/*
* Copyright 1993-2011 NVIDIA Corporation. All rights reserved.
*
* NOTICE TO LICENSEE:
*
* This source code and/or documentation ("Licensed Deliverables") are
* subject to NVIDIA intellectual property rights under U.S. and
* international Copyright laws.
*
* These Licensed Deliverables contained herein is PROPRIETARY and
* CONFIDENTIAL to NVIDIA and is being provided under the terms and
* conditions of a form of NVIDIA software license agreement by and
* between NVIDIA and Licensee ("License Agreement") or electronically
* accepted by Licensee. Notwithstanding any terms or conditions to
* the contrary in the License Agreement, reproduction or disclosure
* of the Licensed Deliverables to any third party without the express
* written consent of NVIDIA is prohibited.
*
* NOTWITHSTANDING ANY TERMS OR CONDITIONS TO THE CONTRARY IN THE
* LICENSE AGREEMENT, NVIDIA MAKES NO REPRESENTATION ABOUT THE
* SUITABILITY OF THESE LICENSED DELIVERABLES FOR ANY PURPOSE. IT IS
* PROVIDED "AS IS" WITHOUT EXPRESS OR IMPLIED WARRANTY OF ANY KIND.
* NVIDIA DISCLAIMS ALL WARRANTIES WITH REGARD TO THESE LICENSED
* DELIVERABLES, INCLUDING ALL IMPLIED WARRANTIES OF MERCHANTABILITY,
* NONINFRINGEMENT, AND FITNESS FOR A PARTICULAR PURPOSE.
* NOTWITHSTANDING ANY T
ERMS OR CONDITIONS TO THE CONTRARY IN THE
* LICENSE AGREEMENT, IN NO EVENT SHALL NVIDIA BE LIABLE FOR ANY
* SPECIAL, INDIRECT, INCIDENTAL, OR CONSEQUENTIAL DAMAGES, OR ANY
* DAMAGES WHATSOEVER RESULTING FROM LOSS OF USE, DATA OR PROFITS,
* WHETHER IN AN ACTION OF CONTRACT, NEGLIGENCE OR OTHER TORTIOUS
* ACTION, ARISING OUT OF OR IN CONNECTION WITH THE USE OR PERFORMANCE
* OF THESE LICENSED DELIVERABLES.
*
* U.S. Government End Users. These Licensed Deliverables are a
* "commercial item" as that term is defined at 48 C.F.R. 2.101 (OCT
* 1995), consisting of "commercial computer software" and "commercial
* computer software documentation" as such terms are used in 48
* C.F.R. 12.212 (SEPT 1995) and is provided to the U.S. Government
* only as a commercial end item. Consistent with 48 C.F.R.12.212 and
* 48 C.F.R. 227.7202-1 through 227.7202-4 (JUNE 1995), all
* U.S. Government End Users acquire the Licensed Deliverables with
* only those rights set forth herein.
*
* Any use of the Licensed Deliverables in individual and commercial
* software must include, in the user documentation and internal
* comments to the code, the above Disclaimer and U.S. Government End
* Users Notice.
*/
/*
* This file contains example Fortran bindings for the CUBLAS library, These
* bindings have been tested with Intel Fortran 9.0 on 32-bit and 64-bit
* Windows, and with g77 3.4.5 on 32-bit and 64-bit Linux. They will likely
* have to be adjusted for other Fortran compilers and platforms.
*/
// includes standard headers
#include <ctype.h>
#include <stdio.h>
#include <string.h>
#include <stddef.h>
#include <stdlib.h>
#if defined(__GNUC__)
#include <stdint.h>
#endif
// includes cuda headers
#include <cuda_runtime.h>
// TODO: replace cublasP.h with cublas_v2.h (remove legacy cublas!)
//#include <cublas_v2.h>
#include "cublasP.h" // CUBLAS public header file
// includes fortran headers
#include <assert.h>
#include <unistd.h>
#include "fortran_common.h"
#include "fortran.h"
int NVP_MALLOC = 2;
int NVP_MEMCPY = 3;
extern cudaStream_t *stream;
/*
int THREADSYNCHRONIZE (void)
{
//cudaThreadSynchronize();
CUDA_ERROR( cudaThreadSynchronize(), "Failed to synchronize the device!" );
return 0;
}
void CUDA_ALL_STREAM_SYNCHRONIZE (void)
{
cudaStreamSynchronize (0);
}
*/
void PERCENT (int *stat, int *Size)
{
int Cpt = (int) ((*stat)/(double)(*Size) * 100);
printf("\r Evolution... %d %%", Cpt);
}
int CUBLAS_GET_ERROR (void)
{
return (int)cublasGetError();
}
void CUBLAS_XERBLA (const char *srName, int *info)
{
cublasXerbla (srName, *info);
}
/*---------------------------------------------------------------------------*/
/*---------------------------------- BLAS1 ----------------------------------*/
/*---------------------------------------------------------------------------*/
int CUBLAS_ISAMAX (const int *n, const devptr_t *devPtrx, const int *incx)
{
float *x = (float *)(*devPtrx);
int retVal;
retVal = cublasIsamax (*n, x, *incx);
return retVal;
}
int CUBLAS_ISAMIN (const int *n, const devptr_t *devPtrx, const int *incx)
{
float *x = (float *)(*devPtrx);
int retVal;
retVal = cublasIsamin (*n, x, *incx);
return retVal;
}
#if CUBLAS_FORTRAN_COMPILER==CUBLAS_G77
double CUBLAS_SASUM (const int *n, const devptr_t *devPtrx, const int *incx)
#else
float CUBLAS_SASUM (const int *n, const devptr_t *devPtrx, const int *incx)
#endif
{
float *x = (float *)(*devPtrx);
float retVal;
retVal = cublasSasum (*n, x, *incx);
return retVal;
}
void CUBLAS_SAXPY (const int *n, const float *alpha, const devptr_t *devPtrx,
const int *incx, const devptr_t *devPtry, const int *incy)
{
float *x = (float *)(*devPtrx);
float *y = (float *)(*devPtry);
cublasSaxpy (*n, *alpha, x, *incx, y, *incy);
}
void CUBLAS_SCOPY (const int *n, const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy)
{
float *x = (float *)(*devPtrx);
float *y = (float *)(*devPtry);
cublasScopy (*n, x, *incx, y, *incy);
}
#if CUBLAS_FORTRAN_COMPILER==CUBLAS_G77
double CUBLAS_SDOT (const int *n, const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy)
#else
float CUBLAS_SDOT (const int *n, const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy)
#endif
{
float *x = (float *)(*devPtrx);
float *y = (float *)(*devPtry);
float res = cublasSdot (*n, x, *incx, y, *incy);
return res;
}
#if CUBLAS_FORTRAN_COMPILER==CUBLAS_G77
double CUBLAS_SNRM2 (const int *n, const devptr_t *devPtrx, const int *incx)
#else
float CUBLAS_SNRM2 (const int *n, const devptr_t *devPtrx, const int *incx)
#endif
{
float *x = (float *)(*devPtrx);
float res = cublasSnrm2 (*n, x, *incx);
return res;
}
void CUBLAS_SROT (const int *n, const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy, const float *sc,
const float *ss)
{
float *x = (float *)(*devPtrx);
float *y = (float *)(*devPtry);
cublasSrot (*n, x, *incx, y, *incy, *sc, *ss);
}
void CUBLAS_SROTG (float *sa, float *sb, float *sc, float *ss)
{
cublasSrotg (sa, sb, sc, ss);
}
void CUBLAS_SROTM (const int *n, const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy,
const float* sparam)
{
float *x = (float *)(*devPtrx);
float *y = (float *)(*devPtry);
cublasSrotm (*n, x, *incx, y, *incy, sparam);
}
void CUBLAS_SROTMG (float *sd1, float *sd2, float *sx1, const float *sy1,
float* sparam)
{
cublasSrotmg (sd1, sd2, sx1, sy1, sparam);
}
void CUBLAS_SSCAL (const int *n, const float *alpha, const devptr_t *devPtrx,
const int *incx)
{
float *x = (float *)(*devPtrx);
cublasSscal (*n, *alpha, x, *incx);
}
void CUBLAS_SSWAP (const int *n, const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy)
{
float *x = (float *)(*devPtrx);
float *y = (float *)(*devPtry);
cublasSswap (*n, x, *incx, y, *incy);
}
void CUBLAS_CAXPY (const int *n, const cuComplex *alpha,
const devptr_t *devPtrx, const int *incx,
const devptr_t *devPtry, const int *incy)
{
cuComplex *x = (cuComplex *)(*devPtrx);
cuComplex *y = (cuComplex *)(*devPtry);
cublasCaxpy (*n, *alpha, x, *incx, y, *incy);
}
void CUBLAS_ZAXPY (const int *n, const cuDoubleComplex *alpha,
const devptr_t *devPtrx, const int *incx,
const devptr_t *
没有合适的资源?快使用搜索试试~ 我知道了~
vasp.5.4.1.05Feb16.tar.gz

温馨提示
VASP全称Vienna Ab-initio Simulation Package VASP是维也纳大学Hafner小组开发的进行电子结构计算和量子力学-分子动力学模拟软件包。它是目前材料模拟和计算物质科学研究中最流行的商用软件之一。
资源推荐
资源详情
资源评论
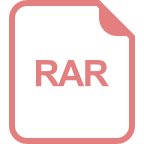
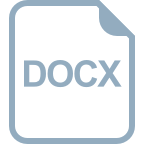
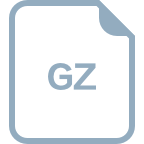
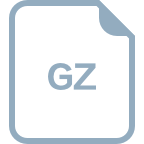
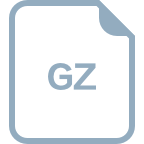
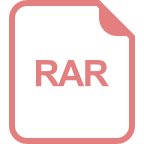
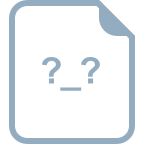
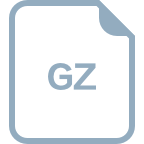
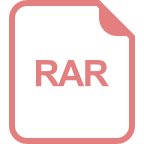
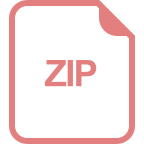
收起资源包目录

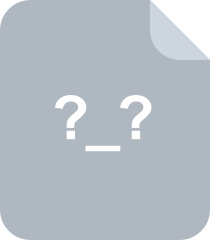
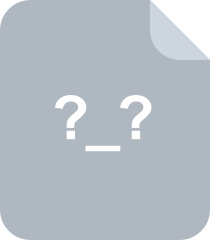
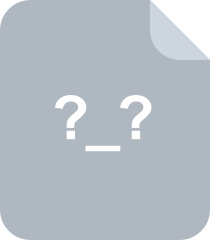
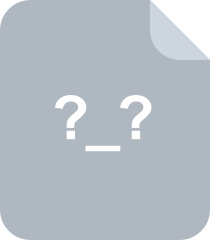
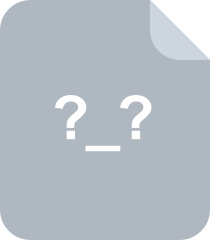
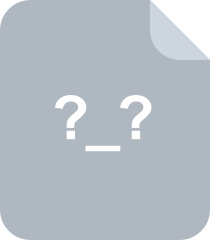
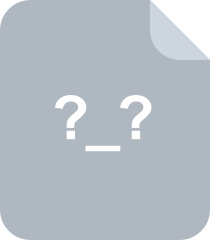
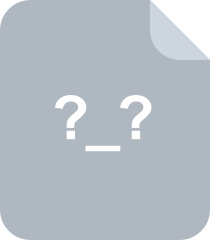
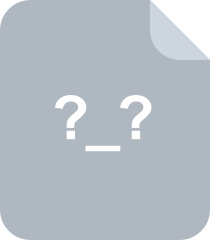
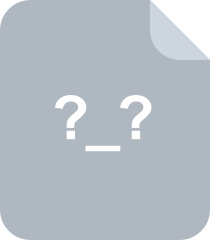
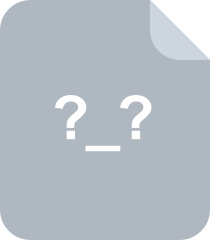
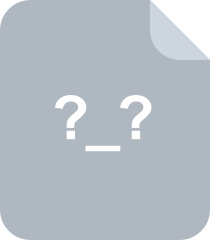
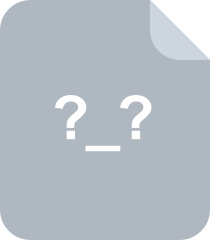
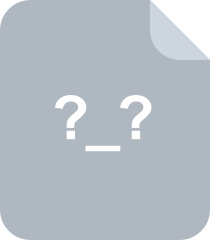
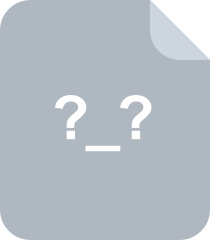
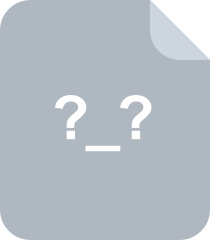
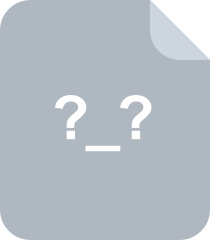
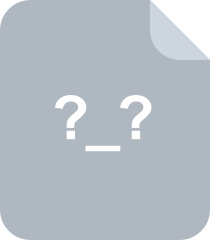
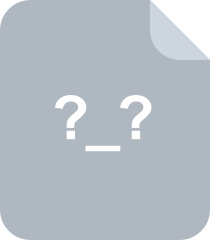
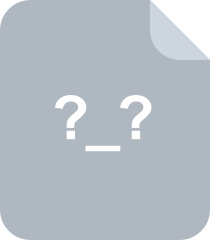
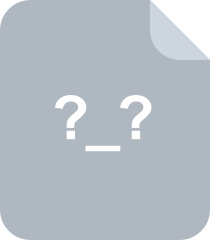
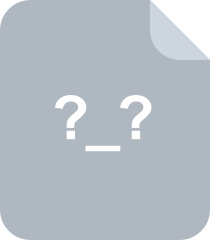
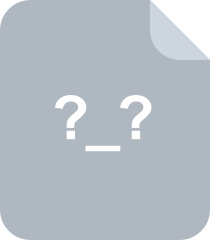
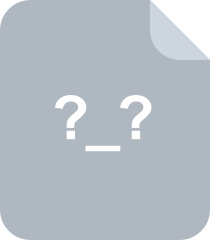
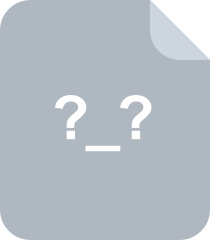
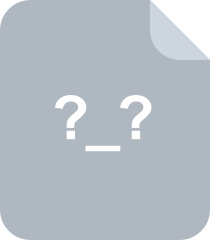
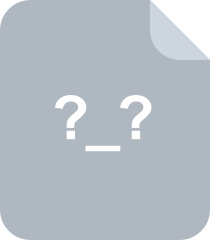
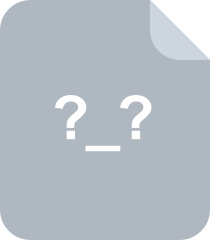
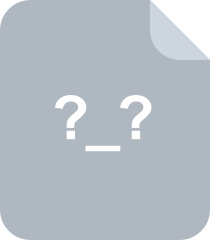
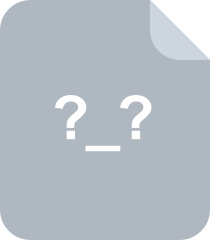
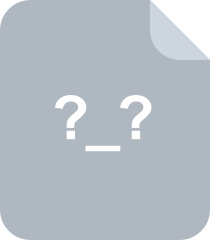
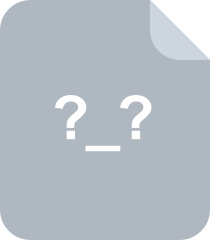
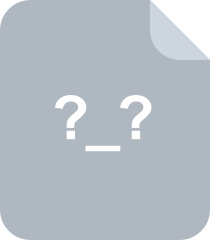
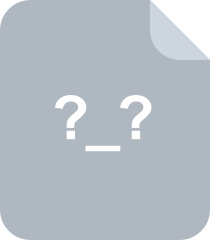
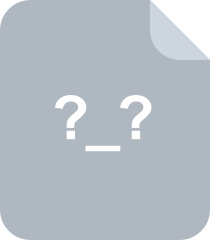
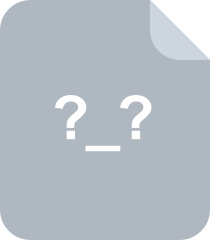
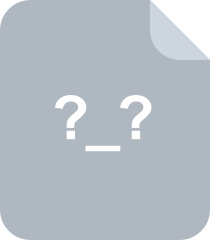
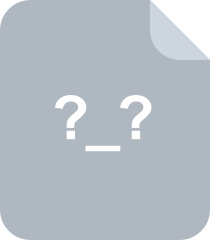
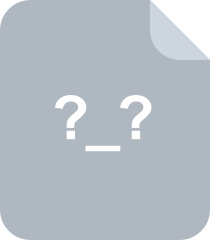
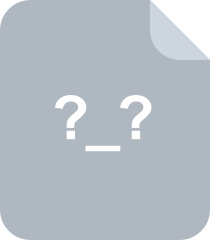
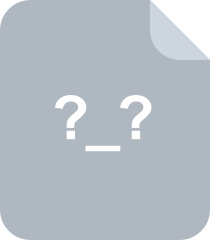
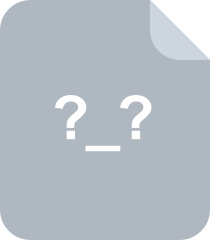
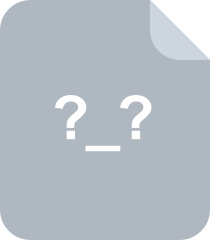
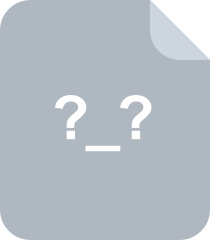
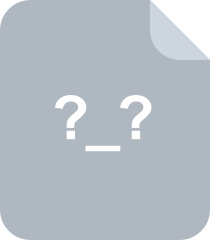
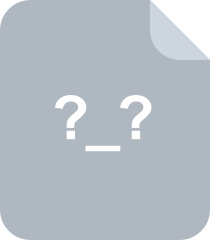
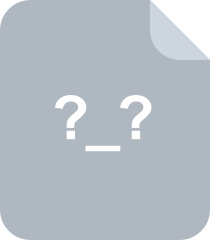
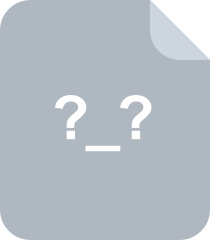
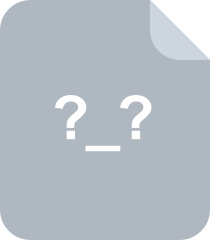
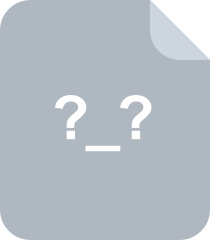
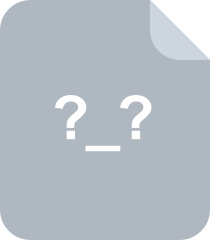
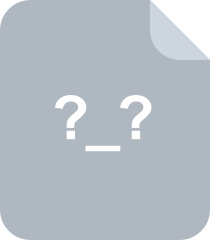
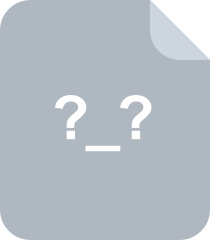
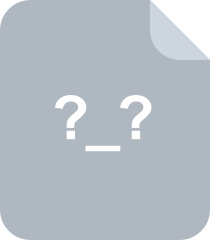
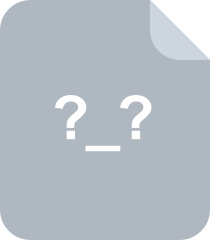
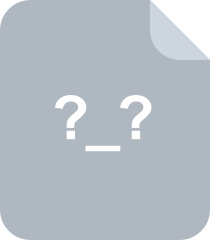
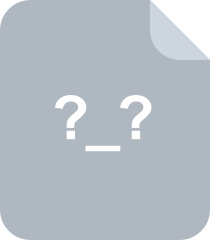
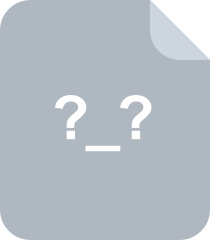
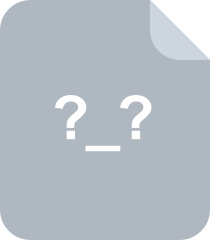
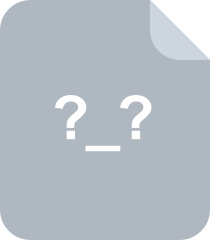
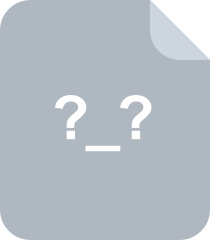
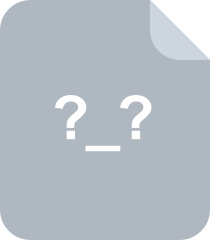
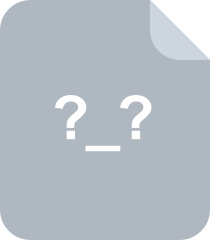
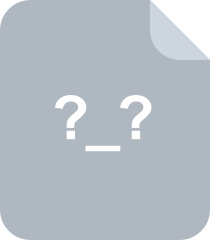
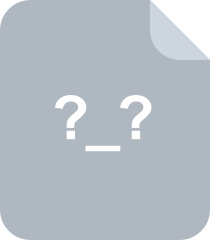
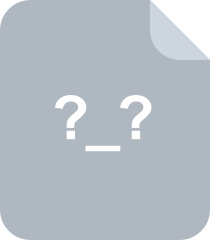
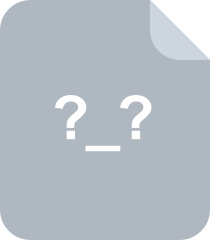
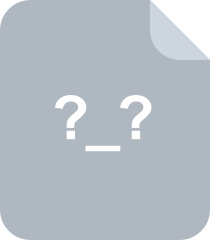
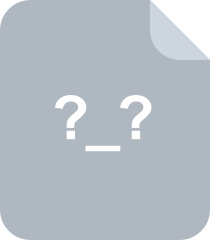
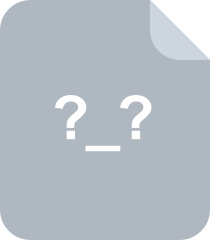
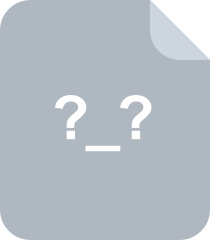
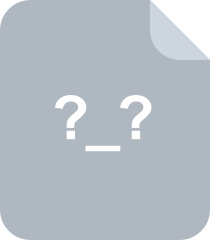
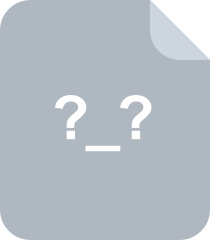
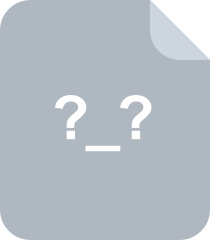
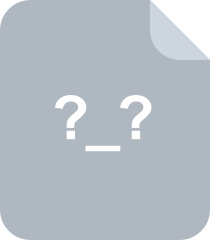
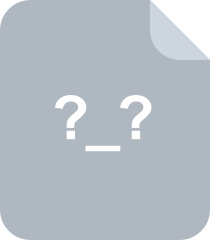
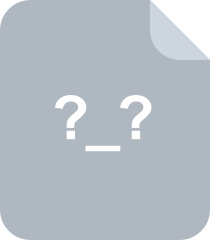
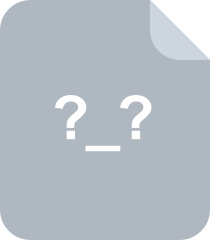
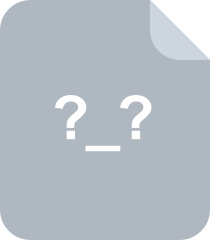
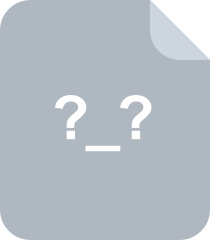
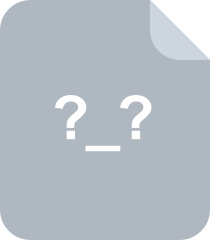
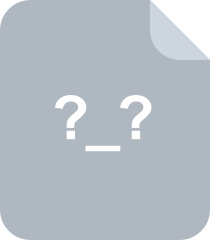
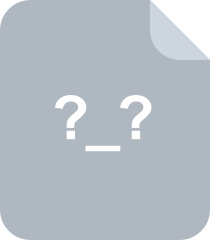
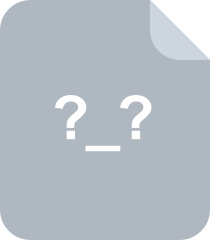
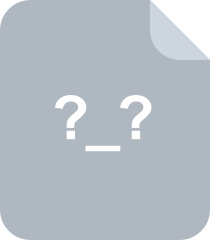
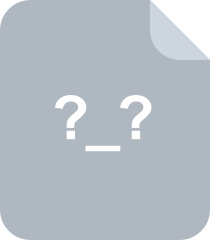
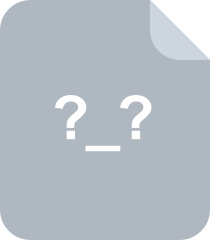
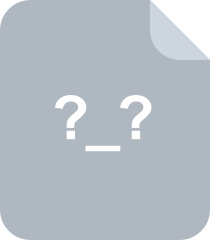
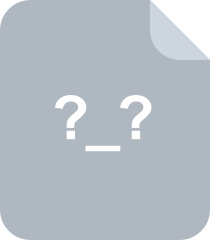
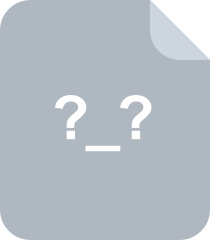
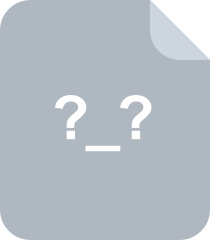
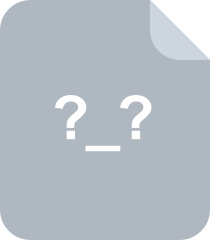
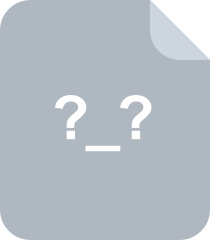
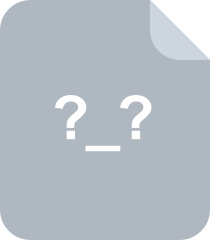
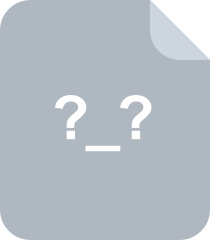
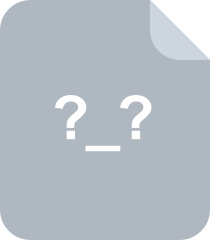
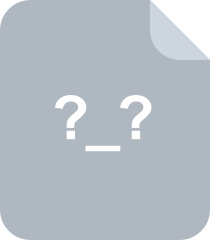
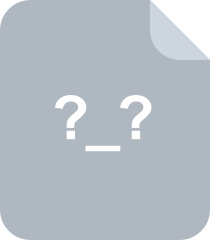
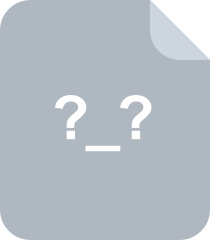
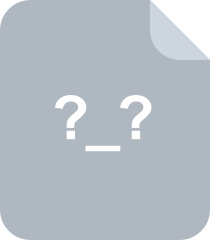
共 317 条
- 1
- 2
- 3
- 4
资源评论
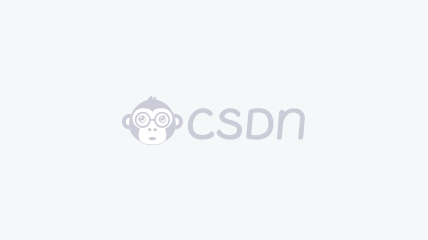
- m0_375098122019-01-13多谢分享,

heas_lin
- 粉丝: 2
- 资源: 6
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

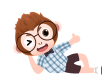
最新资源
- Nginx安装.docx
- 网络路由技术:华为设备上配置直连路由
- 【java毕业设计】交通事故档案管理系统源码(ssm+mysql+说明文档+LW).zip
- 【java毕业设计】健康管理系统源码(ssm+mysql+说明文档).zip
- 【java毕业设计】见福便利店信息管理系统源码(ssm+mysql+说明文档+LW).zip
- 信息打点技术在APP与小程序中的应用探索及实例演示
- 大学生职业生涯规划策划书.pdf
- 【java毕业设计】机房预约系统源码(ssm+mysql+说明文档+LW).zip
- 网络设备配置:交换机与路由器Telnet连接与VLAN配置的实践操作
- 信息打点与CDN绕过技术的深入剖析及应用
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


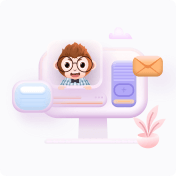
安全验证
文档复制为VIP权益,开通VIP直接复制
