# Introduction
1. This project is a demo project for spring cloud microservice structure, using spring-boot, including:
- spring cloud eureka
- spring cloud config
- ribbon
- feign
- hystrix
- turbine
- Spring Cloud Starters
- multi databases with dynamic select(AOP)
- Global traceId
- velocity
- mybatis, pageHelper (for paging), druid (alibaba druid, for mysql dataSource and connection pool)
- redis (jdk serialization)
- slf4j & logback
- Internationality
- Global Exception catch
- Task executor thread pool
- HealthCheck, globalHealthCheck
2. This project has a login page. After login, you can see the first page of momentList, add new moments to the list, see the comments of each moment, and add new comments to the comment list.
# Run
1. first of all, you should get a redis started, and a mysql started. Then, the sql for creating databases and dbs is as below:
```
create database test;
CREATE TABLE `account` (
`user_id` varchar(127) NOT NULL DEFAULT '',
`user_name` varchar(127) NOT NULL DEFAULT '',
`password` varchar(127) NOT NULL DEFAULT '',
`gmt_created` datetime NOT NULL,
`gmt_modified` datetime DEFAULT NULL,
`is_deleted` tinyint(1) NOT NULL DEFAULT '0',
PRIMARY KEY (`user_id`),
KEY `index_user_id` (`user_id`) KEY_BLOCK_SIZE=10
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
CREATE TABLE `moment` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`user_id` varchar(127) COLLATE utf8mb4_unicode_ci NOT NULL,
`content` text COLLATE utf8mb4_unicode_ci,
`gmt_created` datetime NOT NULL,
`gmt_modified` datetime DEFAULT NULL,
`is_deleted` tinyint(1) DEFAULT '0',
PRIMARY KEY (`id`),
KEY `index_user_id` (`user_id`)
) ENGINE=InnoDB AUTO_INCREMENT=8 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
create database test2;
CREATE TABLE `comment` (
`id` bigint(20) unsigned NOT NULL AUTO_INCREMENT,
`moment_id` bigint(20) unsigned NOT NULL,
`content` text COLLATE utf8mb4_unicode_ci,
`gmt_created` datetime NOT NULL,
`gmt_modified` datetime DEFAULT NULL,
`is_deleted` tinyint(1) DEFAULT '0',
PRIMARY KEY (`id`),
KEY `index_moment_id` (`moment_id`)
) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci;
```
2. Java 8 and maven 3 are required.
3. run command in order:
```
cd spring-cloud-parent
mvn clean install -DskipTests
cd ../spring-cloud-client
mvn clean install -DskipTests
cd ../spring-cloud-starter
mvn clean install -DskipTests
cd spring-cloud-eureka
mvn clean spring-boot:run
cd spring-cloud-account
mvn clean spring-boot:run
cd spring-cloud-biz
mvn clean spring-boot:run
cd spring-cloud-gateway
mvn clean spring-boot:run
```
4. open ```http://127.0.0.1:7001/index``` in brower
# Code explain
1. The dependency of this project is shown below :

2. spring-cloud-parent is just a empty maven project, contains the common poms for other project, so spring-cloud-eureka, spring-cloud-biz, spring-cloud-account and spring-cloud-gateway all inherate from spring-cloud-parent
3. spring-cloud-starter is a demo starter, it cointains the common beans, common bean configurations for spring-cloud-biz, spring-cloud-account and spring-cloud-gateway.
4. spring-cloud-client is a common depency for all, contains some util classes and java Models responding to the db table.
5. spring-cloud-eureka is a server for Service Registration and Service Discovery. Also, I combined spring cloud config with eureka, for dynamic configrations. The heart beat time of eureka is configed to 5s, instead of 15s.
6. spring-cloud-account is the account module
7. spring-cloud-biz is the real business module, contains the moment module and comment module, and this project uses two dbs dynamicly.
8. spring-cloud-gateway is the gateway for all these modules. All outer requests from apps or web pages, should be sent to gateway. GateWay should veirify the login status, do the uploading, do some filters or other aspects.
9. If you want to stop any of spring-cloud-account, spring-cloud-biz or spring-cloud-gateway, use this command: ```curl -H 'Accept:application/json' -X POST localhost:7004/shutdown```, to stop the heartbeat to the eureka cluster.
# Deploy to the production env
1. These projects should be deployed like this:

中文介绍: http://www.jianshu.com/p/c14c47243994
Any questions, please send to chxfantasy@gmail.com
HomePage: http://www.jianshu.com/u/c031bded621b
wx: hurry_words
没有合适的资源?快使用搜索试试~ 我知道了~
毕业设计&课设_Spring Cloud 演示项目:含多种功能,介绍运行、部署,涉及相关技术主题.zip
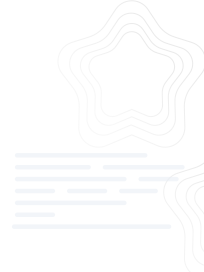
共175个文件
java:118个
xml:21个
properties:18个

1.该资源内容由用户上传,如若侵权请联系客服进行举报
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
2.虚拟产品一经售出概不退款(资源遇到问题,请及时私信上传者)
版权申诉
0 下载量 40 浏览量
2024-11-15
10:35:59
上传
评论
收藏 1.98MB ZIP 举报
温馨提示
1、资源项目源码均已通过严格测试验证,保证能够正常运行; 2、项目问题、技术讨论,可以给博主私信或留言,博主看到后会第一时间与您进行沟通; 3、本项目比较适合计算机领域相关的毕业设计课题、课程作业等使用,尤其对于人工智能、计算机科学与技术等相关专业,更为适合; 4、下载使用后,可先查看README.md文件(如有),本项目仅用作交流学习参考,请切勿用于商业用途。
资源推荐
资源详情
资源评论
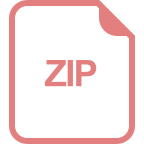
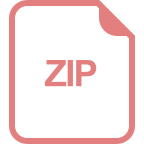
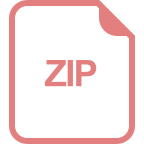
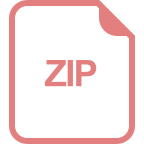
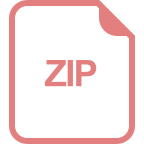
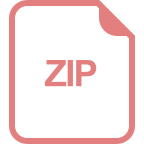
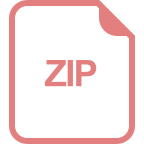
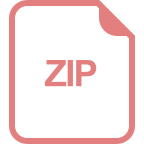
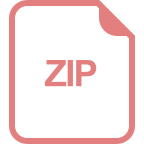
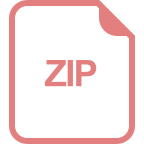
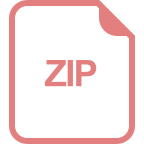
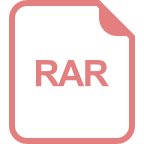
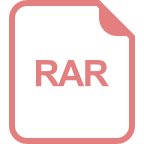
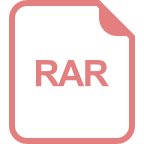
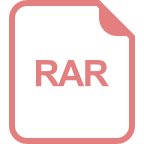
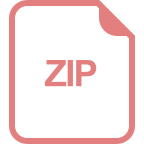
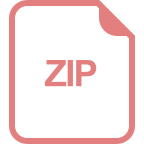
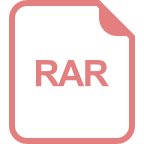
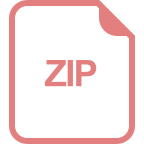
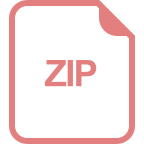
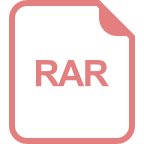
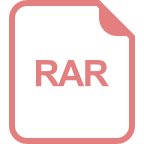
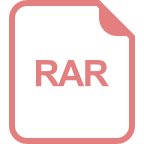
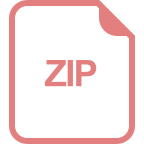
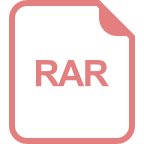
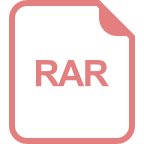
收起资源包目录

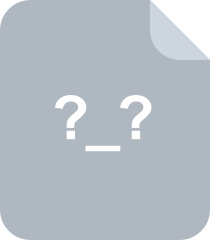
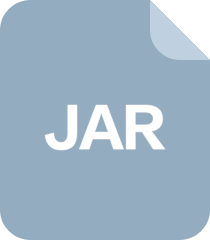
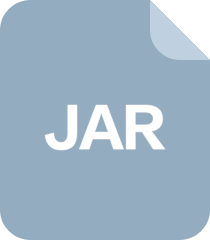
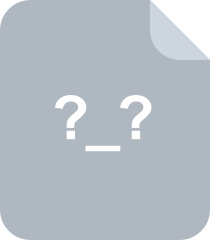
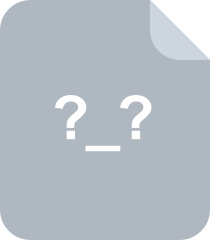
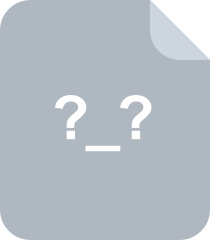
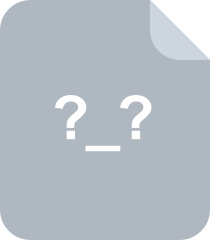
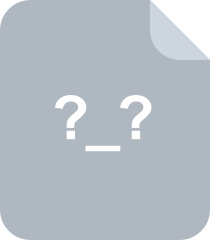
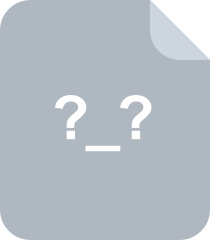
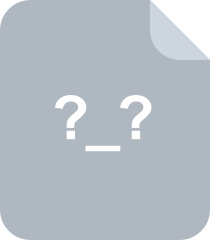
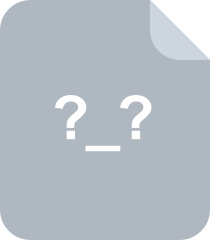
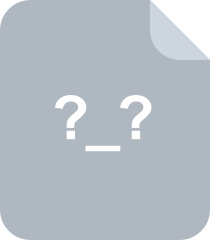
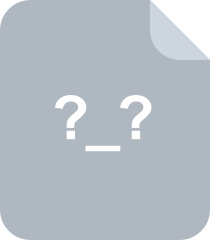
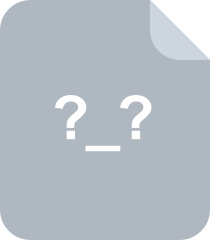
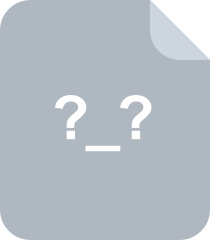
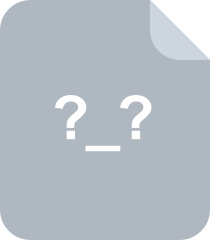
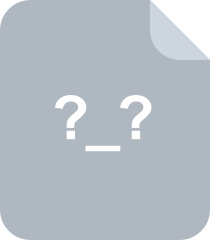
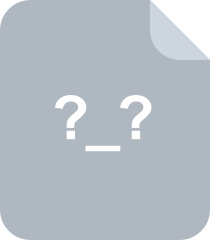
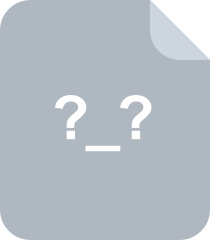
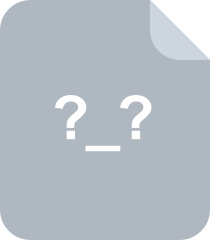
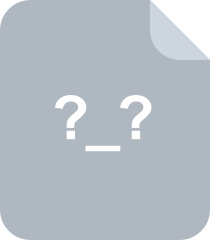
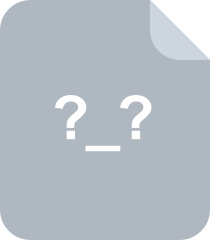
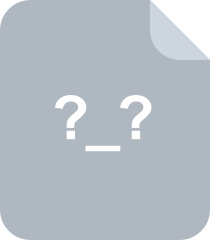
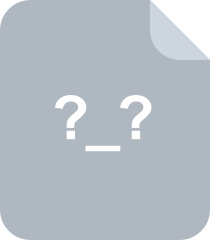
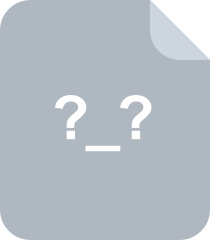
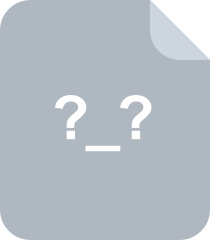
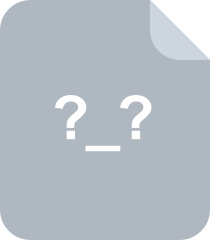
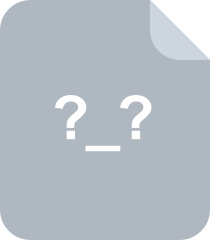
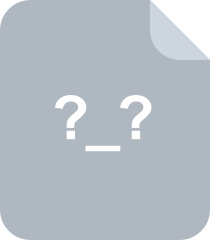
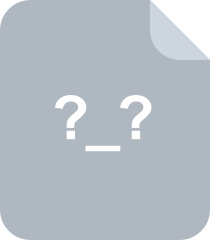
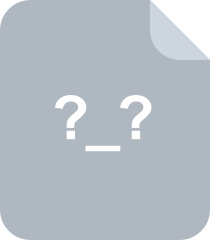
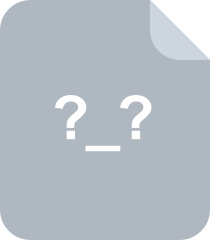
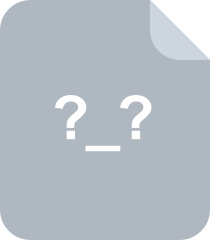
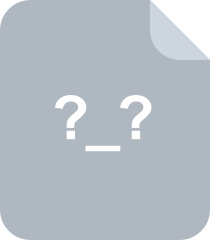
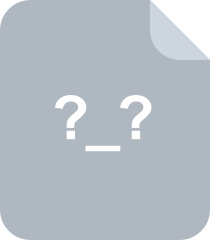
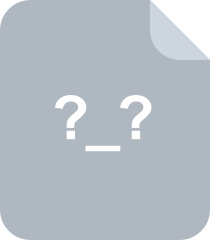
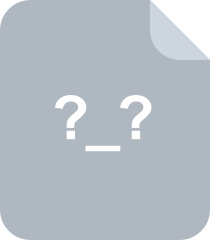
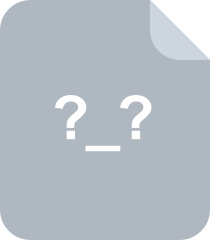
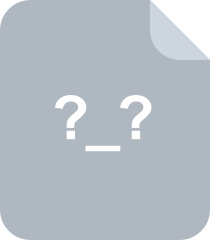
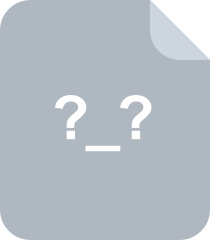
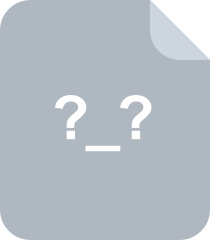
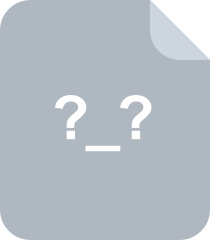
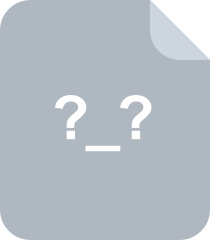
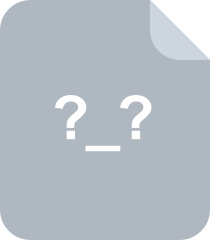
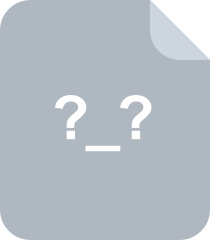
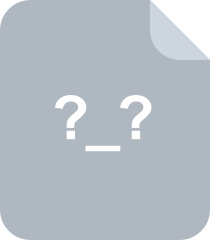
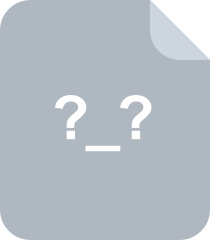
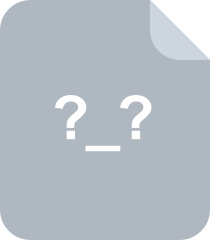
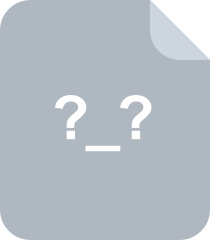
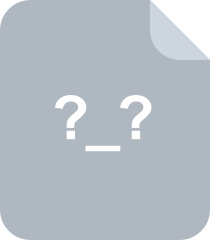
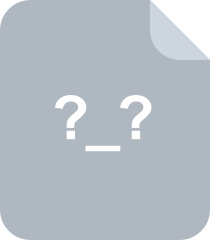
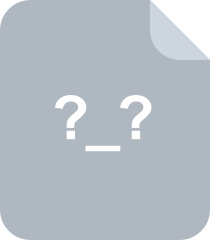
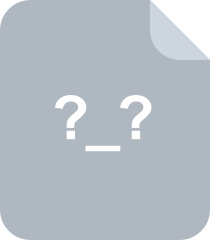
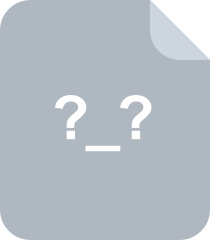
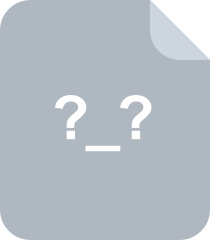
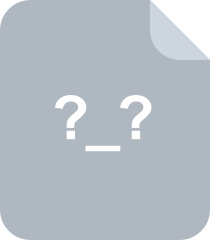
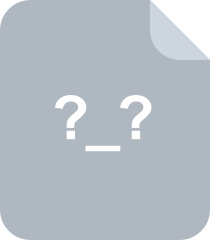
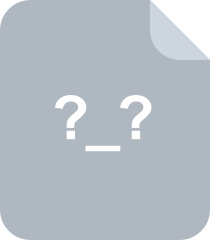
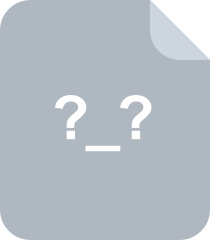
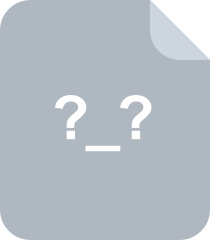
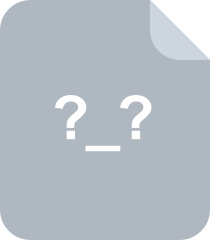
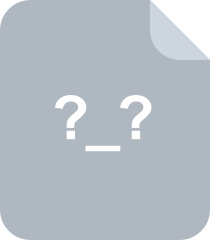
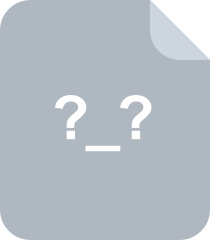
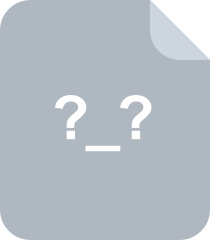
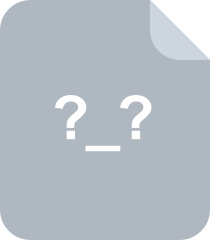
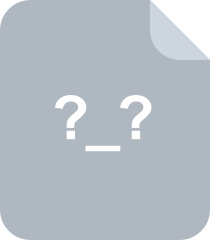
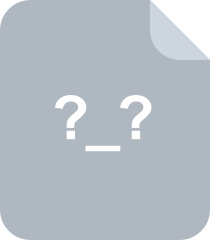
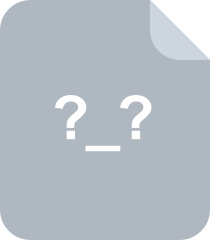
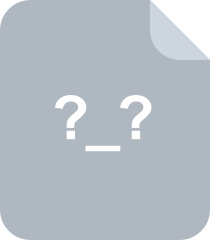
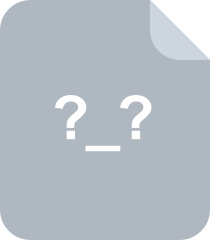
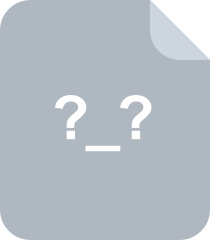
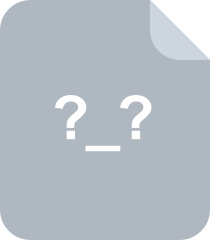
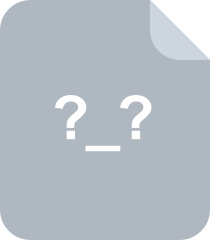
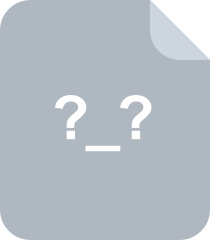
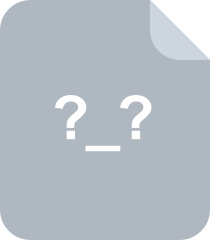
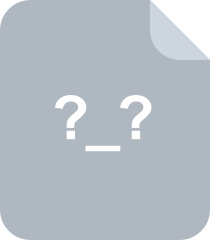
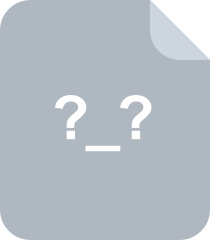
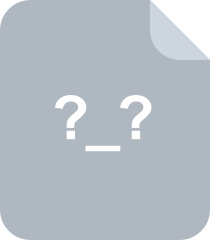
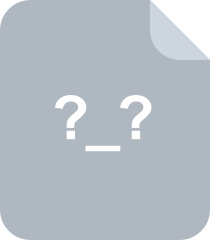
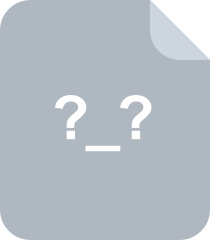
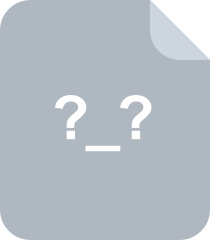
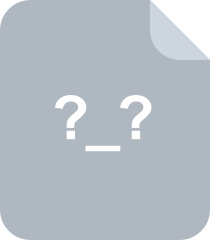
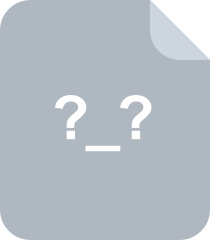
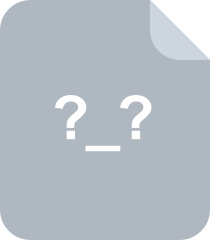
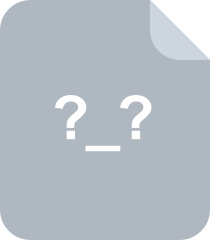
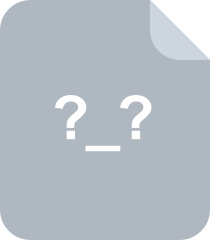
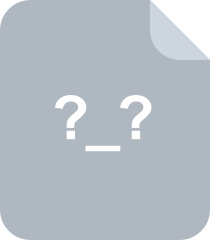
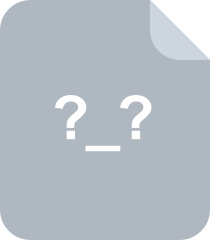
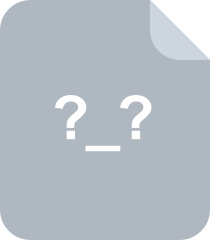
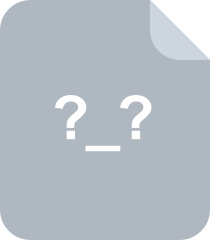
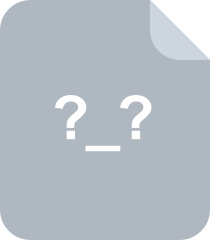
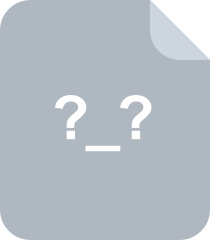
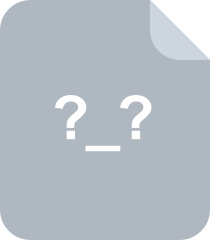
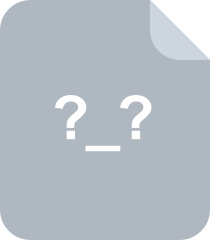
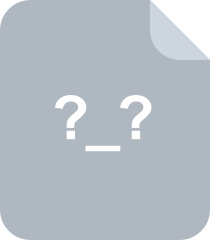
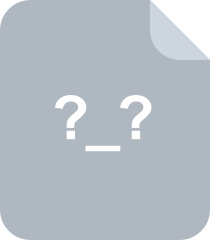
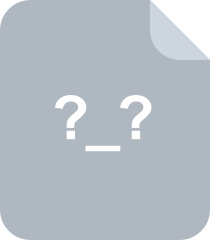
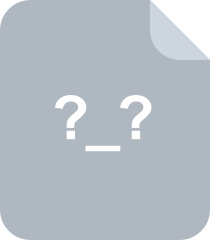
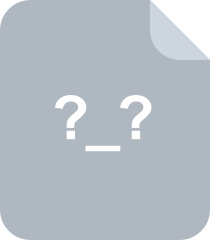
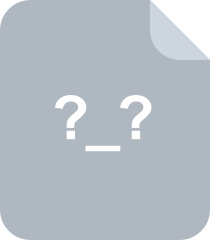
共 175 条
- 1
- 2
资源评论
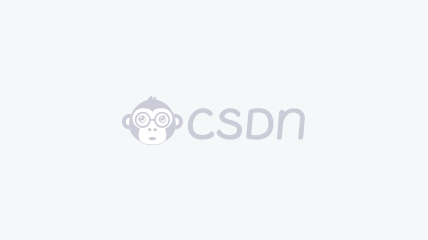

pk_xz123456
- 粉丝: 2064
- 资源: 1356

下载权益

C知道特权

VIP文章

课程特权

开通VIP
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

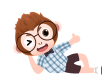
最新资源
- Java《基于springboot框架搭建的B2C商城》+项目源码+文档说明
- 【小程序毕业设计】面向企事业单位的项目申报小程序源码(完整前后端+mysql+说明文档+LW).zip
- 【小程序毕业设计】论坛小程序源码(完整前后端+mysql+说明文档).zip
- Java《基于SSM的高校共享单车管理系统》+项目源码+文档说明
- 【小程序毕业设计】讲座预约系统微信小程序源码(完整前后端+mysql+说明文档+LW).zip
- 【小程序毕业设计】驾校报名小程序源码(完整前后端+mysql+说明文档+LW).zip
- 程序设计竞赛-在线判题系统(OJ系统)【含Web端+判题端】+项目源码+文档说明
- 大数据时代下短视频观看行为数据采集与分析的设计与实现
- 【小程序毕业设计】图书馆座位再利用系统源码(完整前后端+mysql+说明文档).zip
- 【小程序毕业设计】自习室预约系统源码(完整前后端+mysql+说明文档).zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


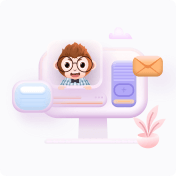
安全验证
文档复制为VIP权益,开通VIP直接复制
