在IT领域,Windows服务是一种特殊的后台应用程序,它可以在没有用户交互的情况下运行,通常用于执行计划任务、管理系统资源或提供持续的系统功能。本教程将深入探讨如何使用C#语言来创建Windows服务,以及如何实现自动启动。 我们需要了解Windows服务的基本概念。Windows服务是Windows操作系统的一个组件,它在系统启动时加载并持续运行,不受用户登录或注销的影响。服务通过`System.ServiceProcess`命名空间中的类来创建和管理,主要包括`ServiceBase`类和`ServiceController`类。 创建Windows服务的第一步是建立一个新的C#项目,并引用`System.ServiceProcess`库。接着,创建一个继承自`ServiceBase`的类,这个类将代表你的服务。在类中,你需要重写`OnStart`和`OnStop`方法,分别定义服务启动和停止时的行为。 ```csharp using System.ServiceProcess; public class MyWindowsService : ServiceBase { public MyWindowsService() { this.ServiceName = "MyCustomService"; } protected override void OnStart(string[] args) { // 在这里添加服务启动时要执行的代码 } protected override void OnStop() { // 在这里添加服务停止时要执行的代码 } } ``` 为了使服务能够响应控制台命令(如手动启动和停止),还需要在类中添加`OnCustomCommand`方法: ```csharp protected override void OnCustomCommand(int command) { switch (command) { case 1: // 执行特定命令 break; // 添加其他命令处理 } } ``` 接下来,创建一个安装程序类,该类继承自`System.Configuration.Install.Installer`,并包含`ServiceProcessInstaller`和`ServiceInstaller`。这使得服务可以通过安装向导进行安装和卸载。 ```csharp using System.Configuration.Install; using System.ComponentModel; [RunInstaller(true)] public partial class ProjectInstaller : Installer { public ProjectInstaller() { InitializeComponent(); ServiceProcessInstaller processInstaller = new ServiceProcessInstaller(); ServiceInstaller serviceInstaller = new ServiceInstaller(); processInstaller.Account = ServiceAccount.LocalSystem; serviceInstaller.StartType = ServiceStartMode.Automatic; // 设置为自动启动 serviceInstaller.ServiceName = "MyCustomService"; serviceInstaller.DisplayName = "我的自定义服务"; this.Installers.Add(processInstaller); this.Installers.Add(serviceInstaller); } } ``` 现在,你可以通过`installutil.exe`工具安装服务。确保`installutil.exe`位于.NET Framework的安装目录(如`C:\Windows\Microsoft.NET\Framework\v4.0.30319`),然后运行以下命令: ```shell installutil.exe MyWindowsService.exe ``` 要启动或停止服务,可以使用`ServiceController`类,或者通过“服务”管理工具。例如,启动服务的代码如下: ```csharp ServiceController sc = new ServiceController("MyCustomService"); sc.Start(); ``` 总结来说,使用C#创建Windows服务涉及以下几个关键步骤: 1. 引用`System.ServiceProcess`命名空间。 2. 创建一个继承自`ServiceBase`的类,重写`OnStart`和`OnStop`方法。 3. 实现安装程序类,使用`ServiceProcessInstaller`和`ServiceInstaller`。 4. 使用`installutil.exe`工具安装服务。 5. 使用`ServiceController`类管理服务的生命周期。 这个过程可以帮助开发者创建定制的、自动化启动的Windows服务,以满足各种系统管理需求。在实际项目中,可能还需要考虑错误处理、日志记录、配置管理等细节,以确保服务的稳定性和可维护性。
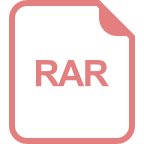
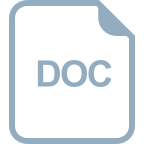
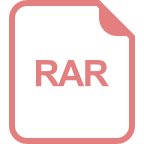
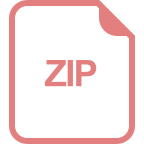
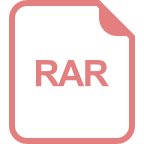
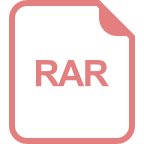
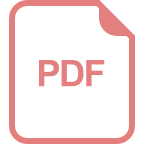
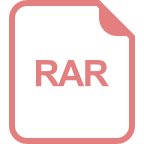
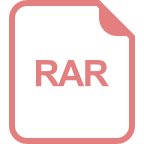
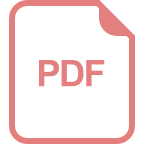
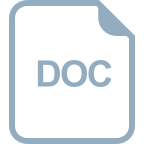
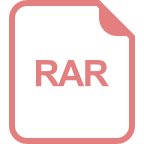
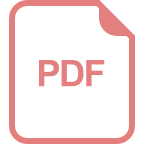
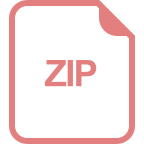
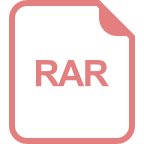
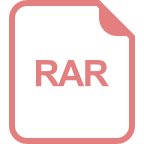
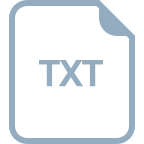
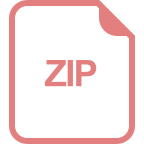
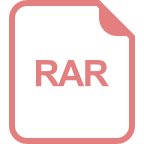
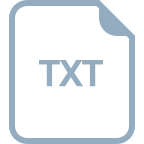
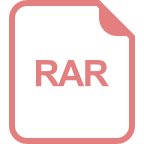
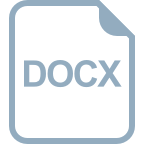
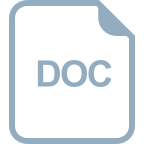
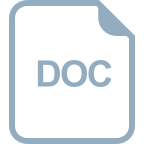
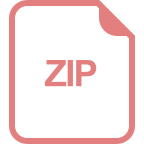





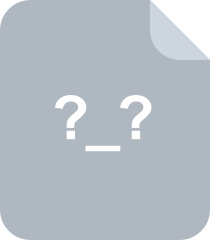
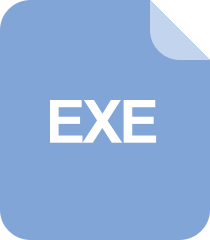
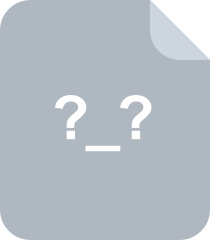
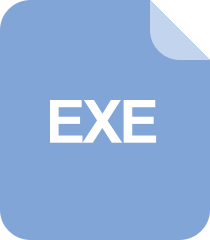
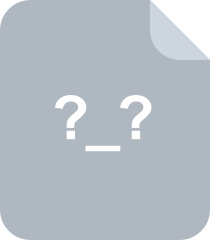
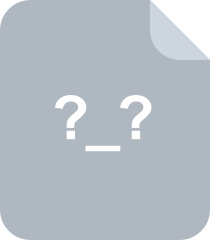
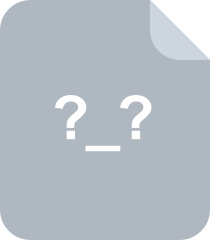



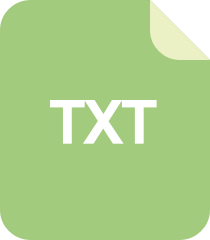
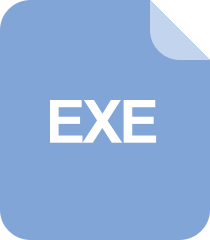
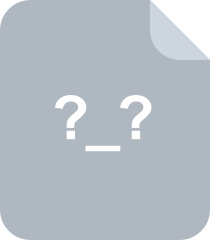
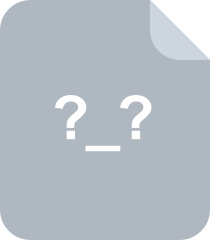
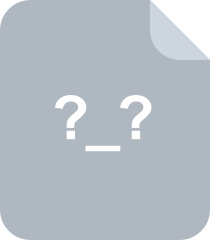
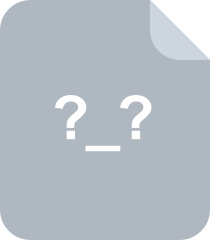
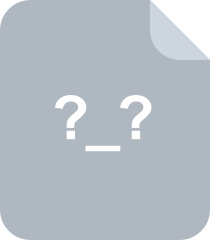
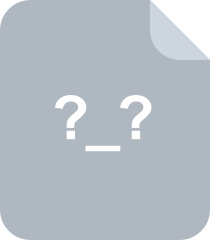

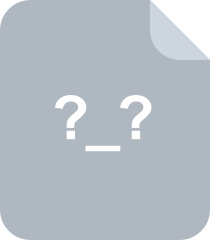
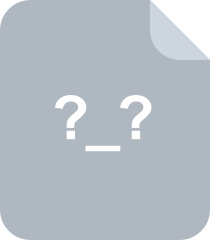
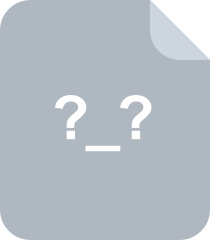
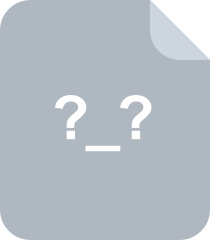
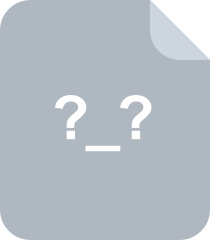
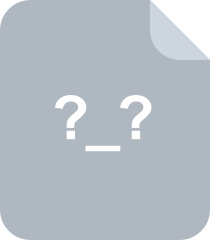
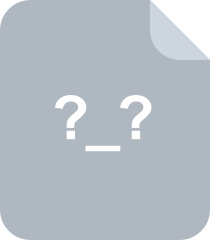
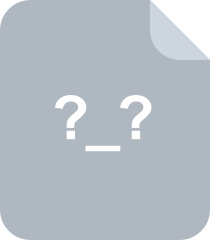

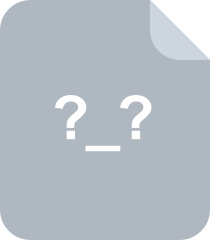
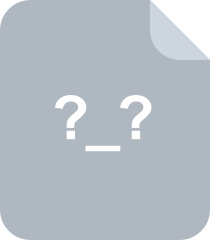



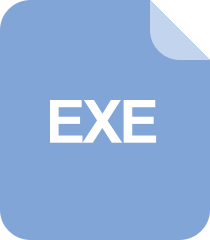
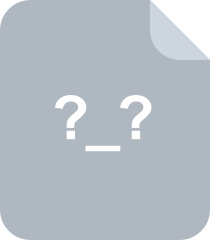
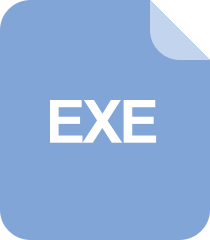
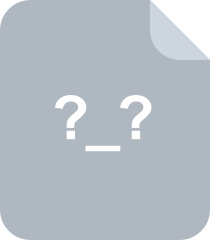
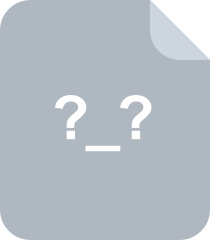
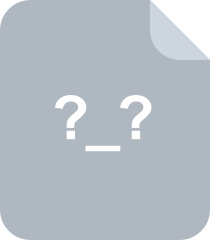
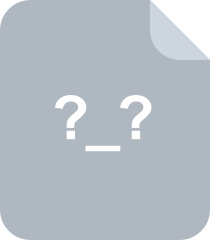



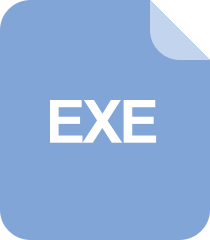
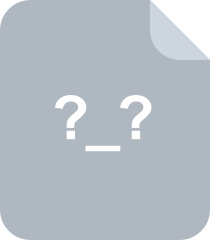
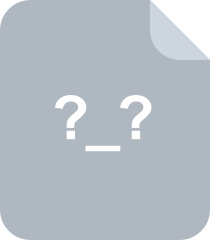
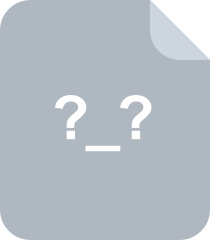
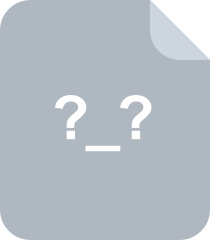
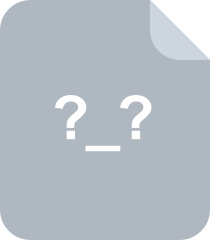

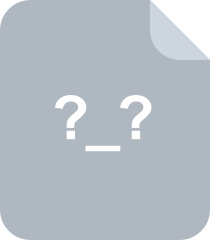
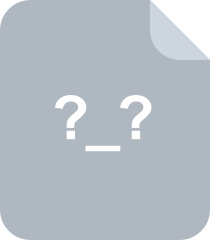
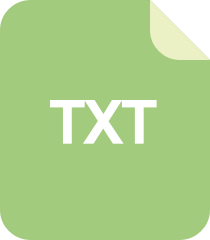
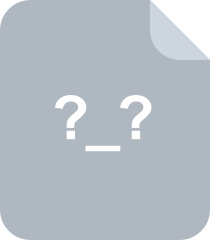
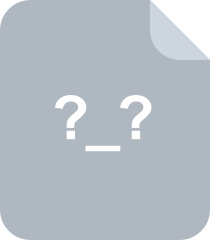

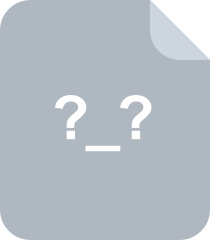
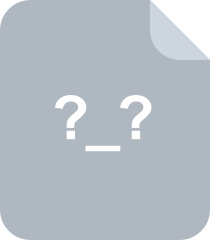
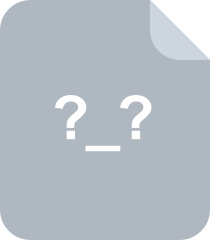
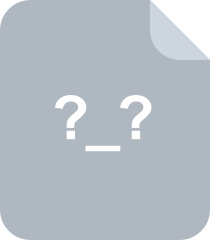
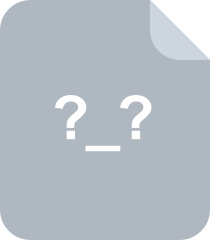
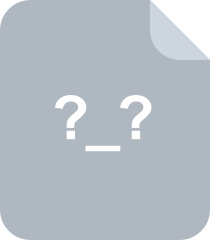
- 1
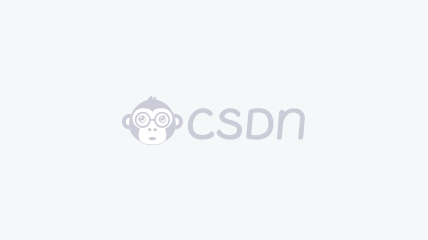

- 粉丝: 402
- 资源: 25
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

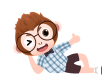
最新资源
- 基于Selenium页面爬取某东商品价格监控:自定义商品价格,降价邮件微信提醒资料齐全+详细文档+源码.zip
- 基于selenium爬取通过搜索关键词采用指定页数的商品信息资料齐全+详细文档+源码.zip
- 基于今日头条自动发文机器人,各大公众平台采集爬虫资料齐全+详细文档+源码.zip
- 基于集众多数据源于一身的爬虫工具箱,旨在安全快捷的帮助用户拿回自己的数据,工具代码开源,流程透明、资料齐全+详细文档+源码.zip
- 基于拼多多爬虫,爬取所有商品、评论等信息资料齐全+详细文档+源码.zip
- 基于爬虫从入门到入狱资料齐全+详细文档+源码.zip
- 基于爬虫学习仓库,适合零基础的人学习,对新手比较友好资料齐全+详细文档+源码.zip
- 基于天眼查爬虫资料齐全+详细文档+源码.zip
- 基于千万级图片爬虫、视频爬虫资料齐全+详细文档+源码.zip
- 基于支付宝账单爬虫资料齐全+详细文档+源码.zip
- 基于SpringBoot+Vue3实现的在线考试系统(三)代码
- 数组-.docx cccccccccccccccccccccc
- Ruby技巧中文最新版本
- Ruby袖珍参考手册pdf英文文字版最新版本
- 融合导航项目全套技术资料100%好用.zip
- 四足机器人技术进展与应用场景

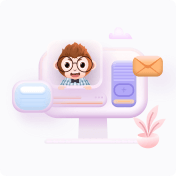
