/* This is AGAST and OAST, an optimal and accelerated corner detector
based on the accelerated segment tests
Below is the original copyright and the references */
/*
Copyright (C) 2010 Elmar Mair
All rights reserved.
Redistribution and use in source and binary forms, with or without
modification, are permitted provided that the following conditions
are met:
*Redistributions of source code must retain the above copyright
notice, this list of conditions and the following disclaimer.
*Redistributions in binary form must reproduce the above copyright
notice, this list of conditions and the following disclaimer in the
documentation and/or other materials provided with the distribution.
*Neither the name of the University of Cambridge nor the names of
its contributors may be used to endorse or promote products derived
from this software without specific prior written permission.
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS
"AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT
LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR
A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/*
The references are:
* Adaptive and Generic Corner Detection Based on the Accelerated Segment Test,
Elmar Mair and Gregory D. Hager and Darius Burschka
and Michael Suppa and Gerhard Hirzinger ECCV 2010
URL: http://www6.in.tum.de/Main/ResearchAgast
*/
#include "agast_score.hpp"
#ifdef _MSC_VER
#pragma warning( disable : 4127 )
#endif
namespace cv
{
void makeAgastOffsets(int pixel[16], int rowStride, int type)
{
static const int offsets16[][2] =
{
{-3, 0}, {-3, -1}, {-2, -2}, {-1, -3}, {0, -3}, { 1, -3}, { 2, -2}, { 3, -1},
{ 3, 0}, { 3, 1}, { 2, 2}, { 1, 3}, {0, 3}, {-1, 3}, {-2, 2}, {-3, 1}
};
static const int offsets12d[][2] =
{
{-3, 0}, {-2, -1}, {-1, -2}, {0, -3}, { 1, -2}, { 2, -1},
{ 3, 0}, { 2, 1}, { 1, 2}, {0, 3}, {-1, 2}, {-2, 1}
};
static const int offsets12s[][2] =
{
{-2, 0}, {-2, -1}, {-1, -2}, {0, -2}, { 1, -2}, { 2, -1},
{ 2, 0}, { 2, 1}, { 1, 2}, {0, 2}, {-1, 2}, {-2, 1}
};
static const int offsets8[][2] =
{
{-1, 0}, {-1, -1}, {0, -1}, { 1, -1},
{ 1, 0}, { 1, 1}, {0, 1}, {-1, 1}
};
const int (*offsets)[2] = type == AgastFeatureDetector::OAST_9_16 ? offsets16 :
type == AgastFeatureDetector::AGAST_7_12d ? offsets12d :
type == AgastFeatureDetector::AGAST_7_12s ? offsets12s :
type == AgastFeatureDetector::AGAST_5_8 ? offsets8 : 0;
CV_Assert(pixel && offsets);
int k = 0;
for( ; k < 16; k++ )
pixel[k] = offsets[k][0] + offsets[k][1] * rowStride;
}
// 16 pixel mask
template<>
int agast_cornerScore<AgastFeatureDetector::OAST_9_16>(const uchar* ptr, const int pixel[], int threshold)
{
int bmin = threshold;
int bmax = 255;
int b_test = (bmax + bmin) / 2;
short offset0 = (short) pixel[0];
short offset1 = (short) pixel[1];
short offset2 = (short) pixel[2];
short offset3 = (short) pixel[3];
short offset4 = (short) pixel[4];
short offset5 = (short) pixel[5];
short offset6 = (short) pixel[6];
short offset7 = (short) pixel[7];
short offset8 = (short) pixel[8];
short offset9 = (short) pixel[9];
short offset10 = (short) pixel[10];
short offset11 = (short) pixel[11];
short offset12 = (short) pixel[12];
short offset13 = (short) pixel[13];
short offset14 = (short) pixel[14];
short offset15 = (short) pixel[15];
while(true)
{
const int cb = *ptr + b_test;
const int c_b = *ptr - b_test;
if(ptr[offset0] > cb)
if(ptr[offset2] > cb)
if(ptr[offset4] > cb)
if(ptr[offset5] > cb)
if(ptr[offset7] > cb)
if(ptr[offset3] > cb)
if(ptr[offset1] > cb)
if(ptr[offset6] > cb)
if(ptr[offset8] > cb)
goto is_a_corner;
else
if(ptr[offset15] > cb)
goto is_a_corner;
else
goto is_not_a_corner;
else
if(ptr[offset13] > cb)
if(ptr[offset14] > cb)
if(ptr[offset15] > cb)
goto is_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
if(ptr[offset8] > cb)
if(ptr[offset9] > cb)
if(ptr[offset10] > cb)
if(ptr[offset6] > cb)
goto is_a_corner;
else
if(ptr[offset11] > cb)
if(ptr[offset12] > cb)
if(ptr[offset13] > cb)
if(ptr[offset14] > cb)
if(ptr[offset15] > cb)
goto is_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
if(ptr[offset10] > cb)
if(ptr[offset11] > cb)
if(ptr[offset12] > cb)
if(ptr[offset8] > cb)
if(ptr[offset9] > cb)
if(ptr[offset6] > cb)
goto is_a_corner;
else
if(ptr[offset13] > cb)
if(ptr[offset14] > cb)
if(ptr[offset15] > cb)
goto is_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
goto is_not_a_corner;
else
if(ptr[offset1] > cb)
if(ptr[offset13] > cb)
if(ptr
没有合适的资源?快使用搜索试试~ 我知道了~
opencv4.5.0编译x86 32位
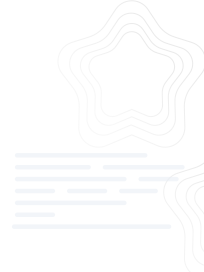
共2000个文件
tlog:2551个
cpp:1890个
obj:1697个

需积分: 0 34 下载量 82 浏览量
2023-03-20
13:56:47
上传
评论 1
收藏 512.74MB RAR 举报
温馨提示
带已经编译好的opencv x86
资源推荐
资源详情
资源评论
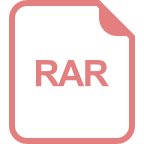
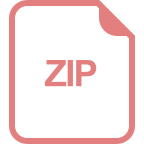
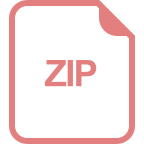
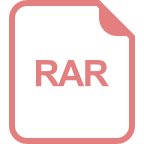
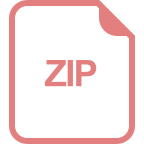
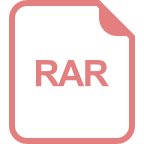
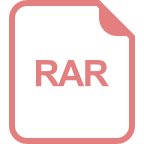
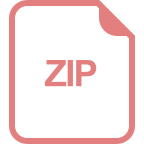
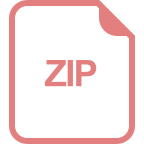
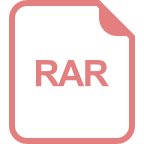
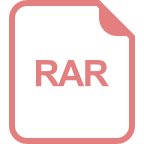
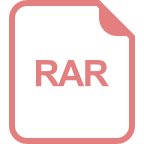
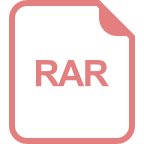
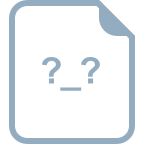
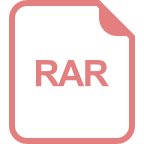
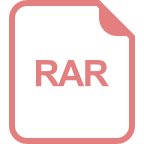
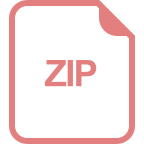
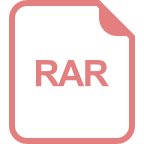
收起资源包目录

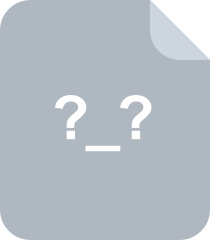
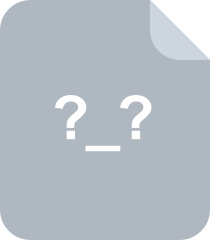
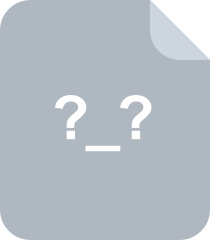
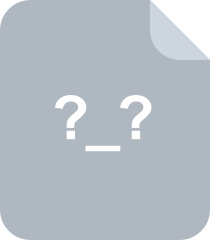
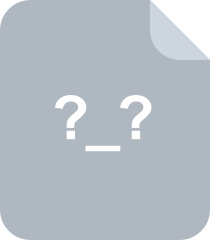
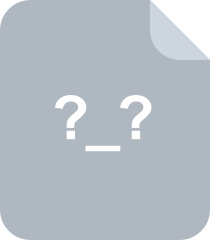
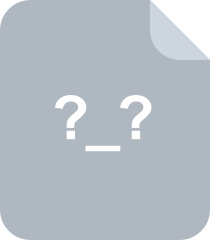
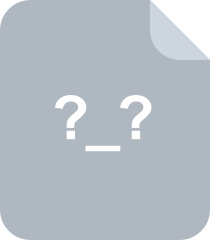
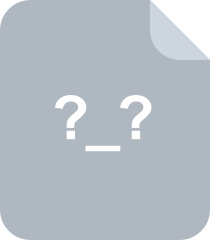
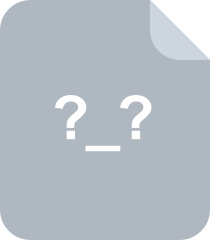
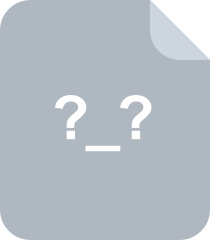
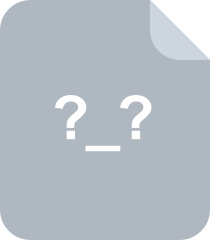
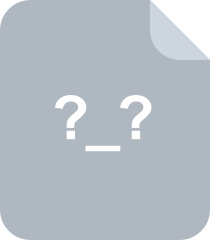
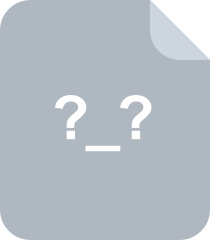
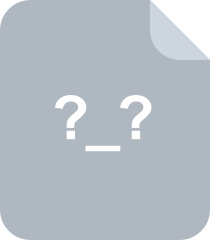
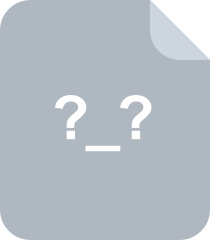
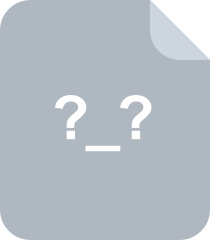
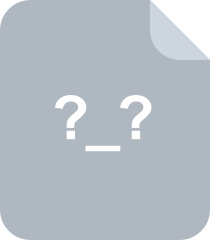
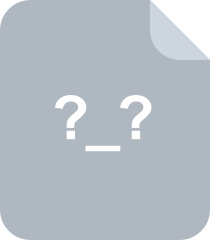
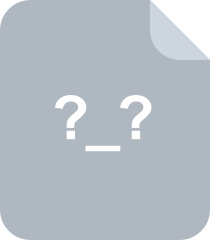
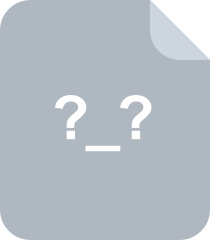
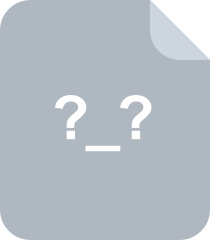
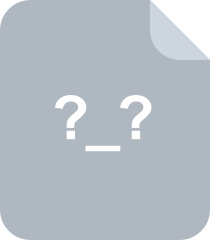
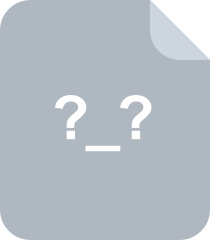
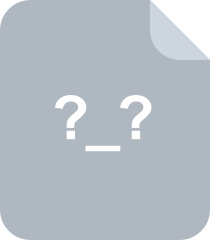
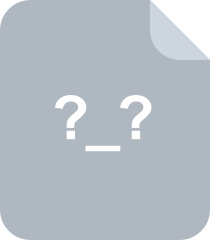
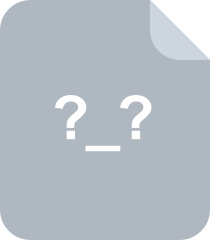
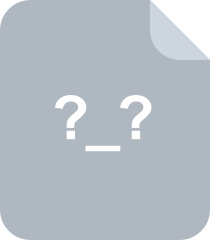
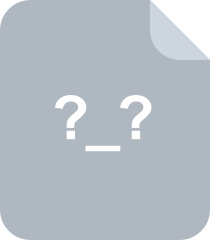
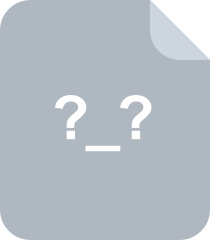
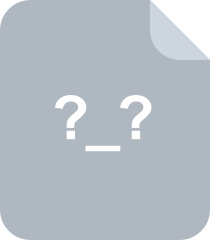
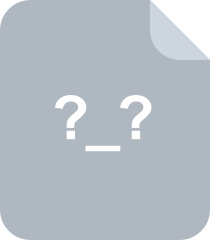
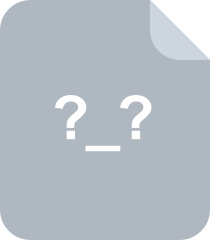
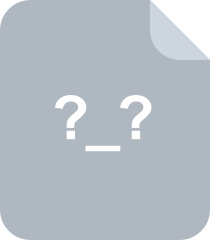
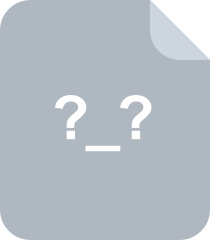
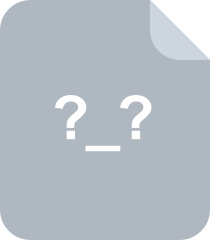
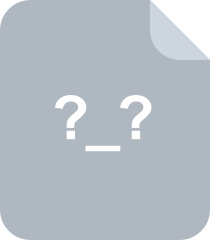
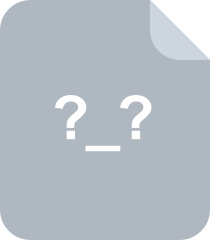
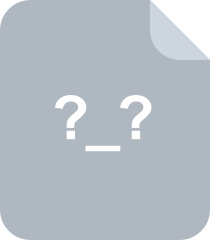
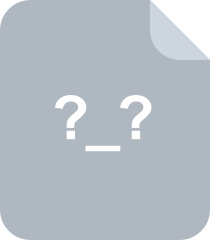
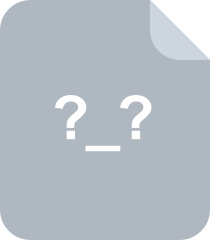
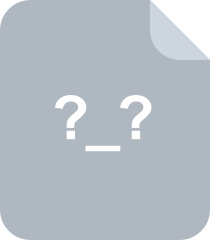
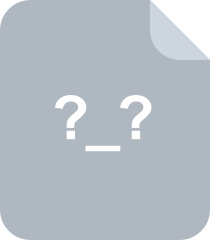
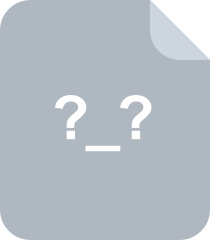
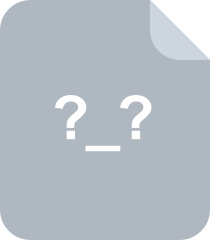
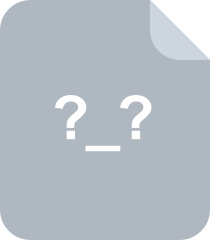
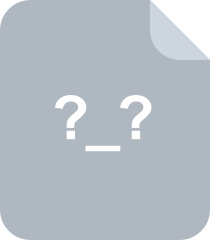
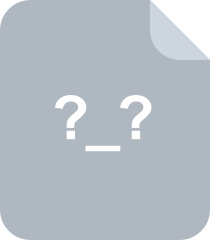
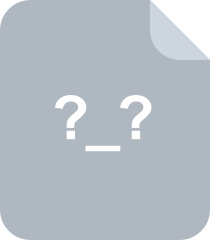
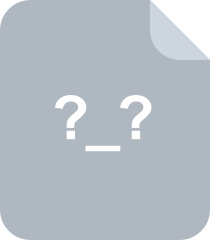
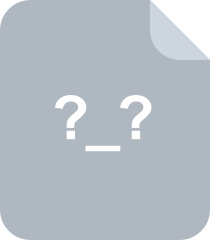
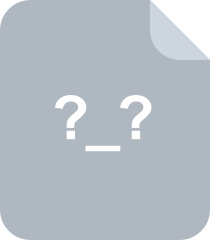
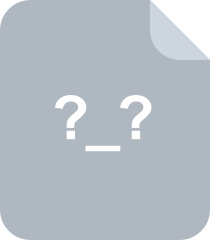
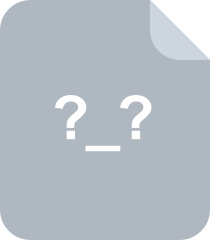
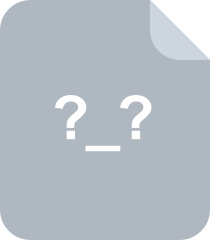
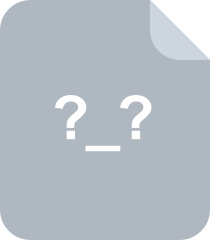
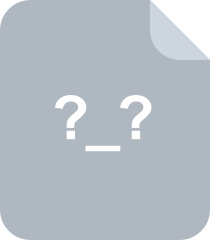
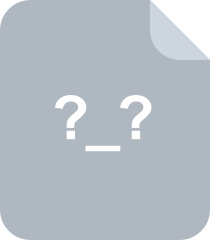
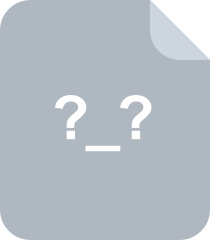
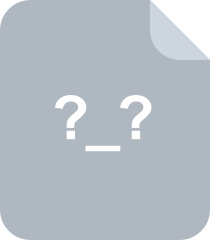
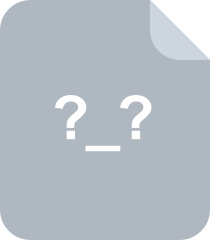
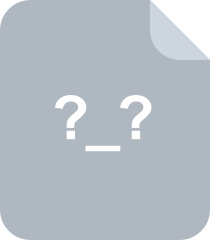
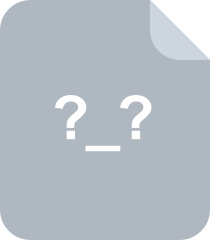
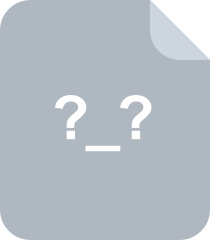
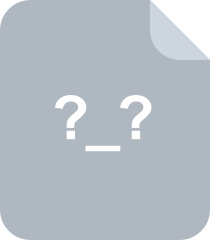
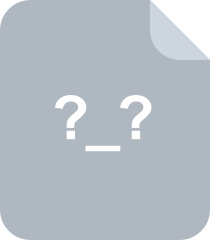
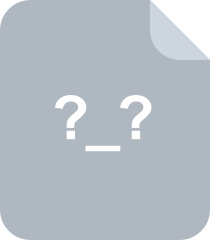
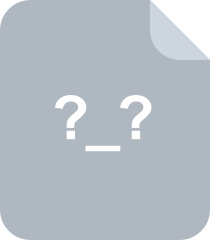
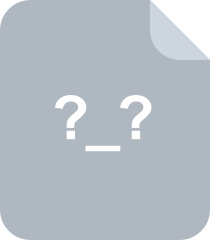
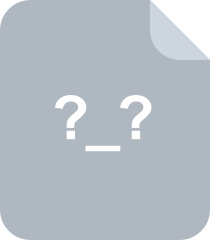
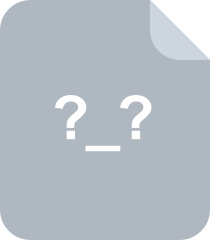
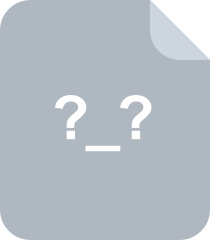
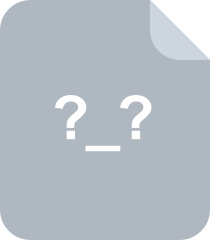
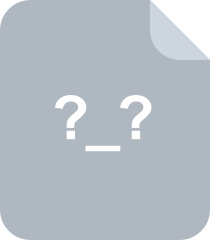
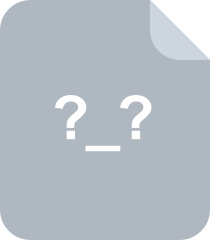
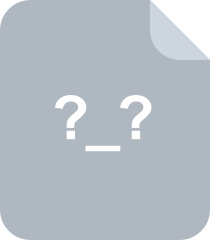
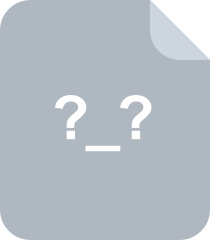
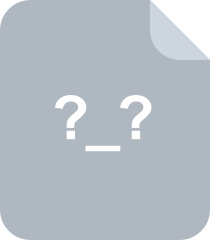
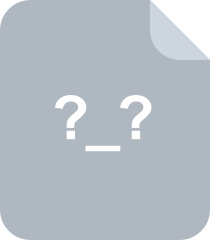
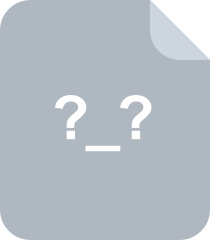
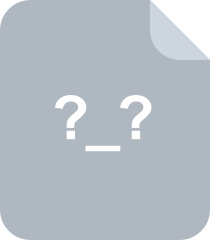
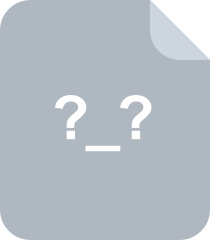
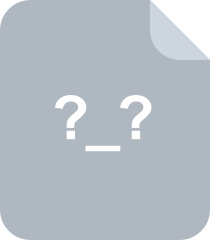
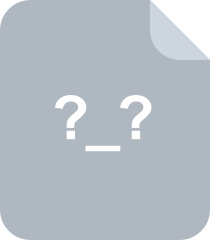
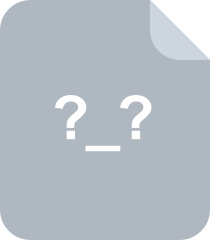
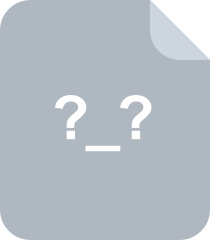
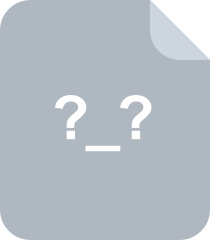
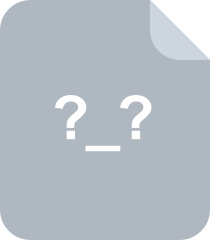
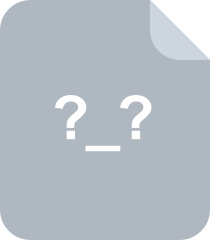
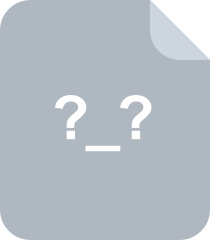
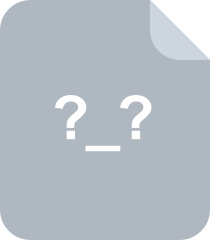
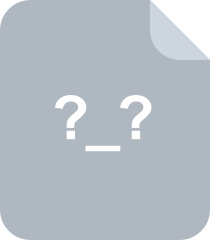
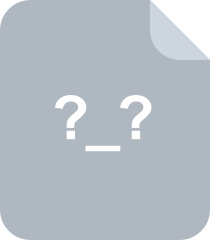
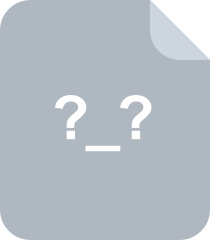
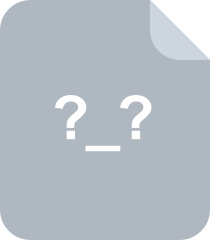
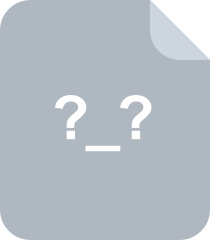
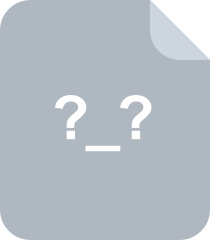
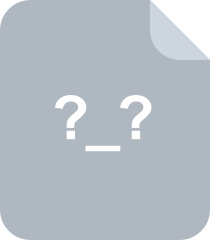
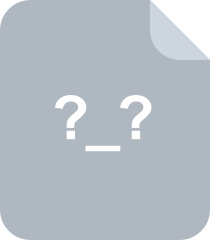
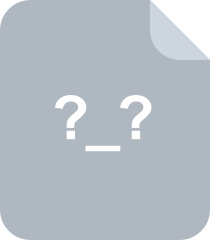
共 2000 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
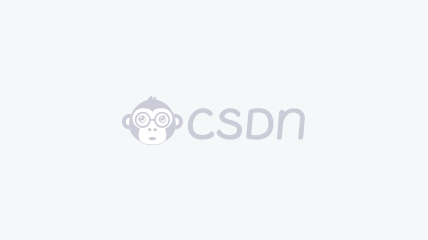

遗忘的背篓elous
- 粉丝: 4
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

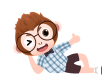
最新资源
- #P0015. 全排列 超级简单
- pta题库答案c语言之排序4统计工龄.zip
- pta题库答案c语言之树结构7堆中的路径.zip
- pta题库答案c语言之树结构3TreeTraversalsAgain.zip
- pta题库答案c语言之树结构2ListLeaves.zip
- pta题库答案c语言之树结构1树的同构.zip
- 基于C++实现民航飞行与地图简易管理系统可执行程序+说明+详细注释.zip
- pta题库答案c语言之复杂度1最大子列和问题.zip
- 三维装箱问题(Three-Dimensional Bin Packing Problem,3D-BPP)是一个经典的组合优化问题
- 以下是一些关于Linux线程同步的基本概念和方法.txt
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


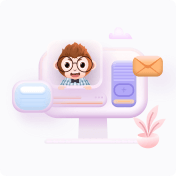
安全验证
文档复制为VIP权益,开通VIP直接复制
