package cn.zwz.tableout;
import cn.zwz.tableout.entity.TableInfo;
import org.apache.poi.hssf.usermodel.HSSFCellStyle;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import cn.hutool.core.collection.CollUtil;
import cn.hutool.db.Db;
import cn.hutool.db.Entity;
import cn.hutool.db.ds.simple.SimpleDataSource;
import java.io.FileOutputStream;
import java.io.IOException;
import java.sql.SQLException;
import java.util.Iterator;
import java.util.List;
import javax.sql.DataSource;
import org.apache.poi.hssf.usermodel.HSSFWorkbook;
import org.apache.poi.ss.usermodel.BorderStyle;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.Font;
import org.apache.poi.ss.usermodel.HorizontalAlignment;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.util.CellRangeAddress;
import org.springframework.boot.autoconfigure.jdbc.DataSourceAutoConfiguration;
import org.springframework.boot.autoconfigure.orm.jpa.HibernateJpaAutoConfiguration;
@SpringBootApplication(
exclude = {DataSourceAutoConfiguration.class, HibernateJpaAutoConfiguration.class}
)
public class TableOutApplication {
private static String baseUrl = "jdbc:mysql://localhost:3306/information_schema";
private static String username = "root";
private static String password = "123456";
private static String schema = "t500";
public TableOutApplication() {
}
public static void main(String[] args) throws SQLException, IOException {
SpringApplication.run(TableOutApplication.class, args);
Workbook wb = new HSSFWorkbook();
Sheet sheet = wb.createSheet(schema);
sheet.setColumnWidth(0, 6000);
sheet.setColumnWidth(1, 6000);
sheet.setColumnWidth(2, 6000);
sheet.setColumnWidth(3, 9000);
CellStyle cellStyle = wb.createCellStyle();
CellStyle titleStyle = wb.createCellStyle();
cellStyle.setBorderBottom(BorderStyle.THIN);
cellStyle.setBorderTop(BorderStyle.THIN);
cellStyle.setBorderLeft(BorderStyle.THIN);
cellStyle.setBorderRight(BorderStyle.THIN);
cellStyle.setAlignment(HorizontalAlignment.CENTER);
titleStyle.setAlignment(HorizontalAlignment.CENTER);
Font font = wb.createFont();
font.setBold(true);
font.setFontHeight((short)240);
font.setFontName("黑体");
titleStyle.setFont(font);
Integer index = 0;
List<String> tableNames = getTableNames(schema);
Integer var9 = index;
index = index + 1;
sheet.createRow(var9);
Row firstRow = sheet.createRow(0);
firstRow.setHeight((short) ((short) 15*20));//设置行高
Cell firstCell = firstRow.createCell(0);
firstCell.setCellValue("Designer小郑 数据库文档导出工具");
firstCell.setCellStyle(titleStyle);
CellRangeAddress region1 = new CellRangeAddress((short)0, (short)0, (short) 0, (short) 3);
sheet.addMergedRegion(region1);
for(int i = 0; i < tableNames.size(); ++i) {
Integer var10 = index;
index = index + 1;
Row row = sheet.createRow(var10);
Cell cell = row.createCell(1);
cell.setCellStyle(titleStyle);
cell.setCellValue((String)tableNames.get(i) + "表");
cell = row.createCell(2);
cell.setCellStyle(titleStyle);
CellRangeAddress cellRangeAddress = new CellRangeAddress(index - 1, index - 1, 1, 2);
sheet.addMergedRegionUnsafe(cellRangeAddress);
Integer var12 = index;
index = index + 1;
row = sheet.createRow(var12);
cell = row.createCell(0);
cell.setCellStyle(cellStyle);
cell.setCellValue("字段名");
cell = row.createCell(1);
cell.setCellStyle(cellStyle);
cell.setCellValue("数据类型");
cell = row.createCell(2);
cell.setCellStyle(cellStyle);
cell.setCellValue("长度");
cell = row.createCell(3);
cell.setCellStyle(cellStyle);
cell.setCellValue("备注");
List<TableInfo> tableInfos = getTableInfos((String)tableNames.get(i), schema);
for(int j = 0; j < tableInfos.size(); ++j) {
String flag = "";
Integer var15 = index;
index = index + 1;
row = sheet.createRow(var15);
cell = row.createCell(0);
cell.setCellStyle(cellStyle);
cell.setCellValue(((TableInfo)tableInfos.get(j)).getColumnName());
cell = row.createCell(1);
cell.setCellStyle(cellStyle);
cell.setCellValue(((TableInfo)tableInfos.get(j)).getDataType());
if ("int".equals(((TableInfo)tableInfos.get(j)).getDataType())) {
flag = "int";
}
if ("bigint".equals(((TableInfo)tableInfos.get(j)).getDataType())) {
flag = "bigint";
}
cell = row.createCell(2);
cell.setCellStyle(cellStyle);
if (!"".equals(((TableInfo)tableInfos.get(j)).getCharacterMaximumLength()) && ((TableInfo)tableInfos.get(j)).getCharacterMaximumLength() != null) {
cell.setCellValue(((TableInfo)tableInfos.get(j)).getCharacterMaximumLength());
} else {
cell.setCellValue("0");
if ("int".equals(flag)) {
cell.setCellValue("11");
}
if ("bigint".equals(flag)) {
cell.setCellValue("20");
}
}
cell = row.createCell(3);
cell.setCellStyle(cellStyle);
cell.setCellValue(((TableInfo)tableInfos.get(j)).getColumnComment());
}
Integer var21 = index;
index = index + 1;
sheet.createRow(var21);
}
FileOutputStream fout = new FileOutputStream(schema + ".xls");
wb.write(fout);
fout.close();
wb.close();
}
private static List<String> getTableNames(String schema) throws SQLException {
DataSource ds = getDatasource();
String sql = "select t.TABLE_NAME from `TABLES` t where TABLE_SCHEMA = ?";
List<Entity> list = Db.use(ds).query(sql, new Object[]{schema});
List<String> tableNames = CollUtil.newArrayList(new String[0]);
Iterator var5 = list.iterator();
while(var5.hasNext()) {
Entity entity = (Entity)var5.next();
String tableName = entity.getStr("table_name");
tableNames.add(tableName);
}
return tableNames;
}
private static List<TableInfo> getTableInfos(String tableName, String schema) throws SQLException {
DataSource ds = getDatasource();
String sql = "select c.COLUMN_NAME,c.DATA_TYPE,c.CHARACTER_MAXIMUM_LENGTH,c.COLUMN_COMMENT from `COLUMNS` c WHERE TABLE_NAME= ? and TABLE_SCHEMA= ?";
List<Entity> list = Db.use(ds).query(sql, new Object[]{tableName, schema});
List<TableInfo> infos = CollUtil.newArrayList(new TableInfo[0]);
Iterator var6 = list.iterator();
while(var6.hasNext()) {
Entity entity = (Entity)var6.next();
TableInfo info = new TableInfo();
String columnName = entity.getStr("column_name");
info.setColumnName(columnName);
String dataType = entity.getStr("data_type");
info.setDataType(dataType);
String characterMaximumLength = entity.getStr("character_maximum_length");
info.setCharacterMaximumLength(characterMaximumLength);
St
没有合适的资源?快使用搜索试试~ 我知道了~
MySql导出表结构到Word文档 支持导出MySQL数据库表结构!! 运行环境:jdk8+,需要Java运行环境
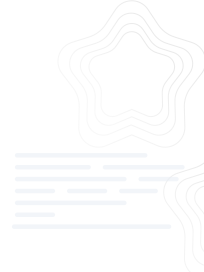
共55个文件
xml:46个
java:3个
yml:2个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 165 浏览量
2025-03-24
06:39:49
上传
评论
收藏 38KB ZIP 举报
温馨提示
MySql导出表结构到Word文档 支持导出MySQL数据库表结构!! 运行环境:jdk8+,需要Java运行环境
资源推荐
资源详情
资源评论





























收起资源包目录


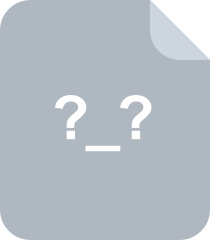






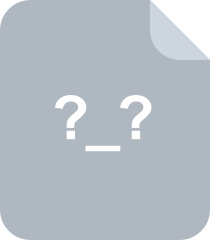


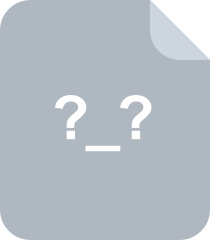




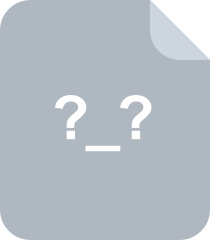

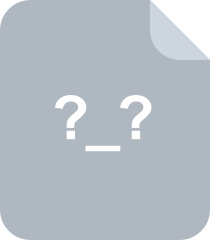

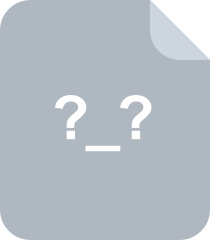

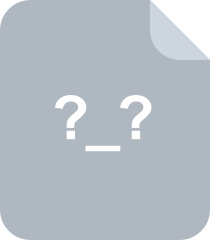
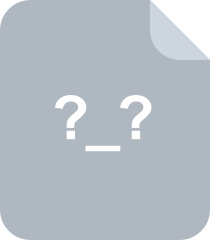
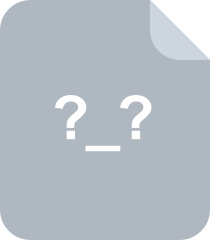
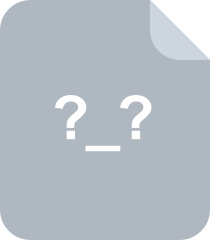
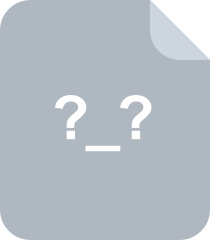
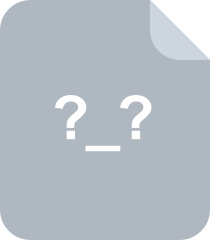
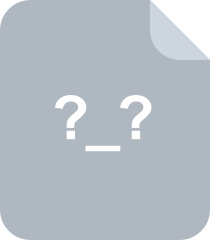
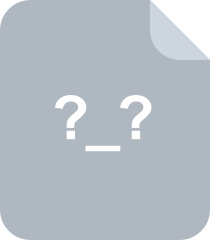
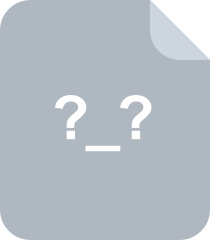
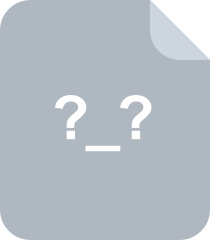
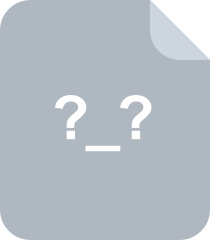
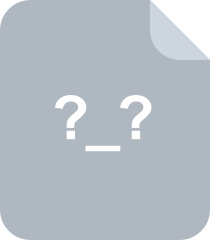
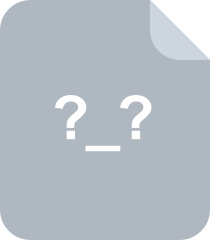
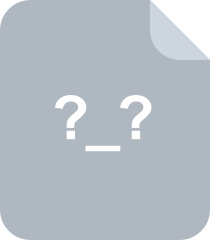
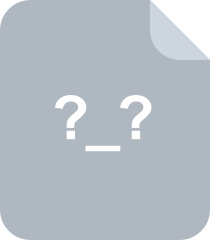
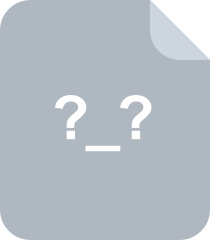
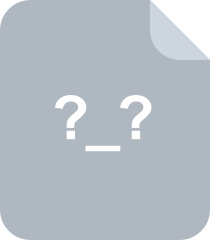
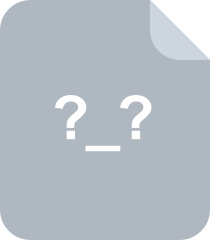
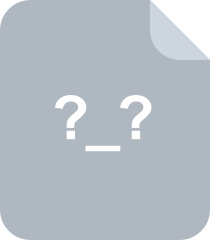
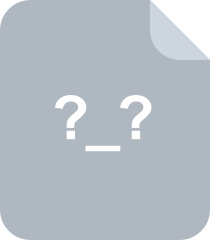
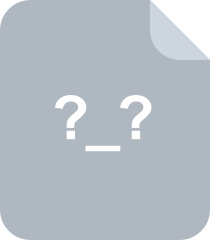
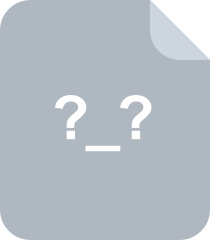
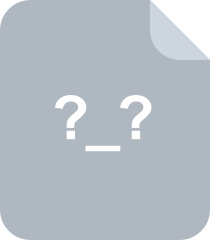
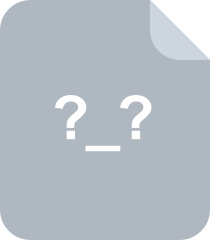
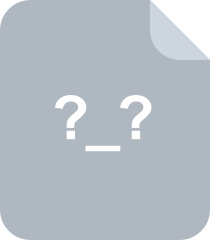
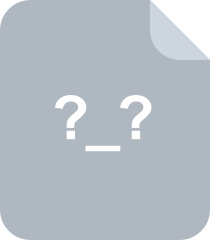
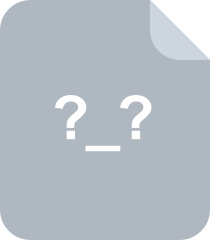
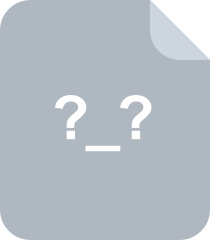
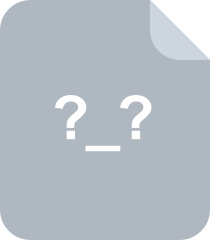
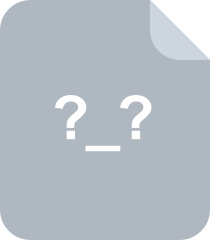
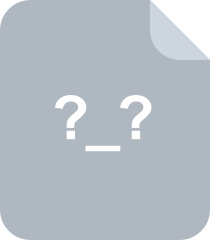
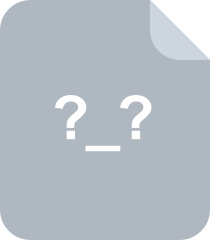
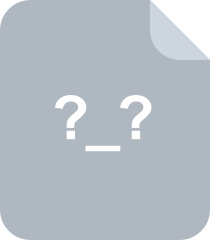
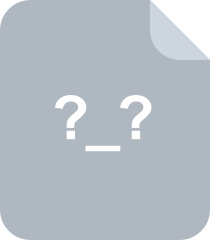
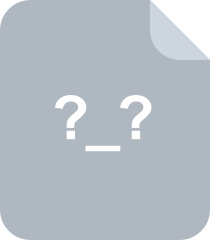
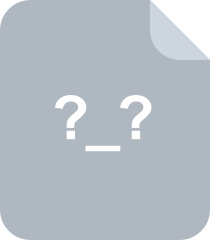
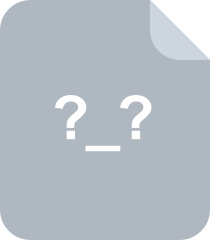
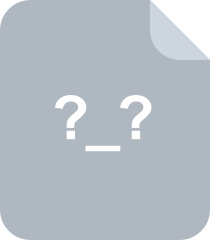
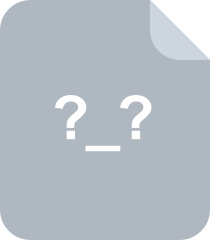
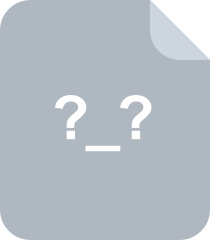
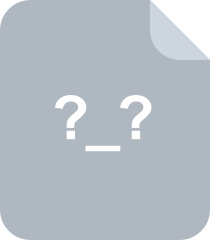
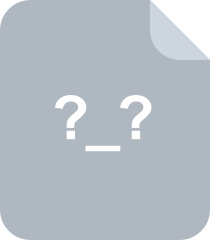
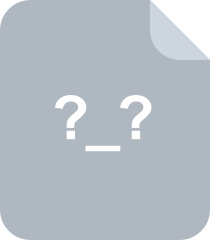
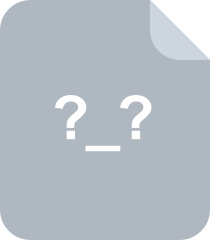
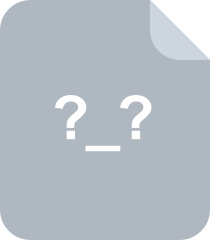
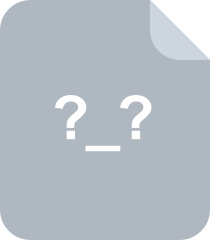





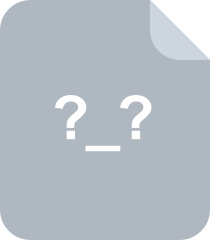

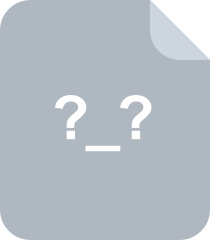
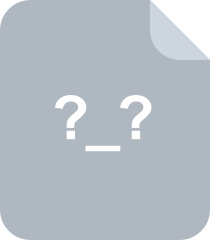


共 55 条
- 1
资源评论
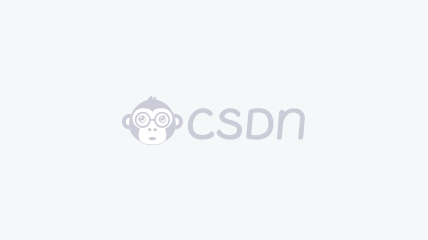
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整


枫叶学长(专业接毕设)
- 粉丝: 1w+
- 资源: 296
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

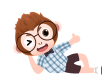
最新资源
- Delphi 12.3控件之SiComponents Tsilang 7.5.0.0 D10.4-D12.7z
- SQL数据库的创建修改与删除实验报告
- Delphi 12.3控件之SiComponents TsiLang Components Suite V7.6.0.1 for D7-DX10.3 WIN32-WIN64 ONLY.7z
- 工业自动化中三菱PLC系统的多机同步与高效产能控制解决方案
- 坦克竞技场UE4实战开发资源包
- Delphi 12.3控件之Steema TeeChart Pro VCL & FMX v2023.38 Support Delphi 12 Athens Full Source.7z
- 计算机等级考试二级Java模拟练习
- 工业自动化领域基于Go语言的远程PLC通讯编程调试监控方案
- Delphi 12.3控件之StreamSec-Tools-4.0.1.324.7z
- 惯导仿真程序.7zmatlab源代码
- PandaX TypeScript 资源大全
- Delphi 12.3控件之StyleControls 5.77.7z
- 前端-JavaScript-图形编辑工具划分定位工具-sysui+canvas
- 三菱PLC功能块FB程序集:涵盖伺服控制与变频器通讯,适用于FX3U与Q系列PLC
- Delphi 12.3控件之StyleControls 5.80.7z
- 全国计算机等级考试二级C语言考试
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


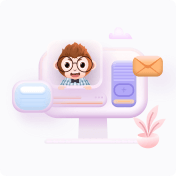
安全验证
文档复制为VIP权益,开通VIP直接复制
