Redis::Objects - Map Redis types directly to Ruby objects
=========================================================
[](https://travis-ci.com/github/nateware/redis-objects)
[](https://codecov.io/gh/nateware/redis-objects)
[](https://www.paypal.com/cgi-bin/webscr?cmd=_s-xclick&hosted_button_id=MJF7JU5M7F8VL)
Important 2.0 changes
=====================
Redis::Objects 2.0 introduces several important backwards incompatible changes.
Currently 2.0 can be installed with `gem install redis-objects --pre` or by listing it
explicitly in your Gemfile:
~~~ruby
# Gemfile
gem 'redis-objects', '>= 2.0.0.beta'
~~~
You're encouraged to try it out in test code (not production) to ensure it works for you.
Official release is expected later in 2023.
Key Naming Changes
------------------
The internal key naming scheme has changed for `Nested::Class::Namespaces` to fix a longstanding bug.
**This means your existing data in Redis will not be accessible until you call `migrate_redis_legacy_keys`.**
To fix this (only needed once), create a script like this:
~~~ruby
class YouClassNameHere < ActiveRecord::Base
include Redis::Objects
# ... your relevant definitions here ...
end
YourClassName.migrate_redis_legacy_keys
~~~
Then, you need to find a time when you can temporarily pause writes to your redis server
so that you can run that script. It uses `redis.scan` internally so it should be able to
handle a high number of keys. For large data sets, it could take a while.
For more details on the issue and fix refer to [#213](https://github.com/nateware/redis-objects/issues/231).
Renaming of `lock` Method
-------------------------
The `lock` method that collided with `ActiveRecord::Base` has been renamed `redis_lock`.
This means your classes need to be updated to call `redis_lock` instead:
~~~ruby
class YouClassNameHere < ActiveRecord::Base
include Redis::Objects
redis_lock :mylock # formerly just "lock"
end
~~~
For more details on the issue and fix refer to [#196](https://github.com/nateware/redis-objects/issues/196).
Overview
--------
This is **not** an ORM. People that are wrapping ORM’s around Redis are missing the point.
The killer feature of Redis is that it allows you to perform _atomic_ operations
on _individual_ data structures, like counters, lists, and sets. The **atomic** part is HUGE.
Using an ORM wrapper that retrieves a "record", updates values, then sends those values back,
_removes_ the atomicity, and thus the major advantage of Redis. Just use MySQL, k?
This gem provides a Rubyish interface to Redis, by mapping [Redis data types](http://redis.io/commands)
to Ruby objects, via a thin layer over the `redis` gem. It offers several advantages
over the lower-level redis-rb API:
1. Easy to integrate directly with existing ORMs - ActiveRecord, DataMapper, etc. Add counters to your model!
2. Complex data structures are automatically Marshaled (if you set :marshal => true)
3. Integers are returned as integers, rather than '17'
4. Higher-level types are provided, such as Locks, that wrap multiple calls
This gem originally arose out of a need for high-concurrency atomic operations;
for a fun rant on the topic, see [An Atomic Rant](http://nateware.com/2010/02/18/an-atomic-rant),
or scroll down to [Atomic Counters and Locks](#atomicity) in this README.
There are two ways to use Redis::Objects, either as an include in a model class (to
tightly integrate with ORMs or other classes), or standalone by using classes such
as `Redis::List` and `Redis::SortedSet`.
Installation and Setup
----------------------
Add it to your Gemfile as:
~~~ruby
gem 'redis-objects'
~~~
Redis::Objects needs a handle created by `Redis.new` or a [ConnectionPool](https://github.com/mperham/connection_pool):
The recommended approach is to use a `ConnectionPool` since this guarantees that most timeouts in the `redis` client
do not pollute your existing connection. However, you need to make sure that both `:timeout` and `:size` are set appropriately
in a multithreaded environment.
~~~ruby
require 'connection_pool'
Redis::Objects.redis = ConnectionPool.new(size: 5, timeout: 5) { Redis.new(:host => '127.0.0.1', :port => 6379) }
~~~
Redis::Objects can also default to `Redis.current` if `Redis::Objects.redis` is not set.
~~~ruby
Redis.current = Redis.new(:host => '127.0.0.1', :port => 6379)
~~~
(If you're on Rails, `config/initializers/redis.rb` is a good place for this.)
Remember you can use Redis::Objects in any Ruby code. There are **no** dependencies
on Rails. Standalone, Sinatra, Resque - no problem.
Alternatively, you can set the `redis` handle directly:
~~~ruby
Redis::Objects.redis = Redis.new(...)
~~~
Finally, you can even set different handles for different classes:
~~~ruby
class User
include Redis::Objects
end
class Post
include Redis::Objects
end
# you can also use a ConnectionPool here as well
User.redis = Redis.new(:host => '1.2.3.4')
Post.redis = Redis.new(:host => '5.6.7.8')
~~~
As of `0.7.0`, `redis-objects` now autoloads the appropriate `Redis::Whatever`
classes on demand. Previous strategies of individually requiring `redis/list`
or `redis/set` are no longer required.
Option 1: Model Class Include
=============================
Including Redis::Objects in a model class makes it trivial to integrate Redis types
with an existing ActiveRecord, DataMapper, Mongoid, or similar class. **Redis::Objects
will work with _any_ class that provides an `id` method that returns a unique value.**
Redis::Objects automatically creates keys that are unique to each object, in the format:
model_name:id:field_name
For illustration purposes, consider this stub class:
~~~ruby
class User
include Redis::Objects
counter :my_posts
def id
1
end
end
user = User.new
user.id # 1
user.my_posts.increment
user.my_posts.increment
user.my_posts.increment
puts user.my_posts.value # 3
user.my_posts.reset
puts user.my_posts.value # 0
user.my_posts.reset 5
puts user.my_posts.value # 5
~~~
Here's an example that integrates several data types with an ActiveRecord model:
~~~ruby
class Team < ActiveRecord::Base
include Redis::Objects
redis_lock :trade_players, :expiration => 15 # sec
value :at_bat
counter :hits
counter :runs
counter :outs
counter :inning, :start => 1
list :on_base
list :coaches, :marshal => true
set :outfielders
hash_key :pitchers_faced # "hash" is taken by Ruby
sorted_set :rank, :global => true
end
~~~
Familiar Ruby array operations Just Work (TM):
~~~ruby
@team = Team.find_by_name('New York Yankees')
@team.on_base << 'player1'
@team.on_base << 'player2'
@team.on_base << 'player3'
@team.on_base # ['player1', 'player2', 'player3']
@team.on_base.pop
@team.on_base.shift
@team.on_base.length # 1
@team.on_base.delete('player2')
@team.on_base = ['player1', 'player2'] # ['player1', 'player2']
~~~
Sets work too:
~~~ruby
@team.outfielders << 'outfielder1'
@team.outfielders << 'outfielder2'
@team.outfielders << 'outfielder1' # dup ignored
@team.outfielders # ['outfielder1', 'outfielder2']
@team.outfielders.each do |player|
puts player
end
player = @team.outfielders.detect{|of| of == 'outfielder2'}
@team.outfielders = ['outfielder1', 'outfielder3'] # ['outfielder1', 'outfielder3']
~~~
Hashes work too:
~~~ruby
@team.pitchers_faced['player1'] = 'pitcher2'
@team.pitchers_faced['player2'] = 'pitcher1'
@team.pitchers_faced = { 'player1' => 'pitcher2', 'player2' => 'pitcher1' }
~~~
And you can do unions and intersections between objects (kinda cool):
~~~ruby
@team1.outfielders | @team2.outfielders # outfielders on both teams
@team1.outfielders & @team2.outfielders # in baseball, should be empty :-)
~~~
Counters can be atomically incremented/decremented (but not assigned):
没有合适的资源?快使用搜索试试~ 我知道了~
将 Redis 类型直接映射到 Ruby 对象.zip
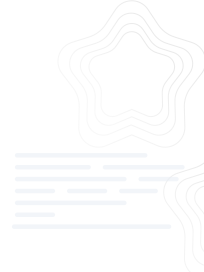
共41个文件
rb:30个
txt:2个
rdoc:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 107 浏览量
2024-12-03
14:40:39
上传
评论
收藏 67KB ZIP 举报
温馨提示
将 Redis 类型直接映射到 Ruby 对象Redis::Objects - 将 Redis 类型直接映射到 Ruby 对象 2.0 版的重要变化Redis::Objects 2.0 引入了几个重要的向后不兼容更改。目前,可以使用以下方式安装 2.0 gem install redis-objects --pre,或者在 Gemfile 中明确列出它# Gemfilegem 'redis-objects', '>= 2.0.0.beta'我们鼓励您在测试代码(而非生产代码)中试用它,以确保它适合您。预计正式发布时间为 2023 年晚些时候。按键命名变更内部键命名方案已更改,以Nested::Class::Namespaces修复长期存在的错误。 这意味着,除非您调用 ,否则 Redis 中的现有数据将无法访问migrate_redis_legacy_keys。为了解决这个问题(只需要一次),创建如下脚本class YouClassNameHere < ActiveRecord::Base include Redis::Objects
资源推荐
资源详情
资源评论
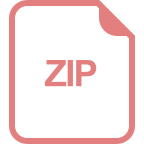
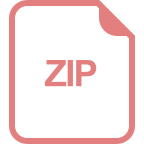
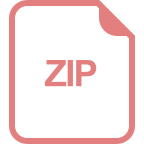
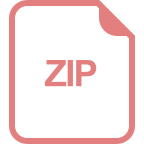
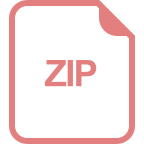
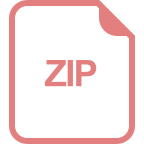
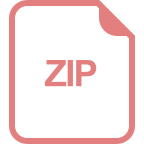
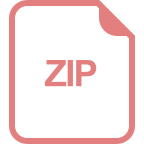
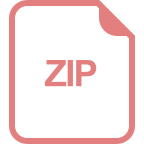
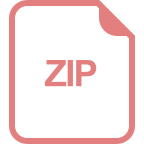
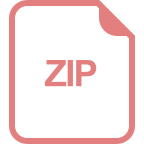
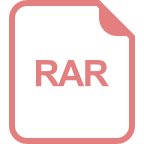
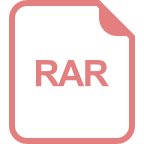
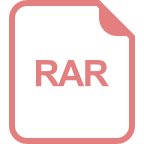
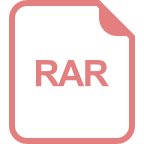
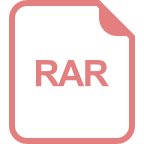
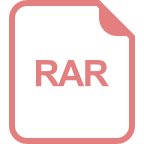
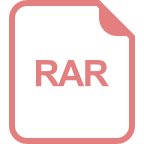
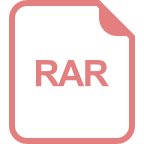
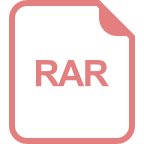
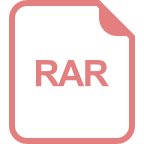
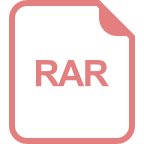
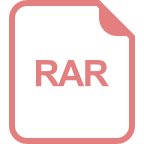
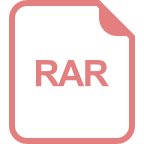
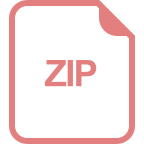
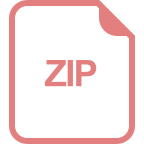
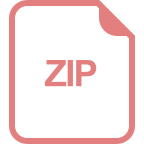
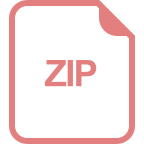
收起资源包目录


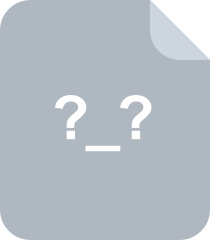

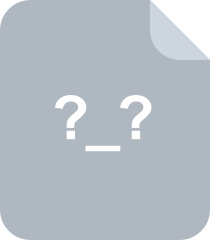
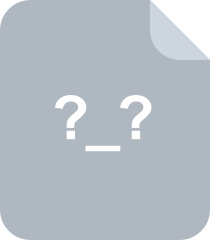

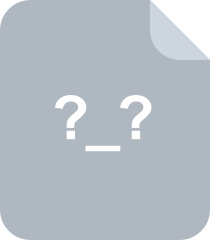
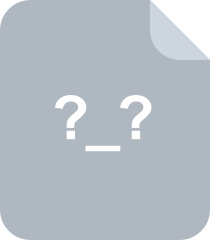

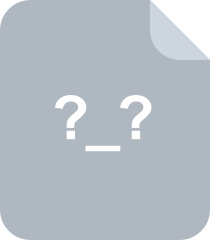
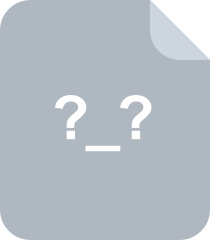
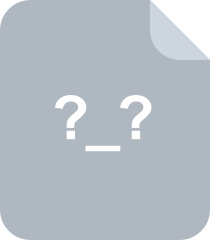
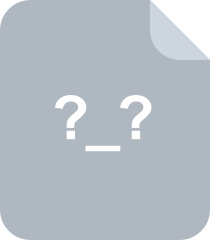
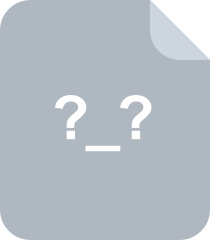
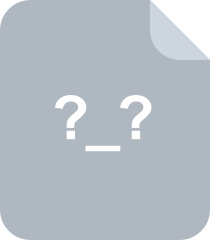
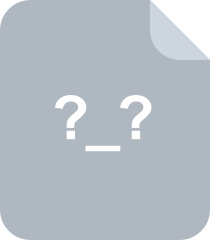
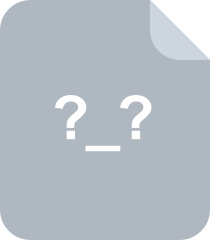
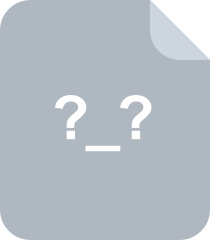
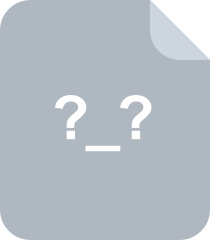
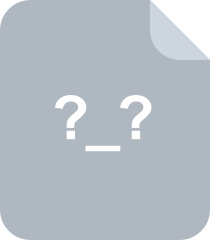
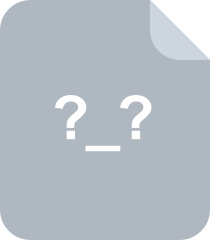
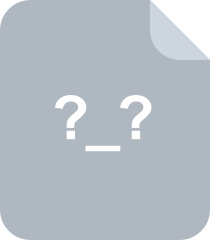
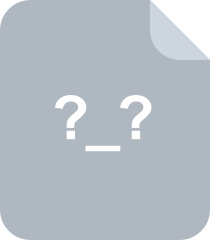
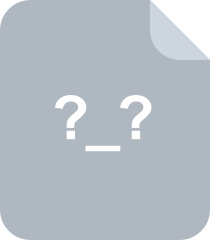
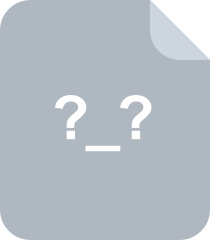
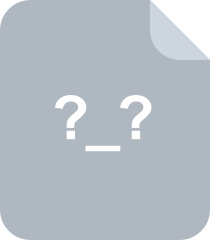
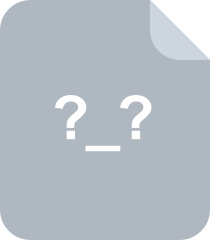
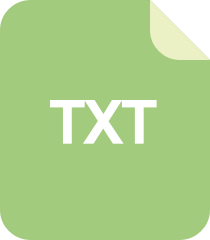
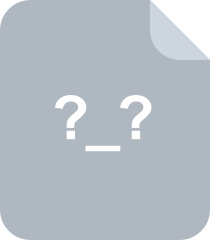
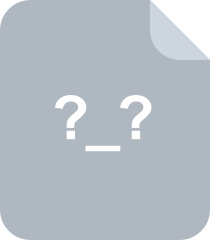

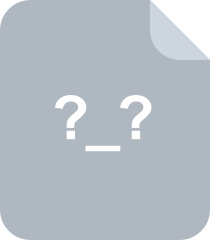
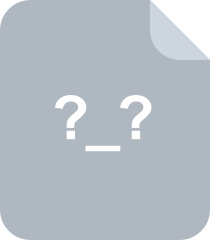
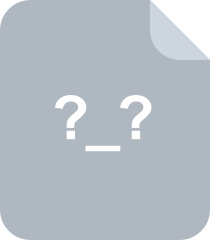
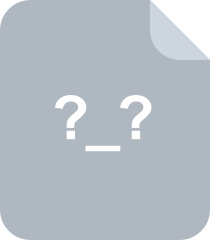
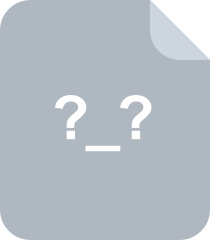
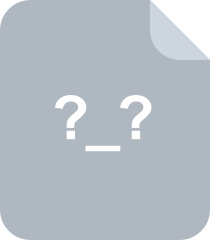
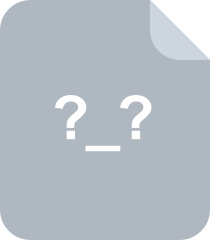
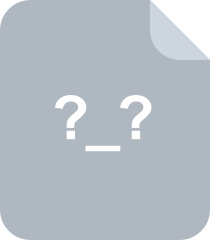
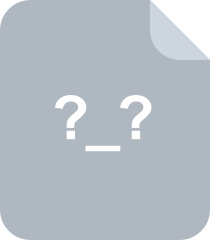
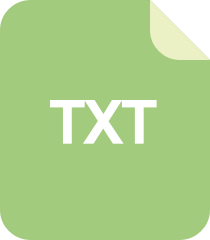
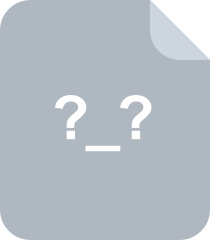
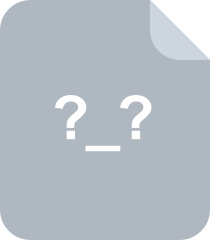
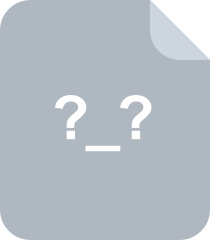
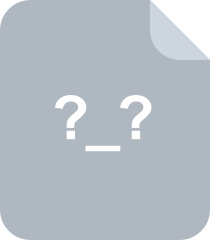
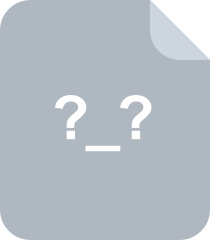
共 41 条
- 1
资源评论
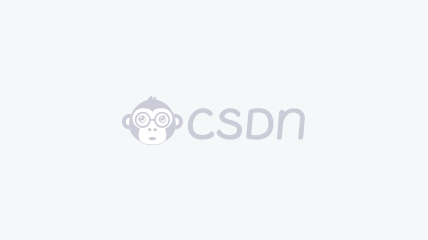

徐浪老师
- 粉丝: 8313
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

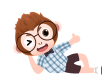
最新资源
- json存取数据,用武器属性作为案例
- 第18章p228页单臂路由实现12月3日.pkt
- varvarvarvarvarvarvarvarvarvarvarvarvarvarvarvarvarvarvar123
- 毕业设计-微麦电影购票小程序(Vue、SpringBoot).zip
- 毕业设计-在线挂号系统APP(VUE).zip
- 月度销售量数据监控(仪表板)
- 毕业设计 - 外包项目网站 - vue+python+flask+uwsgi+nginx.zip
- 工具变量-农民工规模与收入数据集.xls
- 毕业设计 - 基于Android平台的西安市公交路线查询系统的设计与实现.zip
- C# SECS-GEM HSMS 通讯库,使用说明,示例DEMO工程,AIM通讯仿真工具
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


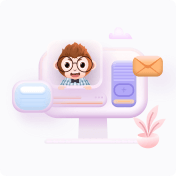
安全验证
文档复制为VIP权益,开通VIP直接复制
