/*!
\file gd32f30x_enet.c
\brief ENET driver
\version 2017-02-10, V1.0.0, firmware for GD32F30x
\version 2018-10-10, V1.1.0, firmware for GD32F30x
\version 2018-12-25, V2.0.0, firmware for GD32F30x
\version 2020-04-02, V2.0.1, firmware for GD32F30x
\version 2020-09-30, V2.1.0, firmware for GD32F30x
*/
/*
Copyright (c) 2020, GigaDevice Semiconductor Inc.
Redistribution and use in source and binary forms, with or without modification,
are permitted provided that the following conditions are met:
1. Redistributions of source code must retain the above copyright notice, this
list of conditions and the following disclaimer.
2. Redistributions in binary form must reproduce the above copyright notice,
this list of conditions and the following disclaimer in the documentation
and/or other materials provided with the distribution.
3. Neither the name of the copyright holder nor the names of its contributors
may be used to endorse or promote products derived from this software without
specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS"
AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT,
INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE)
ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY
OF SUCH DAMAGE.
*/
#include "gd32f30x_enet.h"
#include <stdlib.h>
#ifdef GD32F30X_CL
#if defined (__CC_ARM) /*!< ARM compiler */
__align(4)
enet_descriptors_struct rxdesc_tab[ENET_RXBUF_NUM]; /*!< ENET RxDMA descriptor */
__align(4)
enet_descriptors_struct txdesc_tab[ENET_TXBUF_NUM]; /*!< ENET TxDMA descriptor */
__align(4)
uint8_t rx_buff[ENET_RXBUF_NUM][ENET_RXBUF_SIZE]; /*!< ENET receive buffer */
__align(4)
uint8_t tx_buff[ENET_TXBUF_NUM][ENET_TXBUF_SIZE]; /*!< ENET transmit buffer */
#elif defined ( __ICCARM__ ) /*!< IAR compiler */
#pragma data_alignment=4
enet_descriptors_struct rxdesc_tab[ENET_RXBUF_NUM]; /*!< ENET RxDMA descriptor */
#pragma data_alignment=4
enet_descriptors_struct txdesc_tab[ENET_TXBUF_NUM]; /*!< ENET TxDMA descriptor */
#pragma data_alignment=4
uint8_t rx_buff[ENET_RXBUF_NUM][ENET_RXBUF_SIZE]; /*!< ENET receive buffer */
#pragma data_alignment=4
uint8_t tx_buff[ENET_TXBUF_NUM][ENET_TXBUF_SIZE]; /*!< ENET transmit buffer */
#elif defined (__GNUC__) /* GNU Compiler */
enet_descriptors_struct rxdesc_tab[ENET_RXBUF_NUM] __attribute__ ((aligned (4))); /*!< ENET RxDMA descriptor */
enet_descriptors_struct txdesc_tab[ENET_TXBUF_NUM] __attribute__ ((aligned (4))); /*!< ENET TxDMA descriptor */
uint8_t rx_buff[ENET_RXBUF_NUM][ENET_RXBUF_SIZE] __attribute__ ((aligned (4))); /*!< ENET receive buffer */
uint8_t tx_buff[ENET_TXBUF_NUM][ENET_TXBUF_SIZE] __attribute__ ((aligned (4))); /*!< ENET transmit buffer */
#endif /* __CC_ARM */
/* global transmit and receive descriptors pointers */
enet_descriptors_struct *dma_current_txdesc;
enet_descriptors_struct *dma_current_rxdesc;
/* structure pointer of ptp descriptor for normal mode */
enet_descriptors_struct *dma_current_ptp_txdesc = NULL;
enet_descriptors_struct *dma_current_ptp_rxdesc = NULL;
/* init structure parameters for ENET initialization */
static enet_initpara_struct enet_initpara ={0,0,0,0,0,0,0,0,0,0,0,0,0,0,0};
static uint32_t enet_unknow_err = 0U;
/* array of register offset for debug information get */
static const uint16_t enet_reg_tab[] = {
0x0000, 0x0004, 0x0008, 0x000C, 0x0010, 0x0014, 0x0018, 0x001C, 0x0028, 0x002C, 0x0034,
0x0038, 0x003C, 0x0040, 0x0044, 0x0048, 0x004C, 0x0050, 0x0054, 0x0058, 0x005C, 0x1080,
0x0100, 0x0104, 0x0108, 0x010C, 0x0110, 0x014C, 0x0150, 0x0168, 0x0194, 0x0198, 0x01C4,
0x0700, 0x0704,0x0708, 0x070C, 0x0710, 0x0714, 0x0718, 0x071C, 0x0720, 0x0728, 0x072C,
0x1000, 0x1004, 0x1008, 0x100C, 0x1010, 0x1014, 0x1018, 0x101C, 0x1020, 0x1024, 0x1048,
0x104C, 0x1050, 0x1054};
/* initialize ENET peripheral with generally concerned parameters, call it by enet_init() */
static void enet_default_init(void);
#ifndef USE_DELAY
/* insert a delay time */
static void enet_delay(uint32_t ncount);
#endif /* USE_DELAY */
/*!
\brief deinitialize the ENET, and reset structure parameters for ENET initialization
\param[in] none
\param[out] none
\retval none
*/
void enet_deinit(void)
{
rcu_periph_reset_enable(RCU_ENETRST);
rcu_periph_reset_disable(RCU_ENETRST);
enet_initpara_reset();
}
/*!
\brief configure the parameters which are usually less cared for initialization
note -- this function must be called before enet_init(), otherwise
configuration will be no effect
\param[in] option: different function option, which is related to several parameters,
only one parameter can be selected which is shown as below, refer to enet_option_enum
\arg FORWARD_OPTION: choose to configure the frame forward related parameters
\arg DMABUS_OPTION: choose to configure the DMA bus mode related parameters
\arg DMA_MAXBURST_OPTION: choose to configure the DMA max burst related parameters
\arg DMA_ARBITRATION_OPTION: choose to configure the DMA arbitration related parameters
\arg STORE_OPTION: choose to configure the store forward mode related parameters
\arg DMA_OPTION: choose to configure the DMA descriptor related parameters
\arg VLAN_OPTION: choose to configure vlan related parameters
\arg FLOWCTL_OPTION: choose to configure flow control related parameters
\arg HASHH_OPTION: choose to configure hash high
\arg HASHL_OPTION: choose to configure hash low
\arg FILTER_OPTION: choose to configure frame filter related parameters
\arg HALFDUPLEX_OPTION: choose to configure halfduplex mode related parameters
\arg TIMER_OPTION: choose to configure time counter related parameters
\arg INTERFRAMEGAP_OPTION: choose to configure the inter frame gap related parameters
\param[in] para: the related parameters according to the option
all the related parameters should be configured which are shown as below
FORWARD_OPTION related parameters:
- ENET_AUTO_PADCRC_DROP_ENABLE/ ENET_AUTO_PADCRC_DROP_DISABLE ;
- ENET_TYPEFRAME_CRC_DROP_ENABLE/ ENET_TYPEFRAME_CRC_DROP_DISABLE ;
- ENET_FORWARD_ERRFRAMES_ENABLE/ ENET_FORWARD_ERRFRAMES_DISABLE ;
- ENET_FORWARD_UNDERSZ_GOODFRAMES_ENABLE/ ENET_FORWARD_UNDERSZ_GOODFRAMES_DISABLE .
DMABUS_OPTION related parameters:
- ENET_ADDRESS_ALIGN_ENABLE/ ENET_ADDRESS_ALIGN_DISABLE ;
- ENET_FIXED_BURST_ENABLE/ ENET_FIXED_BURST_DISABLE ;
- ENET_MIXED_BURST_ENABLE/ ENET_MIXED_BURST_DISABLE ;
DMA_MAXBURST_OPTION related parameters:
- ENET_RXDP_1BEAT/ ENET_RXDP_2BEAT/ ENET_RXDP_4BEAT/
ENET_RXDP_8BEAT/ ENET_RXDP_16BEAT/ ENET_RXDP_32BEAT/
ENET_RXDP_4xPGBL_4BEAT/ ENET_RXDP_4xPGBL_8BEAT/
ENET_RXDP_4xPGBL_16BEAT/ ENET_RXDP_4xPGBL_32BEAT/
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
GD32470Z-LCKFB梁山派是立创开发板推出的一款GD32F470系列的开发板,最高主频高达240M,该开发板具有丰富的板载资源,是基于GD32F470ZGT6的全国产化开源开发板,GD32F470ZGT6,主频 240MHz,CPU内核:ARM Cortex-M4,1024KB FLASH ,512KB RAM ,本章节是为需要在 RT-Thread 操作系统上使用更多开发板资源的开发者准备的。通过使用 ENV 工具对 BSP 进行配置,可以开启更多板载资源,实现更多高级功能。本 BSP 为开发者提供 MDK5工程,支持 GCC 开发环境,也可使用RT-Thread Studio开发。下面以 MDK5 开发环境为例,介绍如何将系统运行起来。
资源推荐
资源详情
资源评论
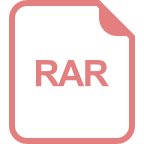
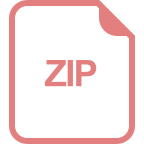
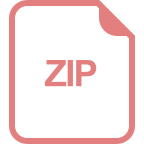
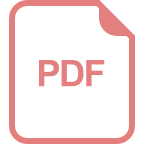
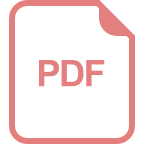
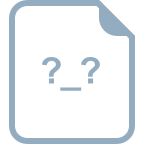
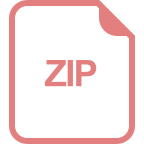
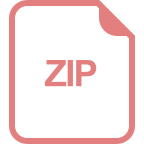
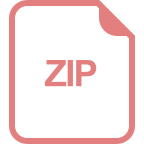
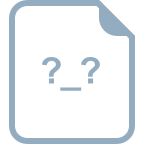
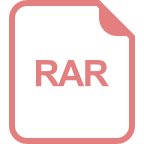
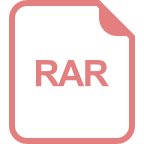
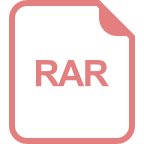
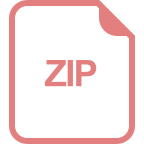
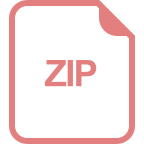
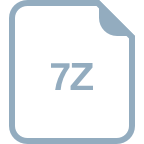
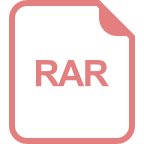
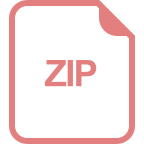
收起资源包目录

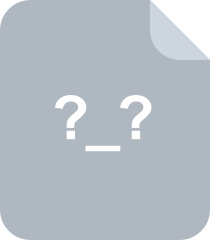
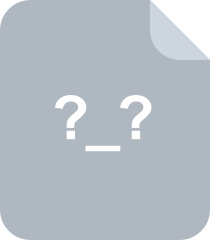
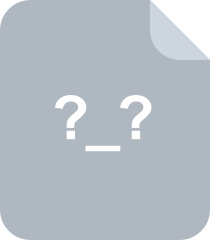
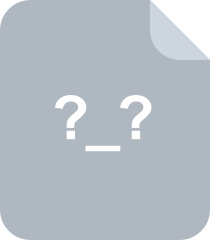
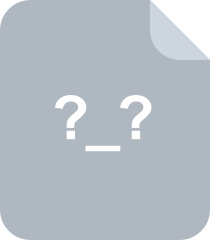
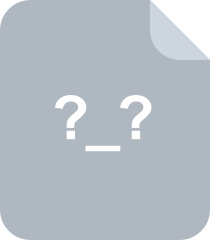
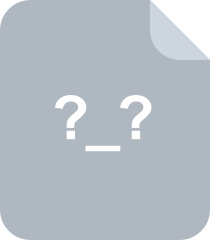
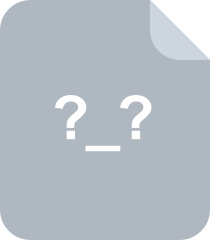
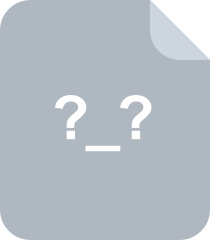
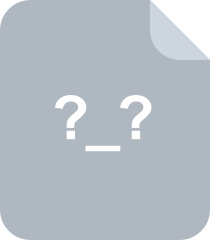
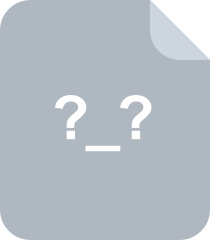
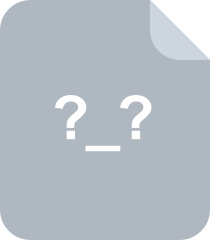
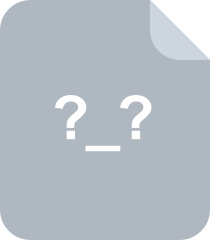
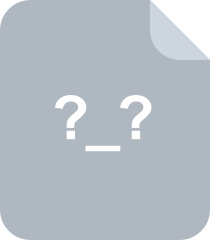
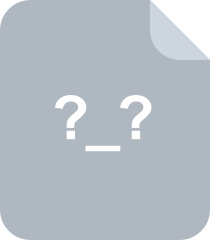
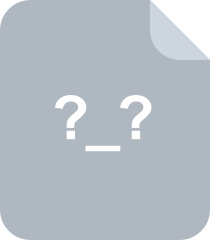
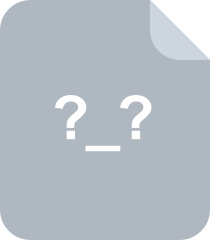
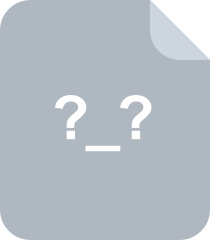
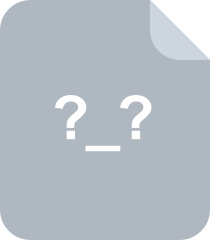
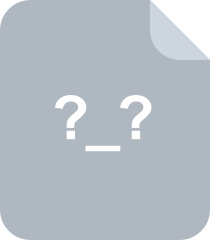
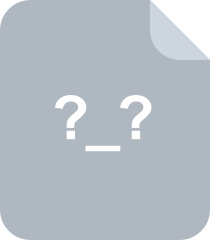
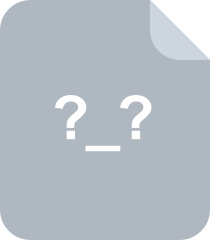
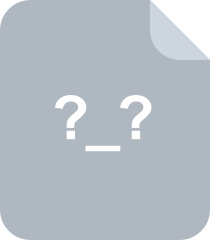
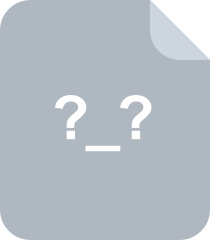
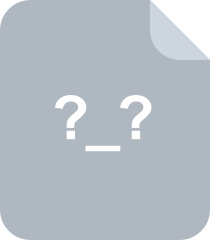
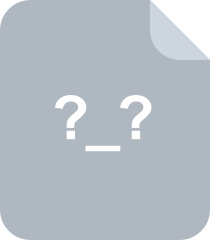
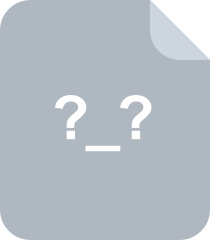
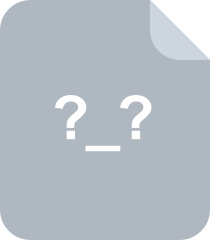
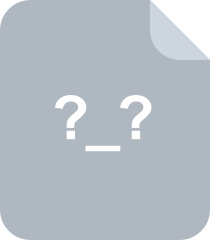
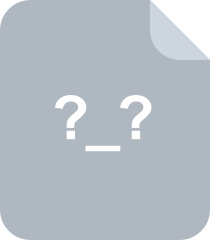
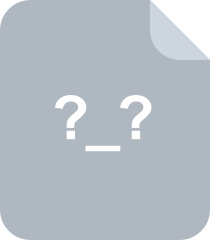
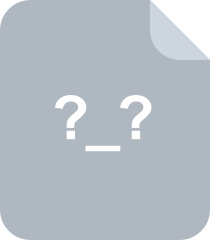
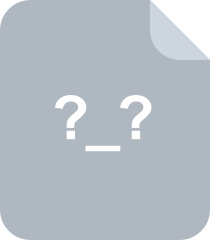
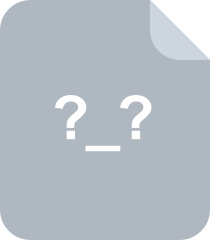
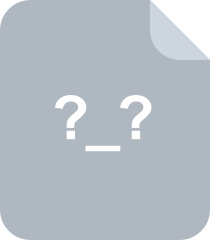
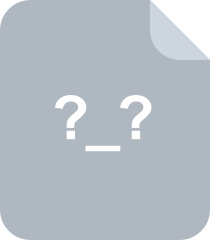
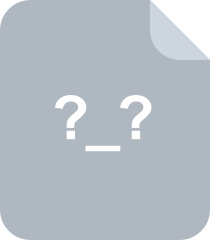
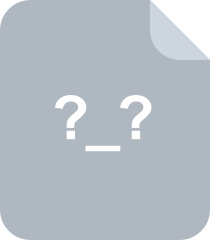
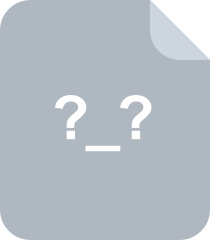
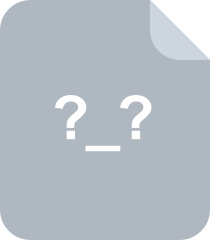
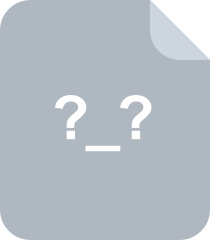
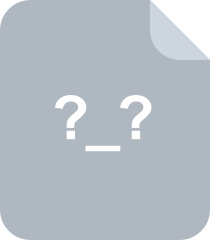
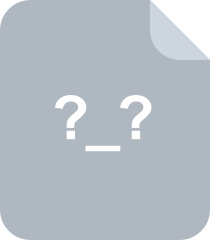
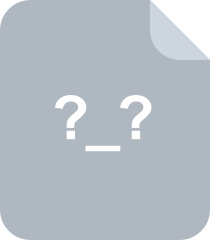
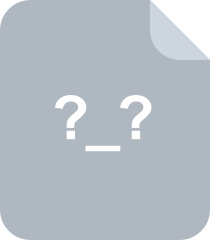
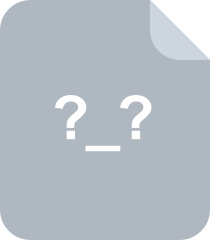
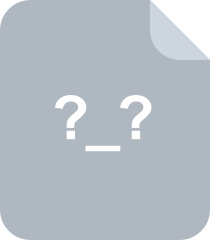
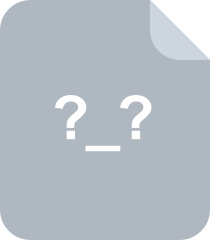
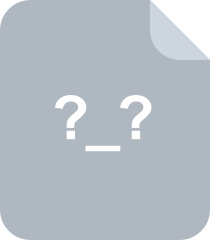
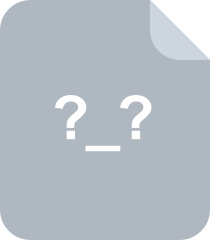
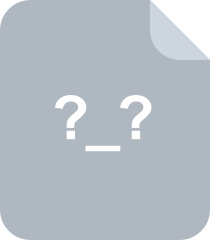
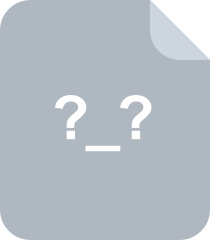
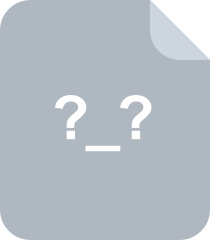
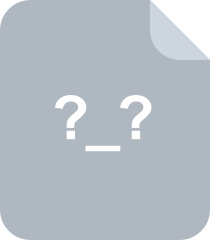
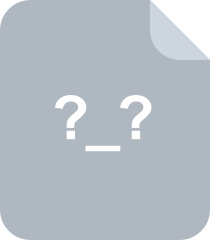
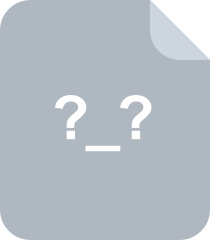
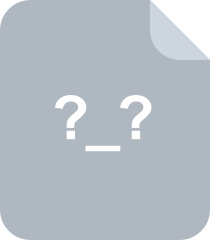
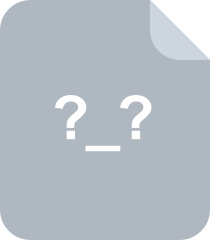
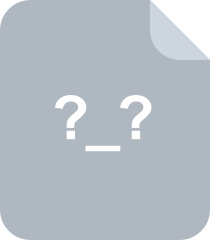
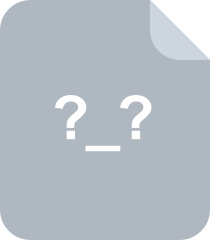
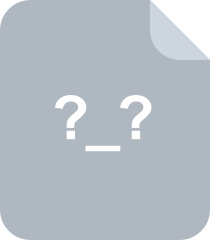
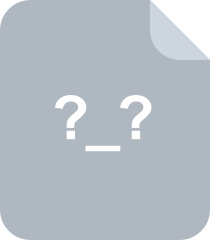
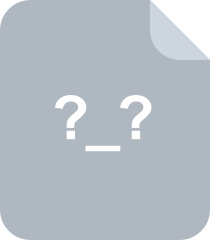
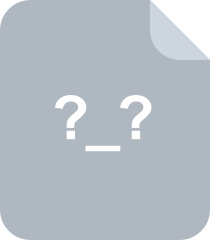
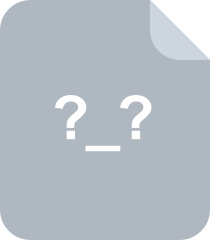
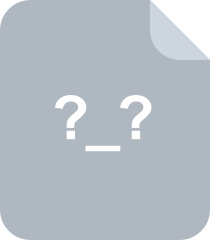
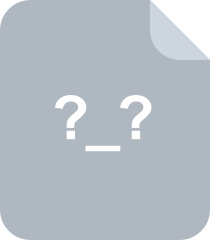
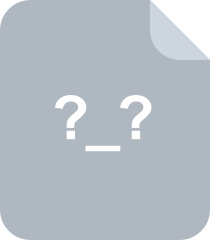
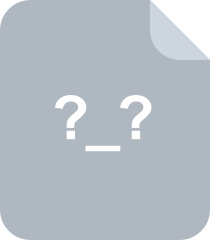
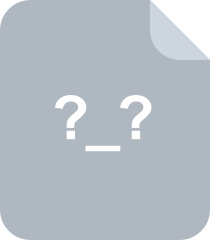
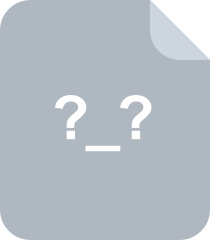
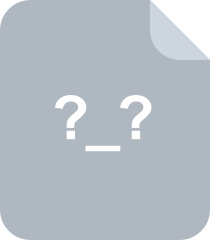
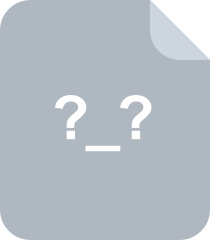
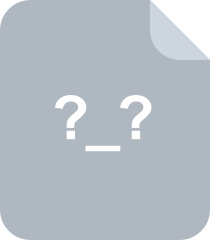
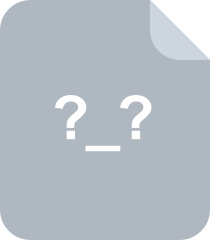
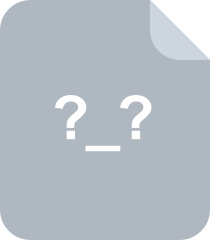
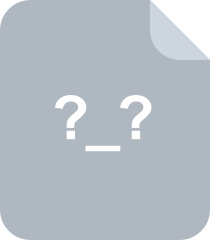
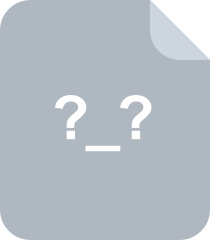
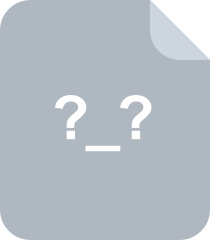
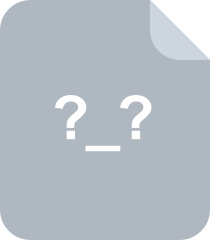
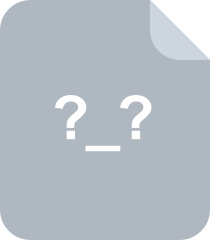
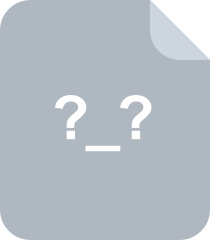
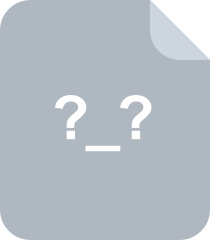
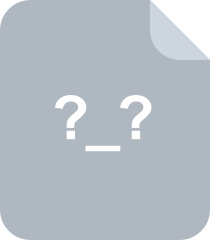
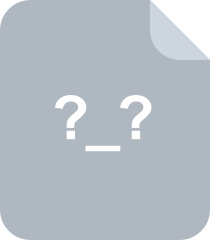
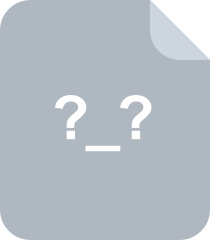
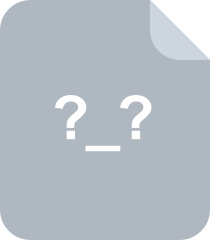
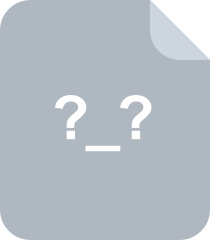
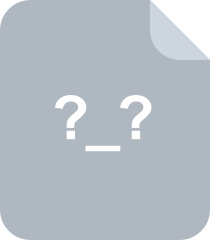
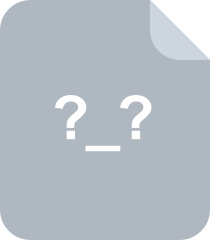
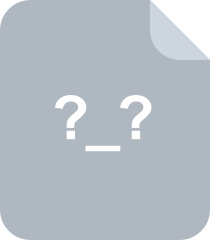
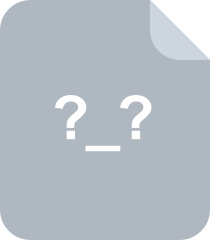
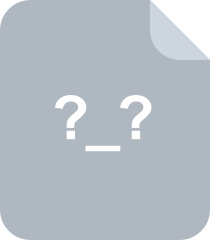
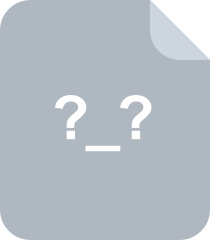
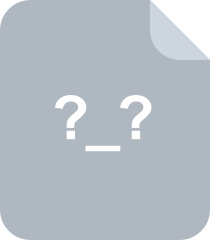
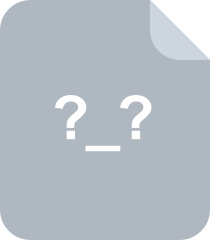
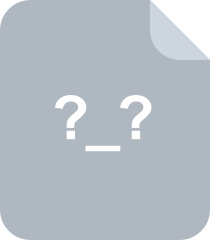
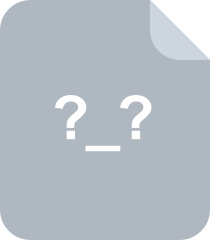
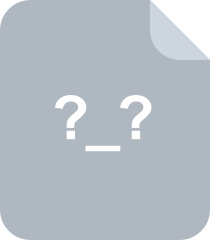
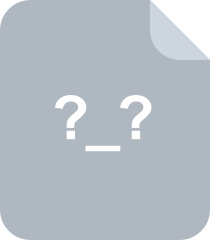
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
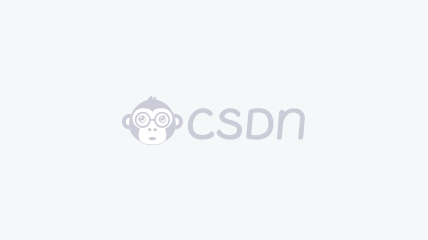


华为奋斗者精神
- 粉丝: 1w+
- 资源: 241
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

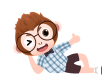
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


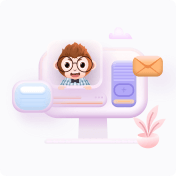
安全验证
文档复制为VIP权益,开通VIP直接复制
