C#获取当前域用户名

根据提供的标题、描述、标签及部分内容,我们可以了解到这篇文章主要讲述的是如何使用C#来获取当前登录用户的名称。这里的关键在于利用了.NET Framework中System.Management命名空间下的类与方法,特别是ManagementObjectSearcher类来查询系统中正在运行的进程及其相关信息。 ### C#获取当前域用户名 #### 前置条件 为了实现这一功能,我们需要确保项目中已经添加了对“System.Management”库的引用。这是因为我们需要用到这个库中的类来进行WMI(Windows Management Instrumentation)查询,以获取系统的管理信息。 ```csharp using System.Diagnostics; using System.Management; ``` #### 代码解析 接下来我们具体分析一下这段代码: 1. **定义命名空间**:首先定义了一个名为`WorkRecord`的命名空间。 2. **定义类**:在命名空间内定义了一个名为`CurrentUser`的类,用于封装获取当前用户的功能。 3. **公共静态方法`GetCurrentUser()`**: - 该方法用于返回当前登录的用户名。 - 方法内部通过遍历所有运行中的进程,尝试获取每个进程的所有者信息。 - 如果找到了一个非“SYSTEM”用户且非空的用户名,则将其作为当前用户返回。 4. **私有静态方法`GetProcessUserName(int pID)`**: - 这个方法接收一个进程ID作为参数,并返回该进程的所有者用户名。 - 使用`ManagementObjectSearcher`类查询WMI中的`Win32_Process`类,其中包含特定进程ID的信息。 - 查询语句为:`"Select * from Win32_Process WHERE processID=" + pID`。 - 通过查询结果,获取到进程的所有者用户名。 #### 具体实现 下面将更详细地解释关键代码段: ```csharp private static string GetProcessUserName(int pID) { string theUser = null; SelectQuery query1 = new SelectQuery("Select * from Win32_Process WHERE processID=" + pID); ManagementObjectSearcher searcher1 = new ManagementObjectSearcher(query1); try { foreach (ManagementObject disk in searcher1.Get()) { ManagementBaseObject inParams = null; ManagementBaseObject outParams = disk.InvokeMethod("GetOwner", inParams, null); theUser = ((string[])outParams["User"])[0]; } } catch (ManagementException e) { Console.WriteLine("The following error occurred: " + e.Message); } return theUser; } ``` - 在`GetProcessUserName`方法中,首先创建了一个`SelectQuery`对象,指定查询条件为进程ID等于传入的参数`pID`。 - 接着创建了一个`ManagementObjectSearcher`对象,并将查询对象传递给它。 - 使用`foreach`循环遍历搜索结果,调用`InvokeMethod`方法执行`GetOwner`方法获取进程的所有者信息。 - 从返回的对象中提取出用户名并返回。 ### 总结 以上就是如何使用C#来获取当前登录用户名的一个实现方案。这种方法利用了.NET Framework提供的强大功能,通过WMI查询系统信息。虽然实现过程稍微复杂,但能够有效地获取到所需的用户名信息。需要注意的是,使用这种方式可能会涉及到权限问题,因此在实际应用时应确保有足够的权限来执行此类操作。此外,考虑到.NET Core或.NET 5及更高版本中System.Management库的支持情况,建议根据实际情况选择合适的实现方式。
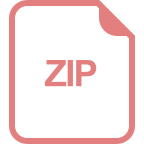
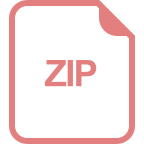
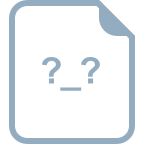
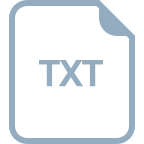
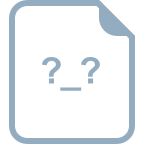
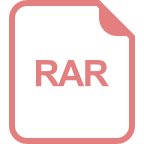
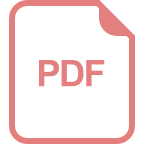
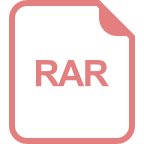
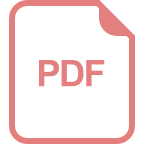
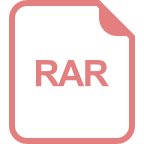
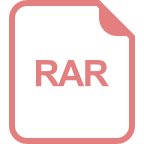
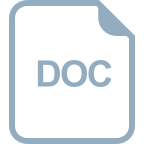
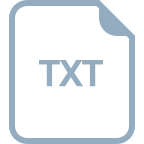
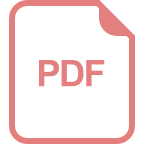
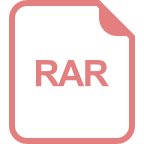
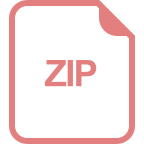
//原文出自:http://blog.csdn.net/xufei96/article/details/5853864
Ensure your project has referenced dll "System.Management", then csharp codes as below:
using System.Diagnostics;
using System.Management;
namespace WorkRecord
{
class CurrentUser
{
public static string GetCurrentUser()
{
string currentUser = null;
foreach (Process p in Process.GetProcesses())
{
currentUser = GetProcessUserName(p.Id);
if (currentUser != "SYSTEM" && currentUser != null && currentUser != string.Empty)
break;
}
return currentUser;
}
private static string GetProcessUserName(int pID)
{
string theUser = null;
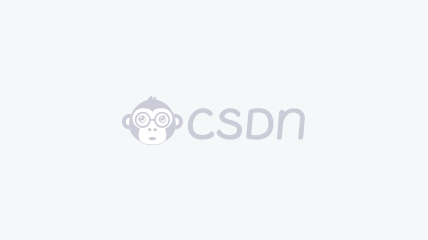
- yanyantian2012-11-03能实现,但是个人感觉有点复杂了,其实一句话就能获取。
- wanggao1234562013-11-08最简单的问题复杂化了,不过这也是一种思路了!
- meiyun_home2012-11-13在js调用当前域名就可以了。这个有点复杂
- ahu10102013-10-24文章不错,楼主继续分享哦

- 粉丝: 14
- 资源: 52
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

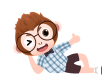
最新资源

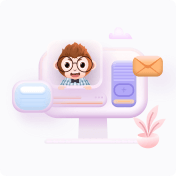
