### 快速入门
##### 1.支付整合配置
```java
/**
* 支付类型
* @author egan
* email egzosn@gmail.com
* date 2016/11/20 0:30
*/
public enum PayType implements BasePayType {
aliPay{
/**
* @see com.egzosn.pay.ali.api.AliPayService
* @param apyAccount
* @return
*/
@Override
public PayService getPayService(ApyAccount apyAccount) {
AliPayConfigStorage aliPayConfigStorage = new AliPayConfigStorage();
aliPayConfigStorage.setPid(apyAccount.getPartner());
aliPayConfigStorage.setAppId(apyAccount.getAppid());
aliPayConfigStorage.setKeyPublic(apyAccount.getPublicKey());
aliPayConfigStorage.setKeyPrivate(apyAccount.getPrivateKey());
aliPayConfigStorage.setNotifyUrl(apyAccount.getNotifyUrl());
aliPayConfigStorage.setReturnUrl(apyAccount.getReturnUrl());
aliPayConfigStorage.setSignType(apyAccount.getSignType());
aliPayConfigStorage.setSeller(apyAccount.getSeller());
aliPayConfigStorage.setPayType(apyAccount.getPayType().toString());
aliPayConfigStorage.setMsgType(apyAccount.getMsgType());
aliPayConfigStorage.setInputCharset(apyAccount.getInputCharset());
return new AliPayService(aliPayConfigStorage);
}
@Override
public TransactionType getTransactionType(String transactionType) {
// com.egzosn.pay.ali.bean.AliTransactionType 17年更新的版本,旧版本请自行切换{@link com.egzosn.pay.ali.before.bean.AliTransactionType}
return AliTransactionType.valueOf(transactionType);
}
},wxPay {
@Override
public PayService getPayService(ApyAccount apyAccount) {
WxPayConfigStorage wxPayConfigStorage = new WxPayConfigStorage();
wxPayConfigStorage.setMchId(apyAccount.getPartner());
wxPayConfigStorage.setAppSecret(apyAccount.getPublicKey());
wxPayConfigStorage.setKeyPublic(apyAccount.getPublicKey());
wxPayConfigStorage.setAppId(apyAccount.getAppid());
wxPayConfigStorage.setKeyPrivate(apyAccount.getPrivateKey());
wxPayConfigStorage.setNotifyUrl(apyAccount.getNotifyUrl());
wxPayConfigStorage.setSignType(apyAccount.getSignType());
wxPayConfigStorage.setPayType(apyAccount.getPayType().toString());
wxPayConfigStorage.setMsgType(apyAccount.getMsgType());
wxPayConfigStorage.setInputCharset(apyAccount.getInputCharset());
return new WxPayService(wxPayConfigStorage);
}
/**
* 根据支付类型获取交易类型
* @param transactionType 类型值
* @see com.egzosn.pay.wx.bean.WxTransactionType
* @return
*/
@Override
public TransactionType getTransactionType(String transactionType) {
return WxTransactionType.valueOf(transactionType);
}
},youdianPay {
@Override
public PayService getPayService(ApyAccount apyAccount) {
// TODO 2017/1/23 14:12 author: egan 集群的话,友店可能会有bug。暂未测试集群环境
WxYouDianPayConfigStorage wxPayConfigStorage = new WxYouDianPayConfigStorage();
wxPayConfigStorage.setKeyPrivate(apyAccount.getPrivateKey());
wxPayConfigStorage.setKeyPublic(apyAccount.getPublicKey());
// wxPayConfigStorage.setNotifyUrl(apyAccount.getNotifyUrl());
// wxPayConfigStorage.setReturnUrl(apyAccount.getReturnUrl());
wxPayConfigStorage.setSignType(apyAccount.getSignType());
wxPayConfigStorage.setPayType(apyAccount.getPayType().toString());
wxPayConfigStorage.setMsgType(apyAccount.getMsgType());
wxPayConfigStorage.setSeller(apyAccount.getSeller());
wxPayConfigStorage.setInputCharset(apyAccount.getInputCharset());
return new WxYouDianPayService(wxPayConfigStorage);
}
/**
* 根据支付类型获取交易类型
* @param transactionType 类型值
* @see com.egzosn.pay.wx.youdian.bean.YoudianTransactionType
* @return
*/
@Override
public TransactionType getTransactionType(String transactionType) {
return YoudianTransactionType.valueOf(transactionType);
}
};
public abstract PayService getPayService(ApyAccount apyAccount);
}
/**
* 支付响应对象
* @author: egan
* email egzosn@gmail.com
* date 2016/11/18 0:34
*/
public class PayResponse {
@Resource
private AutowireCapableBeanFactory spring;
private PayConfigStorage storage;
private PayService service;
private PayMessageRouter router;
public PayResponse() {
}
/**
* 初始化支付配置
* @param apyAccount 账户信息
* @see ApyAccount 对应表结构详情--》 /pay-java-demo/resources/apy_account.sql
*/
public void init(ApyAccount apyAccount) {
//根据不同的账户类型 初始化支付配置
this.service = apyAccount.getPayType().getPayService(apyAccount);
this.storage = service.getPayConfigStorage();
//这里设置代理配置
// service.setRequestTemplateConfigStorage(getHttpConfigStorage());
buildRouter(apyAccount.getPayId());
}
/**
* 获取http配置,如果配置为null则为默认配置,无代理,无证书的请求方式。
* 此处非必需
* @param apyAccount 账户信息
* @return 请求配置
*/
public HttpConfigStorage getHttpConfigStorage(ApyAccount apyAccount){
HttpConfigStorage httpConfigStorage = new HttpConfigStorage();
/*
//http代理地址
httpConfigStorage.setHttpProxyHost("192.168.1.69");
//代理端口
httpConfigStorage.setHttpProxyPort(3308);
//代理用户名
httpConfigStorage.setAuthUsername("user");
//代理密码
httpConfigStorage.setAuthPassword("password");
*/
//设置ssl证书路径
httpConfigStorage.setKeystore(apyAccount.getKeystorePath());
//设置ssl证书对应的密码
httpConfigStorage.setStorePassword(apyAccount.getStorePassword());
return httpConfigStorage;
}
/**
* 配置路由
* @param payId 指定账户id,用户多微信支付多支付宝支付
*/
private void buildRouter(Integer payId) {
router = new PayMessageRouter(this.service);
router
.rule()
.msgType(MsgType.text.name()) //消息类型
.payType(PayType.aliPay.name()) //支付账户事件类型
.transactionType(AliTransactionType.UNAWARE.name())//交易类型,有关回调的可在这处理
.interceptor(new AliPayMessageInterceptor(payId)) //拦截器
.handler(autowire(new AliPayMessageHandler(payId))) //处理器
.end()
.rule()
.msgType(MsgType.xml.name())
.payType(PayType.wxPay.name())
.handler(autowire(new WxPayMessageHandler(payId)))
.end()
.rule()
.msgType(MsgType.json.name())
.payType(PayType.youdianPay.name())
.handler(autowire(new YouDianPayMessageHandler(payId)))
.end()
;
}
private PayMessageHandler autowire(PayMessageHandler handler) {
spring.autowireBean(handler);
return handler;
}
public PayConfigStorage getStorage() {
return storage;
}
public PayService getService() {
return service;
}
public PayMessageRouter ge
没有合适的资源?快使用搜索试试~ 我知道了~
全能第三方支付对接Java开发工具包优雅的轻量级支付模块集成支付对接支付整合
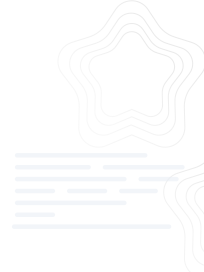
共323个文件
java:277个
xml:16个
md:11个

需积分: 5 0 下载量 52 浏览量
2024-04-08
10:45:37
上传
评论
收藏 1.02MB ZIP 举报
温馨提示
java资源。全能第三方支付对接Java开发工具包.优雅的轻量级支付模块集成支付对接支付整合(微信,支付宝,银联,友店,富友,跨境支付paypal,payoneer(P卡派安盈)易极付)app,扫码,网页支付刷卡付条码付刷脸付转账红包服务商模式,微信分账,合并支付、支持多种支付类型多支付账户,支付与业务完全剥离,简单几行代码即可实现支付。全能第三方支付对接Java开发工具包.优雅的轻量级支付模块集成支付对接支付整合(微信,支付宝,银联,友店,富友,跨境支付paypal,payoneer(P卡派安盈)易极付)app,扫码,网页支付刷卡付条码付刷脸付转账红包服务商模式,微信分账,合并支付、支持多种支付类型多支付账户,支付与业务完全剥离,简单几行代码即可实现支付,简单快速完成支付模块的开发,可轻松嵌入到任何系统里 目前仅是一个开发工具包(即SDK),只提供简单Web实现,建议使用maven或gradle引用本项目即可使用本SDK提供的各种支付相关的功能依赖任何 mvc 框架,依赖极少:httpclient,fastjson,log4j,com.google.zxing,项目精简,不用担心项目
资源推荐
资源详情
资源评论
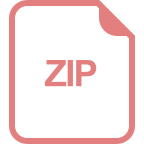
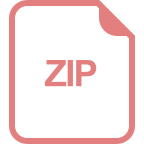
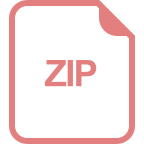
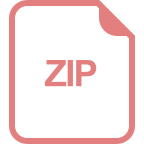
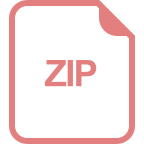
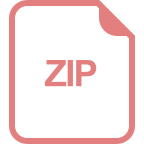
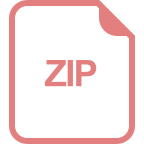
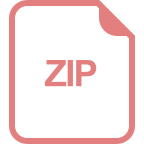
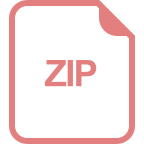
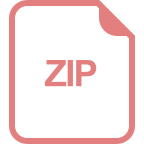
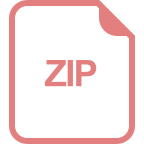
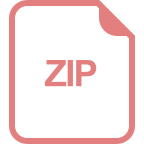
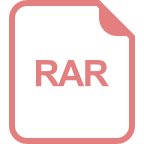
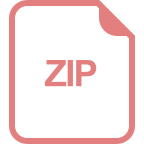
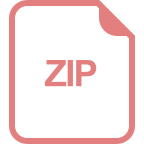
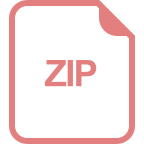
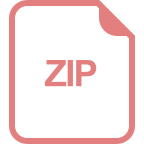
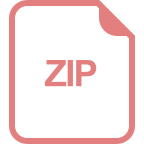
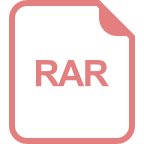
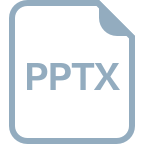
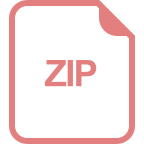
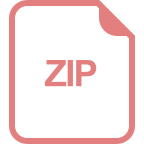
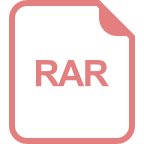
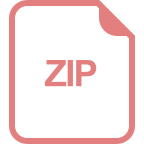
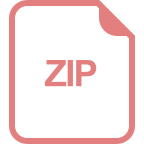
收起资源包目录

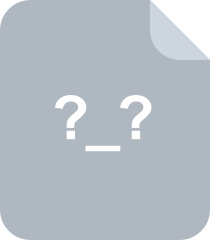
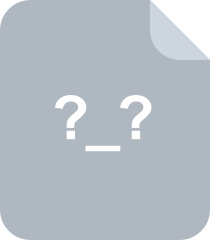
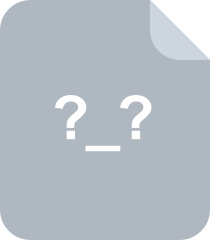
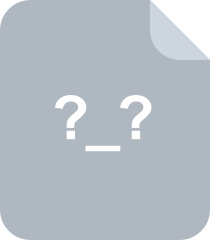
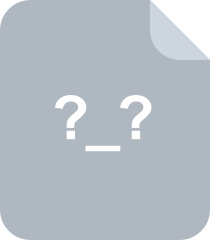
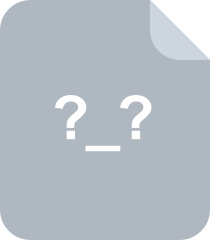
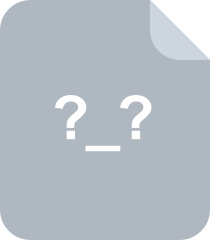
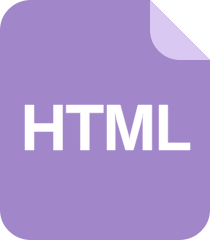
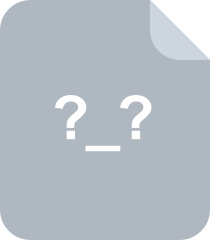
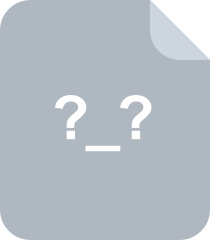
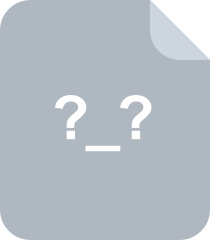
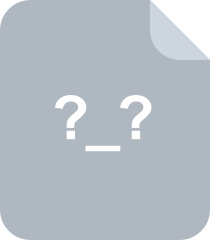
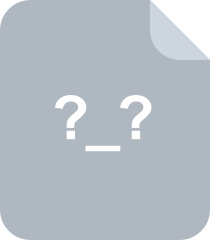
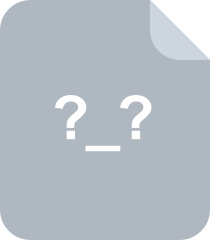
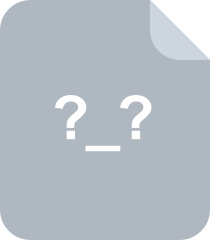
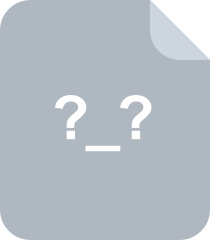
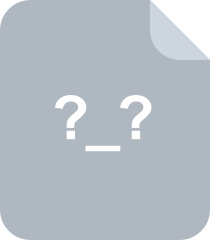
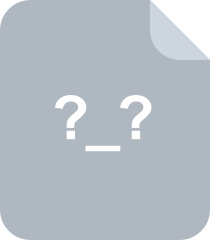
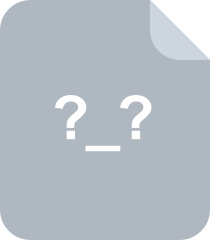
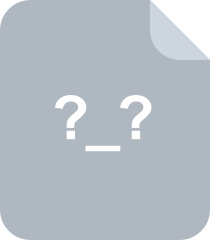
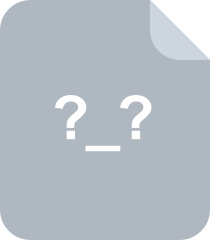
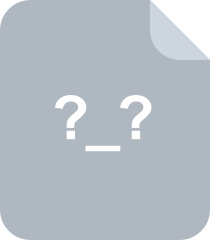
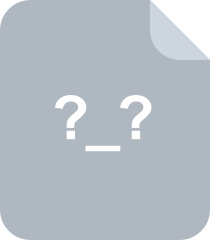
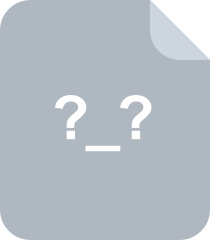
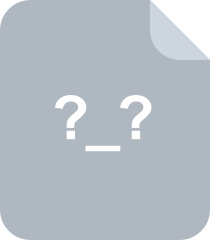
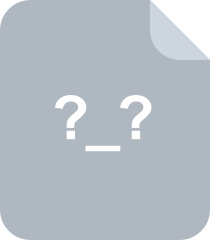
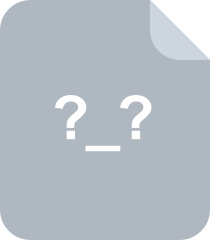
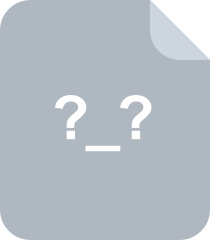
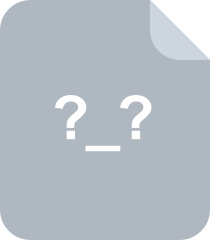
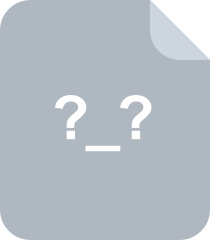
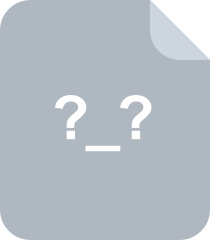
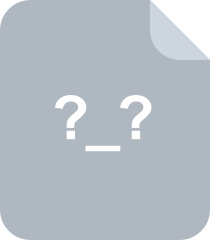
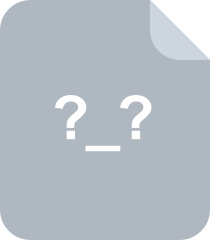
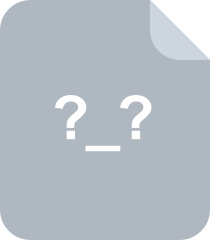
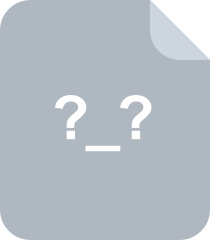
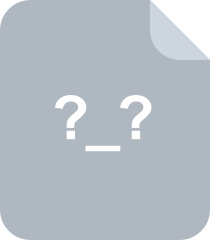
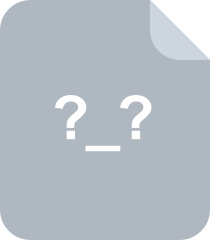
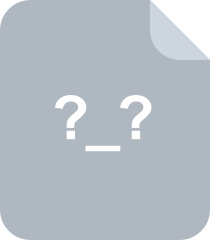
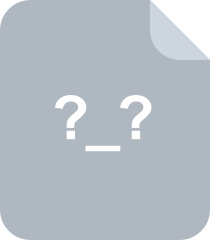
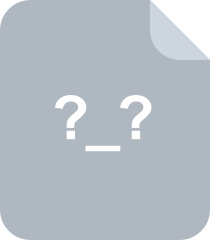
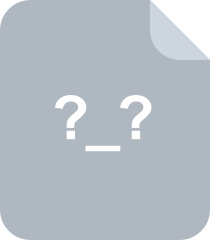
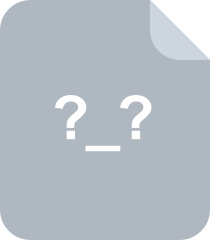
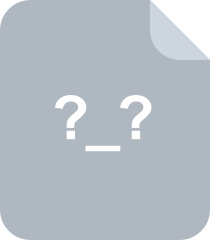
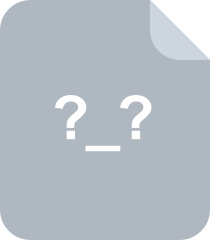
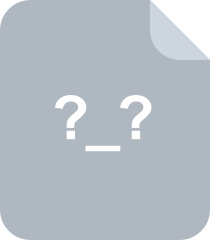
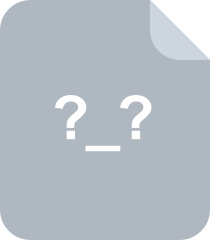
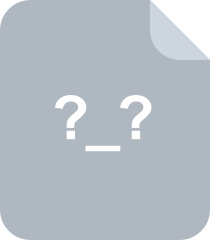
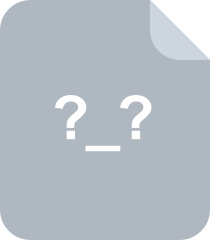
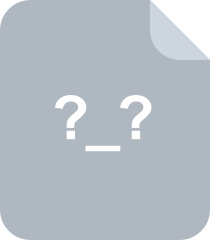
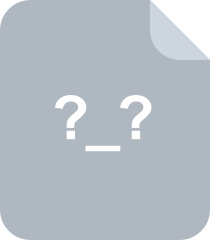
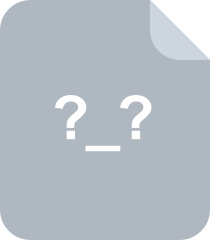
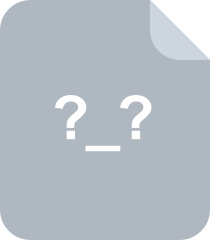
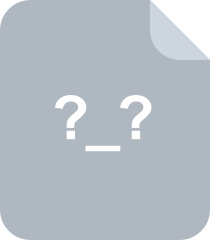
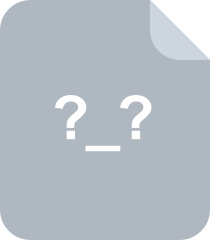
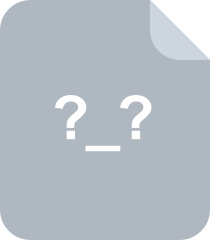
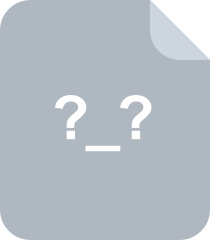
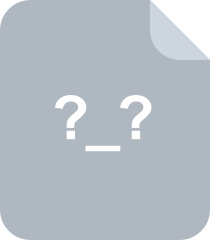
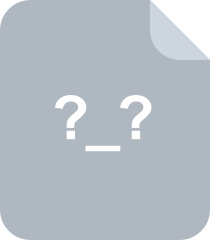
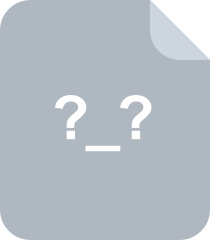
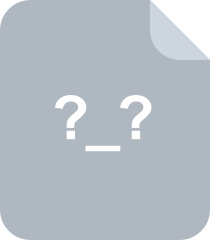
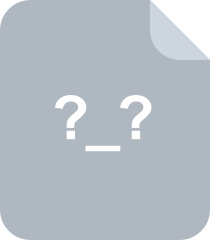
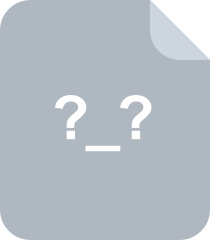
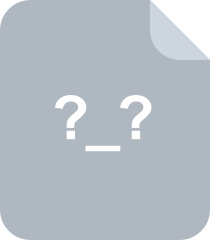
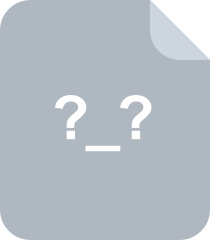
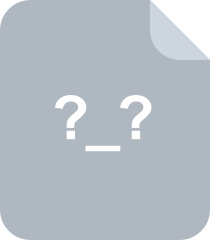
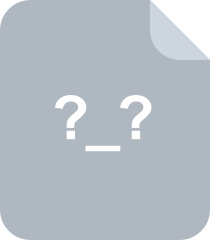
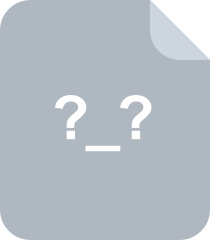
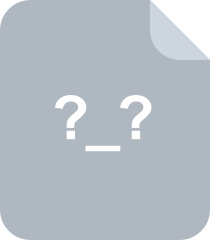
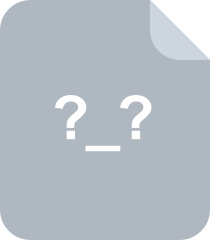
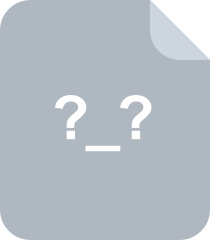
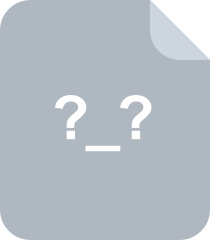
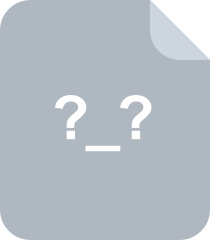
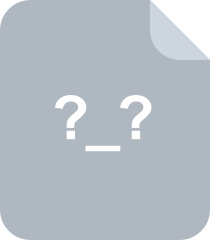
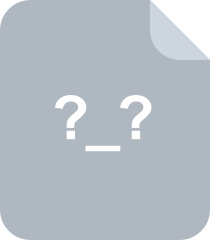
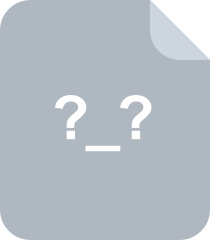
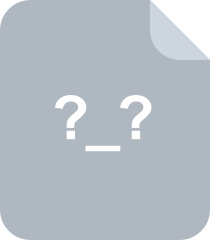
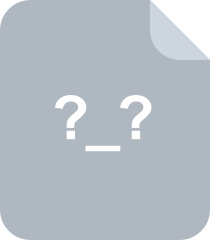
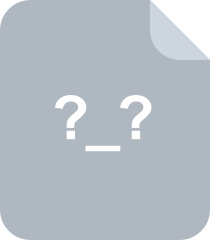
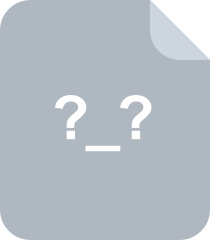
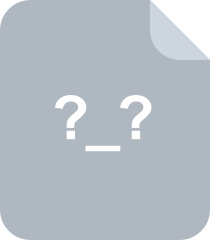
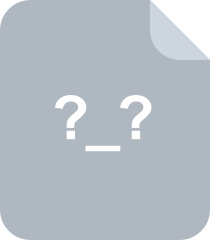
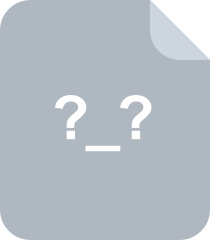
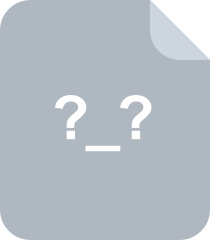
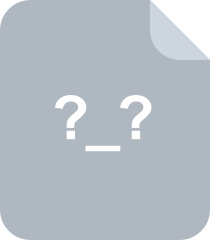
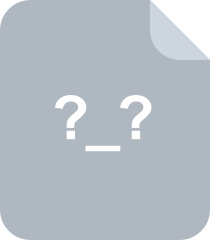
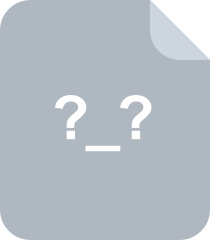
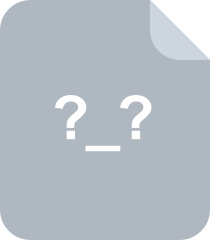
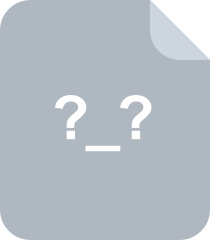
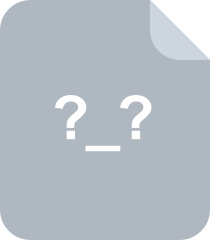
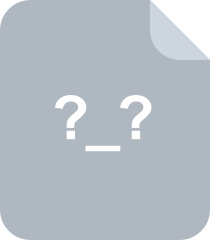
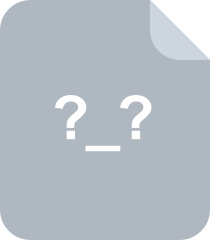
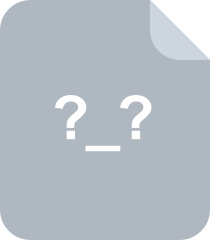
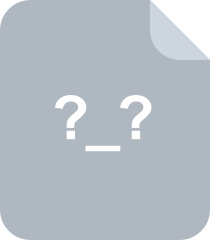
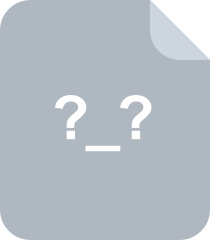
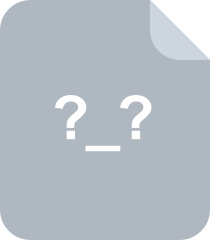
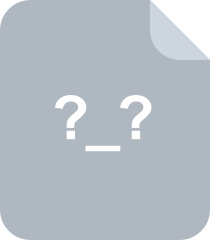
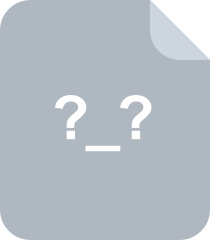
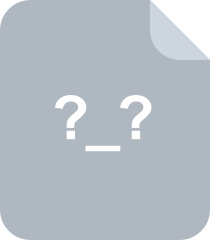
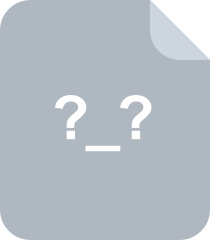
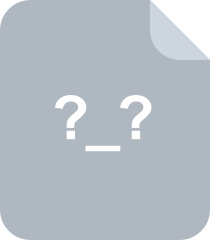
共 323 条
- 1
- 2
- 3
- 4
资源评论
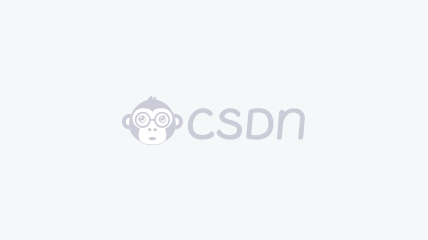

传奇开心果编程
- 粉丝: 1w+
- 资源: 454
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

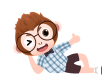
最新资源
- 基于java的三国之家网站设计与实现.docx
- 基于java的图书管理系统V2设计与实现.docx
- 基于java的宿舍管理系统设计与实现.docx
- 基于java的停车场管理系统设计与实现.docx
- 基于java的图书管理系统V3设计与实现.docx
- 基于java的乡村养老服务管理系统设计与实现.docx
- 基于java的图书管理系统设计与实现.docx
- 基于java的项目申报系统设计与实现.docx
- 基于java的校车调度管理系统设计与实现.docx
- 基于java的校园便利平台设计与实现.docx
- 基于java的校园闲置物品交易系统设计与实现.docx
- 基于java的校园一卡通设计与实现.docx
- 基于java的协同过滤电影推荐系统设计与实现.docx
- 基于java的学院个人信息管理系统设计与实现.docx
- 基于java的医院病历管理系统设计与实现.docx
- 基于java的智慧养老中心管理系统设计与实现.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


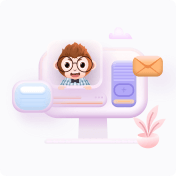
安全验证
文档复制为VIP权益,开通VIP直接复制
