import pygame, sys, easygui, random, time
pygame.init()
screen = pygame.display.set_mode((1200, 650))
pygame.display.set_caption("智能垃圾分类系统")
start = pygame.image.load('./images/start.jpg')
total = pygame.image.load('./images/total.png')
kitchen_detail = pygame.image.load('./images/kitchen_detail.png')
other_detail = pygame.image.load('./images/other_detail.png')
recyclable_detail = pygame.image.load('./images/recyclable_detail.png')
harmful_detail = pygame.image.load('./images/harmful_detail.png')
bg = pygame.image.load('./images/bg.jpeg')
rabbits = pygame.image.load('./images/rabbit.png')
mouse = pygame.image.load('./images/mouse.png')
bottle = pygame.image.load('./images/矿泉水瓶.png')
cabbage = pygame.image.load('./images/白菜叶.png')
eggs = pygame.image.load('./images/蛋壳.png')
battery = pygame.image.load('./images/废电池.png')
mirror = pygame.image.load('./images/镜子.png')
paper = pygame.image.load('./images/卷纸.png')
ceramics = pygame.image.load('./images/陶瓷.png')
thermometer = pygame.image.load('./images/温度计.png')
watermelon = pygame.image.load('./images/西瓜皮.png')
paintBucket = pygame.image.load('./images/油漆桶.png')
umbrella = pygame.image.load('./images/雨伞.png')
clothing = pygame.image.load('./images/旧衣服.png')
harmfulWaster = pygame.image.load('./images/有害.png')
recyclableWaster = pygame.image.load('./images/可回收.png')
otherWaster = pygame.image.load('./images/其他.png')
kitchenWaster = pygame.image.load('./images/厨余.png')
endScore = pygame.image.load('./images/endScore.png')
endBg = pygame.image.load('./images/endBg.jpeg')
#页面标识、是否首次进入标识、4个详细页面碎片获取标识列表、游戏准备标识、垃圾列表、本局展示垃圾列表、本局展示垃圾桶列表、鼠标的x与y、游戏难度列表、选择的游戏难度
global page, flag, flaglist, ready, wasterlist, showrubbishlist, showCanlist, x, y, difficulty, level
# 页面标识
page = 'start'
# 首次进入标识
flag = True
#游戏难度
level = None
# 碎片获取标识列表
flaglist = [False, False, False, False]
# 垃圾列表
wasterlist = [{'img': bottle, 'type': '可回收'}, {'img': clothing, 'type': '可回收'}, {'img': cabbage, 'type': '厨余'},
{'img': eggs, 'type': '厨余'}, {'img': battery, 'type': '有害'}, {'img': mirror, 'type': '可回收'},
{'img': paper, 'type': '可回收'},
{'img': ceramics, 'type': '其他'}, {'img': thermometer, 'type': '有害'},
{'img': watermelon, 'type': '厨余'},
{'img': paintBucket, 'type': '有害'}, {'img': umbrella, 'type': '其他'}]
# 垃圾桶列表
canlist = [{'img': harmfulWaster, 'type': '有害', 'x': 100, 'y': 410},
{'img': recyclableWaster, 'type': '可回收', 'x': 380, 'y': 410},
{'img': otherWaster, 'type': '其他', 'x': 650, 'y': 410},
{'img': kitchenWaster, 'type': '厨余', 'x': 910, 'y': 410}]
# 准备标识
ready = False
showrubbishlist = []
showCanlist = []
difficulty = [{'难度': '简单', '数量': 4, '间隔时间': 1}, {'难度': '困难', '数量': 8, '间隔时间': 0.5},
{'难度': '地狱', '数量': 12, '间隔时间': 0.2}]
# 写文本函数
def drawText(text, pos, size, color=(0, 0, 0)):
font = pygame.font.Font('./fonts/WRYH.ttf', size)
newtext = font.render(text, True, color)
screen.blit(newtext, pos)
# 碰撞检测
def bang(obj1, obj2):
mask1 = pygame.mask.from_surface(obj1.img)
mask2 = pygame.mask.from_surface(obj2.img)
offset = (obj1.x - obj2.x, obj1.y - obj2.y)
result = mask1.overlap(mask2, offset)
return result
# 创造垃圾类
class Rubbish():
def __init__(self, img, x, y, type):
self.img = img
self.x = x
self.y = y
self.type = type
self.lastTime = 0
def move(self):
if not isActionTime(self.lastTime):
return
self.lastTime = time.time()
self.y = self.y + 10
class Can():
def __init__(self, img, x, y, type):
self.img = img
self.x = x
self.y = y
self.type = type
class Game():
def __init__(self, level='', num=4, time=1, score=0):
self.level = level
self.num = num
self.time = time
self.score = score
game = Game()
for i in canlist:
can = Can(i['img'], i['x'], i['y'], i['type'])
showCanlist.append(can)
# 添加时间间隔的方法
def isActionTime(lastTime):
if lastTime == 0:
return True
currentTime = time.time()
return currentTime - lastTime >= game.time
def handleEvent():
global ready, page, flag, index, x, y, lastTime, again, showrubbishlist, level, difficulty, game
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
sys.exit()
if event.type == pygame.MOUSEBUTTONDOWN:
x, y = event.pos
print('鼠标按下的位置:', x, y)
if page == 'start':
page = "introduce"
elif page == 'introduce':
if flag:
easygui.msgbox('集齐四项垃圾分类知识碎片,可解锁隐藏挑战','系统提示')
if (45 <= x <= 580) and (17 <= y <= 310):
page = 'kitchenWasterDetail'
elif (610 <= x <= 1140) and (15 <= y <= 310):
page = 'otherWasterDetail'
elif (45 <= x <= 580) and (330 <= y <= 630):
page = 'recyclableWasterDetail'
elif (610 < x < 1140) and (330 <= y <= 630):
page = 'harmfulWasterDetail'
elif page == 'kitchenWasterDetail' or page == 'otherWasterDetail' or page == 'recyclableWasterDetail' or page == 'harmfulWasterDetail':
page = 'introduce'
flag = False
if flaglist[0] and flaglist[1] and flaglist[2] and flaglist[3]:
easygui.msgbox('恭喜你已全部集齐,开启隐藏挑战','系统提示')
page = 'game'
elif page == 'game':
if not (ready and level):
easygui.msgbox('通过 a s d 移动垃圾到对应的垃圾桶进行分类', '游戏规则')
easygui.msgbox('分类成功加10分,分类错误扣5分,未分类扣1分', '游戏规则')
level = easygui.buttonbox("请选择你的游戏难度", "系统提醒",
choices=[difficulty[0]['难度'], difficulty[1]['难度'],
difficulty[2]['难度']])
if level:
ready = easygui.ynbox("游戏马上开始,是否准备好了呢?", '系统提示')
for i in difficulty:
if i['难度'] == level:
game = Game(level, i['数量'], i['间隔时间'])
if ready:
print(level, game.level)
easygui.msgbox('3', '系统提示')
easygui.msgbox('2', '系统提示')
easygui.msgbox('1', '系统提示')
easygui.msgbox('游戏开始', '系统提示')
rubbishes = random.sample(wasterlist, game.num)
showrubbishlist = []
x = 180
y = 50
lastTime = 0
index = 0
again = False
for i in rubbishes:
x = random.randint(20, 1000)
没有合适的资源?快使用搜索试试~ 我知道了~
python项目 智能垃圾分类系统
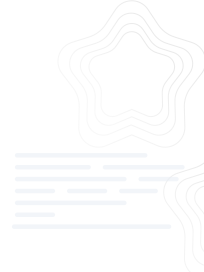
共49个文件
png:29个
xml:4个
ttf:3个

需积分: 5 0 下载量 114 浏览量
2024-11-24
10:57:56
上传
评论
收藏 27.91MB RAR 举报
温馨提示
学习了解垃圾分类、四种不同的模块进行垃圾分类知识的学习,收集垃圾知识碎片,集齐4个垃圾知识碎片后,解锁隐藏游戏挑战。类似俄罗斯方块,通过asd按键来控制垃圾进行移动,将垃圾移动到正确的垃圾箱内,分类成功获得加分,分类失败,扣分! 最后游戏结束,展示分数。最终升华主题,人人做好垃圾分类,保护环境。
资源推荐
资源详情
资源评论
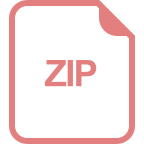
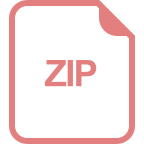
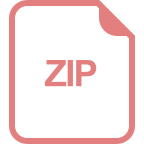
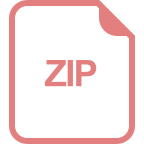
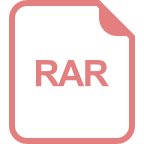
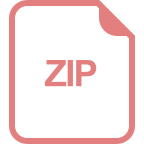
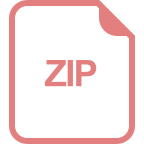
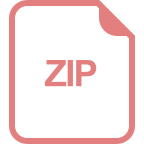
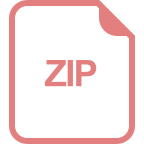
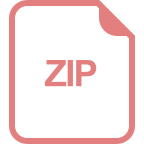
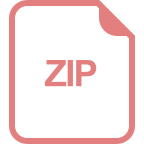
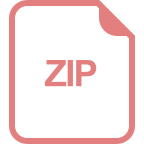
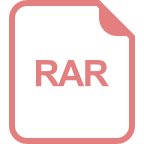
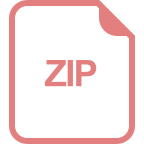
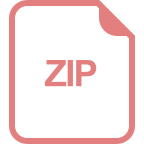
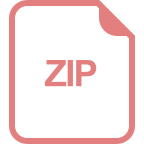
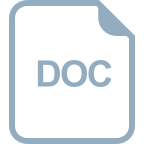
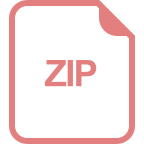
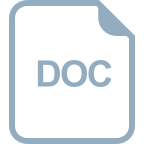
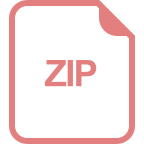
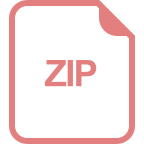
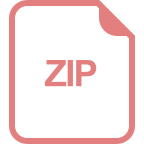
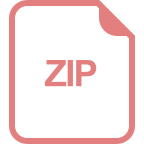
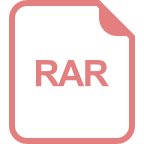
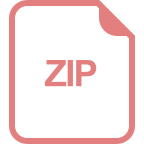
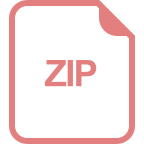
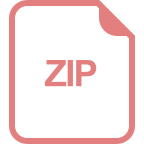
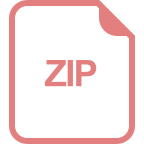
收起资源包目录


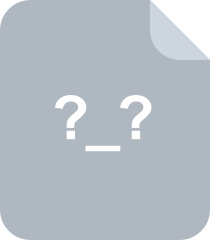

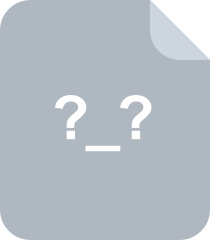
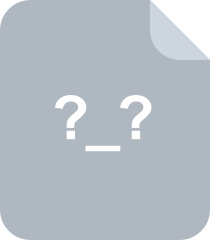

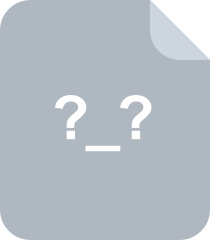
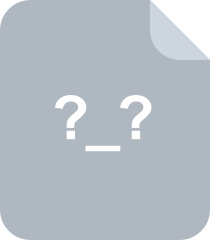
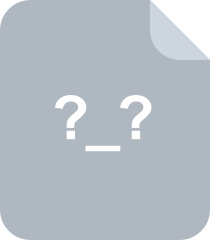
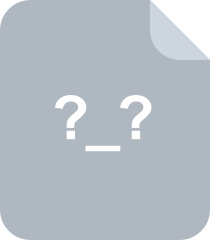
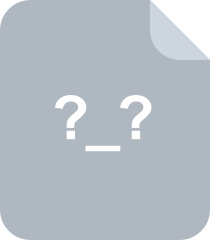

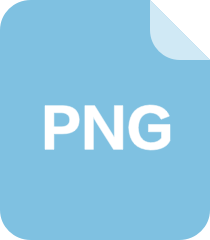
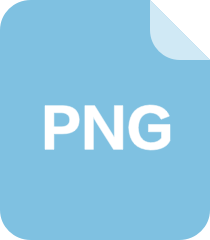
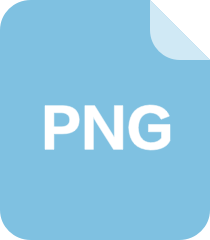
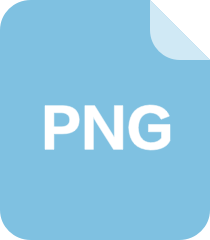
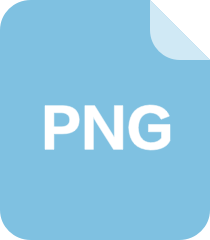
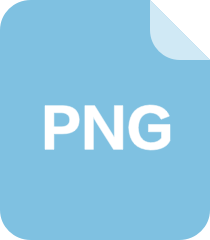
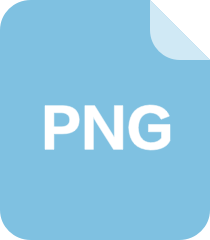
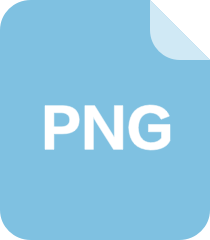
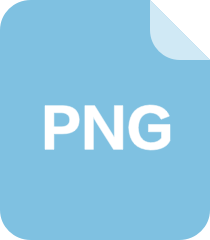
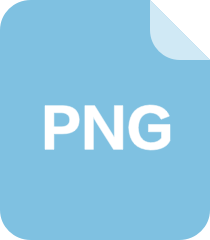
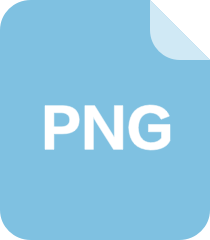
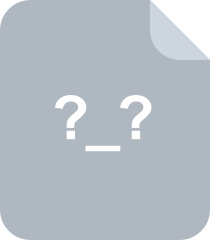
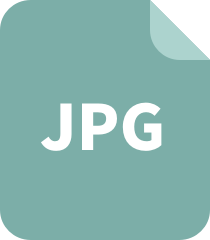
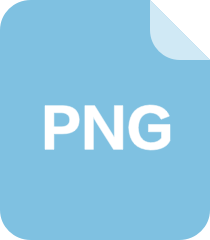
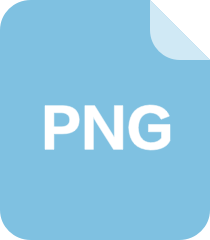
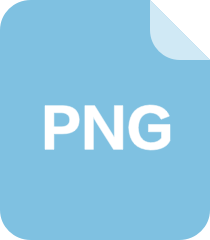
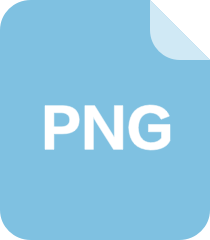
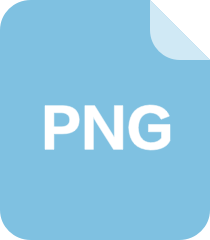
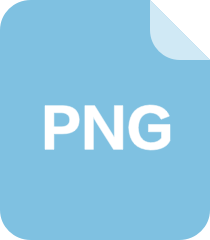
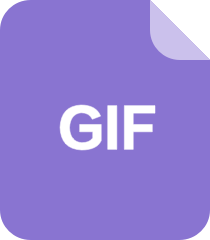
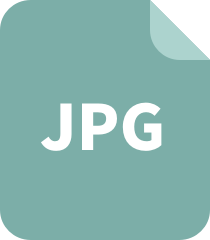
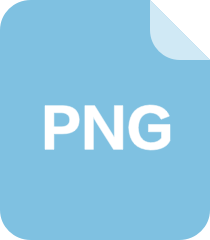
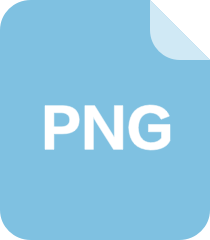
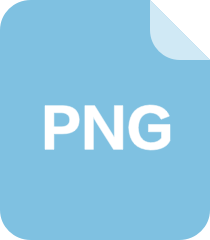
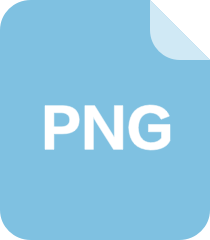
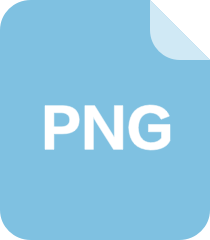
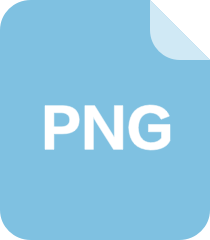
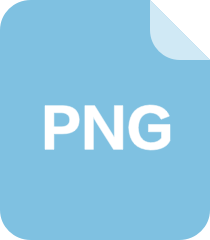
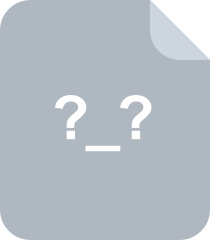
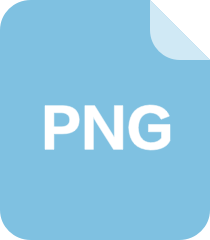
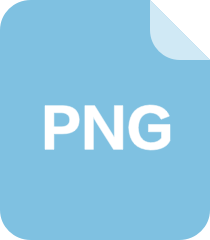
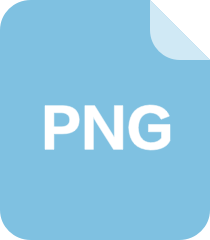
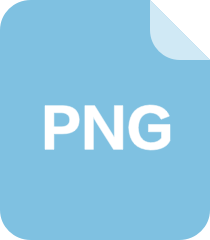
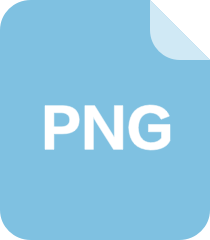


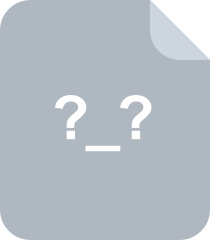
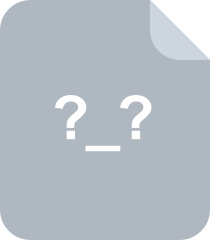
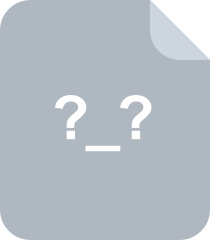
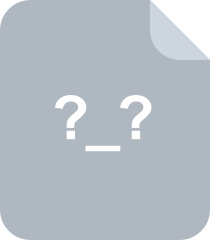

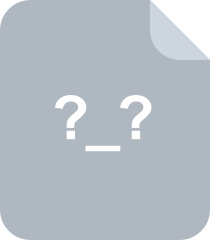
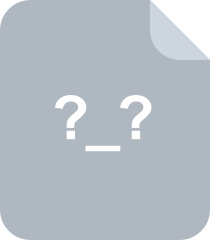
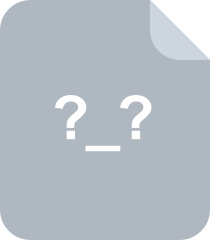
共 49 条
- 1
资源评论
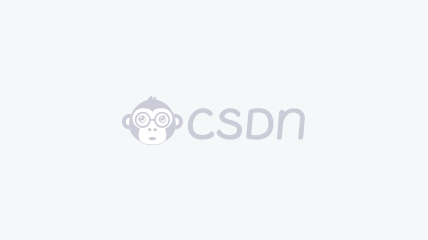

我要当前端工程师
- 粉丝: 4050
- 资源: 15
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

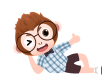
最新资源
- 肝病检测31-CreateML、Paligemma数据集合集.rar
- 2024年最新Redis基础操作与性能调优指南
- 网页昵称检测39-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- xManagementWebApi 测试程序
- 2024年Java开发人员必备常用操作速查指南
- IMG_20241218_130909.jpg
- 网页内容检测49-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 基于Python的文件加密与解密实现方案
- JS使用random随机数实现简单的四则算数验证
- Unity体积雾材质包
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


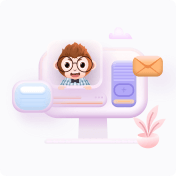
安全验证
文档复制为VIP权益,开通VIP直接复制
