1.给出一个整数数组,求其中任意两个元素之差的最大值。

根据给定文件的信息,我们可以将相关的知识点分为两个部分来详细阐述: ### 第一部分:求整数数组中任意两个元素之差的最大值 #### 题目背景与需求 题目要求给定一个整数数组,计算并返回数组中任意两个元素之差的最大值。 #### 解决方案 为了找到数组中任意两个元素之差的最大值,可以采用排序的方法。首先对数组进行排序,然后计算排序后数组中最大值与最小值之间的差,这个差即为所求的最大值。 #### 代码解析 在给定的部分代码中,作者采用了冒泡排序算法对数组进行排序。具体实现步骤如下: 1. **初始化数组**:创建了一个包含整数的数组`scores`。 2. **冒泡排序**: - 外层循环控制排序轮次; - 内层循环比较相邻元素大小,并交换位置(如果前一个元素大于后一个元素)。 3. **计算最大差值**:通过获取排序后的最大值和最小值,计算两者的差值。 4. **输出结果**:打印出最大差值。 #### 示例代码 ```csharp using System; namespace ConsoleApplication1 { class Program { static void Main(string[] args) { int[] scores = new int[] { 4, 5, 6, 3, 2, 9, 1, 8 }; int i, j; int temp, max = 0, min = 0; // 冒泡排序 for (i = 0; i < scores.Length - 1; i++) { for (j = 0; j < scores.Length - 1 - i; j++) { if (scores[j] > scores[j + 1]) { temp = scores[j]; scores[j] = scores[j + 1]; scores[j + 1] = temp; } } } // 计算最大值和最小值 max = scores[scores.Length - 1]; min = scores[0]; Console.WriteLine(max - min); Console.ReadLine(); } } } ``` ### 第二部分:求整数数组中出现次数最多的数 #### 题目背景与需求 题目要求给定一个整数数组,其中元素的取值范围为0到10000,求其中出现次数最多的数。 #### 解决方案 为了统计数组中每个数出现的次数,可以使用计数器数组的方式。首先创建一个长度为10001的数组(考虑到元素的最大值为10000),然后遍历输入数组,对于每个元素,在对应的计数器数组位置上加1。最后再遍历一次计数器数组,找出计数值最大的元素及其对应的原始数组中的值。 #### 代码解析 在给定的代码片段中,作者实现了上述解决方案: 1. **初始化计数器数组**:创建了一个长度为10001的整数数组`count`。 2. **遍历输入数组**:对于每个元素,更新计数器数组对应位置的计数值。 3. **寻找最大计数值**:遍历计数器数组,记录下计数值最大的元素及其对应的原始数组中的值。 4. **输出结果**:打印出出现次数最多的数及其出现次数。 #### 示例代码 ```csharp using System; namespace shiyan { class Program { public static void Main(string[] args) { int[] i = new int[] { 1, 2, 2, 3, 3, 3, 4, 4, 4, 4, 5, 5, 5, 5, 5, 6, 6, 6, 6, 6, 6, 7, 9, 9, 9, 9, 9, 9, 9, 9 }; int[] count = new int[10001]; int maxCount = 0; int maxResult = 0; // 统计每个元素出现次数 foreach (int index in i) { count[index + 1] += 1; } // 找出出现次数最多的数 for (int index = 0; index < 10000; index++) { if (count[index + 1] > maxCount) { maxCount = count[index + 1]; maxResult = index; } } // 输出结果 Console.Write("最多的是{0},出现了{1}次。", maxResult, maxCount); Console.Write("\nPress any key to continue"); Console.ReadKey(true); } } } ``` 以上就是从给定的文件中提取的相关知识点,包括了求解整数数组中任意两个元素之差的最大值以及求解整数数组中出现次数最多的数的详细解答。





















第一题答案:
namespace ConsoleApplication1
{
class Program
{
static void Main(string[] args)
{
int[] scores = new int[] { 4, 5, 6, 3, 2, 9, 1, 8 };
int i, j;
int temp,max=0,min=0;
for (i = 0; i < scores.Length - 1; i++)
{
for (j = 0; j < scores.Length - 1 - i; j++)
{
if (scores[j] > scores[j + 1])
{
temp = scores[j];
scores[j] = scores[j + 1];
scores[j + 1] = temp;
}
}
}
for (i = 0; i < scores.Length; i++)
{
max=scores[scores.Length-1];
min=scores[0];
}
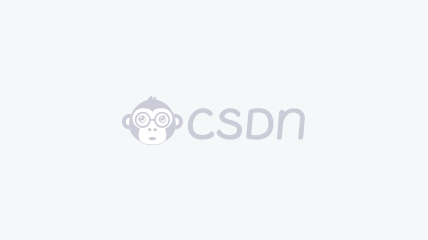
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整
- yuyanfei1252015-01-20资源还是不错的

- 粉丝: 6
- 资源: 5
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

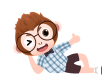
最新资源
- 基于MATLAB的含储能微网双层能量管理模型预测控制算法实现与优化
- B样条曲面拟合的四种形式及其MATLAB实现详解
- 基于Matlab的车型识别:小波变换与盒维数算法的应用及GUI实现
- 基于MATLAB的风光水场景生成与削减:蒙特卡洛法与概率距离快速削减法的应用
- 岩土工程中基于COMSOL的双重介质注浆模型模拟:探讨浆液在多孔介质与裂隙中的时变流动特性
- 基于S7-200PLC与MCGS组态的3x4堆垛式书架式自动化立体车库设计与实现
- 自动驾驶领域中CarSim与Simulink联合仿真的轨迹跟随、车道保持与横向控制实现
- 自动驾驶领域基于HRNet的图像分类:从PyTorch训练到TensorRT部署的技术详解
- 电力传输领域中HVDC双极系统的原理、优势及应用实例解析
- Comsol无偏振转换吸收器的多重干涉理论及Matlab脚本实现
- 混沌系统仿真与应用:Matlab实现混沌系统、电路仿真、同步控制及图像加密
- 电力系统仿真:基于PSCAD的500kV MMC柔性直流输电系统及其控制策略研究
- 电动汽车协调充电调度方法及其Python代码实现与优化
- 新能源汽车电机控制器FOC算法源代码解析及其优化技巧
- 西门子PLC新能源电池焊接1200程序:高效灵活的工业自动化解决方案
- 基于Maxwell的8极48槽V+1型永磁同步电机模型设计与仿真

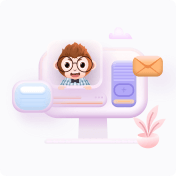
