#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <errno.h>
//arm-linux-gnueabihf-gcc gpio_test_input.c -o gpio_test_input
#define ERROR 0x01
#define IN 0x01
#define OUT 0x02
#define TEST_1_PIN_NUM 434// FMQL45T900 EMIO8 -> gpiochip434
#define TEST_2_PIN_NUM 916// ZYNQ-7000 MIO10 -> gpio916
#define GPIO_DEBUG
#ifdef GPIO_DEBUG
#define dbg_info(format, args...) printf("%d:"format, __LINE__, ##args)
#else
#define dbg_info(format, args...)
#endif
#define dbg_err(format, args...) printf("%d:"format, __LINE__, ##args)
struct pin_mesg{
int pin;
unsigned char inout_flags;
};
static int gpio_unexport(struct pin_mesg* mesg);
static int gpio_read(struct pin_mesg* mesg, char* value);
static int gpio_direction(struct pin_mesg* mesg);
static int gpio_export(struct pin_mesg* mesg);
int main(int argc, char *argv[]){
char value;
struct pin_mesg test_pin_mesg;
test_pin_mesg.pin = TEST_1_PIN_NUM;
test_pin_mesg.inout_flags = IN;
gpio_export(&test_pin_mesg);//生成gpio节点
gpio_direction(&test_pin_mesg);//设置方向
gpio_read(&test_pin_mesg, &value);//读入value,
return 0;
}
static int gpio_export(struct pin_mesg* mesg){
char name[4];
int fd,len;
fd = open("/sys/class/gpio/export", O_WRONLY);
if (fd < 0) {
perror("open export");
return(-1);
}
len = sprintf(name, "%d", mesg->pin);
dbg_info("name = gpio%s\n",name);
if (write(fd, name, sizeof(name)) < 0) {
perror("write export");
return -1;
}
close(fd);
return 0;
}
static int gpio_direction(struct pin_mesg* mesg){
char path[64];
int fd,res;
char *dir;
if(mesg->inout_flags & IN)
dir = "in";
if(mesg->inout_flags & OUT)
dir = "out";
sprintf(path,"/sys/class/gpio/gpio%d/direction", mesg->pin);
dbg_info("direction path: %s,direction = %s\n", path, dir);
fd = open(path, O_WRONLY);
if (fd < 0) {
perror("open direction");
return -1;
}
res = write(fd, dir, sizeof(dir));
if (res <= 0) {
perror("write direction");
return -1;
}
close(fd);
return 0;
}
static int gpio_read(struct pin_mesg* mesg, char* value){
char path[64];
char value_str[4];
int fd;
sprintf(path, "/sys/class/gpio/gpio%d/value", mesg->pin);
dbg_info("value path: %s\n", path);
fd = open(path, O_RDONLY);
if (fd < 0) {
perror("open value");
return -1;
}
if (read(fd, value_str, 3) < 0) {
perror("read value");
return -1;
}
dbg_info("read value: %s\n", value_str);
* value = (atoi(value_str));
close(fd);
return 0;
}
static int gpio_unexport(struct pin_mesg* mesg)
{
char name[4];
int len;
int fd;
fd = open("/sys/class/gpio/unexport", O_WRONLY);
if (fd < 0) {
perror("open unexport");
return -1;
}
len = sprintf(name, "%d", mesg->pin);
dbg_info("name = gpio%s\n",name);
if (write(fd, name, len) < 0) {
perror("write unexport");
return -1;
}
close(fd);
return 0;
}
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
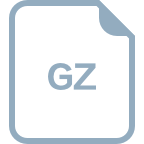
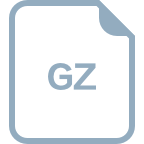
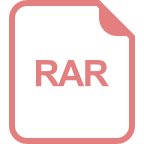
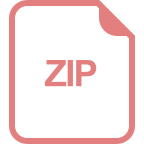
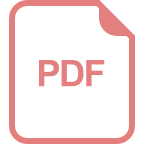
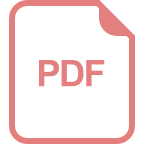
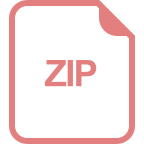
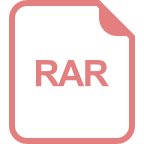
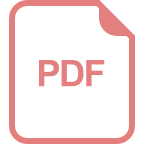
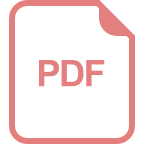
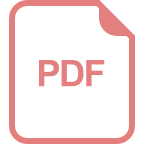
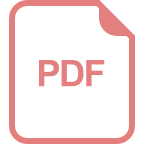
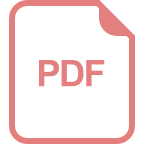
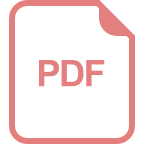
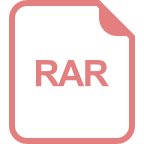
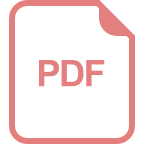
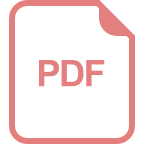
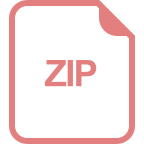
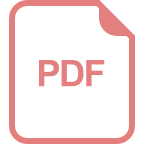
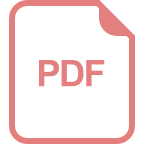
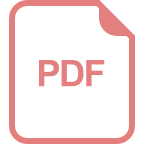
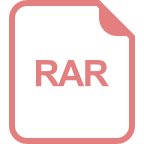
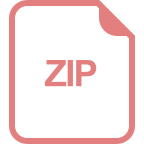
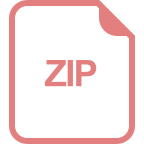
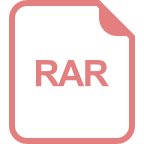
收起资源包目录


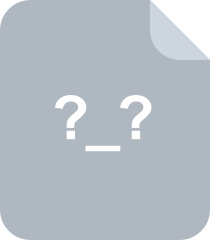
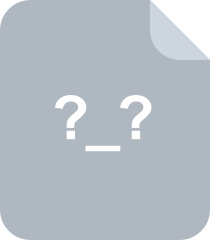
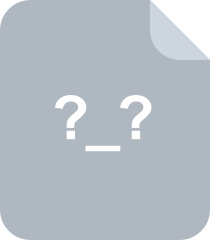
共 3 条
- 1
资源评论
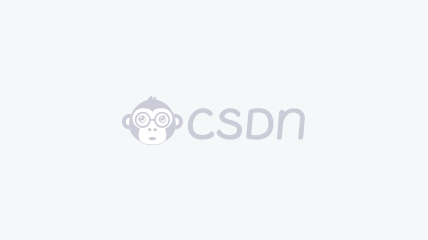

大牛攻城狮
- 粉丝: 1w+
- 资源: 146
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

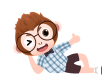
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


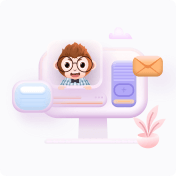
安全验证
文档复制为VIP权益,开通VIP直接复制
