### SQL常用语句详解 #### 创建数据库 在SQL中,创建数据库是一项基本操作,通过`CREATE DATABASE`命令来实现。创建数据库时,可以指定数据库的名字、初始大小、最大大小以及增长方式等属性。 ##### 示例代码解析 ```sql if exists (select * from sys.databases where name = 'students') drop database students; -- 创建名为students的数据库 create database students on (name = 'students_DATA', filename = 'E:\DataBase\S1T5004\(SQL)Students\students_DATA.mdf', size = 10MB, maxsize = 100MB, filegrowth = 10%) log on (name = 'students_LOG', filename = 'E:\DataBase\S1T5004\(SQL)Students\students_LOG.LDF', size = 1MB, maxsize = 5MB, filegrowth = 10%); ``` - **IF EXISTS**: 用于判断名为`students`的数据库是否存在。 - **DROP DATABASE**: 如果存在则删除该数据库。 - **CREATE DATABASE**: 创建一个新数据库`students`。 - **ON** 和 **LOG ON**: 指定数据库文件(`.mdf`)和日志文件(`.ldf`)的位置、名称、初始大小、最大大小以及自动增长比例。 - **FILENAME**: 数据库文件的具体路径。 - **SIZE**: 数据库文件或日志文件的初始大小。 - **MAXSIZE**: 文件的最大大小。 - **FILEGROWTH**: 文件自动增长的比例。 #### 创建表与约束 创建表是存储数据的基本单元。在创建表时,可以通过定义各种类型的字段来满足不同的需求,并且可以添加各种约束来确保数据的完整性。 ##### 示例代码解析 ```sql use students; -- 使用students数据库 if exists (select * from sys.objects where name = 'studentInfo') drop table studentInfo; -- 创建表studentInfo create table studentInfo ( stuNo varchar(10) not null, -- 学号 stuName varchar(20) not null, -- 姓名 stuSex char(2) not null, -- 性别 stuAge int not null, -- 年龄 stuPhone varchar(13), -- 联系电话 stuAddress text -- 地址 ); -- 添加主键约束 alter table studentInfo add constraint PK_stuNo PRIMARY KEY (stuNo); -- 添加检查约束 alter table studentInfo add constraint CK_stuNo CHECK (stuNo like 'N1HB042__'); -- 学号格式必须为N1HB042__ alter table studentInfo add constraint CK_stuSex CHECK (stuSex = '男' or stuSex = '女'); -- 性别只能为男或女 alter table studentInfo add constraint CK_stuAge CHECK (stuAge between 18 and 45); -- 年龄在18到45岁之间 -- 添加默认值约束 alter table studentInfo add constraint DF_stuAddress DEFAULT ('未知地址') for stuAddress; ``` - **USE**: 选择当前要使用的数据库。 - **IF EXISTS**: 检查表`studentInfo`是否存在,如果存在则删除。 - **CREATE TABLE**: 定义表`studentInfo`的结构。 - **ALTER TABLE**: 添加各种约束。 - **ADD CONSTRAINT**: 定义不同的约束类型。 - **PRIMARY KEY**: 主键约束,确保列值唯一。 - **CHECK**: 检查约束,限制列的取值范围。 - **DEFAULT**: 默认值约束,为没有赋值的列提供默认值。 #### 创建课程表与成绩表 创建课程表和成绩表是实现学生成绩管理系统的重要步骤之一。这些表可以帮助管理课程信息及学生的考试成绩。 ##### 示例代码解析 ```sql if exists (select * from sys.objects where name = 'course') drop table course; -- 创建课程表 create table course ( courseId int identity(1,1) not null, -- 课程ID,自增 courseName varchar(20) not null -- 课程名 ); -- 添加主键约束 alter table course add constraint PK_courseId PRIMARY KEY (courseId); if exists (select * from sys.objects where name = 'score') drop table score; -- 创建成绩表 create table score ( scoreId int identity(1,1) not null, -- 成绩ID,自增 score float not null, -- 分数 stuNo varchar(10) not null, -- 学号 courseId int not null -- 课程ID ); -- 添加主键约束 alter table score add constraint PK_scoreId PRIMARY KEY (scoreId); -- 添加检查约束 alter table score add constraint CK_score CHECK (score between 0 and 100); -- 添加外键约束 alter table score add constraint FK_stuNo FOREIGN KEY (stuNo) REFERENCES studentInfo(stuNo); alter table score add constraint FK_courseId FOREIGN KEY (courseId) REFERENCES course(courseId); ``` - **IDENTITY**: 生成连续递增的唯一值,通常用于自增ID。 - **FOREIGN KEY**: 外键约束,确保`score`表中的`stuNo`和`courseId`与`studentInfo`和`course`表中的对应列相匹配。 以上SQL语句覆盖了创建数据库、表及其约束的基本操作。理解这些命令对于构建可靠的数据库系统至关重要。
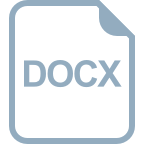
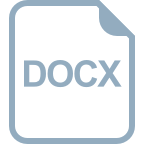
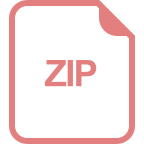
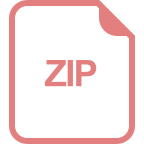
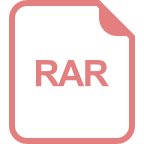
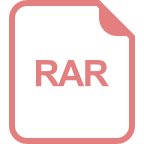
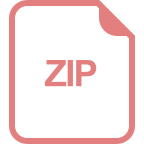
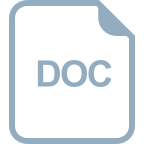
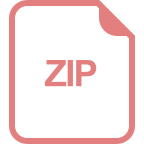
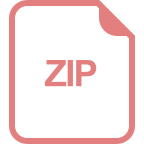
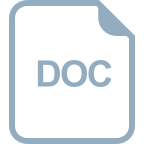
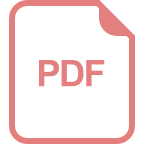
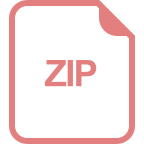
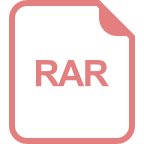
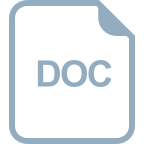
创建数据库
--判断students数据库是否存在,如果存在就删除这个数据库-
if exists(select * from sysdatabases where name='students')
drop database students
--如果不存在就创建student数据库--
create database students
name='students_DATA',--设置主数据文件的逻辑名
fileName='E:\DataBase\S1T5004\(SQL)Students\students_DATA.mdf',--设置数据文件的保存路径,及物理名
size=10mb,--设置数据库的初始化大小
maxSize=100,--设置数据库的最大容量
filegrowth=10%--设置数据库的自动增长率
设置日志文件
name='students_LOG',--设置日志文件的逻辑名
fileName='E:\DataBase\S1T5004\(SQL)Students\students_LOG.ldf',--设置日志文件的保存路径及物理文件名3
size=1mb,--设置日志文件的初始化大小
maxsize=5,--设置日志文件的最大容量L
filegrowth=10%--设置日志文件的自动增长率
创建学员基本信息表
Vuse students--切换students数据库为当前操作的数据库
判断studentinfo表是否存在,如果存在则删除--
if exists(select * from sysObjects where name='studentInfo')
drop table studentInfo
创建studentInfo表--
create table studentInfo
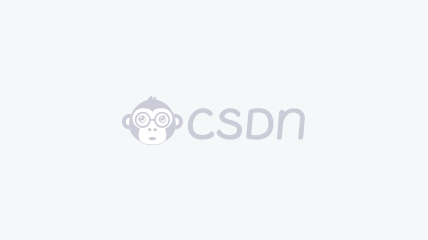

- 粉丝: 4
- 资源: 2
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

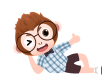
最新资源
- Java+Swing+Mysql的物资信息管理系统源码+文档说明(高分项目)
- (175345440)校园社区跑腿小程序源码.rar
- (175860602)基于51单片机的LCD1602矩阵键盘密码锁(proteus仿真设计)
- (176103642)「数学建模MATLAB必备程序源代码」方程求根源代码
- MATLAB代码:基于列约束生成法CCG的两阶段鲁棒问题求解 关键词:两阶段鲁棒 列约束生成法 CCG算法 鲁棒优化 参考文档:Solving two-stage robust optimizati
- (176167648)基于php+mysql的简易学生信息管理系统.zip
- 20232319 陈正勇.zip
- (176423806)Matlab与数学建模.doc
- (176962054)微同商城开源微信小程序商城(前后端开源:uniapp+Java) 快速搭建一个属于自己的微信小程序商城
- (177391846)毕业设计基于SpringBoot的在线拍卖系统源码含文档
- 酒店预订数据集.zip
- 基于粒子群算法的配电网无功优化 基于IEEE33节点配电网,以无功补偿器的接入位置和容量作为优化变量,以牛拉法进行潮流计算,以配电网网损最小为优化目标,通过优化求解,得到最佳接入位置和容量,优化结果如
- (177400018)pl2303USB转串口线驱动程序.zip
- (177488642)兼容在线/离线身份证读卡插件Windows PC 端SDK
- 中移M5311模块MQTT协议连接阿里云物联网平台(干货)
- (177506410)PHP学生管理系统 .zip

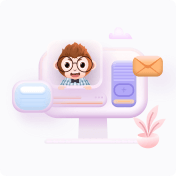
