#!/usr/bin/env python
# -*- coding: utf-8 -*-
'''
A simple, lightweight, WSGI-compatible web framework.
'''
__author__ = 'Michael Liao'
import __builtin__
import types, sys, os, re, cgi, sys, base64, json, time, hashlib, inspect, datetime, functools, mimetypes, threading, logging, urllib, collections, linecache
# thread local object for storing request and response.
ctx = threading.local()
class Dict(dict):
'''
Simple dict but support access as x.y style.
>>> d1 = Dict()
>>> d1['x'] = 100
>>> d1.x
100
>>> d1.y = 200
>>> d1['y']
200
>>> d2 = Dict(a=1, b=2, c='3')
>>> d2.c
'3'
>>> d2['empty']
Traceback (most recent call last):
...
KeyError: 'empty'
>>> d2.empty
Traceback (most recent call last):
...
KeyError: 'empty'
'''
def __getattr__(self, key):
return self[key]
def __setattr__(self, key, value):
self[key] = value
def _json_loads(s, expected=None):
'''
Loads json.
>>> r = _json_loads(r'{"test": "ok", "users": [{"name": "Michael"}, {"name": "Tracy"}]}')
>>> r.test
u'ok'
>>> r.users[0].name
u'Michael'
>>> r.users[1].name
u'Tracy'
>>> r = _json_loads(r'{"test": "ok"}', expected=(list, tuple))
Traceback (most recent call last):
...
TypeError: Object loaded from json is not expected type: (<type 'list'>, <type 'tuple'>)
'''
r = json.loads(s, object_pairs_hook=Dict)
if expected:
if not isinstance(r, expected):
raise TypeError('Object loaded from json is not expected type: %s' % str(expected))
return r
def _json_dumps(obj):
'''
Dumps any object as json string.
>>> class Person(object):
... def __init__(self, name):
... self.name = name
>>> _json_dumps([Person('Bob'), None])
'[{"name": "Bob"}, null]'
'''
def _dump_obj(obj):
if isinstance(obj, dict):
return obj
d = dict()
for k in dir(obj):
if not k.startswith('_'):
d[k] = getattr(obj, k)
return d
return json.dumps(obj, default=_dump_obj)
# all known response statues:
_RESPONSE_STATUSES = {
# Informational
100: 'Continue',
101: 'Switching Protocols',
102: 'Processing',
# Successful
200: 'OK',
201: 'Created',
202: 'Accepted',
203: 'Non-Authoritative Information',
204: 'No Content',
205: 'Reset Content',
206: 'Partial Content',
207: 'Multi Status',
226: 'IM Used',
# Redirection
300: 'Multiple Choices',
301: 'Moved Permanently',
302: 'Found',
303: 'See Other',
304: 'Not Modified',
305: 'Use Proxy',
307: 'Temporary Redirect',
# Client Error
400: 'Bad Request',
401: 'Unauthorized',
402: 'Payment Required',
403: 'Forbidden',
404: 'Not Found',
405: 'Method Not Allowed',
406: 'Not Acceptable',
407: 'Proxy Authentication Required',
408: 'Request Timeout',
409: 'Conflict',
410: 'Gone',
411: 'Length Required',
412: 'Precondition Failed',
413: 'Request Entity Too Large',
414: 'Request URI Too Long',
415: 'Unsupported Media Type',
416: 'Requested Range Not Satisfiable',
417: 'Expectation Failed',
418: "I'm a teapot",
422: 'Unprocessable Entity',
423: 'Locked',
424: 'Failed Dependency',
426: 'Upgrade Required',
# Server Error
500: 'Internal Server Error',
501: 'Not Implemented',
502: 'Bad Gateway',
503: 'Service Unavailable',
504: 'Gateway Timeout',
505: 'HTTP Version Not Supported',
507: 'Insufficient Storage',
510: 'Not Extended',
}
_RE_RESPONSE_STATUS = re.compile(r'^\d\d\d(\ [\w\ ]+)?$')
_RESPONSE_HEADERS = (
'Accept-Ranges',
'Age',
'Allow',
'Cache-Control',
'Connection',
'Content-Encoding',
'Content-Language',
'Content-Length',
'Content-Location',
'Content-MD5',
'Content-Disposition',
'Content-Range',
'Content-Type',
'Date',
'ETag',
'Expires',
'Last-Modified',
'Link',
'Location',
'P3P',
'Pragma',
'Proxy-Authenticate',
'Refresh',
'Retry-After',
'Server',
'Set-Cookie',
'Strict-Transport-Security',
'Trailer',
'Transfer-Encoding',
'Vary',
'Via',
'Warning',
'WWW-Authenticate',
'X-Frame-Options',
'X-XSS-Protection',
'X-Content-Type-Options',
'X-Forwarded-Proto',
'X-Powered-By',
'X-UA-Compatible',
)
_RESPONSE_HEADER_DICT = {}
for hdr in _RESPONSE_HEADERS:
_RESPONSE_HEADER_DICT[hdr.upper()] = hdr
_HEADER_X_POWERED_BY = ('X-Powered-By', 'iTranswarp/1.0')
class HttpError(StandardError):
'''
HttpError that defines http error code.
>>> e = HttpError(404)
>>> e.status
'404 Not Found'
'''
def __init__(self, code):
'''
Init an HttpError with response code.
'''
super(HttpError, self).__init__()
self.status = '%d %s' % (code, _RESPONSE_STATUSES[code])
def header(self, name, value):
if not hasattr(self, '_headers'):
self._headers = [_HEADER_X_POWERED_BY]
self._headers.append((name, value))
@property
def headers(self):
if hasattr(self, '_headers'):
return self._headers
return []
def __str__(self):
return self.status
class RedirectError(StandardError):
'''
RedirectError that defines http redirect code.
>>> e = RedirectError(302, 'http://www.apple.com/')
>>> e.status
'302 Found'
>>> e.location
'http://www.apple.com/'
'''
def __init__(self, code, location):
'''
Init an HttpError with response code.
'''
super(RedirectError, self).__init__()
self.status = '%d %s' % (code, _RESPONSE_STATUSES[code])
self.location = location
def __str__(self):
return self.status
class JsonRpcError(StandardError):
pass
def badrequest():
'''
Send a bad request response.
>>> raise badrequest()
Traceback (most recent call last):
...
HttpError: 400 Bad Request
'''
return HttpError(400)
def unauthorized():
'''
Send an unauthorized response.
>>> raise unauthorized()
Traceback (most recent call last):
...
HttpError: 401 Unauthorized
'''
return HttpError(401)
def forbidden():
'''
Send a forbidden response.
>>> raise forbidden()
Traceback (most recent call last):
...
HttpError: 403 Forbidden
'''
return HttpError(403)
def notfound():
'''
Send a not found response.
>>> raise notfound()
Traceback (most recent call last):
...
HttpError: 404 Not Found
'''
return HttpError(404)
def conflict():
'''
Send a conflict response.
>>> raise conflict()
Traceback (most recent call last):
...
HttpError: 409 Conflict
'''
return HttpError(409)
def internalerror():
'''
Send an internal error response.
>>> raise internalerror()
Traceback (most recent call last):
...
HttpError: 500 Internal Server Error
'''
return HttpError(500)
def _redirect(code, location):
return RedirectError(code, location)
def redirect(location):
'''
Do permanent redirect.
>>> raise redirect('http://www.itranswarp.com/')
Traceback (most recent call last):
...
RedirectError: 301 Moved Permanently
'''
return _redirect(301, location)
def found(location):
'''
Do temporary redirect.
>>> raise found('http://www.itranswarp.com/')
Traceback (most recent call last):
...
RedirectError: 302 Found
'''
return _redirect(302, location)
def seeother(location):
'''
Do temporary redirect.
>>> raise seeother('http://www.itranswarp.com/')
Traceback (most recent call last):
...
RedirectError: 303 See Other
>>> e = seeother('http://www.itranswarp.com/s
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
使用Python开发的基于新浪微博API的迷你微博网站+源码,适合毕业设计、课程设计、项目开发。项目源码已经过严格测试,可以放心参考并在此基础上延申使用~ 使用Python开发的基于新浪微博API的迷你微博网站+源码,适合毕业设计、课程设计、项目开发。项目源码已经过严格测试,可以放心参考并在此基础上延申使用~ 使用Python开发的基于新浪微博API的迷你微博网站+源码,适合毕业设计、课程设计、项目开发。项目源码已经过严格测试,可以放心参考并在此基础上延申使用~ 使用Python开发的基于新浪微博API的迷你微博网站+源码,适合毕业设计、课程设计、项目开发。项目源码已经过严格测试,可以放心参考并在此基础上延申使用~ 使用Python开发的基于新浪微博API的迷你微博网站+源码,适合毕业设计、课程设计、项目开发。项目源码已经过严格测试,可以放心参考并在此基础上延申使用~
资源推荐
资源详情
资源评论
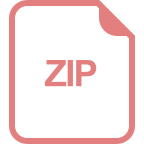
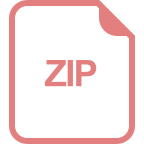
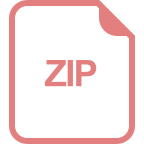
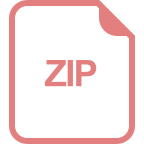
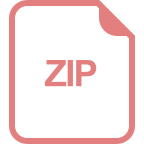
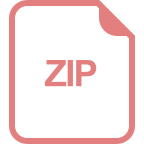
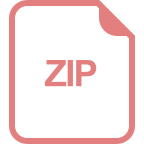
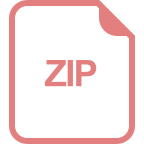
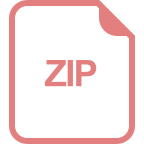
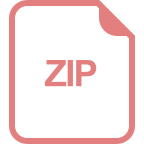
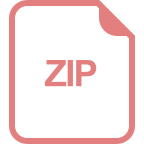
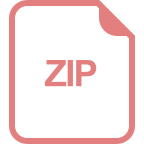
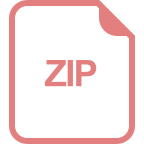
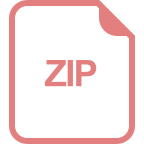
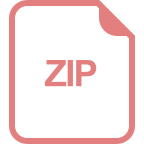
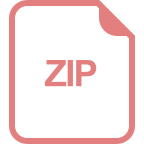
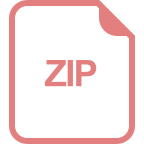
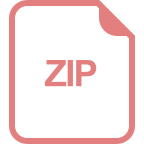
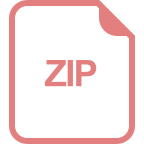
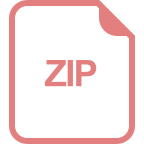
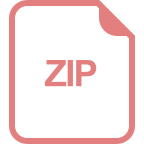
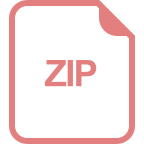
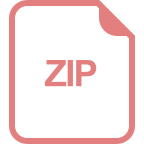
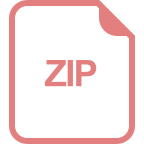
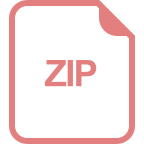
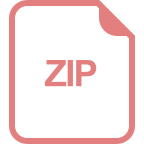
收起资源包目录



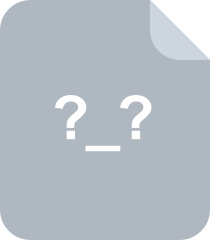
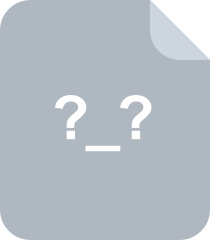
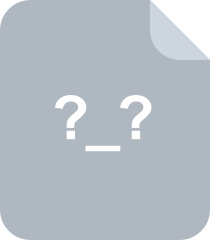
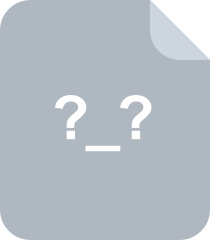
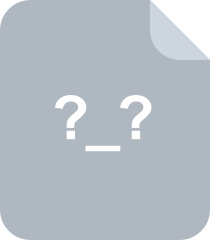
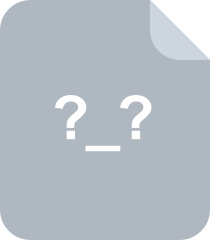
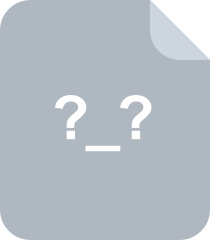
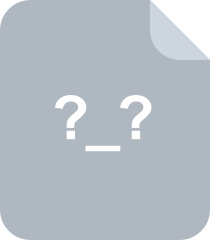
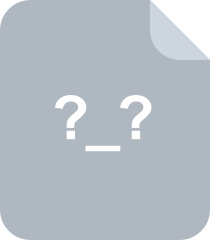
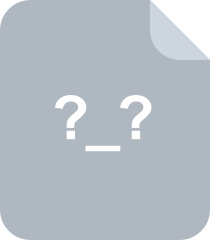
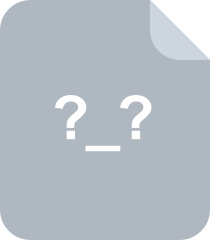
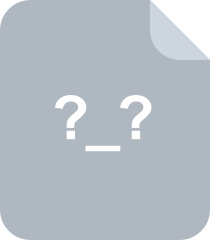
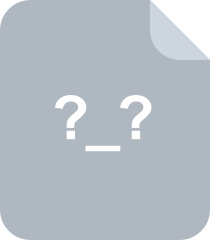
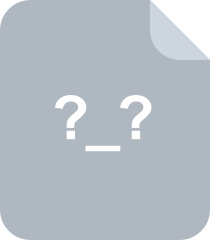
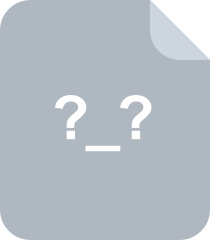
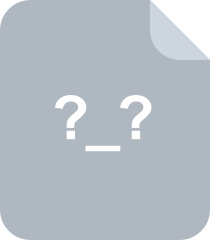


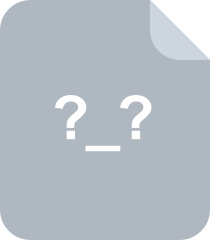
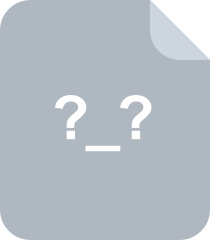
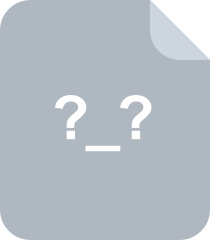
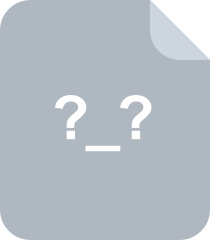
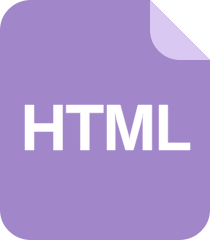

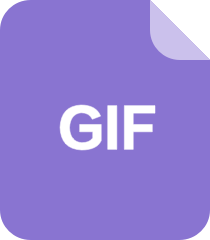
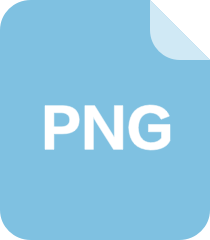
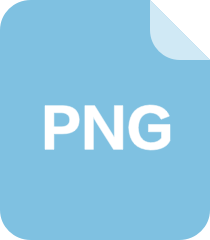

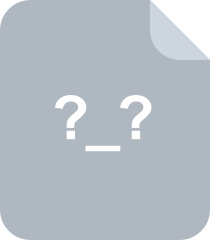
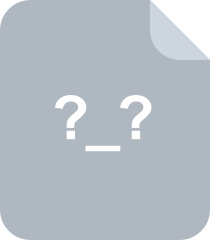
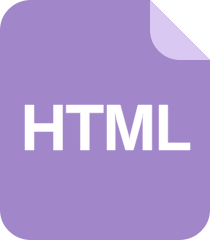
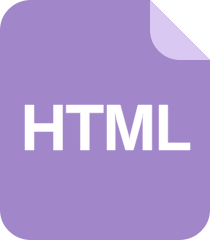
共 28 条
- 1
资源评论
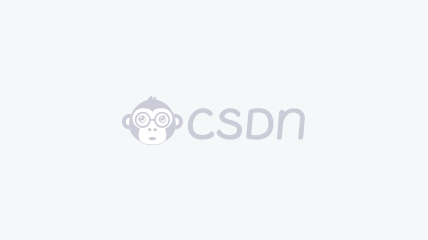


梦回阑珊
- 粉丝: 5409
- 资源: 1707
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

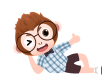
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


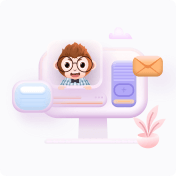
安全验证
文档复制为VIP权益,开通VIP直接复制
