/***************************************************************************
** **
** QCustomPlot, an easy to use, modern plotting widget for Qt **
** Copyright (C) 2011-2022 Emanuel Eichhammer **
** **
** This program is free software: you can redistribute it and/or modify **
** it under the terms of the GNU General Public License as published by **
** the Free Software Foundation, either version 3 of the License, or **
** (at your option) any later version. **
** **
** This program is distributed in the hope that it will be useful, **
** but WITHOUT ANY WARRANTY; without even the implied warranty of **
** MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the **
** GNU General Public License for more details. **
** **
** You should have received a copy of the GNU General Public License **
** along with this program. If not, see http://www.gnu.org/licenses/. **
** **
****************************************************************************
** Author: Emanuel Eichhammer **
** Website/Contact: https://www.qcustomplot.com/ **
** Date: 06.11.22 **
** Version: 2.1.1 **
****************************************************************************/
#include "qcustomplot.h"
/* including file 'src/vector2d.cpp' */
/* modified 2022-11-06T12:45:56, size 7973 */
////////////////////////////////////////////////////////////////////////////////////////////////////
//////////////////// QCPVector2D
////////////////////////////////////////////////////////////////////////////////////////////////////
/*! \class QCPVector2D
\brief Represents two doubles as a mathematical 2D vector
This class acts as a replacement for QVector2D with the advantage of double precision instead of
single, and some convenience methods tailored for the QCustomPlot library.
*/
/* start documentation of inline functions */
/*! \fn void QCPVector2D::setX(double x)
Sets the x coordinate of this vector to \a x.
\see setY
*/
/*! \fn void QCPVector2D::setY(double y)
Sets the y coordinate of this vector to \a y.
\see setX
*/
/*! \fn double QCPVector2D::length() const
Returns the length of this vector.
\see lengthSquared
*/
/*! \fn double QCPVector2D::lengthSquared() const
Returns the squared length of this vector. In some situations, e.g. when just trying to find the
shortest vector of a group, this is faster than calculating \ref length, because it avoids
calculation of a square root.
\see length
*/
/*! \fn double QCPVector2D::angle() const
Returns the angle of the vector in radians. The angle is measured between the positive x line and
the vector, counter-clockwise in a mathematical coordinate system (y axis upwards positive). In
screen/widget coordinates where the y axis is inverted, the angle appears clockwise.
*/
/*! \fn QPoint QCPVector2D::toPoint() const
Returns a QPoint which has the x and y coordinates of this vector, truncating any floating point
information.
\see toPointF
*/
/*! \fn QPointF QCPVector2D::toPointF() const
Returns a QPointF which has the x and y coordinates of this vector.
\see toPoint
*/
/*! \fn bool QCPVector2D::isNull() const
Returns whether this vector is null. A vector is null if \c qIsNull returns true for both x and y
coordinates, i.e. if both are binary equal to 0.
*/
/*! \fn QCPVector2D QCPVector2D::perpendicular() const
Returns a vector perpendicular to this vector, with the same length.
*/
/*! \fn double QCPVector2D::dot() const
Returns the dot/scalar product of this vector with the specified vector \a vec.
*/
/* end documentation of inline functions */
/*!
Creates a QCPVector2D object and initializes the x and y coordinates to 0.
*/
QCPVector2D::QCPVector2D() :
mX(0),
mY(0)
{
}
/*!
Creates a QCPVector2D object and initializes the \a x and \a y coordinates with the specified
values.
*/
QCPVector2D::QCPVector2D(double x, double y) :
mX(x),
mY(y)
{
}
/*!
Creates a QCPVector2D object and initializes the x and y coordinates respective coordinates of
the specified \a point.
*/
QCPVector2D::QCPVector2D(const QPoint &point) :
mX(point.x()),
mY(point.y())
{
}
/*!
Creates a QCPVector2D object and initializes the x and y coordinates respective coordinates of
the specified \a point.
*/
QCPVector2D::QCPVector2D(const QPointF &point) :
mX(point.x()),
mY(point.y())
{
}
/*!
Normalizes this vector. After this operation, the length of the vector is equal to 1.
If the vector has both entries set to zero, this method does nothing.
\see normalized, length, lengthSquared
*/
void QCPVector2D::normalize()
{
if (mX == 0.0 && mY == 0.0) return;
const double lenInv = 1.0/length();
mX *= lenInv;
mY *= lenInv;
}
/*!
Returns a normalized version of this vector. The length of the returned vector is equal to 1.
If the vector has both entries set to zero, this method returns the vector unmodified.
\see normalize, length, lengthSquared
*/
QCPVector2D QCPVector2D::normalized() const
{
if (mX == 0.0 && mY == 0.0) return *this;
const double lenInv = 1.0/length();
return QCPVector2D(mX*lenInv, mY*lenInv);
}
/*! \overload
Returns the squared shortest distance of this vector (interpreted as a point) to the finite line
segment given by \a start and \a end.
\see distanceToStraightLine
*/
double QCPVector2D::distanceSquaredToLine(const QCPVector2D &start, const QCPVector2D &end) const
{
const QCPVector2D v(end-start);
const double vLengthSqr = v.lengthSquared();
if (!qFuzzyIsNull(vLengthSqr))
{
const double mu = v.dot(*this-start)/vLengthSqr;
if (mu < 0)
return (*this-start).lengthSquared();
else if (mu > 1)
return (*this-end).lengthSquared();
else
return ((start + mu*v)-*this).lengthSquared();
} else
return (*this-start).lengthSquared();
}
/*! \overload
Returns the squared shortest distance of this vector (interpreted as a point) to the finite line
segment given by \a line.
\see distanceToStraightLine
*/
double QCPVector2D::distanceSquaredToLine(const QLineF &line) const
{
return distanceSquaredToLine(QCPVector2D(line.p1()), QCPVector2D(line.p2()));
}
/*!
Returns the shortest distance of this vector (interpreted as a point) to the infinite straight
line given by a \a base point and a \a direction vector.
\see distanceSquaredToLine
*/
double QCPVector2D::distanceToStraightLine(const QCPVector2D &base, const QCPVector2D &direction) const
{
return qAbs((*this-base).dot(direction.perpendicular()))/direction.length();
}
/*!
Scales this vector by the given \a factor, i.e. the x and y components are multiplied by \a
factor.
*/
QCPVector2D &QCPVector2D::operator*=(double factor)
{
mX *= factor;
mY *= factor;
return *this;
}
/*!
Scales this vector by the given \a divisor, i.e. the x and y components are divided by \a
divisor.
*/
QCPVector2D &QCPVector2D::operator/=(double divisor)
{
mX /= divisor;
mY /= divisor;
return *this;
}
/*!
Adds the given \a vector to this vector component-wise.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
本项目为DAQ122 IPC SDK,是一款多语言兼容的设计源码,支持C++、C、C#三种编程语言。该套件包含506个文件,其中包含408个头文件(.h)、32个PNG图片文件、12个Markdown文件、10个VI编辑器文件、7个C#源文件(.cs)、6个C++源文件(.cpp)、3个动态链接库文件(.dll)以及其他类型的文件。该SDK旨在为用户提供灵活的工业数据采集解决方案。
资源推荐
资源详情
资源评论
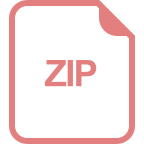
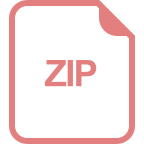
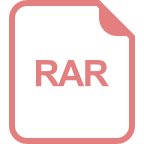
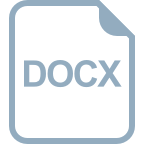
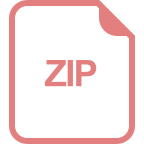
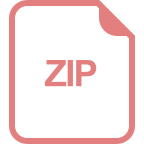
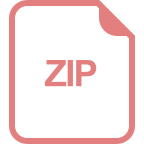
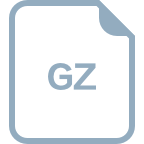
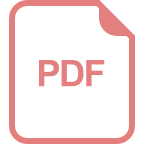
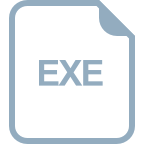
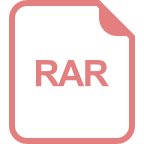
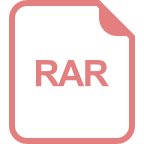
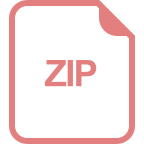
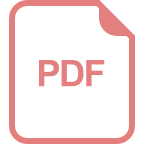
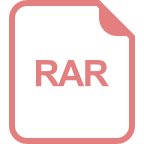
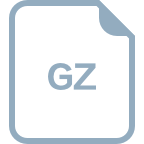
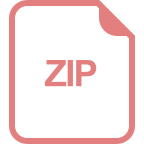
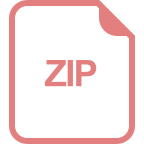
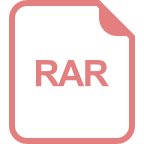
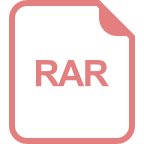
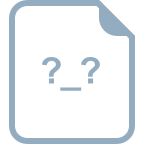
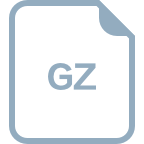
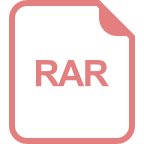
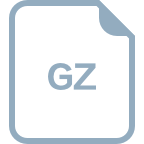
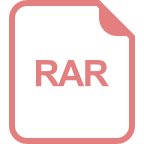
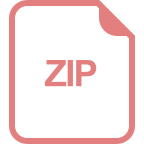
收起资源包目录

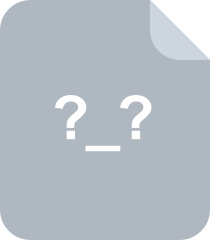
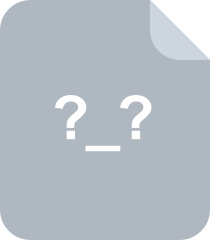
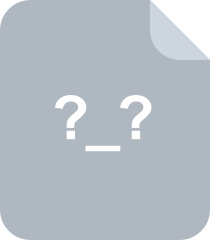
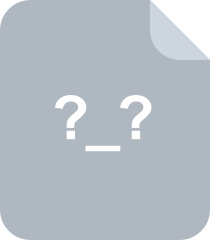
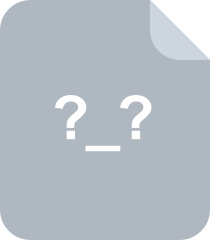
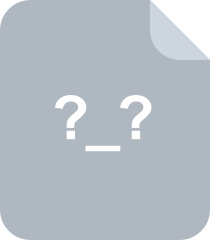
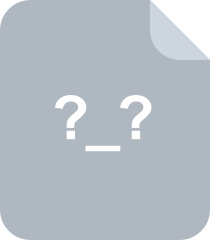
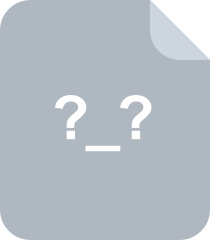
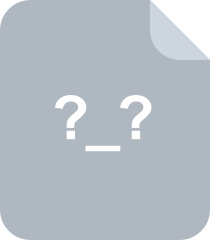
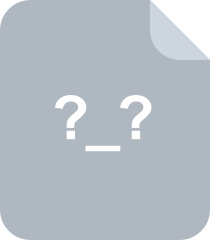
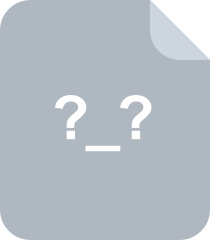
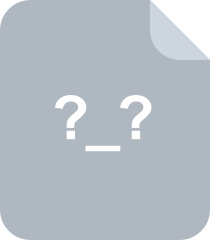
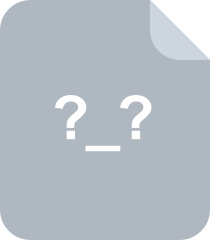
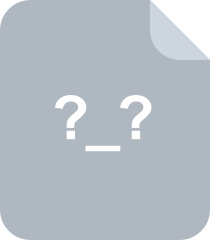
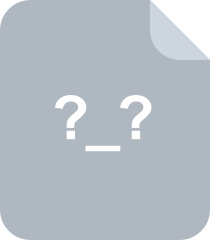
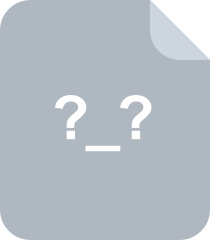
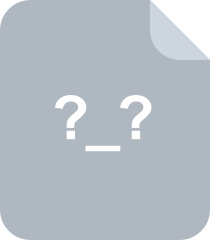
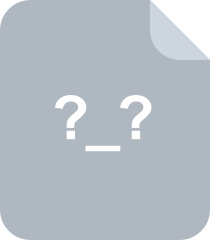
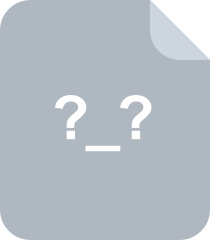
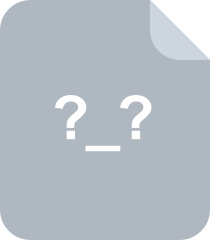
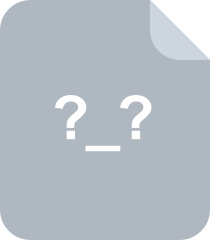
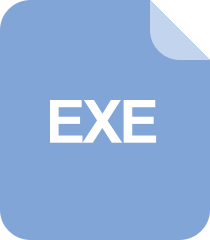
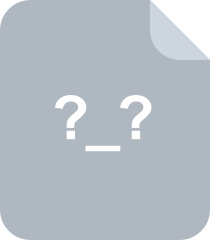
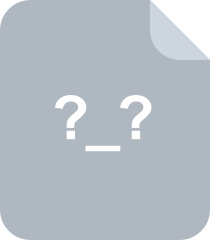
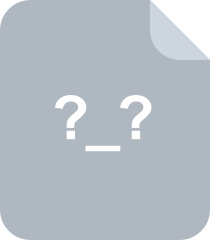
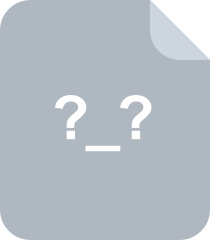
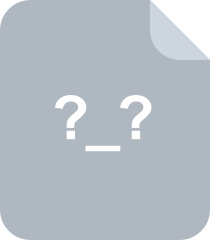
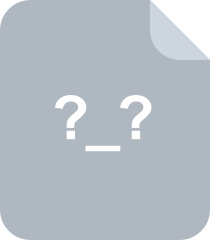
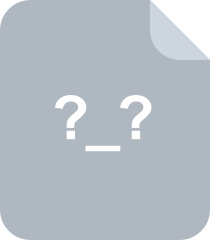
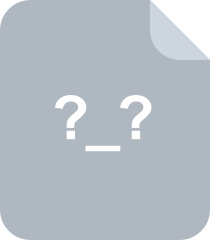
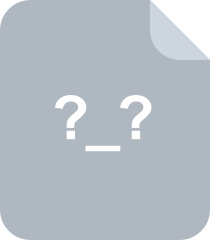
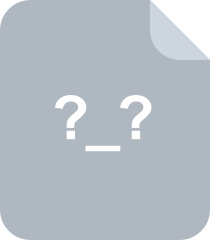
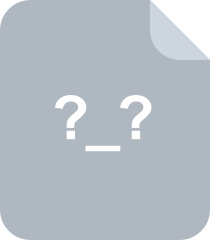
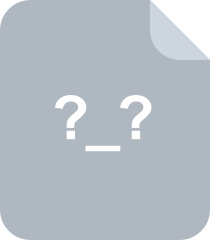
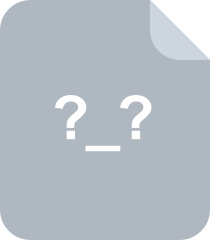
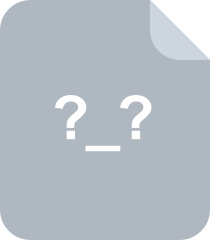
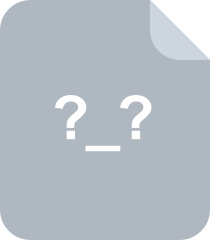
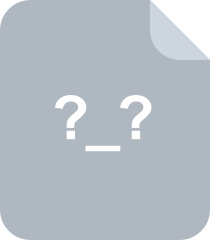
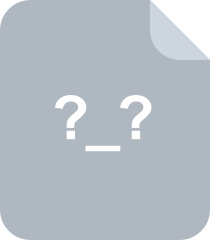
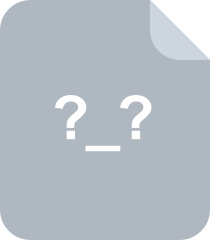
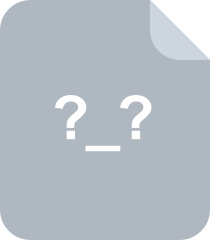
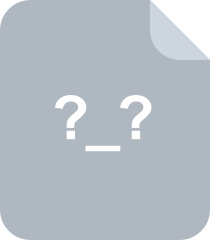
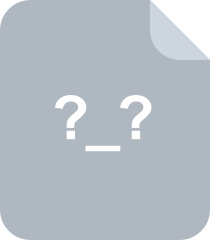
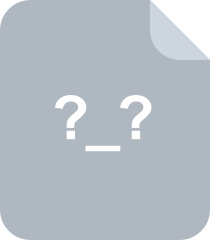
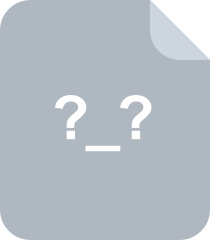
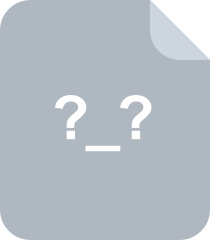
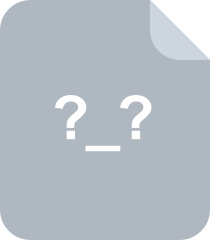
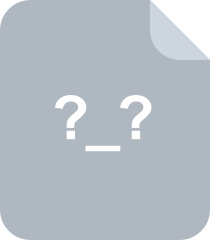
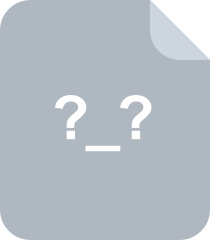
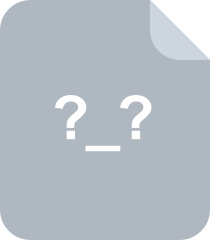
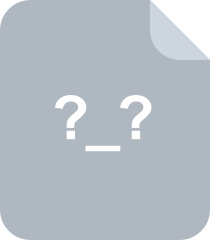
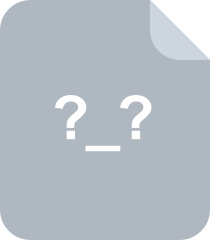
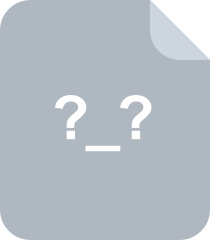
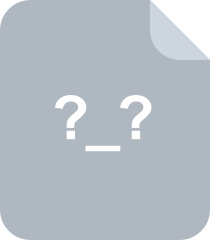
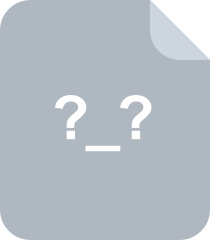
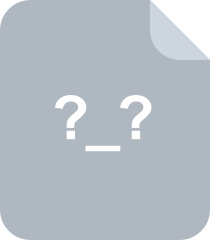
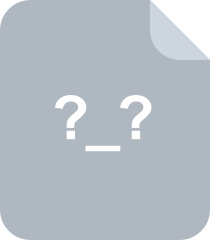
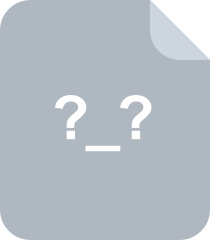
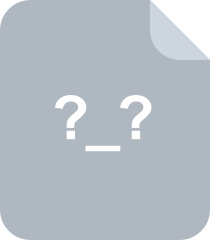
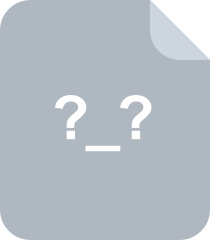
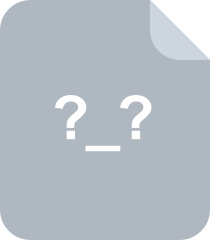
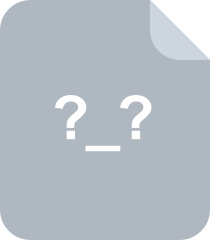
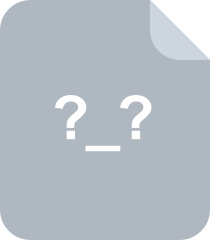
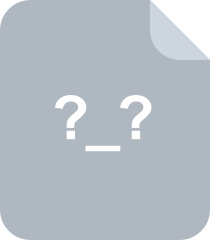
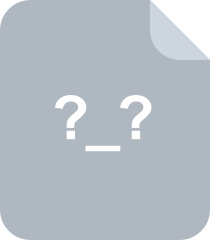
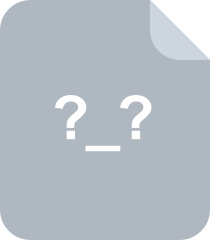
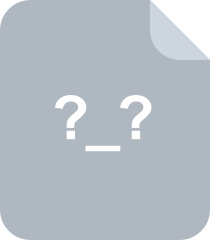
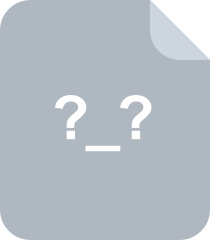
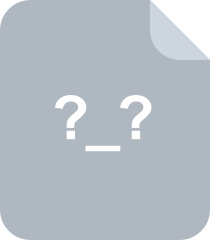
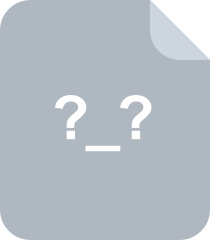
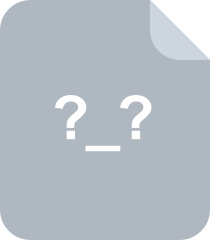
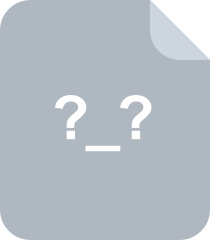
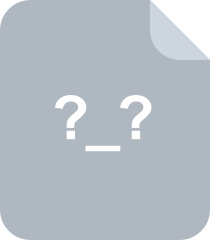
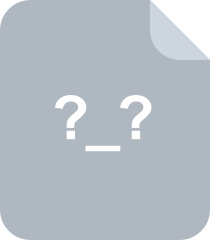
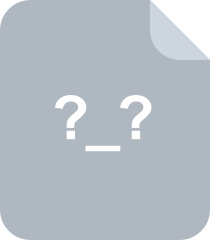
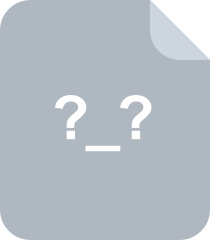
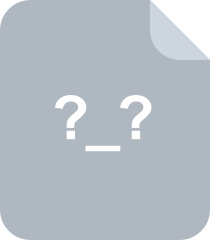
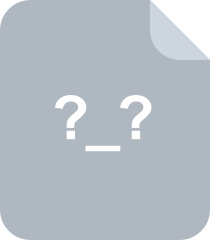
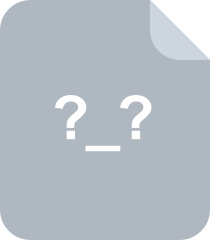
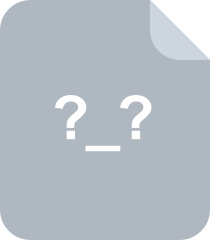
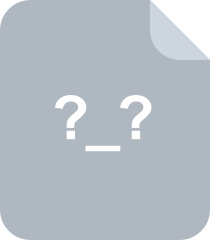
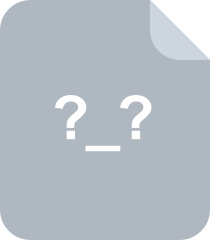
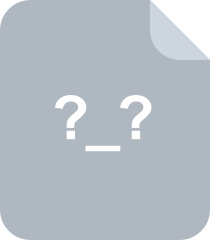
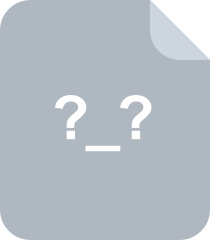
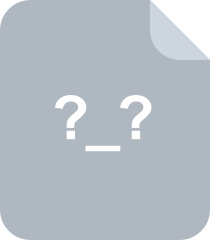
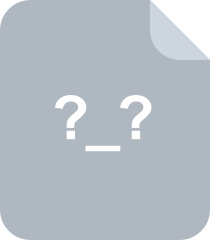
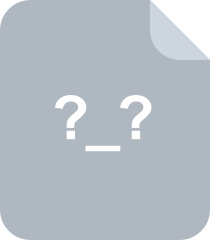
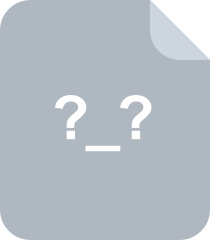
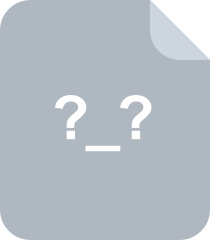
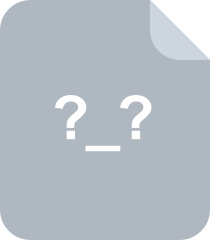
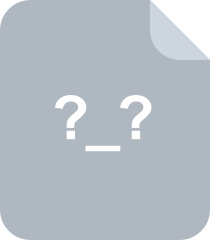
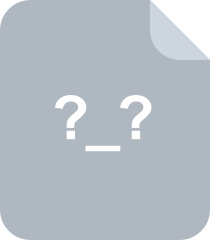
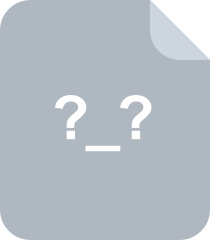
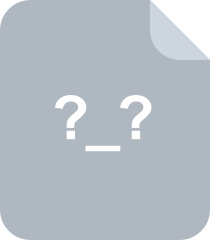
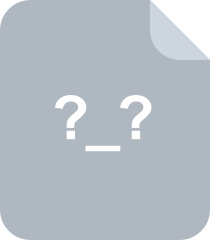
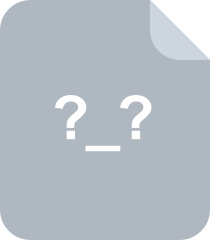
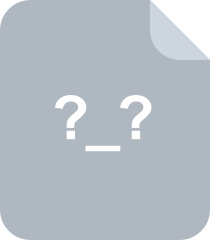
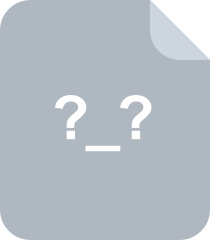
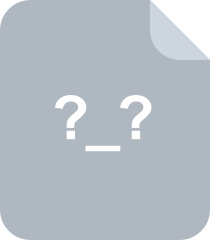
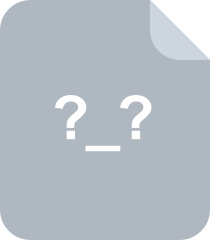
共 498 条
- 1
- 2
- 3
- 4
- 5
资源评论
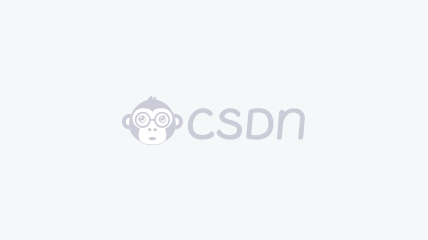

csbysj2020
- 粉丝: 2456
- 资源: 5457
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

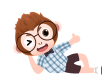
最新资源
- 快手APP大学生用户数据集【数据格式已处理】.zip
- 《编译原理》课件-第4章文法和语言
- 【java毕业设计】校园博客系统源码(springboot+vue+mysql+说明文档+LW).zip
- 【java毕业设计】springbootjava付费自习室管理系统(springboot+vue+mysql+说明文档).zip
- Shell脚本中变量与字符串操作的实战指南
- 【java毕业设计】springbootjava在线考试系统(springboot+vue+mysql+说明文档).zip
- grendel-gs(3D gs gpus)
- 【java毕业设计】校友社交系统源码(springboot+vue+mysql+说明文档+LW+LW).zip
- 打造完美圣诞装饰球:使用 CSS `border-radius` 创建圆形
- 大数据笔记自己记录用的
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


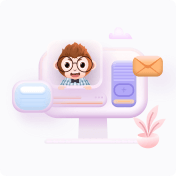
安全验证
文档复制为VIP权益,开通VIP直接复制
