没有合适的资源?快使用搜索试试~ 我知道了~
计算机图形学之渲染算法:Path Tracing:BRDF理论与实现.docx
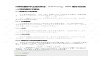

试读
19页
需积分: 0 0 下载量 54 浏览量
更新于2024-11-17
收藏 34KB DOCX 举报
计算机图形学之渲染算法:Path Tracing:BRDF理论与实现.docx
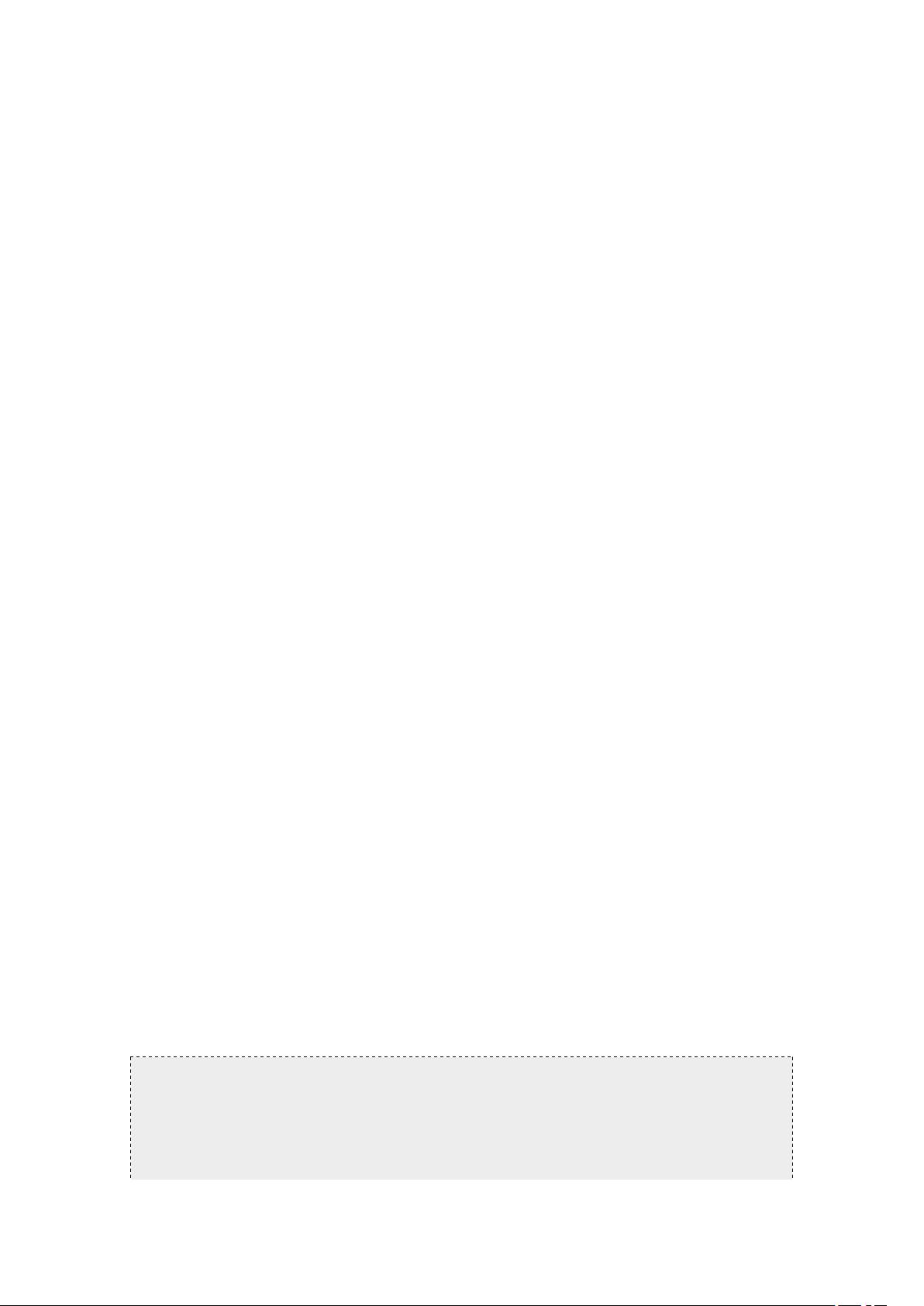
1
计算机图形学之渲染算法:Path Tracing:BRDF 理论与实现
1 计算机图形学基础
1.1 光线与几何基础
在计算机图形学中,光线(ray)是模拟光在三维空间中传播的一种数学模
型。光线由一个原点和一个方向向量定义,可以表示为:
r
(
t
)
=
o
+
t
d
其中,
r
(
t
)
是光线上的点,
o
是光线的原点,
d
是光线的方向向量,而
t
是沿
着光线方向的距离参数。
1.1.1 几何基础
几何基础包括了如何在三维空间中表示和操作物体。在计算机图形学中,
物体通常被表示为多边形网格,其中每个多边形由顶点(vertex)定义。顶点可
以有位置、法线(normal)、纹理坐标等属性。
1.1.1.1 法线
法线是垂直于物体表面的向量,用于计算光照效果。在渲染过程中,法线
的方向对于确定光线如何与表面交互至关重要。
1.1.1.2 顶点位置
顶点的位置是三维空间中的坐标,用于定义物体的形状。这些坐标可以是
世界坐标、模型坐标或屏幕坐标,具体取决于渲染管线的阶段。
1.1.2 光线与几何体的相交测试
光线与几何体相交测试是渲染算法中的关键步骤。对于一个简单的几何体,
如一个平面或一个球体,可以通过解析几何方法来计算光线与几何体的交点。
例如,对于一个球体,其方程为:
(
x
−
x
c
)
2
+
(
y
−
y
c
)
2
+
(
z
−
z
c
)
2
=
r
2
其中,
(
x
c
,
y
c
,
z
c
)
是球体的中心,
r
是球体的半径。如果光线
r
(
t
)
=
o
+
t
d
与球
体相交,那么存在一个
t
值,使得上述方程成立。通过解这个方程,可以找到交
点的
t
值,从而确定光线是否与球体相交以及交点的位置。
// C++
示例代码:光线与球体相交测试
#include <iostream>
#include <cmath>
struct Vector3 {
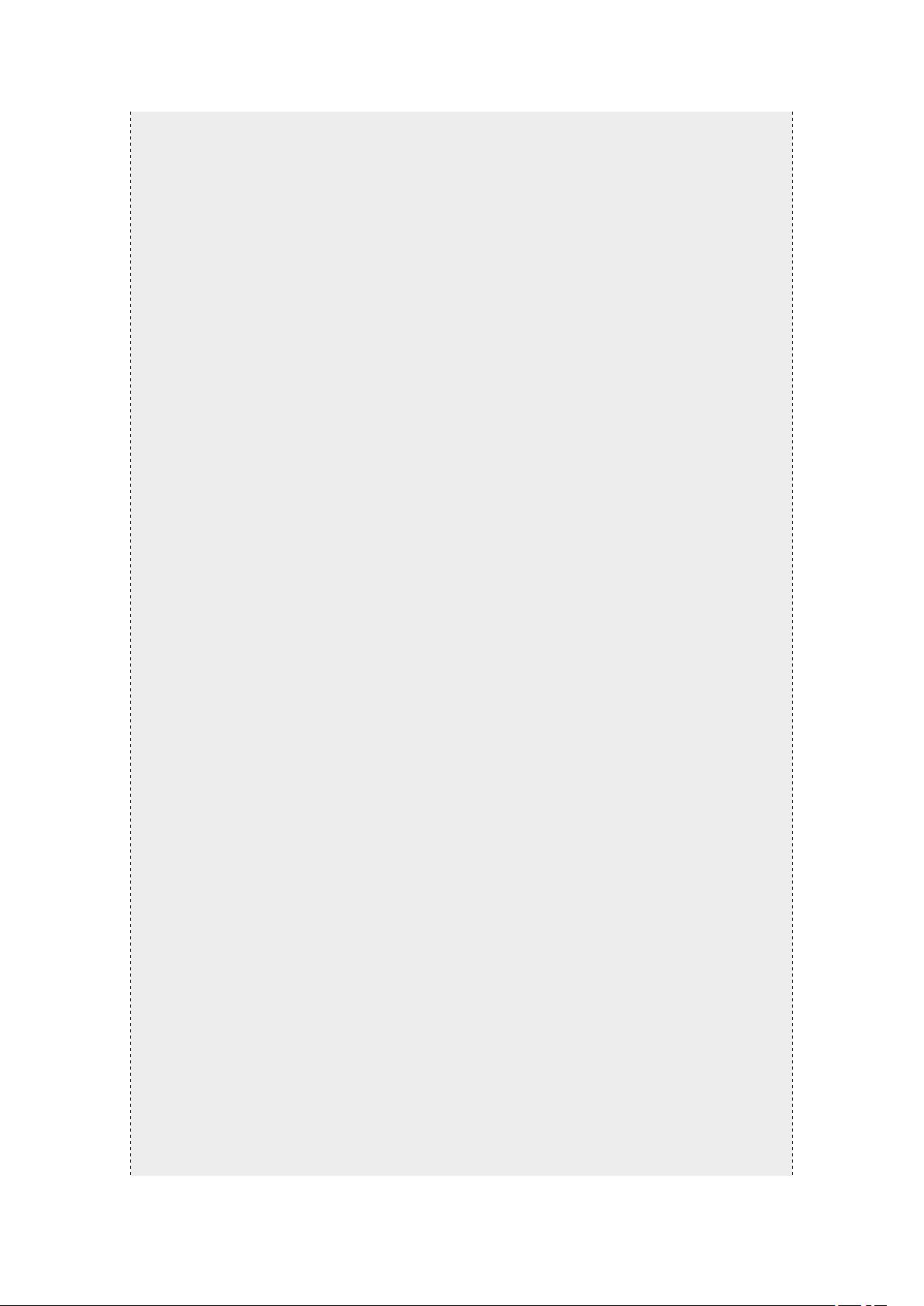
2
float x, y, z;
Vector3(float x, float y, float z) : x(x), y(y), z(z) {}
Vector3 operator-(const Vector3& v) const {
return Vector3(x - v.x, y - v.y, z - v.z);
}
float dot(const Vector3& v) const {
return x * v.x + y * v.y + z * v.z;
}
Vector3 cross(const Vector3& v) const {
return Vector3(y * v.z - z * v.y, z * v.x - x * v.z, x * v.y - y * v.x);
}
Vector3 normalize() const {
float len = sqrt(x * x + y * y + z * z);
return Vector3(x / len, y / len, z / len);
}
};
struct Ray {
Vector3 origin;
Vector3 direction;
Ray(Vector3 origin, Vector3 direction) : origin(origin), direction(direction.normalize()) {}
};
struct Sphere {
Vector3 center;
float radius;
Sphere(Vector3 center, float radius) : center(center), radius(radius) {}
};
bool intersect(const Ray& ray, const Sphere& sphere, float& t) {
Vector3 oc = ray.origin - sphere.center;
float a = ray.direction.dot(ray.direction);
float b = 2.0f * oc.dot(ray.direction);
float c = oc.dot(oc) - sphere.radius * sphere.radius;
float discriminant = b * b - 4.0f * a * c;
if (discriminant < 0.0f) {
return false; //
没有交点
} else {
float sqrt_discriminant = sqrt(discriminant);
float t1 = (-b - sqrt_discriminant) / (2.0f * a);
float t2 = (-b + sqrt_discriminant) / (2.0f * a);
if (t1 > 0.0f) {
t = t1;
return true;
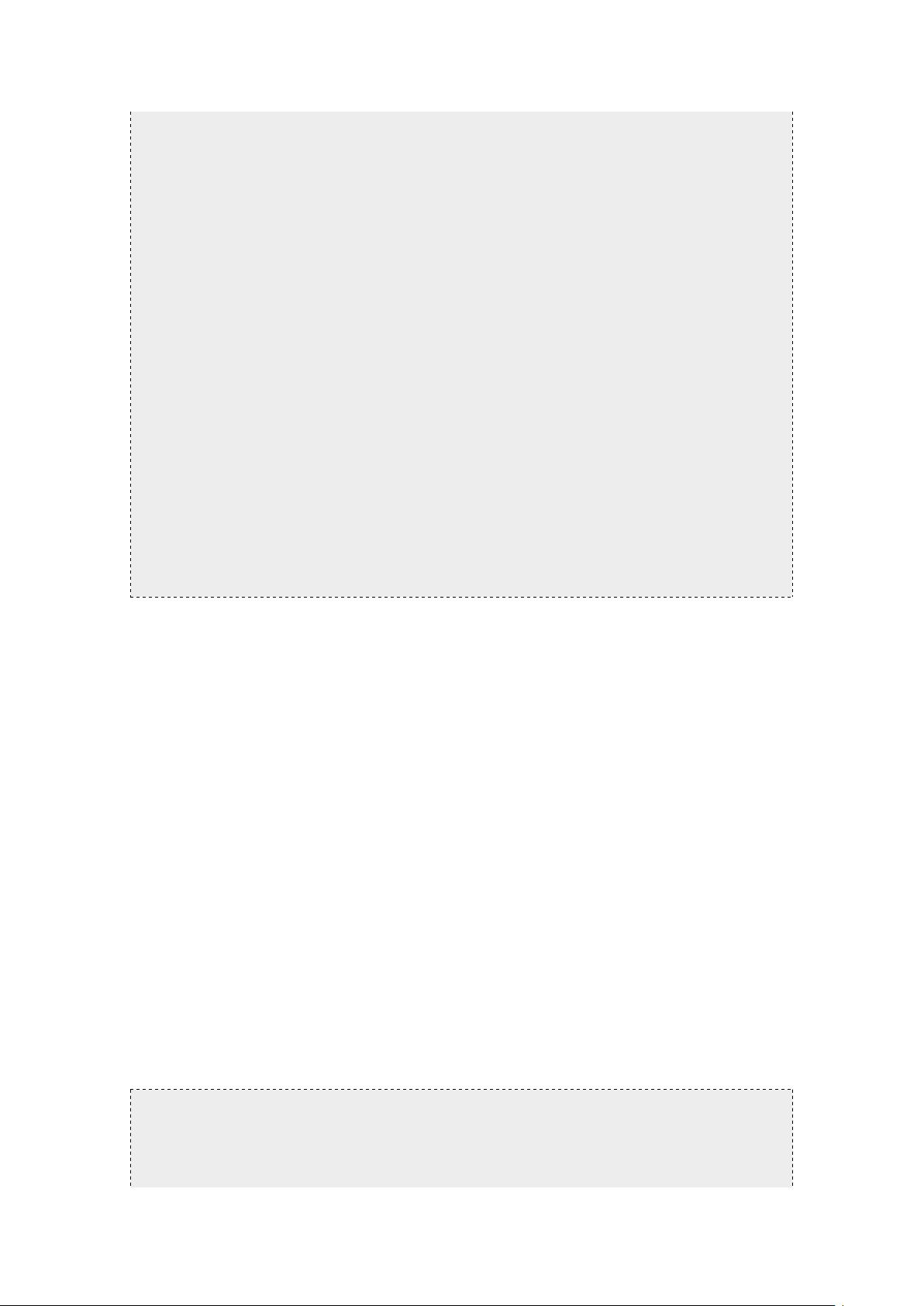
3
} else if (t2 > 0.0f) {
t = t2;
return true;
} else {
return false; //
交点在光线的反方向
}
}
}
int main() {
Ray ray(Vector3(0, 0, 0), Vector3(0, 0, 1));
Sphere sphere(Vector3(0, 0, 5), 2);
float t;
if (intersect(ray, sphere, t)) {
std::cout << "光线与球体相交,交点距离为: " << t << std::endl;
} else {
std::cout << "光线与球体不相交" << std::endl;
}
return 0;
}
1.2 颜色与光照模型
颜色在计算机图形学中是通过红、绿、蓝(RGB)三个颜色通道的组合来
表示的。光照模型描述了光线如何与物体表面交互,以及如何计算物体表面的
颜色。其中,Phong 光照模型是一个常用的模型,它包括了环境光、漫反射和
镜面反射三个部分。
1.2.1 Phong 光照模型
Phong 光照模型的计算公式如下:
I
=
I
a
+
I
d
(
n
⋅
l
)
+
I
s
(
r
⋅
v
)
n
其中,
I
是最终的颜色强度,
I
a
是环境光强度,
I
d
是漫反射强度,
I
s
是镜面反
射强度,
n
是表面法线,
l
是光源方向向量,
r
是反射光线方向向量,
v
是观察者方
向向量,而
n
是镜面反射的高光指数。
1.2.2 实现 Phong 光照模型
在实际的渲染算法中,Phong 光照模型的计算通常在每个像素上进行。下
面是一个使用 Phong 光照模型计算物体表面颜色的 C++示例代码:
// C++
示例代码:
Phong
光照模型
#include <iostream>
struct Color {
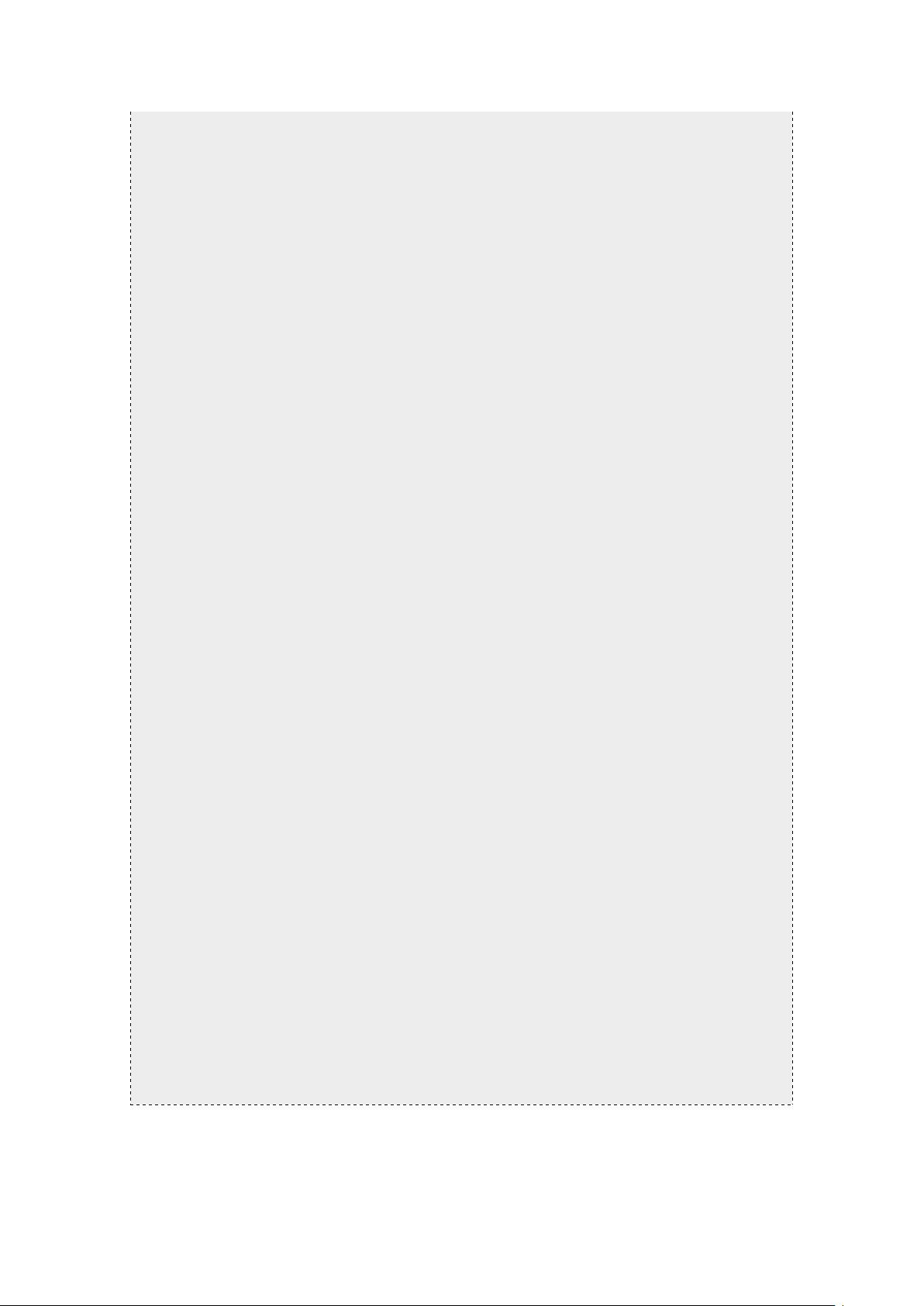
4
float r, g, b;
Color(float r, float g, float b) : r(r), g(g), b(b) {}
Color operator*(const Color& c) const {
return Color(r * c.r, g * c.g, b * c.b);
}
Color operator*(float f) const {
return Color(r * f, g * f, b * f);
}
};
struct Light {
Vector3 direction;
Color color;
Light(Vector3 direction, Color color) : direction(direction), color(color) {}
};
Color phongShading(const Vector3& normal, const Vector3& lightDir, const Vector3& viewDir, co
nst Light& light, const Color& ambient, const Color& diffuse, const Color& specular, float shinine
ss) {
Vector3 reflection = normal * (2.0f * normal.dot(lightDir)) - lightDir;
float diffuseFactor = std::max(0.0f, normal.dot(lightDir));
float specularFactor = std::pow(std::max(0.0f, reflection.dot(viewDir)), shininess);
return ambient + (diffuse * light.color) * diffuseFactor + (specular * light.color) * specularFacto
r;
}
int main() {
Vector3 normal(0, 0, 1);
Vector3 lightDir(0, 0, -1);
Vector3 viewDir(0, 0, 1);
Light light(lightDir, Color(1, 1, 1));
Color ambient(0.1, 0.1, 0.1);
Color diffuse(0.5, 0.5, 0.5);
Color specular(1, 1, 1);
float shininess = 32.0f;
Color color = phongShading(normal, lightDir, viewDir, light, ambient, diffuse, specular, shinines
s);
std::cout << "计算后的颜色为: (" << color.r << ", " << color.g << ", " << color.b << ")" << std::en
dl;
return 0;
}
剩余18页未读,继续阅读
资源推荐
资源评论
112 浏览量
2009-05-14 上传
2022-03-30 上传

105 浏览量
186 浏览量
198 浏览量
2022-03-30 上传
123 浏览量
164 浏览量
133 浏览量

123 浏览量

154 浏览量
176 浏览量
132 浏览量
资源评论
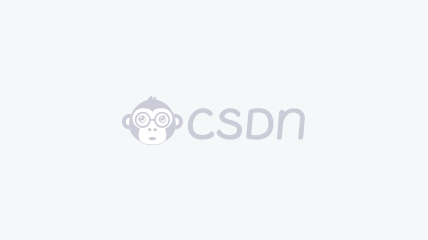

chenlz2007
- 粉丝: 9606
- 资源: 425
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

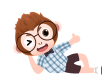
最新资源
- 该仿真主要应用于路径规划和轨迹跟踪的研究 主要有五个文件(内部包含3个算法,两个仿真),1.A星算法自动生成避障的最短路径,2.两轮小车及其四轮小车的运动学建模3.纯路径跟踪算法,包括预锚点的选择算
- Edifier Connect_8.4.11.apk
- anaconda配置pytorch环境.md
- 机械设计自动倒角机sw18可编辑非常好的设计图纸100%好用.zip
- 基于28027滑膜算法的水泵驱动方案,带有初始定位算法,启动不反转,pfc采用硬件方案
- 机械设计自动PCM板胶纸机sw18非常好的设计图纸100%好用.zip
- 三相PWM整流器闭环仿真,电压电流双闭环控制,输出直流电压做外环 模型中包含主电路,坐标变,电压电流双环PI控制器,SVPWM控制,PWM发生器 matlab simulink模型 功率因数1,低TH
- anaconda配置pytorch环境.md
- Cytoscape-3-10-3-windows-64bit-仅限个人学习
- DDR3测试模块 fpga测试接口模块,提供测试模块工程 Vivado2019.1或者Vivado2017.4;语言vhdl 可以修改ip引脚,测试初始化完成
- 网络工程领域竞赛试题:网络搭建与应用的技术实战项目
- 山东大学软件学院人工智能导论22级复习资料
- 机械设计自动切断面条机ZD80sw16非常好的设计图纸100%好用.zip
- 毕设基于stm32的风机监控系统程序设计源码.zip
- 机械设计自动贴E型定位胶纸机sw18非常好的设计图纸100%好用.zip
- 机械设计自动贴E型胶纸机sw18非常好的设计图纸100%好用.zip
安全验证
文档复制为VIP权益,开通VIP直接复制
