Jayway JsonPath
=====================
**A Java DSL for reading JSON documents.**
[](https://travis-ci.org/json-path/JsonPath)
[](https://maven-badges.herokuapp.com/maven-central/com.jayway.jsonpath/json-path)
[](http://www.javadoc.io/doc/com.jayway.jsonpath/json-path)
Jayway JsonPath is a Java port of [Stefan Goessner JsonPath implementation](http://goessner.net/articles/JsonPath/).
Getting Started
---------------
JsonPath is available at the Central Maven Repository. Maven users add this to your POM.
```xml
<dependency>
<groupId>com.jayway.jsonpath</groupId>
<artifactId>json-path</artifactId>
<version>2.9.0</version>
</dependency>
```
If you need help ask questions at [Stack Overflow](http://stackoverflow.com/questions/tagged/jsonpath). Tag the question 'jsonpath' and 'java'.
JsonPath expressions always refer to a JSON structure in the same way as XPath expression are used in combination
with an XML document. The "root member object" in JsonPath is always referred to as `$` regardless if it is an
object or array.
JsonPath expressions can use the dot–notation
`$.store.book[0].title`
or the bracket–notation
`$['store']['book'][0]['title']`
Operators
---------
| Operator | Description |
| :------------------------ | :----------------------------------------------------------------- |
| `$` | The root element to query. This starts all path expressions. |
| `@` | The current node being processed by a filter predicate. |
| `*` | Wildcard. Available anywhere a name or numeric are required. |
| `..` | Deep scan. Available anywhere a name is required. |
| `.<name>` | Dot-notated child |
| `['<name>' (, '<name>')]` | Bracket-notated child or children |
| `[<number> (, <number>)]` | Array index or indexes |
| `[start:end]` | Array slice operator |
| `[?(<expression>)]` | Filter expression. Expression must evaluate to a boolean value. |
Functions
---------
Functions can be invoked at the tail end of a path - the input to a function is the output of the path expression.
The function output is dictated by the function itself.
| Function | Description | Output type |
|:------------|:-------------------------------------------------------------------------------------|:---------------------|
| `min()` | Provides the min value of an array of numbers | Double |
| `max()` | Provides the max value of an array of numbers | Double |
| `avg()` | Provides the average value of an array of numbers | Double |
| `stddev()` | Provides the standard deviation value of an array of numbers | Double |
| `length()` | Provides the length of an array | Integer |
| `sum()` | Provides the sum value of an array of numbers | Double |
| `keys()` | Provides the property keys (An alternative for terminal tilde `~`) | `Set<E>` |
| `concat(X)` | Provides a concatinated version of the path output with a new item | like input |
| `append(X)` | add an item to the json path output array | like input |
| `first()` | Provides the first item of an array | Depends on the array |
| `last()` | Provides the last item of an array | Depends on the array |
| `index(X)` | Provides the item of an array of index: X, if the X is negative, take from backwards | Depends on the array |
Filter Operators
-----------------
Filters are logical expressions used to filter arrays. A typical filter would be `[?(@.age > 18)]` where `@` represents the current item being processed. More complex filters can be created with logical operators `&&` and `||`. String literals must be enclosed by single or double quotes (`[?(@.color == 'blue')]` or `[?(@.color == "blue")]`).
| Operator | Description |
| :----------------------- | :-------------------------------------------------------------------- |
| `==` | left is equal to right (note that 1 is not equal to '1') |
| `!=` | left is not equal to right |
| `<` | left is less than right |
| `<=` | left is less or equal to right |
| `>` | left is greater than right |
| `>=` | left is greater than or equal to right |
| `=~` | left matches regular expression [?(@.name =~ /foo.*?/i)] |
| `in` | left exists in right [?(@.size in ['S', 'M'])] |
| `nin` | left does not exists in right |
| `subsetof` | left is a subset of right [?(@.sizes subsetof ['S', 'M', 'L'])] |
| `anyof` | left has an intersection with right [?(@.sizes anyof ['M', 'L'])] |
| `noneof` | left has no intersection with right [?(@.sizes noneof ['M', 'L'])] |
| `size` | size of left (array or string) should match right |
| `empty` | left (array or string) should be empty |
Path Examples
-------------
Given the json
```javascript
{
"store": {
"book": [
{
"category": "reference",
"author": "Nigel Rees",
"title": "Sayings of the Century",
"price": 8.95
},
{
"category": "fiction",
"author": "Evelyn Waugh",
"title": "Sword of Honour",
"price": 12.99
},
{
"category": "fiction",
"author": "Herman Melville",
"title": "Moby Dick",
"isbn": "0-553-21311-3",
"price": 8.99
},
{
"category": "fiction",
"author": "J. R. R. Tolkien",
"title": "The Lord of the Rings",
"isbn": "0-395-19395-8",
"price": 22.99
}
],
"bicycle": {
"color": "red",
"price": 19.95
}
},
"expensive": 10
}
```
| JsonPath | Result |
|:-------------------------------------------------------------------| :----- |
| `$.store.book[*].author` | The authors of all books |
| `$..author` | All authors |
| `$.store.*` | All things, both books and bicycles |
| `$.store..price` | The price of everything
没有合适的资源?快使用搜索试试~ 我知道了~
Java JsonPath 实现.zip
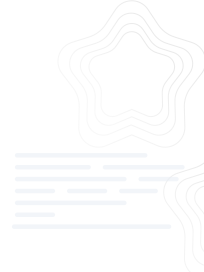
共232个文件
java:198个
json:13个
gradle:4个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 24 浏览量
2024-11-25
08:49:07
上传
评论
收藏 332KB ZIP 举报
温馨提示
Java JsonPath 实现Jayway JsonPath用于读取 JSON 文档的 Java DSL。 Jayway JsonPath 是Stefan Goessner JsonPath 实现的 Java 端口。入门JsonPath 可在中央 Maven 存储库中找到。Maven 用户将其添加到您的 POM 中。<dependency> <groupId>com.jayway.jsonpath</groupId> <artifactId>json-path</artifactId> <version>2.9.0</version></dependency>如果您需要帮助,请在Stack Overflow上提问。标记问题“jsonpath”和“java”。JsonPath 表达式始终引用 JSON 结构,就像 XPath 表达式与 XML 文档结合使用一样。JsonPath 中的“根成员对象”始终被引用,$无论它是对象还是数组。JsonPath 表达式可以使用点符号$.store.book[0].title或
资源推荐
资源详情
资源评论
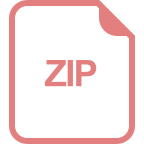
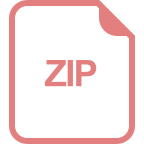
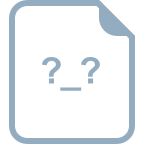
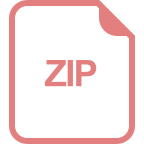
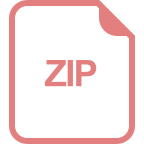
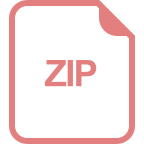
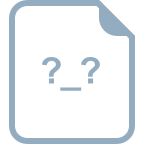
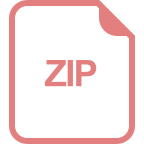
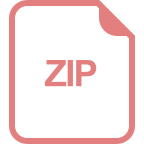
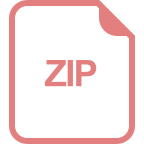
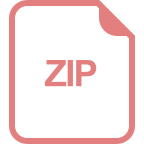
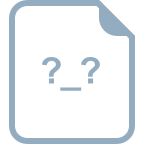
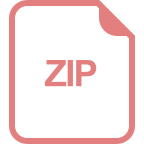
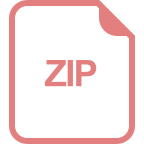
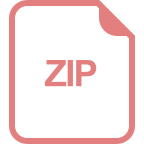
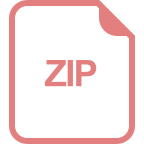
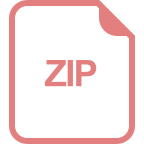
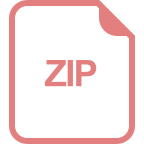
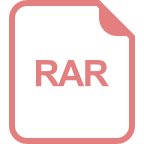
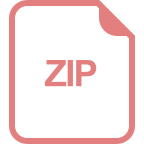
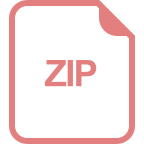
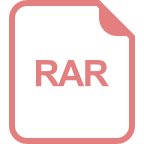
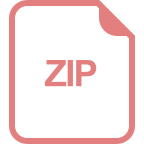
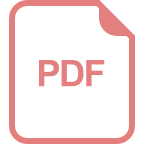
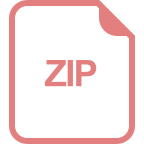
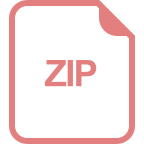
收起资源包目录

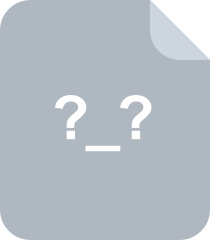
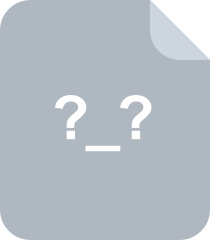
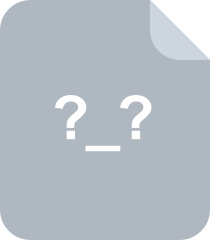
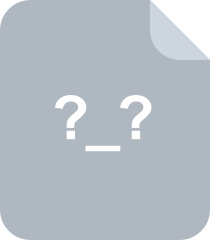
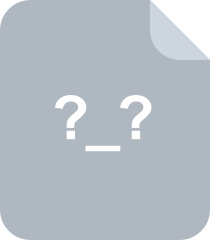
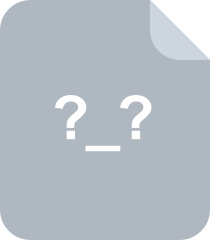
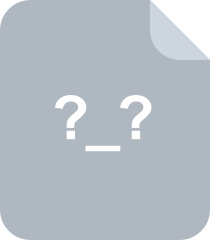
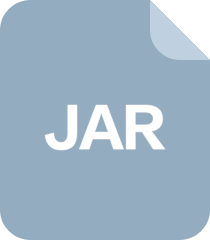
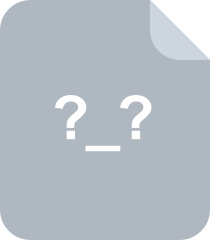
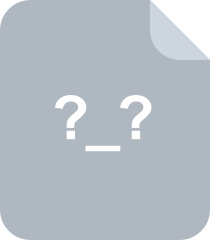
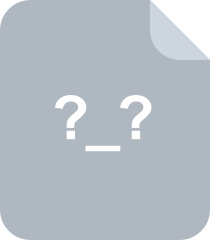
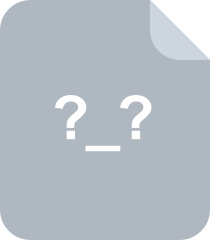
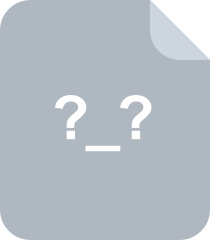
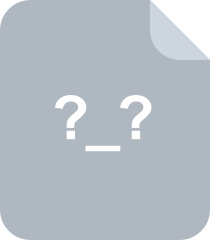
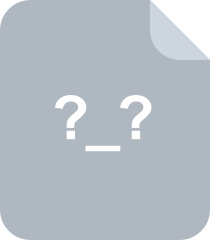
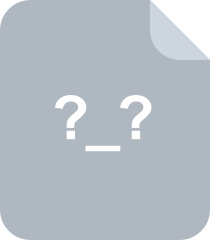
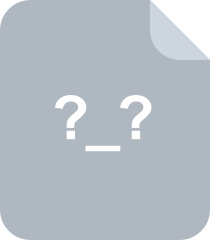
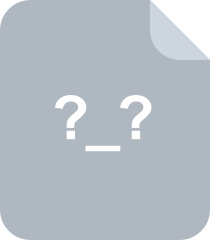
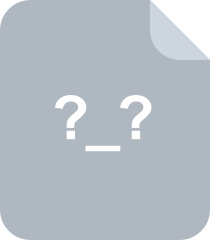
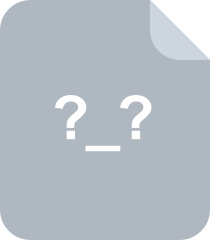
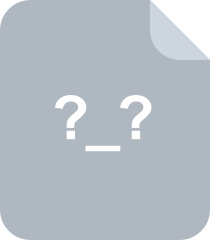
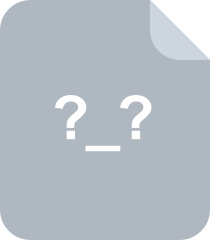
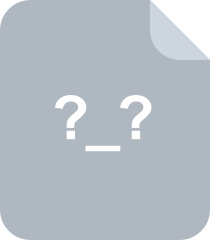
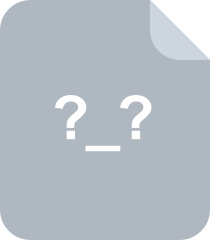
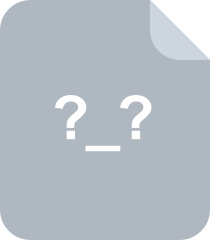
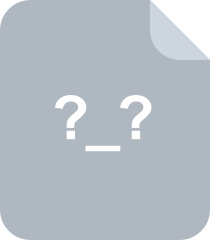
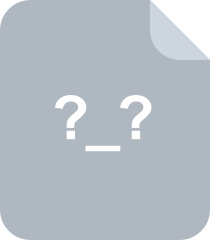
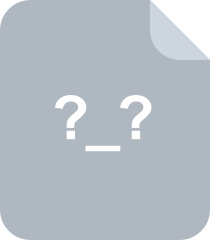
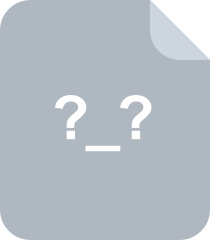
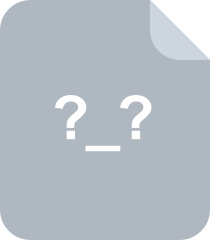
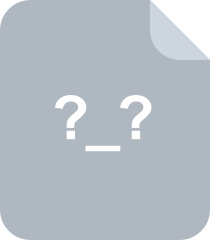
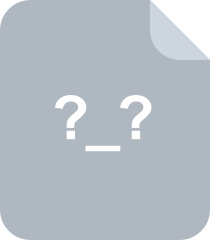
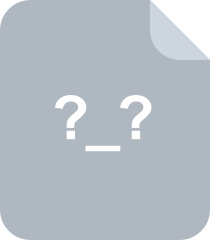
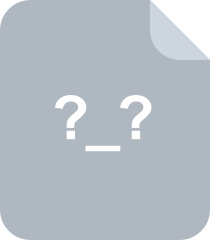
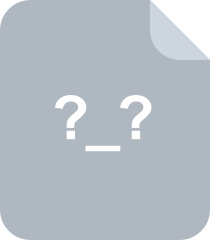
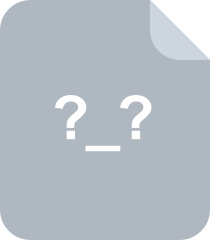
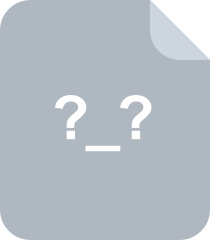
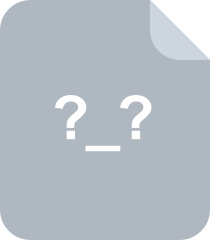
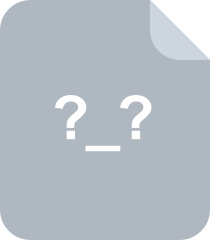
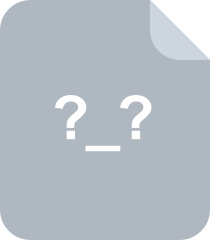
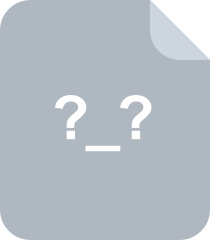
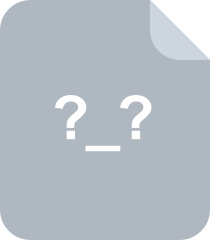
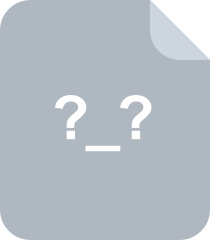
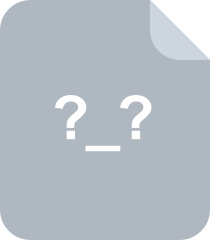
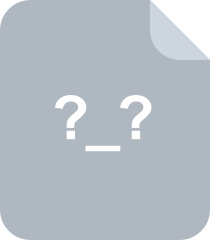
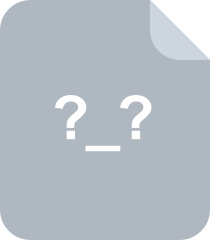
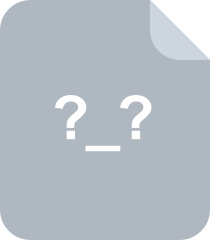
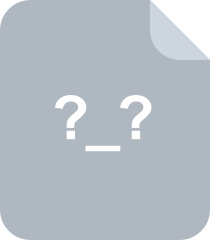
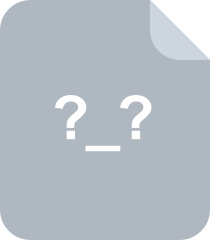
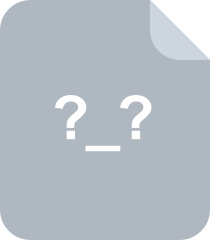
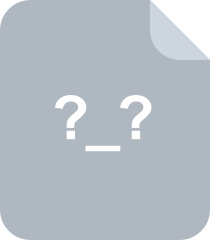
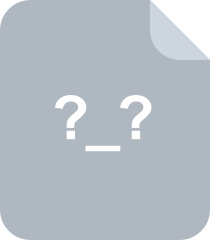
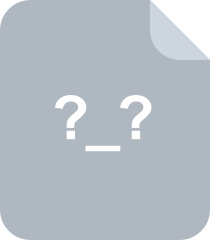
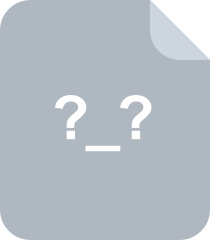
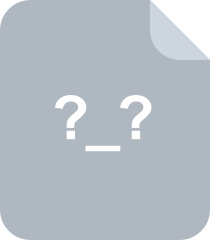
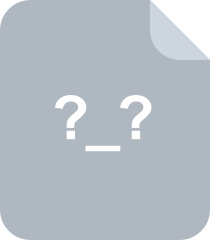
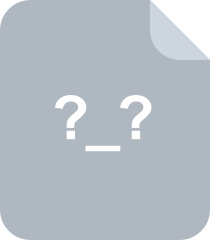
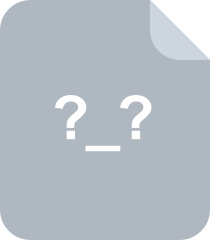
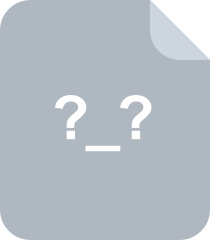
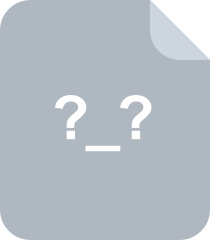
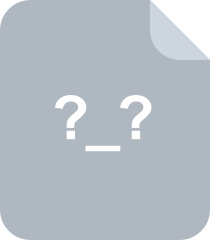
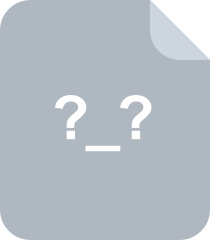
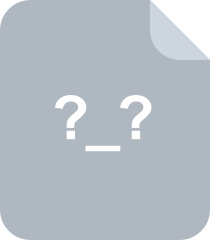
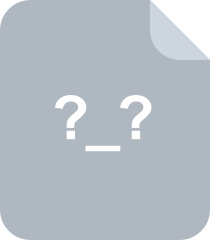
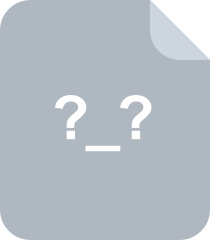
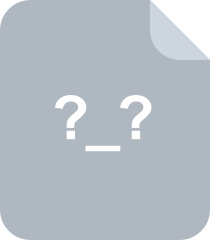
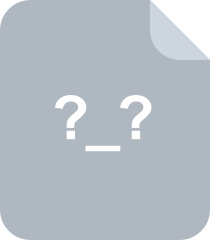
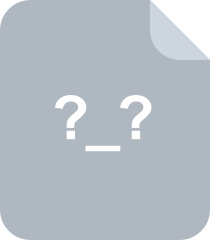
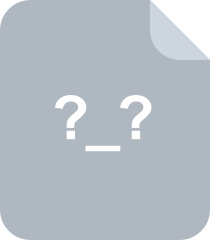
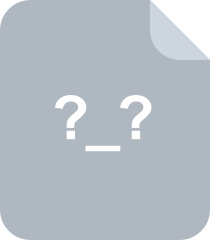
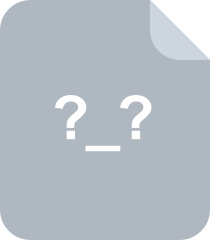
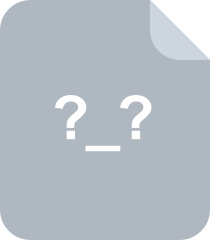
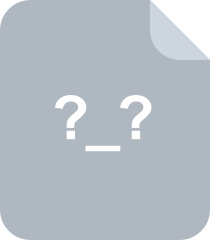
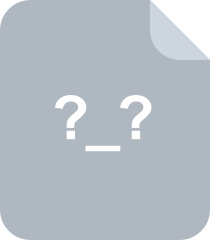
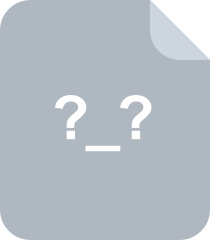
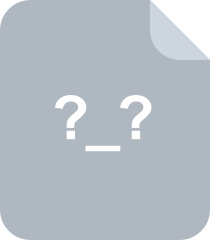
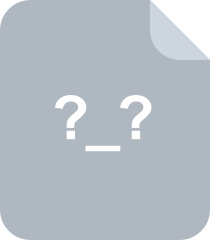
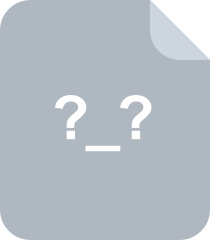
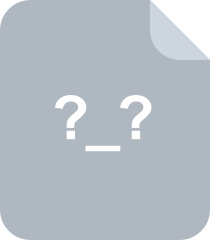
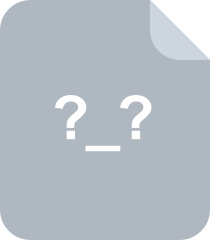
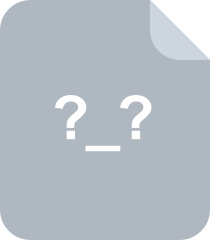
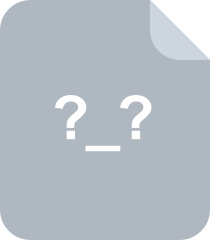
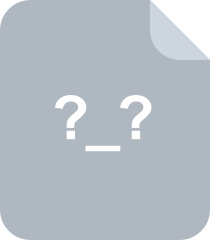
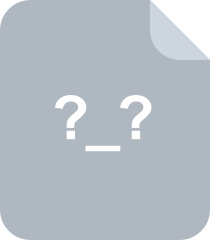
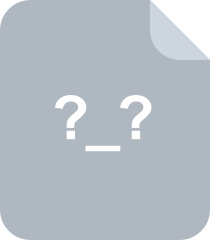
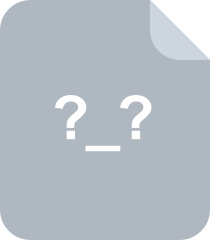
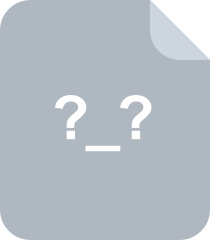
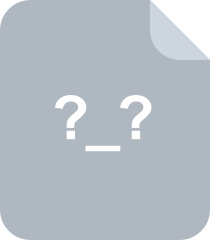
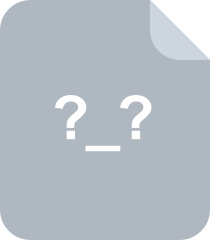
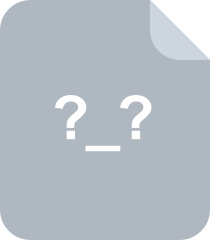
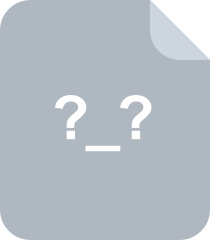
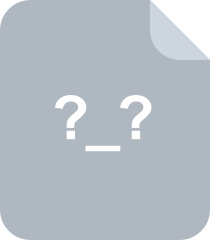
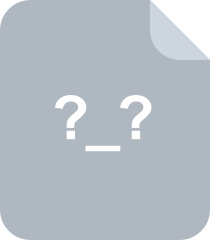
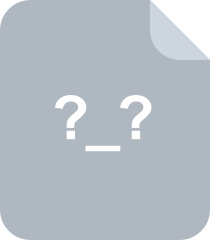
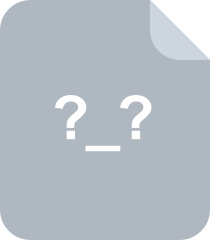
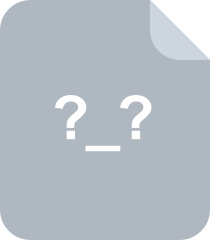
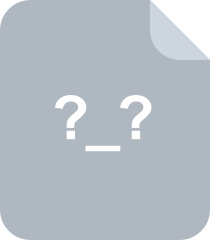
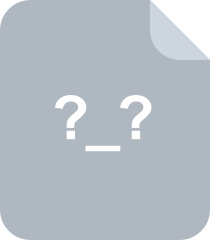
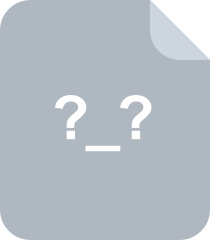
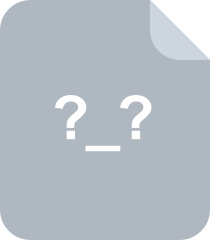
共 232 条
- 1
- 2
- 3
资源评论
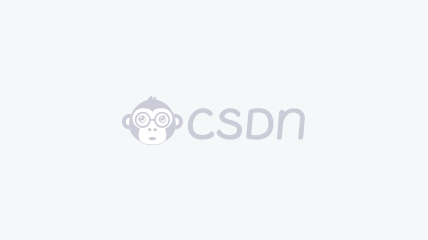

徐浪老师
- 粉丝: 8162
- 资源: 8889
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

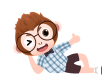
安全验证
文档复制为VIP权益,开通VIP直接复制
