<?php
/****************************************************
SIMPLEPIE
A PHP-Based RSS and Atom Feed Framework
Takes the hard work out of managing a complete RSS/Atom solution.
Version: 1.0 Beta 2
Updated: 30 May 2006
Copyright: 2004-2006 Ryan Parman, Geoffrey Sneddon
http://simplepie.org
*****************************************************
LICENSE:
GNU Lesser General Public License 2.1 (LGPL)
http://creativecommons.org/licenses/LGPL/2.1/
*****************************************************
Please submit all bug reports and feature requests to the SimplePie forums.
http://simplepie.org/support/
****************************************************/
class SimplePie {
// SimplePie Information
var $name = 'SimplePie';
var $version = '1.0 Beta 2';
var $build = '20060530';
var $url = 'http://simplepie.org/';
var $useragent;
var $linkback;
// Run-time Variables
var $rss_url;
var $encoding;
var $xml_dump = false;
var $caching = true;
var $max_minutes = 60;
var $cache_location = './data/simplepie_cache';
var $bypass_image_hotlink = 'i';
var $bypass_image_hotlink_page = false;
var $replace_headers = false;
var $remove_div = true;
var $order_by_date = true;
var $strip_ads = false;
var $strip_htmltags = 'blink,body,doctype,embed,font,form,frame,frameset,html,iframe,input,marquee,meta,noscript,object,param,script,style';
var $strip_attributes = 'class,id,style,onclick,onmouseover,onmouseout,onfocus,onblur';
var $encode_instead_of_strip = false;
// RSS Auto-Discovery Variables
var $parsed_url;
var $local = array();
var $elsewhere = array();
// XML Parsing Variables
var $xml;
var $tagName;
var $insideItem;
var $insideChannel;
var $insideImage;
var $insideAuthor;
var $itemNumber = 0;
var $authorNumber = 0;
var $categoryNumber = 0;
var $enclosureNumber = 0;
var $linkNumber = 0;
var $itemLinkNumber = 0;
var $data = false;
var $attribs;
var $xmldata;
var $feed_xmlbase;
var $item_xmlbase;
var $xhtml_prefix;
/****************************************************
CONSTRUCTOR
Initiates a couple of variables. Accepts feed_url, cache_location,
and cache_max_minutes.
****************************************************/
function SimplePie($feed_url = null, $cache_location = null, $cache_max_minutes = null) {
$this->useragent = $this->name . '/' . $this->version . ' (Feed Parser; ' . $this->url . '; Allow like Gecko) Build/' . $this->build;
$this->linkback = '<a href="' . $this->url . '" title="' . $this->name . ' ' . $this->version . '">' . $this->name . '</a>';
if (!is_null($feed_url)) {
$this->feed_url($feed_url);
}
if (!is_null($cache_location)) {
$this->cache_location($cache_location);
}
if (!is_null($cache_max_minutes)) {
$this->cache_max_minutes($cache_max_minutes);
}
if (!is_null($feed_url)) {
return $this->init();
}
// If we've passed an xmldump variable in the URL, snap into XMLdump mode
if (isset($_GET['xmldump'])) {
$this->enable_xmldump($_GET['xmldump']);
}
}
/****************************************************
CONFIGURE OPTIONS
Set various options (feed URL, XML dump, caching, etc.)
****************************************************/
// Feed URL
function feed_url($url) {
$url = $this->fix_protocol($url, 1);
$this->rss_url = $url;
return true;
}
// XML Dump
function enable_xmldump($enable) {
$this->xml_dump = (bool) $enable;
return true;
}
// Bypass Image Hotlink
function bypass_image_hotlink($getvar='i') {
$this->bypass_image_hotlink = (string) $getvar;
return true;
}
// Bypass Image Hotlink Page
function bypass_image_hotlink_page($page = false) {
$this->bypass_image_hotlink_page = (string) $page;
return true;
}
// Caching
function enable_caching($enable) {
$this->caching = (bool) $enable;
return true;
}
// Cache Timeout
function cache_max_minutes($minutes) {
$this->max_minutes = (int) $minutes;
return true;
}
// Cache Location
function cache_location($location) {
$this->cache_location = (string) $location;
return true;
}
// Replace H1, H2, and H3 tags with the less important H4 tags.
function replace_headers($enable) {
$this->replace_headers = (bool) $enable;
return true;
}
// Remove outer div in XHTML content within Atom
function remove_div($enable) {
$this->remove_div = (bool) $enable;
return true;
}
// Order the items by date
function order_by_date($enable) {
$this->order_by_date = (bool) $enable;
return true;
}
// Strip out certain well-known ads
function strip_ads($enable) {
$this->strip_ads = (bool) $enable;
return true;
}
// Strip out potentially dangerous tags
function strip_htmltags($tags, $encode=false) {
$this->strip_htmltags = (string) $tags;
$this->encode_instead_of_strip = (bool) $encode;
return true;
}
// Encode dangerous tags instead of stripping them
function encode_instead_of_strip($encode=true) {
$this->encode_instead_of_strip = (bool) $encode;
return true;
}
// Strip out potentially dangerous attributes
function strip_attributes($attrib) {
$this->strip_attributes = (string) $attrib;
return true;
}
/****************************************************
MAIN INITIALIZATION FUNCTION
Rewrites the feed so that it actually resembles XML, processes the XML,
and builds an array from the feed.
****************************************************/
function init() {
// If Bypass Image Hotlink is enabled, send image to the page and quit.
if ($this->bypass_image_hotlink) {
if (isset($_GET[$this->bypass_image_hotlink]) && !empty($_GET[$this->bypass_image_hotlink])) {
$this->display_image($_GET[$this->bypass_image_hotlink]);
exit;
}
}
// If Bypass Image Hotlink is enabled, send image to the page and quit.
if (isset($_GET['js'])) {
// JavaScript for the Odeo Player
$embed='';
$embed.='function embed_odeo(link) {';
$embed.='document.writeln(\'';
$embed.='<object classid="clsid:d27cdb6e-ae6d-11cf-96b8-444553540000" ';
$embed.=' codebase="http://fpdownload.macromedia.com/pub/shockwave/cabs/flash/swflash.cab#version=7,0,0,0" ';
$embed.=' width="440" ';
$embed.=' height="80" ';
$embed.=' align="middle">';
$embed.='<param name="movie" value="http://odeo.com/flash/audio_player_fullsize.swf" />';
$embed.='<param name="allowScriptAccess" value="any" />';
$embed.='<param name="quality" value="high">';
$embed.='<param name="wmode" value="transparent">';
$embed.='<param name="flashvars" value="valid_sample_rate=true&external_url=\'+link+\'" />';
$embed.='<embed src="http://odeo.com/flash/audio_player_fullsize.swf" ';
$embed.=' pluginspage="http://www.macromedia.com/go/getflashplayer" ';
$embed.=' type="application/x-shockwave-flash" ';
$embed.=' quality="high" ';
$embed.=' width="440" ';
$embed.=' height="80" ';
$embed.=' wmode="transparent" ';
$embed.=' allowScriptAccess="any" ';
$embed.=' flashvars="valid_sample_rate=true&external_url=\'+link+\'">';
$embed.='</embed>';
$embed.='</object>';
$embed.='\');';
$embed.='}';
$embed.="\r\n";
$embed.='function embed_quicktime(type, bgcolor, width, height, link, placeholder, loop) {';
$embed.='document.writeln(\'';
$embed.='<object classid="clsid:02BF25D5-8C17-4B23-BC80-D3488ABDDC6B" ';
$embed.=' style="cursor:hand; cursor:pointer;" ';
$embed.=' type="\'+type+\'" ';
$embed.=' codebase="http://www.apple.com/qtactivex/qtplugin.cab" ';
$embed.=' bgcolor="\'+bgcolor+\'" ';
$embed.=' width="\'+width+\'" ';
$embed.=' height="\'+height+\'">';
$embed.='<param name="href" value="\'+link+\'" />';
$embed.='<param name="src" value="\'+placeho

jiesenfeng
- 粉丝: 1
- 资源: 5
最新资源
- 毕设和企业适用springboot汽车管理类及教育评价系统源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及基因数据分析平台源码+论文+视频.zip
- 毕设和企业适用springboot区域电商平台类及电力系统优化平台源码+论文+视频.zip
- 毕设和企业适用springboot区域电商平台类及个性化推荐系统源码+论文+视频.zip
- 毕设和企业适用springboot区域电商平台类及国际贸易平台源码+论文+视频.zip
- 毕设和企业适用springboot人工智能客服系统类及视频监控平台源码+论文+视频.zip
- 毕设和企业适用springboot人工智能客服系统类及旅游规划平台源码+论文+视频.zip
- 毕设和企业适用springboot人工智能客服系统类及市场营销自动化平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及企业管理平台源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及气象数据管理系统源码+论文+视频.zip
- 毕设和企业适用springboot汽车管理类及数字内容管理平台源码+论文+视频.zip
- 毕设和企业适用springboot区域电商平台类及跨境物流平台源码+论文+视频.zip
- 毕设和企业适用springboot区域电商平台类及互联网金融平台源码+论文+视频.zip
- 毕设和企业适用springboot区域电商平台类及活动管理平台源码+论文+视频.zip
- 毕设和企业适用springboot人工智能客服系统类及视频监控系统源码+论文+视频.zip
- 毕设和企业适用springboot人工智能客服系统类及数字内容管理平台源码+论文+视频.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


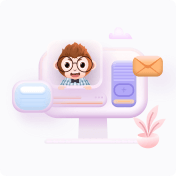