#import <Foundation/Foundation.h>
#import "sqlite3.h"
#import "FMResultSet.h"
#import "FMDatabasePool.h"
#if ! __has_feature(objc_arc)
#define FMDBAutorelease(__v) ([__v autorelease]);
#define FMDBReturnAutoreleased FMDBAutorelease
#define FMDBRetain(__v) ([__v retain]);
#define FMDBReturnRetained FMDBRetain
#define FMDBRelease(__v) ([__v release]);
#define FMDBDispatchQueueRelease(__v) (dispatch_release(__v));
#else
// -fobjc-arc
#define FMDBAutorelease(__v)
#define FMDBReturnAutoreleased(__v) (__v)
#define FMDBRetain(__v)
#define FMDBReturnRetained(__v) (__v)
#define FMDBRelease(__v)
// If OS_OBJECT_USE_OBJC=1, then the dispatch objects will be treated like ObjC objects
// and will participate in ARC.
// See the section on "Dispatch Queues and Automatic Reference Counting" in "Grand Central Dispatch (GCD) Reference" for details.
#if OS_OBJECT_USE_OBJC
#define FMDBDispatchQueueRelease(__v)
#else
#define FMDBDispatchQueueRelease(__v) (dispatch_release(__v));
#endif
#endif
#if !__has_feature(objc_instancetype)
#define instancetype id
#endif
typedef int(^FMDBExecuteStatementsCallbackBlock)(NSDictionary *resultsDictionary);
/** A SQLite ([http://sqlite.org/](http://sqlite.org/)) Objective-C wrapper.
### Usage
The three main classes in FMDB are:
- `FMDatabase` - Represents a single SQLite database. Used for executing SQL statements.
- `<FMResultSet>` - Represents the results of executing a query on an `FMDatabase`.
- `<FMDatabaseQueue>` - If you want to perform queries and updates on multiple threads, you'll want to use this class.
### See also
- `<FMDatabasePool>` - A pool of `FMDatabase` objects.
- `<FMStatement>` - A wrapper for `sqlite_stmt`.
### External links
- [FMDB on GitHub](https://github.com/ccgus/fmdb) including introductory documentation
- [SQLite web site](http://sqlite.org/)
- [FMDB mailing list](http://groups.google.com/group/fmdb)
- [SQLite FAQ](http://www.sqlite.org/faq.html)
@warning Do not instantiate a single `FMDatabase` object and use it across multiple threads. Instead, use `<FMDatabaseQueue>`.
*/
#pragma clang diagnostic push
#pragma clang diagnostic ignored "-Wobjc-interface-ivars"
@interface FMDatabase : NSObject {
sqlite3* _db;
NSString* _databasePath;
BOOL _logsErrors;
BOOL _crashOnErrors;
BOOL _traceExecution;
BOOL _checkedOut;
BOOL _shouldCacheStatements;
BOOL _isExecutingStatement;
BOOL _inTransaction;
NSTimeInterval _maxBusyRetryTimeInterval;
NSTimeInterval _startBusyRetryTime;
NSMutableDictionary *_cachedStatements;
NSMutableSet *_openResultSets;
NSMutableSet *_openFunctions;
NSDateFormatter *_dateFormat;
}
///-----------------
/// @name Properties
///-----------------
/** Whether should trace execution */
@property (atomic, assign) BOOL traceExecution;
/** Whether checked out or not */
@property (atomic, assign) BOOL checkedOut;
/** Crash on errors */
@property (atomic, assign) BOOL crashOnErrors;
/** Logs errors */
@property (atomic, assign) BOOL logsErrors;
/** Dictionary of cached statements */
@property (atomic, retain) NSMutableDictionary *cachedStatements;
///---------------------
/// @name Initialization
///---------------------
/** Create a `FMDatabase` object.
An `FMDatabase` is created with a path to a SQLite database file. This path can be one of these three:
1. A file system path. The file does not have to exist on disk. If it does not exist, it is created for you.
2. An empty string (`@""`). An empty database is created at a temporary location. This database is deleted with the `FMDatabase` connection is closed.
3. `nil`. An in-memory database is created. This database will be destroyed with the `FMDatabase` connection is closed.
For example, to create/open a database in your Mac OS X `tmp` folder:
FMDatabase *db = [FMDatabase databaseWithPath:@"/tmp/tmp.db"];
Or, in iOS, you might open a database in the app's `Documents` directory:
NSString *docsPath = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES)[0];
NSString *dbPath = [docsPath stringByAppendingPathComponent:@"test.db"];
FMDatabase *db = [FMDatabase databaseWithPath:dbPath];
(For more information on temporary and in-memory databases, read the sqlite documentation on the subject: [http://www.sqlite.org/inmemorydb.html](http://www.sqlite.org/inmemorydb.html))
@param inPath Path of database file
@return `FMDatabase` object if successful; `nil` if failure.
*/
+ (instancetype)databaseWithPath:(NSString*)inPath;
/** Initialize a `FMDatabase` object.
An `FMDatabase` is created with a path to a SQLite database file. This path can be one of these three:
1. A file system path. The file does not have to exist on disk. If it does not exist, it is created for you.
2. An empty string (`@""`). An empty database is created at a temporary location. This database is deleted with the `FMDatabase` connection is closed.
3. `nil`. An in-memory database is created. This database will be destroyed with the `FMDatabase` connection is closed.
For example, to create/open a database in your Mac OS X `tmp` folder:
FMDatabase *db = [FMDatabase databaseWithPath:@"/tmp/tmp.db"];
Or, in iOS, you might open a database in the app's `Documents` directory:
NSString *docsPath = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES)[0];
NSString *dbPath = [docsPath stringByAppendingPathComponent:@"test.db"];
FMDatabase *db = [FMDatabase databaseWithPath:dbPath];
(For more information on temporary and in-memory databases, read the sqlite documentation on the subject: [http://www.sqlite.org/inmemorydb.html](http://www.sqlite.org/inmemorydb.html))
@param inPath Path of database file
@return `FMDatabase` object if successful; `nil` if failure.
*/
- (instancetype)initWithPath:(NSString*)inPath;
///-----------------------------------
/// @name Opening and closing database
///-----------------------------------
/** Opening a new database connection
The database is opened for reading and writing, and is created if it does not already exist.
@return `YES` if successful, `NO` on error.
@see [sqlite3_open()](http://sqlite.org/c3ref/open.html)
@see openWithFlags:
@see close
*/
- (BOOL)open;
/** Opening a new database connection with flags and an optional virtual file system (VFS)
@param flags one of the following three values, optionally combined with the `SQLITE_OPEN_NOMUTEX`, `SQLITE_OPEN_FULLMUTEX`, `SQLITE_OPEN_SHAREDCACHE`, `SQLITE_OPEN_PRIVATECACHE`, and/or `SQLITE_OPEN_URI` flags:
`SQLITE_OPEN_READONLY`
The database is opened in read-only mode. If the database does not already exist, an error is returned.
`SQLITE_OPEN_READWRITE`
The database is opened for reading and writing if possible, or reading only if the file is write protected by the operating system. In either case the database must already exist, otherwise an error is returned.
`SQLITE_OPEN_READWRITE | SQLITE_OPEN_CREATE`
The database is opened for reading and writing, and is created if it does not already exist. This is the behavior that is always used for `open` method.
If vfs is given the value is passed to the vfs parameter of sqlite3_open_v2.
@return `YES` if successful, `NO` on error.
@see [sqlite3_open_v2()](http://sqlite.org/c3ref/open.html)
@see open
@see close
*/
#if SQLITE_VERSION_NUMBER >= 3005000
- (BOOL)openWithFlags:(int)flags;
- (BOOL)openWithFlags:(int)flags vfs:(NSString *)vfsName;
#endif
/** Closing a database connection
@return `YES` if success, `NO` on error.
@see [sqlite3_close()](http://sqlite.org/c3ref/close.html)
@see open
@see openWithFlags:
IOS数据库操作FMDB简单入门实例及文章讲解
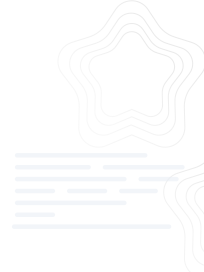

在iOS应用开发中,数据库操作是必不可少的一部分,用于存储和检索数据。FMDB是一个流行的、Objective-C编写的SQLite数据库管理库,它为开发者提供了一种简单易用的方式来与SQLite进行交互。本文将深入探讨FMDB的基本用法和关键概念,帮助你快速上手。 FMDB基于SQLite,一个轻量级、文件级的数据库系统,适用于移动设备和嵌入式系统。SQLite无需服务器进程,可以直接在本地文件系统上运行,非常适合iOS应用。FMDB提供了面向对象的API,使得开发者可以使用Objective-C的语法来执行SQL语句和管理数据库。 **安装FMDB** 在你的Xcode项目中,你可以通过CocoaPods或者Carthage来添加FMDB依赖。如果你选择CocoaPods,只需在Podfile中添加`pod 'FMDB'`,然后运行`pod install`。对于Carthage,你则需要在Cartfile中写入`github "ccgus/fmdb"`,并执行`carthage update`。 **初始化数据库** 在使用FMDB之前,你需要创建一个`FMDatabase`对象,这代表了一个SQLite数据库连接。你可以使用`[FMDatabase databaseWithPath:]`方法指定数据库文件路径来初始化数据库。如果数据库文件不存在,FMDB会尝试创建它。 ```objc NSString *databasePath = [NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES) objectAtIndex:0]; databasePath = [databasePath stringByAppendingPathComponent:@"myDatabase.sqlite"]; FMDatabase *db = [FMDatabase databaseWithPath:databasePath]; ``` **打开和关闭数据库** 在开始操作数据库前,需要调用`-open`方法打开数据库。当不再使用时,记得调用`-close`以释放资源。 ```objc if (![db open]) { NSLog(@"Failed to open database"); } else { // 数据库操作 } ``` **执行SQL语句** FMDB提供了`-executeQuery:withArgumentsInArray:`和`-executeUpdate:`方法来执行查询和更新操作。查询结果会被封装在一个`FMResultSet`对象中,你可以遍历这个结果集来获取数据。 ```objc // 执行查询 FMResultSet *rs = [db executeQuery:@"SELECT * FROM myTable"]; while ([rs next]) { // 获取列值 NSString *name = [rs stringForColumn:@"name"]; NSInteger age = [rs intForColumn:@"age"]; NSLog(@"Name: %@, Age: %ld", name, (long)age); } // 执行更新 BOOL success = [db executeUpdate:@"INSERT INTO myTable (name, age) VALUES (?, ?)", @"John", @(25)]; if (!success) { NSLog(@"Insert failed"); } ``` **事务处理** 在FMDB中,你可以使用`beginTransaction`、`commit`和`rollback`方法来处理事务,确保一系列操作的原子性。 ```objc [db beginTransaction]; BOOL success = [db executeUpdate:@"UPDATE myTable SET age = age + 1 WHERE name = ?", @"John"]; if (success) { [db commit]; } else { [db rollback]; } ``` **错误处理** FMDB在执行操作失败时,会自动设置一个错误对象。你可以通过`lastErrorMessage`和`lastErrorCode`属性获取错误信息。 ```objc if (![db executeUpdate:@"INVALID SQL STATEMENT"]) { NSLog(@"Error: %@", db.lastErrorMessage); } ``` FMDB简化了iOS应用中的SQLite操作,使开发者能够更专注于业务逻辑。通过理解和实践这些基本操作,你将能够高效地管理你的应用程序的数据存储。记住,始终确保正确处理数据库的生命周期,避免资源泄露,并确保在执行SQL语句时考虑到安全性和性能。
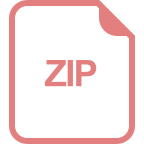
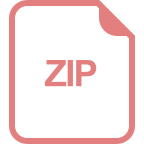
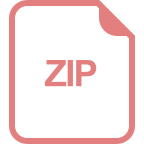
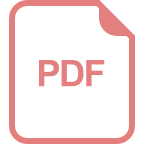
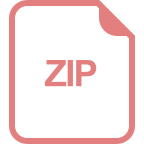
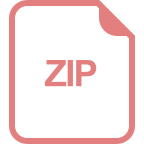
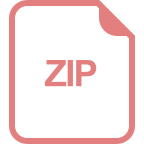
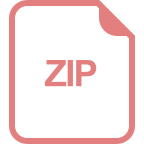
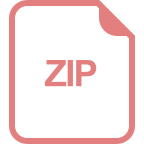
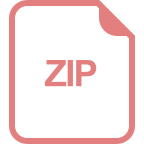
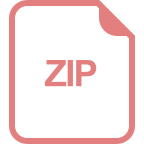
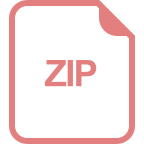
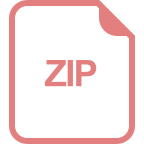
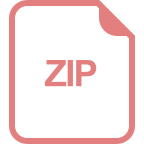
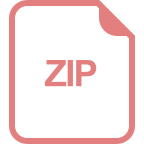



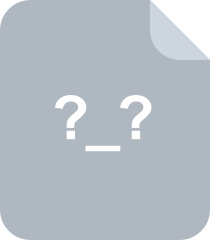
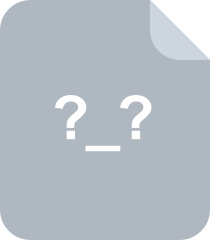
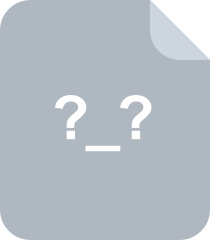
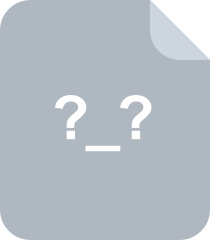
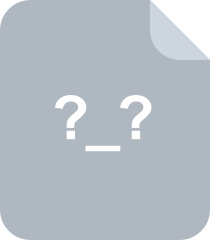
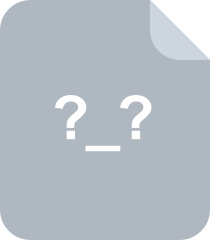
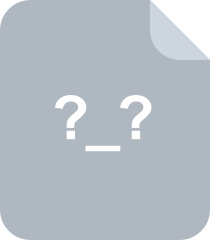
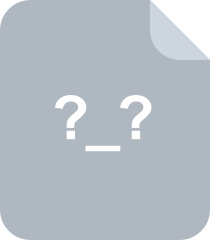
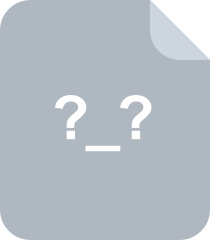
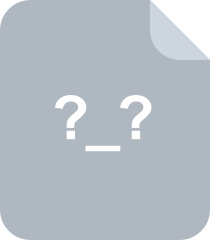
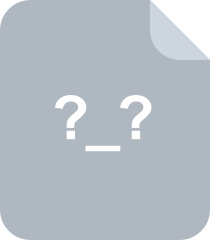
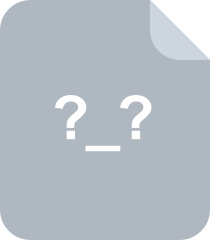
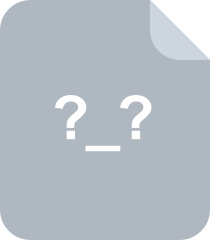

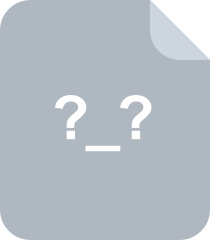
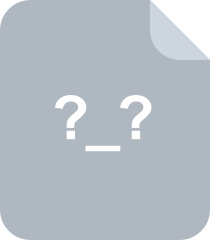

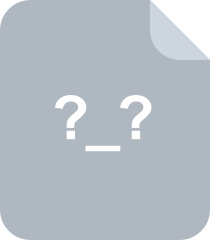
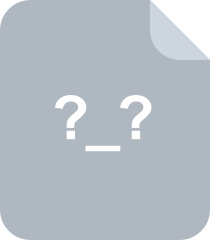
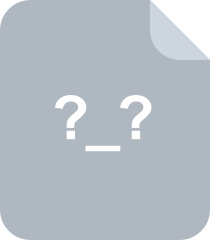
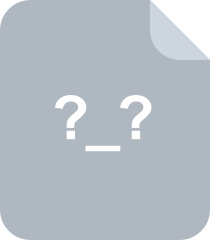

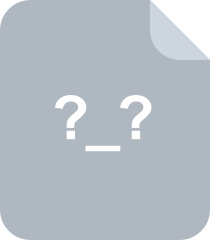
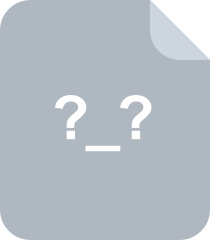
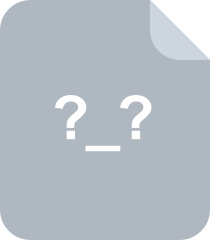


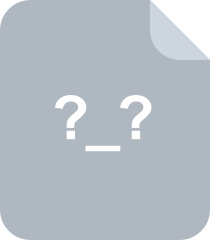
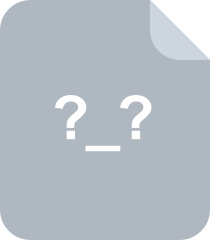


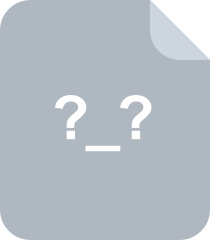


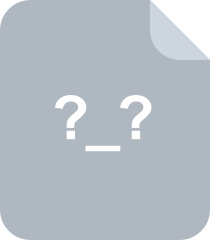
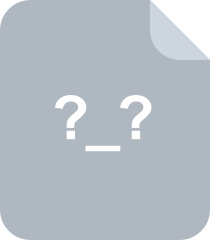



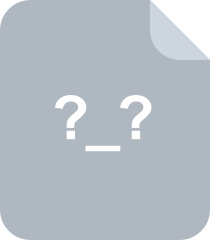
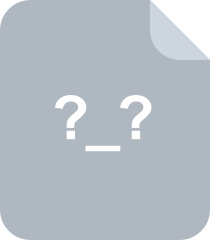
- 1
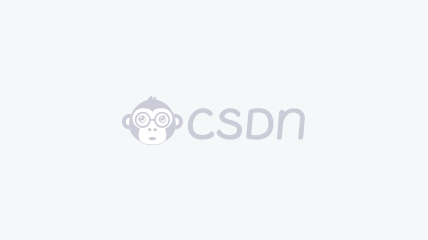
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整

- 粉丝: 53
- 资源: 32
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

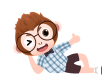
最新资源
- 4S店车辆管理系-JAVA-基于springBoot4S店车辆管理系统设计与实现(论文+PPT)
- 遗迹·v15(巅峰版)(8).zip
- JAVA编码-通过cannal同步mysql数据
- 基于matlab Simulink的双闭环三相和五相永磁同步电机仿真模型
- image_download_1736578874477.jpg
- STM32单片机步进电机8种算法全套STM32单片机步进电机S曲线SPTA梯形加减速步进算法:8种算法全套
- UE5 插件KawaiiPhysics
- 天牛须优化算法BAS优化支持向量机SVM的c和g参数做多输入单输出的拟合预测建模 程序内注释详细直接替数据就可以使用 程序语言为matlab 程序直接运行可以出拟合预测图,迭代优化图,线性拟合预
- 高考志愿智能推荐-JAVA-基于springBoot爬虫高考志愿智能推荐系统(毕业论文+PPT)
- TMS320F28335电机控制程序,bldc,pmsm无感有感,异步vf程序等,源代码和开发资料,开发资料包括源代码,原理图,说明文档等
- 模型概况comsol激光熔覆 单道多层 激光焊接 熔池形貌 版本升级目前,针对之前的模型进行了全面的修改和优化,熔池形貌更加复合实际情况 基本原理激光直接沉积过程中,快速熔化凝固和多组
- MATLAB代码:基于主从博弈的电热综合能源系统动态定价与能量管理 关键词:主从博弈 电热综合能源 动态定价 需求响应 参考文档:自编文档,完全复现 上下层算法:差分进化算法和MATLAB-cp
- matlab simulink 三机九节点系统,风电调频,双馈风机调频,一次调频,火电调频,同步机调频 带有调速器等部分 并网电压电流 风电附带下垂控制,惯性控制,风电渗透率20%,有参考文献
- 餐饮连锁店管理-JAVA-基于springBoot餐饮连锁店管理系统的设计与实现(毕业论文+PPT)
- ASP.NET大型快运快递管理系统源码 带完整文档 ASP.NET大型快运(快递)管理系统源码带完整文档 大型快递(快运)管理系统基于webservice的分布式系统,集成快递物流业务流程管理,快递物
- 《使用YOLO-v3-tiny和B-CNN实现街头车辆的检测和车辆属性的多标签识别使用》计算机相关专业毕业设计&大作业 (源码、说明、论文、数据集一站式服务,拿来就能用的绝对好资源)

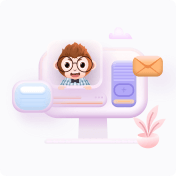
