#include "matlabsrvr.h"
typedef interface tagMLApp_IMLApp_Interface MLApp_IMLApp_Interface;
typedef struct tagMLApp_IMLApp_VTable
{
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *QueryInterface )( MLApp_IMLApp_Interface __RPC_FAR * This,
REFIID riid,
void __RPC_FAR *__RPC_FAR *ppvObject);
ULONG ( STDMETHODCALLTYPE __RPC_FAR *AddRef )( MLApp_IMLApp_Interface __RPC_FAR * This);
ULONG ( STDMETHODCALLTYPE __RPC_FAR *Release )( MLApp_IMLApp_Interface __RPC_FAR * This);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *GetFullMatrix_) (MLApp_IMLApp_Interface __RPC_FAR *This,
BSTR name,
BSTR workspace,
SAFEARRAY **pr,
SAFEARRAY **pi);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *PutFullMatrix_) (MLApp_IMLApp_Interface __RPC_FAR *This,
BSTR name,
BSTR workspace,
SAFEARRAY *pr,
SAFEARRAY *pi);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *Execute_) (MLApp_IMLApp_Interface __RPC_FAR *This,
BSTR name);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *MinimizeCommandWindow_) (MLApp_IMLApp_Interface __RPC_FAR *This);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *MaximizeCommandWindow_) (MLApp_IMLApp_Interface __RPC_FAR *This);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *Quit_) (MLApp_IMLApp_Interface __RPC_FAR *This);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *GetCharArray_) (MLApp_IMLApp_Interface __RPC_FAR *This,
BSTR name,
BSTR workspace,
BSTR *mlString);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *PutCharArray_) (MLApp_IMLApp_Interface __RPC_FAR *This,
BSTR name,
BSTR workspace,
BSTR charArray);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *GetVisible_) (MLApp_IMLApp_Interface __RPC_FAR *This,
long *pVal);
HRESULT ( STDMETHODCALLTYPE __RPC_FAR *SetVisible_) (MLApp_IMLApp_Interface __RPC_FAR *This,
long pVal);
} MLApp_IMLApp_VTable;
typedef interface tagMLApp_IMLApp_Interface
{
CONST_VTBL MLApp_IMLApp_VTable __RPC_FAR *lpVtbl;
} MLApp_IMLApp_Interface;
/*const IID IID_IDispatch =
{
0x669CEC93, 0x6E22, 0x11CF, 0xA4, 0xD6, 0x0, 0xA0, 0x24, 0x58, 0x3C, 0x19
};
const IID MLApp_IID_IMLApp =
{
0x669CEC92, 0x6E22, 0x11CF, 0xA4, 0xD6, 0x0, 0xA0, 0x24, 0x58, 0x3C, 0x19
}; */
const IID MLApp_IID_DIMLEval;
const IID MLApp_IID_IMLApp;
HRESULT CVIFUNC MLApp_NewDIMLApp (const char *server, int supportMultithreading,
LCID locale, int reserved,
CAObjHandle *objectHandle)
{
HRESULT __result = S_OK;
GUID clsid = {0x554F6053, 0x79D4, 0x11D4, 0xB0, 0x67, 0x0, 0x90, 0x27,
0xBA, 0x5F, 0x81};
__result = CA_CreateObjectByClassIdEx (&clsid, server, &IID_IDispatch,
supportMultithreading, locale,
reserved, objectHandle);
return __result;
}
HRESULT CVIFUNC MLApp_OpenDIMLApp (const char *fileName, const char *server,
int supportMultithreading, LCID locale,
int reserved, CAObjHandle *objectHandle)
{
HRESULT __result = S_OK;
GUID clsid = {0x554F6053, 0x79D4, 0x11D4, 0xB0, 0x67, 0x0, 0x90, 0x27,
0xBA, 0x5F, 0x81};
__result = CA_LoadObjectFromFileByClassIdEx (fileName, &clsid, server,
&IID_IDispatch,
supportMultithreading, locale,
reserved, objectHandle);
return __result;
}
HRESULT CVIFUNC MLApp_ActiveDIMLApp (const char *server,
int supportMultithreading, LCID locale,
int reserved, CAObjHandle *objectHandle)
{
HRESULT __result = S_OK;
GUID clsid = {0x554F6053, 0x79D4, 0x11D4, 0xB0, 0x67, 0x0, 0x90, 0x27,
0xBA, 0x5F, 0x81};
__result = CA_GetActiveObjectByClassIdEx (&clsid, server,
&IID_IDispatch,
supportMultithreading, locale,
reserved, objectHandle);
return __result;
}
HRESULT CVIFUNC MLApp_DIMLAppGetFullMatrix (CAObjHandle objectHandle,
ERRORINFO *errorInfo,
const char *name,
const char *workspace,
SAFEARRAY **pr, SAFEARRAY **pi)
{
HRESULT __result = S_OK;
unsigned int __paramTypes[] = {CAVT_CSTRING, CAVT_CSTRING,
CAVT_DOUBLE | CAVT_ARRAY | CAVT_BYREFIO,
CAVT_DOUBLE | CAVT_ARRAY | CAVT_BYREFIO};
__result = CA_MethodInvokeEx (objectHandle, errorInfo, &IID_IDispatch,
0x60010000, CAVT_EMPTY, NULL, 4,
__paramTypes, name, workspace, pr, pi);
return __result;
}
HRESULT CVIFUNC MLApp_DIMLAppPutFullMatrix (CAObjHandle objectHandle,
ERRORINFO *errorInfo,
const char *name,
const char *workspace, SAFEARRAY *pr,
SAFEARRAY *pi)
{
HRESULT __result = S_OK;
unsigned int __paramTypes[] = {CAVT_CSTRING, CAVT_CSTRING,
CAVT_DOUBLE | CAVT_ARRAY,
CAVT_DOUBLE | CAVT_ARRAY};
__result = CA_MethodInvokeEx (objectHandle, errorInfo, &IID_IDispatch,
0x60010001, CAVT_EMPTY, NULL, 4,
__paramTypes, name, workspace, pr, pi);
return __result;
}
HRESULT CVIFUNC MLApp_DIMLAppExecute (CAObjHandle objectHandle,
ERRORINFO *errorInfo, const char *name,
char **returnValue)
{
HRESULT __result = S_OK;
unsigned int __paramTypes[] = {CAVT_CSTRING};
__result = CA_MethodInvokeEx (objectHandle, errorInfo, &IID_IDispatch,
0x60010002, CAVT_CSTRING, returnValue, 1,
__paramTypes, name);
return __result;
}
HRESULT CVIFUNC MLApp_DIMLAppMinimizeCommandWindow (CAObjHandle objectHandle,
ERRORINFO *errorInfo)
{
HRESULT __result = S_OK;
__result = CA_MethodInvokeEx (objectHandle, errorInfo, &IID_IDispatch,
0x60010003, CAVT_EMPTY, NULL, 0, NULL);
return __result;
}
HRESULT CVIFUNC MLApp_DIMLAppMaximizeCommandWindow (CAObjHandle objectHandle,
ERRORINFO *errorInfo)
{
HRESULT __result = S_OK;
__result = CA_MethodInvokeEx (objectHandle, errorInfo, &IID_IDispatch,
0x60010004, CAVT_EMPTY, NULL, 0, NULL)

honglei19880829
- 粉丝: 0
- 资源: 1
最新资源
- 自制数据库迁移工具-C版-06-HappySunshineV1.5-(支持南大Gbase8a、PostgreSQL、达梦DM)
- 车载以太网IEEE 802 规范
- 基于java+springboot+mysql+微信小程序的开放实验室预约管理系统 源码+数据库+论文(高分毕业设计).zip
- 手机外观尺寸检测设备工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- 基于java+springboot+mysql+微信小程序的流浪动物救助系统 源码+数据库+论文(高分毕业设计).zip
- 基于java+springboot+mysql+微信小程序的企业内部员工管理系统 源码+数据库+论文(高分毕业设计).zip
- 基于java+springboot+mysql+微信小程序的社区志愿者服务平台 源码+数据库+论文(高分毕业设计).zip
- 基于java+springboot+mysql+微信小程序的社区物业信息管理系统 源码+数据库+论文(高分毕业设计).zip
- 基于java+springboot+mysql+微信小程序的上门维修系统 源码+数据库+论文(高分毕业设计).zip
- 深度卷积神经网络在MNIST数据集上的应用
- 基于转子磁链模型的改进滑模观测器 1.对滑模观测器进行改进,采用与转速相关的自适应反馈增益,避免恒定增益导致的低速下抖振明显的问题; 2.区别传统滑模从反电势中提取位置和转速信息,改进滑模观测器中利用
- 汇编语言教程、案例与相关项目资源汇总
- 双工位手机外壳抛光机工程图机械结构设计图纸和其它技术资料和技术方案非常好100%好用.zip
- docker-ubuntu24.10-tesseract5.5.0
- 基于微信的高校教务管理系统设计与实现springboot.zip
- 中国剪纸微信小程序的设计与实现ssm.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


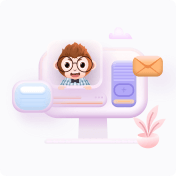