#include<iostream>
#include<fstream>
#include<string>
#include<new>
using namespace std;
class AFD; //类声明
class MFD;
//用户文件目录
class UFD{
protected:
string fname; //文件名
int pcode; //保护码
int length; //文件长度(AFD中为写指针)
public:
UFD *next;
virtual void printfile();
UFD *findfile1(string fname);
string getfname();
int getpcode();
int getlength();
void addfile();
void editfile(int len); //更新文件长度
void createfile();
void deletefile(AFD *run);
void openfile(AFD *run);
void bye(AFD *run);
};
//运行目录文件
class AFD:public UFD{
private:
int readp; //读指针
public:
AFD *next; //下一打开文件
virtual void printfile();
AFD *findfile2(string fname);
int getreadp();
void addfile(AFD *run,string name,int code,int len); //添加打开文件
void editfile(int wlen,int rlen); //更新读写指针
void readfile();
void writefile();
void closefile(UFD *user);
};
//主文件目录
//MFD带头结点
class MFD{
public:
string uname; //用户名
UFD *dir; //该用户文件目录指针
MFD *next; //下一用户
void init(); //新建MFD初始化
UFD* finduser(string username); //搜索用户
};
//初始化MFD内容
void MFD::init(){
uname="\n";
dir=NULL;
next=NULL;
MFD *temp1=this;
UFD *temp2;
int usernum;
int filenum;
cout<<"===========新建MFD==========="<<endl;
cout<<"创建用户数:";
cin>>usernum;
for(int i=1;i<=usernum;i++){
temp1->next=new MFD;
cout<<"\n第"<<i<<"个用户名字:";
cin>>temp1->next->uname;
cout<<"用户"<<temp1->next->uname<<"文件数:";
cin>>filenum;
temp1->next->dir=new UFD;
temp2=temp1->next->dir;
temp2->next=NULL;
for(int j=0;j<filenum;j++){
temp2->next=new UFD;
temp2->next->addfile();
temp2=temp2->next;
}
temp1->next->next=NULL;
temp1=temp1->next;
}
}
//传入用户名
//在MFD查找用户
//返回用户UFD指针
UFD* MFD::finduser(string username){
MFD *temp;
for(temp=next;temp;temp=temp->next){ //查找用户
if(0==temp->uname.compare(username))return temp->dir; //用户名比较
}
return NULL; //没找到用户
}
//------------------------------------------------------------------------------------
//增加UFD文件
//用于修改文件内容
void UFD::addfile(){
cout<<"创建文件名:";
cin>>fname;getchar();
cout<<"文件"<<fname<<"保护码(1.读 2.写 3.执行):";
for(cin>>pcode;pcode!=1&&pcode!=2&&pcode!=3;){
cout<<"重新输入保护码(1.读 2.写 3.执行):";
cin>>pcode;
}
cout<<"文件"<<fname<<"长度:";
for(cin>>length;cin.fail();){
cerr<<"长度输入错误"<<endl;
cin.clear();
cout<<"重新输入文件"<<fname<<"长度:";
}
next=NULL;
}
//创建UFD文件
void UFD::createfile(){
UFD *temp;
temp=new UFD;
temp->addfile();
temp->next=next;
next=temp;
}
//查找用户文件
//返回相应文件前一指针
UFD* UFD::findfile1(string fname){
UFD *temp1,*temp2;
for(temp1=this,temp2=temp1->next;temp2;){
if(0==temp2->fname.compare(fname))break; //比较找到文件
temp1=temp2;
temp2=temp2->next;
}
if(!temp2)return NULL; //没找到
else return temp1; //找到返回前一指针
}
//传入AFD
//查找并删除要求文件
void UFD::deletefile(AFD *run){
string name;
UFD *delf,*temp;
AFD *delf2,*temp2;
printfile();
cout<<"输入要删除文件名:";
cin>>name;getchar();
delf=findfile1(name);
if(!delf)cout<<"不存在该文件"<<endl;
else{ //删除UFD文件
temp=delf->next;
delf->next=delf->next->next;
delete temp;
delf2=run->findfile2(name);
if(delf2){ //删除AFD中相应文件
temp2=delf2->next;
delf2->next=delf2->next->next;
delete temp2;
}
}
printfile();
}
//返回文件名
string UFD::getfname(){return fname;}
//获得文件保护码
int UFD::getpcode(){return pcode;}
//获得文件长度
int UFD::getlength(){return length;}
//传入AFD
//检查并打开文件
void UFD::openfile(AFD *run){
UFD *openf;
string name;
printfile();
cout<<"输入要打开文件名:";
cin>>name;getchar();
openf=findfile1(name);
if(!openf)cout<<"不存在该文件"<<endl;
else{
if(!run->findfile2(name)){ //要打开文件不在AFD
openf=openf->next; //openf下一文件才是要找文件(见finffile)
AFD *add=new AFD;
add->addfile(run,name,openf->getpcode(),openf->getlength());
}
run->printfile();
}
}
//修改文件长度
void UFD::editfile(int len){length=len;}
//显示用户文件情况
void UFD::printfile(){
UFD *temp;
int i;
cout<<"===============用户文件================"<<endl;
cout<<"NO. 文件名 保护码 文件长度"<<endl;
for(temp=next,i=1;temp;temp=temp->next,i++){
cout<<i<<"\t"
<<temp->getfname()<<"\t"
<<temp->getpcode()<<"\t"
<<temp->getlength()<<endl;
}
}
//关闭打开文件
//保存用户文件
//保存MFD
void UFD::bye(AFD *run){
AFD *temp;
UFD *editf;
temp=run->next;
for(;run->next;temp=run->next){
editf=findfile1(run->next->getfname()); //查找文件
editf->next->editfile(run->next->getlength()); //修改UFD对应文件
run->next=run->next->next; //从AFD中删文件
delete temp;
}
}
//------------------------------------------------------------------------------------
//显示已打开文件情况
void AFD::printfile(){
AFD *temp;
int i;
cout<<"==============已打开文件==============="<<endl;
cout<<"NO. 文件名 保护码 读指针 写指针"<<endl;
for(temp=next,i=1;temp;temp=temp->next,i++){
cout<<i<<"\t"
<<temp->getfname()<<"\t"
<<temp->getpcode()<<"\t"
<<temp->getreadp()<<"\t"
<<temp->getlength()<<endl;
}
}
//查找AFD用户文件
//返回相应文件前一指针
AFD* AFD::findfile2(string fname){
AFD *temp1,*temp2;
for(temp1=this,temp2=temp1->next;temp2;){
if(0==temp2->fname.compare(fname))break; //比较找到文件
temp1=temp2;
temp2=temp2->next;
}
if(!temp2)return NULL; //没找到
else return temp1; //找到返回前一指针
}
//AFD搜索要读文件
//输入读长度并修改读指针
void AFD::readfile(){
string name;
int rlen;
AFD *readf;
printfile();
cout<<"要读文件:";
cin>>name;getchar();
readf=findfile2(name);
if(!readf)cout<<"文件"<<name<<"未打开!"<<endl;
else{
readf=readf->next;
cout<<"读文件"<<name<<"长度:";
cin>>rlen;
readf->editfile(0,rlen); //修改读指针
cout<<"读文件"<<name<<"成功"<<endl;
}
}
//AFD搜索要写文件
//输入写长度并修改写指针
void AFD::writefile(){
string name;
int wlen;
AFD *writef;
printfile();
cout<<"要写文件:";
cin>>name;getchar();
writef=findfile2(name);
if(!writef)cout<<"文件"<<name<<"未打开!"<<endl;
else{
writef=writef->next;
if(writef->getpcode()<2)
cout<<"文件"<<name<<"不能进行写操作!"<<endl;
else{
cout<<"写文件"<<name<<"长度:";
cin>>wlen;
writef->editfile(wlen,0); //修改写指针
cout<<"写文件"<<name<<"成功"<<endl;
}
}
}
//传入用户UFD
//保存并关闭文件
void AFD::closefile(UFD *user){
string name;
AFD *clof,*temp;
printfile();
cout<<"输入要关闭文件:";
cin>>name;getchar();
clof=findfile2(name);
if(clof){ //要关闭文件在AFD中
UFD *editf;
editf=user->findfile1(name);
editf->next->editfile(clof->next->getlength()); //保存
temp=clof->next;
clof->next=clof->next->next; //修改AFD
delete temp;
}
printfile();
}
//传入AFD,打开文件名及长度
//增加文件到AFD
void AFD::addfile(AFD *run,string name,int code,int len){
fname=name;
cout<<"打开文件"<<fname<<"能用 "<<code<<" 以下的保护码"<<endl;
cout<<"输入保护码(1.读 2.写 3.执行):";
for(cin>>pcode;pcode<1||pcode>code;){
cout<<"重新输入保护码(1.读 2.写 3.执行):";
cin>>pcode;
}
length=len;
readp=0;
next=run->next; //作为第一结点插入
run->next=this;
//for(AFD *temp=this;temp->next;temp=temp->next); //表尾
}
//修改文件读写指针
void AFD::editfile(int wlen,int rlen){
length+=wlen;
readp+=rlen;
if(readp>length)readp=length;
}
//返回读指针
int AFD::getreadp(){return readp;}
//------------------------------------------------------------------------------------
void main(){
MFD *mfd=NULL;
mfd=new MFD;
mfd->init();
cout<<"新建成功!"<<endl;
char sel;
string username;
UFD *user=NULL;
AFD *run=new AFD; //头
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
广东工业大学 操作系统 实验四 文件系统 一、实验目的 模拟文件系统实现的基本功能,了解文件系统的基本结构和文件的各种管理方法,加深理解文件系统的内部功能及内部实现。通过用高级语言编写和调试一个简单的文件系统,模拟文件管理的工作过程,从而对各种文件操作命令的实质内容和执行过程有比较深入的了解。 二、实验内容和要求 编程模拟一个简单的文件系统,实现文件系统的管理和控制功能。要求本文件系统采用 两级目录,即设置主文件目录[MFD]和用户文件目录[UED]。另外,为打开文件设置运行文件 目录[AFD]。设计一个10个用户的文件系统,每次用户可保存10个文件,一次运行用户可 以打开5个文件,并对文件必须设置保护措施。在用户程序中通过使用文件系统提供的Create、open、read、write、close、delete等文件命令,对文件进行操作。 …… …… ……
资源推荐
资源详情
资源评论
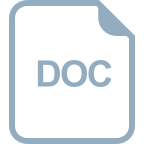
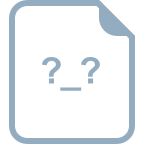
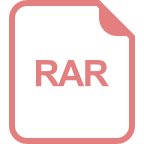
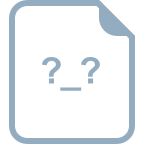
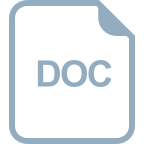
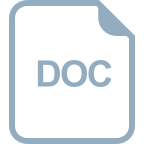
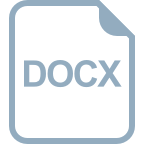
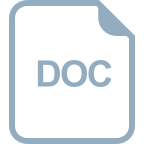
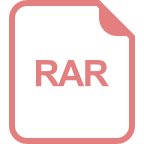
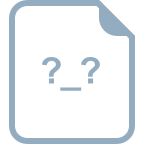
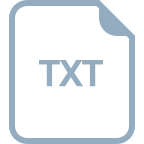
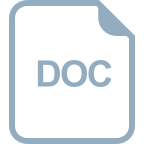
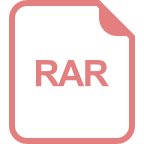
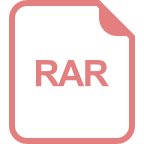
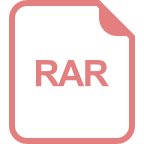
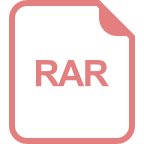
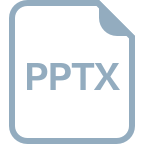
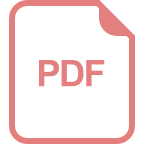
收起资源包目录

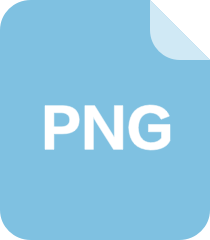
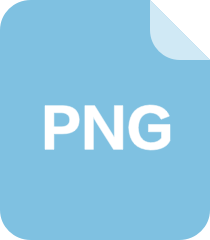
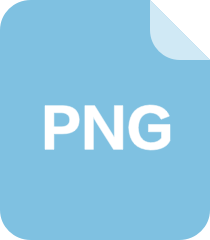
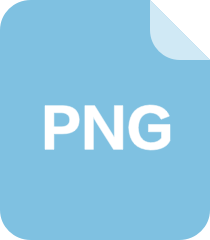
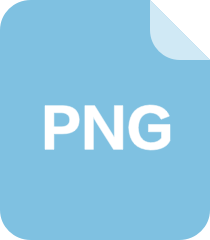
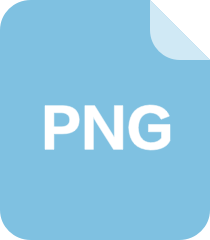
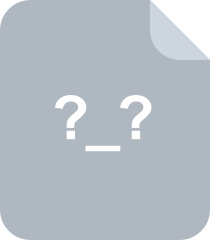
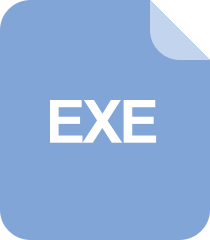
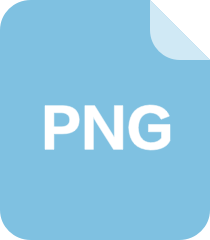
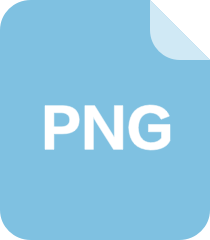
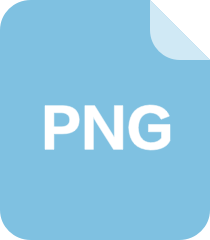
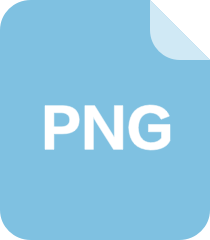
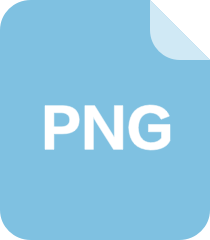
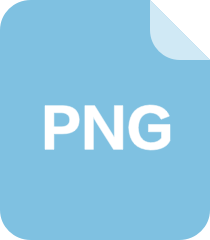
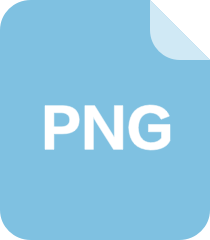
共 15 条
- 1
资源评论
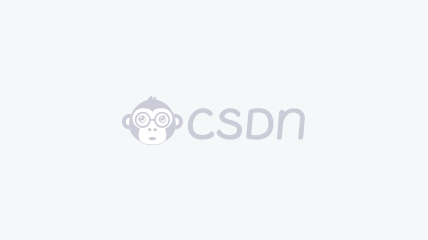
- killuayugi2013-01-09对于我来说很有用,谢谢
- gxmzjn2213142013-05-19很不错!完全符合
- beckylola2014-03-30对我来说没什么用,谢谢

jal960
- 粉丝: 13
- 资源: 78
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

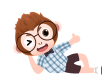
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


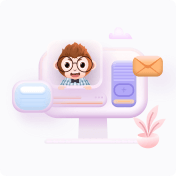
安全验证
文档复制为VIP权益,开通VIP直接复制
