#include "HelloWorldScene.h"
#include "cloud.h"
#include "Missile.h"
#include "Box.h"
using namespace cocos2d;
//天空的颜色
static const ccColor4B SKY_COLOR = {30, 66, 78, 255};
static HelloWorld* layer;
//导弹精灵
static Missile* MissileSprite;
//天空背景图层
static cocos2d::CCLayerColor* skyLayer;
enum {
//设定一个父节点的tag
kTagParentNode = 1,
};
enum {
Z_PHYSICS_DEBUG = 100,
};
HelloWorld::HelloWorld(){
}
HelloWorld::~HelloWorld()
{
// manually Free rogue shapes
for( int i=0;i<4;i++) {
cpShapeFree( m_pWalls[i] );
}
cpSpaceFree( m_pSpace );
}
//调试模式下可以看到为刚体定义的形状,否则形状会不可见
void HelloWorld::toggleDebugCallback(CCObject* pSender)
{
#if CC_ENABLE_CHIPMUNK_INTEGRATION
m_pDebugLayer->setVisible(! m_pDebugLayer->isVisible());
#endif
}
CCScene* HelloWorld::scene()
{
CCScene * scene = NULL;
do
{
// 'scene' is an autorelease object
scene = CCScene::create();
CC_BREAK_IF(! scene);
// 'layer' is an autorelease object
layer = HelloWorld::create();
CC_BREAK_IF(! layer);
// add layer as a child to scene
scene->addChild(layer,0,1);
} while (0);
// return the scene
return scene;
}
// on "init" you need to initialize your instance
bool HelloWorld::init()
{
bool bRet = false;
do
{
//////////////////////////////////////////////////////////////////////////
// super init first
//////////////////////////////////////////////////////////////////////////
CC_BREAK_IF(! CCLayer::init());
//////////////////////////////////////////////////////////////////////////
// add your codes below...
//////////////////////////////////////////////////////////////////////////
// 1. Add a menu item with "X" image, which is clicked to quit the program.
// Create a "close" menu item with close icon, it's an auto release object.
CCMenuItemImage *pCloseItem = CCMenuItemImage::create(
"CloseNormal.png",
"CloseSelected.png",
this,
menu_selector(HelloWorld::menuCloseCallback));
CC_BREAK_IF(! pCloseItem);
// Place the menu item bottom-right conner.
pCloseItem->setPosition(ccp(CCDirector::sharedDirector()->getWinSize().width - 20, 20));
// Create a menu with the "close" menu item, it's an auto release object.
CCMenu* pMenu = CCMenu::create(pCloseItem, NULL);
pMenu->setPosition(CCPointZero);
CC_BREAK_IF(! pMenu);
// Add the menu to HelloWorld layer as a child layer.
this->addChild(pMenu, 1);
this->setTouchEnabled(true);
// Get window size and place the label upper.
CCSize size = CCDirector::sharedDirector()->getWinSize();
//建立天空颜色图层
CCLayerColor *skyLayer = CCLayerColor::create(SKY_COLOR);
addChild(skyLayer);
// 创建地面精灵.
CCSprite* pSprite = CCSprite::create("Terrain.png");
pSprite->setScale(0.5f);
CC_BREAK_IF(! pSprite);
// Place the sprite on the center of the screen
pSprite->setPosition(ccp(size.width/2, size.height/2));
// Add the sprite to HelloWorld layer as a child layer.
this->addChild(pSprite, 0);
//加入箱子的表情纹理
CCTextureCache::sharedTextureCache()->addImage("BoxUpset.png");
CCTextureCache::sharedTextureCache()->addImage("BoxHappy.png");
initPhysics();
//创建并设置自定义的精灵-愤怒的乌云
cloudSprite=cloud::create("Cloud.png");
cloudSprite->setScale(0.5f);
addChild(cloudSprite);
cloudSprite->setPosition(ccp(240,280));
// menu for debug layer 设定物理引擎运行的模式用的菜单按钮
CCMenuItemFont::setFontSize(24);
CCMenuItemFont *item = CCMenuItemFont::create("Toggle debug", this, menu_selector(HelloWorld::toggleDebugCallback));
CCMenu *menu = CCMenu::create(item, NULL);
this->addChild(menu);
menu->setPosition(ccp(VisibleRect::right().x-100, VisibleRect::top().y-60));
Box* box=Box::create("BoxHappy.png",ccp(110,100),m_pSpace);
box->setScale(0.6f);
addChild(box);
//调用计时器,此类计时器只认update方法,也就是层里定义的那个
scheduleUpdate();
bRet = true;
} while (0);
return bRet;
}
void HelloWorld::ccTouchesBegan(CCSet* touches, CCEvent* event)
{
//Add a new body/atlas sprite at the touched location
//根据触屏位置添加刚体精灵
CCSetIterator it;
CCTouch* touch;
for( it = touches->begin(); it != touches->end(); it++)
{
touch = (CCTouch*)(*it);
if(!touch)
break;
//根据触屏点创建一个导弹
MissileSprite=Missile::create("Missile.png",cloudSprite->getPosition(),cpv(this->getParent()->convertTouchToNodeSpace(touch).x,this->convertTouchToNodeSpace(touch).y),m_pSpace);
MissileSprite->setScale(0.5f);
addChild(MissileSprite);
//更新乌云眼神的角度
cloudSprite->lookAt=touch->getLocation();
}
}
//触摸移动时的回调函数,用于引导导弹
void HelloWorld::ccTouchesMoved(CCSet* touches, CCEvent* event){
CCSetIterator it;
CCTouch* touch;
for( it = touches->begin(); it != touches->end(); it++)
{
touch = (CCTouch*)(*it);
if(!touch)
break;
}
if(MissileSprite){
//开启导弹追踪属性并设置重力矢量点到当前触屏移动到的位置
MissileSprite->isTracking=true;
MissileSprite->target=cpv(this->getParent()->convertTouchToNodeSpace(touch).x,this->convertTouchToNodeSpace(touch).y);
}
//更新乌云眼神的角度
cloudSprite->lookAt=touch->getLocation();
}
//触摸结束时的回调
void HelloWorld::ccTouchesEnded(CCSet* touches, CCEvent* event){
//去除导弹的跟踪属性
if(MissileSprite){
MissileSprite->isTracking=false;
}
}
//物理系统初始化
void HelloWorld::initPhysics()
{
#if CC_ENABLE_CHIPMUNK_INTEGRATION
// init chipmunk
//cpInitChipmunk();
//创建一个物理空间
m_pSpace = cpSpaceNew();
//设定空间的重力点
m_pSpace->gravity = cpv(0, -100);
//
// rogue shapes
// We have to free them manually
//这里创建了四个形状用于创建一个矩形的墙体,其对应的刚体是静态刚体,也就是保持静止不动的刚体
//cpSegmentShapeNew用来建立一个段状形状,其它定义请看源码
// bottom
m_pWalls[0] = cpSegmentShapeNew( m_pSpace->staticBody,
cpv(VisibleRect::leftBottom().x,VisibleRect::leftBottom().y),
cpv(VisibleRect::rightBottom().x, VisibleRect::rightBottom().y), 9.0f);
// top
m_pWalls[1] = cpSegmentShapeNew( m_pSpace->staticBody,
cpv(VisibleRect::leftTop().x, VisibleRect::leftTop().y),
cpv(VisibleRect::rightTop().x, VisibleRect::rightTop().y), 0.0f);
// left
m_pWalls[2] = cpSegmentShapeNew( m_pSpace->staticBody,
cpv(VisibleRect::leftBottom().x,VisibleRect::leftBottom().y),
cpv(VisibleRect::leftTop().x,VisibleRect::leftTop().y), 0.0f);
// right
m_pWalls[3] = cpSegmentShapeNew( m_pSpace->staticBody,
cpv(VisibleRect::rightBottom().x, VisibleRect::rightBottom().y),
cpv(VisibleRect::rightTop().x, VisibleRect::rightTop().y), 0.0f);
//为形状设定弹性及摩擦系数并加入到物理空间
for( int i=0;i<4;i++) {
m_pWalls[i]->e = 1.0f;
m_pWalls[i]->u = 1.0f;
//设定碰撞类型标识
m_pWalls[i]->collision_type=i;
//添加墙体到物理空间
cpSpaceAddStaticShape(m_pSpace, m_pWalls[i] );
//设定碰撞的回调函数,5是导弹的碰撞类型标识
cpSpaceAddCollisionHandler(m_pSpace,5,i,beginCollision,NULL,NULL,NULL,NULL);
}
// Physics debug layer
m_pDebugLayer = CCPhysicsDebugNode::create(m_pSpace);
this->addChild(m_pDebugLayer, Z_PHYSICS_DEBUG);
#endif
}
void HelloWorld::update(float delta)
{
// Should use a fixed size step based on the animation interval.
int steps = 2;
flo
在本实践篇中,我们将深入探讨如何在Cocos2d-x游戏引擎中使用Chipmunk物理库进行简单的碰撞检测。Cocos2d-x是一个广泛使用的2D游戏开发框架,而Chipmunk则是一个轻量级的2D物理引擎,特别适合处理游戏中的碰撞和运动模拟。 `Missile.cpp` 和 `Missile.h` 文件是关于导弹对象的实现和声明。导弹在游戏中通常作为玩家的攻击手段,需要精确的碰撞检测来判断是否击中目标。在这些文件中,你可能看到导弹类的定义,包括其形状、速度和碰撞行为的设置。 Chipmunk提供了`cpShape`对象来表示物理形状,导弹可能被表示为一个`cpCircleShape`或`cpSegmentShape`,取决于其形状。 接着,`Box.cpp` 和 `Box.h` 文件则包含了盒子对象的代码。盒子可能代表游戏场景中的障碍物或者其他可交互的物体。在Chipmunk中,你可以使用`cpBoxShape`来创建矩形形状,这有助于构建各种静态和动态的物理对象。 `cloud.cpp` 和 `cloud.h` 文件可能与"cloudBomb"示例中的云朵对象有关。云朵可能是一个动态物体,它可以通过`cpBody`对象来管理其位置和速度,并通过`cpShape`来定义其碰撞属性。在`cloudBomb`例子中,云朵可能带有炸弹,当导弹与其碰撞时,会触发爆炸效果。 `main.cpp` 是程序的主要入口点,它包含了游戏的初始化、更新循环和渲染逻辑。在这里,你会看到如何设置Chipmunk的物理世界,如何添加物体,以及如何处理碰撞事件。`update`函数会调用Chipmunk的`cpSpaceStep`来模拟物理世界的一帧,并检查发生的碰撞。 `CloudBomb.win32.vcxproj.filters` 是Visual Studio项目的过滤器文件,它帮助组织源代码文件在IDE中的显示。 至于`resource.h` 和 `main.h` ,它们分别包含了资源管理和主程序的全局头文件。`resource.h`可能包含了游戏资源的定义,如图像、声音或字体,而`main.h`则可能包含了所有其他头文件的包含以及游戏的主要类和常量的定义。 通过这些源代码,我们可以学习到如何在Cocos2d-x和Chipmunk的结合中创建物理对象,定义它们的碰撞属性,以及如何响应和处理碰撞事件。这对于开发涉及物理互动的游戏是非常关键的。理解并熟练运用这些概念,将有助于你构建更复杂、更具真实感的游戏场景。














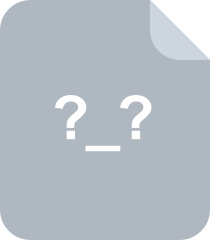
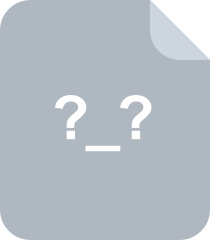

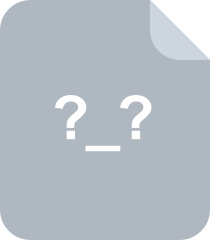
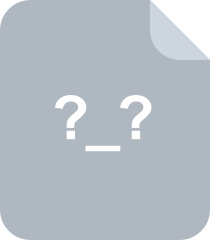
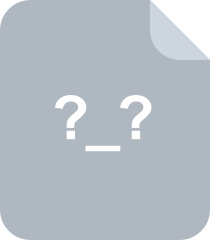
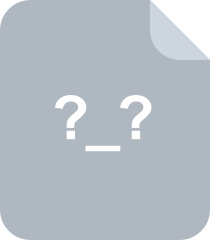
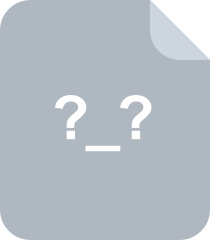

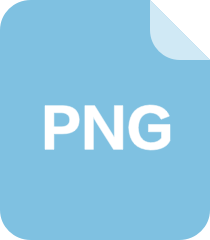
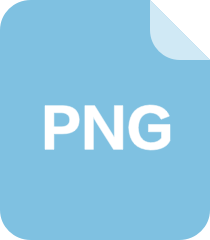
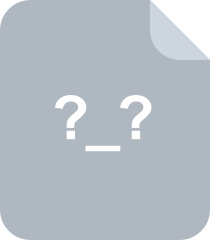
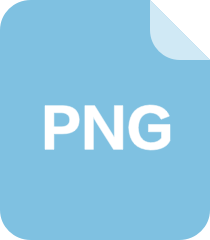
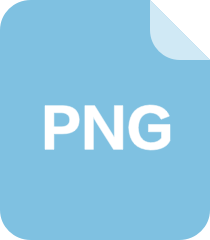
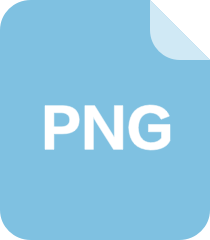
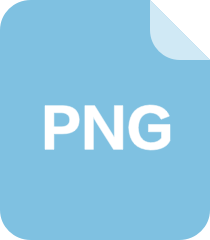
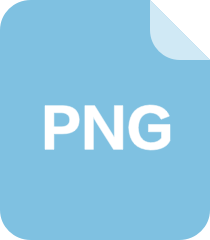
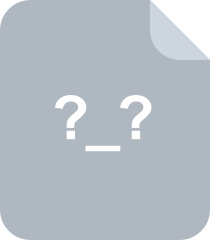
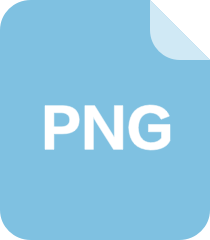
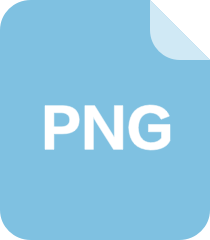
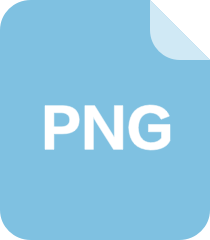
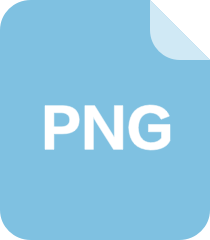
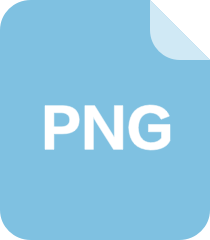
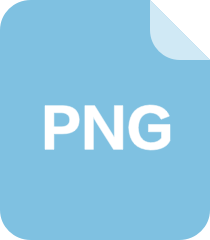
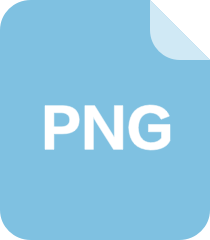
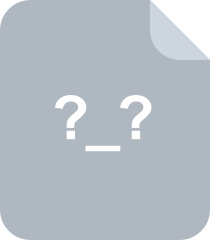
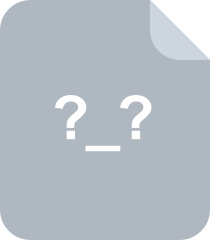
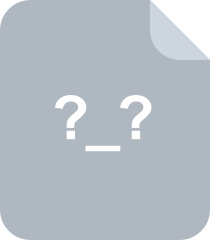
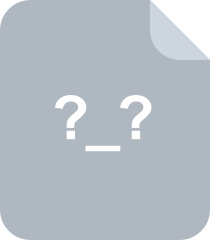
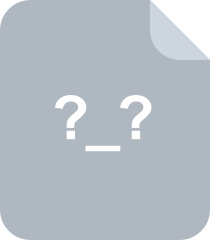
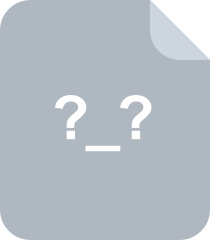

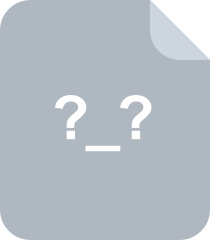
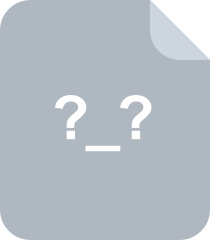
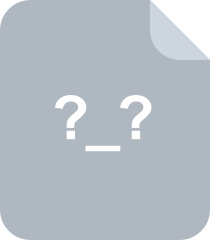
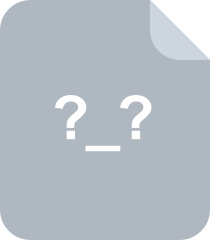
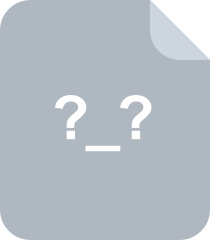
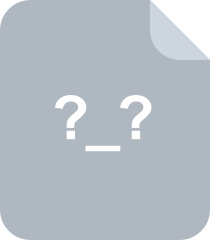
- 1
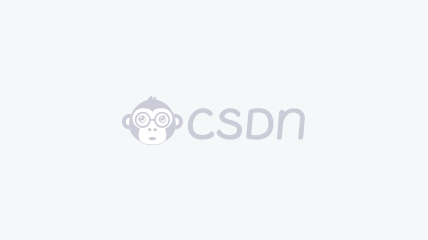
- #完美解决问题
- #运行顺畅
- #内容详尽
- #全网独家
- #注释完整
- baidu_286557312015-07-10还行 可以了解下

- 粉丝: 129
- 资源: 36
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

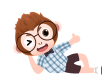
最新资源
- 2024年中国省、市、县驻地点位数据.zip
- 2011-2022年 省级-人均拥有公共图书馆藏数量.zip
- 基于MATLAB语音信号去噪实现(含GUI)
- matlab程序代码项目案例:使用 Simulink 进行自适应 MPC 设计
- 完整性访问控制系统-计算机系统安全实验
- 基于PCA算法的人脸识别MATLAB源码
- 大二计算机系统综合(SoC)实验的一些参考资料
- 基于Python+Pytorch的轴承故障分析(含CNN、SVM、KNN算法)
- 一个计算机系统实验课程-流水线CPU的设计
- 基于SIFT算法的图像拼接MATLAB源码(含GUI)
- 2023年 中国环境统计年鉴.zip
- 基于微信小程序的商场电子优惠券系统设计全套代码+数据库
- 2024年 专精特新中小企业基本信息库.zip
- 2000-2023年 上市公司-气候风险总词频、气候风险指数.zip
- 2000-2022年 上市公司-战略性新兴产业企业面板数据及企业名单.zip
- 2008-2023年 上市公司-企业研发操纵数据.zip

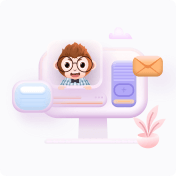
