package com.wyf.controller;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.servlet.ModelAndView;
import com.wyf.model.User;
import com.wyf.service.impl.UserOperationsServiceImpl;
@Controller
@RequestMapping(value = "/redis")
public class UserController {
@Autowired
private UserOperationsServiceImpl userOperationsService;
private User user;
@RequestMapping(value = "/addUser", method = RequestMethod.POST)
public ModelAndView addUser(
@RequestParam(value = "Id", required = true) String Id,
@RequestParam(value = "name", required = true) String name,
@RequestParam(value = "password", required = true) String password) {
user = new User(Id, name, password);
userOperationsService.add(user);
List<User> lists=userOperationsService.getUsers();
Map<String, Object>mapList=new HashMap<String, Object>();
mapList.put("userList", lists);
return new ModelAndView("/WEB-INF/jsp/userList.jsp","user",mapList);
}
@RequestMapping(value = "/addUser", method = RequestMethod.GET)
public String addUser() {
return "/WEB-INF/jsp/AddUser.jsp";
}
@RequestMapping(value = "/queryUser")
public String queryUser() {
return "/WEB-INF/jsp/getUser.jsp";
}
@RequestMapping(value = "/getUser", method = RequestMethod.POST)
@ResponseBody
public Map<String, Object> getUser(
@RequestParam(value = "key", required = true) String key,Model model) {
user = userOperationsService.getUser(key);
Map<String, Object> maps=new HashMap<String, Object>();
maps.put("uid", user.getId());
maps.put("uname", user.getName());
maps.put("upwd", user.getPassword());
return maps;
}
@RequestMapping(value = "/getUsers")
public ModelAndView getUsers() {
List<User> lists=userOperationsService.getUsers();
Map<String, Object>mapList=new HashMap<String, Object>();
mapList.put("userList", lists);
return new ModelAndView("/WEB-INF/jsp/userList.jsp","user",mapList);
}
@RequestMapping(value = "/updateUser", method = RequestMethod.POST)
public ModelAndView updateUser(
@RequestParam(value = "Id", required = true) String Id,
@RequestParam(value = "name", required = true) String name,
@RequestParam(value = "password", required = true) String password) {
user = new User(Id, name, password);
userOperationsService.add(user);
List<User> lists=userOperationsService.getUsers();
Map<String, Object>mapList=new HashMap<String, Object>();
mapList.put("userList", lists);
return new ModelAndView("/WEB-INF/jsp/userList.jsp","user",mapList);
}
@RequestMapping(value = "/delUser",method = RequestMethod.GET)
public ModelAndView delUser(
@RequestParam(value = "uid", required = true) String uid) {
userOperationsService.deldate(uid);
List<User> lists=userOperationsService.getUsers();
Map<String, Object>mapList=new HashMap<String, Object>();
mapList.put("userList", lists);
return new ModelAndView("/WEB-INF/jsp/userList.jsp","user",mapList);
}
}
没有合适的资源?快使用搜索试试~ 我知道了~
在springMVC中java操作redis的封装的增删改查demo
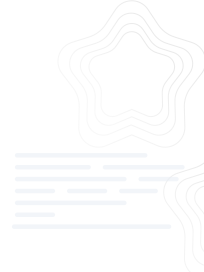
共53个文件
xml:11个
class:7个
java:7个

需积分: 10 11 下载量 106 浏览量
2018-11-07
15:11:03
上传
评论
收藏 1.31MB RAR 举报
温馨提示
在springMVC中java操作redis的封装的增删改查demo --------------
资源推荐
资源详情
资源评论
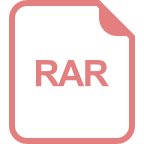
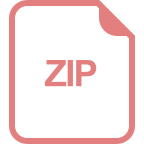
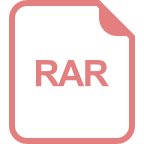
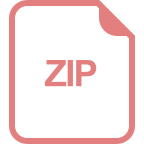
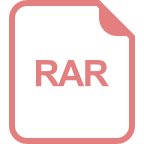
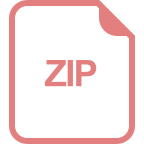
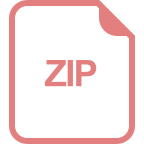
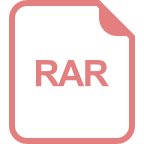
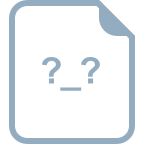
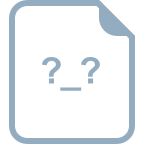
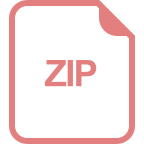
收起资源包目录



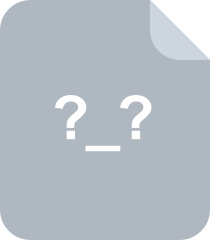
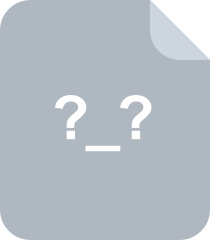






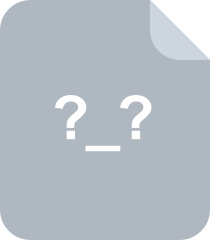
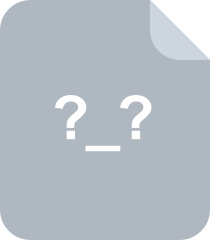




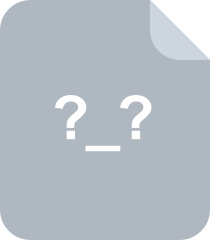
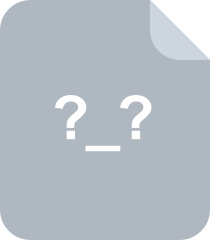
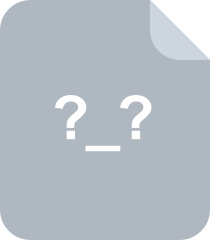


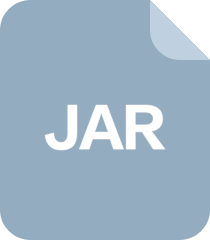
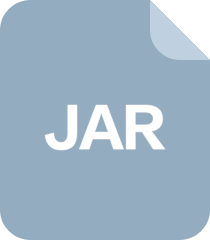
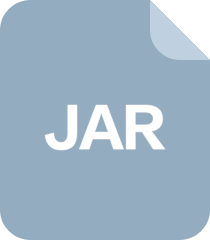

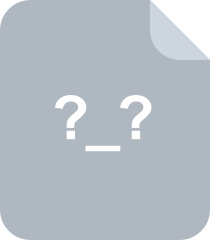
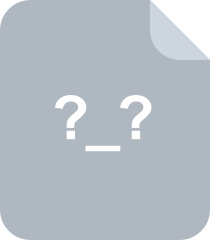
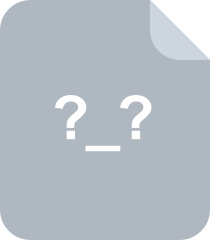

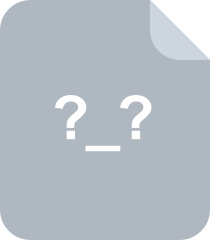
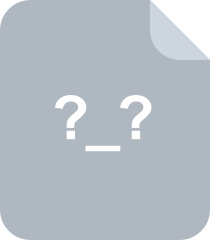

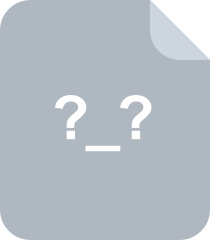
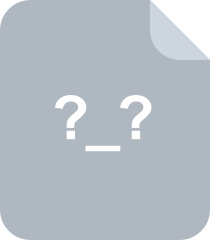

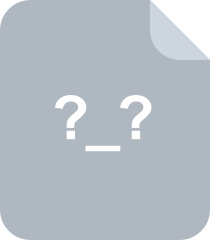


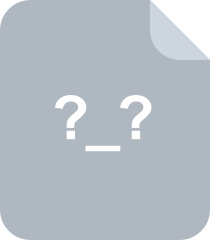
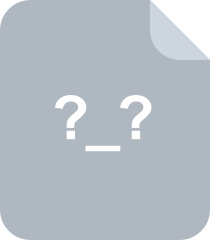

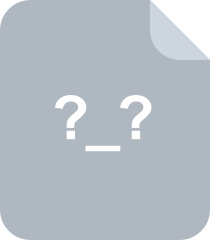




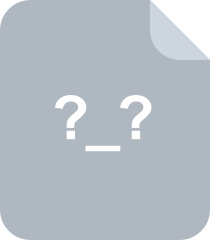

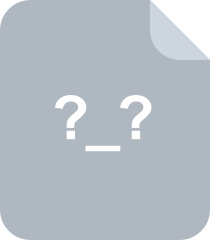
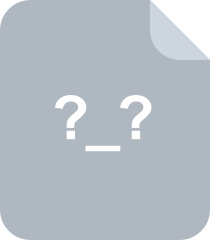

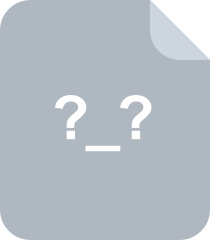

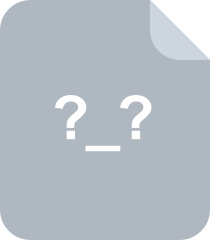




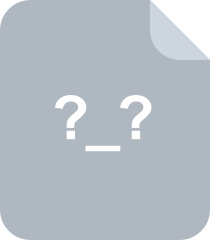



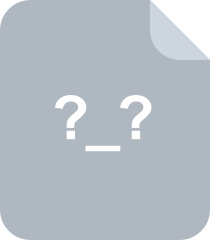
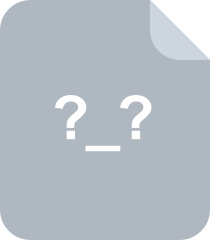



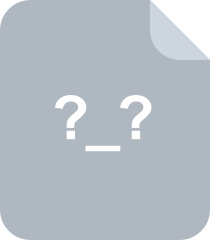



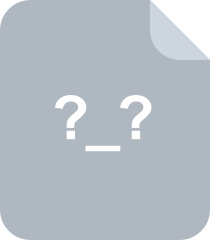
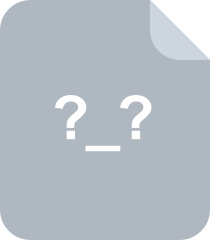




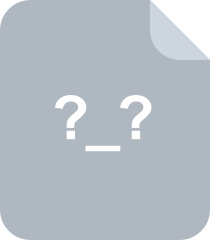

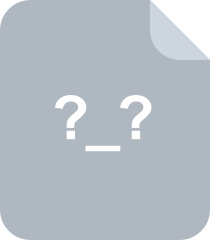
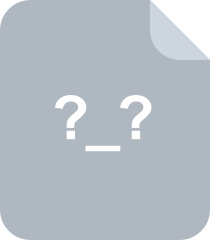

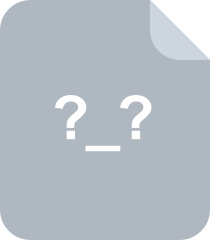

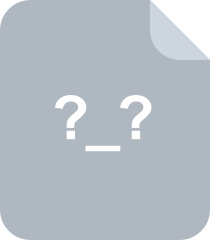

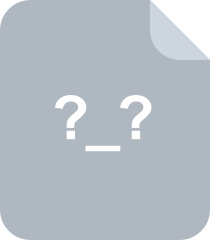
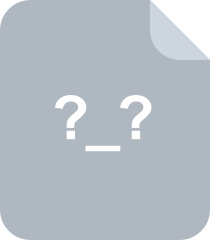

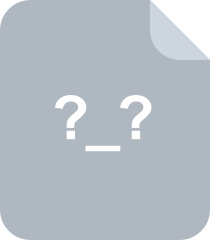
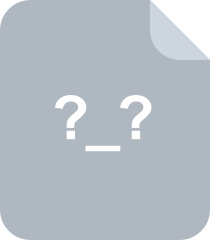

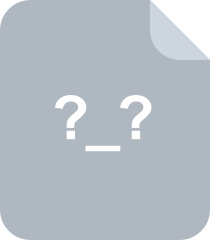



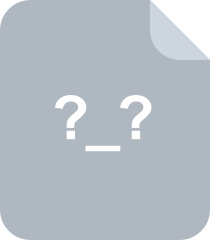
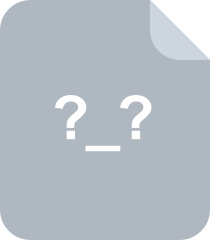
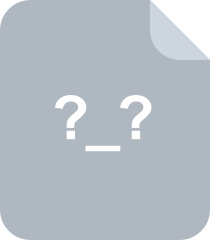
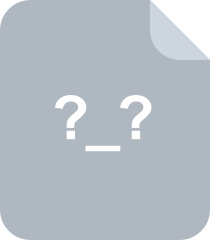
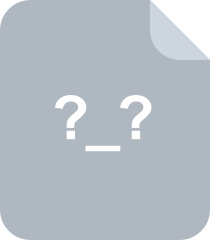
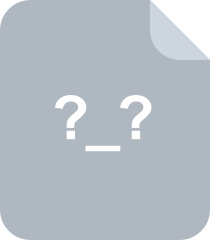
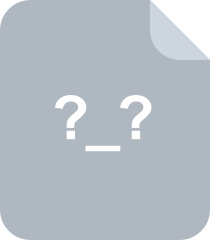
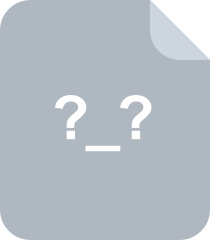
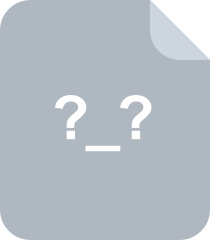
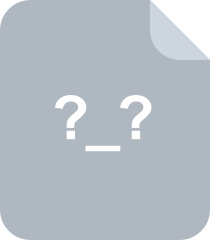
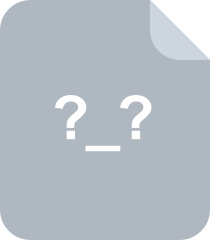
共 53 条
- 1
资源评论
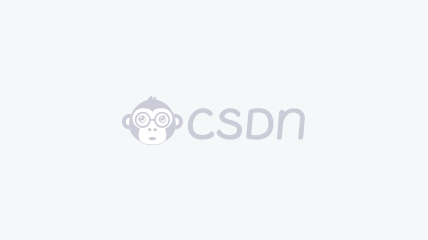

juone929
- 粉丝: 8
- 资源: 74
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

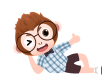
安全验证
文档复制为VIP权益,开通VIP直接复制
