# Yolo v4 and Yolo v3 Tiny
Yolo v4 & Yolo v3 Tiny using TensorFlow 2.x
This Tensorflow adaptation of the release 4 of the famous deep network Yolo is based on the original Yolo source code in C++ that you can find here: https://github.com/pjreddie/darknet and https://github.com/AlexeyAB/darknet + the WIKI https://github.com/AlexeyAB/darknet/wiki
The method to adapt this deep network is based on the method used by *Jason Brownlee* for the previous release v3 and presented here https://machinelearningmastery.com/how-to-perform-object-detection-with-yolov3-in-keras/
But I have made several changes due to the new features added by the release 4 of Yolo.
All the steps are included in the jupyter notebook **YoloV4_tf.ipynb**
In addition, I have defined the **loss function** so you can train the model as described later. The corresponding steps are included in the jupyter notebook **YoloV4_Train_tf.ipynb**
The release numbers are:
- TensorFlow version: 2.1.0
- Keras version: 2.2.4-tf
# The steps to use Yolo-V4 with TensorFlow 2.x are the following
## 1. Build the TensorFlow model
The model is composed of 161 layers.
Most of them are *Conv2D*, there are also 3 *MaxPool2D* and one *UpSampling2D*.
In addtion there are few shorcuts with some concatenate.
Two activation methods are used, *LeakyReLU* with alpha=0.1 and *Mish* with a threshold = 20.0. I have defined Mish as a custom object as Mish is not included in the core TF release yet.
The specifc Yolo output layers *yolo_139*, *yolo_150* and *yolo_161* are not defined in my Tensorflow model because they handle cutomized processing. So I have defined no activation for these layers but I have built the corresponding processing in a specifig python function run after the model prediction.
## 2. Get and compute the weights
The yolo weight have been retreived from https://github.com/AlexeyAB/darknet/releases/download/darknet_yolo_v3_optimal/yolov4.weights.
The file contain the kernel weights but also the biases and the Batch Normalisation parameters scale, mean and var.
Instead of using Batch normalisation layers into the model, I have directly normalized the weights and biases with the values of scale, mean and var.
- bias = bias - scale * mean / (np.sqrt(var + 0.00001)
- weights = weights* scale / (np.sqrt(var + 0.00001))
I have kept the Batch normalisation layers in the model for training purpose. By defaut, the corresponding parameters are not applicable (weight = 1 and bias = 0) but they are updated if you train the model.
As these parameters as stored in the Caffe mode, I have applied several transformation to map the TF requirements.
## 3. Save the model
The model is saved in a h5 file after building it and computing the weights.
## 4. Load the model
The model previously saved is loaded from the h5 file and then ready to be used.
## 5. Pre-processing
During the pre-processing the 80 labels and the image to predict are loaded.
The labels are in the file *coco_classes.txt*.
The image is resized in the Yolo format 608*608 using interpolation = 'bilinear'.
As usual, the values of the pixels are divided by 255.
## 6. Run the model
The model is run with the resized image as input with a shape=(1,608,608,3).
The model provides 3 output layers 139, 150 and 161 with the shapes respectively (1, 76, 76, 255), (1, 38, 38, 255), (1, 19, 19, 255).
The number of channels is 255 = ( bx,by,bh,bw,pc + 80 classes ) * 3 anchor boxes, where *(bx,by,bh,bw)* define the position and size of the box, and *pc* is the probability to find an object in the box.
3 anchor boxes per Yolo output layers are defined:
- output layer 139 (76,76,255): (12, 16), (19, 36), (40, 28)
- output layer 150 (38,38,255): (36, 75), (76, 55), (72, 146)
- output layer 161 (19,19,255): (142, 110), (192, 243), (459, 40)
## 7. Compute the Yolo layers
As explained before, the 3 final Yolo layers are computed outside the TF model by the python function *decode_netout*.
The steps of this function are the following:
- apply the sigmoid activation on everything except bh and bw.
- scale bx and by using the factor *scales_x_y* 1.2, 1.1, 1.05 defined for each Yolo layer.
- (bx,by)=(bx,by)scales_x_y - 0.5(scales_x_y - 1.0)
- get the boxes parameters for prediction *pc* > 0.25
- x = (col + x) / grid_w (=76, 38 or 19)
- y = (row + y) / grid_h (=76, 38 or 19)
- w = anchors_w * exp(w) / network width (=608)
- h = anchors_h * exp(h) / network height (=608)
- classes = classes*pc
## 8. Correct the boxes according the inital size of the image
## 9. Suppress the non Maximal boxes
## 10. Get the details of the detected objects for a threshold > 0.6
## 11. Draw the result
# The steps to train Yolo-V4 with TensorFlow 2.x are the following
## 1. Build the TensorFlow model
The model is composed of 161 layers.
Most of them are *Conv2D*, there are also 3 *MaxPool2D* and one *UpSampling2D*.
In addtion there are few shorcuts with some concatenate.
Two activation methods are used, *LeakyReLU* with alpha=0.1 and *Mish* with a threshold = 20.0. I have defined Mish as a custom object as Mish is not included in the core TF release yet.
The specifc Yolo output layers *yolo_139*, *yolo_150* and *yolo_161* are not defined in my Tensorflow model because they handle cutomized processing. So I have defined no activation for these layers but I have built the corresponding processing in a specifig python function run after the model prediction.
## 2. Get and compute the weights (you can skip this part if you want to train a empty model)
The yolo weight have been retreived from https://github.com/AlexeyAB/darknet/releases/download/darknet_yolo_v3_optimal/yolov4.weights.
The file contain the kernel weights but also the biases and the Batch Normalisation parameters scale, mean and var.
Instead of using Batch normalisation layers into the model, I have directly normalized the weights and biases with the values of scale, mean and var.
- bias = bias - scale * mean / (np.sqrt(var + 0.00001)
- weights = weights* scale / (np.sqrt(var + 0.00001))
I have kept the Batch normalisation layers in the model for training purpose. By defaut, the corresponding parameters are not applicable (weight = 1 and bias = 0) but they are updated if you train the model.
As these parameters as stored in the Caffe mode, I have applied several transformation to map the TF requirements.
## 3. Save the model
The model is saved in a h5 file after building it and computing the weights.
## 4. Load the model
The model previously saved is loaded from the h5 file.
## 5. Freeze the backbone
You need to define until which layer you want to freese the model. To free the backbone Yolo v4, set fine_tune_at = "convn_136"
## 6. Get the Pascal VOC dataset
I have used the Pascal VOC dataset to train the model.
You can find the dataset here: https://pjreddie.com/projects/pascal-voc-dataset-mirror/ in order to get the images and the corresponding annotations in xml format.
## 7. Build the labels files for VOC train dataset
One label file per image and per box is created (3 boxes are defined in Yolo4).
The label file contains the position and the size of the box, the probability to find an object in the box and the class id of the object.
This file contains one line per object in the image.
## 8. Build the labels files for VOC validate dataset
Same thing than above but for the dataset used to validate the training.
## 9. Compute the data for training
Train data are created based on the Label files previously created and the images.
You can define how many data do you want to train.
## 10. Compute the data for validation
Same thing than above but for the data used to validate the training.
## 11. Choose the optimizer
Several optimizers are available in Tensorflow: SGD, RMSprop, Adam...
## 12. Fit the model including validation data
Fit the model using all the Tensorflow features you w
没有合适的资源?快使用搜索试试~ 我知道了~
使用 TensorFlow 2.x 的 Yolo v4.zip
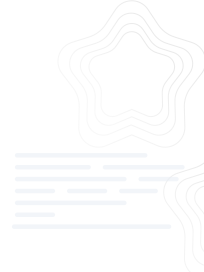
共112个文件
png:40个
py:26个
sh:6个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 176 浏览量
2024-11-26
13:37:45
上传
评论
收藏 9.01MB ZIP 举报
温馨提示
Yolo v4 和 Yolo v3 Tiny使用 TensorFlow 2.x 的 Yolo v4 和 Yolo v3 Tiny这个 Tensorflow 改编自著名深度网络 Yolo 的第 4 版,基于 C++ 中的原始 Yolo 源代码,您可以在此处找到https://github.com/pjreddie/darknet和https://github.com/AlexeyAB/darknet + WIKI https://github.com/AlexeyAB/darknet/wiki适应这个深度网络的方法基于Jason Brownlee在之前的版本 v3 中使用方法,并在此处介绍https://machinelearningmastery.com/how-to-perform-object-detection-with-yolov3-in-keras/但由于 Yolo 4 版新增的功能,我做了一些更改。所有步骤都包含在 jupyter 笔记本YoloV4_tf.ipynb中此外,我定义了损失函数,以便您可以按照后面所述训练模型。相应的步骤包含在 jupy
资源推荐
资源详情
资源评论
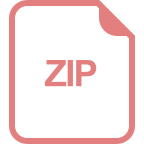
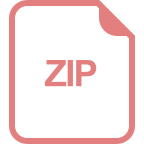
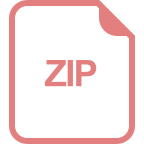
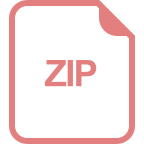
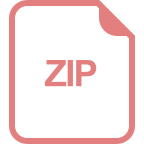
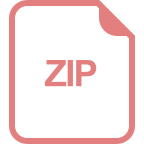
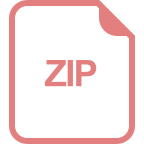
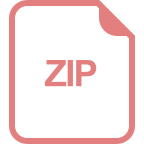
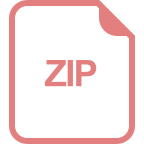
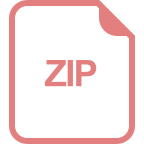
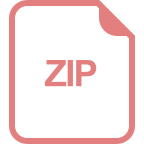
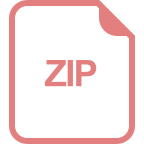
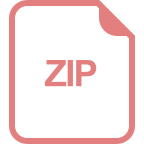
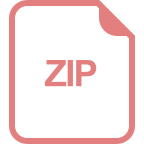
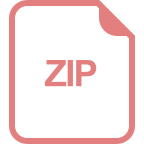
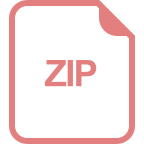
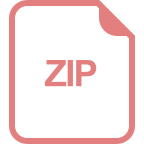
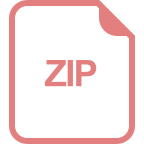
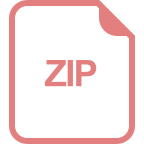
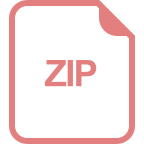
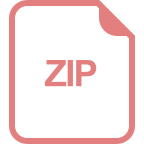
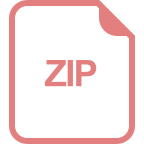
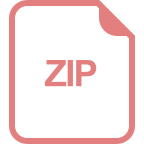
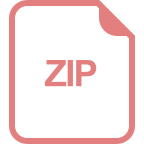
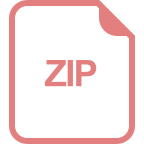
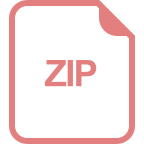
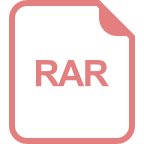
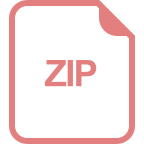
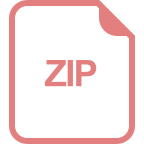
收起资源包目录

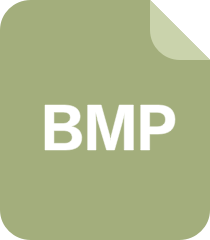
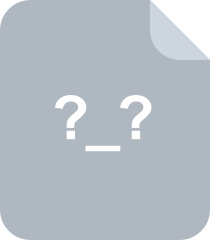
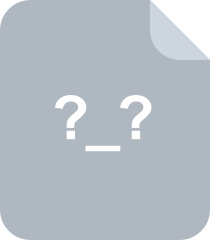
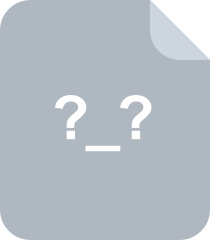
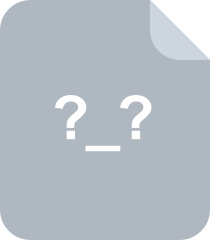
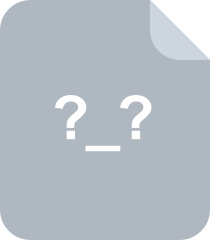
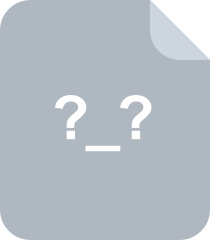
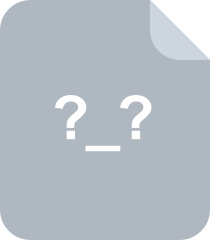
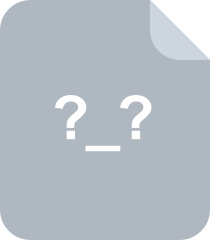
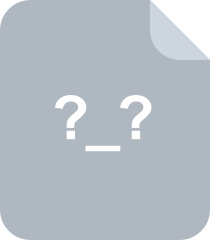
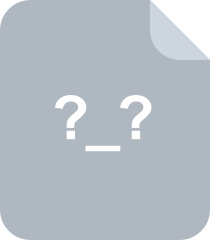
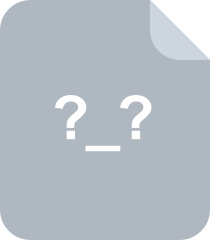
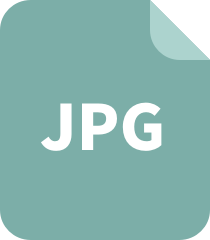
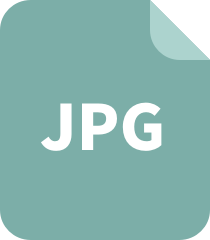
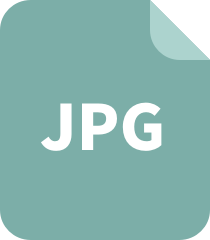
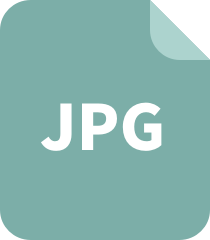
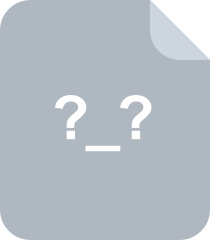
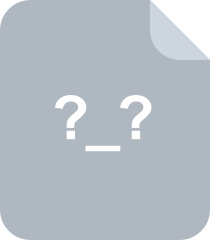
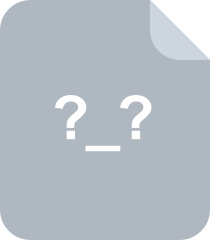
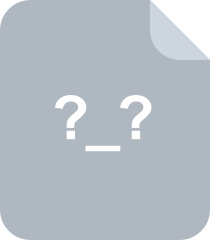
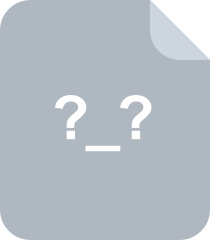
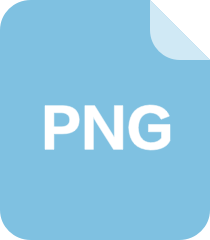
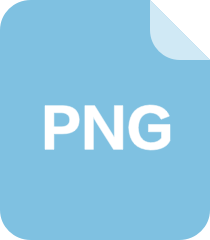
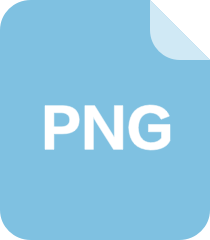
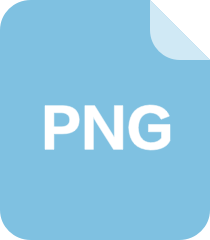
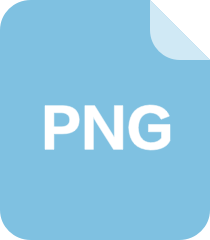
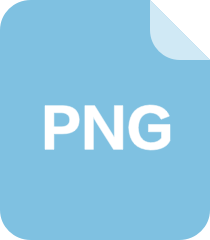
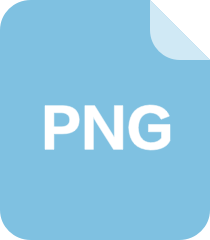
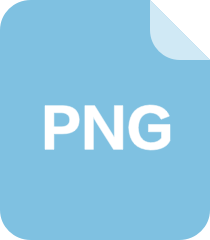
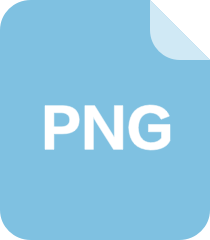
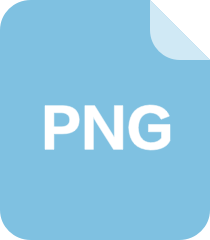
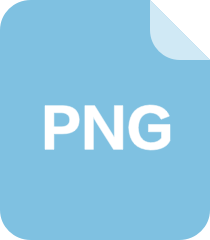
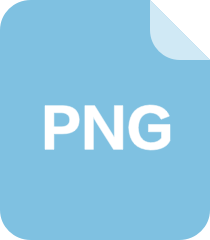
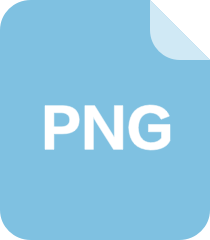
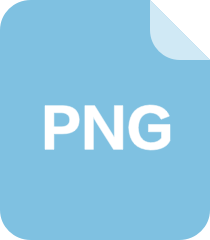
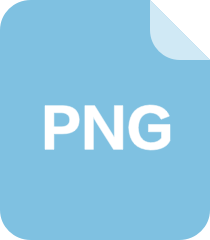
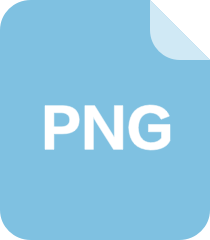
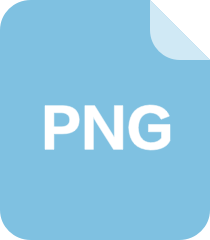
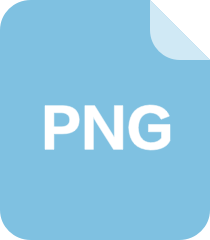
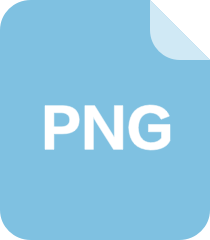
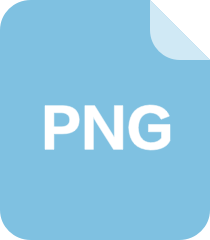
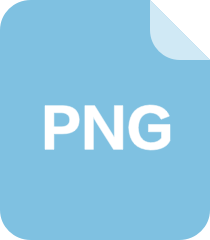
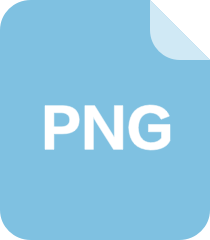
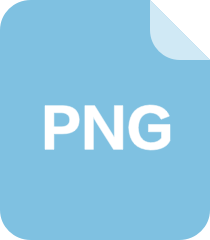
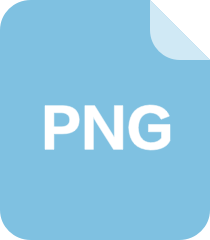
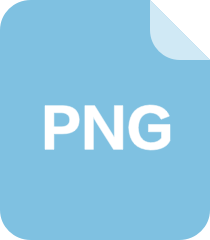
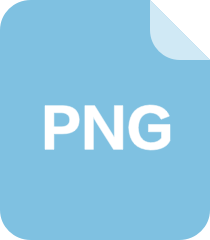
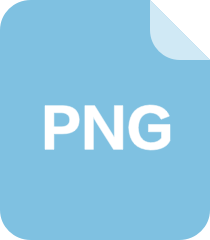
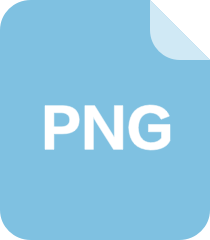
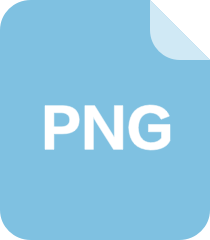
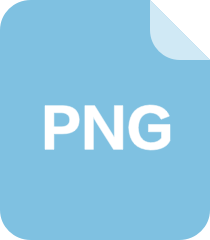
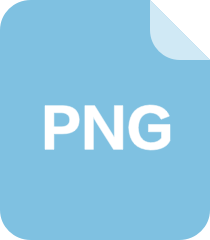
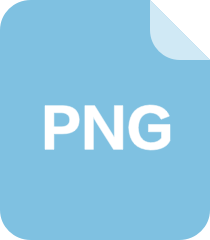
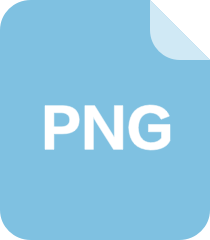
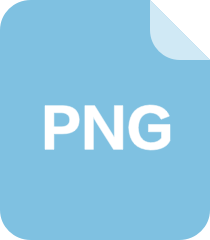
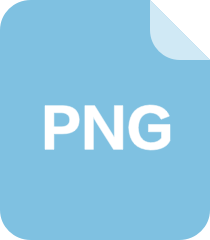
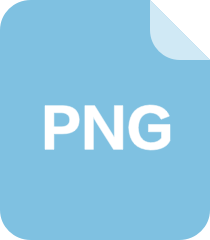
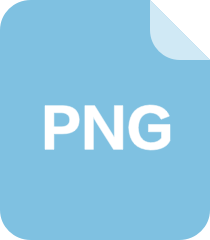
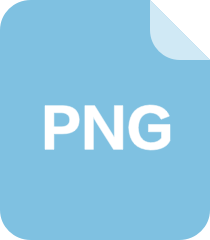
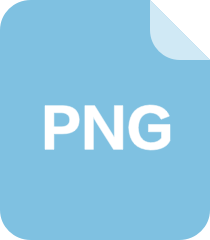
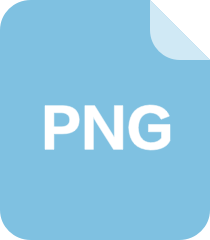
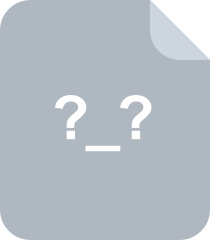
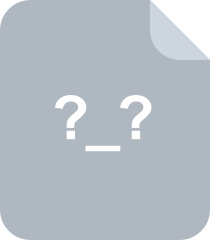
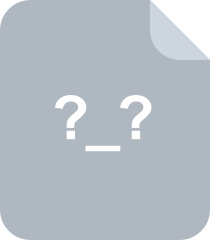
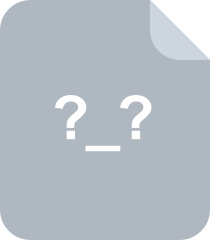
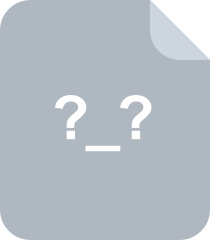
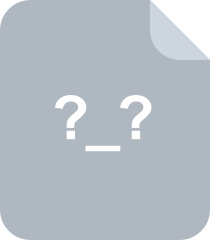
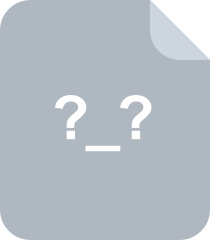
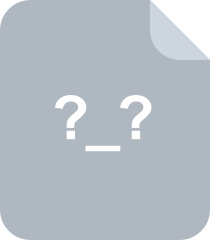
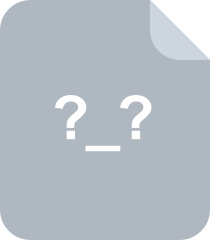
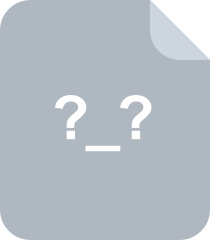
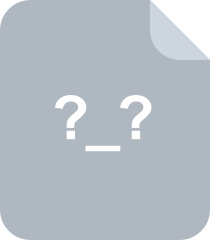
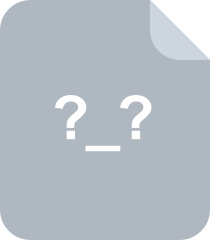
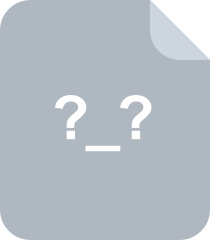
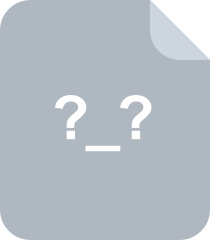
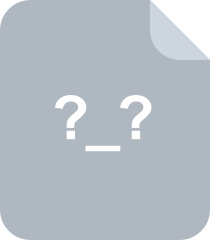
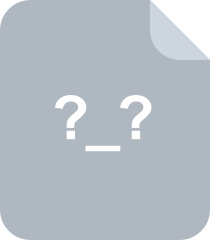
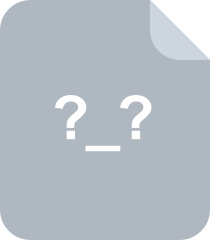
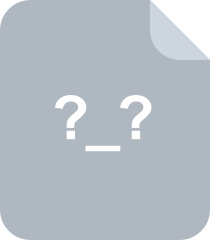
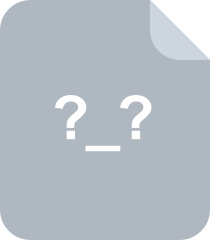
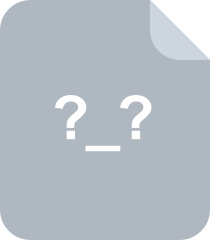
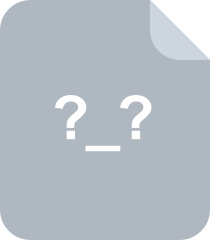
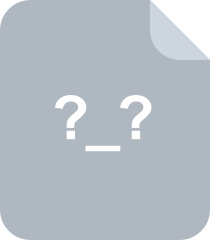
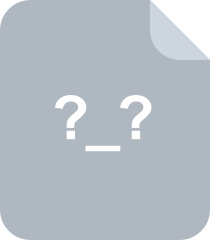
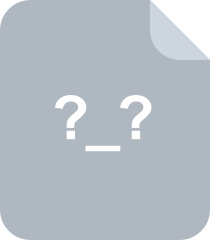
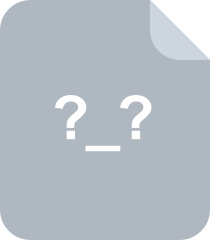
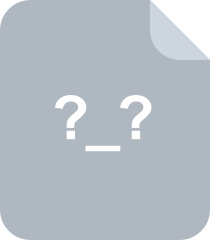
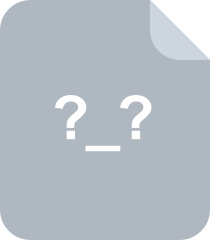
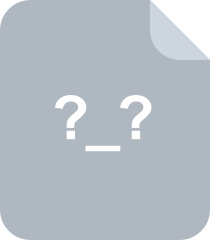
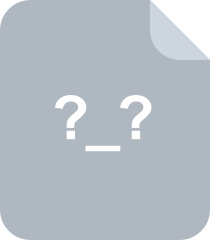
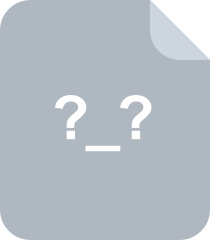
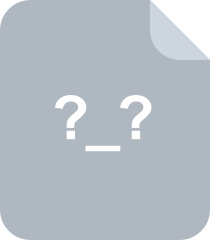
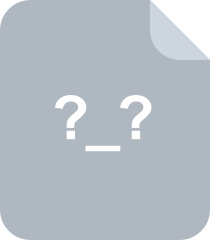
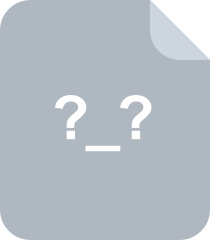
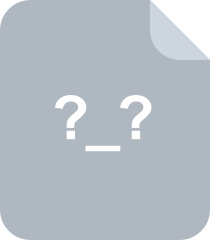
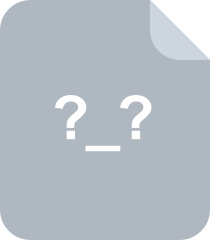
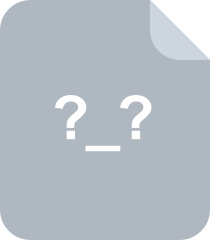
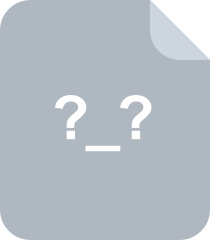
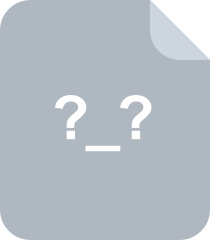
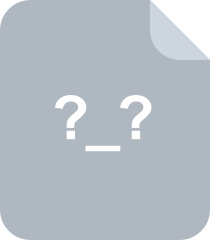
共 112 条
- 1
- 2
资源评论
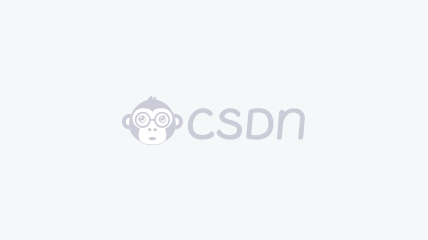

徐浪老师
- 粉丝: 8550
- 资源: 1万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

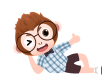
最新资源
- jsp+sql智能交通道路管理系统+任务书+外文翻译+开题报告+文献综述(+说明文档+mysql+lw).zip
- jsp+基于JB的人事管理系统源代码+(+说明文档+mysql+lw).zip
- jspOA办公自动化系统-毕业设计(+说明文档+mysql).zip
- JSPGenCMS_(+说明文档+mysql).zip
- JSP+SQL计算机等级考试查询系统(源代码++答辩PPT)(+说明文档+mysql+lw).zip
- jsp报名系统(+说明文档+mysql).zip
- jsp+sql书店(+说明文档+mysql+lw).zip
- 结构相同的excel表格,如何实现自动批量合并?
- Emlog搜云数据采集插件,一键自动云抓取分类发布,列表、内容
- 半监督学习Tri-training算法在命名实体识别领域的Python实现与应用
- 公考大宝小包言语理解资料
- 计算机图形学网格处理的项目
- 434JSP新生学生宿舍分配系统毕业课程源码设计+论文资料
- 基于卡尔曼滤波的锂电池SOC估计 Matlab Simulink仿真模型(成品) 扩展卡尔曼滤波(EFK)实验、参数辨识和仿真
- 基于LCL的三相逆变器并网仿真(QP控制),thd值为2.38%,从左到右依次是直流母线,三相逆变器lc l滤波器,负载以及380v电源,逆变器采用pq策略(联系不送单独的svpwm仿真以及文档说明
- iDealshare VideoGo for Mac v6.8.1
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


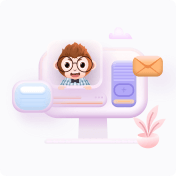
安全验证
文档复制为VIP权益,开通VIP直接复制
