#include "videoplayer.h"
videoPlayer::videoPlayer(QWidget *parent)
: QWidget(parent)
{
bPlayOrPause = false;
bRepaint = false; // 换背景色的变量
bTop = false;
bMaxSize = false; // 窗口最大化
bFull = false;
this->setGeometry(300,30,640,480);
this->setWindowIcon(QIcon(":img/image/play.png"));
this->setWindowTitle(tr("视频播放器"));
this->setWindowFlags(Qt::FramelessWindowHint);
this->setAttribute(Qt::WA_TranslucentBackground);
this->setAutoFillBackground(true);
this->setAttribute(Qt::WA_DeleteOnClose); // 关闭时释放占用的内存
createMediaObjects(); // 媒体的各种控件
createBtnLink(); // 按键
createLayout(); // 布局管理
// videoWidget之上的背景图
QPixmap pixMap(":img/image/vbg2.png");
labelBackGround = new QLabel(this);
labelBackGround->setPixmap(pixMap);
labelBackGround->setGeometry(10,10,620,360);
}
videoPlayer::~videoPlayer()
{
}
void videoPlayer::createMediaObjects()
{
mediaObject = new Phonon::MediaObject(this); // 媒体对象
metaInfomationResolver = new Phonon::MediaObject(this); // 当前媒体对象
connect(mediaObject,SIGNAL(aboutToFinish()),this,SLOT(aboutToFinish()));
// 与播放结束的链接 finished重载了 实现循环播放
connect(mediaObject,SIGNAL(finished()),this,SLOT(finished()));
// LCDNumber 每秒更新显示 和 获取当前歌曲总时间 类的私有槽函数
connect(mediaObject,SIGNAL(tick(qint64)),this,SLOT(tickAndTotalTime(qint64)));
connect(mediaObject,SIGNAL(tick(qint64)),this,SLOT(showSubtitle(qint64))); // 字幕
// 用于显示图像的窗口 不能少 Phonon::VideoWidget
videoWidget = new Phonon::VideoWidget(this);
videoWidget->setAspectRatio(Phonon::VideoWidget::AspectRatioAuto);
Phonon::createPath(mediaObject, videoWidget); // 创建图像与mediaObject的连接
videoWidget->installEventFilter(this); // 安装事件过滤器 在eventFileter中实现
audioOutput = new Phonon::AudioOutput(Phonon::VideoCategory, this);
Phonon::createPath(mediaObject, audioOutput); // 创建声音与mediaObject的连接
// 音量标签
volumeLabel = new QLabel(tr("Volume:"),this);
volumeLabel->setStyleSheet("QLabel {color:black}");
volumeLabel->setFont(QFont(tr("Times New Roman"),12));
// 音量条
volumeSlider = new Phonon::VolumeSlider(this);
volumeSlider->setAudioOutput(audioOutput); // 设置声音的输出设备
volumeSlider->setSizePolicy(QSizePolicy::Maximum,QSizePolicy::Maximum);
volumeSlider->setStyleSheet(
"QSlider::groove:horizontal { /*糟部分*/ "
" border: 1px solid #999999; "
" height: 2px; "
" margin: 0px 0; "
" left: 12px; right: 12px; "
" } "
"QSlider::handle:horizontal { /*滑块部分*/ "
" border: 1px solid #5c5c5c; "
" border-image:url(:/images/smile.png); "
" width: 18px; "
" margin: -7px -7px -7px -7px; "
" } "
" "
"QSlider::sub-page:horizontal{ /*已经滑过部分*/ "
" background: rgba(57,57,57,200); "
"} "
"QSlider::add-page:horizontal{ /*未滑过部分*/ "
" background: rgba(150,150,150,200);;}");
// 进度条
seekSlider = new Phonon::SeekSlider(this);
seekSlider->setMediaObject(mediaObject); // 设置进度条的的连接媒体源 mediaObject
seekSlider->setStyleSheet(
"QSlider::groove:horizontal { /*糟部分*/ "
" border: 1px solid #999999; "
" height: 2px; "
" margin: 0px 0; "
" left: 12px; right: 12px; "
" } "
"QSlider::handle:horizontal { /*滑块部分*/ "
" border: 1px solid #5c5c5c; "
" border-image:url(:/images/heart.png); "
" width: 18px; "
" margin: -7px -7px -7px -7px; "
" } "
" "
"QSlider::sub-page:horizontal{ /*已经滑过部分*/ "
" background: rgba(57,57,57,200); "
"} "
"QSlider::add-page:horizontal{ /*未滑过部分*/ "
" background: rgba(150,150,150,200);;}");
// 时间标签
musicTimeLabel = new QLabel(tr("0:00:00/0:00:00"),this);
musicTimeLabel->setFont(QFont(tr("Times New Roman"),16));
musicTimeLabel->show();
// 字幕标签
subTitleLabel = new QLabel(videoWidget);
subTitleLabel->setStyleSheet("QLabel {color: white;}");
subTitleLabel->setFont(QFont(tr("Times New Roman"),14));
subTitleLabel->setWindowFlags(Qt::WindowStaysOnTopHint);
subTitleLabel->setAttribute(Qt::WA_TranslucentBackground);
subTitleLabel->show();
subTitleLabel->move(200, 400);
bDesLrc = true;
}
// 获取电影的文件名和路径,以便查找字幕文件
void videoPlayer::getVideoPathAndName()
{
/* ***************** 重新获取歌曲名称和路径 以便显示歌词 ******************/
QString fileName = mediaObject->currentSource().fileName();
musicFileName = "";
musicFilePath = "";
musicFileName = fileName.right(fileName.length() - fileName.lastIndexOf('/') - 1); // 音乐文件名
musicFilePath = fileName.left(fileName.length() - musicFileName.length()); // 路径名
qDebug() << musicFileName;
}
// 显示电影字幕
void videoPlayer::showSubtitle(qint64 time)
{
//getVideoPathAndName(); // 获取电影名和路径
if(lrcHash.isEmpty()){ // 换了歌曲的话 要重读文件
subTitleLabel->setText(tr("Loading..."));
subTitleLabel->update();
bDesLrc = true;
lrcHash.squeeze(); // resize the hash<>
}
long timeFlag = (long)time;
QString t = QString::number(timeFlag);
if(timeFlag < 9000)
t = t.mid(0,1);
if(timeFlag > 9000 && timeFlag < 100000)
t = t.mid(0,2);
else
t = t.mid(0,3);
int realTime = t.toInt();
QFile file; // 歌词文件
if(bDesLrc){ // 需要重读文件 bDesLrc是控制变量
QDir::setCurrent(musicFilePath); // 设置音乐文件路径为当前路径
QString lrcFileName(musicFileName); // 设置文件名为正在播放的文件名
lrcFileName = lrcFileName.remove(musicFileName.right(3)) + "srt";
file.setFileName(lrcFileName);
if(file.open(QIODevice::ReadOnly | QIODevice::Text)) // 叛断歌词文件是否打开
bDesLrc = false;
}
QString lrcCache = ""; // 从文件中读进来的内容存进里面 缓存
QTextStream lrcStream(&file);
QTextCodec *codec = QTextCodec::codecForName("gb18030"); //解决乱码问题
lrcStream.setCodec(codec);
// read the lrc stream
while(!(lrcStream.atEnd())){ // 没有读完的时候
int timeTwo,timeThr;
bool bTwo = false;
bool bThr = false;
lrcCache = lrcStream.readLine(); // read line
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
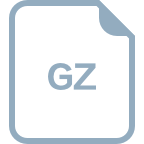
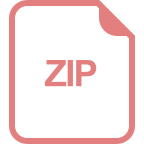
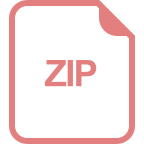
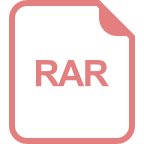
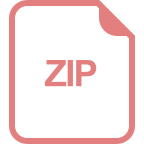
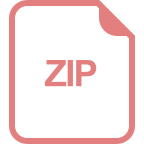
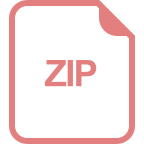
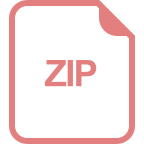
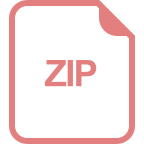
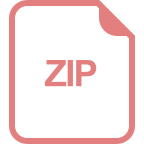
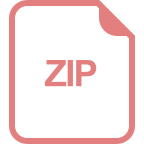
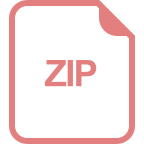
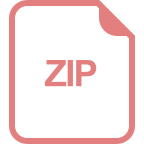
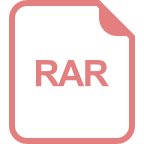
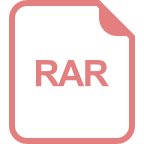
收起资源包目录

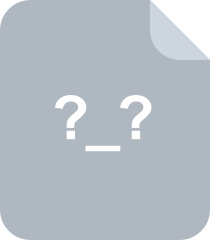
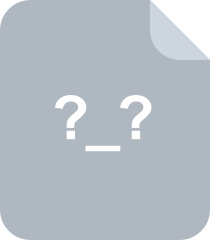
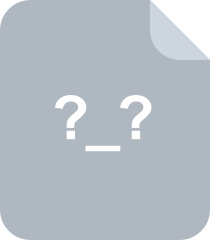
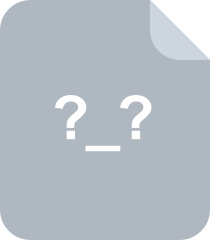
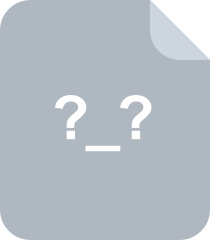
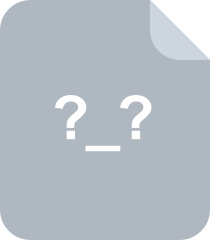
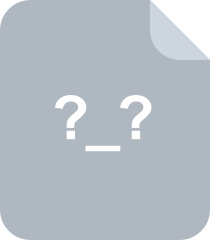
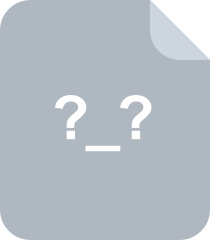
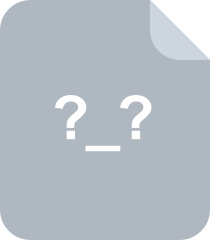
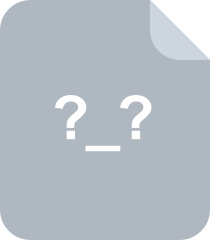
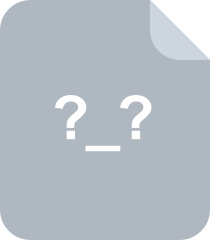
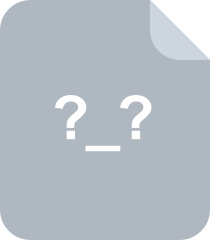
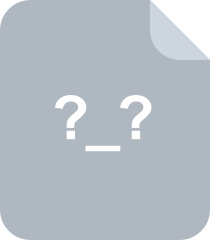
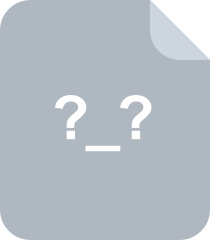
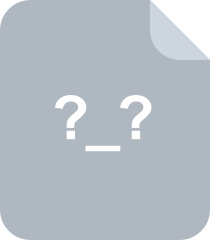
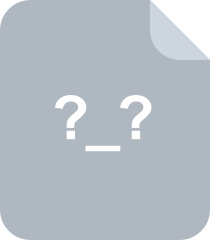
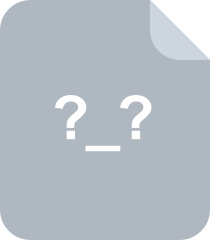
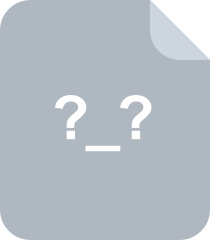
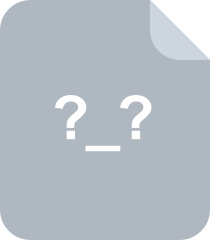
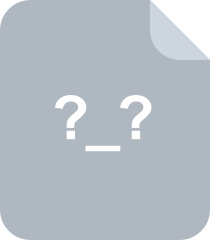
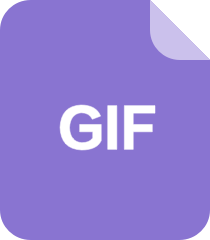
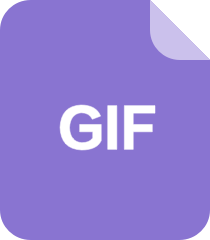
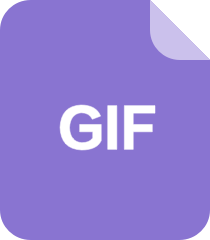
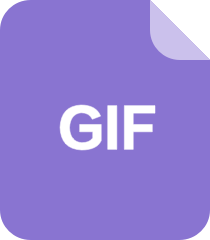
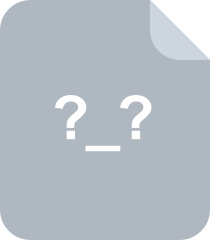
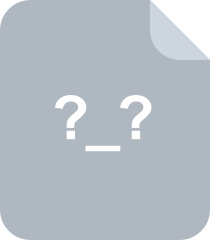
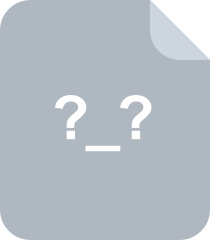
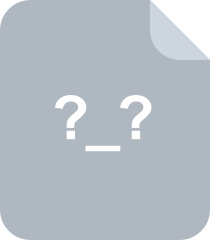
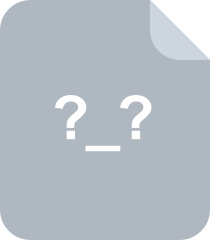
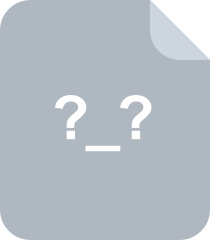
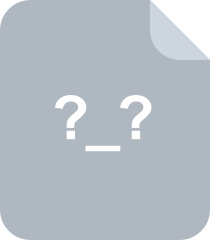
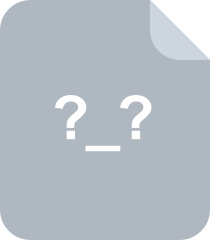
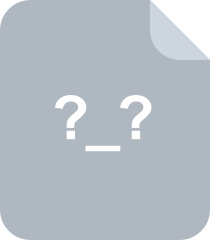
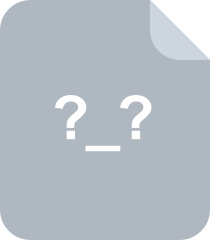
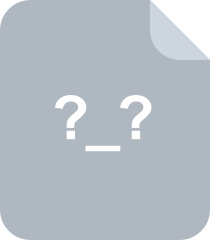
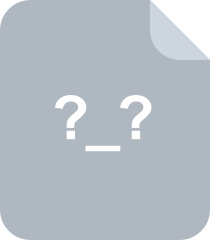
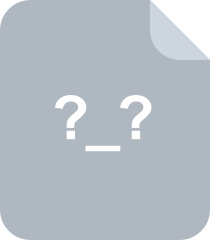
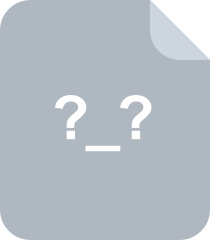
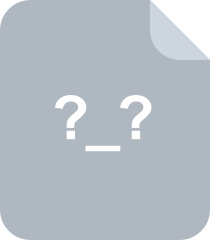
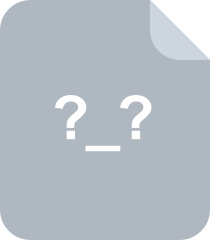
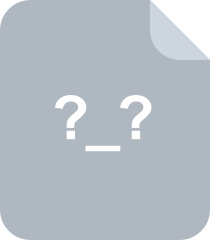
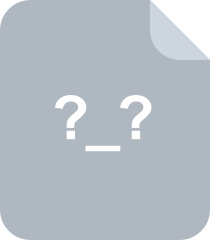
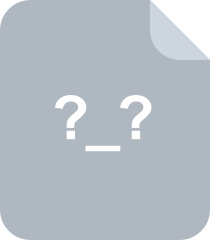
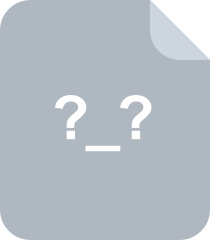
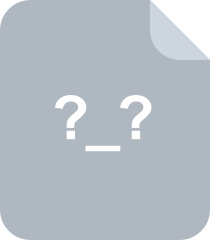
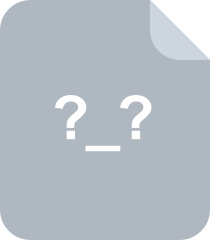
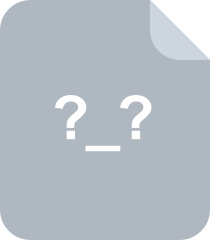
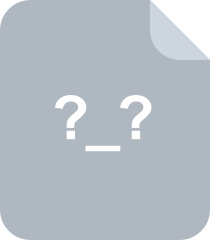
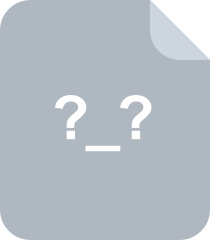
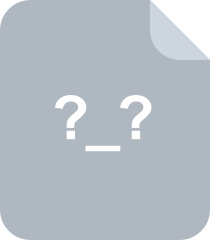
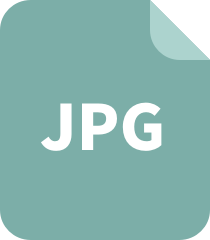
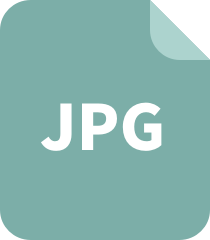
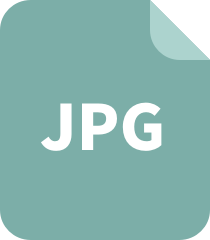
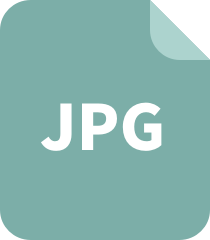
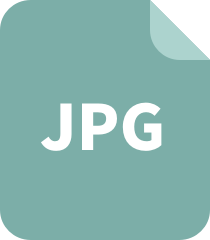
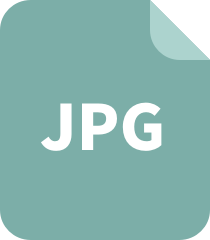
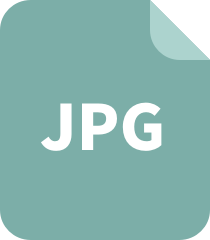
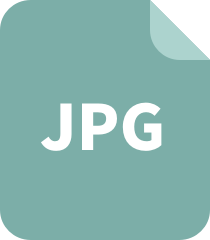
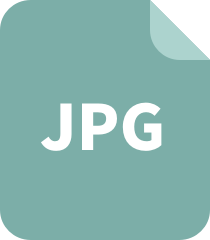
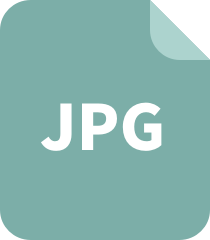
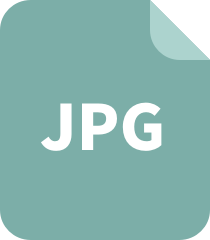
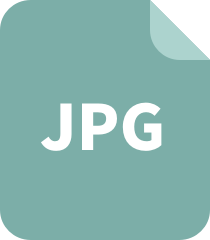
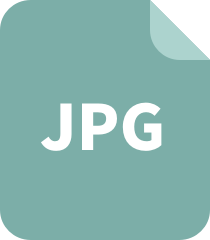
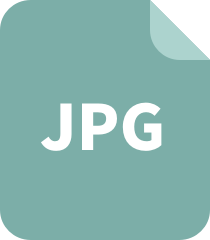
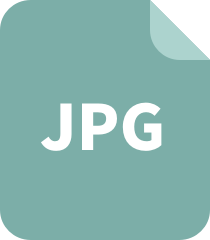
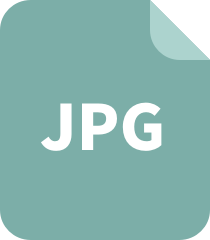
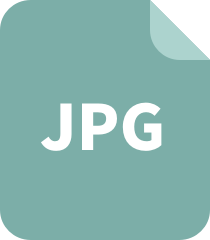
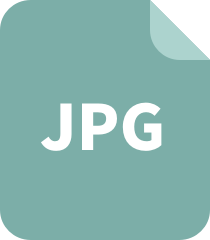
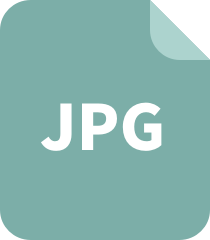
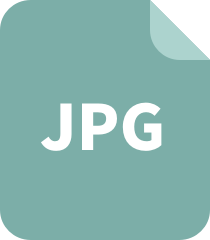
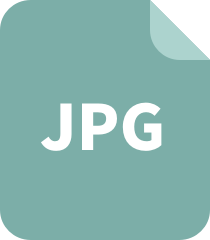
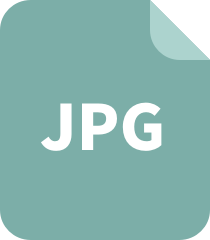
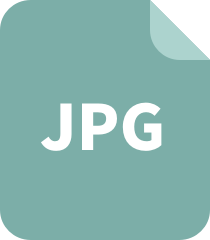
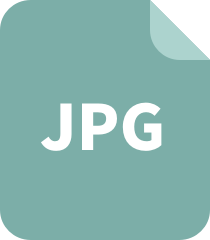
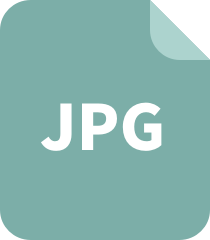
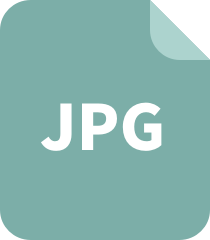
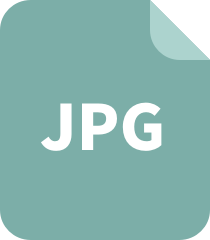
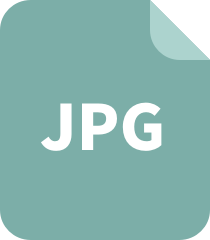
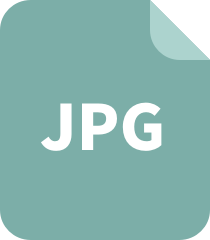
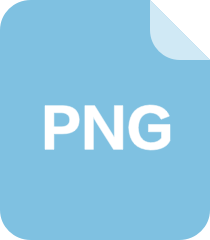
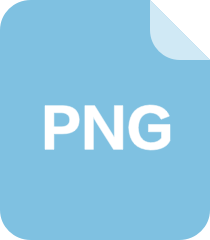
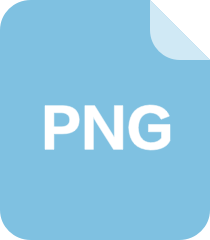
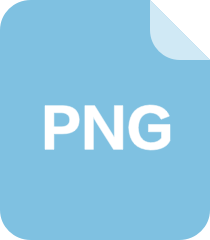
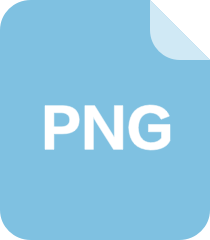
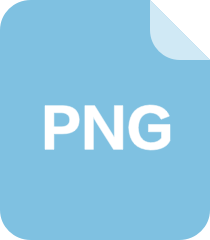
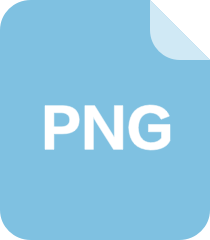
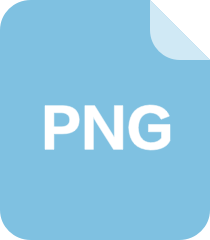
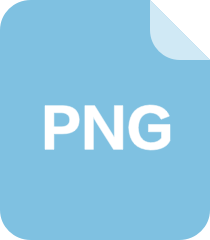
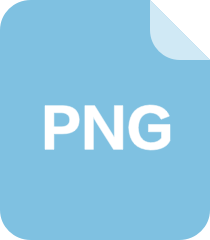
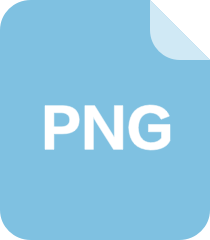
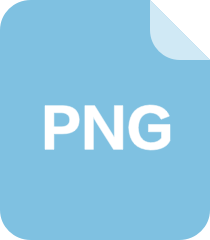
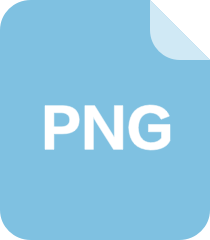
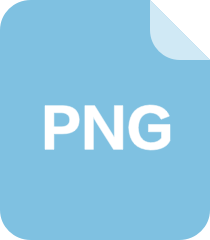
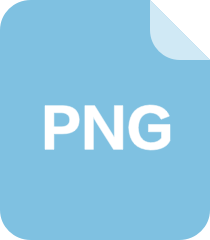
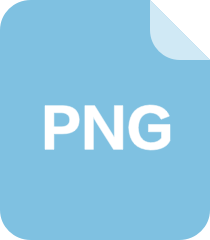
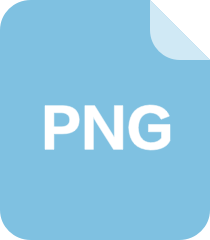
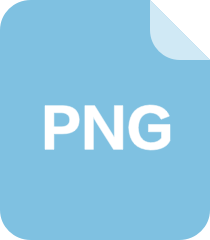
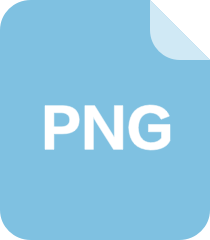
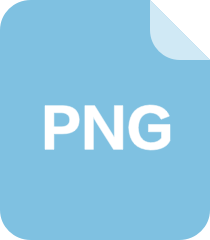
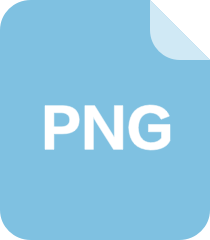
共 316 条
- 1
- 2
- 3
- 4

不吃咸鱼的猫
- 粉丝: 3
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

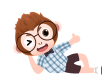
最新资源
- CocosCreator源码资源SrcPackage2(6款源码合集)
- (源码)基于Spring Boot和Spring Cloud的权限管理系统.zip
- CocosCreator源码资源SrcPackage1(11款源码合集)
- (源码)基于Python和Kafka的微博热搜情感分析系统.zip
- 毕业设计《HTML5-Bootstrap-SSM校园导游咨询网(可升级SpringBoot)》+Java项目源码+文档说明
- (源码)基于Arduino的智能导盲犬系统.zip
- sentinel-dashboard的1.8.6版本集成nacos,对接gateway的限流
- CocosCreator源码资源Snaker(贪吃蛇 精品)
- (源码)基于C语言的智能仓库管理系统(IWMS).zip
- (源码)基于Unity的通用升级系统.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


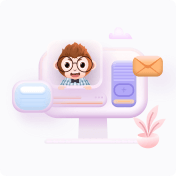
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页