(function(t,e,i){function n(i,r){var s=e[i];if(!s){var o=t[i];if(!o)return;var a={};s=e[i]={exports:a},o[0]((function(t){return n(o[1][t]||t)}),s,a)}return s.exports}for(var r=0;r<i.length;r++)n(i[r])})({1:[(function(t,e,i){"use strict";t("../core/platform/CCClass");var n=t("../core/utils/misc");cc.Action=cc.Class({name:"cc.Action",ctor:function(){this.originalTarget=null,this.target=null,this.tag=cc.Action.TAG_INVALID},clone:function(){var t=new cc.Action;return t.originalTarget=null,t.target=null,t.tag=this.tag,t},isDone:function(){return!0},startWithTarget:function(t){this.originalTarget=t,this.target=t},stop:function(){this.target=null},step:function(t){cc.logID(1006)},update:function(t){cc.logID(1007)},getTarget:function(){return this.target},setTarget:function(t){this.target=t},getOriginalTarget:function(){return this.originalTarget},setOriginalTarget:function(t){this.originalTarget=t},getTag:function(){return this.tag},setTag:function(t){this.tag=t},retain:function(){},release:function(){}}),cc.Action.TAG_INVALID=-1,cc.FiniteTimeAction=cc.Class({name:"cc.FiniteTimeAction",extends:cc.Action,ctor:function(){this._duration=0},getDuration:function(){return this._duration*(this._timesForRepeat||1)},setDuration:function(t){this._duration=t},reverse:function(){return cc.logID(1008),null},clone:function(){return new cc.FiniteTimeAction}}),cc.Speed=cc.Class({name:"cc.Speed",extends:cc.Action,ctor:function(t,e){this._speed=0,this._innerAction=null,t&&this.initWithAction(t,e)},getSpeed:function(){return this._speed},setSpeed:function(t){this._speed=t},initWithAction:function(t,e){return t?(this._innerAction=t,this._speed=e,!0):(cc.errorID(1021),!1)},clone:function(){var t=new cc.Speed;return t.initWithAction(this._innerAction.clone(),this._speed),t},startWithTarget:function(t){cc.Action.prototype.startWithTarget.call(this,t),this._innerAction.startWithTarget(t)},stop:function(){this._innerAction.stop(),cc.Action.prototype.stop.call(this)},step:function(t){this._innerAction.step(t*this._speed)},isDone:function(){return this._innerAction.isDone()},reverse:function(){return new cc.Speed(this._innerAction.reverse(),this._speed)},setInnerAction:function(t){this._innerAction!==t&&(this._innerAction=t)},getInnerAction:function(){return this._innerAction}}),cc.speed=function(t,e){return new cc.Speed(t,e)},cc.Follow=cc.Class({name:"cc.Follow",extends:cc.Action,ctor:function(t,e){this._followedNode=null,this._boundarySet=!1,this._boundaryFullyCovered=!1,this._halfScreenSize=null,this._fullScreenSize=null,this.leftBoundary=0,this.rightBoundary=0,this.topBoundary=0,this.bottomBoundary=0,this._worldRect=cc.rect(0,0,0,0),t&&(e?this.initWithTarget(t,e):this.initWithTarget(t))},clone:function(){var t=new cc.Follow,e=this._worldRect,i=new cc.Rect(e.x,e.y,e.width,e.height);return t.initWithTarget(this._followedNode,i),t},isBoundarySet:function(){return this._boundarySet},setBoudarySet:function(t){this._boundarySet=t},initWithTarget:function(t,e){if(!t)return cc.errorID(1022),!1;e=e||cc.rect(0,0,0,0),this._followedNode=t,this._worldRect=e,this._boundarySet=!(0===e.width&&0===e.height),this._boundaryFullyCovered=!1;var i=cc.winSize;return this._fullScreenSize=cc.v2(i.width,i.height),this._halfScreenSize=this._fullScreenSize.mul(.5),this._boundarySet&&(this.leftBoundary=-(e.x+e.width-this._fullScreenSize.x),this.rightBoundary=-e.x,this.topBoundary=-e.y,this.bottomBoundary=-(e.y+e.height-this._fullScreenSize.y),this.rightBoundary<this.leftBoundary&&(this.rightBoundary=this.leftBoundary=(this.leftBoundary+this.rightBoundary)/2),this.topBoundary<this.bottomBoundary&&(this.topBoundary=this.bottomBoundary=(this.topBoundary+this.bottomBoundary)/2),this.topBoundary===this.bottomBoundary&&this.leftBoundary===this.rightBoundary&&(this._boundaryFullyCovered=!0)),!0},step:function(t){var e=this.target.convertToWorldSpaceAR(cc.Vec2.ZERO),i=this._followedNode.convertToWorldSpaceAR(cc.Vec2.ZERO),r=e.sub(i),s=this.target.parent.convertToNodeSpaceAR(r.add(this._halfScreenSize));if(this._boundarySet){if(this._boundaryFullyCovered)return;this.target.setPosition(n.clampf(s.x,this.leftBoundary,this.rightBoundary),n.clampf(s.y,this.bottomBoundary,this.topBoundary))}else this.target.setPosition(s.x,s.y)},isDone:function(){return!this._followedNode.activeInHierarchy},stop:function(){this.target=null,cc.Action.prototype.stop.call(this)}}),cc.follow=function(t,e){return new cc.Follow(t,e)}}),{"../core/platform/CCClass":199,"../core/utils/misc":301}],2:[(function(t,e,i){"use strict";function n(t,e,i,n,r,s){var o=s*s,a=o*s,l=(1-r)/2,h=l*(2*o-a-s),c=l*(-a+o)+(2*a-3*o+1),u=l*(a-2*o+s)+(-2*a+3*o),_=l*(a-o),f=t.x*h+e.x*c+i.x*u+n.x*_,d=t.y*h+e.y*c+i.y*u+n.y*_;return cc.v2(f,d)}function r(t,e){return t[Math.min(t.length-1,Math.max(e,0))]}function s(t){for(var e=[],i=t.length-1;i>=0;i--)e.push(cc.v2(t[i].x,t[i].y));return e}function o(t){for(var e=[],i=0;i<t.length;i++)e.push(cc.v2(t[i].x,t[i].y));return e}cc.CardinalSplineTo=cc.Class({name:"cc.CardinalSplineTo",extends:cc.ActionInterval,ctor:function(t,e,i){this._points=[],this._deltaT=0,this._tension=0,this._previousPosition=null,this._accumulatedDiff=null,void 0!==i&&cc.CardinalSplineTo.prototype.initWithDuration.call(this,t,e,i)},initWithDuration:function(t,e,i){return e&&0!==e.length?!!cc.ActionInterval.prototype.initWithDuration.call(this,t)&&(this.setPoints(e),this._tension=i,!0):(cc.errorID(1024),!1)},clone:function(){var t=new cc.CardinalSplineTo;return t.initWithDuration(this._duration,o(this._points),this._tension),t},startWithTarget:function(t){cc.ActionInterval.prototype.startWithTarget.call(this,t),this._deltaT=1/(this._points.length-1),this._previousPosition=cc.v2(this.target.x,this.target.y),this._accumulatedDiff=cc.v2(0,0)},update:function(t){var e,i;t=this._computeEaseTime(t);var s=this._points;if(1===t)e=s.length-1,i=1;else{var o=this._deltaT;i=(t-o*(e=0|t/o))/o}var a,l,h=n(r(s,e-1),r(s,e-0),r(s,e+1),r(s,e+2),this._tension,i);if(cc.macro.ENABLE_STACKABLE_ACTIONS&&(a=this.target.x-this._previousPosition.x,l=this.target.y-this._previousPosition.y,0!==a||0!==l)){var c=this._accumulatedDiff;a=c.x+a,l=c.y+l,c.x=a,c.y=l,h.x+=a,h.y+=l}this.updatePosition(h)},reverse:function(){var t=s(this._points);return cc.cardinalSplineTo(this._duration,t,this._tension)},updatePosition:function(t){this.target.setPosition(t),this._previousPosition=t},getPoints:function(){return this._points},setPoints:function(t){this._points=t}}),cc.cardinalSplineTo=function(t,e,i){return new cc.CardinalSplineTo(t,e,i)},cc.CardinalSplineBy=cc.Class({name:"cc.CardinalSplineBy",extends:cc.CardinalSplineTo,ctor:function(t,e,i){this._startPosition=cc.v2(0,0),void 0!==i&&this.initWithDuration(t,e,i)},startWithTarget:function(t){cc.CardinalSplineTo.prototype.startWithTarget.call(this,t),this._startPosition.x=t.x,this._startPosition.y=t.y},reverse:function(){for(var t,e=this._points.slice(),i=e[0],n=1;n<e.length;++n)t=e[n],e[n]=t.sub(i),i=t;var r=s(e);i=r[r.length-1],r.pop(),i.x=-i.x,i.y=-i.y,r.unshift(i);for(n=1;n<r.length;++n)(t=r[n]).x=-t.x,t.y=-t.y,t.x+=i.x,t.y+=i.y,r[n]=t,i=t;return cc.cardinalSplineBy(this._duration,r,this._tension)},updatePosition:function(t){var e=this._startPosition,i=t.x+e.x,n=t.y+e.y;this._previousPosition.x=i,this._previousPosition.y=n,this.target.setPosition(i,n)},clone:function(){var t=new cc.CardinalSplineBy;return t.initWithDuration(this._duration,o(this._points),this._tension),t}}),cc.cardinalSplineBy=function(t,e,i){return new cc.CardinalSplineBy(t,e,i)},cc.CatmullRomTo=cc.Class({name:"cc.CatmullRomTo",extends:cc.CardinalSplineTo,ctor:function(t,e){e&&this.initWithDuration(t,e)},initWithDuration:function(t,e){return cc.CardinalSplineTo.prototype.initWithDuration.call(this,t,e,.5)},clone:function(){var t=new cc.CatmullRomTo;return t.initWithDuration(this._duration,o(this._points)),t}}),cc.catmullRomTo=function(t,e){return new cc.CatmullRomTo(t,e)},cc.CatmullRomBy=cc.Class({name:"cc.CatmullRomBy",extends:cc.CardinalSplineBy,ctor:function(t,e){e&&this
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
java毕业设计,项目包含完整前后端源码,带数据库sql文件。项目可正常运行! 环境说明: 开发语言:Java 前端框架:小程序 JDK版本:JDK1.8 数据库:mysql 5.7+ 部署容器:tomcat7+ 数据库工具:Navicat11+ 开发软件:eclipse/myeclipse/idea(推荐idea) Maven包:Maven3.3.9
资源推荐
资源详情
资源评论
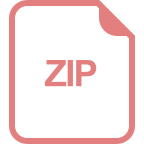
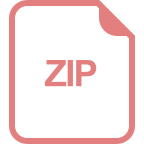
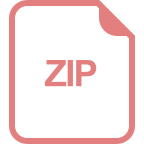
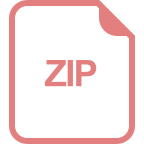
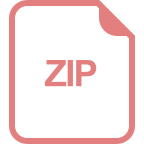
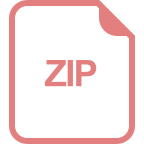
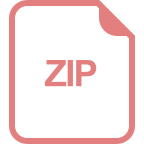
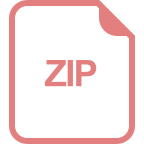
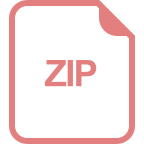
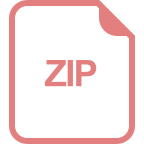
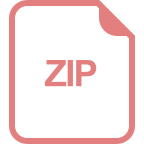
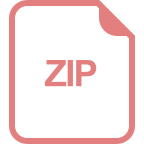
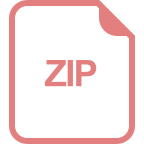
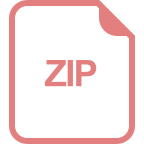
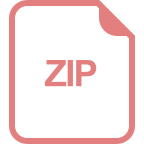
收起资源包目录

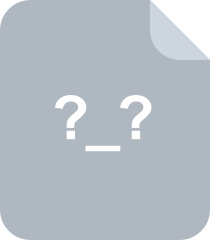
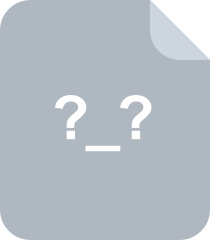
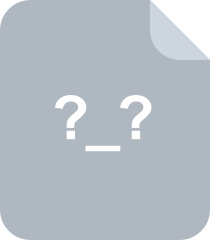
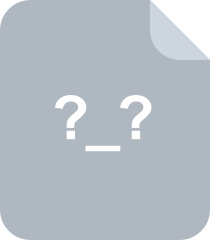
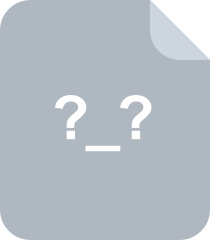
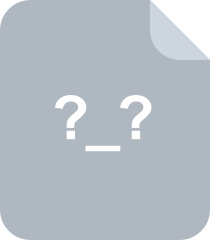
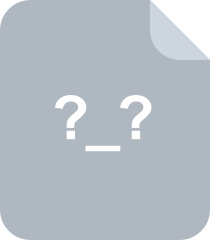
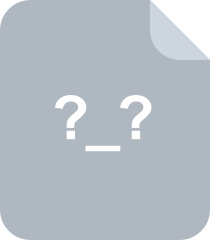
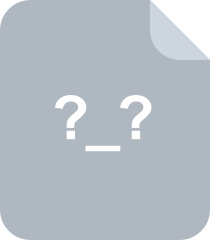
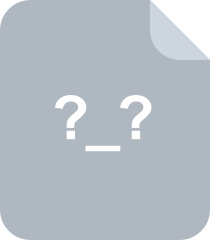
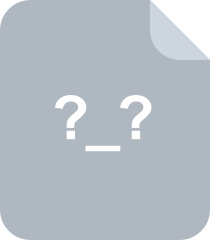
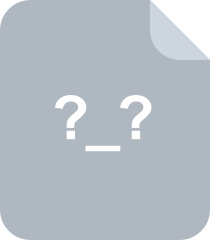
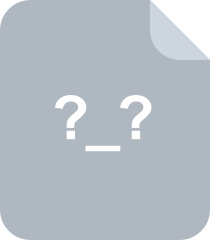
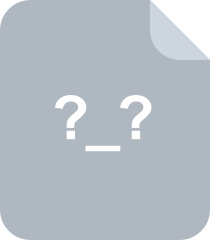
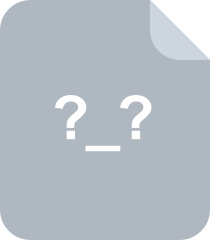
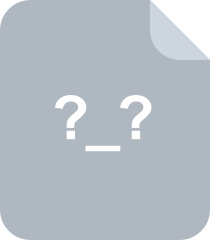
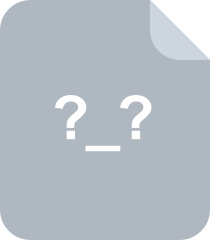
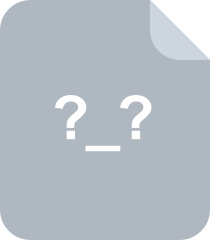
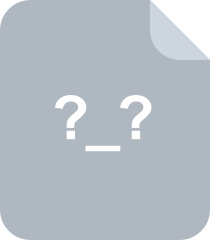
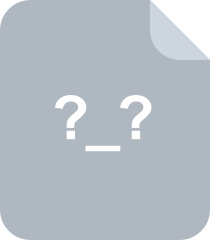
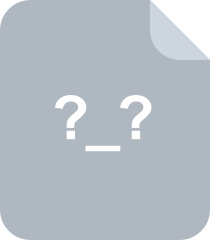
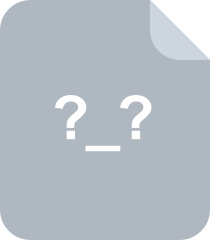
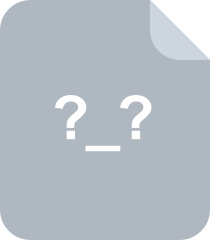
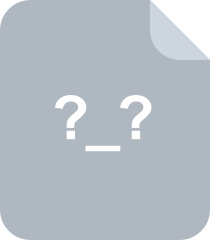
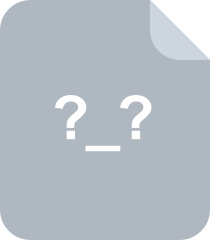
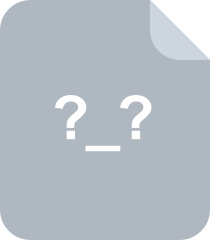
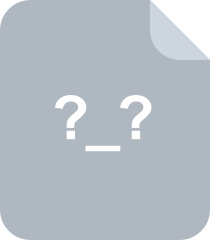
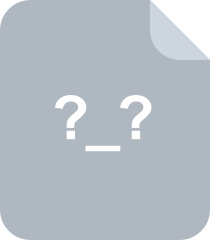
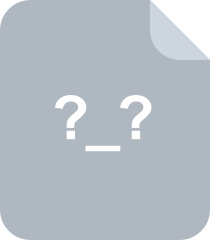
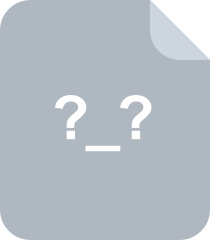
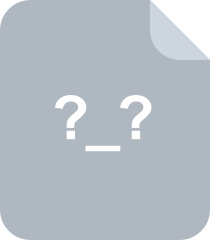
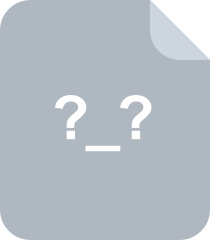
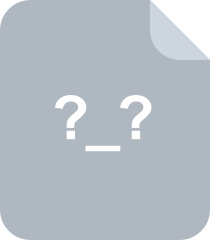
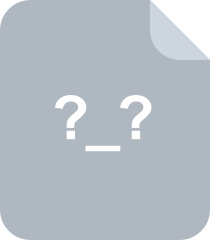
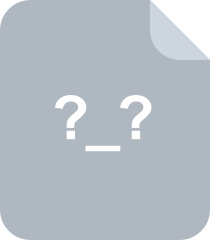
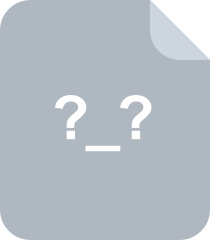
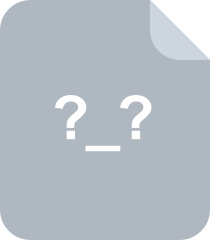
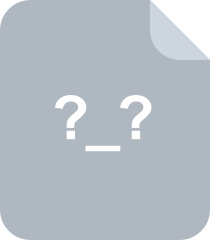
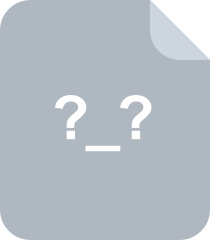
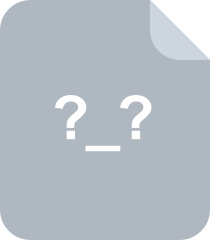
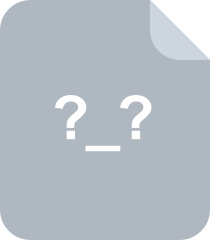
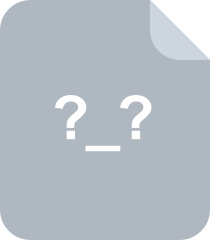
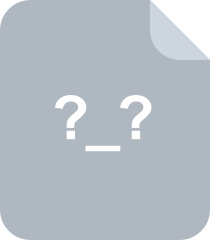
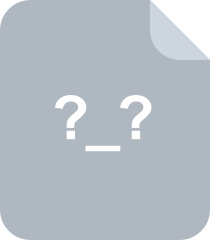
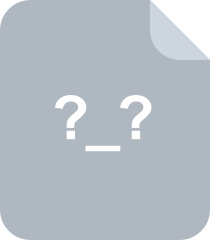
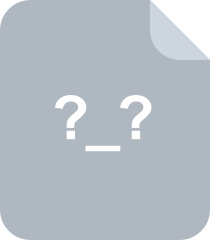
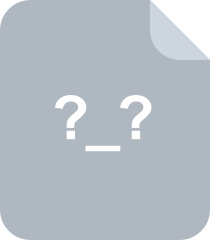
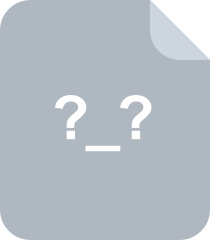
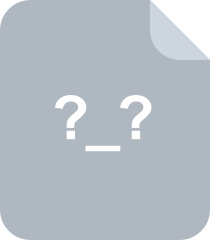
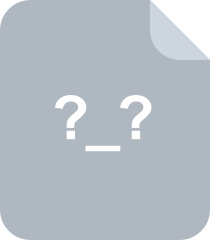
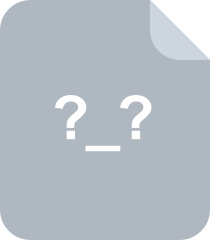
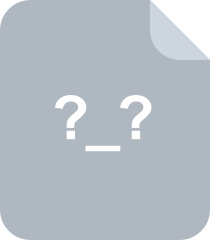
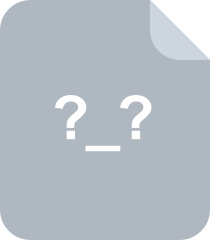
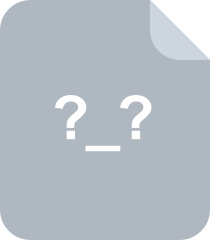
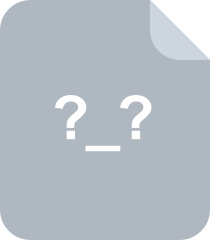
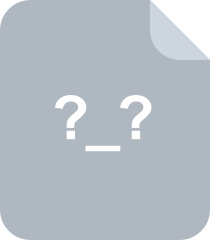
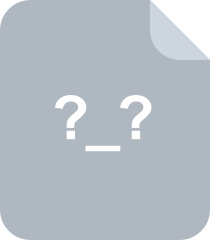
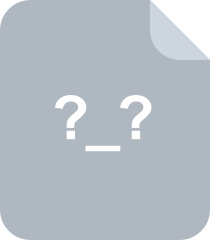
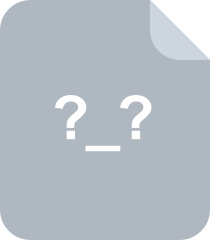
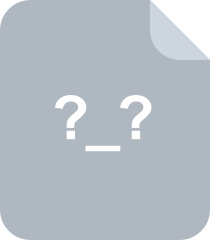
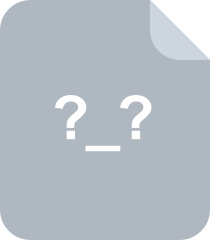
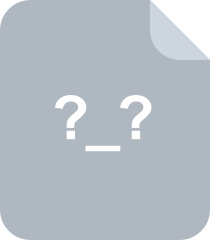
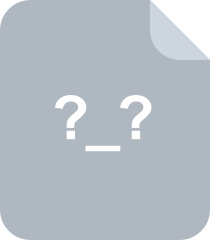
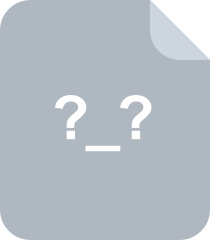
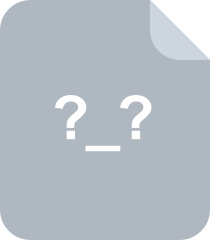
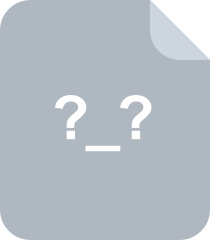
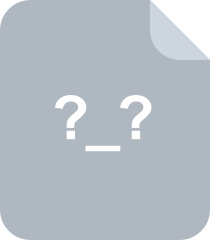
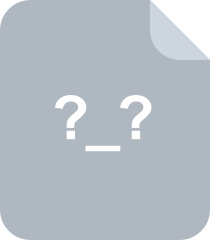
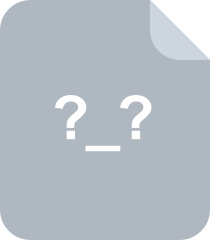
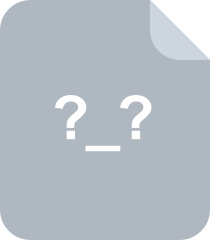
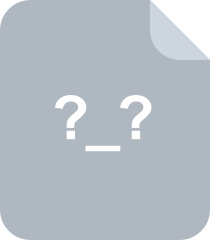
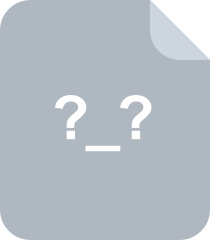
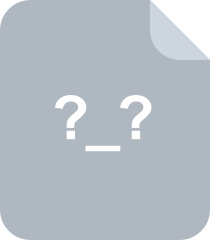
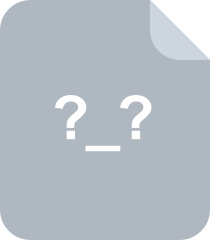
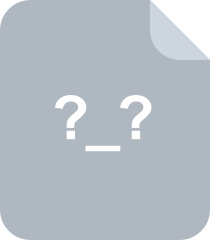
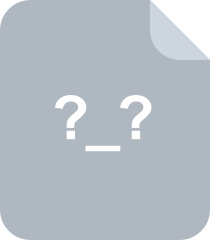
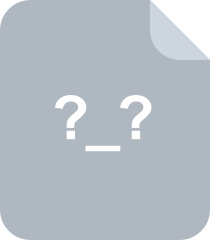
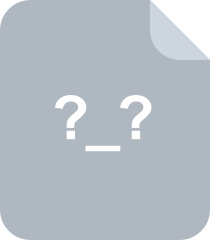
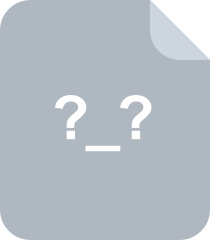
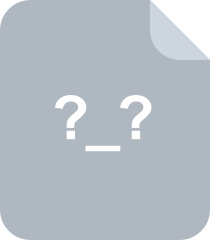
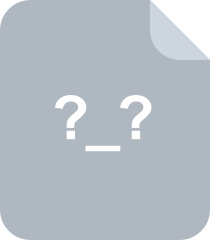
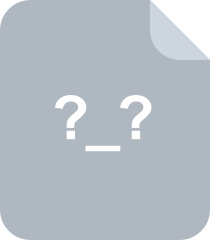
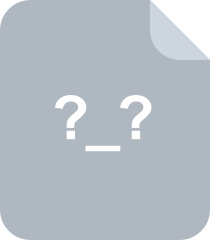
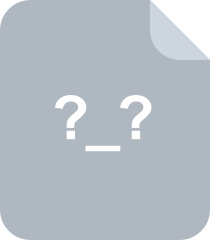
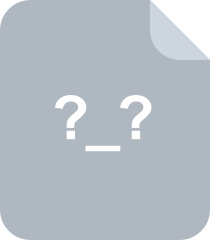
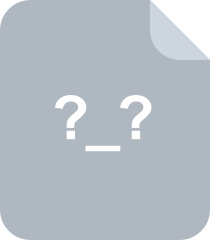
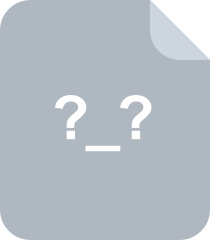
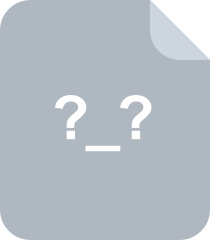
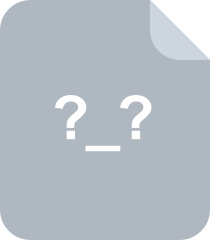
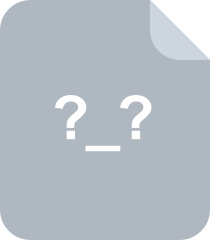
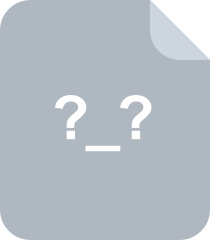
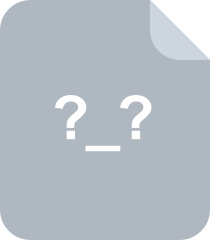
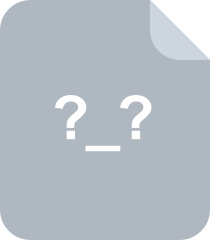
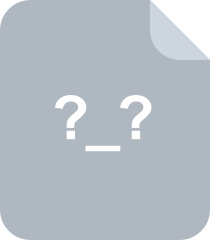
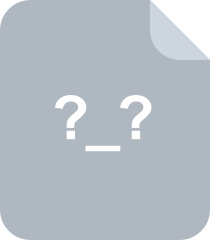
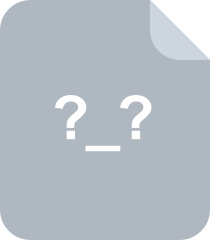
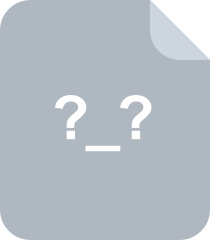
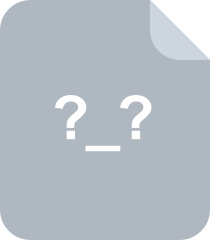
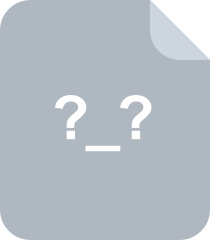
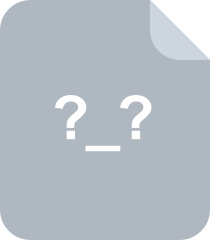
共 350 条
- 1
- 2
- 3
- 4
资源评论
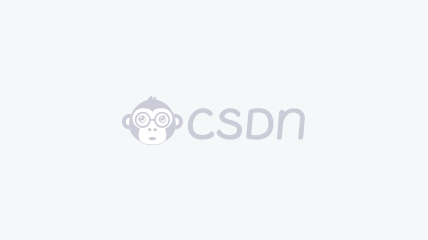

大学生资源网
- 粉丝: 138
- 资源: 1334
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

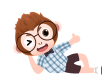
安全验证
文档复制为VIP权益,开通VIP直接复制
