没有合适的资源?快使用搜索试试~ 我知道了~
BOM浏览器对象模型,大小写转换,window窗口对象,正则表达式.
资源推荐
资源详情
资源评论
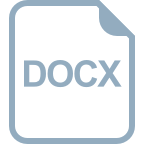
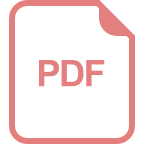
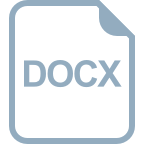
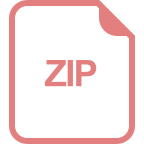
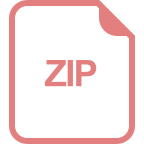
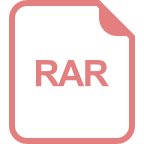
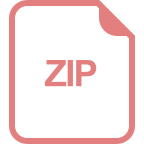
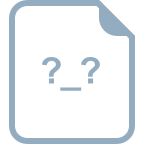
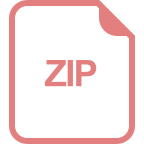
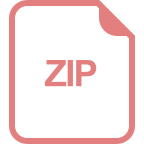
1、如果变量是一种引用类型或是null类型的,用typeof将返回“object”;null被认为是对象的占位符。
newarrivals
2、undefined是表示已声明但未赋值的变量,可以对未声明的值使用typeof,其他运算符不可以;当函数无明确返回值是
返回的也是值undefined。
http://www.gsmarena.com/sony_ericsson_xperia_arc-3619.php
3、Null类型,它只有一个专用值null,alert(null==undefined);//outputs "true"。
http://negrielectronics.com/htc-t328e-desire-x-unlocked-blue.html#.UK8VIWfpLlc
4、Number类型,边界值Number.MIN_VALUE和Number.MAX_VALUE。所有ECMAScript书都必须在这两个值之间,
不过计算生成的数可以不落在这两个数之间;isFinit(sValue)确保概述不是无穷大;isNaN()判断是不是数字,
不是的话就返回true。
5、object类,Contructor--对创建对象的函数的引用;Prototype--对该对象的对象原型的引用;
HasOwnProperty--判断对象是否有某个特定属性;IsPrototypeOf(object)--判断该对象是否为另一对象的原型;
PropertyIsEnumerable(property)--判断给定的属性是否可以用for..in语句;ToString()--返回对象的原始字符串表示;
ValueOf()--返回最适合该对象的原始值。
6、Number类,toFixed()返回具有指定位数小树的数字的字符串表示。
var oNumberObject = new Number(99);
alert(oNumberObject .toFixed(2));//outputs "99.00";
toExponential()返回的是用科学技术法表示的数字的字符串形式;
var oNumberObject = new Number(99);
alert(oNumberObject .toExponential(1));//outputs "9.9e+1";
toPrecision()根据最有意义的形式来返回数字的预定形式或字数形式,会对数字进行四舍五入;
var oNumberObject = new Number(99);
alert(oNumberObject.toPrecision(1));//outputs "le+2";
alert(oNumberObject.toPrecision(2));//outputs "99";
alert(oNumberObject.toPrecision(3));//outputs "99.0";
7、String对象的valueOf()和toString()方法都会返回String型的原始值:
var oStringObject = new String("hello world");
alert(oStringObject.valueOf() == oStringObject .toString());//outputs "true";
newarrivals
2、undefined是表示已声明但未赋值的变量,可以对未声明的值使用typeof,其他运算符不可以;当函数无明确返回值是
返回的也是值undefined。
http://www.gsmarena.com/sony_ericsson_xperia_arc-3619.php
3、Null类型,它只有一个专用值null,alert(null==undefined);//outputs "true"。
http://negrielectronics.com/htc-t328e-desire-x-unlocked-blue.html#.UK8VIWfpLlc
4、Number类型,边界值Number.MIN_VALUE和Number.MAX_VALUE。所有ECMAScript书都必须在这两个值之间,
不过计算生成的数可以不落在这两个数之间;isFinit(sValue)确保概述不是无穷大;isNaN()判断是不是数字,
不是的话就返回true。
5、object类,Contructor--对创建对象的函数的引用;Prototype--对该对象的对象原型的引用;
HasOwnProperty--判断对象是否有某个特定属性;IsPrototypeOf(object)--判断该对象是否为另一对象的原型;
PropertyIsEnumerable(property)--判断给定的属性是否可以用for..in语句;ToString()--返回对象的原始字符串表示;
ValueOf()--返回最适合该对象的原始值。
6、Number类,toFixed()返回具有指定位数小树的数字的字符串表示。
var oNumberObject = new Number(99);
alert(oNumberObject .toFixed(2));//outputs "99.00";
toExponential()返回的是用科学技术法表示的数字的字符串形式;
var oNumberObject = new Number(99);
alert(oNumberObject .toExponential(1));//outputs "9.9e+1";
toPrecision()根据最有意义的形式来返回数字的预定形式或字数形式,会对数字进行四舍五入;
var oNumberObject = new Number(99);
alert(oNumberObject.toPrecision(1));//outputs "le+2";
alert(oNumberObject.toPrecision(2));//outputs "99";
alert(oNumberObject.toPrecision(3));//outputs "99.0";
7、String对象的valueOf()和toString()方法都会返回String型的原始值:
var oStringObject = new String("hello world");
alert(oStringObject.valueOf() == oStringObject .toString());//outputs "true";
8、indexOf()和lastIndexOf()方法返回的都是指定的子串在另一个字符串中的位置(如果没找到返回-1);
localeCompare()对字符串进行排序。
var oStringObject = new String("hello");
alert(oStringObject.localeCompare("brick"));// outputs "1";
alert(oStringObject.localeCompare("hello"));// outputs "0";
alert(oStringObject.localeCompare("zero"));// outputs "-1";
slice()和substring();
alert(oStringObject.slice(1));// outputs "llo";
alert(oStringObject.substring(1));//outputs "llo";
alert(oStringObject.slice(1,2));// outputs "ll";
alert(oStringObject.substring(1,2));//outputs "ll";
alert(oStringObject.slice(1,-1));// outputs "llo";
alert(oStringObject.substring(1,-1));//outputs "h";
9、大小写转换,toLowerCase()、toLocaleLowerCase()、toUpperCase()、toLocaleUpperCase();
10、instanceof运算符确认对象为模特定类型。
11、<script type="text/javascript">
code
</script>
<script type="text/javascript">
//<![CDATA[
code
//]]>
</script>
12、BOM浏览器对象模型:window->document/frames/history/location/navigator/screen
剩余5页未读,继续阅读
资源评论
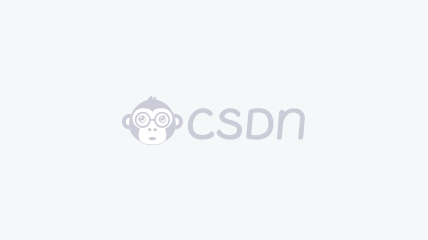

guowen321
- 粉丝: 0
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

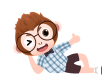
最新资源
- Screenshot_20240427_031602.jpg
- 网页PDF_2024年04月26日 23-46-14_QQ浏览器网页保存_QQ浏览器转格式(6).docx
- 直接插入排序,冒泡排序,直接选择排序.zip
- 在排序2的基础上,再次对快排进行优化,其次增加快排非递归,归并排序,归并排序非递归版.zip
- 实现了7种排序算法.三种复杂度排序.三种nlogn复杂度排序(堆排序,归并排序,快速排序)一种线性复杂度的排序.zip
- 冒泡排序 直接选择排序 直接插入排序 随机快速排序 归并排序 堆排序.zip
- 课设-内部排序算法比较 包括冒泡排序、直接插入排序、简单选择排序、快速排序、希尔排序、归并排序和堆排序.zip
- Python排序算法.zip
- C语言实现直接插入排序、希尔排序、选择排序、冒泡排序、堆排序、快速排序、归并排序、计数排序,并带图详解.zip
- 常用工具集参考用于图像等数据处理
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


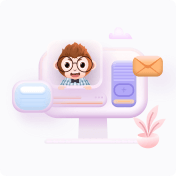
安全验证
文档复制为VIP权益,开通VIP直接复制
