基于MATLAB的条形码数字分割和识别算法仿真-源码
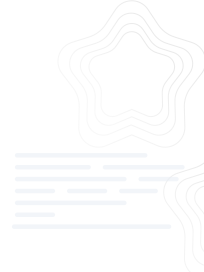

2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
在IT领域,条形码数字分割与识别是自动识别技术中的一个重要组成部分,广泛应用于物流、零售、仓储等行业的数据管理。本项目以MATLAB为平台,实现了一套条形码数字分割和识别的算法仿真,这有助于理解条形码识别的基本原理,并为实际应用提供参考。MATLAB是一款强大的数学计算软件,同时也非常适合进行图像处理和算法开发。 在MATLAB中,我们可以利用其丰富的图像处理函数来处理条形码图像。条形码数字分割涉及预处理步骤,包括图像去噪、二值化和边缘检测。在这个阶段,可以使用`imfilter`进行滤波操作,`im2bw`将图像转换为黑白二值图像,以及`edge`函数检测图像边缘。这些步骤旨在突出条形码的条和空,以便后续的分析。 接下来,对二值图像进行细化处理,如使用Canny算法或Hough变换来准确地找到条形码的垂直线条。MATLAB中的`canny`函数可用于Canny边缘检测,而`hough`函数则支持Hough变换,这两种方法都能有效地检测出图像中的直线。 在条形码的数字分割阶段,需要确定每个数字的边界。这通常通过连通组件分析实现,MATLAB的`bwlabel`函数能标识并标记图像中的连通区域。通过分析这些连通组件的大小和位置,可以将条形码的每个数字区分开来。 一旦数字被分割,接下来就是识别阶段。这里可以采用模板匹配或者机器学习方法,如SVM(支持向量机)或神经网络。MATLAB提供了`templateMatch`函数用于模板匹配,以及`svmtrain`和`svmclassify`函数支持SVM训练和分类。对于更复杂的识别任务,可以利用`neuralnet`构建和训练神经网络模型。 在提供的源码中,读者可以深入学习如何在MATLAB中实现这些步骤,包括数据结构的处理、图像函数的应用以及算法的调优。通过阅读和理解代码,不仅可以提升MATLAB编程技能,还能掌握条形码识别的核心技术,为实际项目开发打下坚实基础。 此外,了解如何在MATLAB中开发软件或插件也是很重要的。MATLAB提供了App Designer工具,使得用户能够创建交互式的图形用户界面(GUI)。通过结合算法代码和GUI,可以将条形码识别功能封装成一个易于使用的软件工具。 这个基于MATLAB的条形码数字分割和识别算法仿真项目,涵盖了图像处理、模式识别、算法设计等多个IT领域的核心知识点。无论是对学术研究还是工业应用,都具有很高的参考价值。通过对源码的深入学习和实践,开发者可以增强自己的图像处理技能,提高解决实际问题的能力。
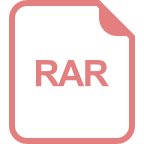
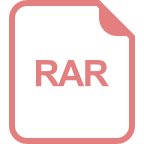
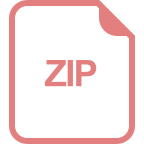
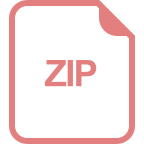
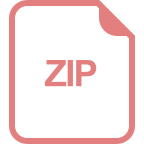
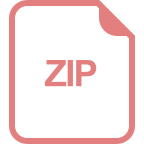
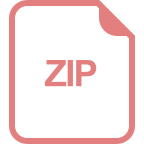
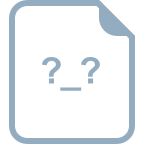
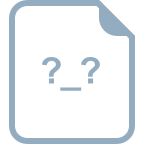
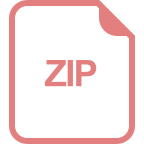
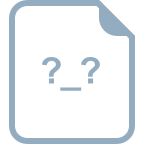
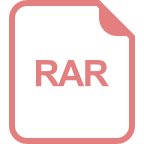
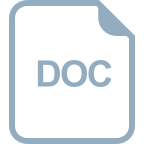
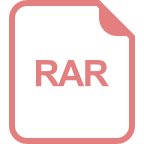
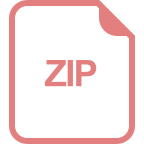
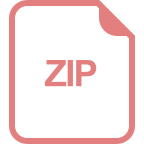
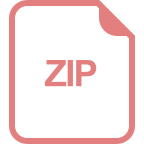
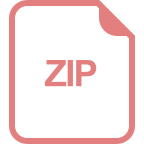
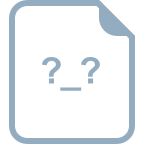
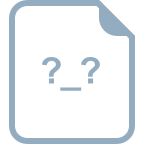
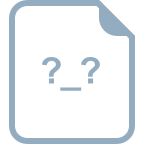
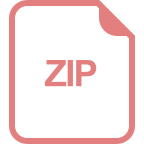
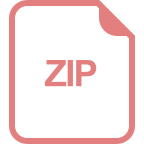



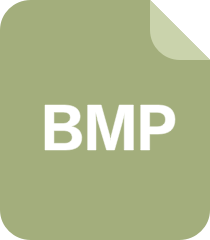
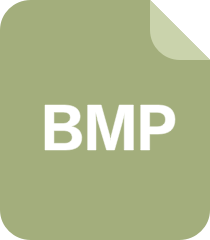
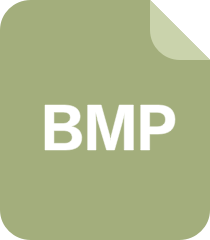
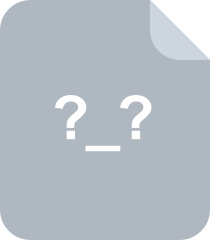
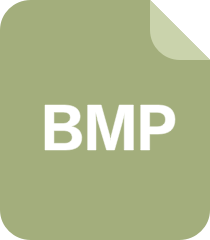
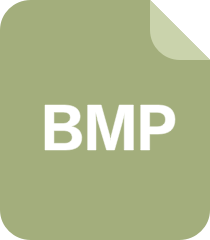

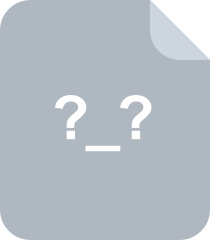
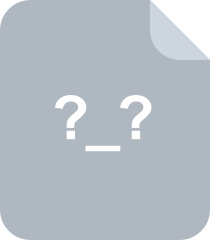
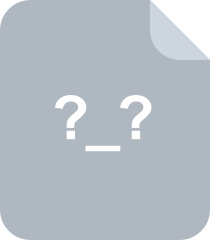
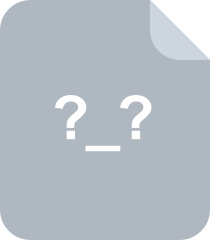
- 1
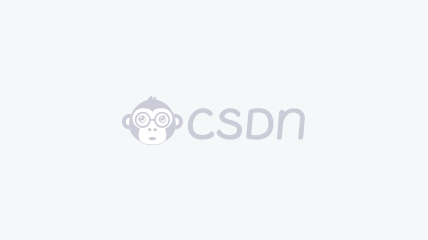

- 粉丝: 2230
- 资源: 19万+





我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

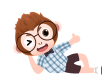
最新资源
- 机械设计巧克力自动切割成型机sw17可编辑非常好的设计图纸100%好用.zip
- 机械设计全自动多功能压力机sw17可编辑非常好的设计图纸100%好用.zip
- 三种步长的MPPT仿真效果对比(变步长、大步长、小步长) ①仿真模型:包含三种仿真 放在同一个仿真中进行比对 1大步长扰动观察法:虽然能够迅速到达最大功率点,但是稳定的时候稳态震荡比较大(如下图
- yhhhhbnhjbhbhj
- 机械设计潜伏式AGV小车sw18可编辑非常好的设计图纸100%好用.zip
- 永磁同步电机新型非奇异快速终端滑模电流预测控制 速度控制器是一种新型非奇异滑模面,电流控制器是一种无差拿电流预测控制,同时使用扩张观测器观测负载扰动
- 直流电压源+双向Buck-Boost DCDC变器+负载+锂离子电池+控制系统,Simulink仿真模型 有两种工作模式: 1锂离子电池经双向DCDC变器为负载供电 2直流可控电压源为负载供电同时经
- dsp28335基于模型的设计,自动代码生成,还有各种外设的驱动库
- ArcGIS地理空间平台任意文件读取漏洞及其复现方法详解与安全测试案例
- ad9371参考设计,移植 基于kcu105+adrv9371板卡,通过adi iio oscilloscope软件进行操控和查看 提供移植支持和工程 包含hdl工程、vitis工程、各种文档、文件
- 顺景ERP管理系统UploadInvtSpBuzPlanFile接口任意文件上传漏洞详细分析与POC验证
- 蓝桥杯java算法学习笔记(强烈推荐!!!!对新手小白非常友好)
- 网络存储设备安全:D-Link NAS(account-mgr.cgi)未授权远程命令执行漏洞解析与POC
- 1000万豆瓣电影,评论,名人,评分数据源码采集分享(内含千万电影数据集,可下载).zip
- 基于线性预测的语音合成实验MATLAB代码
- AI for Science 论文解读合集(持续更新ing),论文,数据集,教程下载hyper.ai.zip

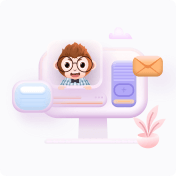
