/* -*- Mode:C; tab-width: 8 -*-
* remote.c --- remote control of Netscape Navigator for Unix.
* version 1.1.3, for Netscape Navigator 1.1 and newer.
*
* Copyright � 1996 Netscape Communications Corporation, all rights reserved.
* Created: Jamie Zawinski <jwz@netscape.com>, 24-Dec-94.
*
* Permission to use, copy, modify, distribute, and sell this software and its
* documentation for any purpose is hereby granted without fee, provided that
* the above copyright notice appear in all copies and that both that
* copyright notice and this permission notice appear in supporting
* documentation. No representations are made about the suitability of this
* software for any purpose. It is provided "as is" without express or
* implied warranty.
*
* To compile:
*
* cc -o netscape-remote remote.c -DSTANDALONE -lXmu -lX11
*
* To use:
*
* netscape-remote -help
*
* Documentation for the protocol which this code implements may be found at:
*
* http://home.netscape.com/newsref/std/x-remote.html
*
* Bugs and commentary to x_cbug@netscape.com.
*/
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <string.h>
#include <X11/Xlib.h>
#include <X11/Xatom.h>
#include <X11/Xmu/WinUtil.h> /* for XmuClientWindow() */
/* vroot.h is a header file which lets a client get along with `virtual root'
window managers like swm, tvtwm, olvwm, etc. If you don't have this header
file, you can find it at "http://home.netscape.com/newsref/std/vroot.h".
If you don't care about supporting virtual root window managers, you can
comment this line out.
*/
#include "vroot.h"
#ifdef STANDALONE
static const char *progname = 0;
static const char *expected_mozilla_version = "1.1";
#else /* !STANDALONE */
extern const char *progname;
extern const char *expected_mozilla_version;
#endif /* !STANDALONE */
#define MOZILLA_VERSION_PROP "_MOZILLA_VERSION"
#define MOZILLA_LOCK_PROP "_MOZILLA_LOCK"
#define MOZILLA_COMMAND_PROP "_MOZILLA_COMMAND"
#define MOZILLA_RESPONSE_PROP "_MOZILLA_RESPONSE"
static Atom XA_MOZILLA_VERSION = 0;
static Atom XA_MOZILLA_LOCK = 0;
static Atom XA_MOZILLA_COMMAND = 0;
static Atom XA_MOZILLA_RESPONSE = 0;
static void
mozilla_remote_init_atoms (Display *dpy)
{
if (! XA_MOZILLA_VERSION)
XA_MOZILLA_VERSION = XInternAtom (dpy, MOZILLA_VERSION_PROP, False);
if (! XA_MOZILLA_LOCK)
XA_MOZILLA_LOCK = XInternAtom (dpy, MOZILLA_LOCK_PROP, False);
if (! XA_MOZILLA_COMMAND)
XA_MOZILLA_COMMAND = XInternAtom (dpy, MOZILLA_COMMAND_PROP, False);
if (! XA_MOZILLA_RESPONSE)
XA_MOZILLA_RESPONSE = XInternAtom (dpy, MOZILLA_RESPONSE_PROP, False);
}
static Window
mozilla_remote_find_window (Display *dpy)
{
int i;
Window root = RootWindowOfScreen (DefaultScreenOfDisplay (dpy));
Window root2, parent, *kids;
unsigned int nkids;
Window result = 0;
Window tenative = 0;
unsigned char *tenative_version = 0;
if (! XQueryTree (dpy, root, &root2, &parent, &kids, &nkids))
{
fprintf (stderr, "%s: XQueryTree failed on display %s\n", progname,
DisplayString (dpy));
exit (2);
}
/* root != root2 is possible with virtual root WMs. */
if (! (kids && nkids))
{
fprintf (stderr, "%s: root window has no children on display %s\n",
progname, DisplayString (dpy));
exit (2);
}
for (i = nkids-1; i >= 0; i--)
{
Atom type;
int format, status;
unsigned long nitems, bytesafter;
unsigned char *version = 0;
Window w;
w = XmuClientWindow (dpy, kids[i]);
status = XGetWindowProperty (dpy, w, XA_MOZILLA_VERSION,
0, (65536 / sizeof (long)),
False, XA_STRING,
&type, &format, &nitems, &bytesafter,
&version);
if (! version)
continue;
if (strcmp ((char *) version, expected_mozilla_version) &&
!tenative)
{
tenative = w;
tenative_version = version;
continue;
}
XFree (version);
if (status == Success && type != None)
{
result = w;
break;
}
}
if (result && tenative)
{
fprintf (stderr,
"%s: warning: both version %s (0x%x) and version\n"
"\t%s (0x%x) are running. Using version %s.\n",
progname, tenative_version, (unsigned int) tenative,
expected_mozilla_version, (unsigned int) result,
expected_mozilla_version);
XFree (tenative_version);
return result;
}
else if (tenative)
{
#if 0
fprintf (stderr,
"%s: warning: expected version %s but found version\n"
"\t%s (0x%x) instead.\n",
progname, expected_mozilla_version,
tenative_version, (unsigned int) tenative);
#endif
XFree (tenative_version);
return tenative;
}
else if (result)
{
return result;
}
else
{
fprintf (stderr, "%s: not running on display %s\n", progname,
DisplayString (dpy));
exit (1);
}
}
static void
mozilla_remote_check_window (Display *dpy, Window window)
{
Atom type;
int format;
unsigned long nitems, bytesafter;
unsigned char *version = 0;
int status = XGetWindowProperty (dpy, window, XA_MOZILLA_VERSION,
0, (65536 / sizeof (long)),
False, XA_STRING,
&type, &format, &nitems, &bytesafter,
&version);
if (status != Success || !version)
{
fprintf (stderr, "%s: window 0x%x is not a Netscape window.\n",
progname, (unsigned int) window);
exit (6);
}
else if (strcmp ((char *) version, expected_mozilla_version))
{
fprintf (stderr,
"%s: warning: window 0x%x is Netscape version %s;\n"
"\texpected version %s.\n",
progname, (unsigned int) window,
version, expected_mozilla_version);
}
XFree (version);
}
static char *lock_data = 0;
static void
mozilla_remote_obtain_lock (Display *dpy, Window window)
{
Bool locked = False;
Bool waited = False;
if (! lock_data)
{
lock_data = (char *) malloc (255);
sprintf (lock_data, "pid%d@", getpid ());
if (gethostname (lock_data + strlen (lock_data), 100))
{
perror ("gethostname");
exit (-1);
}
}
do
{
int result;
Atom actual_type;
int actual_format;
unsigned long nitems, bytes_after;
unsigned char *data = 0;
XGrabServer (dpy); /* ################################# DANGER! */
result = XGetWindowProperty (dpy, window, XA_MOZILLA_LOCK,
0, (65536 / sizeof (long)),
False, /* don't delete */
XA_STRING,
&actual_type, &actual_format,
&nitems, &bytes_after,
&data);
if (result != Success || actual_type == None)
{
/* It's not now locked - lock it. */
#ifdef DEBUG_PROPS
fprintf (stderr, "%s: (writing " MOZILLA_LOCK_PROP
" \"%s\" to 0x%x)\n",
progname, lock_data, (unsigned int) window);
#endif
XChangeProperty (dpy, window, XA_MOZILLA_LOCK, XA_STRING, 8,
PropModeReplace, (unsigned char *) lock_data,
strlen (lock_data));
locked = True;
}
XUngrabServer (dpy); /* ################################# danger over */
XSync (dpy, False);
if (! locked)
{
/* We tried to grab the lock this time, and failed because someone
else is holding it already. So, wait for a PropertyDelete event
to come in, and try again. */
fprintf (stderr, "%s: window 0x%x is locked by %s; waiting...\n",
progname, (unsigned int) window, data);
waited = True;
while (1)
{
XEvent event;
XNextEvent (dpy, &event);
if (event.xany.type == DestroyNotify &&
event.xdestroywindow.window == window)
{
fprintf (stderr, "%s: window 0x%x unexpectedly destroyed.\n",
progname, (unsigned int) window);
exit (6);
}
else if (event.xany.type == PropertyNotify &&
event.xproperty.state == PropertyDelete &&
event.xproperty.window == window &&
event.xproperty.atom == XA_MOZILLA_LOCK)
{
/* Ok! Someone deleted their lock, so n
没有合适的资源?快使用搜索试试~ 我知道了~
网络工具网络 监控

温馨提示
网络 监控网络 监控网络 监控
资源推荐
资源详情
资源评论
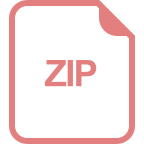
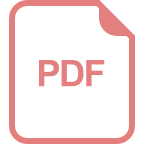
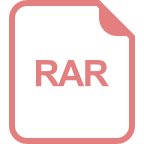
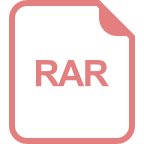
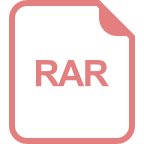
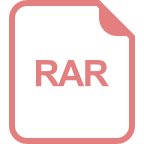
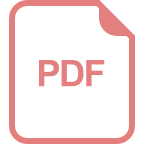
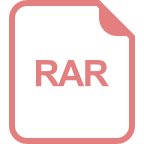
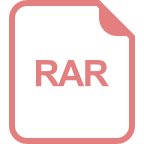
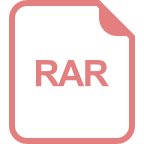
收起资源包目录

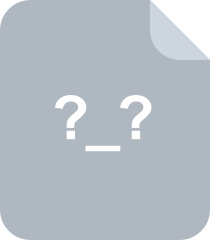
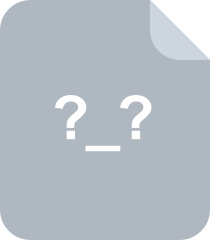
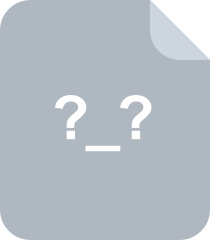
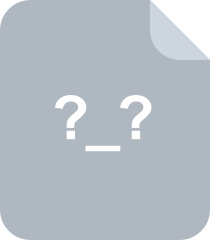
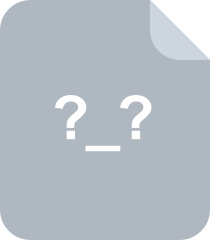
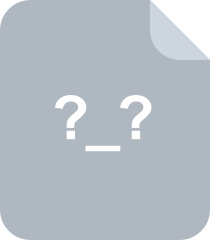
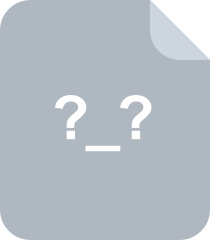
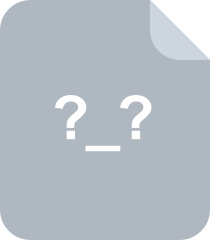
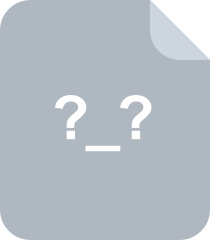
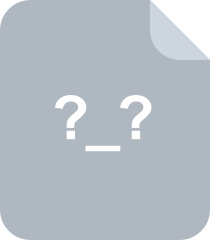
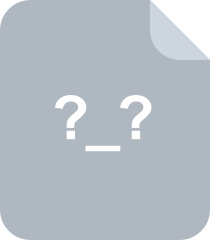
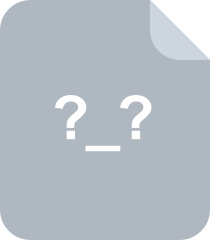
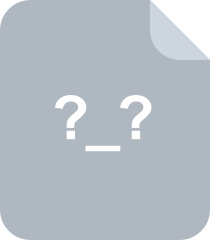
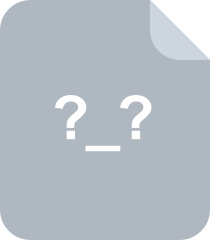
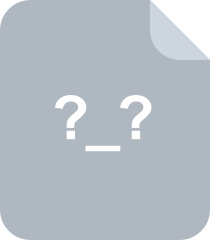
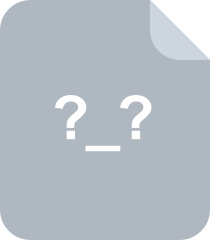
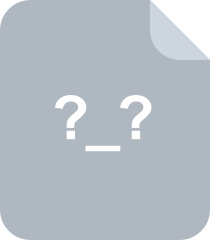
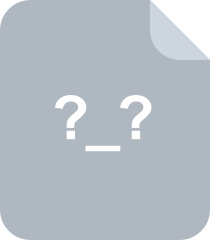
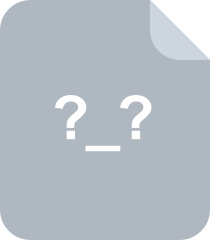
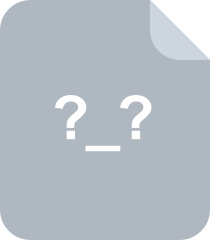
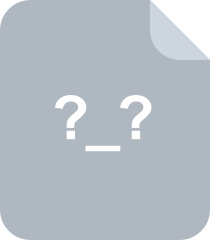
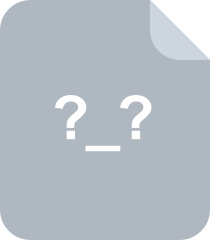
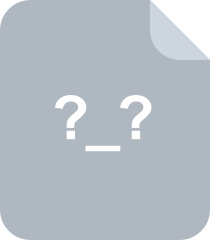
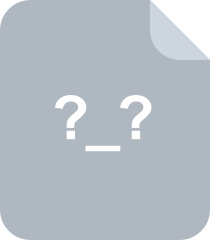
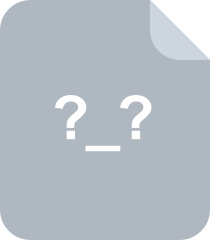
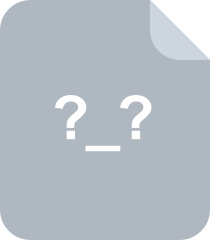
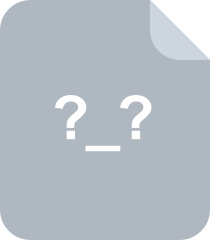
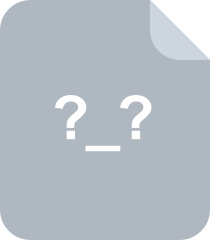
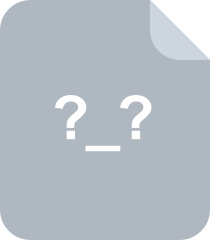
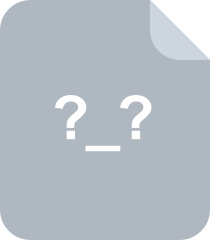
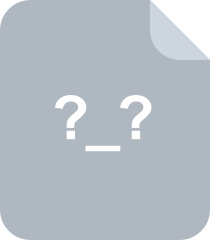
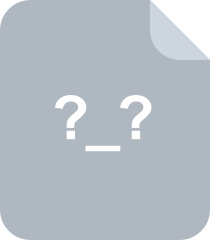
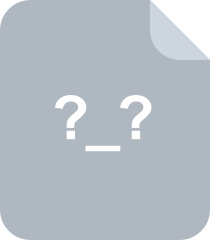
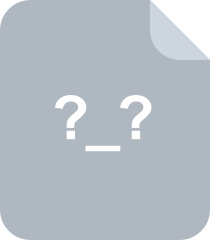
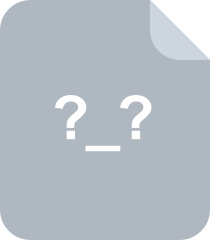
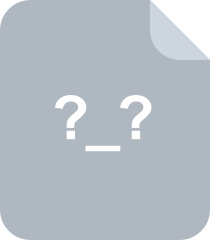
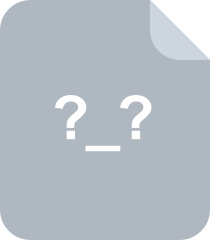
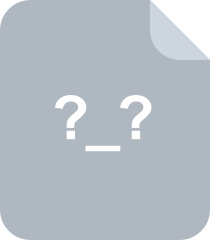
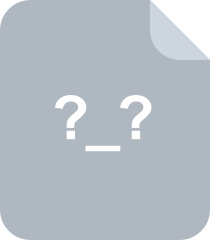
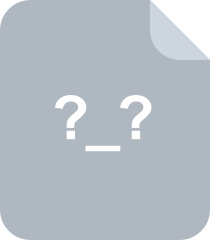
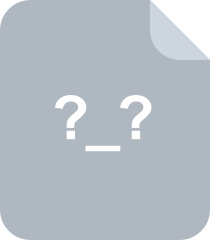
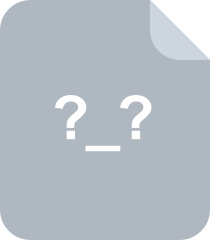
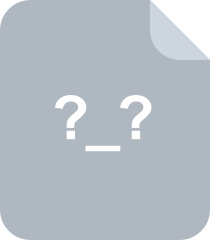
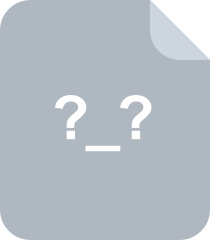
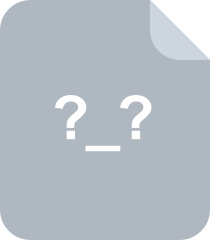
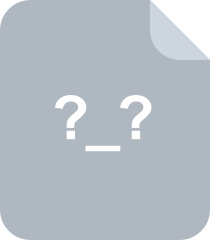
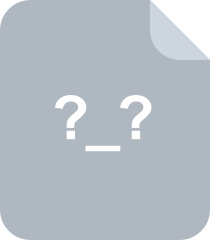
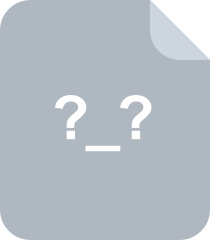
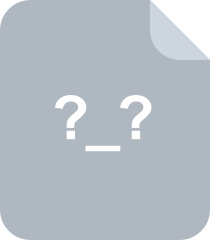
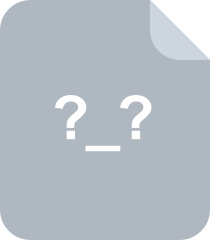
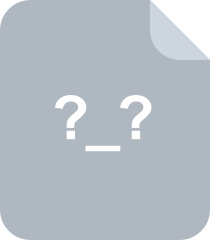
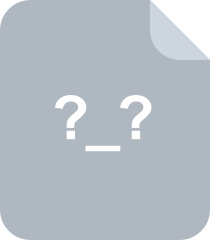
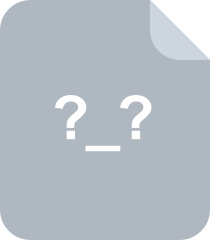
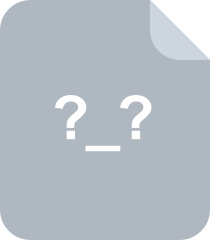
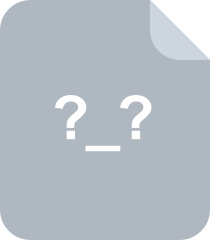
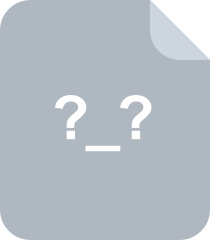
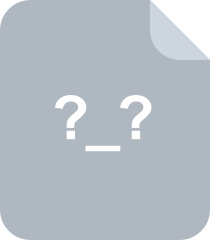
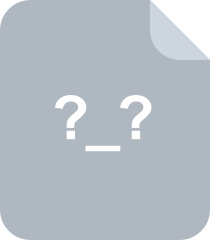
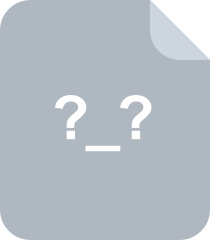
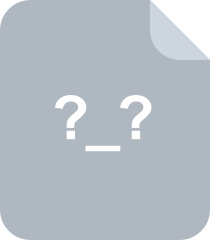
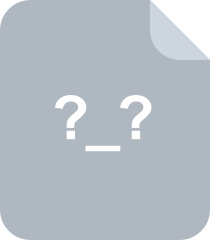
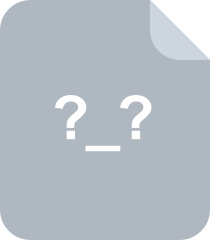
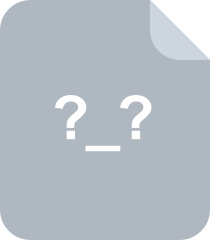
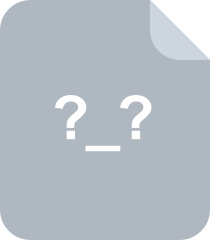
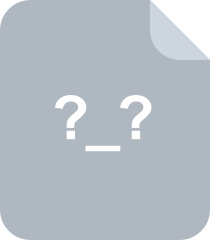
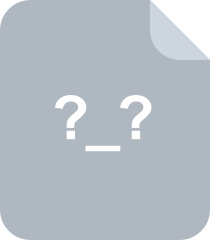
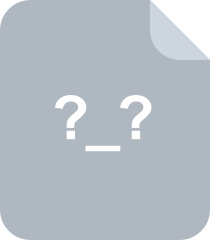
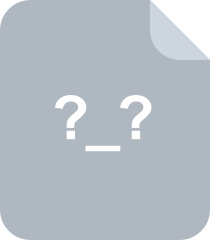
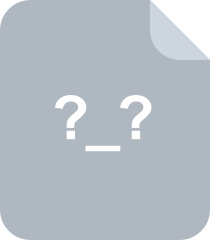
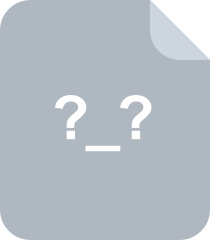
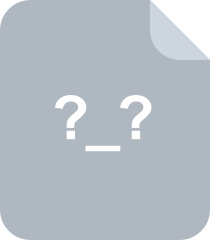
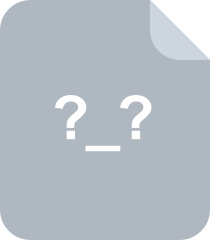
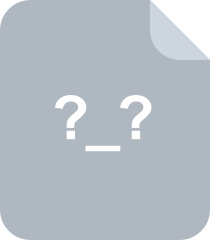
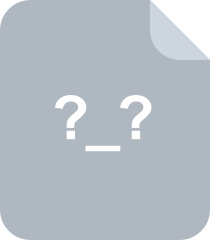
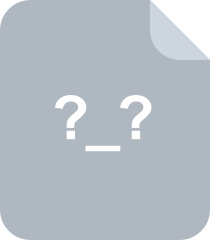
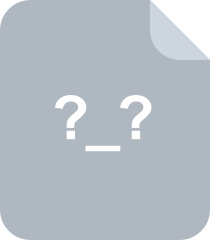
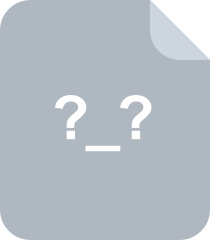
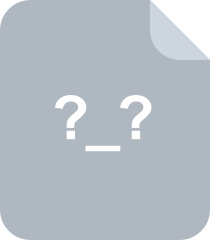
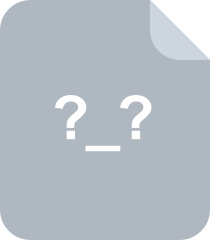
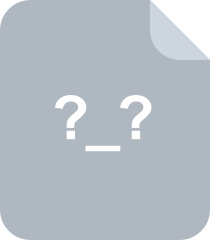
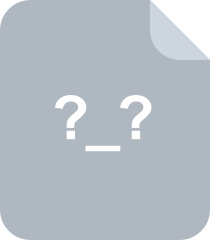
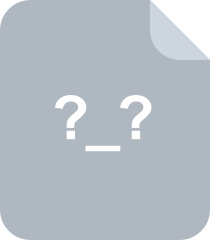
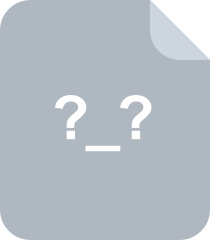
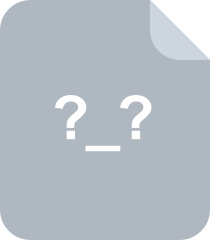
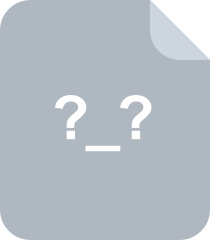
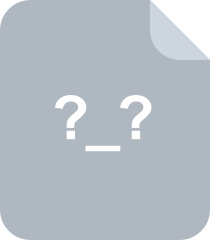
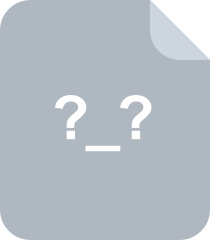
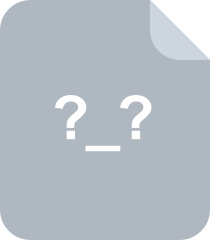
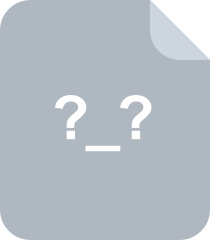
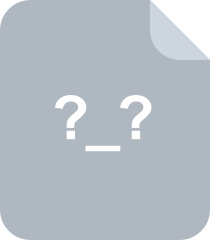
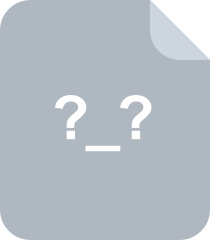
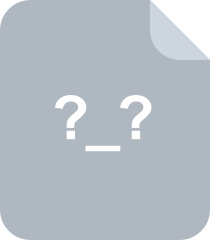
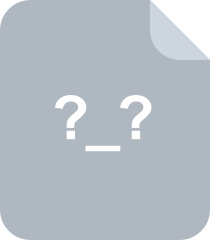
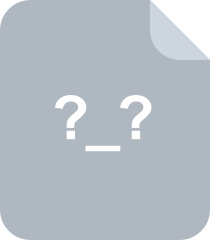
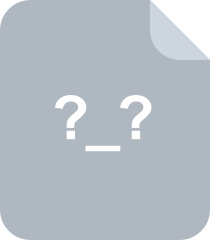
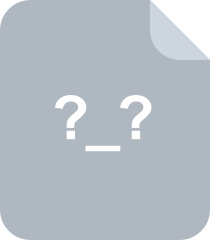
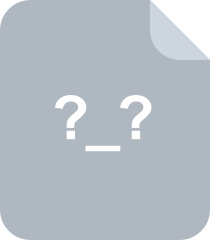
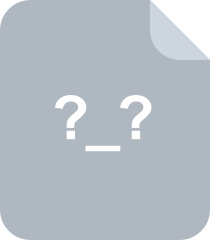
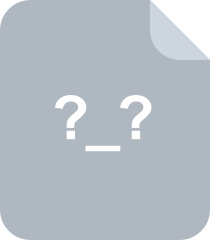
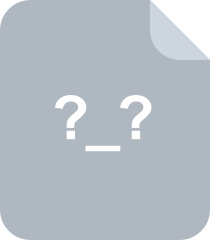
共 129 条
- 1
- 2
资源评论
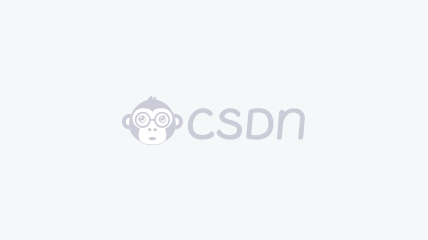
- 梁Gavin2013-06-04是源代码,没有装平台,需要的是程序
- ruanlinlin2011-10-18全部都是代码,有点小乱,不容易看懂。。。
- k7_xiaoyuyu2013-08-26代码看得头有点晕,表示有点压力!

dkqqos
- 粉丝: 0
- 资源: 1
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

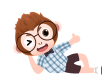
最新资源
- 爱心流星雨背景_超好看.zip
- 基于springboot+mybatis+mysql+vue音乐网站管理系统源码+数据库(高分毕业设计)
- DirectX 12图形引擎+网格算法库.zip
- 创维8K10机芯 U1系列 主程序软件 电视刷机 固件升级包 V014.002.251
- DirectX 12 编程第 4 卷示例.zip
- DirectX 12 编程第 1 卷示例.zip
- DirectX 12 离线安装程序适用于那些无法在其系统上运行在线安装程序的用户!.zip
- 计算机专业数据结构入门
- python《基于BERT的电商评论观点挖掘和情感分析》+项目源码+文档说明(高分作品)
- DirectX 12 示例实时体素化利用曲面细分进行原始处理和外推,以及利用深度剥离进行实体体素化 .zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


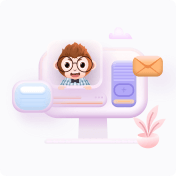
安全验证
文档复制为VIP权益,开通VIP直接复制
