package sc.util;
import java.math.BigDecimal;
import java.sql.Date;
import java.sql.Time;
import java.sql.Timestamp;
import java.text.DateFormat;
import java.text.NumberFormat;
import java.text.ParseException;
import java.text.SimpleDateFormat;
import java.util.Locale;
/**
* 类型转换辅助工具
* @version:1.0
*/
public class TypeCaseHelper {
/**
* 转换核心实现方法
*
* @param obj
* @param type
* @param format
* @return Object
* @throws TypeCastException
*/
public static Object convert(Object obj, String type, String format) throws TypeCastException {
Locale locale = new Locale("zh", "CN", "");
if (obj == null)
return null;
if (obj.getClass().getName().equals(type))
return obj;
if ("Object".equals(type) || "java.lang.Object".equals(type))
return obj;
String fromType = null;
if (obj instanceof String) {
fromType = "String";
String str = (String) obj;
if ("String".equals(type) || "java.lang.String".equals(type))
return obj;
if (str.length() == 0)
return null;
if ("Boolean".equals(type) || "java.lang.Boolean".equals(type)) {
Boolean value = null;
if (str.equalsIgnoreCase("TRUE"))
value = new Boolean(true);
else
value = new Boolean(false);
return value;
}
if ("Double".equals(type) || "java.lang.Double".equals(type))
try {
Number tempNum = getNf(locale).parse(str);
return new Double(tempNum.doubleValue());
} catch (ParseException e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
if ("BigDecimal".equals(type) || "java.math.BigDecimal".equals(type))
try {
BigDecimal retBig = new BigDecimal(str);
int iscale = str.indexOf(".");
int keylen = str.length();
if (iscale > -1) {
iscale = keylen - (iscale + 1);
return retBig.setScale(iscale, 5);
} else {
return retBig.setScale(0, 5);
}
} catch (Exception e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
if ("Float".equals(type) || "java.lang.Float".equals(type))
try {
Number tempNum = getNf(locale).parse(str);
return new Float(tempNum.floatValue());
} catch (ParseException e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
if ("Long".equals(type) || "java.lang.Long".equals(type))
try {
NumberFormat nf = getNf(locale);
nf.setMaximumFractionDigits(0);
Number tempNum = nf.parse(str);
return new Long(tempNum.longValue());
} catch (ParseException e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
if ("Integer".equals(type) || "java.lang.Integer".equals(type))
try {
NumberFormat nf = getNf(locale);
nf.setMaximumFractionDigits(0);
Number tempNum = nf.parse(str);
return new Integer(tempNum.intValue());
} catch (ParseException e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
if ("Date".equals(type) || "java.sql.Date".equals(type)) {
if (format == null || format.length() == 0)
try {
return Date.valueOf(str);
} catch (Exception e) {
try {
DateFormat df = null;
if (locale != null)
df = DateFormat.getDateInstance(3, locale);
else
df = DateFormat.getDateInstance(3);
java.util.Date fieldDate = df.parse(str);
return new Date(fieldDate.getTime());
} catch (ParseException e1) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
}
try {
SimpleDateFormat sdf = new SimpleDateFormat(format);
java.util.Date fieldDate = sdf.parse(str);
return new Date(fieldDate.getTime());
} catch (ParseException e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
}
if ("Timestamp".equals(type) || "java.sql.Timestamp".equals(type)) {
if (str.length() == 10)
str = str + " 00:00:00";
if (format == null || format.length() == 0)
try {
return Timestamp.valueOf(str);
} catch (Exception e) {
try {
DateFormat df = null;
if (locale != null)
df = DateFormat.getDateTimeInstance(3, 3, locale);
else
df = DateFormat.getDateTimeInstance(3, 3);
java.util.Date fieldDate = df.parse(str);
return new Timestamp(fieldDate.getTime());
} catch (ParseException e1) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
}
try {
SimpleDateFormat sdf = new SimpleDateFormat(format);
java.util.Date fieldDate = sdf.parse(str);
return new Timestamp(fieldDate.getTime());
} catch (ParseException e) {
throw new TypeCastException("Could not convert " + str + " to " + type + ": ", e);
}
} else {
throw new TypeCastException("Conversion from " + fromType + " to " + type + " not currently supported");
}
}
if (obj instanceof BigDecimal) {
fromType = "BigDecimal";
BigDecimal bigD = (BigDecimal) obj;
if ("String".equals(type))
return getNf(locale).format(bigD.doubleValue());
if ("BigDecimal".equals(type) || "java.math.BigDecimal".equals(type))
return obj;
if ("Double".equals(type))
return new Double(bigD.doubleValue());
if ("Float".equals(type))
return new Float(bigD.floatValue());
if ("Long".equals(type))
return new Long(Math.round(bigD.doubleValue()));
if ("Integer".equals(type))
return new Integer((int) Math.round(bigD.doubleValue()));
else
throw new TypeCastException("Conversion from " + fromType + " to " + type + " not currently supported");
}
if (obj instanceof Double) {
fromType = "Double";
Double dbl = (Double) obj;
if ("String".equals(type) || "java.lang.String".equals(type))
return getNf(locale).format(dbl.doubleValue());
if ("Double".equals(type) || "java.lang.Double".equals(type))
return obj;
if ("Float".equals(type) || "java.lang.Float".equals(type))
return new Float(dbl.floatValue());
if ("Long".equals(type) || "java.lang.Long".equals(type))
return new Long(Math.round(dbl.doubleValue()));
if ("Integer".equals(type) || "java.lang.Integer".equals(type))
return new Integer((int) Math.round(dbl.doubleValue()));
if ("BigDecimal".equals(type) || "java.math.BigDecimal".equals(type))
return new BigDecimal(dbl.toString());
else
throw new TypeCastException("Conversion from " + fromType + " to " + type + " not currently supported");
}
if (obj instanceof Float) {
fromType = "Float";
Float flt = (Float) obj;
if ("String".equals(type))
return getNf(locale).format(flt.doubleValue());
if ("BigDecimal".equals(type) || "java.math.BigDecimal".equals(type))
return new BigDecimal(flt.doubleValue());
if ("Double".equals(type))
return new Double(flt.doubleValue());
if ("Float".equals(type))
return obj;
if ("Long".equals(type))
return new Long(Math.round(flt.doubleValue()));
if ("Integer".equals(type))
return new Integer((int) Math.round(flt.doubleValue()));
else
throw new TypeCastException("Conversion from " + fromType + " to " + type + " not currently supported");
}
if (obj instanceof Long) {
fromType = "Long";
Long lng = (Long) obj;
if ("String".equals(type) || "java.lang.String".equals(type))
return getNf(locale).format(lng.longValue());
if ("Double".equals(type) || "java.lang.Double".equals(type))
return new Double(lng.doubleValue());
if ("Float".equals(type) || "java.lang.Float".equals(type))
return new Float(lng.floatValue());
if ("BigDecimal".equals(type) || "java.math.BigDecimal".equals(type))
return new BigDecimal(lng.toString());
if ("Long".equals(type) || "java.lang.Long".equals(type))
return obj;
if ("Integer".equals(type) || "java.lang.Integer".equals(type))
return new Int
没有合适的资源?快使用搜索试试~ 我知道了~
爬虫项目实例源码资源分享
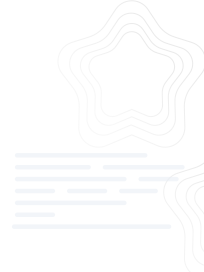
共344个文件
png:116个
jar:63个
class:39个

需积分: 1 0 下载量 82 浏览量
2024-01-16
21:13:35
上传
评论
收藏 40.55MB ZIP 举报
温馨提示
爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享爬虫项目实例源码资源分享
资源推荐
资源详情
资源评论
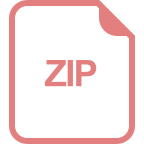
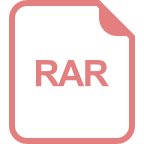
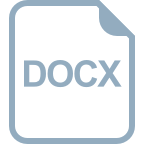
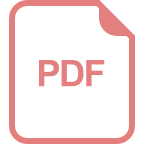
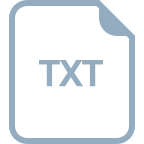
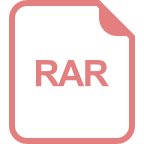
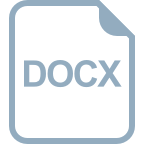
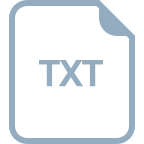
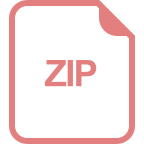
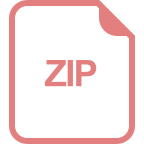
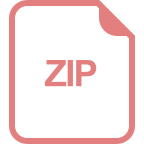
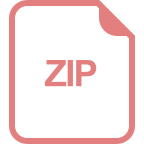
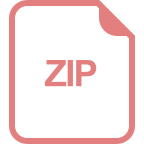
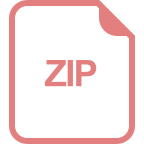
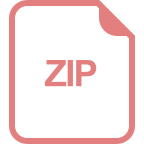
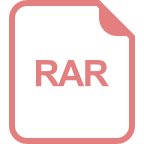
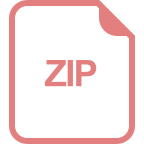
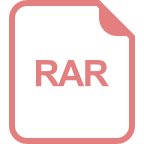
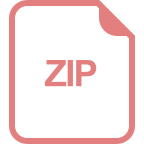
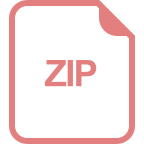
收起资源包目录

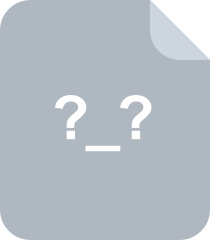
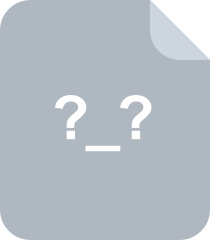
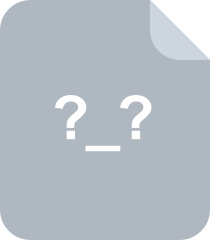
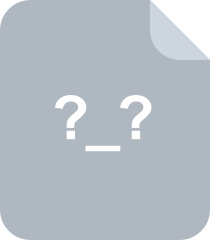
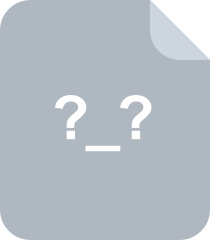
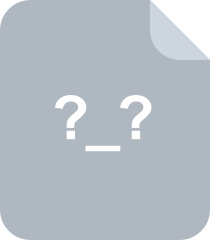
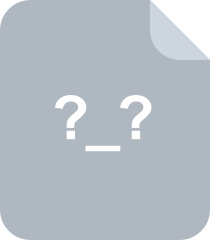
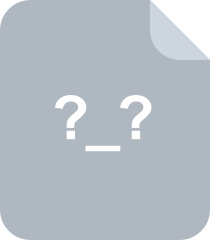
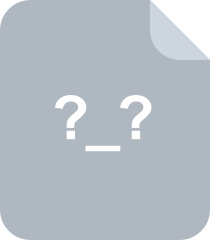
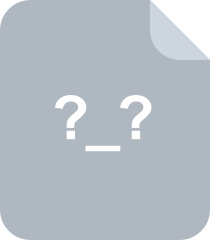
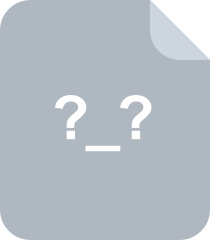
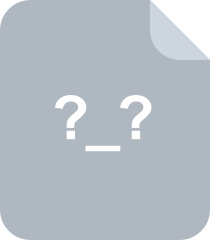
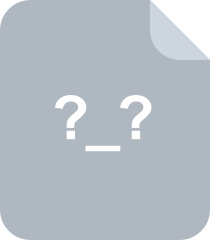
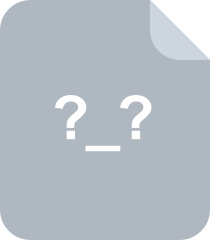
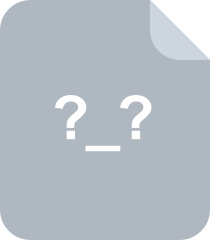
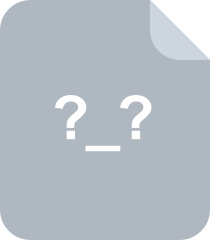
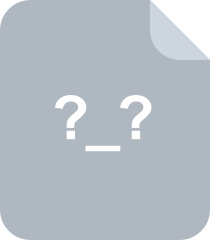
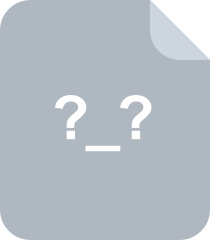
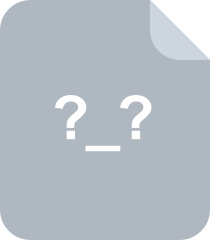
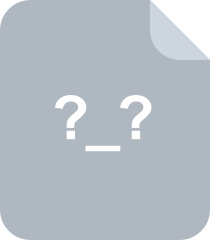
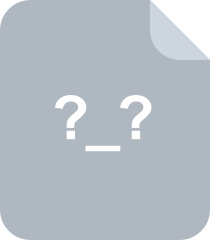
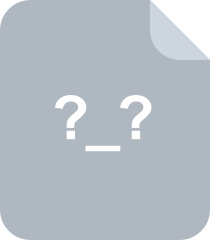
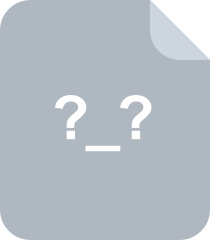
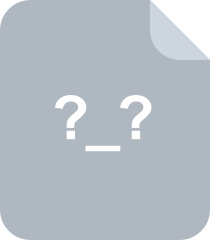
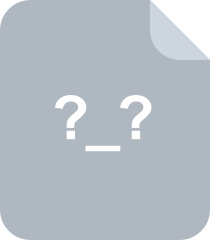
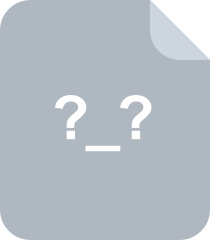
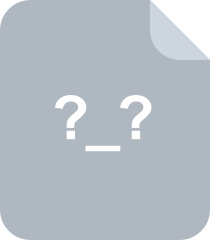
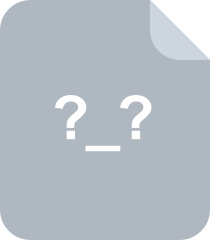
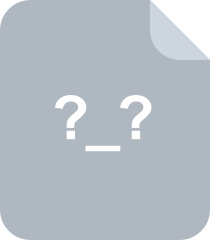
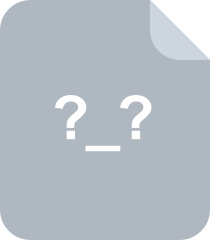
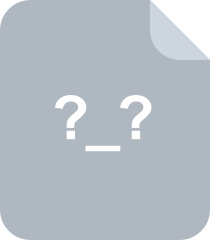
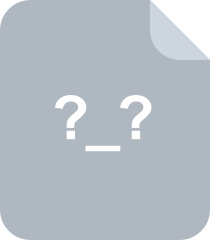
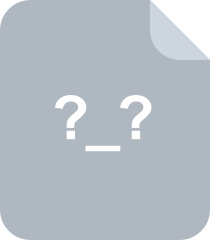
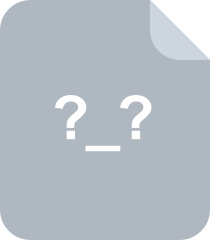
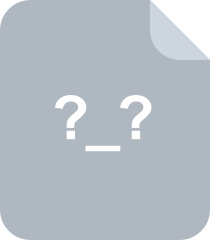
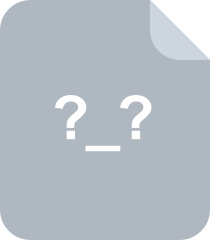
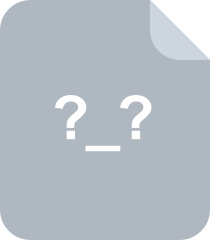
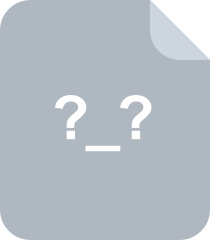
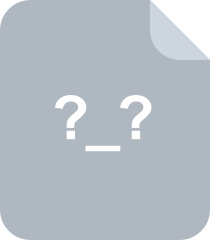
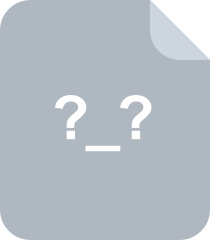
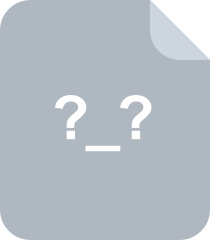
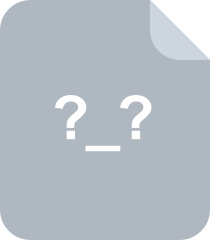
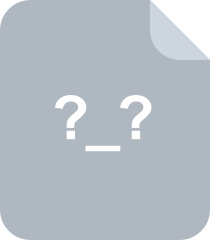
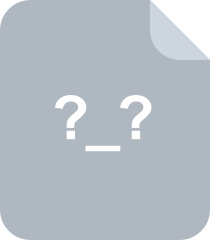
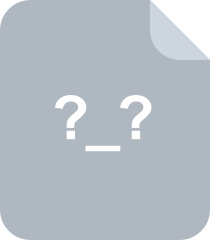
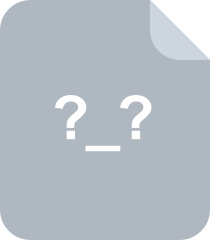
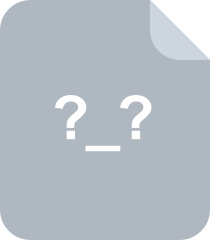
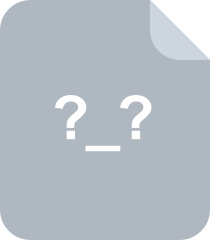
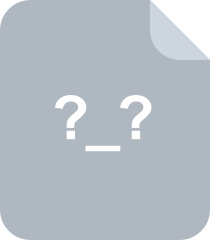
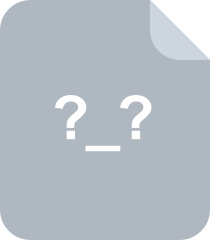
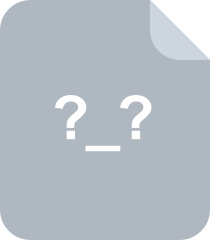
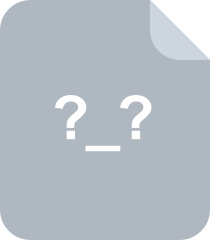
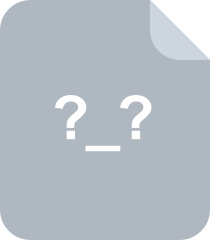
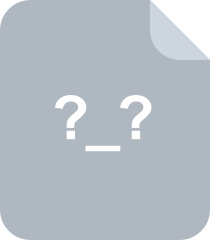
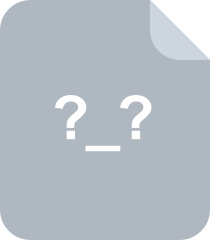
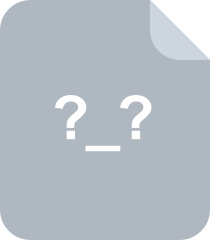
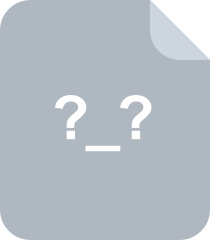
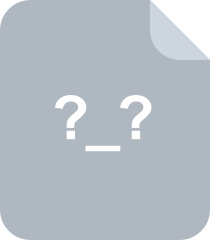
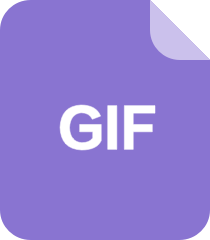
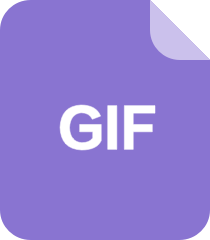
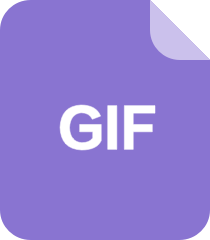
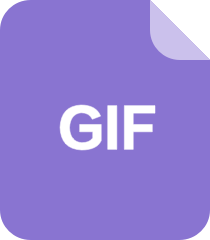
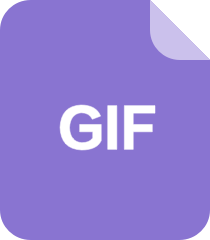
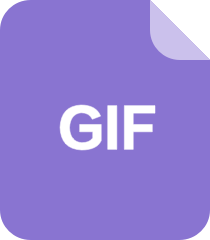
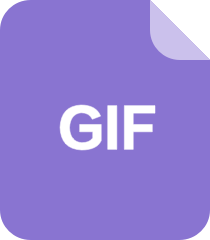
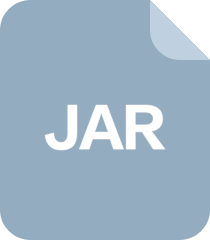
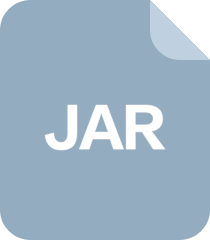
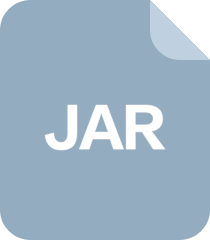
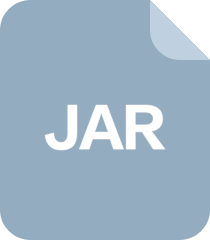
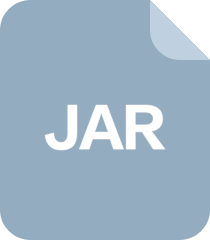
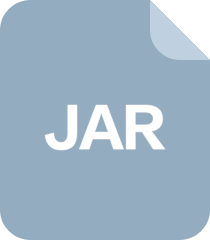
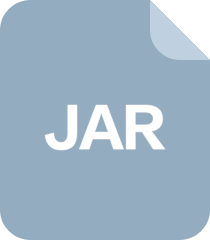
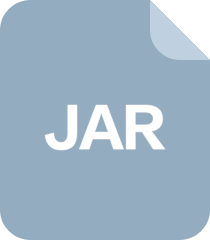
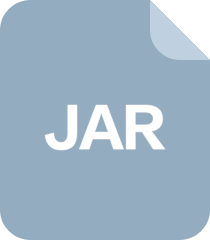
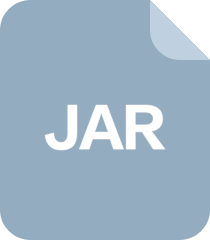
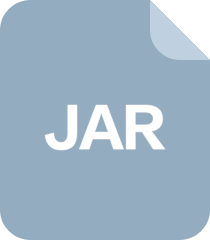
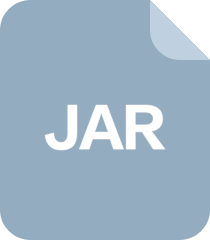
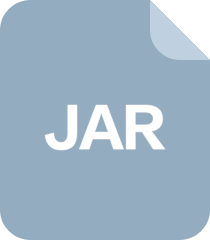
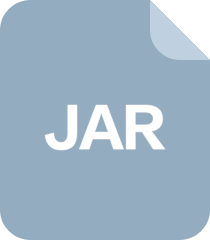
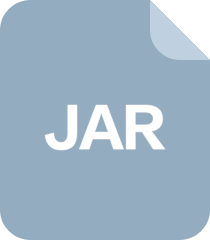
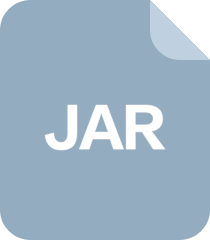
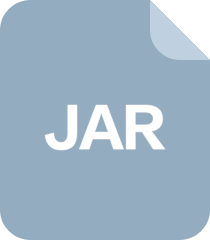
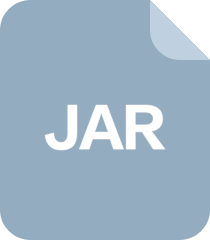
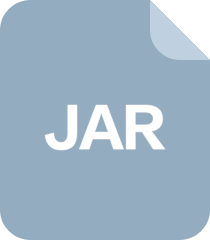
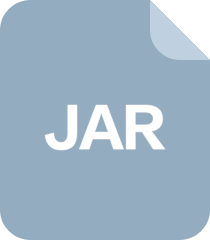
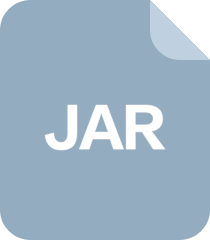
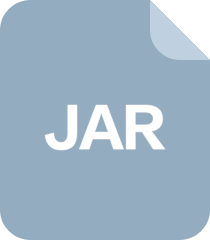
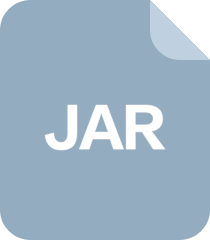
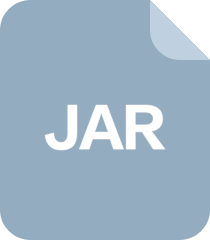
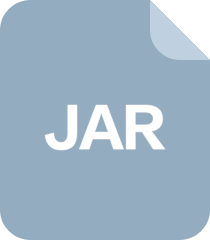
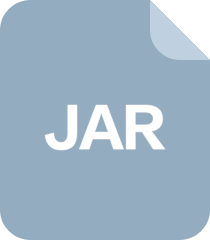
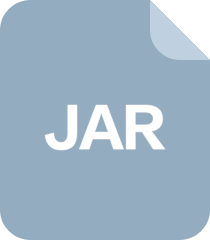
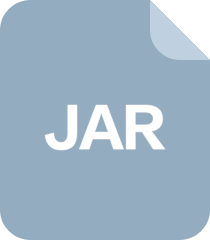
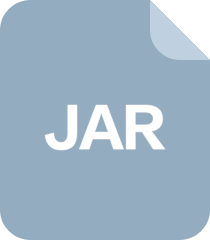
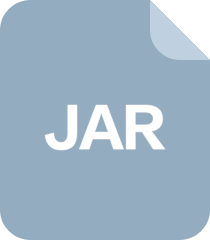
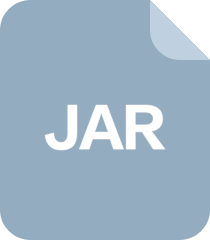
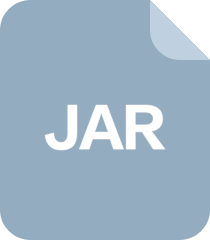
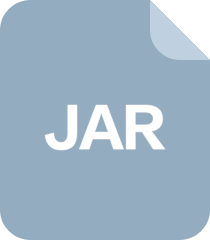
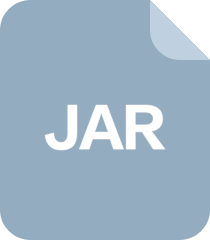
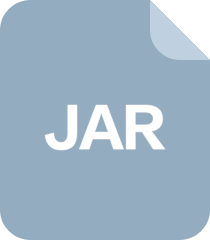
共 344 条
- 1
- 2
- 3
- 4
资源评论
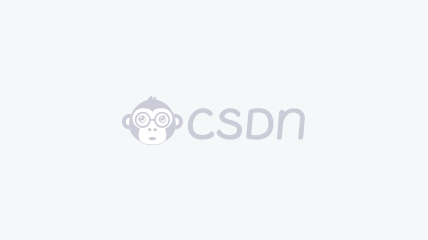

广寒舞雪
- 粉丝: 1392
- 资源: 155
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

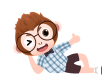
最新资源
- 全球前8GDP数据图(python动态柱状图)
- 汽车检测7-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 检测高压线电线-YOLO(v5至v9)、COCO、Darknet、VOC数据集合集.rar
- 检测行路中的人脸-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- Image_17083039753012.jpg
- 检测生锈铁片生锈部分-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、VOC数据集合集.rar
- 检测桌面物体-YOLO(v5至v9)、COCO、CreateML、Darknet、Paligemma、TFRecord、VOC数据集合集.rar
- 基于Java实现的动态操作实体属性及数据类型转换的设计源码
- x32dbg-And-x64dbg-for-windows逆向调试
- 检测是否佩戴口罩-YOLO(v5至v9)、Paligemma、TFRecord、VOC数据集合集.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


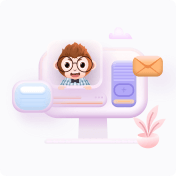
安全验证
文档复制为VIP权益,开通VIP直接复制
