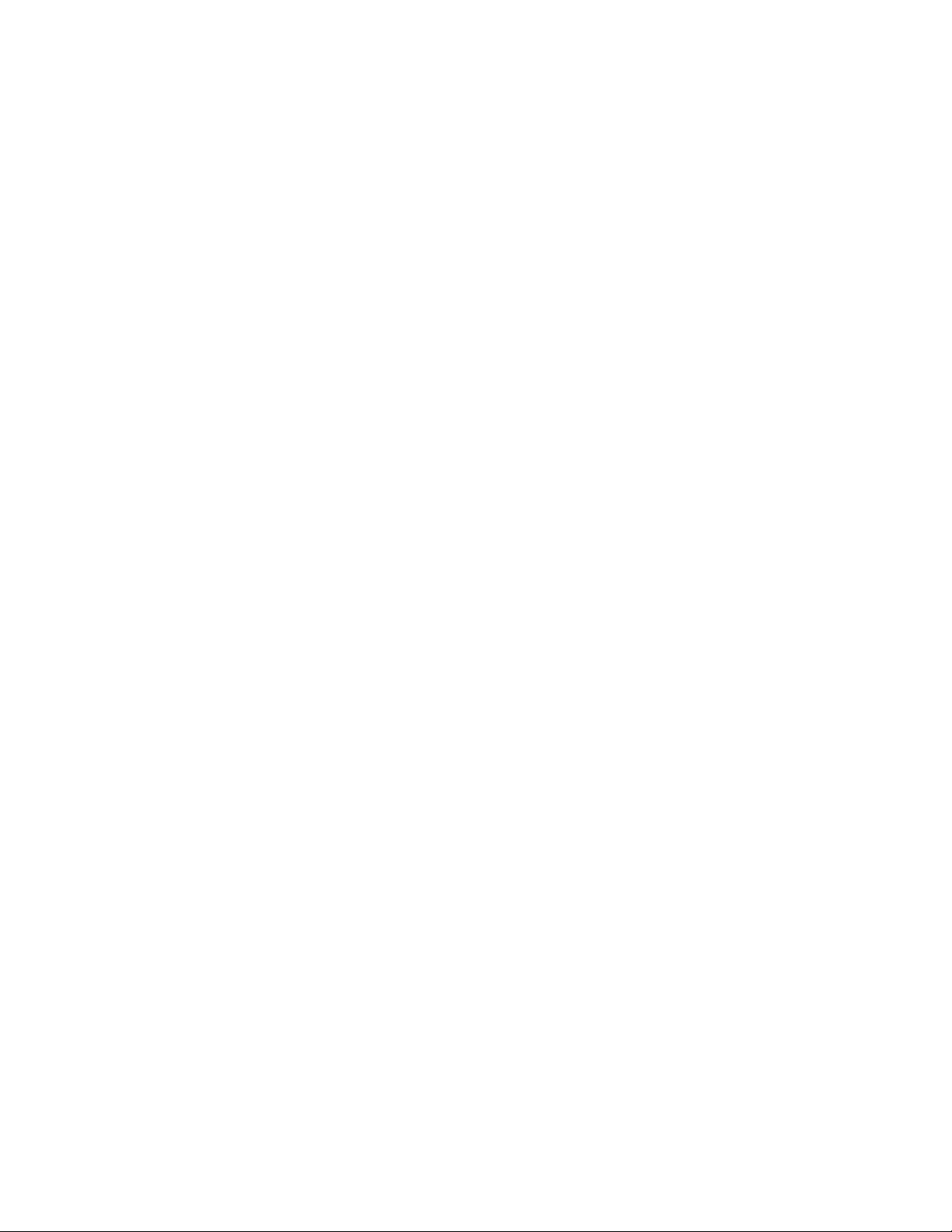
The OpenGL Utility Toolkit (GLUT)
Programming Interface
API Version 3
Mark J. Kilgard
Silicon Graphics, Inc.
November 13, 1996
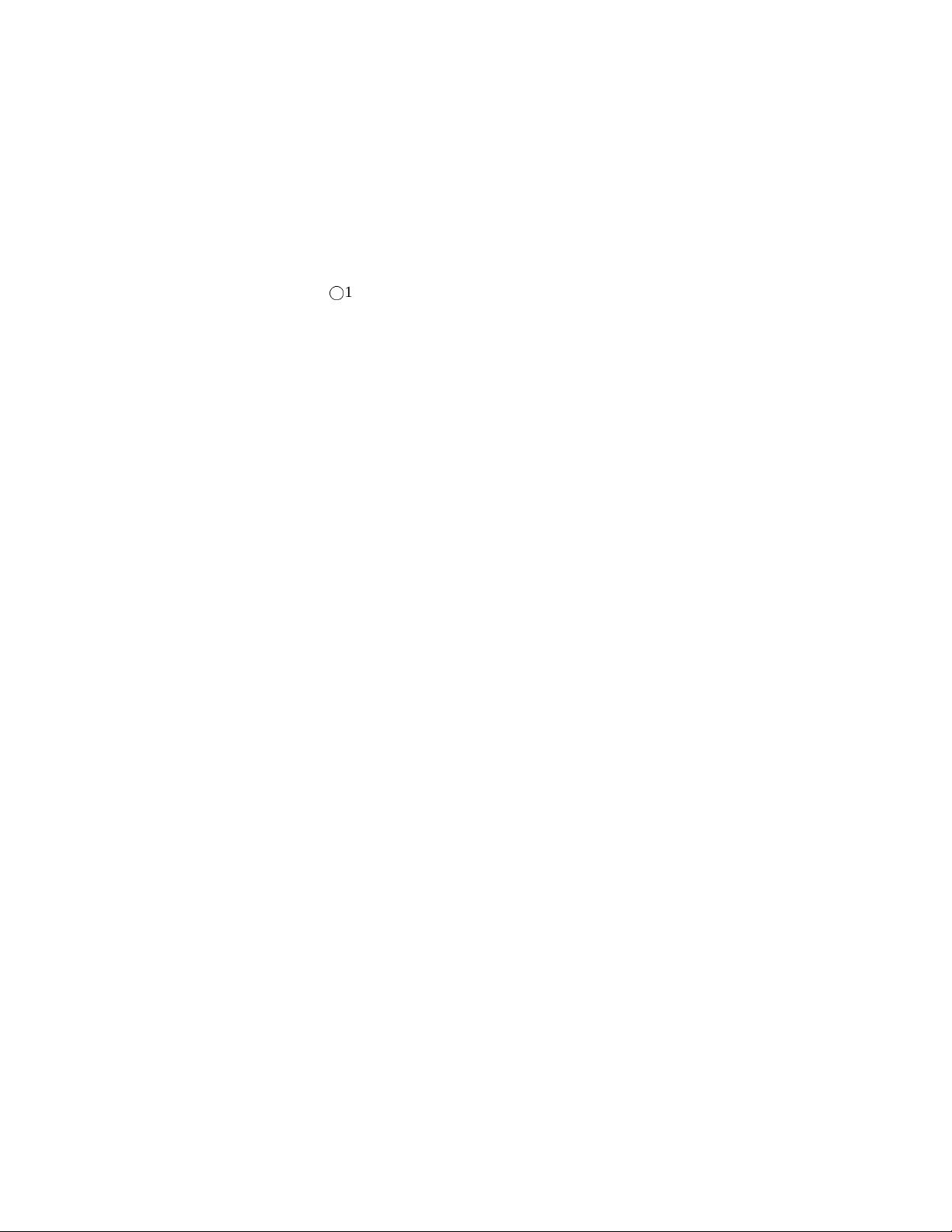
OpenGL is a trademark of Silicon Graphics, Inc. X Window System is a trademark of X Consortium, Inc.
Spaceball is a registered trademark of Spatial Systems Inc.
The author has taken care in preparation of this documentation but makes no expressed or implied warranty
of any kind and assumes no responsibility for errors or omissions. No liability is assumed for incidental or
consequential damages in connection with or arising from the use of information or programs contained herein.
Copyright
c
1994, 1995, 1996. Mark J. Kilgard. All rights reserved.
All rights reserved. No part of this documentation may be reproduced, in any form or by any means, without
permission in writing from the author.
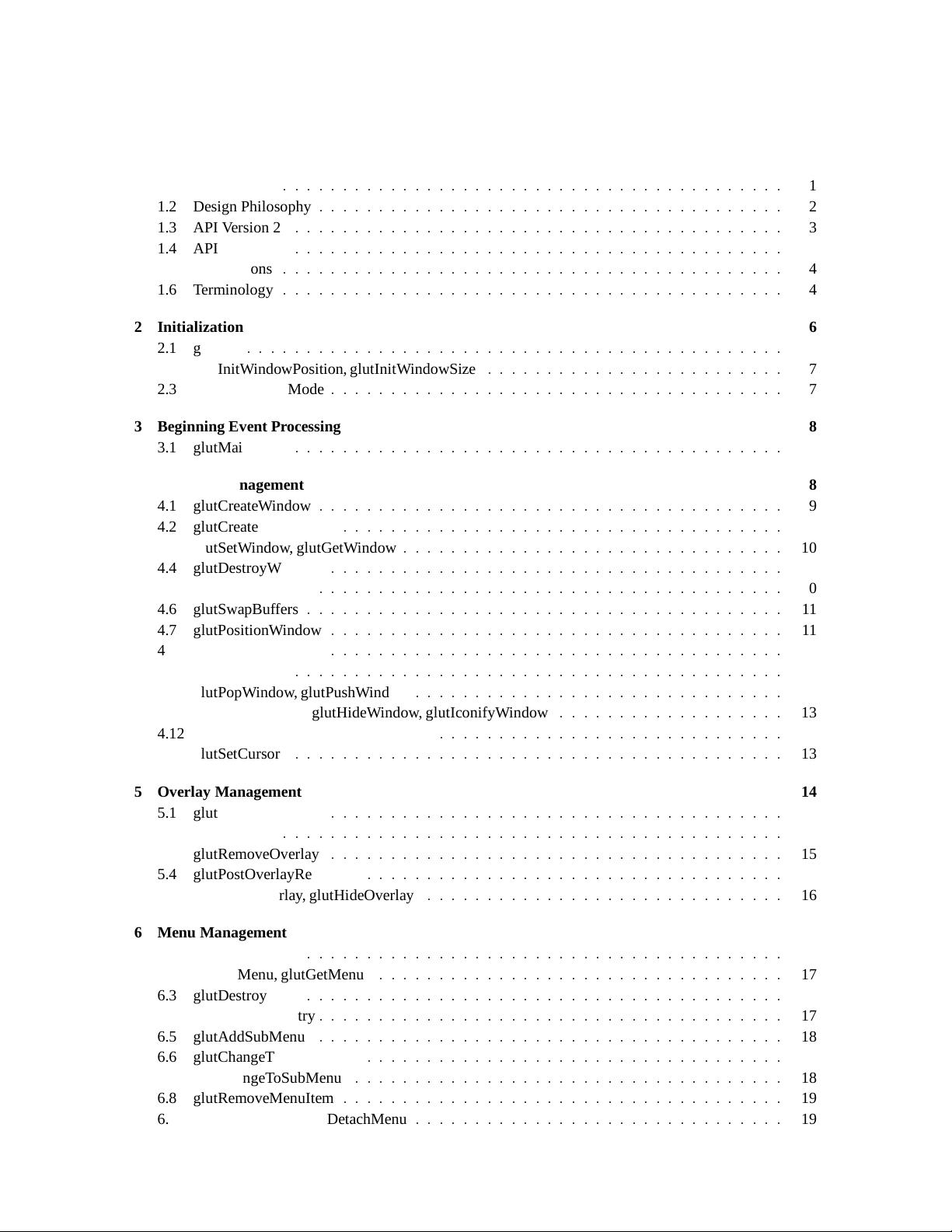
CONTENTS
i
Contents
1 Introduction 1
1.1 Background
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
1
1.2 Design Philosophy
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
2
1.3 API Version 2
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
3
1.4 API Version 3
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
3
1.5 Conventions
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
4
1.6 Terminology
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
4
2 Initialization 6
2.1 glutInit
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :::: ::: :
6
2.2 glutInitWindowPosition, glutInitWindowSize
::::::: ::: :::: ::: :::: ::: :
7
2.3 glutInitDisplayMode
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
7
3 Beginning Event Processing 8
3.1 glutMainLoop
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
8
4 Window Management 8
4.1 glutCreateWindow
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
9
4.2 glutCreateSubWindow
::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
9
4.3 glutSetWindow, glutGetWindow
::::::: ::: :::: ::: :::: ::: :::: ::::
10
4.4 glutDestroyWindow
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
10
4.5 glutPostRedisplay
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
10
4.6 glutSwapBuffers
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
11
4.7 glutPositionWindow
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
11
4.8 glutReshapeWindow
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
11
4.9 glutFullScreen
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
12
4.10 glutPopWindow, glutPushWindow
:::::: ::: :::: ::: :::: ::: :::: ::::
12
4.11 glutShowWindow, glutHideWindow, glutIconifyWindow
:::: :::: ::: :::: ::: :
13
4.12 glutSetWindowTitle, glutSetIconTitle
::::::: :::: ::: :::: ::: :::: ::: :
13
4.13 glutSetCursor
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
13
5 Overlay Management 14
5.1 glutEstablishOverlay
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
14
5.2 glutUseLayer
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
15
5.3 glutRemoveOverlay
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
15
5.4 glutPostOverlayRedisplay
:::::: :::: ::: :::: ::: :::: ::: ::::::: :
16
5.5 glutShowOverlay, glutHideOverlay
::::: ::: :::: ::: :::: ::: :::: ::::
16
6 Menu Management 16
6.1 glutCreateMenu
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
16
6.2 glutSetMenu, glutGetMenu
::::: :::: ::: :::: ::: :::: ::: ::::::: :
17
6.3 glutDestroyMenu
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
17
6.4 glutAddMenuEntry
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
17
6.5 glutAddSubMenu
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
18
6.6 glutChangeToMenuEntry
:::::: :::: ::: :::: ::: :::: ::: ::::::: :
18
6.7 glutChangeToSubMenu
::::::: :::: ::: :::: ::: :::: ::: ::::::: :
18
6.8 glutRemoveMenuItem
::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
19
6.9 glutAttachMenu, glutDetachMenu
:::::: ::: :::: ::: :::: ::: :::: ::::
19
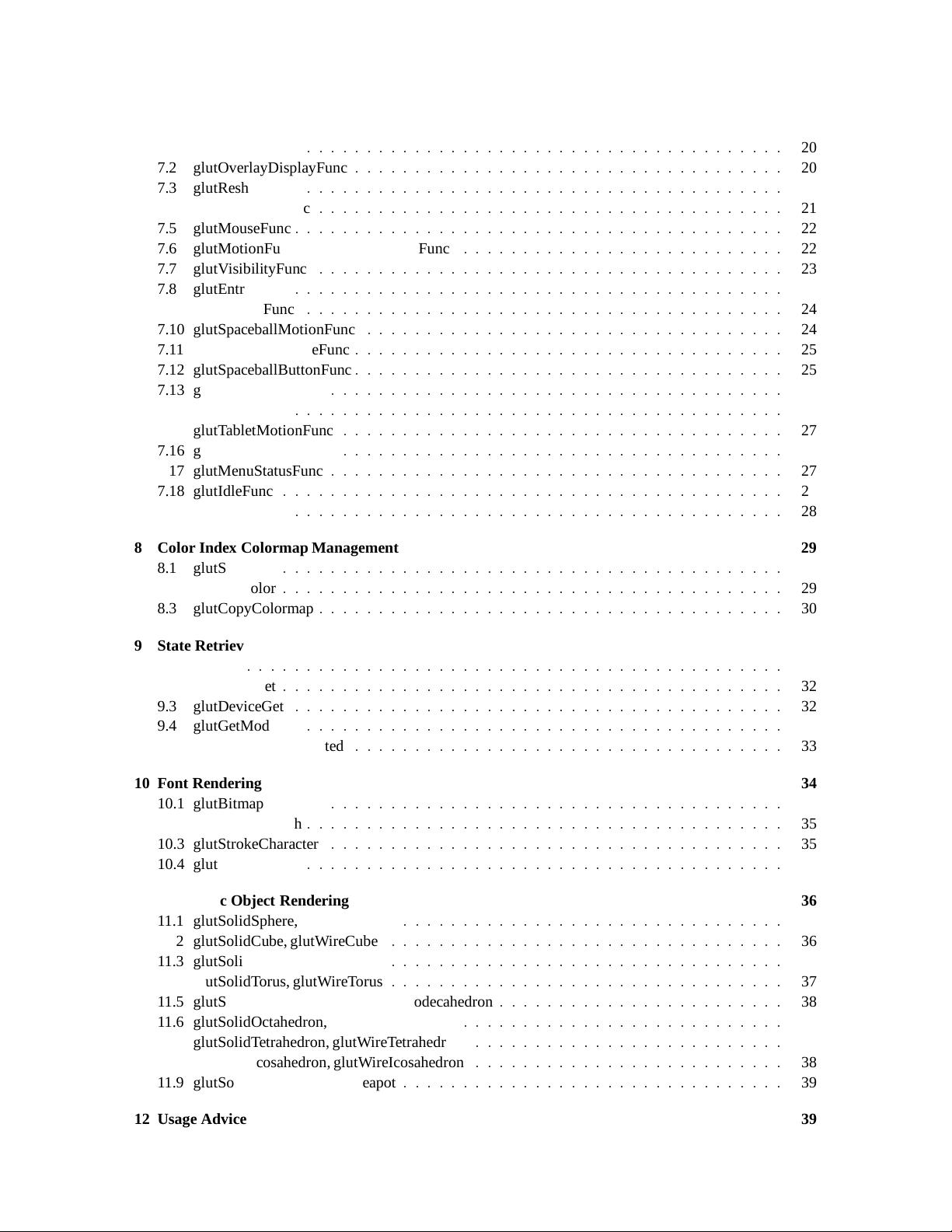
ii
CONTENTS
7 Callback Registration 19
7.1 glutDisplayFunc
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
20
7.2 glutOverlayDisplayFunc
::::::: :::: ::: :::: ::: :::: ::: ::::::: :
20
7.3 glutReshapeFunc
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
21
7.4 glutKeyboardFunc
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
21
7.5 glutMouseFunc
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
22
7.6 glutMotionFunc, glutPassiveMotionFunc
::::: :::: ::: :::: ::: :::: ::: :
22
7.7 glutVisibilityFunc
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
23
7.8 glutEntryFunc
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
23
7.9 glutSpecialFunc
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
24
7.10 glutSpaceballMotionFunc
:::::: :::: ::: :::: ::: :::: ::: ::::::: :
24
7.11 glutSpaceballRotateFunc
::::::: :::: ::: :::: ::: :::: ::: ::::::: :
25
7.12 glutSpaceballButtonFunc
::::::: :::: ::: :::: ::: :::: ::: ::::::: :
25
7.13 glutButtonBoxFunc
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
26
7.14 glutDialsFunc
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
26
7.15 glutTabletMotionFunc
::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
27
7.16 glutTabletButtonFunc
::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
27
7.17 glutMenuStatusFunc
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
27
7.18 glutIdleFunc
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
28
7.19 glutTimerFunc
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
28
8 Color Index Colormap Management 29
8.1 glutSetColor
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
29
8.2 glutGetColor
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
29
8.3 glutCopyColormap
::::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
30
9 State Retrieval 30
9.1 glutGet
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :::: ::: :
30
9.2 glutLayerGet
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
32
9.3 glutDeviceGet
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
32
9.4 glutGetModifiers
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
33
9.5 glutExtensionSupported
::::::: :::: ::: :::: ::: :::: ::: ::::::: :
33
10 Font Rendering 34
10.1 glutBitmapCharacter
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
34
10.2 glutBitmapWidth
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
35
10.3 glutStrokeCharacter
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
35
10.4 glutStrokeWidth
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
36
11 Geometric Object Rendering 36
11.1 glutSolidSphere,glutWireSphere
::::::: ::: :::: ::: :::: ::: :::: ::::
36
11.2 glutSolidCube, glutWireCube
:::: :::: ::: :::: ::: :::: ::: ::::::: :
36
11.3 glutSolidCone, glutWireCone
:::: :::: ::: :::: ::: :::: ::: ::::::: :
37
11.4 glutSolidTorus, glutWireTorus
:::: :::: ::: :::: ::: :::: ::: ::::::: :
37
11.5 glutSolidDodecahedron, glutWireDodecahedron
:::::: ::: :::: ::: :::: ::: :
38
11.6 glutSolidOctahedron,glutWireOctahedron
::::: :::: ::: :::: ::: :::: ::: :
38
11.7 glutSolidTetrahedron,glutWireTetrahedron
:::: :::: ::: :::: ::: :::: ::: :
38
11.8 glutSolidIcosahedron, glutWireIcosahedron
:::: :::: ::: :::: ::: :::: ::: :
38
11.9 glutSolidTeapot, glutWireTeapot
::::::: ::: :::: ::: :::: ::: :::: ::::
39
12 Usage Advice 39
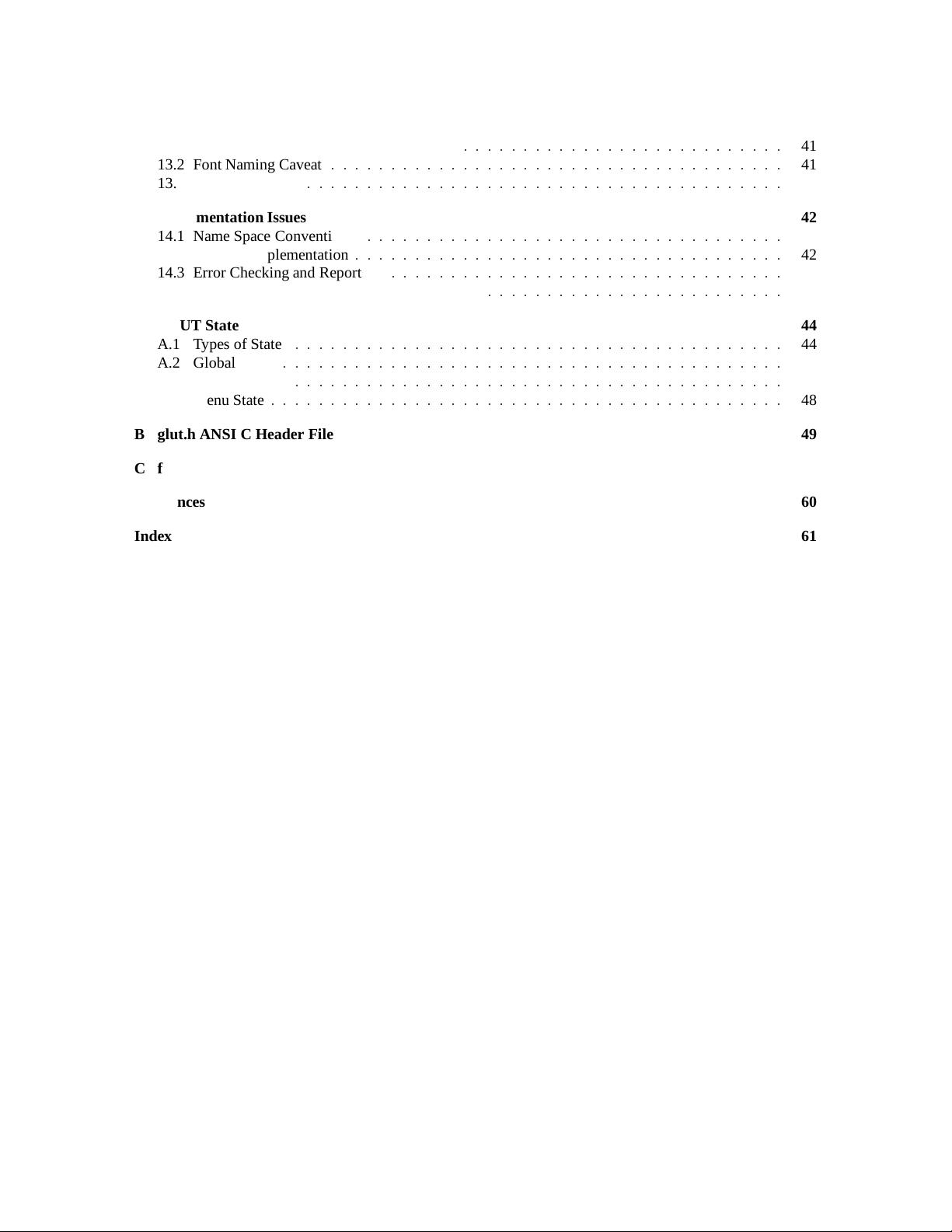
CONTENTS
iii
13 FORTRAN Binding 41
13.1 Names for the FORTRAN GLUT Binding
::::: :::: ::: :::: ::: :::: ::: :
41
13.2 Font Naming Caveat
:::::: ::: :::: ::: :::: ::: :::: ::::::: ::: :
41
13.3 NULL Callback
:::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
42
14 Implementation Issues 42
14.1 Name Space Conventions
:::::: :::: ::: :::: ::: :::: ::: ::::::: :
42
14.2 Modular Implementation
::::::: :::: ::: :::: ::: :::: ::: ::::::: :
42
14.3 Error Checking and Reporting
:::: :::: ::: :::: ::: :::: ::: ::::::: :
42
14.4 Avoid Unspecified GLUT Usage Restrictions
::::::: ::: :::: ::: :::: ::: :
42
A GLUT State 44
A.1 Types of State
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
44
A.2 Global State
:::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
44
A.3 Window State
::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
45
A.4 Menu State
::::::: :::: ::: :::: ::: :::: ::: ::::::: :::: ::: :
48
B glut.h ANSI C Header File 49
C fglut.h FORTRAN Header File 55
References 60
Index 61
评论0
最新资源