package com.v512.demo.hibernate;
import org.hibernate.HibernateException;
import org.hibernate.Session;
import org.hibernate.cfg.Configuration;
/**
* Configures and provides access to Hibernate sessions, tied to the
* current thread of execution. Follows the Thread Local Session
* pattern, see {@link http://hibernate.org/42.html }.
*/
public class HibernateSessionFactory {
/**
* Location of hibernate.cfg.xml file.
* Location should be on the classpath as Hibernate uses
* #resourceAsStream style lookup for its configuration file.
* The default classpath location of the hibernate config file is
* in the default package. Use #setConfigFile() to update
* the location of the configuration file for the current session.
*/
private static String CONFIG_FILE_LOCATION = "/hibernate.cfg.xml";
private static final ThreadLocal<Session> threadLocal = new ThreadLocal<Session>();
private static Configuration configuration = new Configuration();
private static org.hibernate.SessionFactory sessionFactory;
private static String configFile = CONFIG_FILE_LOCATION;
static {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
private HibernateSessionFactory() {
}
/**
* Returns the ThreadLocal Session instance. Lazy initialize
* the <code>SessionFactory</code> if needed.
*
* @return Session
* @throws HibernateException
*/
public static Session getSession() throws HibernateException {
Session session = (Session) threadLocal.get();
if (session == null || !session.isOpen()) {
if (sessionFactory == null) {
rebuildSessionFactory();
}
session = (sessionFactory != null) ? sessionFactory.openSession()
: null;
threadLocal.set(session);
}
return session;
}
/**
* Rebuild hibernate session factory
*
*/
public static void rebuildSessionFactory() {
try {
configuration.configure(configFile);
sessionFactory = configuration.buildSessionFactory();
} catch (Exception e) {
System.err
.println("%%%% Error Creating SessionFactory %%%%");
e.printStackTrace();
}
}
/**
* Close the single hibernate session instance.
*
* @throws HibernateException
*/
public static void closeSession() throws HibernateException {
Session session = (Session) threadLocal.get();
threadLocal.set(null);
if (session != null) {
session.close();
}
}
/**
* return session factory
*
*/
public static org.hibernate.SessionFactory getSessionFactory() {
return sessionFactory;
}
/**
* return session factory
*
* session factory will be rebuilded in the next call
*/
public static void setConfigFile(String configFile) {
HibernateSessionFactory.configFile = configFile;
sessionFactory = null;
}
/**
* return hibernate configuration
*
*/
public static Configuration getConfiguration() {
return configuration;
}
}
hibernate,hibernate实例源码
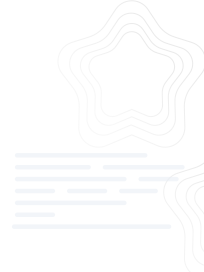

【hibernate,hibernate实例源码】 Hibernate是一个开源的对象关系映射(ORM)框架,它极大地简化了Java应用程序与数据库之间的交互。通过提供一套API和元数据配置,Hibernate可以将Java对象自动持久化到关系数据库中,使得开发者无需直接编写SQL语句,而是以面向对象的方式来操作数据。 一、Hibernate核心概念 1. 实体(Entity):在Hibernate中,实体通常对应于数据库中的表,是一个具有唯一标识的对象。它们通过@Entity注解标记,并通过@Id注解指定主键字段。 2. 映射(Mapping):Hibernate使用XML文件(hbm.xml)或注解来描述对象和表之间的映射关系,包括字段对应、关系映射等。 3. 会话(Session):是Hibernate与数据库交互的桥梁,负责在应用程序和数据库之间管理临时状态。它提供了事务处理、缓存管理和对象持久化的功能。 4. 事务(Transaction):在Hibernate中,事务处理由Session管理,可以确保数据的一致性和完整性。 5. 查询(Querying):Hibernate提供HQL(Hibernate Query Language)和Criteria API进行数据查询,它们都是面向对象的查询语言,更接近于Java语法。 二、Hibernate实例详解 在Hibernate实例中,通常包括以下几个步骤: 1. 配置:创建hibernate.cfg.xml文件,配置数据库连接信息、实体类扫描路径等。 2. 实体类定义:创建Java类,使用注解或XML文件定义实体类与数据库表的映射关系。 3. SessionFactory创建:通过Configuration类加载配置文件,构建SessionFactory对象,它是线程不安全的,通常在应用启动时创建一次。 4. Session操作:使用SessionFactory创建Session对象,通过Session执行CRUD(Create, Read, Update, Delete)操作。 5. 事务处理:在Session中开启和提交事务,确保数据一致性。 6. 查询数据:使用Session的createQuery()或createCriteria()方法,执行HQL或Criteria查询。 三、源码分析 源码分析是深入理解Hibernate工作原理的关键。通过阅读和理解以下关键组件的源码: 1. Configuration:配置加载和解析的过程。 2. SessionFactory:如何根据配置信息创建SessionFactory,以及其内部的缓存机制。 3. Session:如何执行SQL语句,如何管理一级缓存和二级缓存。 4. Query和Criteria:查询构造过程,如何生成最终的SQL语句。 5. Persistence Context:了解Hibernate如何跟踪对象的状态(瞬时、持久化、脱管)。 四、实例应用场景 1. 网络购物系统:用于用户信息、商品信息、订单数据的存储和检索。 2. 社交媒体平台:用户资料、帖子、评论的数据管理。 3. 在线教育平台:课程、学生、教师信息的持久化。 4. CRM系统:客户、销售、订单等业务数据的处理。 通过深入学习和实践Hibernate,开发者可以提高开发效率,减少对SQL的依赖,同时利用其强大的查询能力和缓存机制优化数据库操作。源码分析则有助于理解其内部机制,为优化性能和解决问题提供依据。






























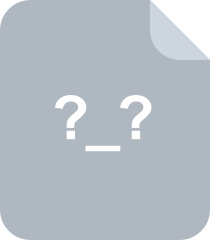





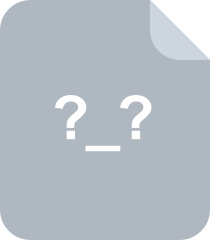
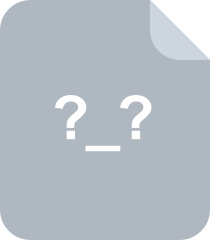
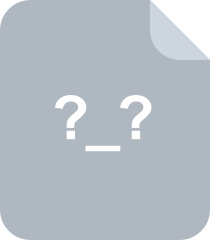
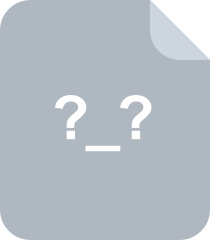
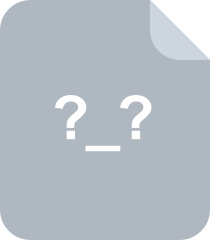
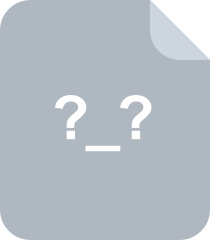





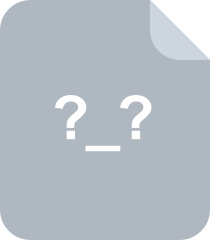
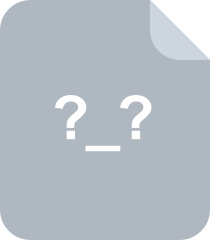
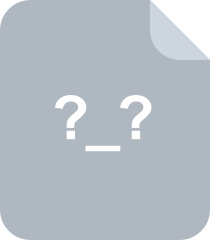
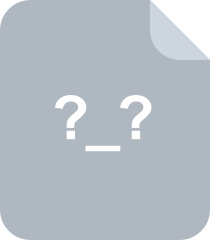
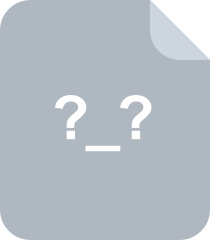
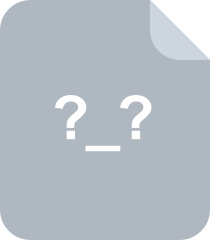
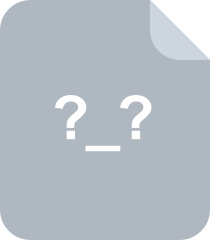
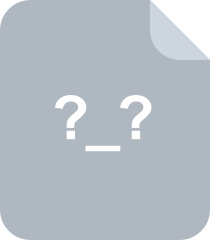
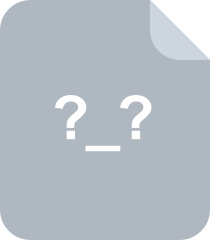

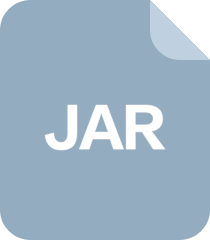
- 1
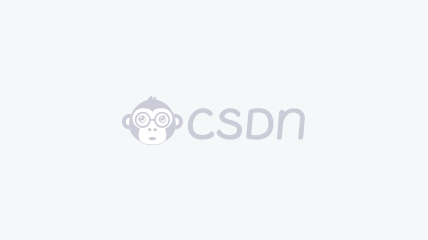
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 0
- 资源: 34
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

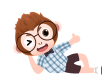
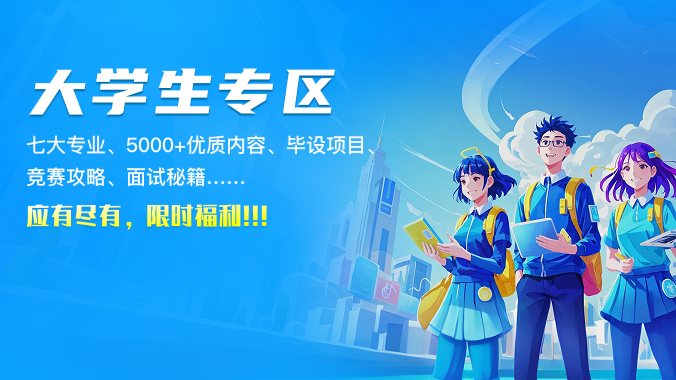
最新资源
- 【大数据技术】HBase从安装到实操全面解析:涵盖环境搭建、基础操作及故障排除HBase的安装
- 论文模板111111.doc
- 基于springboot技术的经方药食两用服务平台(完整Java源码+数据库sql文件+项目文档+Java项目编程实战+编程练手好项目).zip
- 基于springboot技术的在线旅游管理系统平台(完整Java源码+数据库sql文件+项目文档+Java项目编程实战+编程练手好项目).zip
- 南宁周边乡村游系统 2025免费JAVA微信小程序毕设
- 旅游社交系统 2025免费JAVA微信小程序毕设
- 基于springboot技术的高校教师电子名片系统(完整Java源码+数据库sql文件+项目文档+Java项目编程实战+编程练手好项目).zip
- 基于SpringBoot技术的企业员工薪酬关系系统的设计(完整Java源码+数据库sql文件+项目文档+Java项目编程实战+编程练手好项目).zip
- 农场驿站平台 2025免费JAVA微信小程序毕设
- ca26b508c584466e872d6427e2fe6842.part13
- 社区团购 2025免费JAVA微信小程序毕设
- 跑腿管理系统 2025免费JAVA微信小程序毕设
- 基于SpringBoot技术的在线远程考试系统的设计与实现(完整Java源码+数据库sql文件+项目文档+Java项目编程实战+编程练手好项目).zip
- 基于SpringBoot+Vue技术的乡政府管理系统(完整Java源码+数据库sql文件+项目文档+Java项目编程实战+编程练手好项目).zip
- 书橱管理系统 2025免费JAVA微信小程序毕设
- 随堂测系统 2025免费JAVA微信小程序毕设

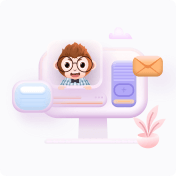
