/*
文件名: GameLayer.cpp
描 述: 游戏界面的实现
创建人: 郝萌主 (博客:http://blog.csdn.net/haomengzhu)
创建日期: 2013.12.05
*/
#include "GameLayer.h"
using namespace cocos2d;
GameLayer::~GameLayer() {
CC_SAFE_RELEASE(_jamMove);
CC_SAFE_RELEASE(_jamAnimate);
}
CCScene* GameLayer::scene()
{
CCScene * scene = NULL;
do
{
// 'scene' is an autorelease object
scene = CCScene::create();
CC_BREAK_IF(! scene);
// 'layer' is an autorelease object
GameLayer *layer = GameLayer::create();
CC_BREAK_IF(! layer);
// add layer as a child to scene
scene->addChild(layer);
} while (0);
// return the scene
return scene;
}
// on "init" you need to initialize your instance
bool GameLayer::init()
{
bool bRet = false;
do
{
//////////////////////////////////////////////////////////////////////////
// super init first
//////////////////////////////////////////////////////////////////////////
CC_BREAK_IF(! CCLayer::init());
//获取屏幕大小
_screenSize = CCDirector::sharedDirector()->getWinSize();
//创建游戏界面
createGameScreen();
//重新开始游戏
resetGame();
//listen for touches
this->setTouchEnabled(true);
//create main loop
this->schedule(schedule_selector(GameLayer::update));
bRet = true;
} while (0);
return bRet;
}
//重新开始游戏
void GameLayer::resetGame () {
//初始化配置
_score = 0;
_speedIncreaseInterval = 15;
_speedIncreaseTimer = 0;
//显示积分
char szValue[100] = {0};
sprintf(szValue, "%i", (int) _score);
_scoreDisplay->setString (szValue);
_scoreDisplay->setAnchorPoint(ccp(1,0));
_scoreDisplay->setPosition(ccp(_screenSize.width * 0.95f, _screenSize.height * 0.88f));
_state = kGameIntro;
_intro->setVisible(true);
_mainMenu->setVisible(true);
_jam->setPosition(ccp(_screenSize.width * 0.19f, _screenSize.height * 0.47f));
_jam->setVisible(true);
_jam->runAction(_jamAnimate);
_running = true;
}
void GameLayer::update(float dt) {
//CCLog("_running:%d",_running);
if (!_running)
return;
//获取主角位置
if (_player->getPositionY() < -_player->getHeight() ||
_player->getPositionX() < -_player->getWidth() * 0.5f) {
if (_state == kGamePlay) {
_running = false;
//create GAME OVER state
_state = kGameOver;
_tryAgain->setVisible(true);
_scoreDisplay->setAnchorPoint(ccp(0.5f, 0.5f));
_scoreDisplay->setPosition(ccp(_screenSize.width * 0.5f,
_screenSize.height * 0.88f));
_hat->setPosition(ccp(_screenSize.width * 0.2f, -_screenSize.height * 0.1f));
_hat->setVisible(true);
//旋转精灵,以度为单位
CCAction * rotate = CCRotateBy::create(2.0f, 660);
CCAction * jump = CCJumpBy::create(2.0f, ccp(0,10), _screenSize.height * 0.8f, 1);
//主角死亡,帽子旋转跳起
_hat->runAction(rotate);
_hat->runAction(jump);
//SimpleAudioEngine::sharedEngine()->stopBackgroundMusic();
//SimpleAudioEngine::sharedEngine()->playEffect("crashing.wav");
}
}
//更新主角状态
_player->update(dt);
_terrain->move(_player->getVector().x);
//检查碰撞冲突
if (_player->getState() != kPlayerDying)
_terrain->checkCollision(_player);
//放置主角
_player->place();
if (_player->getNextPosition().y > _screenSize.height * 0.6f) {
_gameBatchNode->setPositionY( (_screenSize.height * 0.6f - _player->getNextPosition().y) * 0.8f);
} else {
_gameBatchNode->setPositionY ( 0 );
}
//update paralax
if (_player->getVector().x > 0) {
//一直往左移
_background->setPositionX(_background->getPosition().x - _player->getVector().x * 0.25f);
float diffx;
//移完一个宽度时,重新把位置设置为接近0的位置
if (_background->getPositionX() < -_background->getContentSize().width) {
diffx = fabs(_background->getPositionX()) - _background->getContentSize().width;
_background->setPositionX(-diffx);
}
_foreground->setPositionX(_foreground->getPosition().x - _player->getVector().x * 4);
if (_foreground->getPositionX() < -_foreground->getContentSize().width * 4) {
diffx = fabs(_foreground->getPositionX()) - _foreground->getContentSize().width * 4;
_foreground->setPositionX(-diffx);
}
int count = _clouds->count();
CCSprite * cloud;
for (int i = 0; i < count; i++) {
cloud = (CCSprite *) _clouds->objectAtIndex(i);
cloud->setPositionX(cloud->getPositionX() - _player->getVector().x * 0.15f);
if (cloud->getPositionX() + cloud->boundingBox().size.width * 0.5f < 0 )
cloud->setPositionX(_screenSize.width + cloud->boundingBox().size.width * 0.5f);
}
}
if (_jam->isVisible()) {
if (_jam->getPositionX() < -_screenSize.width * 0.2f) {
_jam->stopAllActions();
_jam->setVisible(false);
}
}
if (_terrain->getStartTerrain() && _player->getVector().x > 0) {
_score += dt * 50;
char szValue[100] = {0};
sprintf(szValue, "%i", (int) _score);
_scoreDisplay->setString (szValue);
_speedIncreaseTimer += dt;
if (_speedIncreaseTimer > _speedIncreaseInterval) {
_speedIncreaseTimer = 0;
_player->setMaxSpeed (_player->getMaxSpeed() + 4);
}
}
if (_state > kGameTutorial) {
if (_state == kGameTutorialJump) {
if (_player->getState() == kPlayerFalling && _player->getVector().y < 0) {
_player->stopAllActions();
_jam->setVisible(false);
_jam->stopAllActions();
_running = false;
_tutorialLabel->setString("While in the air, tap the screen to float.");
_state = kGameTutorialFloat;
}
} else if (_state == kGameTutorialFloat) {
if (_player->getPositionY() < _screenSize.height * 0.95f) {
_player->stopAllActions();
_running = false;
_tutorialLabel->setString("While floating, tap the screen again to drop.");
_state = kGameTutorialDrop;
}
} else {
_tutorialLabel->setString("That's it. Tap the screen to play.");
_state = kGameTutorial;
}
}
}
void GameLayer::ccTouchesBegan(CCSet* pTouches, CCEvent* event) {
CCTouch *touch = (CCTouch *)pTouches->anyObject();
if (touch) {
CCPoint tap = touch->getLocation();
switch (_state) {
case kGameIntro:
break;
case kGameOver:
if (_tryAgain->boundingBox().containsPoint(tap)) {
_hat->setVisible(false);
_hat->stopAllActions();
_tryAgain->setVisible(false);
_terrain->reset();
_player->reset();
resetGame();
}
break;
case kGamePlay:
if (_player->getState() == kPlayerFalling) {
_player->setFloating ( _player->getFloating() ? false : true );
} else {
if (_player->getState() != kPlayerDying) {
//SimpleAudioEngine::sharedEngine()->playEffect("jump.wav");
_player->setJumping(true);
}
}
_terrain->activateChimneysAt(_player);
break;
case kGameTutorial:
_tutorialLabel->setString("");
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
尊重开发者的劳动成果,转载的时候请务必注明出处:http://blog.csdn.net/haomengzhu/article/details/17333123 【cocos2d-x IOS游戏开发-城市跑酷】跑酷游戏介绍 游戏特色: 1、自己创建属于自己的城市以及繁荣的街区 2、随机的屋顶与意想不到的坑等你来挑战 3、操作教程 4、简单的操作:你只要点击屏幕就能跳起来,双击屏幕你就能飞起来,是不是感觉很刺激!! 5、主角跳起来的时候屋顶的烟囱会冒烟来为主角加油 6、主角与街区、屋顶、以及坑的碰撞 7、主角死亡的动画 8、跑酷积分统计
资源推荐
资源详情
资源评论
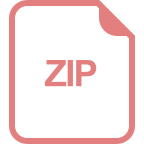
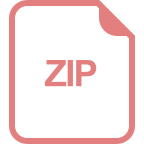
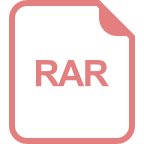
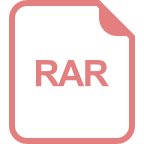
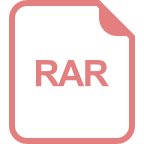
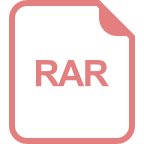
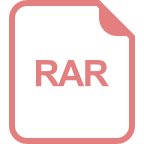
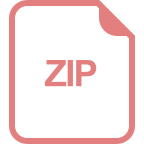
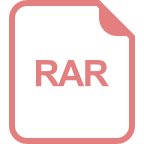
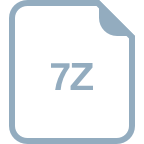
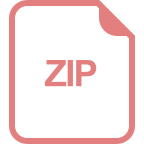
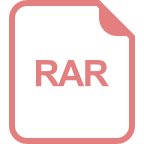
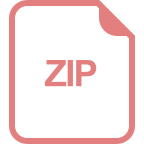
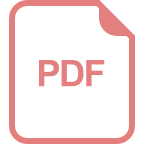
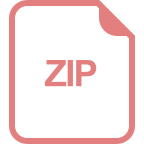
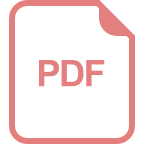
收起资源包目录



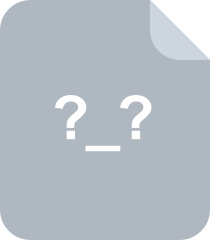
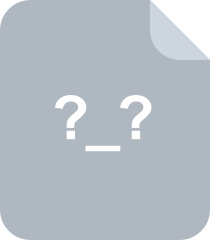
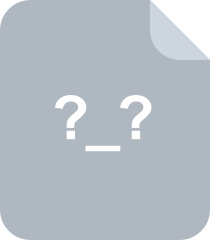

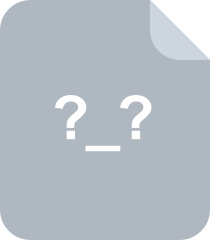

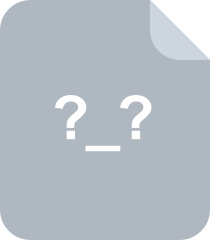
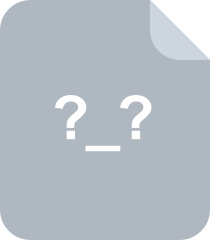
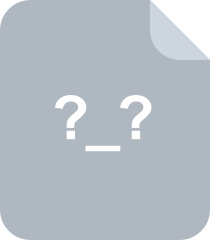
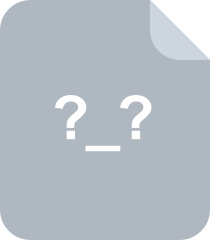
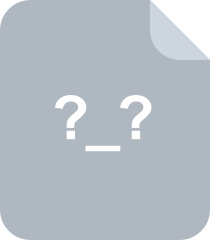
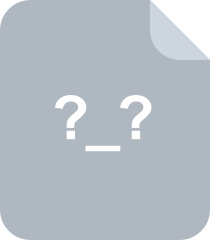
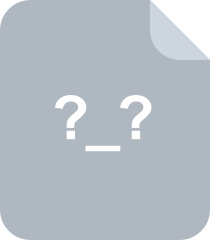
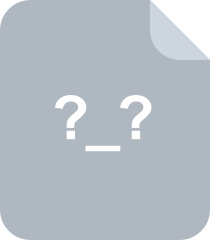
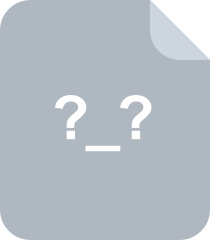
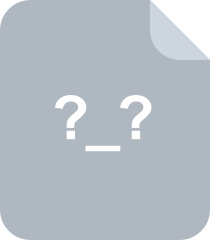
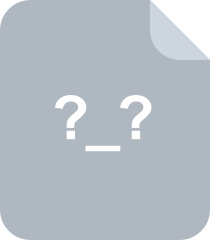
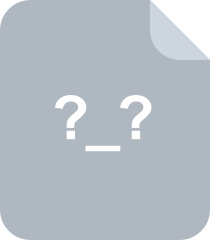

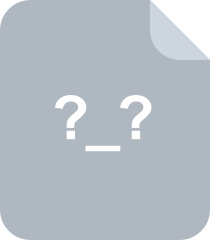
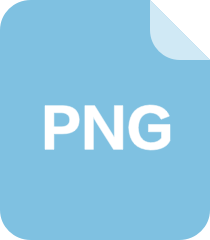
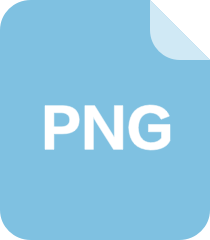
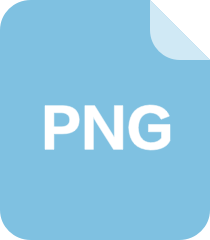
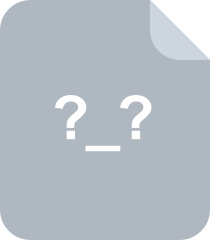

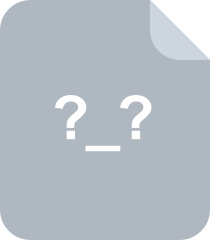
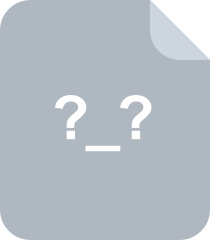
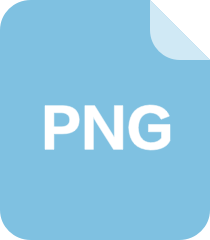
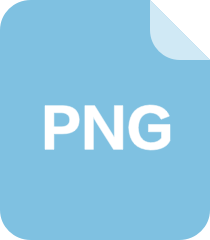
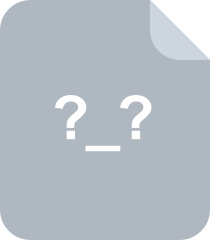
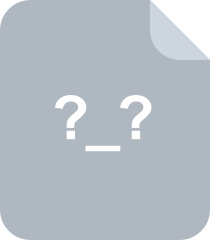
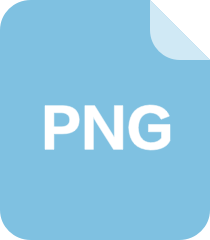
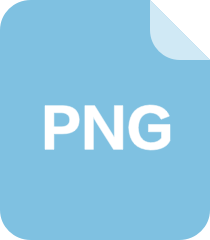
共 29 条
- 1

这是一个神秘的博客
- 粉丝: 529
- 资源: 20
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

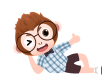
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


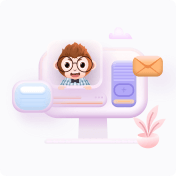
安全验证
文档复制为VIP权益,开通VIP直接复制

- 1
- 2
- 3
- 4
前往页