/*
* Filename: EditEx.h
* Description: CEditEx class implementation
* Copyright: Julijan 較ibar, 2011
*
* This software is provided 'as-is', without any express or implied
* warranty. In no event will the author(s) be held liable for any damages
* arising from the use of this software.
*
* Permission is granted to anyone to use this software for any purpose,
* including commercial applications, and to alter it and redistribute it
* freely, subject to the following restrictions:
*
* 1. The origin of this software must not be misrepresented; you must not
* claim that you wrote the original software. If you use this software
* in a product, an acknowledgment in the product documentation would be
* appreciated but is not required.
* 2. Altered source versions must be plainly marked as such, and must not be
* misrepresented as being the original software.
* 3. This notice may not be removed or altered from any source distribution.
*/
#include "stdafx.h"
#include "EditEx.h"
//////////////////////////////////////////////////////////////////////////
// CEditEx::CInsertTextCommand
CEditEx::CInsertTextCommand::CInsertTextCommand(CEditEx* const pEditControl, const CString& text)
: CEditExCommand(pEditControl)
, m_text(text)
{
int nEnd;
pEditControl->GetSel(m_nStart, nEnd);
}
void CEditEx::CInsertTextCommand::DoUndo()
{
m_pEditControl->DeleteText(m_nStart, m_text.GetLength());
m_pEditControl->SetSel(m_nStart, m_nStart);
}
void CEditEx::CInsertTextCommand::DoExecute()
{
m_pEditControl->InsertText(m_text, m_nStart);
int nEnd = m_nStart + m_text.GetLength();
m_pEditControl->SetSel(nEnd, nEnd);
}
//////////////////////////////////////////////////////////////////////////
// CEditEx::CDeleteSelectionCommand
CEditEx::CDeleteSelectionCommand::CDeleteSelectionCommand(CEditEx* const pEditControl, CursorOnUndo cursorOnUndo)
: CEditExCommand(pEditControl)
, m_textDeleted(pEditControl->GetSelectionText())
{
m_pEditControl->GetSel(m_nStart, m_nEnd);
m_cursorOnUndo = cursorOnUndo;
}
void CEditEx::CDeleteSelectionCommand::DoUndo()
{
m_pEditControl->InsertText(m_textDeleted, m_nStart);
switch (m_cursorOnUndo)
{
case Selection:
m_pEditControl->SetSel(m_nStart, m_nEnd);
break;
case End:
m_pEditControl->SetSel(m_nEnd, m_nEnd);
break;
case Start:
m_pEditControl->SetSel(m_nStart, m_nStart);
break;
default:
ASSERT(FALSE);
break;
}
}
void CEditEx::CDeleteSelectionCommand::DoExecute()
{
m_pEditControl->DeleteText(m_nStart, m_nEnd - m_nStart);
m_pEditControl->SetSel(m_nStart, m_nStart);
}
//////////////////////////////////////////////////////////////////////////
// CEditEx::CDeleteCharacterCommand
CEditEx::CDeleteCharacterCommand::CDeleteCharacterCommand(CEditEx* pEditControl, bool isBackspace)
: CEditExCommand(pEditControl)
, m_isBackspace(isBackspace)
{
ASSERT(m_pEditControl->IsSelectionEmpty());
m_pEditControl->GetSel(m_nStart, m_nStart);
CString text;
m_pEditControl->GetWindowText(text);
if (m_isBackspace)
--m_nStart;
m_charDeleted = text[m_nStart];
}
void CEditEx::CDeleteCharacterCommand::DoUndo()
{
m_pEditControl->InsertChar(m_charDeleted, m_nStart);
if (m_isBackspace)
m_pEditControl->SetSel(m_nStart + 1, m_nStart + 1);
else
m_pEditControl->SetSel(m_nStart, m_nStart);
}
void CEditEx::CDeleteCharacterCommand::DoExecute()
{
m_pEditControl->DeleteText(m_nStart, 1);
m_pEditControl->SetSel(m_nStart, m_nStart);
}
//////////////////////////////////////////////////////////////////////////
// CEditEx::CReplaceSelectionCommand
CEditEx::CReplaceSelectionCommand::CReplaceSelectionCommand(CEditEx* const pEditControl, const CString& text)
: CEditExCommand(pEditControl)
{
ASSERT(pEditControl->IsSelectionEmpty() == false);
m_pDeleteCommand = new CDeleteSelectionCommand(pEditControl, CDeleteSelectionCommand::Selection);
m_pInsertCommand = new CInsertTextCommand(pEditControl, text);
ASSERT(m_pDeleteCommand != NULL);
ASSERT(m_pInsertCommand != NULL);
}
CEditEx::CReplaceSelectionCommand::~CReplaceSelectionCommand()
{
delete m_pDeleteCommand;
delete m_pInsertCommand;
}
void CEditEx::CReplaceSelectionCommand::DoUndo()
{
m_pInsertCommand->Undo();
m_pDeleteCommand->Undo();
}
void CEditEx::CReplaceSelectionCommand::DoExecute()
{
m_pDeleteCommand->Execute();
m_pInsertCommand->Execute();
}
//////////////////////////////////////////////////////////////////////////
// CEditEx::CCommandHistory
CEditEx::CCommandHistory::CCommandHistory()
{
}
CEditEx::CCommandHistory::~CCommandHistory()
{
DestroyUndoCommands();
DestroyRedoCommands();
}
void CEditEx::CCommandHistory::AddCommand(CEditExCommand* pCommand)
{
m_undoCommands.push(pCommand);
DestroyRedoCommands();
}
bool CEditEx::CCommandHistory::Undo()
{
if (!CanUndo())
return false;
CEditExCommand* pCommand = m_undoCommands.top();
pCommand->Undo();
m_undoCommands.pop();
m_redoCommands.push(pCommand);
return true;
}
bool CEditEx::CCommandHistory::Redo()
{
if (!CanRedo())
return false;
CEditExCommand* pCommand = m_redoCommands.top();
pCommand->Execute();
m_redoCommands.pop();
m_undoCommands.push(pCommand);
return true;
}
bool CEditEx::CCommandHistory::CanUndo() const
{
return m_undoCommands.empty() == false;
}
bool CEditEx::CCommandHistory::CanRedo() const
{
return m_redoCommands.empty() == false;
}
void CEditEx::CCommandHistory::DestroyCommands(CommandStack& stack)
{
while (!stack.empty())
{
CEditExCommand* pCommand = stack.top();
delete pCommand;
stack.pop();
}
}
void CEditEx::CCommandHistory::DestroyUndoCommands()
{
DestroyCommands(m_undoCommands);
}
void CEditEx::CCommandHistory::DestroyRedoCommands()
{
DestroyCommands(m_redoCommands);
}
//////////////////////////////////////////////////////////////////////////
// CEditEx implementation
IMPLEMENT_DYNAMIC(CEditEx, CEdit)
CEditEx::CEditEx()
{
m_contextMenuShownFirstTime = false;
}
CEditEx::~CEditEx()
{
}
// public methods
inline bool CEditEx::IsMultiLine() const
{
return (GetStyle() & ES_MULTILINE) == ES_MULTILINE;
}
inline bool CEditEx::IsSelectionEmpty() const
{
int nStart, nEnd;
GetSel(nStart, nEnd);
return nEnd == nStart;
}
int CEditEx::GetCursorPosition() const
{
ASSERT(IsSelectionEmpty());
return GetSel() & 0xFFFF;
}
CString CEditEx::GetSelectionText() const
{
CString text;
GetWindowText(text);
int nStart, nEnd;
GetSel(nStart, nEnd);
return text.Mid(nStart, nEnd - nStart);
}
void CEditEx::ReplaceSel(LPCTSTR lpszNewText, BOOL bCanUndo /*= FALSE*/)
{
if (bCanUndo)
CreateInsertTextCommand(lpszNewText);
CEdit::ReplaceSel(lpszNewText, bCanUndo);
}
BOOL CEditEx::Undo()
{
return m_commandHistory.Undo();
}
BOOL CEditEx::Redo()
{
return m_commandHistory.Redo();
}
BOOL CEditEx::CanUndo() const
{
return m_commandHistory.CanUndo();
}
BOOL CEditEx::CanRedo() const
{
return m_commandHistory.CanRedo();
}
// command pattern receiver methods
void CEditEx::InsertText(const CString& textToInsert, int nStart)
{
ASSERT(nStart <= GetWindowTextLength());
CString text;
GetWindowText(text);
text.Insert(nStart, textToInsert);
SetWindowText(text);
}
void CEditEx::InsertChar(TCHAR charToInsert, int nStart)
{
ASSERT(nStart <= GetWindowTextLength());
CString text;
GetWindowText(text);
text.Insert(nStart, charToInsert);
SetWindowText(text);
}
void CEditEx::DeleteText(int nStart, int nLen)
{
ASSERT(nLen > 0);
ASSERT(GetWindowTextLength() > 0);
CString text;
GetWindowText(text);
text.Delete(nStart, nLen);
SetWindowText(text);
}
// CEditEx overrides
// intercept commands to add them to the history
BOOL CEditEx::PreTranslateMessage(MSG* pMsg)
{
switch (pMsg->message)
{
case WM_KEYDOWN:
i
没有合适的资源?快使用搜索试试~ 我知道了~
资源详情
资源评论
资源推荐
收起资源包目录





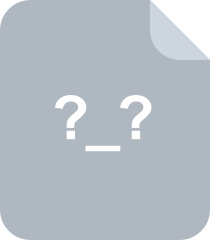
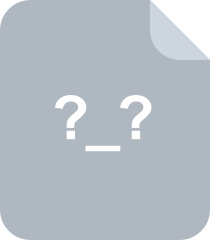

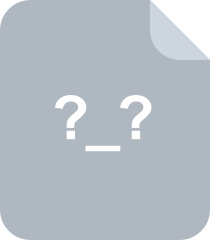

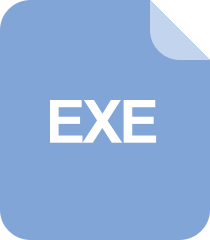

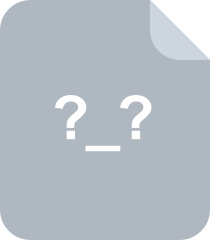
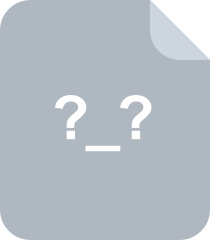
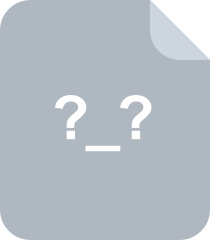
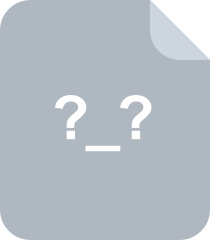

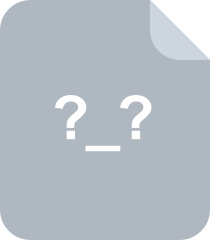
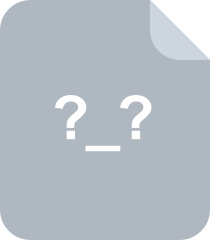
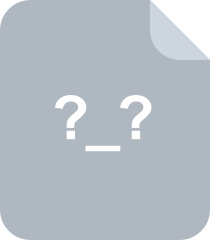
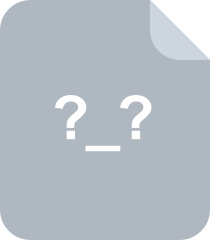
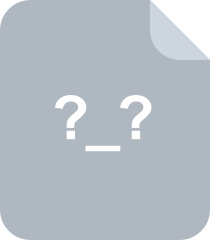
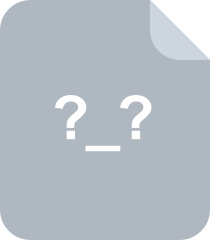
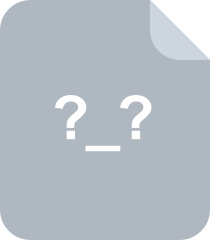
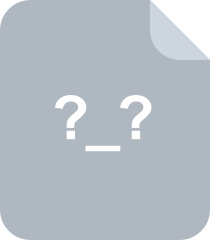
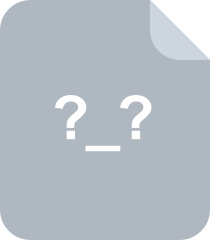
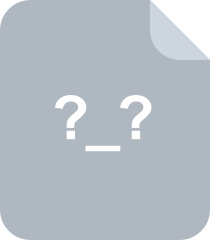
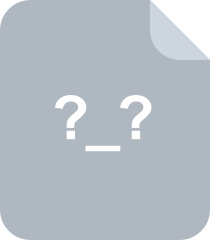
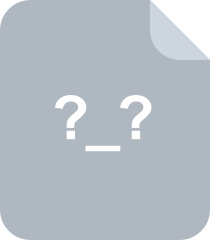

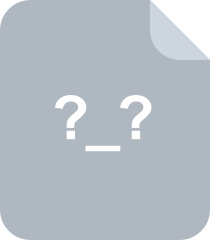
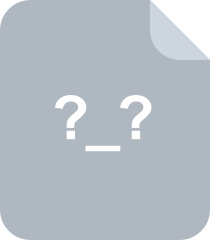
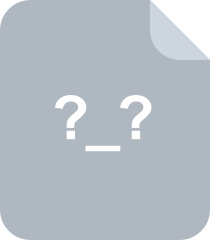
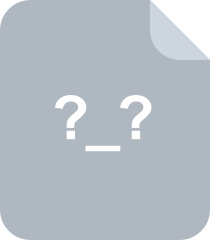
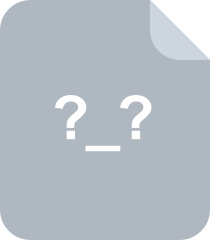
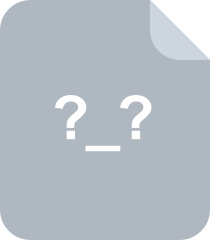
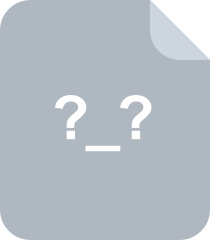
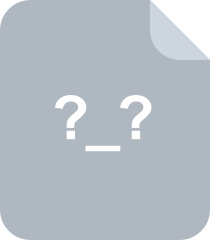
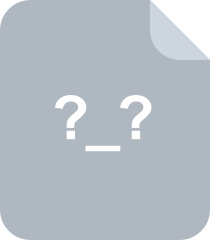

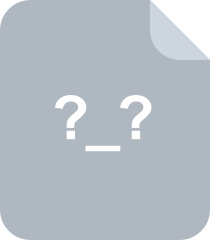
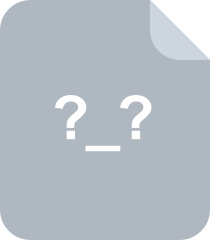
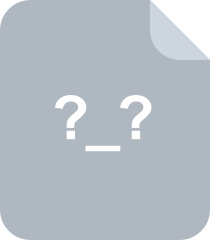
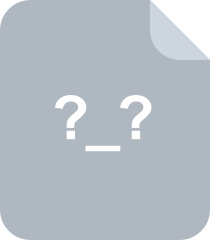
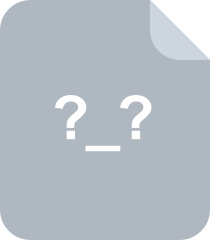
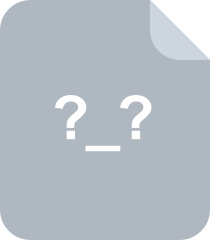
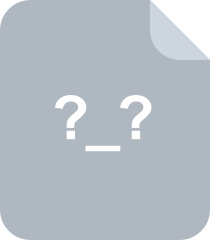
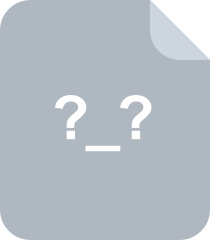
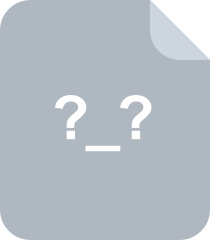
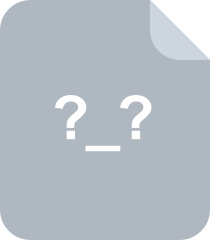
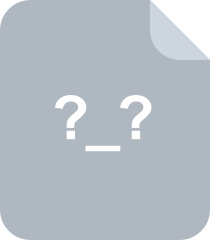
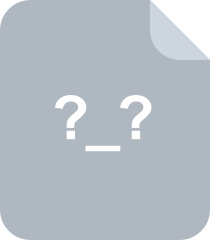
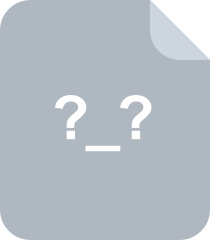
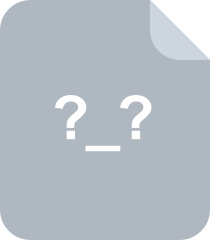


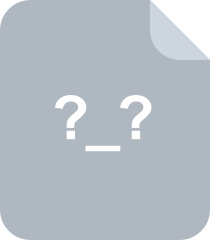
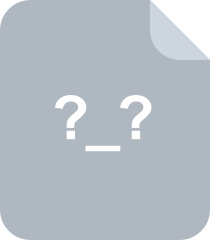

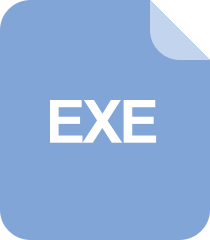
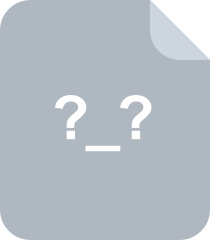
共 47 条
- 1
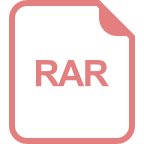
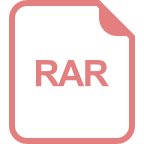
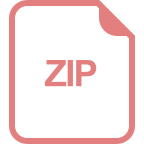
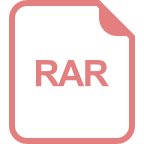
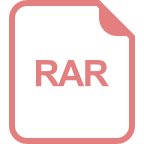
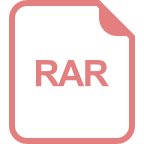
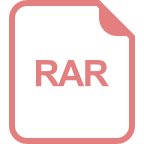
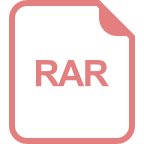
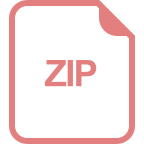
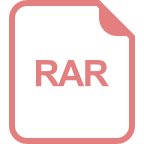
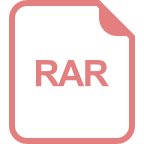
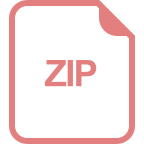
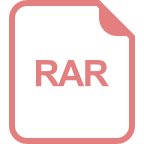
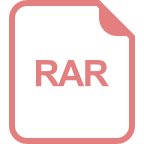
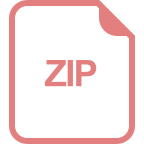
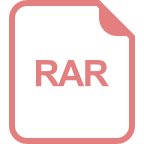
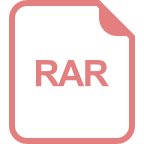
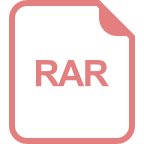
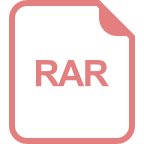
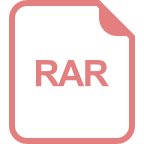
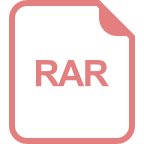
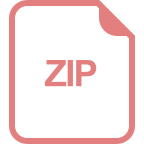
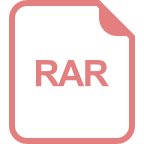
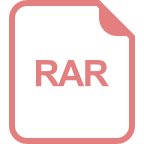
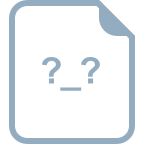
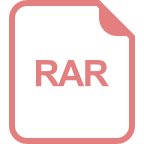

五一编程
- 粉丝: 1w+
- 资源: 882
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

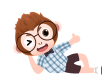
最新资源
- COMSOL中三相变压器电磁场部分
- COMSOL中场路耦合实现及方法对比(使用电路接口、全局方程分别实现电磁场的场路耦合)
- 基于YOLO的轴承生产缺陷检测,数据集大小568张,类别三类
- C#asp.net问卷调查系统源码数据库 SQL2008源码类型 WebForm
- wiwf-web-manage
- PUBG MOBILE CHINA.html
- C语言毕设项目之基于C51芯片单片机设计的简易交通灯控制系统.zip
- C#ASP.NET最新版基于知识树的多课程网络教学平台源码数据库 SQL2008源码类型 WebForm
- 基于C++控制台(Windows平台)的一个吃豆人小游戏.zip
- C++ primer 习题上半部分
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


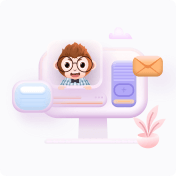
安全验证
文档复制为VIP权益,开通VIP直接复制

评论0