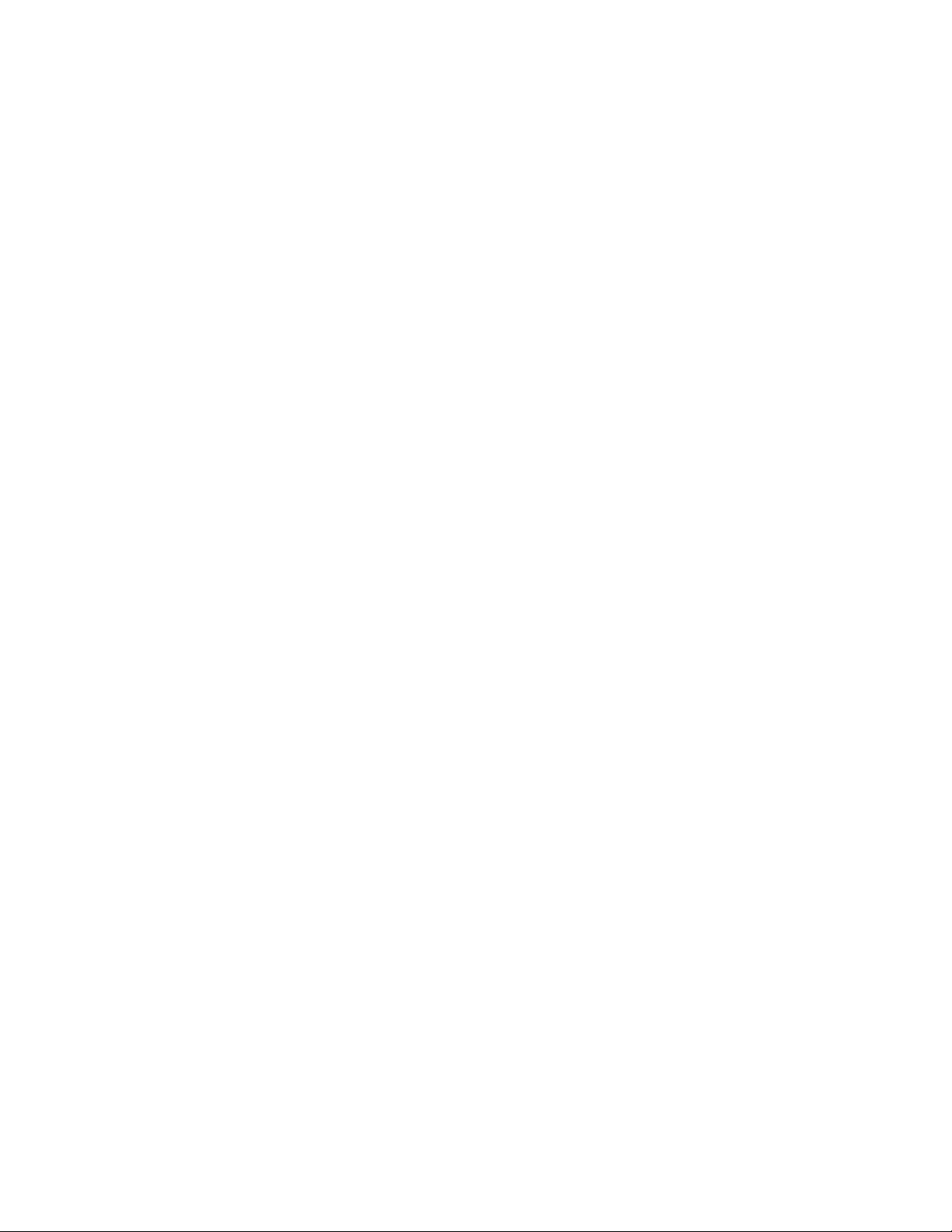
3
}
/* Output line of pixels */
linepic(line);
if (line_y % 10 == 0)
{
printf( "\nDone line %d", line_y );
fflush( stdout );
}
}
endpic();
}
2.2 Include Files
Include files define structure types, global variables,
constants, and functions types. Their use simplifies later
modification of the program.
The first include file,
typedefs.h
, defines the
structures for the basic ray tracer. Structures allow
conceptual grouping of data and simplify information
passing between routines. New attributes can easily be
added to structures.
Spheres, boxes, triangles, and superquadrics are
defined. Each object is decribed in two structures. The first
structure has the fields which are common to all objects.
The second has the fields that are unique to a given object.
o_sphere
describes spheres. It has fields for the
sphere's radius and its x,y,z center position.
The box object is defined by the
o_box
structure. It
has x,y,z center position, sizes for the x, y, and z sides and
sidehit. Sidehit is used in the computation of the surface
normal of the box.
Triangles are described by the
o_triangle
structure. It
include the triangle's plane description, three edge vectors,
and three edge constants.
The superquadric object structure,
o_superq
, has all
of the values of the box structure, plus some new values.
Thes values are described in Section 3.5.
The
t_object
structure contains the fields which are
common to all objects. It has object id, which is unique for
each object, surface type number, which specifies the color
of the surface, object type, and object pointer, which points
to one of the object type structures defined above.
The
t_light
type defines a point light source. It has an
x,y,z position in space and a brightness value.
The
t_surface
structure describes the properties of an
object's surfaces. The color is specified by red, green, and
blue components for each of ambient, diffuse, and specular
lighting. The specular coefficient controls the size and
intensity of the specular highlight. It is a positive integer
and the higher the coeficient, the smaller and brighter the
specular highlight. The reflectivity of the surface is
specified by a number from zero to one, with zero being no
reflection, one being a perfect mirror. Transparency is
specified in a similar manner, with zero being opaque, one
completely transparent. Reflections and transparency are
not included in the basic program; they are added later.
Three-dimensional vectors are represented by the
t_3d
structure. It is used throughout the program for both
positions and vectors in 3 space.
Lastly,
t_color
structure specifies a red, green, blue
color trio. Color values are passed throughout the program
in this form.
/*** typedefs.h ***/
typedef struct
{
double x,y,z;
} t_3d;
typedef struct
{
double r; /* radius */
double x,y,z; /* position */
} o_sphere;
typedef struct
{
double sidehit;/* side intersected */
double xs,ys,zs; /* size of sides */
double x,y,z; /* center position */
} o_box;
typedef struct
{
t_3d nrm; /* triangle normal */
double d; /* plane constant */
t_3d e1,e2,e3; /* edge vectors */
double d1,d2,d3;/*plane constants */
} o_triangle;
typedef struct
{
int sidehit; /* side intersected */
double xs,ys,zs; /* size of sides */
double x,y,z; /* center position */
double pow; /* n in formula */
double a,b,c,r; /* coefficients */
double err; /* error measure */
} o_superq;
typedef struct
{
int id; /* object number */
int objtyp; /* object type */
int surfnum; /* surface number */
union
{
o_sphere *p_sphere;
o_box *p_box;
o_triangle *p_triangle;
o_superq *p_superq;
} objpnt; /* object pointer */
} t_object;
typedef struct
{
double x,y,z; /* position */
double bright;
} t_light;
typedef struct
{
double ar,ag,ab; /* ambient r,g,b */
double dr,dg,db; /* diffuse r,g,b */
double sr,sg,sb;/* specular r,g,b */
double coef; /* specular coef */
double refl; /* reflection 0-1 */
double transp;/* transparency 0-1 */
} t_surface;