# DEPRECATED
This library is not maintained anymore and there will be no further releases except for very critical bug fixes. Use [WorkManager](https://github.com/evernote/android-job#workmanager) instead of this library.
# ~~Android-Job~~
A utility library for Android to run jobs delayed in the background. Depending on the Android version either the `JobScheduler`, `GcmNetworkManager` or `AlarmManager` is getting used. You can find out in [this blog post](https://blog.evernote.com/tech/2015/10/26/unified-job-library-android/) or in [these slides](https://speakerdeck.com/vrallev/doo-z-z-z-z-z-e?slide=50) why you should prefer this library than each separate API. All features from Android Oreo are backward compatible back to Ice Cream Sandwich.
## Target SDK 31
When targeting API 31 and you still use this library (you really should not and migrate to WorkManager instead), then don't forget to add the [exact alarm permission](https://developer.android.com/about/versions/12/behavior-changes-12#exact-alarm-permission) when using exact jobs.
## Download
Download [the latest version](http://search.maven.org/#search|gav|1|g:"com.evernote"%20AND%20a:"android-job") or grab via Gradle:
```groovy
dependencies {
implementation 'com.evernote:android-job:1.4.3'
}
```
Starting with version `1.3.0` the library will use the `WorkManager` internally, please read the [documentation](https://github.com/evernote/android-job#workmanager) and opt-in.
If you didn't turn off the manifest merger from the Gradle build tools, then no further step is required to setup the library. Otherwise you manually need to add the permissions and services like in this [AndroidManifest](library/src/main/AndroidManifest.xml).
You can read the [JavaDoc here](https://evernote.github.io/android-job/javadoc/).
## Usage
The class `JobManager` serves as entry point. Your jobs need to extend the class `Job`. Create a `JobRequest` with the corresponding builder class and schedule this request with the `JobManager`.
Before you can use the `JobManager` you must initialize the singleton. You need to provide a `Context` and add a `JobCreator` implementation after that. The `JobCreator` maps a job tag to a specific job class. It's recommended to initialize the `JobManager` in the `onCreate()` method of your `Application` object, but there is [an alternative](https://github.com/evernote/android-job/wiki/FAQ#i-cannot-override-the-application-class-how-can-i-add-my-jobcreator), if you don't have access to the `Application` class.
```java
public class App extends Application {
@Override
public void onCreate() {
super.onCreate();
JobManager.create(this).addJobCreator(new DemoJobCreator());
}
}
```
```java
public class DemoJobCreator implements JobCreator {
@Override
@Nullable
public Job create(@NonNull String tag) {
switch (tag) {
case DemoSyncJob.TAG:
return new DemoSyncJob();
default:
return null;
}
}
}
```
After that you can start scheduling jobs.
```java
public class DemoSyncJob extends Job {
public static final String TAG = "job_demo_tag";
@Override
@NonNull
protected Result onRunJob(Params params) {
// run your job here
return Result.SUCCESS;
}
public static void scheduleJob() {
new JobRequest.Builder(DemoSyncJob.TAG)
.setExecutionWindow(30_000L, 40_000L)
.build()
.schedule();
}
}
```
## Advanced
The `JobRequest.Builder` class has many extra options, e.g. you can specify a required network connection, make the job periodic, pass some extras with a bundle, restore the job after a reboot or run the job at an exact time.
Each job has a unique ID. This ID helps to identify the job later to update requirements or to cancel the job.
```java
private void scheduleAdvancedJob() {
PersistableBundleCompat extras = new PersistableBundleCompat();
extras.putString("key", "Hello world");
int jobId = new JobRequest.Builder(DemoSyncJob.TAG)
.setExecutionWindow(30_000L, 40_000L)
.setBackoffCriteria(5_000L, JobRequest.BackoffPolicy.EXPONENTIAL)
.setRequiresCharging(true)
.setRequiresDeviceIdle(false)
.setRequiredNetworkType(JobRequest.NetworkType.CONNECTED)
.setExtras(extras)
.setRequirementsEnforced(true)
.setUpdateCurrent(true)
.build()
.schedule();
}
private void schedulePeriodicJob() {
int jobId = new JobRequest.Builder(DemoSyncJob.TAG)
.setPeriodic(TimeUnit.MINUTES.toMillis(15), TimeUnit.MINUTES.toMillis(5))
.build()
.schedule();
}
private void scheduleExactJob() {
int jobId = new JobRequest.Builder(DemoSyncJob.TAG)
.setExact(20_000L)
.build()
.schedule();
}
private void runJobImmediately() {
int jobId = new JobRequest.Builder(DemoSyncJob.TAG)
.startNow()
.build()
.schedule();
}
private void cancelJob(int jobId) {
JobManager.instance().cancel(jobId);
}
```
If a non periodic `Job` fails, then you can reschedule it with the defined back-off criteria.
```java
public class RescheduleDemoJob extends Job {
@Override
@NonNull
protected Result onRunJob(Params params) {
// something strange happened, try again later
return Result.RESCHEDULE;
}
@Override
protected void onReschedule(int newJobId) {
// the rescheduled job has a new ID
}
}
```
#### Proguard
The library doesn't use reflection, but it relies on three `Service`s and two `BroadcastReceiver`s. In order to avoid any issues, you shouldn't obfuscate those four classes. The library bundles its own Proguard config and you don't need to do anything, but just in case you can add [these rules](library/proguard.cfg) in your configuration.
## More questions?
See the [FAQ](https://github.com/evernote/android-job/wiki/FAQ) in the [Wiki](https://github.com/evernote/android-job/wiki).
## WorkManager
[WorkManager](https://developer.android.com/topic/libraries/architecture/workmanager) is a new architecture component from Google and tries to solve a very similar problem this library tries to solve: implementing background jobs only once for all Android versions. The API is very similar to this library, but provides more features like chaining work items and it runs its own executor.
If you start a new project, you should be using `WorkManager` instead of this library. You should also start migrating your code from this library to `WorkManager`. At some point in the future this library will be deprecated.
Starting with version `1.3.0` this library will use the `WorkManager` internally for scheduling jobs. That should ease the transition to the new architecture component. You only need to add the `WorkManager` to your classpath, e.g.
```groovy
dependencies {
implementation "android.arch.work:work-runtime:$work_version"
}
```
Please take a look at the [Wiki](https://github.com/evernote/android-job/wiki/Migrating-to-WorkManager) for a complete transition guide.
The API and feature set of `android-job` and `WorkManager` are really similar. However, some features are unique and only supported by one or the other
| Feature | android-job | WorkManager |
| --- | --- | --- |
| Exact jobs | Yes | No |
| Transient jobs | Yes | No |
| Daily jobs | Yes | No |
| Custom Logger | Yes | No |
| Observe job status | No | Yes |
| Chained jobs | No | Yes |
| Work sequences | No | Yes |
## Google Play Services
This library does **not** automatically bundle the Google Play Services, because the dependency is really heavy and not all apps want to include them. That's why you need to add the dependency manually, if you want that the library uses the `GcmNetworkManager` on Android 4, then include the following dependency.
```groovy
dependen
没有合适的资源?快使用搜索试试~ 我知道了~
android-job用于运行后台延迟的作业
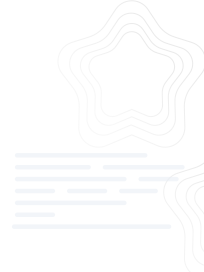
共147个文件
java:98个
xml:13个
png:8个

需积分: 5 0 下载量 198 浏览量
2023-08-21
23:03:23
上传
评论
收藏 296KB ZIP 举报
温馨提示
Android的实用程序库,用于运行后台延迟的作业。根据Android版本,将使用“JobScheduler”、“GcmNetworkManager”或“AlarmManager”。你可以在[这篇博客文章]中找到(https://blog.evernote.com/tech/2015/10/26/unified-job-library-android/)或在[这些幻灯片]中(https://speakerdeck.com/vrallev/doo-z-z-z-z-z-e?slide=50)为什么您应该更喜欢这个库而不是每个单独的API。安卓奥利奥的所有功能都向后兼容到冰淇淋三明治。
资源推荐
资源详情
资源评论
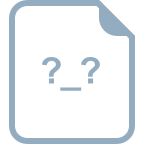
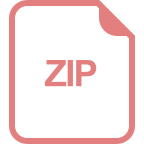
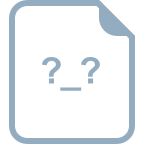
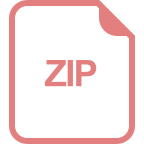
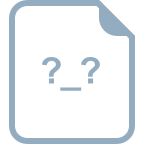
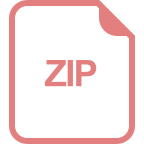
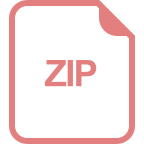
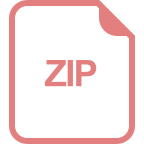
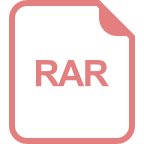
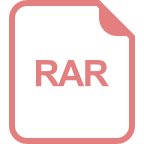
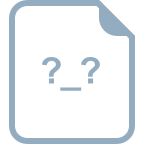
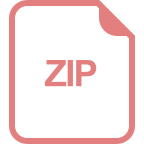
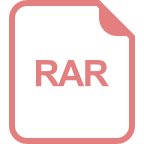
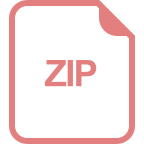
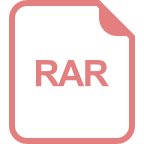
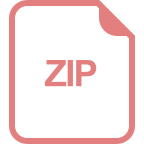
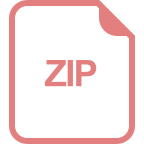
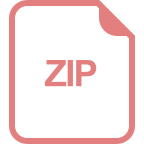
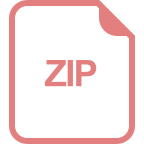
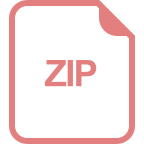
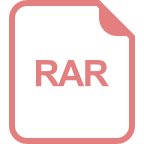
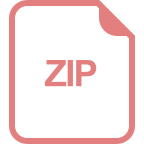
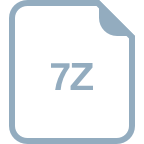
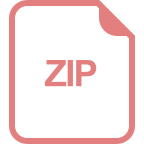
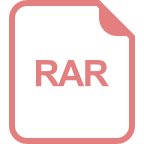
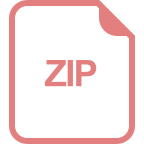
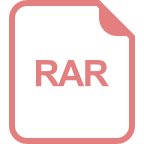
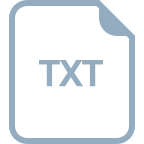
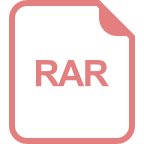
收起资源包目录

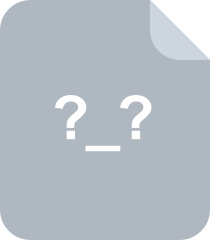
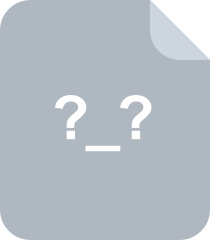
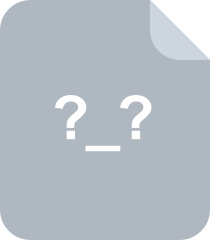
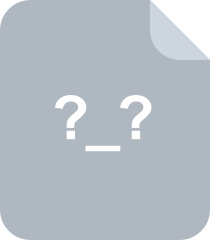
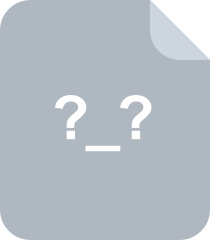
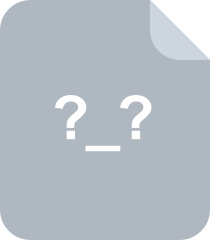
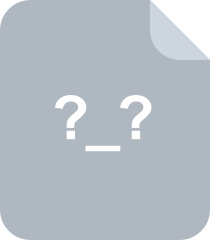
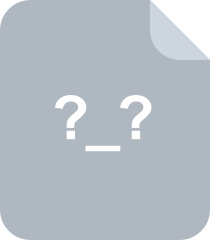
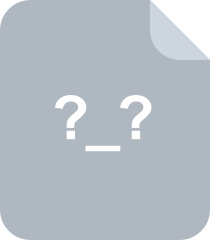
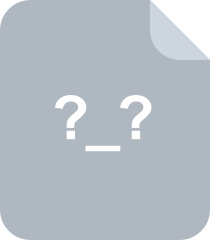
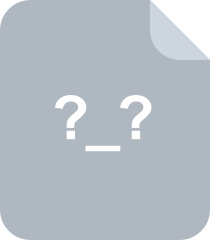
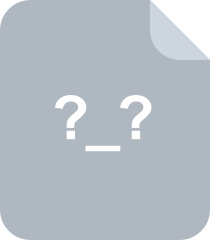
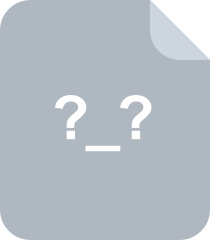
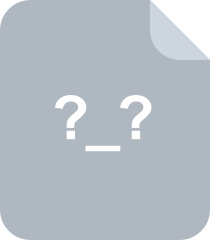
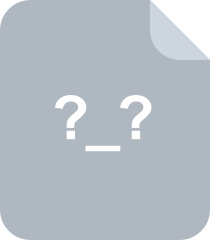
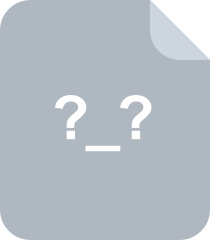
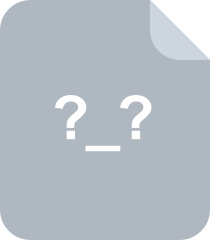
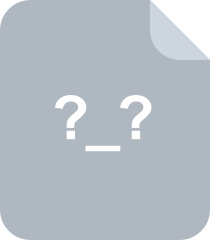
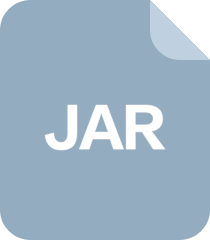
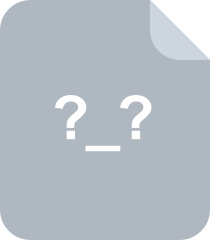
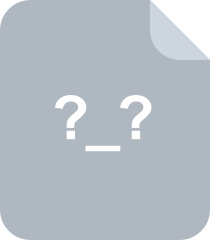
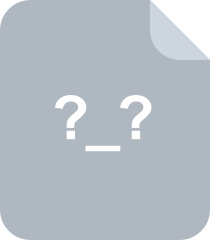
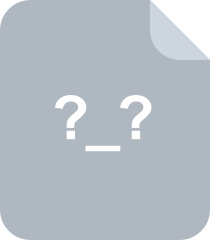
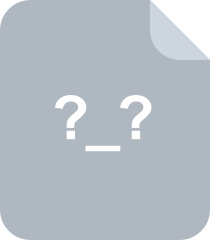
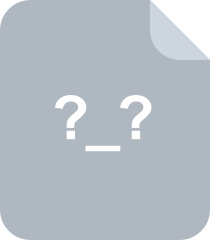
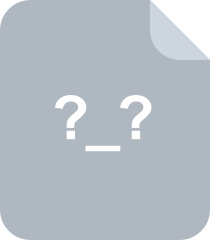
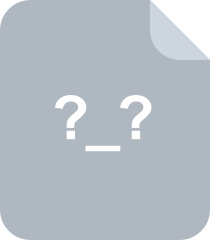
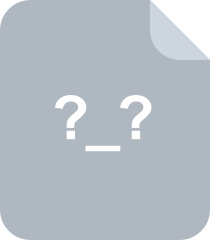
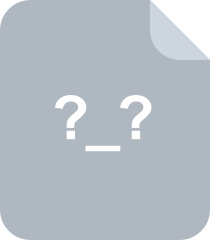
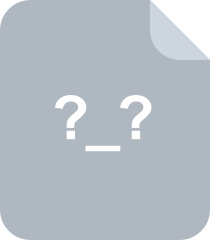
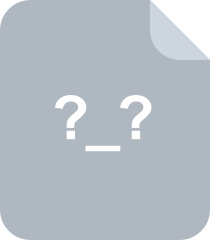
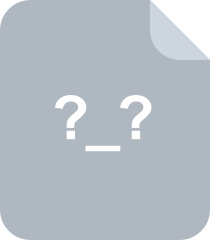
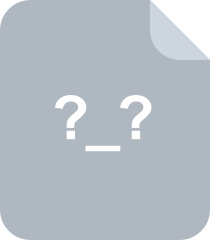
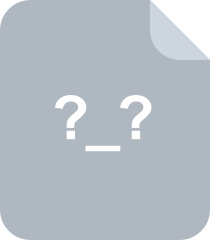
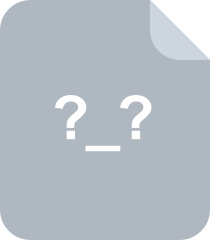
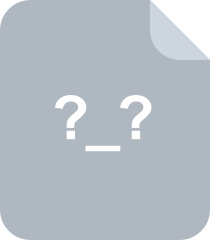
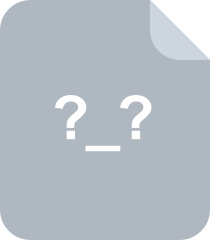
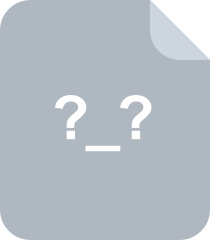
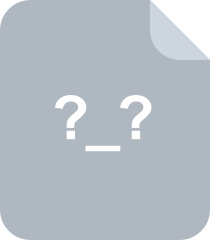
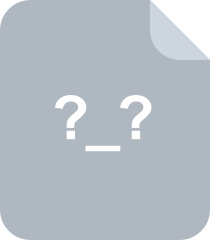
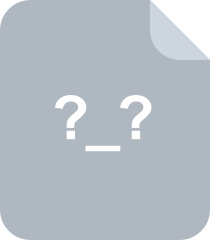
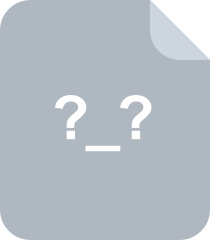
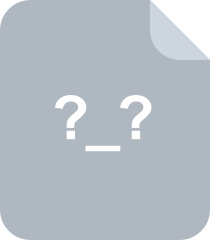
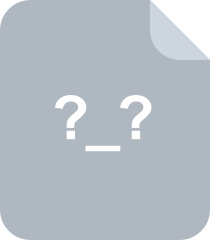
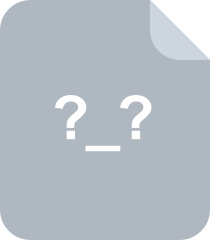
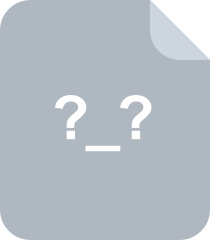
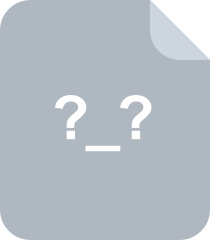
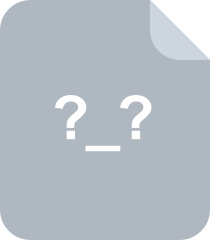
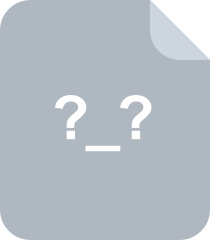
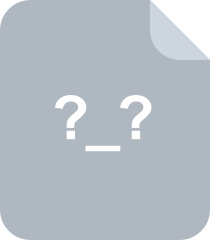
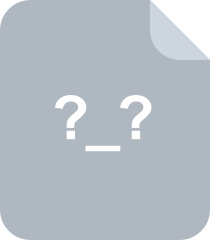
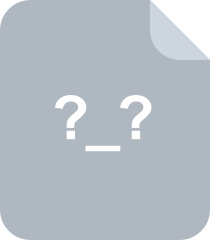
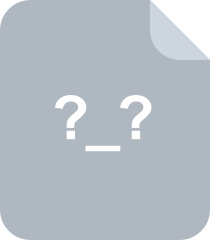
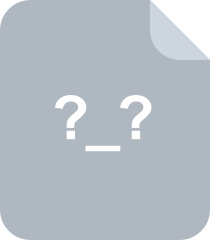
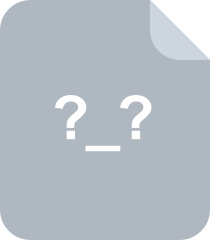
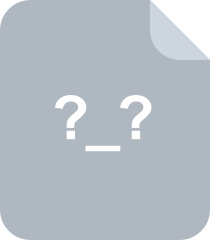
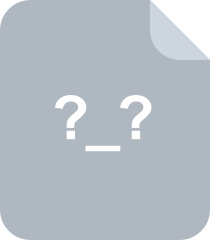
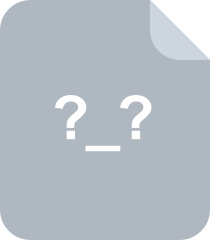
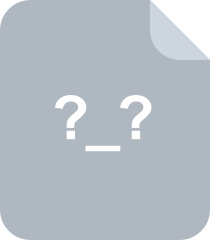
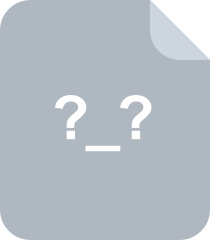
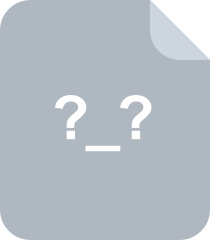
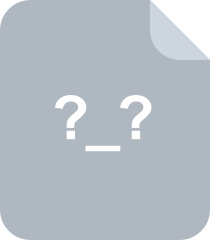
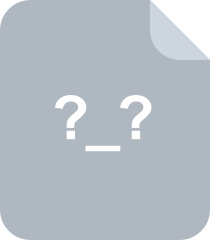
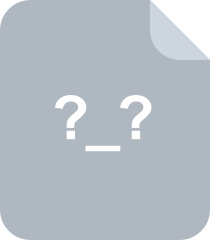
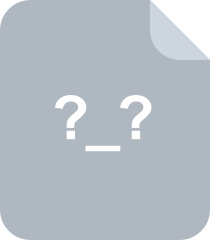
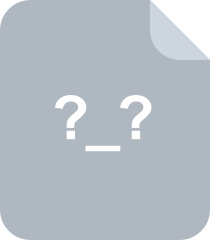
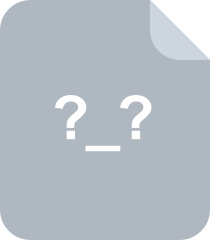
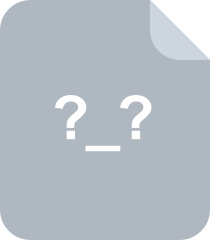
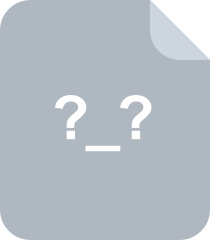
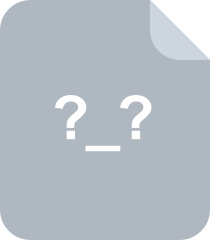
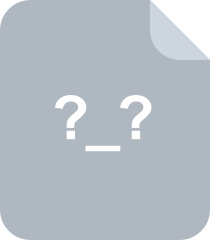
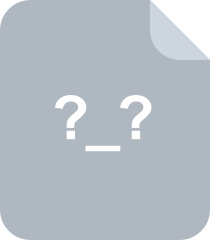
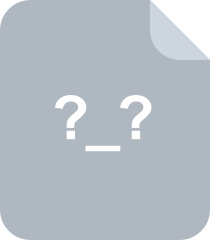
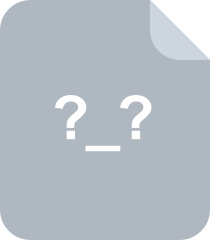
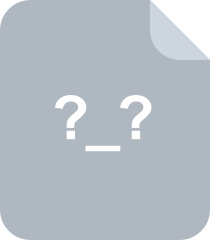
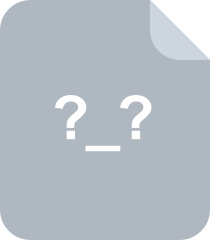
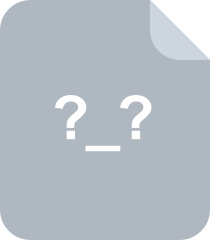
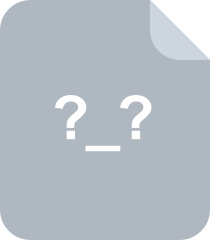
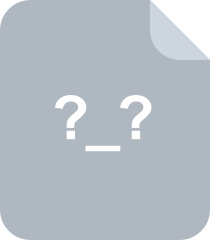
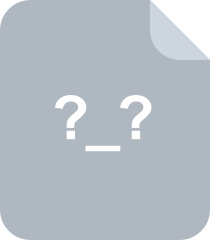
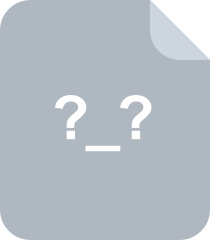
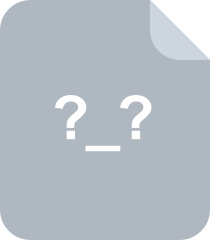
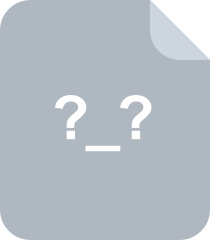
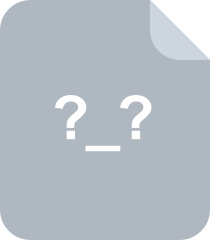
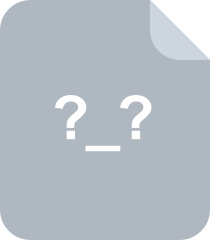
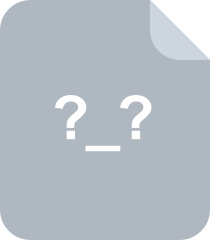
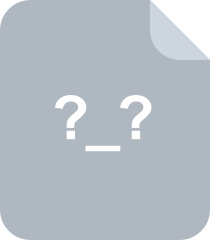
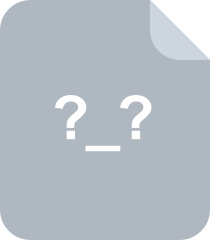
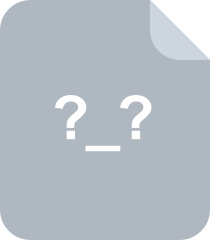
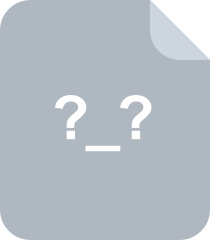
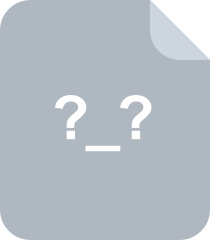
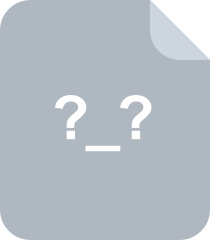
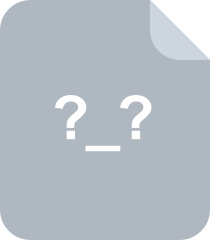
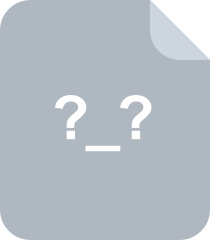
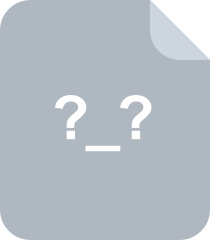
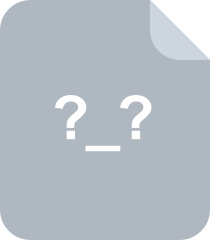
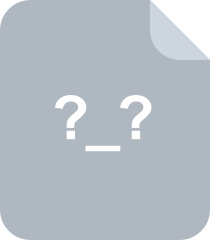
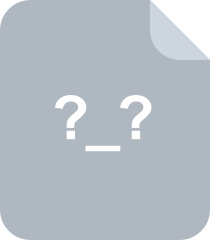
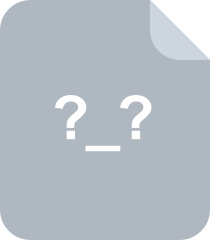
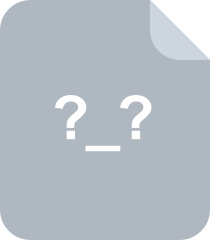
共 147 条
- 1
- 2
资源评论
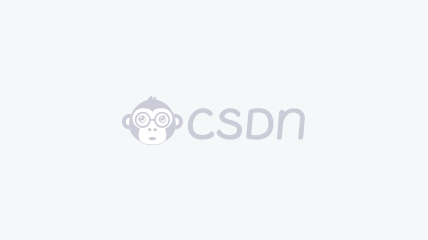

hello_中年人
- 粉丝: 7
- 资源: 324
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

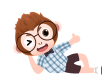
最新资源
- HtmlMate标签使用详解中文最新版本
- ATM机旁危险物品检测数据集VOC+YOLO格式1251张5类别.zip
- 网页优化meta标签使用方法及规则中文最新版本
- 网页万能复制 浏览器插件
- IMG_20241123_093226.jpg
- JavaScript的表白代码项目源码.zip
- springboot vue3前后端分离开发入门介绍,分享给有需要的人,仅供参考
- 全国297个地级市城市辖区数据1990-2022年末实有公共汽车出租车数人均城市道路建成区绿地面积供水供气总量医院卫生机构数医生人数GDP第一二三产业增加值分行业从业人员水资源农产品产量利用外资
- Python客流量时间序列预测模型.zip
- 故障预测-灰色预测模型C++源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


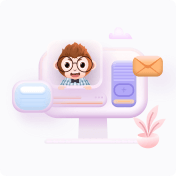
安全验证
文档复制为VIP权益,开通VIP直接复制
