/*
* Copyright 2009 Cedric Priscal
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.android_serialport_api.src.android_serialport_api.sample;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Timer;
import java.util.TimerTask;
import com.io.jniio;
import android.R.bool;
import android.R.string;
import android.app.Activity;
import android.app.ProgressDialog;
import android.content.Intent;
import android.os.Bundle;
import android.os.Handler;
import android.os.Message;
import android.os.SystemClock;
import android.util.Log;
import android.view.KeyEvent;
import android.view.View;
import android.widget.AdapterView;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ListView;
import android.widget.SimpleAdapter;
import android.widget.TextView;
import android.widget.Toast;
import android.widget.AdapterView.OnItemClickListener;
import android.widget.AdapterView.OnItemSelectedListener;
import android.widget.TextView.OnEditorActionListener;
import com.io.R;
import com.zeph.android.fileoperate.FileHelper;
import com.zeph.android.fileoperate.excelutil;
public class ConsoleActivity extends Activity {
EditText mReception;
static final int LED_ON =1;
static final int LED_OFF= 0;
static final int INPUT = 2;
static final int OUTPUT = 3;
static final int ADINIT = 4;
static final int ADREAD = 5;
static final int SPI0INITIAL= 6;
static final float iodelay0 = (float) 1.0;//MCUÉϵçÑÓʱiodelay0ºó¿ªÊ¼P10Êä³ö¸ß£¬ËùÓÐIOĬÈÏÊÇÉϵçµÍµçƽ£¬P10---P17 ¶ø¶óÍóÎÊÎÊ
static final float iodelay1 = 0 ; //P11ͬÉÏ
static final float iodelay2 = 0 ; //P12ͬÉÏ
static final float iodelay3 = 0 ; //P13ͬÉÏ
static final float iodelay4 = 0 ; //P14ͬÉÏ
static final float iodelay5 = 0 ; //P15ͬÉÏ
static final float iodelay6 = 0 ; //P16ͬÉÏ
static final float iodelay7 = 0 ; //P17ͬÉÏ
static final float iohigh1= (float)0.2 ; //MCUÉϵçÑÓʱiodelay0ºó¿ªÊ¼Êä³ö¸ßµçƽ³ÖÐøiohigh1£¬
static final float iolow1 =(float)1 ; // ¸ßµç½áÊøºó¿ªÊ¼Êä³öµÍµçƽ³ÖÐøÊ±¼äÊÇiolow1
static final float iohigh2 =(float)0.5 ; // ͬÉÏ iohigh1
static final float iolow2 = (float)1.5 ; // ͬÉÏ iolow1
static final float iohigh3 =(float)0.4 ; // ͬÉÏ iohigh1
static final float iolow3 = (float)0.5 ; // ͬÉÏ iolow1
static final float just_once =0 ;
long[] io_l1=new long[8];
long[] io_h1=new long[8];
long[] io_l2=new long[8];
long[] io_h2=new long[8];
long[] io_l3=new long[8];
long[] io_h3=new long[8];
boolean justonce=false;
long [] count=new long[8];
char [] stepflag=new char[8];
long [] paracount1=new long[8];
private FileHelper helper1;
private int exit;
long [] io_delay ={ (long)iodelay0*20
, (long)iodelay1*20
, (long)iodelay2*20
,(long)iodelay3*20
,(long)iodelay4*20
,(long)iodelay5*20
,(long)iodelay6*20
,(long)iodelay7*20} ;
long count_action[]=new long [8];
int[]key_flag =new int [8];
jniio ok6410ioport=new jniio();
private ListView mListView;
ArrayList<HashMap<String, String>> list =new ArrayList<HashMap<String, String>>();
//生成两个HashMap类型的变量map1 , map2
//HashMpa为键值对类型。第一个参数为建,第二个参数为值
HashMap<String, String> map1 =new HashMap<String, String>();
HashMap<String, String> map2 =new HashMap<String, String>();
SimpleAdapter listAdapter;
excelutil excel;
ArrayList<ArrayList<String>> excelcontent=new ArrayList<ArrayList<String>> ();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.console);
setTitle("Loopback test");
//生成一个ArrayList类型的变量list
excel=new excelutil(this.getBaseContext()) ;
//把数据填充到map1和map2中。 1 row
map1.put("power_delay", "张三");
map1.put("h_pluse1", "192.168.1.52");
map1.put("l_pluse1", "192.168.1.52");
map1.put("h_pluse2", "192.168.1.52");
map1.put("l_pluse2", "192.168.1.52");
map1.put("h_pluse3", "192.168.1.52");
map1.put("l_pluse3", "192.168.1.52");
//2 row
map2.put("power_delay", "李四");
map2.put("h_pluse1", "192.168.1.52");
map2.put("l_pluse1", "192.168.1.52");
map2.put("h_pluse2", "192.168.1.52");
map2.put("l_pluse2", "192.168.1.52");
map2.put("h_pluse3", "192.168.1.52");
map2.put("l_pluse3", "192.168.1.52");
//把map1和map2添加到list中
list.add(map1);
list.add(map2);
//生成一个SimpleAdapter类型的变量来填充数据
listAdapter =new SimpleAdapter(this, list, R.layout.user,
new String[]{
"port","power_delay" ,
"h_pluse1","l_pluse1",
"h_pluse2","l_pluse2",
"h_pluse3","l_pluse3",
"feadback"
},
new int[]{R.id.port ,
R.id.power_delay ,
R.id.h_pluse1,R.id.l_pluse1,
R.id.h_pluse2,R.id.l_pluse2,
R.id.h_pluse3,R.id.l_pluse3,
R.id.feadback
});
//设置显示ListView
exit=0;
helper1 = new FileHelper(getApplicationContext());
mListView = (ListView) findViewById(R.id.listView1);
mListView.setAdapter(listAdapter);
mListView.setOnItemClickListener(new OnItemClickListener()
{
@SuppressWarnings("unchecked")
@Override
public void onItemClick(AdapterView<?> arg0, View arg1, int arg2, long arg3) {
// TODO Auto-generated method stub
Intent intent = new Intent();
ListView listView = (ListView)arg0;
HashMap<String, String> map = (HashMap<String, String>) listView.getItemAtPosition(arg2);
String power_delay =map.get("power_delay");
String h_pluse1 = map.get("h_pluse1");
String l_pluse1 = map.get("l_pluse1");
String h_pluse2 = map.get("h_pluse2");
String l_pluse2 = map.get("l_pluse2");
String h_pluse3 = map.get("h_pluse3");
String l_pluse3 = map.get("l_pluse3");
//Toast.makeText(ConsoleActivity.this, h_pluse1 +" , "+ l_pluse1 +" , "+ h_pluse2 ,Toast.LENGTH_LONG).show();
Bundle bundle = new Bundle();
bundle.putString("power_delay", power_delay);
bundle.putString("h_pluse1", h_pluse1);
bundle.putString("l_pluse1", l_pluse1);
bundle.putString("h_pluse2", h_pluse2);
bundle.putString("l_pluse2", l_pluse2);
bundle.putString("h_pluse3", h_pluse3);
bundle.putString("l_pluse3", l_pluse3);
bundle.putInt ("pos", arg2);//("l_pluse3", l_pluse3);
intent.putExtras(bundle);
intent.setClass(ConsoleActivity.this, modifytextActivity.class);
startActivity(intent);
//timer.cancel();
//task.cancel();
//ok6410ioport.close();
//ConsoleActivity.this.finish();
}
});
/* mListView.setOnItemSelectedListener(new OnItemSelectedListener(){
@Override
public void on
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
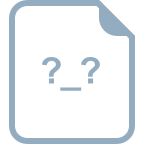
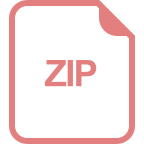
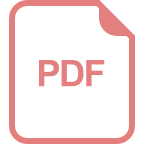
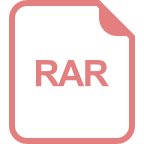
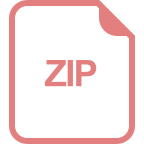
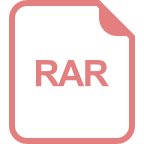
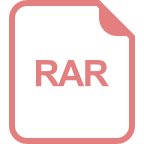
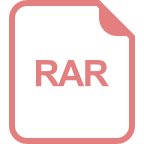
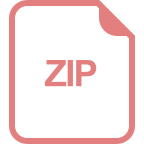
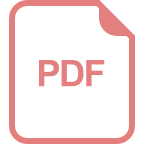
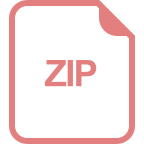
收起资源包目录

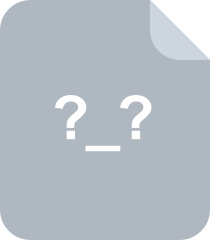
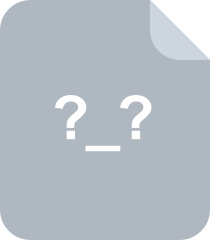
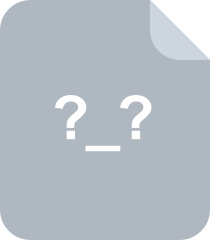
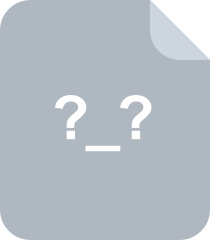
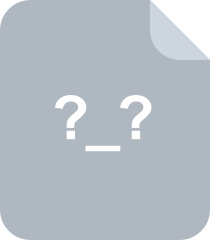
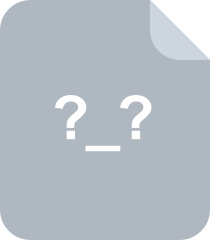
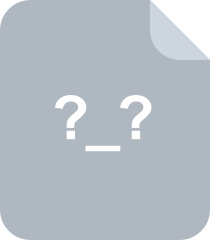
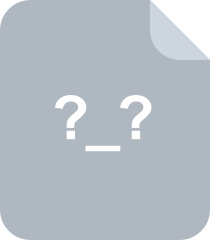
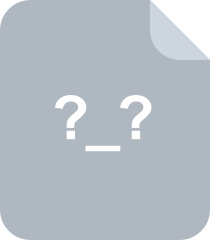
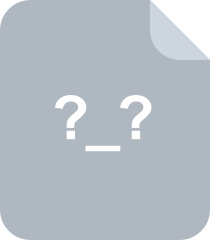
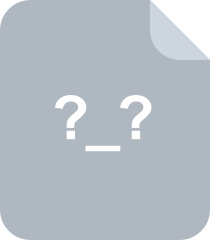
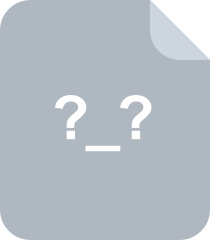
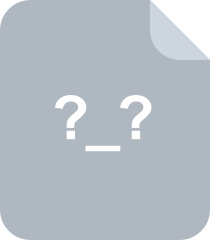
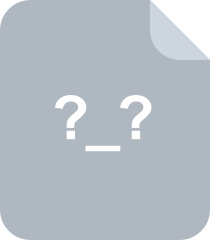
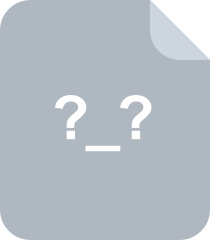
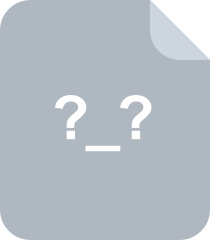
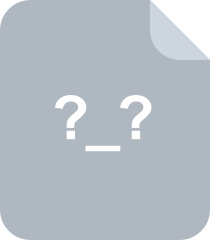
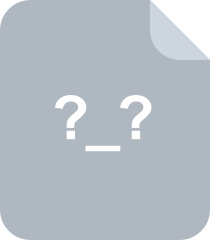
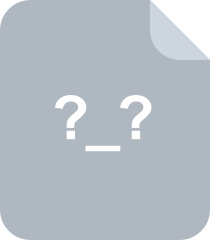
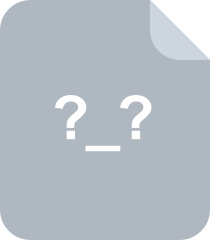
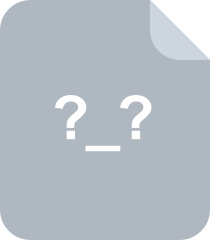
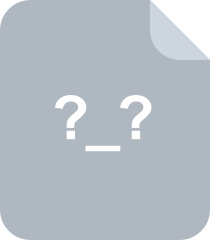
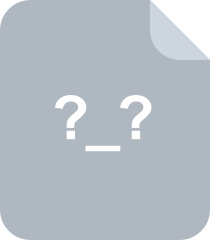
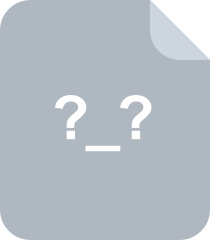
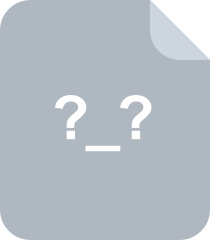
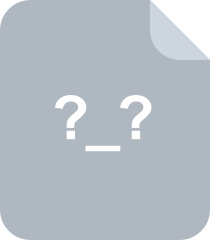
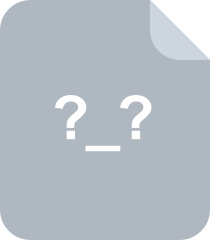
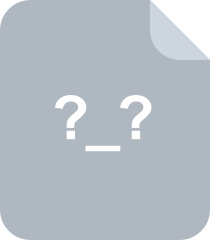
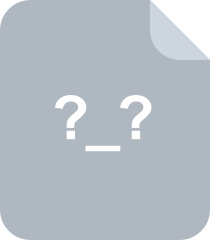
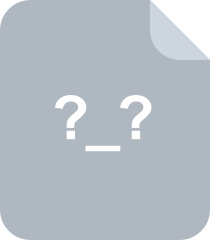
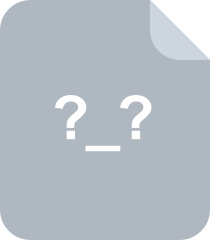
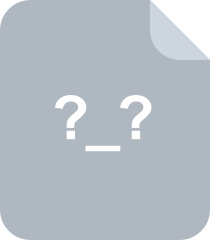
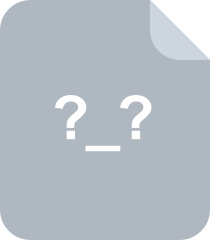
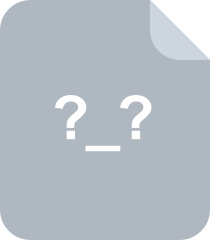
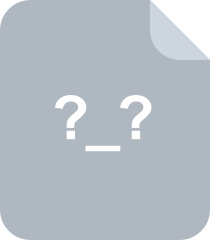
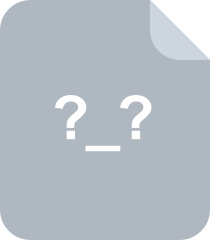
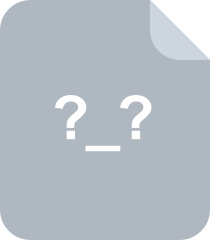
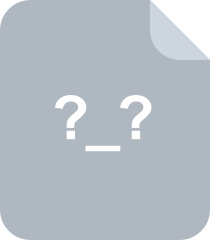
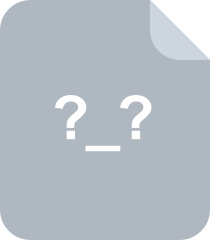
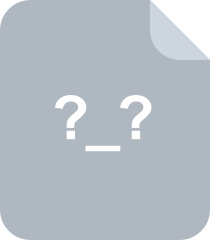
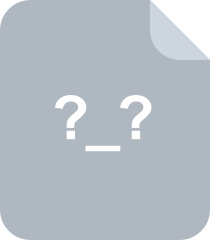
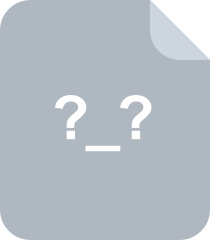
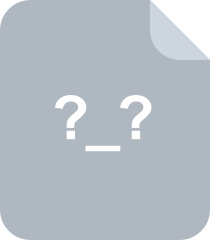
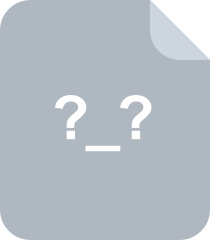
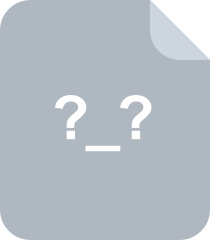
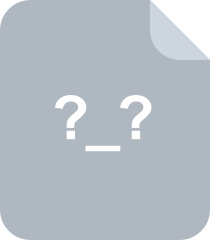
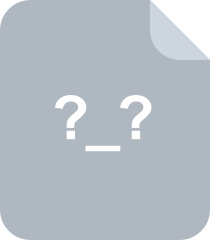
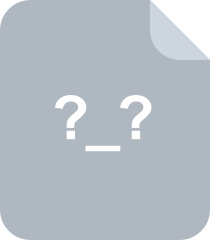
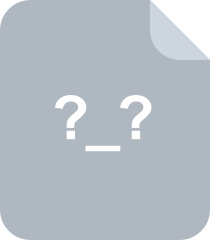
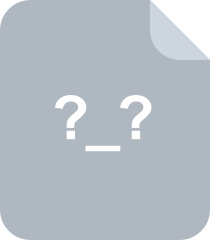
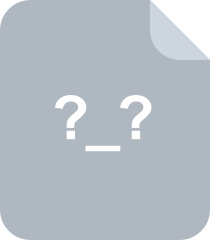
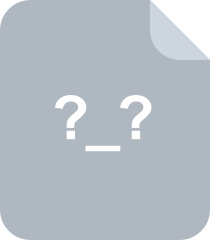
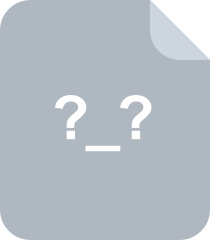
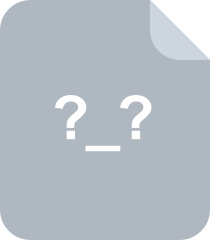
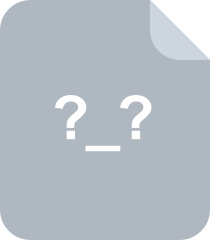
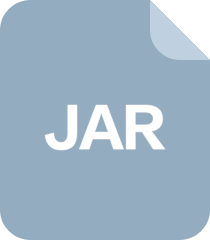
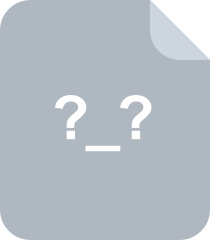
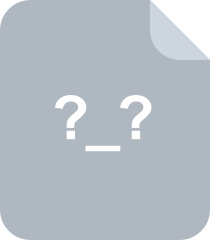
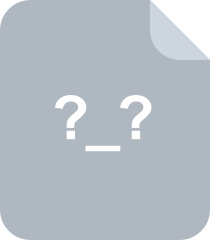
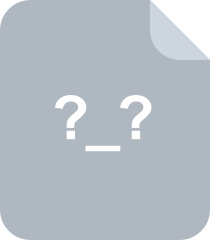
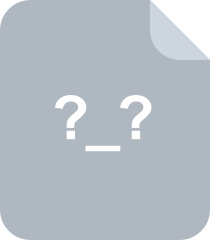
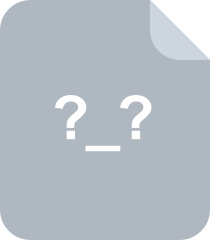
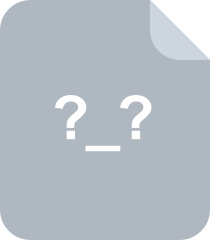
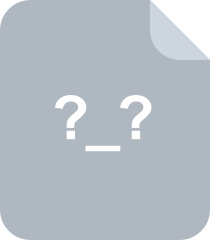
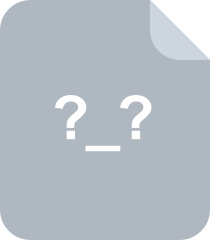
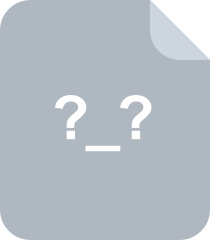
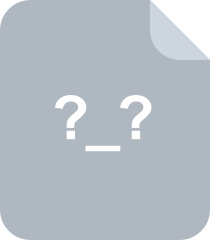
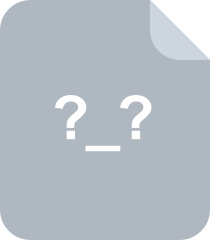
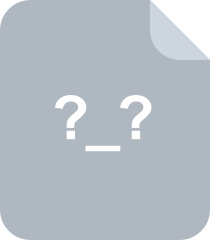
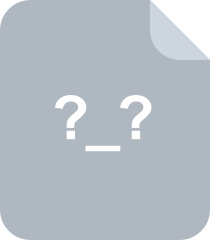
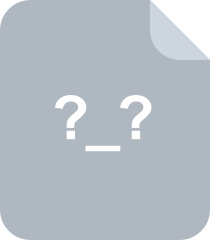
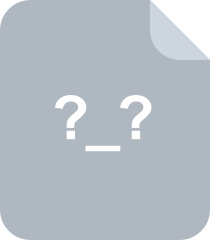
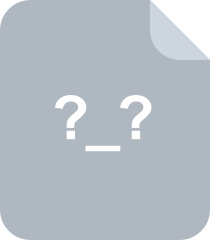
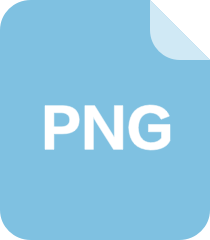
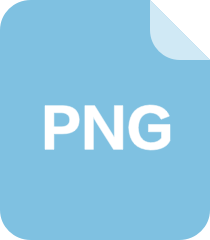
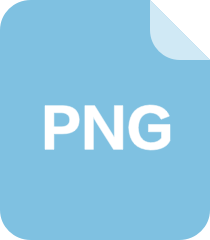
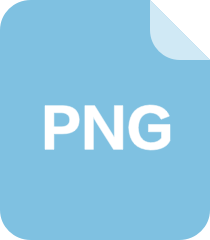
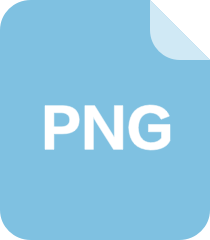
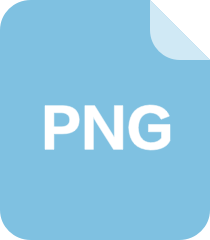
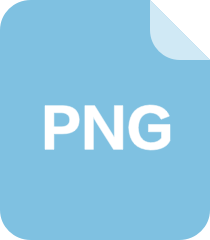
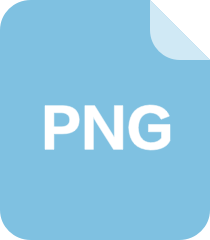
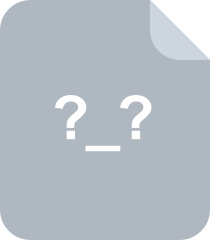
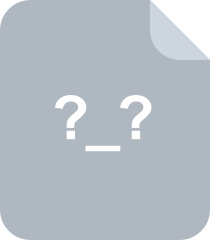
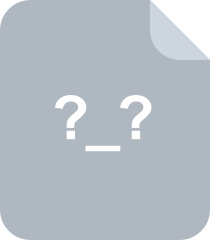
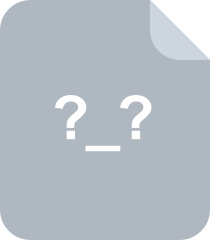
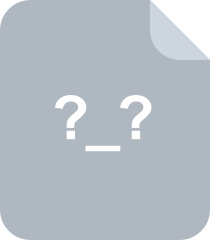
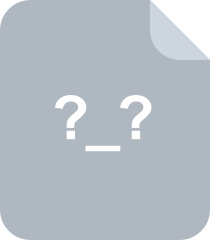
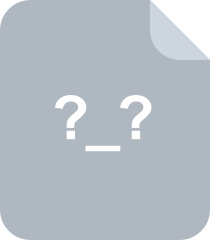
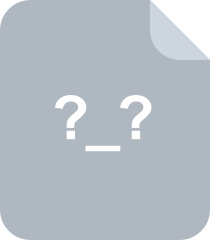
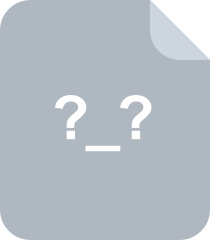
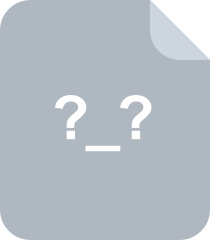
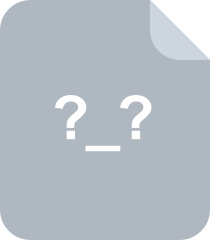
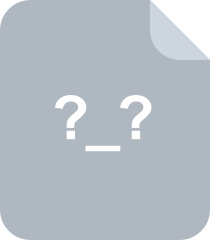
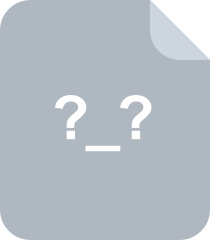
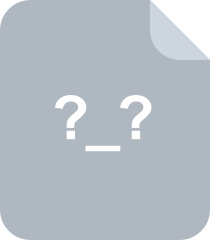
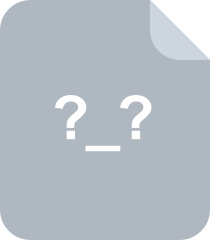
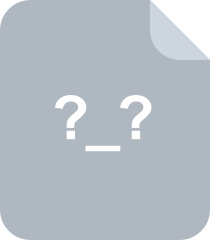
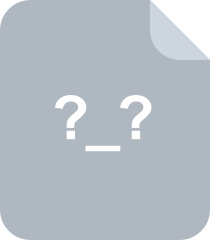
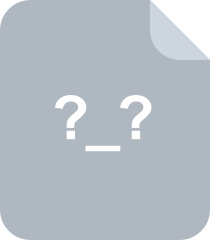
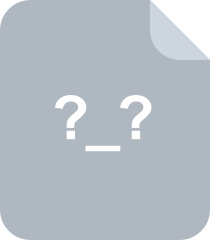
共 102 条
- 1
- 2

fanting247615584
- 粉丝: 1
- 资源: 25
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

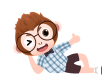
最新资源
- 白色简洁风格的Zero企业网站模板.zip
- 白色简洁风格的奥迪mini跑车企业网站模板.zip
- 白色简洁风格的办公office企业网站模板下载.zip
- 白色简洁风格的办公管理后台系统源码下载.zip
- 白色简洁风格的办公室装修公司企业网站模板.zip
- 白色简洁风格的办公平台登录表源码下载.zip
- 白色简洁风格的办公室室内设计门户网站模板下载.zip
- 白色简洁风格的别墅设计装修整站网站模板.zip
- 白色简洁风格的别墅整站网站模板.zip
- 白色简洁风格的博客论坛后台系统源码下载.zip
- 白色简洁风格的餐厅菜品系列源码下载.zip
- 白色简洁风格的博客论坛后台统计源码下载.zip
- 白色简洁风格的餐厅会员登录框源码下载.zip
- 白色简洁风格的餐厅服务团队整站网站源码下载.zip
- 白色简洁风格的餐厅美味食谱整站网站源码下载.zip
- 白色简洁风格的餐饮食材食谱整站网站源码下载.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


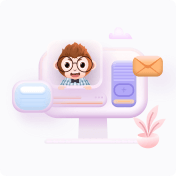
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
前往页