# Microsoft.IO.RecyclableMemoryStream [](https://www.nuget.org/packages/Microsoft.IO.RecyclableMemoryStream/)
A library to provide pooling for .NET `MemoryStream` objects to improve application performance, especially in the area of garbage collection.
## Get Started
Install the latest version from [NuGet](https://www.nuget.org/packages/Microsoft.IO.RecyclableMemoryStream/)
```
Install-Package Microsoft.IO.RecyclableMemoryStream
```
## Purpose
`Microsoft.IO.RecyclableMemoryStream` is a `MemoryStream` replacement that offers superior behavior for performance-critical systems. In particular it is optimized to do the following:
* Eliminate Large Object Heap allocations by using pooled buffers
* Incur far fewer gen 2 GCs, and spend far less time paused due to GC
* Avoid memory leaks by having a bounded pool size
* Avoid memory fragmentation
* Allow for multiple ways to read and write data that will avoid extraneous allocations
* Provide excellent debuggability and logging
* Provide metrics for performance tracking
## Features
- The semantics are close to the original `System.IO.MemoryStream` implementation, and is intended to be a drop-in replacement as much as possible.
- Rather than pooling the streams themselves, the underlying buffers are pooled. This allows you to use the simple `Dispose` pattern to release the buffers back to the pool, as well as detect invalid usage patterns (such as reusing a stream after it’s been disposed).
- `RecyclableMemoryStreamManager` is thread-safe (streams themselves are inherently NOT thread safe).
- Implementation of `IBufferWrite<byte>`.
- Support for enormous streams through abstracted buffer chaining.
- Extensive support for newer memory-related types like `Span<byte>`, `ReadOnlySpan<byte>`, `ReadOnlySequence<byte>`, and `Memory<byte>`.
- Each stream can be tagged with an identifying string that is used in logging - helpful when finding bugs and memory leaks relating to incorrect pool use.
- Debug features like recording the call stack of the stream allocation to track down pool leaks
- Maximum free pool size to handle spikes in usage without using too much memory.
- Flexible and adjustable limits to the pooling algorithm.
- Metrics tracking and events so that you can see the impact on the system.
## Build Targets
At least MSBuild 16.8 is required to build the code. You get this with Visual Studio 2019.
Supported build targets in v2.0 are: net462, netstandard2.0, netstandard2.1, and netcoreapp2.1 (net40, net45, net46 and netstandard1.4 were deprecated). Starting with v2.1, net5.0 target has been added.
## Testing
A minimum of .NET 5.0 is required for executing the unit tests. Requirements:
- NUnit test adapter (VS Extension)
- Be sure to set the default processor architecture for tests to x64 (or the giant allocation test will fail)
## Benchmark tests
The results are available [here](https://microsoft.github.io/Microsoft.IO.RecyclableMemoryStream/dev/bench/)
## Change Log
Read the change log [here](https://github.com/microsoft/Microsoft.IO.RecyclableMemoryStream/blob/master/CHANGES.md).
## How It Works
`RecyclableMemoryStream` improves GC performance by ensuring that the larger buffers used for the streams are put into the gen 2 heap and stay there forever. This should cause full collections to happen less frequently. If you pick buffer sizes above 85,000 bytes, then you will ensure these are placed on the large object heap, which is touched even less frequently by the garbage collector.
The `RecyclableMemoryStreamManager` class maintains two separate pools of objects:
1. **Small Pool** - Holds small buffers (of configurable size). Used by default for all normal read/write operations. Multiple small buffers are chained together in the `RecyclableMemoryStream` class and abstracted into a single stream.
2. **Large Pool** - Holds large buffers, which are only used when you must have a single, contiguous buffer, such as when you plan to call `GetBuffer()`. It is possible to create streams larger than is possible to be represented by a single buffer because of .NET's array size limits.
A `RecyclableMemoryStream` starts out by using a small buffer, chaining additional ones as the stream capacity grows. Should you ever call `GetBuffer()` and the length is greater than a single small buffer's capacity, then the small buffers are converted to a single large buffer. You can also request a stream with an initial capacity; if that capacity is larger than the small pool block size, multiple blocks will be chained unless you call an overload with `asContiguousBuffer` set to true, in which case a single large buffer will be assigned from the start. If you request a capacity larger than the maximum poolable size, you will still get a stream back, but the buffers will not be pooled. (Note: This is not referring to the maximum array size. You can limit the poolable buffer sizes in `RecyclableMemoryStreamManager`)
There are two versions of the large pool:
* **Linear** (default) - You specify a multiple and a maximum size, and an array of buffers, from size (1 * multiple), (2 * multiple), (3 * multiple), ... maximum is created. For example, if you specify a multiple of 1 MB and maximum size of 8 MB, then you will have an array of length 8. The first slot will contain 1 MB buffers, the second slot 2 MB buffers, and so on.
* **Exponential** - Instead of linearly growing, the buffers double in size for each slot. For example, if you specify a multiple of 256KB, and a maximum size of 8 MB, you will have an array of length 6, the slots containing buffers of size 256KB, 512KB, 1MB, 2MB, 4MB, and 8MB.
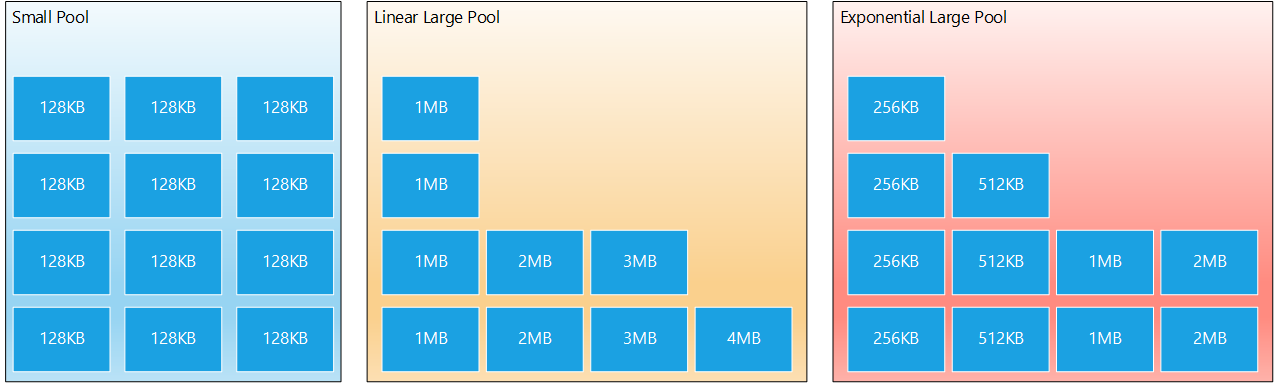
Which one should you use? That depends on your usage pattern. If you have an unpredictable large buffer size, perhaps the linear one will be more suitable. If you know that a longer stream length is unlikely, but you may have a lot of streams in the smaller size, picking the exponential version could lead to less overall memory usage (which was the reason this form was added).
Buffers are created, on demand, the first time they are requested and nothing suitable already exists in the pool. After use, these buffers will be returned to the pool through the `RecyclableMemoryStream`'s `Dispose` method. When that return happens, the `RecyclableMemoryStreamManager` will use the properties `MaximumFreeSmallPoolBytes` and `MaximumFreeLargePoolBytes` to determine whether to put those buffers back in the pool, or let them go (and thus be garbage collected). It is through these properties that you determine how large your pool can grow. If you set these to 0, you can have unbounded pool growth, which is essentially indistinguishable from a memory leak. For every application, you must determine through analysis and experimentation the appropriate balance between pool size and garbage collection.
If you forget to call a stream's `Dispose` method, this could cause a memory leak. To help you prevent this, each stream has a finalizer that will be called by the CLR once there are no more references to the stream. This finalizer will raise an event or log a message about the leaked stream.
Note that for performance reasons, the buffers are not ever pre-initialized or zeroed-out. It is your responsibility to ensure their contents are valid and safe to use buffer recycling.
If you want to avoid accidental data leakage, you can set `ZeroOutBuffer` to true. This will zero out the buffers on allocation and before returning them to the pool. Be aware of the performance implications.
## Usage
You can jump right in with no fuss by just doing a simple replacement of `MemoryStream` with some
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
如无法下载请看主页文章《移动曲面法数字高程模型内插程序算法(C#桌面窗体)》 DEM移动拟合算法程序,《数字高程模型》,适用于地理信息科学等专业。 根据提供的10个数据点的坐标(X,Y,Z)和待求点的平面坐标(Xp,Yp),利用移动二次曲面拟合法,由格网点P(Xp,Yp)周围的10个已知点内插出待求格网点P的高程,编制相应程序解算出格网点P的高程。 实现功能:自定义打开读取excel文件,并用dataview显示其内容。选择移动曲面拟合插值方法,自定义输入(X,Y)坐标,计算显示对应的高程。
资源推荐
资源详情
资源评论
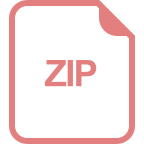
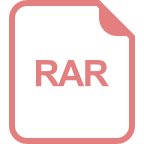
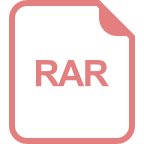
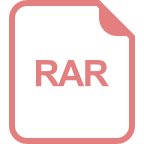
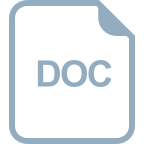
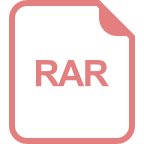
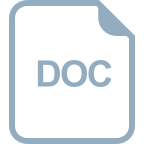
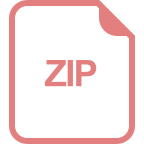
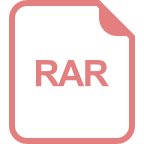
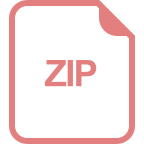
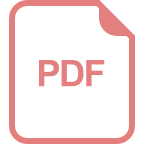
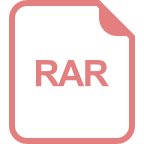
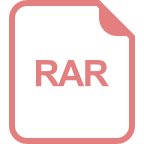
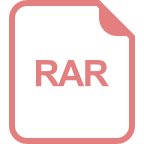
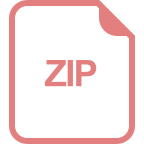
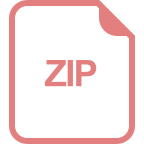
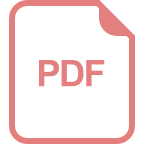
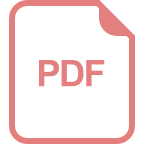
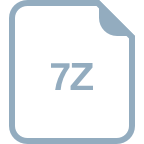
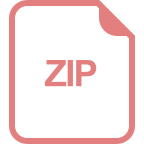
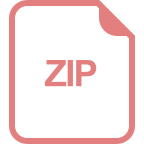
收起资源包目录

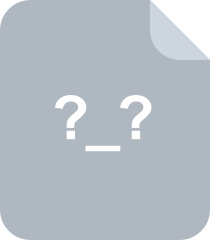
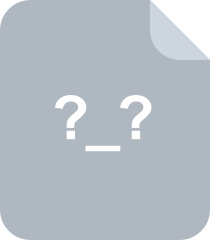
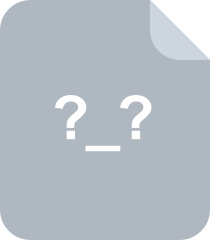
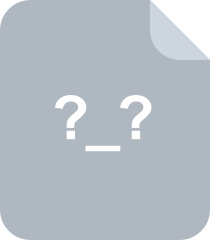
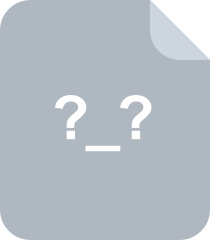
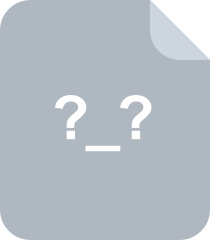
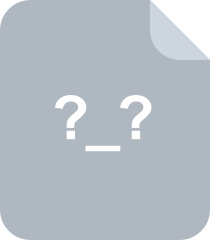
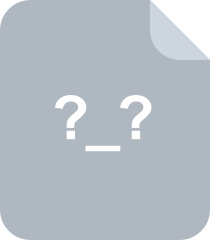
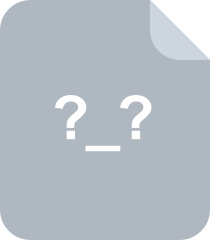
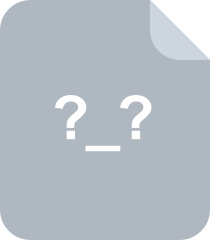
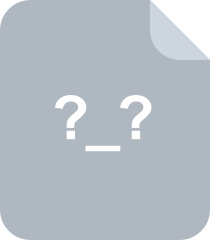
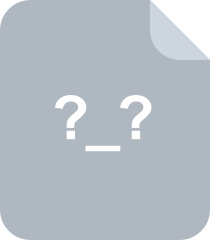
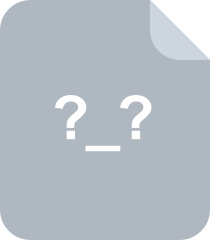
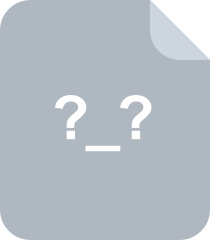
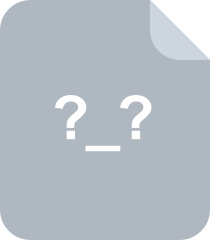
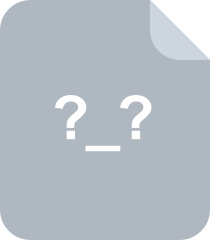
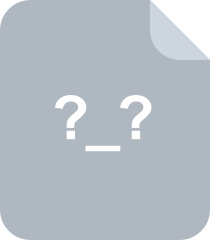
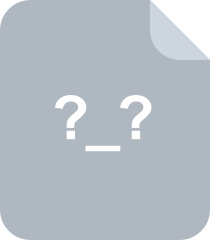
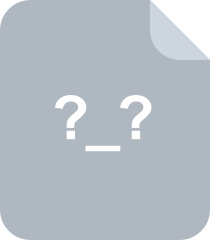
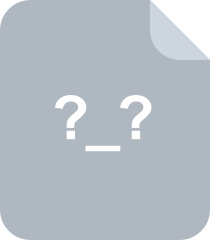
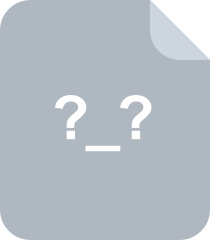
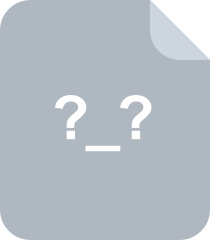
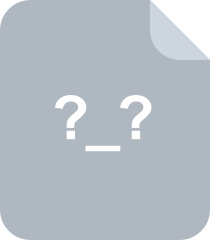
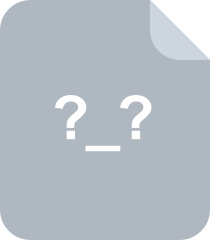
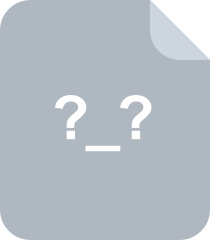
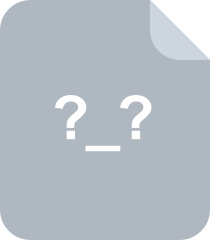
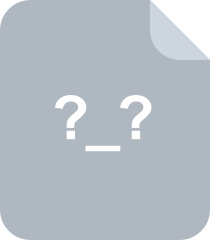
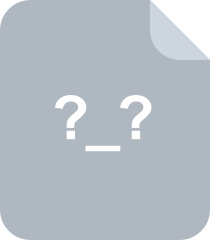
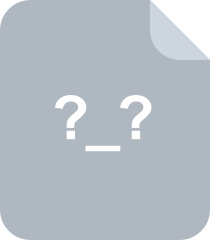
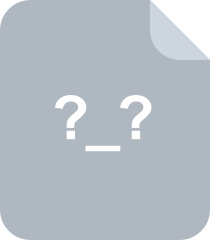
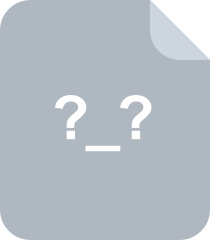
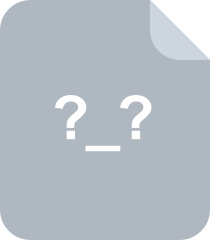
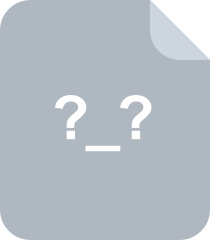
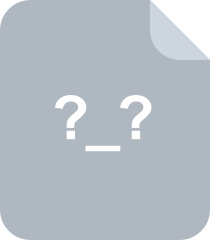
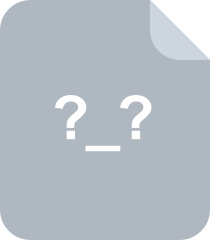
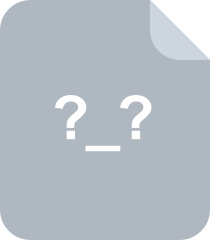
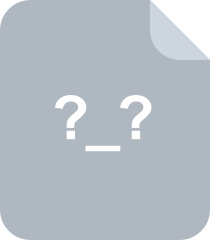
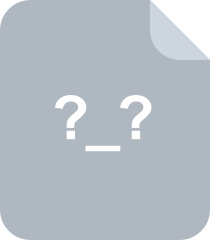
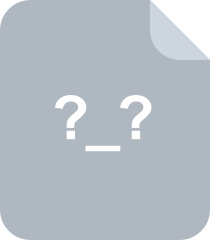
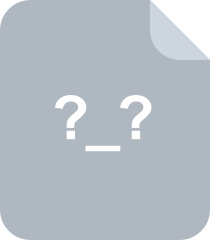
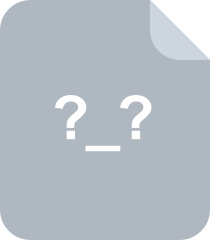
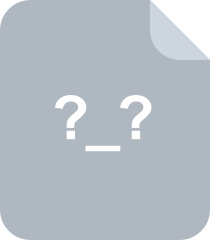
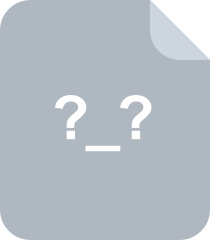
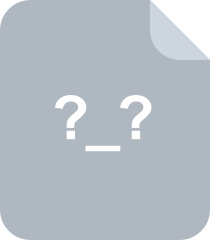
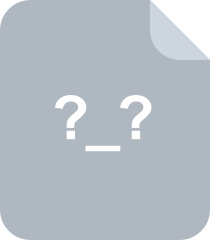
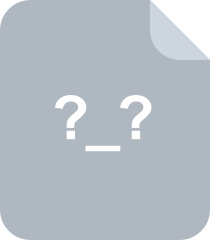
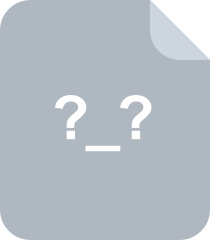
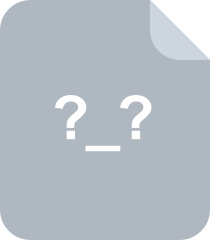
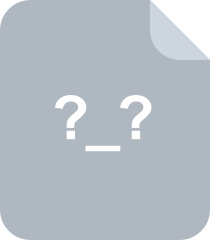
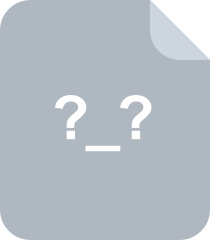
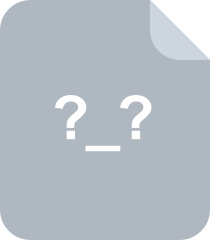
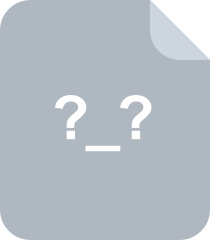
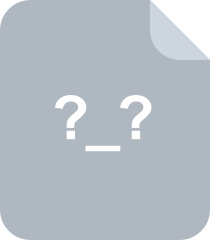
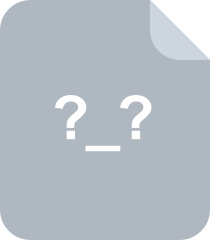
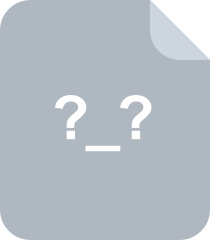
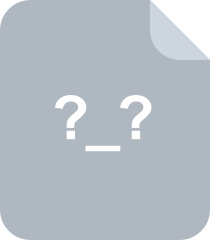
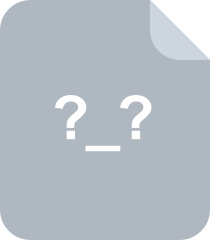
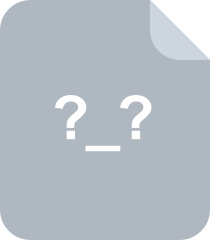
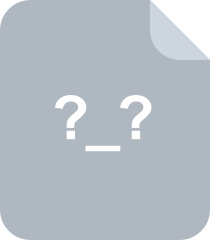
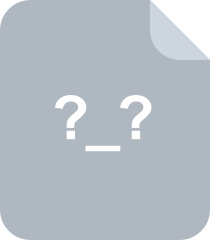
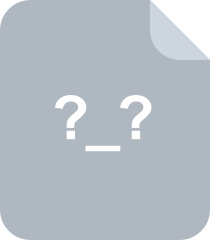
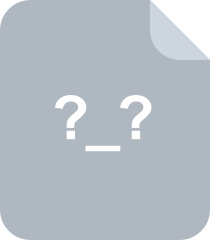
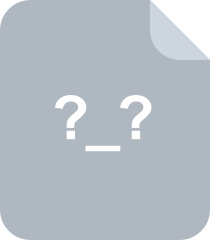
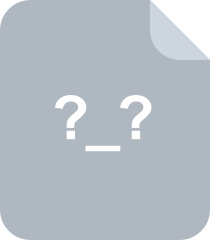
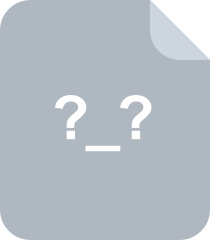
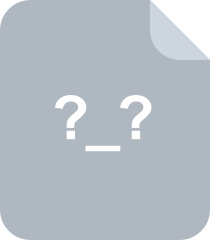
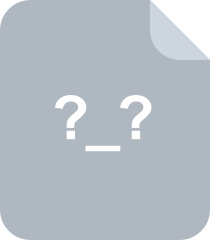
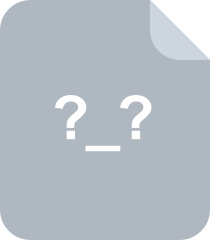
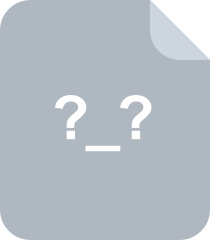
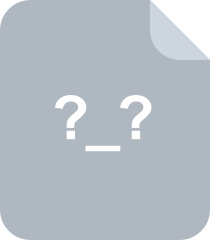
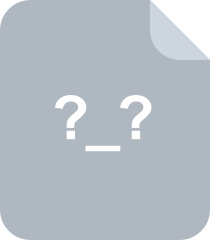
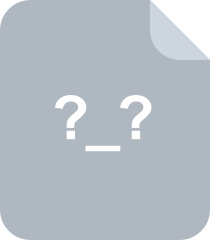
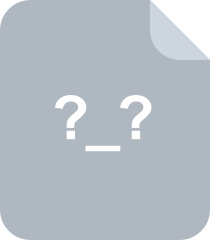
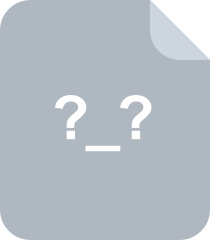
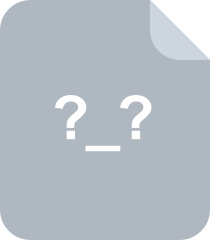
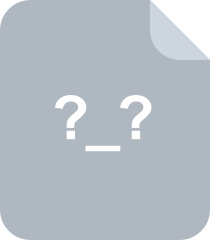
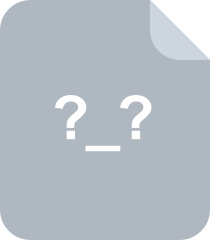
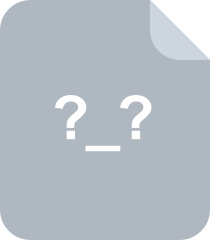
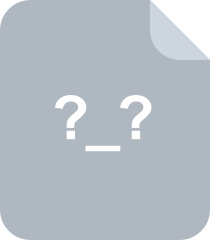
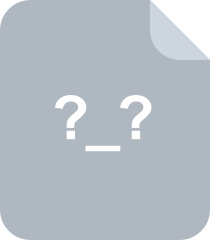
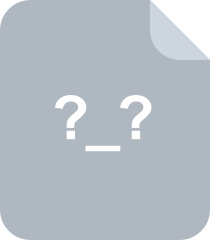
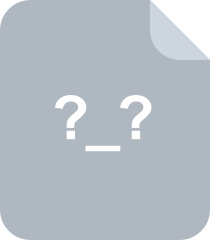
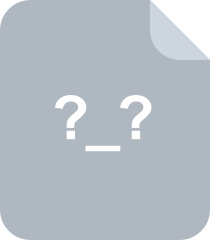
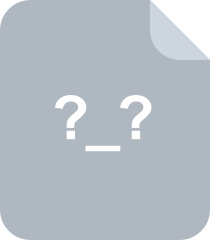
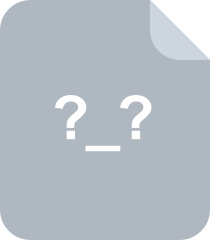
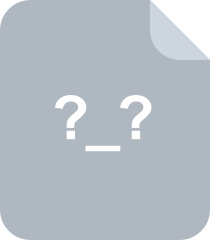
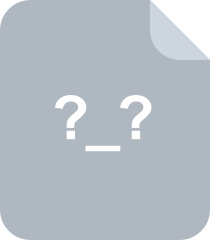
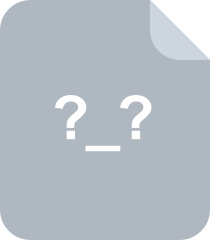
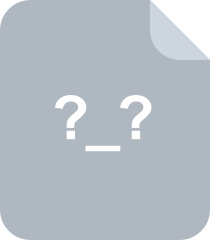
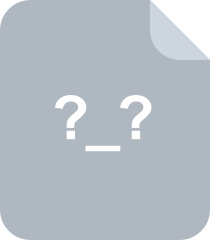
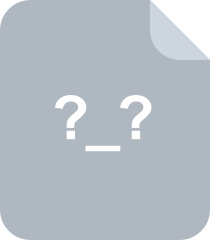
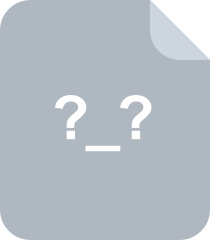
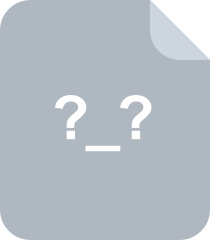
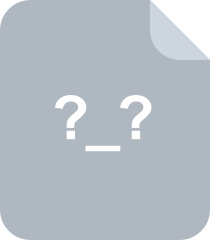
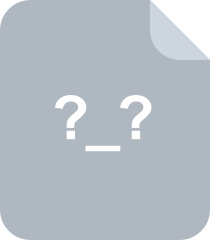
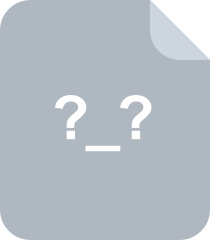
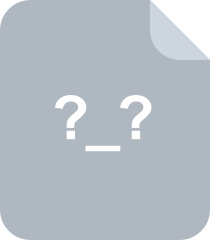
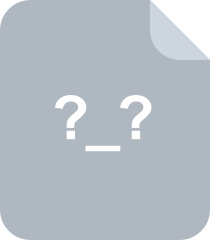
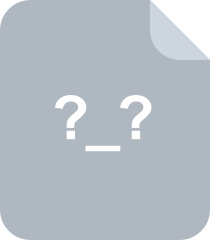
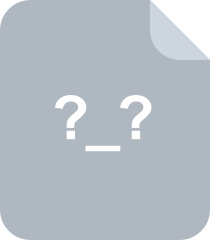
共 551 条
- 1
- 2
- 3
- 4
- 5
- 6
资源评论
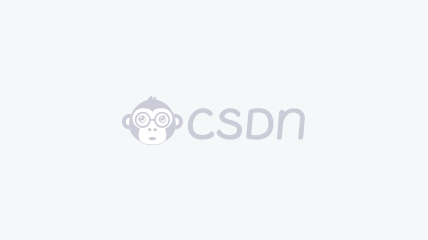

万物此臻
- 粉丝: 227
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

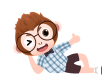
最新资源
- SQL查询一个值 的所处的数据库表和列名
- sql server 不同服务器之间数据库同步.zip
- 始终加密(Always Encrypted)在SQL Server中的应用.pdf
- matplotlib详细介绍(Python的2D绘图库)
- 超市管理系统java源代码+数据库100%好用.zip
- 非常好的点餐系统全部项目资料100%好用.zip
- 这个仓库包含多个包含Spring Boot的智能车示例,涵盖了各种功能和用例,适合学习和参考
- 非常好的会议预约管理系统源代码资料100%好用.zip
- 功能齐全的任务管理系统的设计方案,涵盖了基本的CRUD操作、用户管理、基本的安全控制以及前后端分离的设计
- 超市进销管理系统源代码资料.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


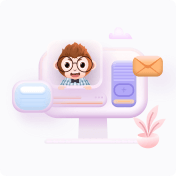
安全验证
文档复制为VIP权益,开通VIP直接复制
