java-core-assessment-framework.pdf
在当前的编程语言领域中,Java 无疑是最受欢迎的编程语言之一。由于其强大的社区支持、广泛的应用范围以及企业级应用的稳定性,Java 已经成为了许多公司的技术栈核心。根据文中的描述,Java 相关的工作需求在美国持续增长,根据数据目前有超过20,000个职位需要具备 Java 编程知识的候选人。因此,为了准确评估求职者的 Java 技能,开发一个具有权威性和科学性的 Java 面试评估框架变得尤为重要。 目前的技术招聘现状是,招聘者常常将简历作为技能水平的代理,这种方法导致了招聘和评估过程中的偏见和效率低下。尽管一些前瞻性的公司已经开始使用自动化评估工具来创建他们自己的测试,作为面试流程的第一个步骤,但这种方法存在两大问题。创建这些评估的内部团队不是测试设计方面的专家,这可能导致创建出不符合平等就业机会委员会(EEOC)规定的测试,或者过分强调某些技能,而忽视了其他可能对职位至关重要的技能。内部团队投入大量时间创建这些评估后,这些评估很多最终被发布在诸如 Glassdoor 和 Stack Overflow 等网站上,这使得测试结果的有效性变得值得质疑。 针对这一挑战,本文提出了一种名为 JavaSCA(Specialized Coding Assessment)的框架,旨在创建标准化测试来衡量 Java 编程技能和知识。基于研究确保这些测试具有高度的一致性和遵守 EEOC 的规定。此外,基于框架的测试使得可以大规模地创建题库,所有题目都遵循相同的指导方针。每次测试执行时,可以随机从题库中选择题目,从而降低考生提前记忆题目而获得不公平优势的风险。 JavaSCA 框架的设计不仅帮助了招聘人员了解求职者的能力,同时也为求职者和教育者提供了一种快速了解测试者技能的途径。它能够测量每位测试者的代码编写和调试能力,进而提供一个全面的评估结果。 在介绍 JavaSCA 框架的同时,文章指出,当前的教育资源越来越丰富,有关掌握 Java 的教育资料层出不穷。这为框架的建立提供了坚实的基础,使得标准化测试更加科学和有效。 总体来看,JavaSCA 框架的创建,意图超越简历评估,直接衡量求职者在 Java 编程上的实际能力。这不仅对技术招聘行业具有重大意义,而且对于教育者和求职者自身来说也是一个宝贵的学习和自我评估工具。通过这种方式,Java 技术社区可以朝着更加高效和公正的方向发展。
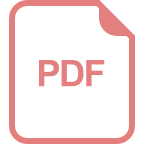
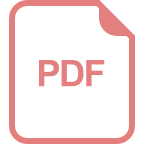
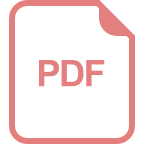
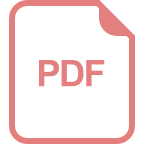
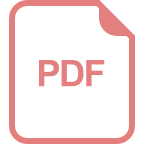
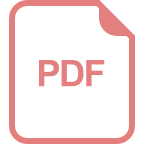
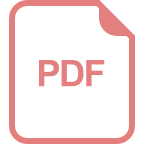
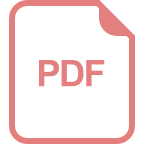
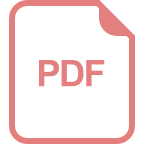
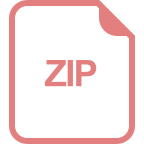
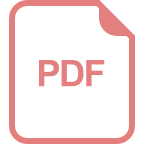
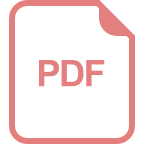
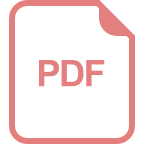
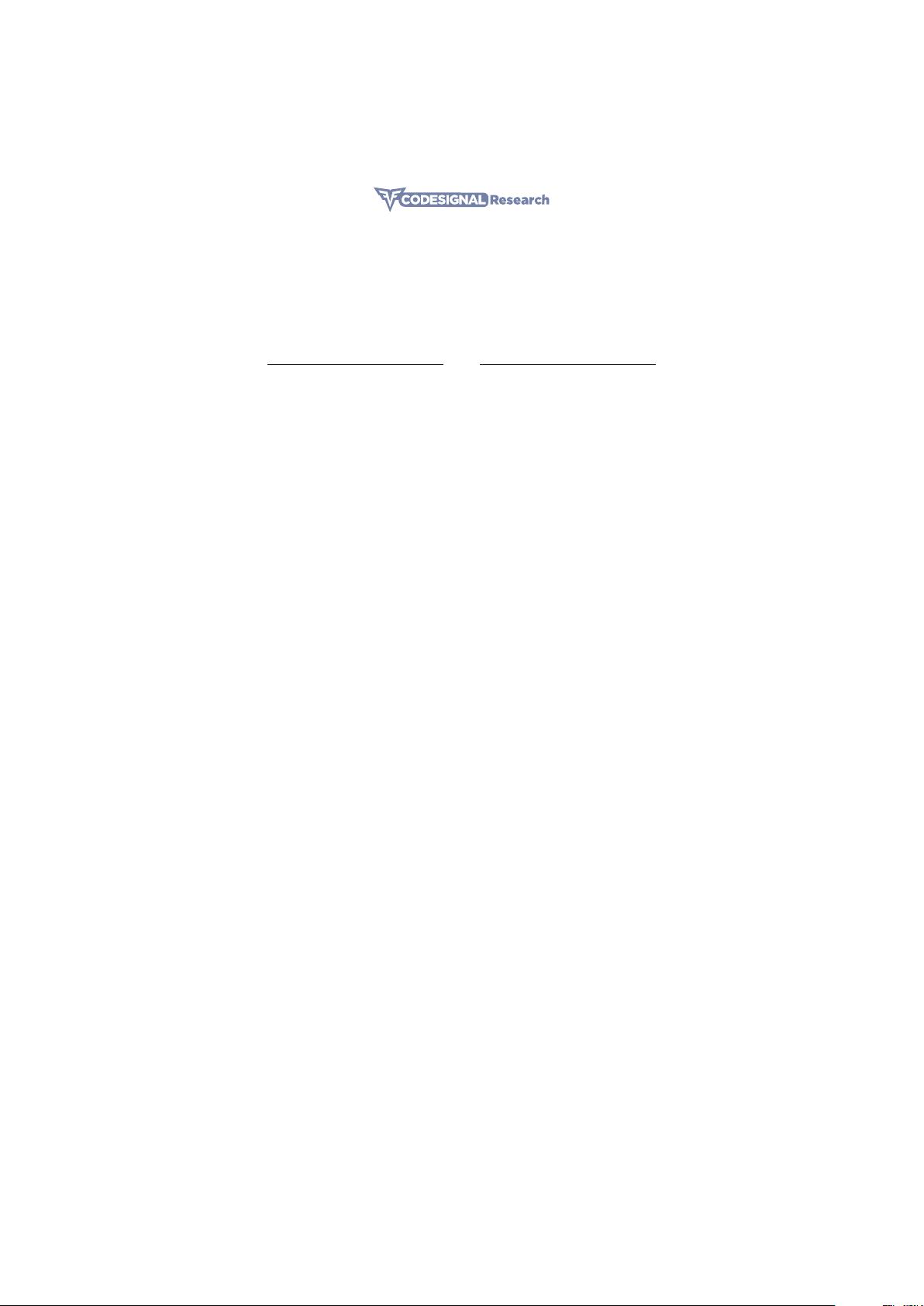
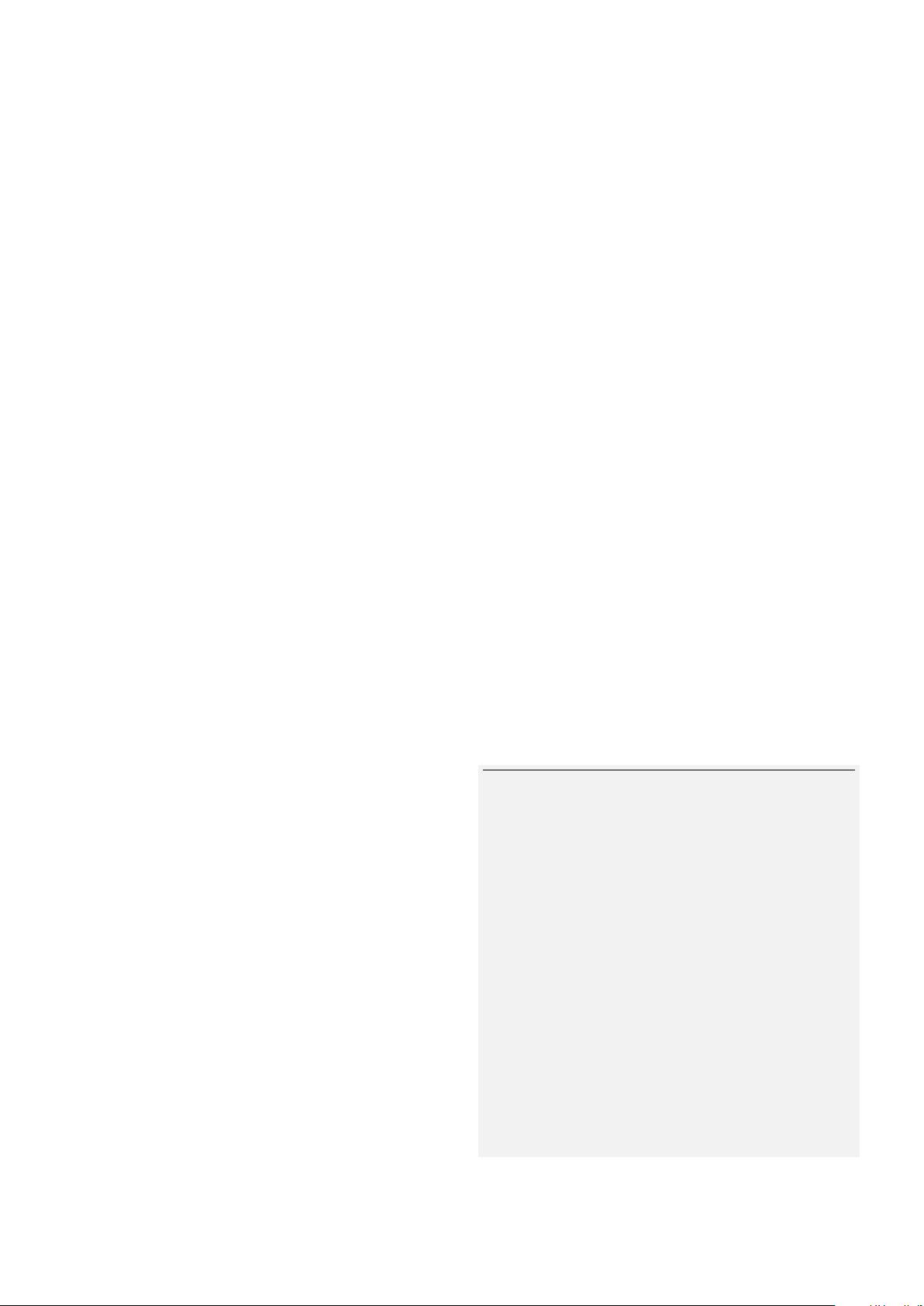
剩余9页未读,继续阅读
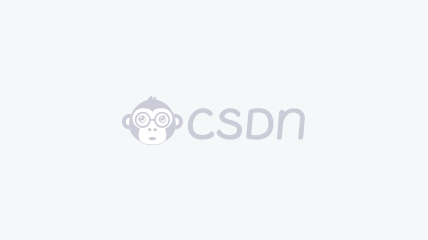

- 粉丝: 32
- 资源: 51
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

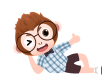
最新资源
- 毕设和企业适用springboot智慧交通平台类及物流管理平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及信息管理系统源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及远程教育平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及虚拟现实体验平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及用户行为分析平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及职业技能培训平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及智能客服系统源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及智能农场管理系统源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及自动化测试平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧教育平台类及AR技术平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧交通平台类及智能图像识别系统源码+论文+视频.zip
- 毕设和企业适用springboot智慧教育平台类及共享经济平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧教育平台类及大数据云平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧教育平台类及电子产品维修平台源码+论文+视频.zip
- 毕设和企业适用springboot智慧教育平台类及健康数据分析系统源码+论文+视频.zip
- 5Pin插针设备工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip

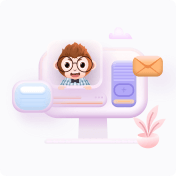
