import java.net.InetAddress;
import java.net.UnknownHostException;
import org.apache.log4j.Logger;
import org.apache.log4j.Priority;
import org.eclipse.hyades.logging.core.Guid;
import org.eclipse.hyades.logging.events.cbe.CommonBaseEvent;
import org.eclipse.hyades.logging.events.cbe.ComponentIdentification;
import org.eclipse.hyades.logging.events.cbe.EventFactory;
import org.eclipse.hyades.logging.events.cbe.ReportSituation;
import org.eclipse.hyades.logging.events.cbe.Situation;
import org.eclipse.hyades.logging.events.cbe.impl.EventFactoryContext;
/**********************************************************************
* Copyright (c) 2005 IBM Corporation and others.
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
* $Id: HyadesLoggingLog4JSample.java,v 1.3 2005/04/19 03:04:11 paules Exp $
*
* Contributors:
* IBM - Initial API and implementation
**********************************************************************/
/**
* CLASS: HyadesLoggingLog4JSample.java
*
*
* DESCRIPTION: Sample class to demonstrate the usage of the Hyades support for logging Common Base Event log records using Apache Log4J logging APIs.
*
*
* ASSUMPTIONS: 1) This class must be executed using JRE 1.4.0 or above.
* 2) The required JAR files must be specified in the JVM's classpath system variable.
* 3) JRE 1.4.0 or above must be specified in the PATH environment variable.
* 4) When launching this application from the Profiling and Logging Perspective of the Workbench, logging is enabled in the Profiling and Logging preferences (Window --> Preferences --> Profiling and Logging --> Enable logging)
* and the 'org.eclipse.hyades.tests.logging.logging.log4j.HyadesLoggingLog4JSample' logging agent is added to the Profiling and Logging preferences (Window --> Preferences --> Profiling and Logging --> Logging Agents --> New agent --> Add Agent).
* 5) When either launching this application from the Profiling and Logging Perspective of the Workbench or attaching to a logging agent using the Workbench, the Hyades Data Collection Engine application or service is configured and running.
* 6) When launching this application from the Profiling and Logging Perspective of the Workbench, the application launch configuration or the Hyades Data Collection Engine application or service classpath contains Apache Log4J 1.2.8 or above (not provided) in the classpath.
* 7) The 'log4j.configuration' environment variable is set to the Apache Log4J logging's configuration file's (HyadesLoggingLog4JSample.xml) absolute path and name in the form of a URL. For example, <i>file:/<workspace></i>/HyadesLoggingLog4JProject/cfg/HyadesLoggingLog4JSample.xml.
*
*
* DEPENDENCIES: The following JAR files are required to compile and execute HyadesLoggingLog4JSample.java:
*
* hexr.jar - org.eclipse.hyades.execution.remote
* hllog4j.jar - org.eclipse.hyades.logging.log4j
* hlcore.jar - org.eclipse.hyades.logging.core
* hlcb101.jar - org.eclipse.hyades.logging.core
* common.jar - org.eclipse.emf.common\runtime
* ecore.jar - org.eclipse.emf.ecore\runtime
* log4j-1.2.8.jar - org.apache.jakarta_log4j_logging
*
* *Not provided.
* <p>
*
*
* @author Paul E. Slauenwhite
* @version April 18, 2005
* @since July 20, 2004
* @since 1.0.1
* @see org.apache.log4j.Logger
* @see org.apache.log4j.Level
* @see org.apache.log4j.Appender
* @see org.apache.log4j.Layout
* @see org.apache.log4j.spi.Filter
* @see org.eclipse.hyades.logging.log4j.LoggingAgentAppender
* @see org.eclipse.hyades.logging.log4j.SingleLoggingAgentAppender
* @see org.eclipse.hyades.logging.events.cbe.CommonBaseEvent
* @see org.eclipse.hyades.logging.events.cbe.EventFactory
* @see org.eclipse.hyades.logging.events.cbe.impl.EventFactoryContext
*/
public class HyadesLoggingLog4JSample {
//Amount of time (seconds) to wait to allow time to attach to the logging agent:
private static final int WAITTIME = 30;
//The name of the Log4J logger:
private static final String LOGGERS_NAME = "HyadesLoggingLog4JSample";
/**
* IP address (IPv4) of the local host, otherwise "127.0.0.1".
*/
private static String localHostIP = null;
static {
try {
localHostIP = InetAddress.getLocalHost().getHostAddress();
}
catch (UnknownHostException u) {
localHostIP = "127.0.0.1";
}
}
public static void main(String[] args) {
try {
//OPTIONAL: Re-load the Log4J logging configuration XML file:
//DOMConfigurator.configure("HyadesLoggingLog4JSample.xml");
//Create a logger named 'org.eclipse.hyades.logging.log4j.sample.HyadesLoggingLog4JSample':
Logger logger = Logger.getLogger(LOGGERS_NAME);
//NOTE: Alternatively, set the logger's level programmatically:
//Set the logger to log warning or lower messages:
//logger.setLevel(Level.WARN);
//NOTE: Alternatively, create and set the logger's console appender programmatically:
//Create a new instance of a console appender:
//Appender consoleAppender = new ConsoleAppender();
//NOTE: Alternatively, set the console appender's log record filter programmatically via the appender.addFilter() API:
//Set the console appender's log record filter:
//consoleAppender.addFilter(new CommonBaseEventFilter());
//NOTE: Alternatively, set the logger's console appender programmatically via the logger.addAppender() API:
//Add the console appender to the logger:
//logger.addAppender(consoleAppender);
//NOTE: Alternatively, create and set the logger's Logging Agent appender programmatically:
//Create a new instance of a Logging Agent appender:
//Appender loggingAgentAppender = new LoggingAgentAppender();
//NOTE: Alternatively, set the Logging Agent appender's log record filter programmatically via the appender.addFilter() API:
//Set the Logging Agent appender's log record filter:
//loggingAgentAppender.addFilter(new CommonBaseEventFilter());
//NOTE: Alternatively, set the logger's Logging Agent appender programmatically via the logger.addAppender() API:
//Add the Logging Agent appender to the logger:
//logger.addAppender(loggingAgentAppender);
//NOTE: Alternatively, create and set the logger's Logging Agent appender programmatically using the Single Logging Agent appender:
//Create a new instance of a Single Logging Agent appender:
//Appender singleLoggingAgentAppender = new SingleLoggingAgentAppender();
//NOTE: Alternatively, set the Single Logging Agent appender's log record filter programmatically via the appender.addFilter() API:
//Set the Single Logging Agent appender's log record filter:
//singleLoggingAgentAppender.addFilter(new CommonBaseEventFilter());
//NOTE: Alternatively, set the logger's Single Logging Agent appender programmatically via the logger.addAppender() API:
//Add the Single Logging Agent appender to the logger:
//logger.addAppender(singleLoggingAgentAppender);
//Retrieve the instance of the Event Factory:
EventFactory eventFactory = EventFactoryContext.getInstance().getSimpleEventFactoryHome().getAnonymousEventFactory();
//Create a new instance of a report situation:
ReportSituation reportSituation = eventFactory.createReportSituation();
reportSituation.setReasoningScope("INTERNAL");
reportSituation.setReportCategory("LOG");
//Create a new instance of a situation:
Situation situation = eventFactory.createSituation();
situation.setCategoryName("ReportSituation");
situation.setSituationType(reportSituation);
//Create a new
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
eclipse tptp THE ACCOMPANYING PROGRAM IS PROVIDED UNDER THE TERMS OF THIS ECLIPSE PUBLIC LICENSE ("AGREEMENT"). ANY USE, REPRODUCTION OR DISTRIBUTION OF THE PROGRAM CONSTITUTES RECIPIENT'S ACCEPTANCE OF THIS AGREEMENT.
资源推荐
资源详情
资源评论
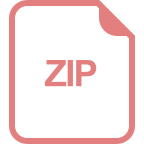
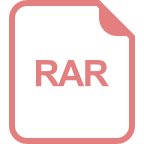
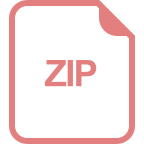
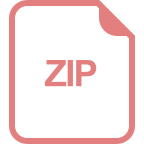
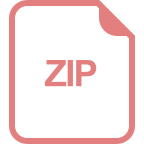
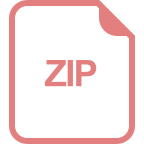
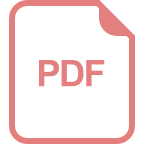
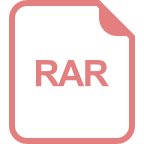
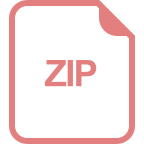
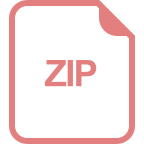
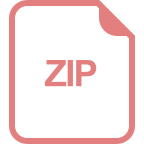
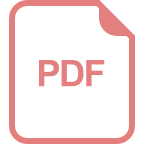
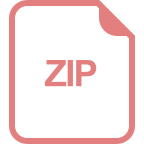
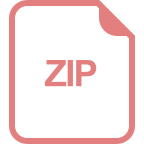
收起资源包目录

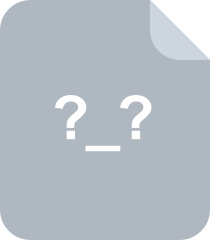
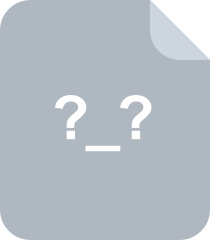
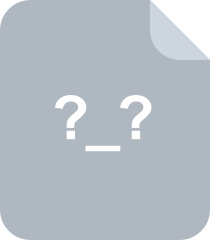
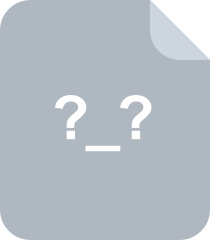
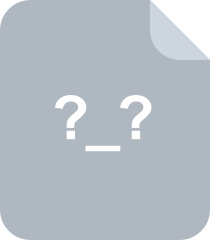
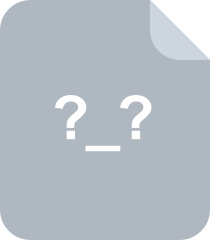
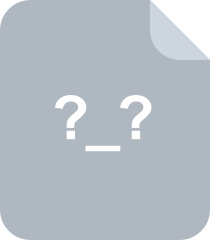
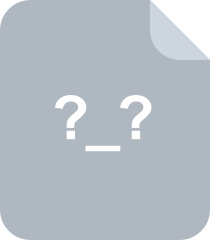
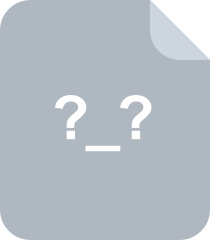
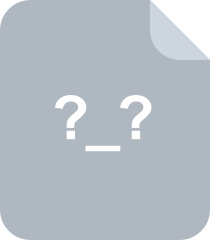
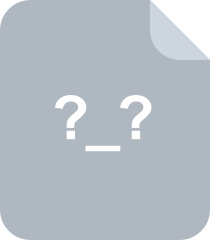
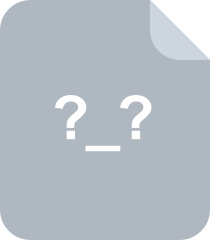
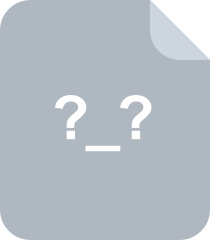
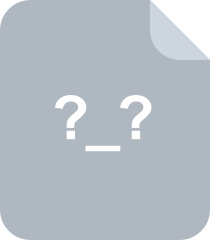
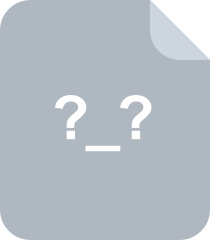
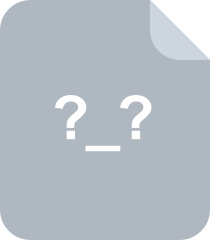
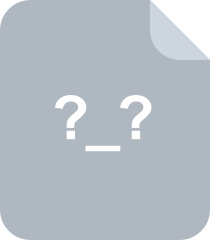
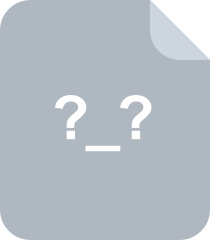
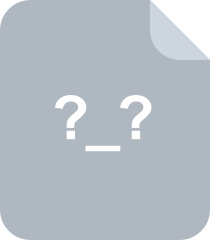
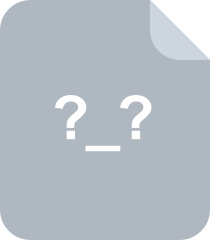
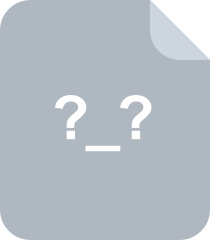
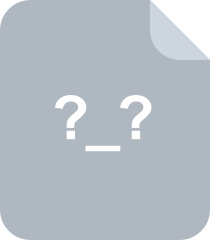
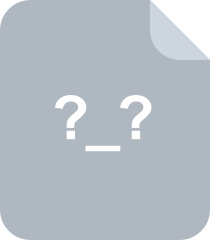
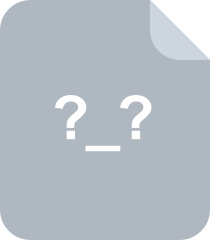
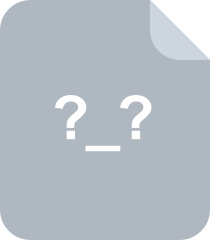
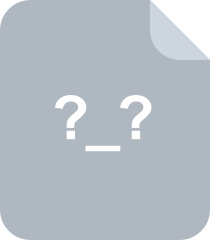
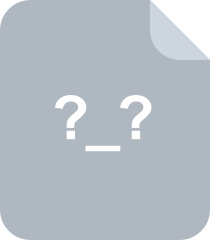
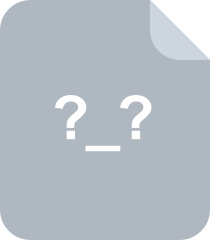
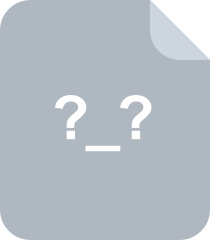
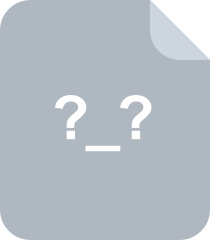
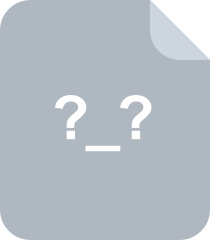
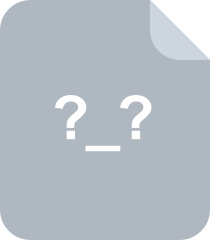
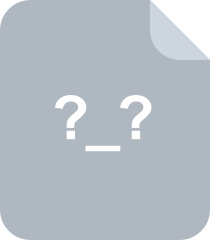
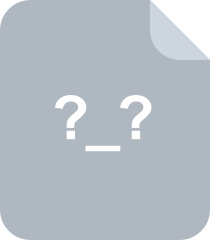
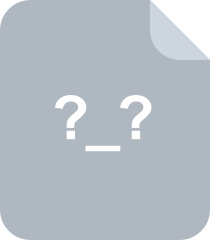
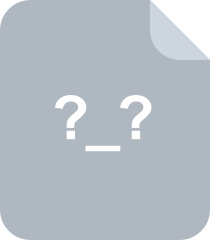
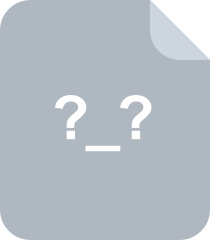
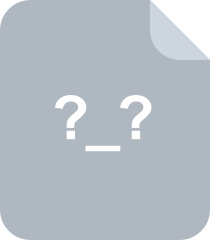
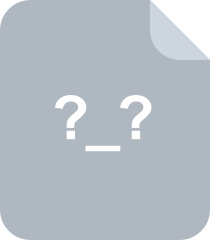
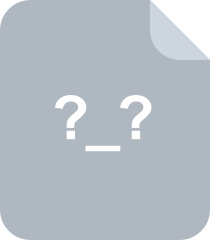
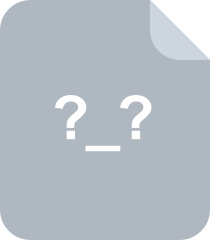
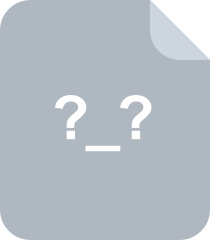
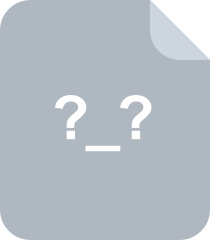
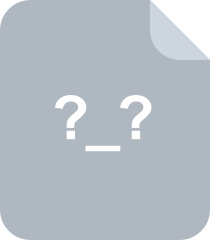
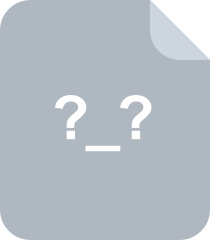
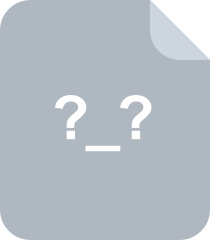
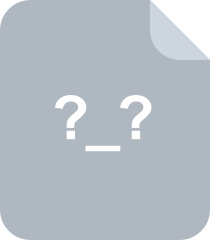
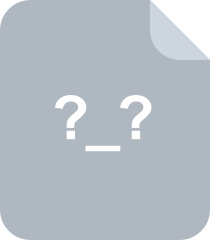
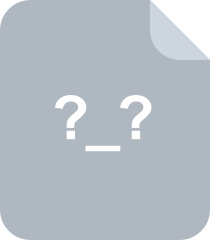
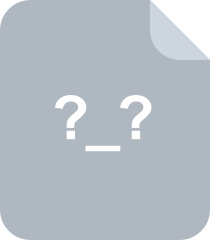
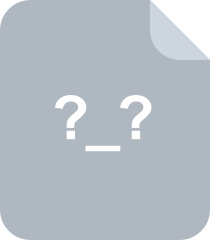
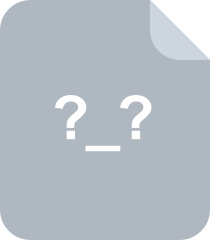
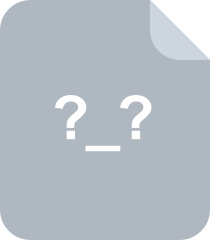
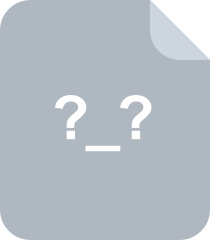
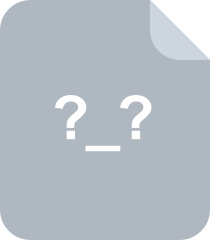
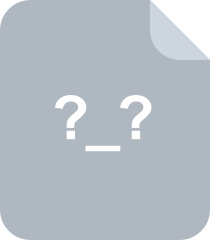
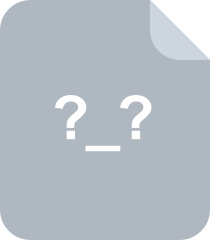
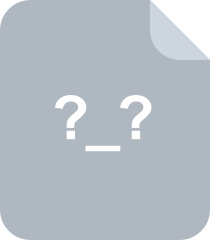
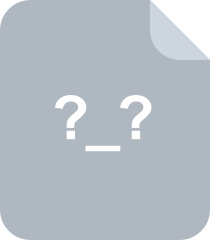
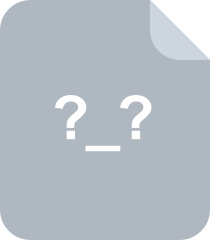
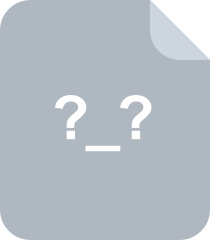
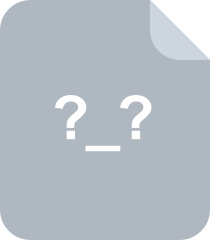
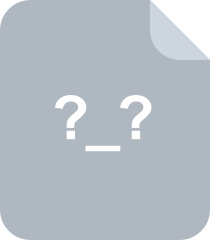
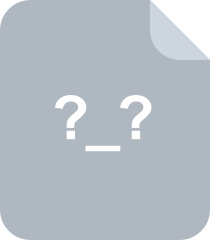
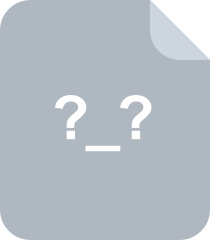
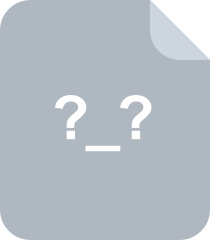
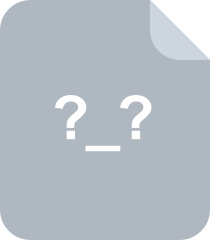
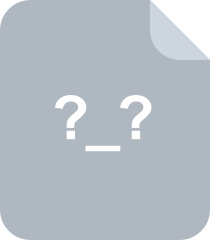
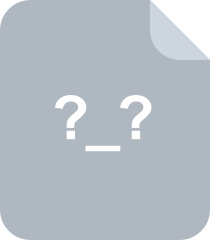
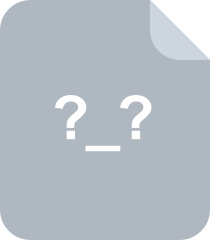
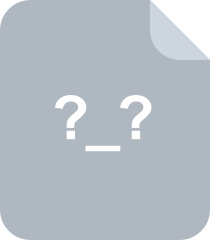
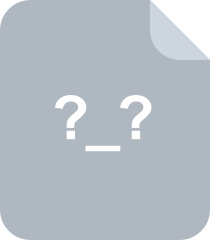
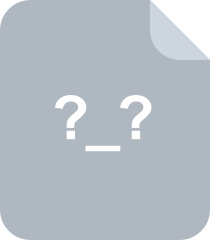
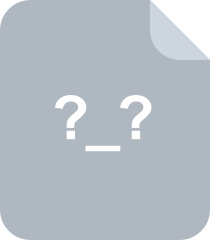
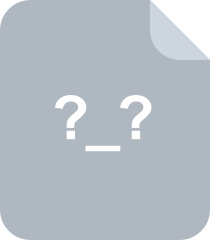
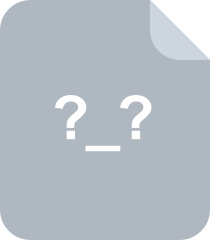
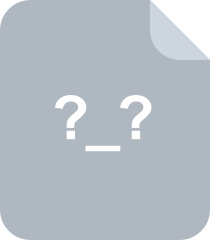
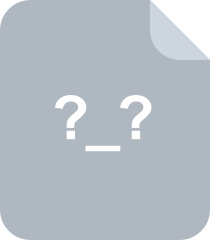
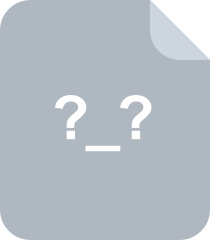
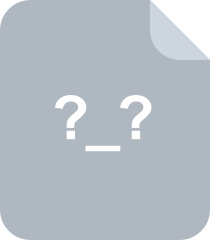
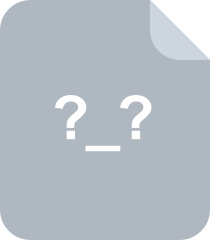
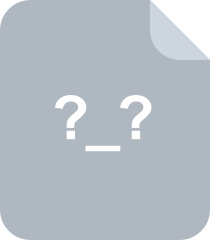
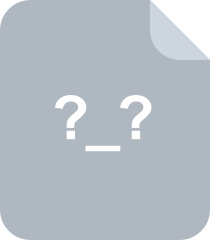
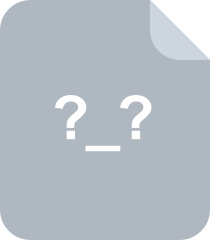
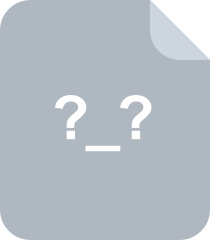
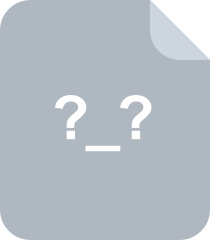
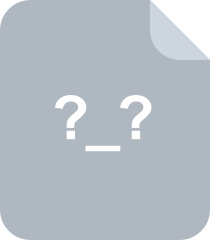
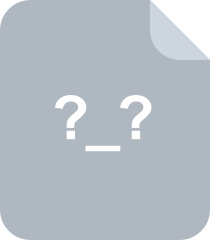
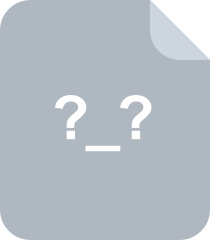
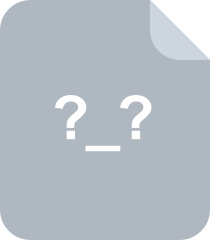
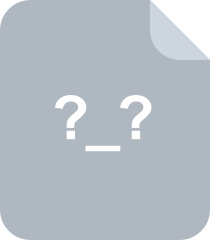
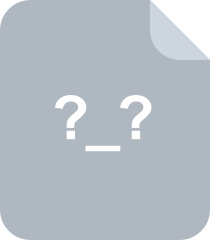
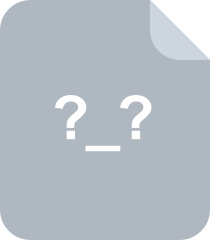
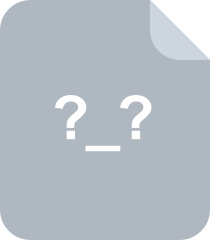
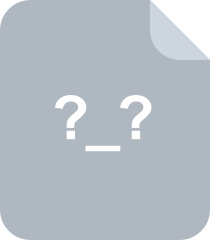
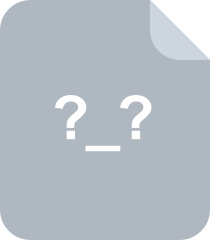
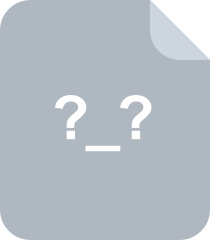
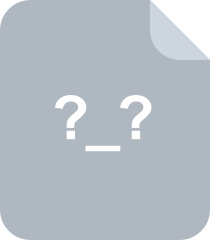
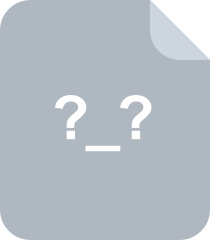
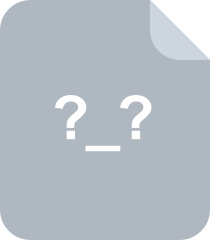
共 1988 条
- 1
- 2
- 3
- 4
- 5
- 6
- 20
资源评论
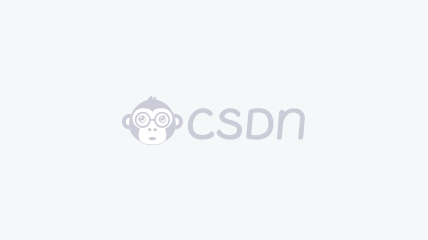
- idealwangqing2012-08-10可以下载,但用link的方式一直没有成功,最后选择直接在eclipse里面下载的。

huyimin12
- 粉丝: 1
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

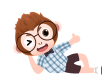
最新资源
- CC2530无线zigbee裸机代码实现液晶LCD显示.zip
- CC2530无线zigbee裸机代码实现中断唤醒系统.zip
- 车辆、飞机、船检测24-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 基于51单片机的火灾烟雾红外人体检测声光报警系统(protues仿真)-毕业设计
- 高仿抖音滑动H5随机短视频源码带打赏带后台 网站引流必备源码
- 车辆、飞机、船检测25-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- 四足机器人示例代码pupper-example-master.zip
- Python人工智能基于深度学习的农作物病虫害识别项目源码.zip
- 基于MIT mini-cheetah 的四足机器人控制quadruped-robot-master.zip
- 菠萝狗四足机器人py-apple-bldc-quadruped-robot-main.zip
- 基于51单片机的篮球足球球类比赛计分器设计(protues仿真)-毕业设计
- 第3天实训任务--电子22级.pdf
- 基于FPGA 的4位密码锁矩阵键盘 数码管显示 报警仿真
- 车辆、飞机、船检测5-YOLO(v5至v11)、COCO、CreateML、Paligemma、VOC数据集合集.rar
- 河南大学(软工免浪费时间)
- NOIP-学习建议-C++
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


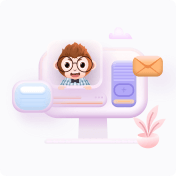
安全验证
文档复制为VIP权益,开通VIP直接复制
