/////////////////////////////////////////////////////////////////////////
//
// imagereader.cpp --a part of libdecodeqr
//
// Copyright(C) 2007 NISHI Takao <zophos@koka-in.org>
// JMA (Japan Medical Association)
// NaCl (Network Applied Communication Laboratory Ltd.)
//
// This is free software with ABSOLUTELY NO WARRANTY.
// You can redistribute and/or modify it under the terms of LGPL.
//
// $Id: imagereader.cpp 36 2007-02-21 23:22:03Z zophos $
//
#include "imagereader.h"
namespace Qr{
//
// avoid cvBoundingRect() fxxcin' bug.
//
typedef struct{
CvRect feret;
CvSeq *contour;
} ImageReaderCandidate;
/////////////////////////////////////////////////////////////////////
//
//
//
ImageReader::ImageReader()
{
this->_init();
}
ImageReader::ImageReader(int width,int height,int depth,int channel)
{
this->_init();
this->_alloc_image(width,height,depth,channel);
}
ImageReader::~ImageReader()
{
if(this->qr)
delete this->qr;
if(this->_seq_code_area_contour)
cvRelease((void **)&this->_seq_code_area_contour);
cvRelease((void **)&this->_seq_finder_pattern);
cvReleaseMemStorage(&this->_stor_tmp);
cvReleaseMemStorage(&this->_stor);
this->release_image();
}
void ImageReader::_init()
{
memset(this->_coderegion_vertexes,0,sizeof(CvPoint)*4);
memset(this->_finderpattern_boxes,0,sizeof(CvBox2D)*3);
this->_img_src_internal=NULL;
this->_img_src=NULL;
this->_img_transformed=NULL;
this->_img_binarized=NULL;
this->_img_tmp_1c=NULL;
this->_stor=cvCreateMemStorage(0);
this->_stor_tmp=cvCreateMemStorage(0);
this->_seq_finder_pattern=cvCreateSeq(CV_SEQ_ELTYPE_GENERIC,
sizeof(CvSeq),
sizeof(CvBox2D),
this->_stor);
this->_seq_code_area_contour=NULL;
this->status=0;
this->qr=NULL;
}
void ImageReader::_alloc_image(int width,int height,int depth,int channel)
{
this->_img_src_internal=cvCreateImage(cvSize(width,height),
depth,channel);
cvZero(this->_img_src_internal);
this->_img_src=this->_img_src_internal;
this->_img_transformed=cvCloneImage(this->_img_src);
this->_img_binarized=cvCreateImage(cvSize(this->_img_src->width,
this->_img_src->height),
IPL_DEPTH_8U,1);
cvZero(this->_img_binarized);
this->_img_tmp_1c=cvCloneImage(this->_img_binarized);
}
IplImage *ImageReader::set_image(IplImage *src)
{
this->release_image();
this->_img_src=src;
this->_img_transformed=cvCloneImage(this->_img_src);
this->_img_binarized=cvCreateImage(cvSize(this->_img_src->width,
this->_img_src->height),
IPL_DEPTH_8U,1);
cvZero(this->_img_binarized);
this->_img_tmp_1c=cvCloneImage(this->_img_binarized);
return(this->_img_src);
}
IplImage *ImageReader::set_image(int width,int height,
int depth,int channel)
{
this->release_image();
this->_alloc_image(width,height,depth,channel);
return(this->_img_src);
}
void ImageReader::release_image()
{
if(this->_img_tmp_1c)
cvReleaseImage(&this->_img_tmp_1c);
if(this->_img_binarized)
cvReleaseImage(&this->_img_binarized);
if(this->_img_transformed)
cvReleaseImage(&this->_img_transformed);
if(this->_img_src_internal)
cvReleaseImage(&this->_img_src_internal);
this->_img_tmp_1c=NULL;
this->_img_binarized=NULL;
this->_img_transformed=NULL;
this->_img_src_internal=NULL;
this->_img_src=NULL;
}
IplImage *ImageReader::src_buffer()
{
return(this->_img_src);
}
IplImage *ImageReader::transformed_buffer()
{
return(this->_img_transformed);
}
IplImage *ImageReader::binarized_buffer()
{
return(this->_img_binarized);
}
IplImage *ImageReader::tmp_buffer()
{
return(this->_img_tmp_1c);
}
CvPoint *ImageReader::coderegion_vertexes()
{
return(this->_coderegion_vertexes);
}
CvBox2D *ImageReader::finderpattern_boxes()
{
return(this->_finderpattern_boxes);
}
Qr *ImageReader::decode(int adaptive_th_size,
int adaptive_th_delta)
{
if(this->status&QR_IMAGEREADER_WORKING)
return(NULL);
if(!this->_img_src){
this->status=(QR_IMAGEREADER_ERROR|
QR_IMAGEREADER_NOT_INVALID_SRC_IMAGE);
return(NULL);
}
this->status=QR_IMAGEREADER_WORKING;
cvResetImageROI(this->_img_transformed);
cvResetImageROI(this->_img_binarized);
cvResetImageROI(this->_img_tmp_1c);
Qr *ret=this->_decode(adaptive_th_size,adaptive_th_delta);
this->status^=QR_IMAGEREADER_WORKING;
return(ret);
}
Qr *ImageReader::decode(IplImage *src,
int adaptive_th_size,
int adaptive_th_delta)
{
if(this->status&QR_IMAGEREADER_WORKING)
return(NULL);
this->status=QR_IMAGEREADER_WORKING;
this->set_image(src);
Qr *ret=this->_decode(adaptive_th_size,adaptive_th_delta);
this->_img_src=NULL;
this->status^=QR_IMAGEREADER_WORKING;
return(ret);
}
Qr *ImageReader::_decode(int adaptive_th_size,int adaptive_th_delta)
{
if(this->qr){
delete this->qr;
this->qr=NULL;
}
memset(this->_coderegion_vertexes,0,sizeof(CvPoint)*4);
memset(this->_finderpattern_boxes,0,sizeof(CvBox2D)*3);
//
// binarize
//
if(this->_img_src->nChannels>1)
cvCvtColor(this->_img_src,this->_img_tmp_1c,CV_BGR2GRAY);
else
cvCopy(this->_img_src,this->_img_tmp_1c);
cvSmooth(this->_img_tmp_1c,this->_img_binarized,CV_MEDIAN,3);
cvCopy(this->_img_binarized,this->_img_tmp_1c);
if(adaptive_th_size>0)
cvAdaptiveThreshold(this->_img_tmp_1c,
this->_img_binarized,
128,CV_ADAPTIVE_THRESH_MEAN_C,
CV_THRESH_BINARY_INV,
adaptive_th_size,
adaptive_th_delta);
else
cvThreshold(this->_img_tmp_1c,
this->_img_binarized,
adaptive_th_delta,
255,CV_THRESH_BINARY_INV);
//
// find finder patterns from binarized image
//
this->_find_finder_pattern();
if(this->_seq_finder_pattern->total!=3){
this->status|=(QR_IMAGEREADER_ERROR|
QR_IMAGEREADER_NOT_FOUND_FINDER_PATTERN);
return(NULL);
}
//
// find code area from binarized image using finder patterns
//
this->_find_code_area_contour(FIND_CODE_AREA_POLY_APPROX_TH);
if(!this->_seq_code_area_contour||
this->_seq_code_area_contour->total!=4){
this->status|=(QR_IMAGEREADER_ERROR|
QR_IMAGEREADER_NOT_FOUND_CODE_AREA);
return(NULL);
}
//
// perspective transform from source image
//
this->_transform_image();
IplImage *code_matri
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
QR code是最近很熱門的二維條碼,常可以在網站上看到,主要用來對url、文字、電話號碼或簡訊作編碼,然後透過手機上的CMOS相機作辨識。libdecoderq是一個open source的C/C++ QR code library,可以在 http://trac.koka-in.org/libdecodeqr 下載 libdecodeqr-0.9.3.tar.bz2,不过这个网站现在已经下载不了了!
资源推荐
资源详情
资源评论
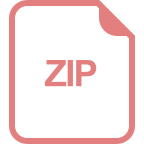
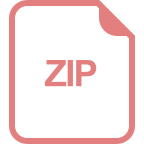
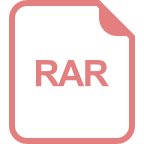
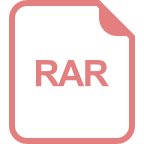
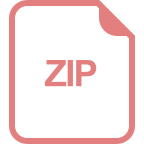
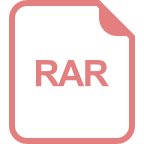
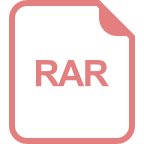
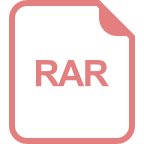
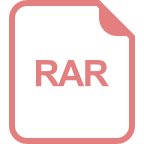
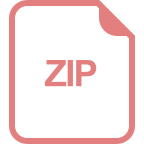
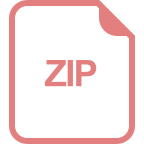
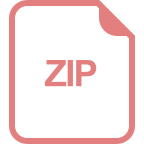
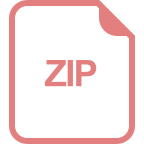
收起资源包目录



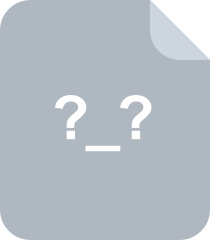
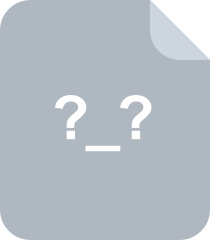

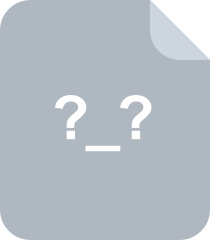

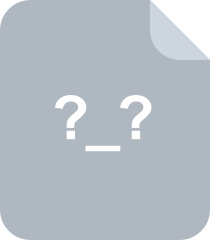
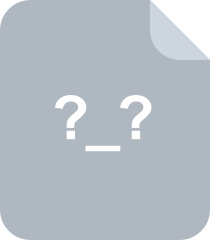
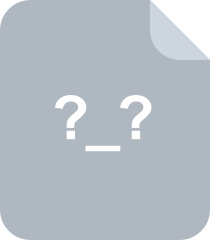
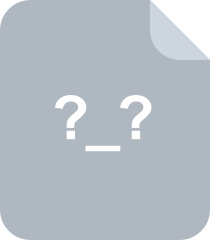
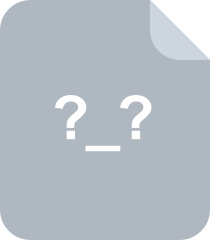
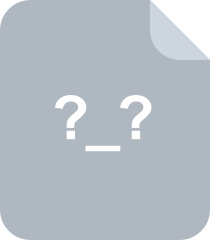

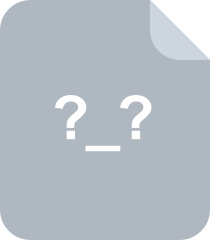
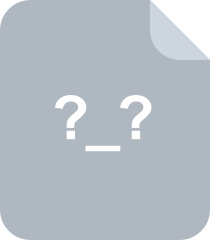
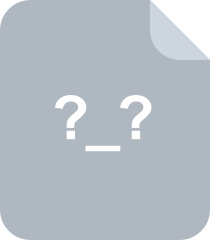
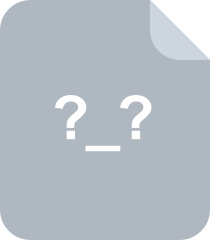
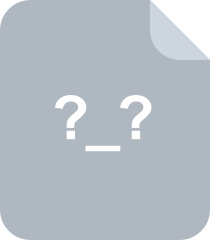
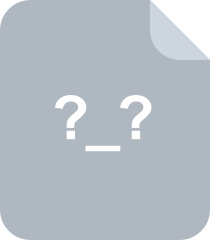
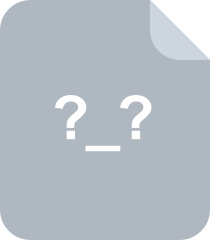
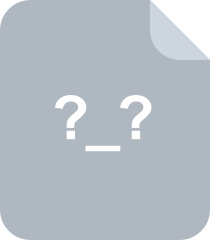

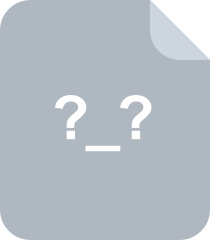
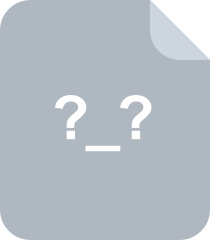
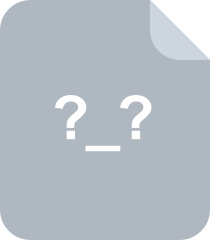
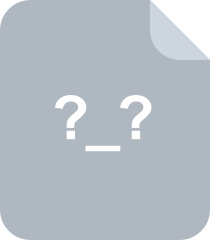
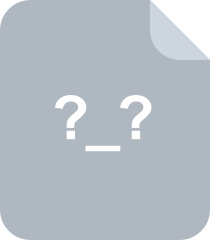
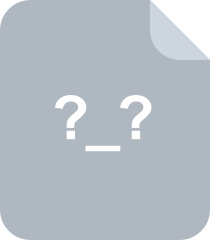
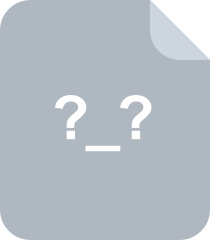
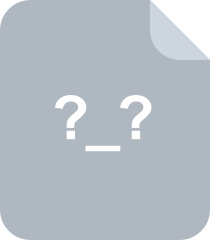
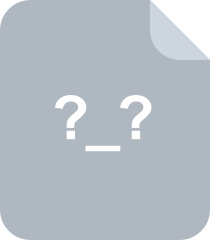
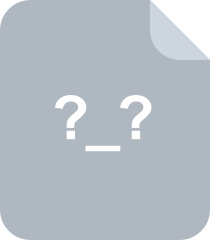
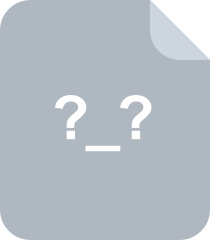
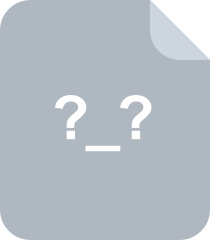
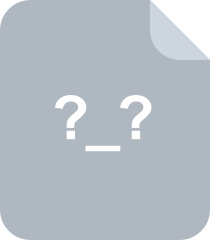
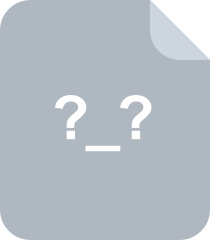
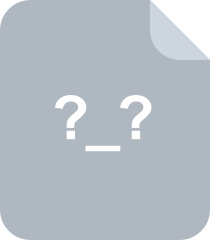
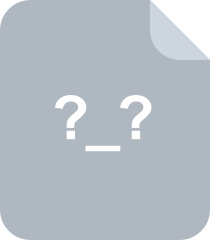
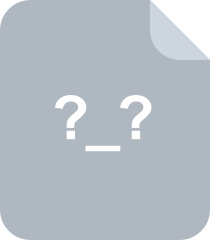
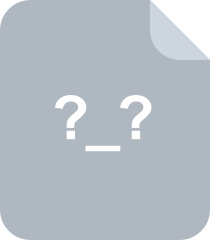
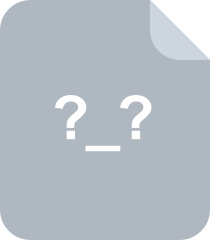
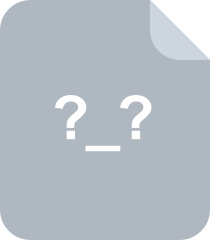
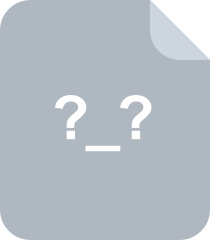
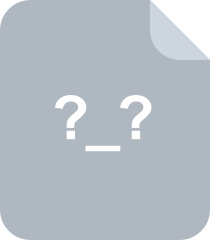
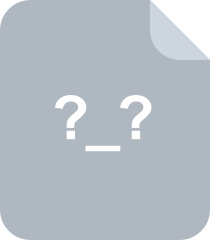
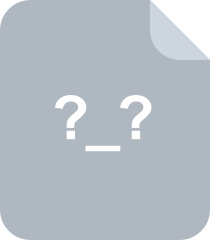

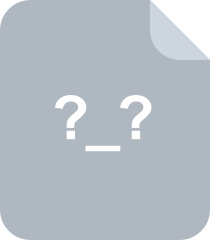
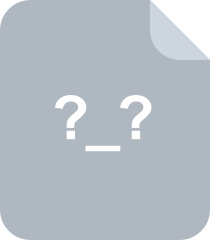
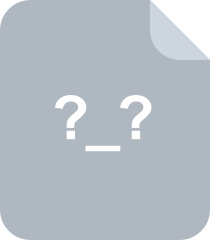

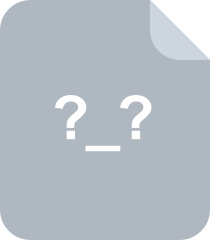
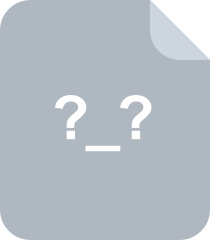
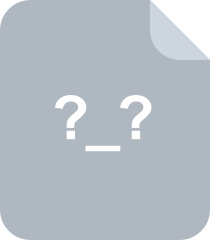
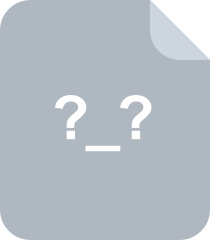

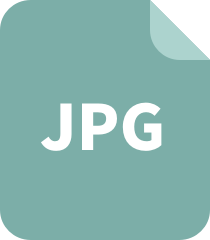
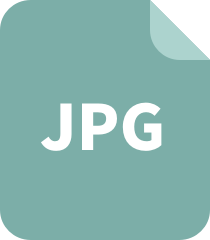
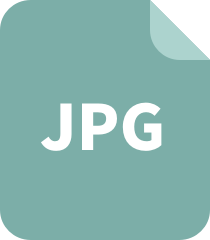
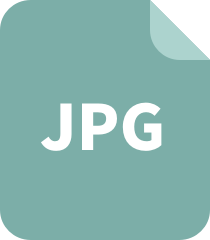
共 52 条
- 1

大自在天1
- 粉丝: 1
- 资源: 7
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

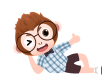
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


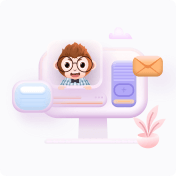
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
- 5
- 6
前往页