/*
Module : GPS.CPP
Purpose: "C" style Implementation to GPS32
Created: PJN / 28-12-1997
History: PJN / 20-01-1998 1. A number of problems were discovered when GPSLIB was being
used in a UNICODE build. These problems have been fixed
2. Mak file now includes all the possible build options
3. Mak file no longer hard codes output to d:\winnt\system32
4. Included release binaries in distribution
PJN / 26-08-1998 1. Small change to stdafx.h to include afxcview.h in gps32exe
2. The GpsShowControlPanel function now actually works
3. Minor tweaks to the GPS Mak files
4. Removed a number of unused symbols
5. Change ID of a number of GPS32EXE menu items to avoid conflict
with new VC 6 defines.
6. Updated all Version Infos to 1.02
7. Removed a level 4 warning from the Test app.
8. Removed unused toolbar from GPS CPL file
9. GPS cpl file now uses standard F2 accelerator for Rename
10. GPS32EXE toolbar is now shown flat
11. Number of extra TRACE statements have been added to
aid in debugging.
PJN / 11-08-1999 1. Now uses the WINAPI calling convention meaning that GPSLIB is now
callable from VB.
2. Now also ships a GPS.BAS file for use of GPSLIB in VB
PJN / 27-09-2001 1. Fixed a problem where the background serial port thread using causing
heavy CPU utilization.
2. Updated the project settings so that all binaries get build to the Bin
directory and all Lib files get created in a Lib directory
3. Updated the version info's in all the binaries
4. Updated the copyright message in the code modules
PJN / 21-08-2002 1. Fixed a synchronisation problem between the worker thread and the
GpsGetPosition method. Thanks to Bill Oatman for spotting this problem.
Copyright (c) 1997 - 2002 by PJ Naughter. (Web: www.naughter.com, Email: pjna@naughter.com)
All rights reserved.
Copyright / Usage Details:
You are allowed to include the source code in any product (commercial, shareware, freeware or otherwise)
when your product is released in binary form. You are allowed to modify the source code in any way you want
except you cannot modify the copyright details at the top of each module. If you want to distribute source
code with your application, then you are only allowed to distribute versions released by the author. This is
to maintain a single distribution point for the source code.
*/
///////////////////////////////// Includes //////////////////////////////////
#include "stdafx.h"
#include "gps.h"
#include "serport.h"
#include "resource.h"
#include "nmea.h"
#include "math.h"
#include "gpssetup.h"
////////////////////////////////// Macros / Defines //////////////////////////
#ifdef _DEBUG
#undef THIS_FILE
static char BASED_CODE THIS_FILE[] = __FILE__;
#define new DEBUG_NEW
#endif
////////////////////////////////// Locals /////////////////////////////////////
class GPSHandle
{
public:
GPSHandle();
~GPSHandle();
GPSPOSITION m_Position; //the actual data
CEvent* m_pKillEvent; //Event to signal thread to exit
CEvent* m_pStartEvent; //Is the background thread running
GPSDEVINFO m_DevInfo; //Settings of the comms port to use
BOOL m_bRunning; //Is the background thread running
CWinThread* m_pThread; //The pointer to the background thread
CCriticalSection m_csPosition; //Critical section used to serialized access to m_Position
};
BOOL GetGpsDevice(DWORD dwDevice, LPGPSDEVINFO lpGpsDevInfo);
BOOL SetGpsDevice(DWORD dwDevice, LPCGPSDEVINFO lpGpsDevInfo, BOOL bCheckDefault = TRUE);
BOOL SetGpsNumDevices(DWORD dwDevices);
UINT GpsMonitorThead(LPVOID pParam);
////////////////////////////////// Implementation /////////////////////////////
GPSHandle::GPSHandle()
{
ZeroMemory(&m_Position, sizeof(GPSPOSITION));
m_pKillEvent = new CEvent(FALSE, TRUE);
m_pStartEvent = new CEvent(FALSE, TRUE);
m_pThread = NULL;
}
GPSHandle::~GPSHandle()
{
delete m_pStartEvent;
m_pStartEvent = NULL;
delete m_pKillEvent;
m_pKillEvent = NULL;
}
BOOL GetGpsDevice(DWORD dwDevice, LPGPSDEVINFO lpGpsDevInfo)
{
BOOL bSuccess = FALSE;
HKEY hKey;
CString sValueKey;
sValueKey.Format(_T("SOFTWARE\\PJ Naughter\\GPS32\\%d"), dwDevice);
LONG nError = RegOpenKeyEx(HKEY_LOCAL_MACHINE, sValueKey, 0, KEY_ALL_ACCESS, &hKey);
if (nError == ERROR_SUCCESS)
{
DWORD dwType;
DWORD dwSize;
RegQueryValueEx(hKey, _T("DeviceName"), 0, &dwType, NULL, &dwSize);
TCHAR* pszName = new TCHAR[dwSize / sizeof(TCHAR)];
RegQueryValueEx(hKey, _T("DeviceName"), 0, &dwType, (LPBYTE) pszName, &dwSize);
_tcscpy(lpGpsDevInfo->szDeviceName, pszName);
delete [] pszName;
RegQueryValueEx(hKey, _T("DefaultReceiver"), 0, &dwType, (LPBYTE) &lpGpsDevInfo->bDefaultReceiver, &dwSize);
RegQueryValueEx(hKey, _T("Port"), 0, &dwType, (LPBYTE) &lpGpsDevInfo->wCommPort, &dwSize);
RegQueryValueEx(hKey, _T("BaudRate"), 0, &dwType, (LPBYTE) &lpGpsDevInfo->dwCommBaudRate, &dwSize);
RegQueryValueEx(hKey, _T("DataBits"), 0, &dwType, (LPBYTE) &lpGpsDevInfo->wCommDataBits, &dwSize);
RegQueryValueEx(hKey, _T("StopBits"), 0, &dwType, (LPBYTE) &lpGpsDevInfo->wCommStopBits, &dwSize);
bSuccess = TRUE;
RegCloseKey(hKey);
}
else
{
TRACE(_T("GetGpsDevice, Failed to open a registry key, Error was: %d\n"), nError);
}
return bSuccess;
}
BOOL GpsSetNumDevices(DWORD dwDevices)
{
BOOL bSuccess = FALSE;
HKEY hKey;
DWORD dwDisposition;
LONG nError = RegCreateKeyEx(HKEY_LOCAL_MACHINE, _T("SOFTWARE\\PJ Naughter\\GPS32"), 0, _T(""),
REG_OPTION_NON_VOLATILE, KEY_ALL_ACCESS, NULL, &hKey, &dwDisposition);
if (nError == ERROR_SUCCESS)
{
RegSetValueEx(hKey, _T("NumberOfDevices"), 0, REG_DWORD, (CONST BYTE*) &dwDevices, sizeof(DWORD));
RegCloseKey(hKey);
bSuccess = TRUE;
}
else
{
TRACE(_T("GpsSetNumDevices, Failed in call to create a registry key, Error was: %d\n"), nError);
}
return bSuccess;
}
BOOL SetGpsDevice(DWORD dwDevice, LPCGPSDEVINFO lpDevice, BOOL bCheckDefault)
{
//Fix up the case where we have just made this device
//the default by removing the default flag from all
//other devices
if (bCheckDefault && lpDevice->bDefaultReceiver)
{
//make all other devices non default
for (DWORD i=0; i<GpsGetNumDevices(); i++)
{
GPSDEVINFO devInfo;
GetGpsDevice(i, &devInfo);
devInfo.bDefaultReceiver = FALSE;
SetGpsDevice(i, &devInfo, FALSE);
}
}
BOOL bSuccess = FALSE;
HKEY hKey;
DWORD dwDisposition;
CString sValueKey;
sValueKey.Format(_T("SOFTWARE\\PJ Naughter\\GPS32\\%d"), dwDevice);
LONG nError = RegCreateKeyEx(HKEY_LOCAL_MACHINE, sValueKey, 0, _T(""), REG_OPTION_NON_VOLATILE,
KEY_ALL_ACCESS, NULL, &hKey, &dwDisposition);
if (nError == ERROR_SUCCESS)
{
RegSetValueEx(hKey, _T("DeviceName"), 0, REG_SZ, (CONST BYTE*) lpDevice->szDeviceName, _tcslen(lpDevice->szDeviceName));
RegSetValueEx(hKey, _T("DefaultReceiver"), 0, REG_DWORD, (CONST BYTE*) &lpDevice->bDefaultReceiver, sizeof(BOOL));
DWORD dwData = lpDevice->wCommPort;
RegSetValueEx(hKey, _T("Port"), 0, REG_DWORD, (CONST BYTE*) &dwData, sizeof(DWORD));

普通网友
- 粉丝: 882
- 资源: 2万+
最新资源
- 半桥型流阀损耗解析计算模型 分析半桥型MMC损耗分为通态损耗和开关损耗,依据桥臂电流方向建立各器件的通态损耗模型;依据桥臂电压变化和电流方向分段建立器件的开关损耗模型 在MATLAB中进行仿真对比分
- 两极式单相光伏并网仿真 前极:Boost电路+扰动观察法 后极:桥式逆变+L型滤波+电压外环电流内环控制 并网电流和电网电压同频同相,单位功率因数并网,谐波失真率0.39%,并网效率高
- 两极式单相光伏并网仿真 前极:Boost电路+扰动观察法 后极:桥式逆变+L型滤波+电压外环电流内环控制 并网电流和电网电压同频同相,单位功率因数并网,谐波失真率0.39%,并网效率高 有配套vide
- Comsol等离子体仿真,Ar棒板流注放电 电子密度,电子温度,三维视图,电场强度等
- 考虑电动汽车接入的主动配电网优化调度
- 一种采用RRT*机械臂轨迹避障算法,然后采用三次B 样条函数对 所 规 划 路 径 进 行 拟 合 优 化 带有较为详细的注视 rrt路径规划结合机械臂仿真 基于matlab,6自由度,机械臂+rr
- 改进共生搜索算法(CSOS),测试函数效果如下,采用多种改进策略,与多种群智能算法在初始种群数量为30,最大运行次数为500,独立运行次数为30,对比效果如下
- 三电平逆变器运行在三相不平衡电网仿真 可选基于延时相消法(DSC)和双二阶广义积分器(DSOGI)的正负序分离控制 默认DSC 控制交流侧输出为对称三相电流波形,注入电网 电流谐波含量低 SVPW
- 永磁同步模型电流预测控制+滑模控制 1速度环采用滑模控制 滑模控制器采用新型趋近律与扰动观测器结合,提高系统鲁棒性和稳态特性 2电流环采用预测控制双矢量改进算法 含有对应学习文献
- jdk1.8版本,有window64和32位、linux版本
- 计算机网络技术领域《高级网络技术》实训指导书-网络规划与设备配置实训
- 注浆模型: 1.随机裂隙网络注浆模型,含ppt,考虑不同注浆压力下的注浆效果 2.基于两相达西定律、多孔介质及达西定律的注浆模型 3.基于层流和水平集的注浆扩散模型
- 智混合动力汽车SIMULINK整车模型,并联P2构型,基于规则的控制策略,模型运行及仿真无误
- 基于Python的学生信息管理系统的实现与应用
- MATLAB路径规划仿真 轨迹规划,船舶轨迹跟踪控制,数学模型基于两轮差速的小车模型,用PID环节对航向角进行控制,迫使小车走向目标,或用PID环节对航向角和距离进行控制,迫使小车走向目标 LQR 算
- MATLAB代码:电-气-热综合能源系统耦合优化调度 关键词:综合能源系统 优化调度 电气热耦合 参考文档:自编文档,非常细致详细,可联系我查阅 仿真平台:MATLAB YALMIP+cplex
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


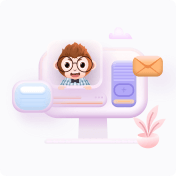