#include "GameScene.h"
#include "Utility.h"
#include <algorithm>
GameScene::GameScene():
m_state(GS_START),
m_nGoldenBubbleCount(0),
m_nSliveryBubbleCount(0),
m_nScore(0)
{
SimpleAudioEngine::sharedEngine()->playBackgroundMusic("game_scene_bg.mp3",true);
SimpleAudioEngine::sharedEngine()->playEffect("ready_go.mp3");
srand(time(NULL));
}
GameScene::~GameScene()
{
//clear();
}
CCScene* GameScene::scene()
{
CCScene* scene = NULL;
do
{
scene = CCScene::create();
CC_BREAK_IF(!scene);
GameScene* layer = GameScene::create();
CC_BREAK_IF(!layer);
scene->addChild(layer);
} while (0);
return scene;
}
bool GameScene::init()
{
bool bRet = false;
do
{
CC_BREAK_IF(!CCLayer::init());
//加载背景
CCSprite *background = CCSprite::create("background1.jpg");
CC_BREAK_IF(!background);
background->setAnchorPoint(CCPointZero);
background->setPosition(CCPointZero);
this->addChild(background);
CC_BREAK_IF(!GameScene::initScheduler());
CC_BREAK_IF(!GameScene::initBoard());
CC_BREAK_IF(!GameScene::initReadyBubble());
CC_BREAK_IF(!GameScene::initWaitBubble());
this->setEnable();
bRet = true;
} while (0);
return bRet;
}
bool GameScene::initScheduler()
{
//this->schedule(schedule_selector(GameScene::loop), 1.0f);
//this->scheduleUpdate();
return true;
}
//初始化泡泡队列,
bool GameScene::initBoard()
{
bool bRet = false;
do
{
for (int row = 0; row < MAX_ROWS; row++)
{
for (int col = 0; col < MAX_COLS - row % 2; col++)
{
//初始化前INIT_LINE行, 其他行为NULL
if (row >= INIT_LINE)
{
m_board[row][col] = NULL;
continue;
}
Bubble* pBubble = randomBubble();
if (pBubble == NULL)
{
CC_BREAK_IF(!pBubble);
}
//
pBubble->setPosition(getPosByRowAndCol(row, col));
this->addChild(pBubble);
m_board[row][col] = pBubble;
m_board[row][col]->setRowColIndex(row, col);
m_listBubble.push_back(pBubble);
bRet = true;
}
}
} while (0);
return bRet;
}
//初如化泡泡发射器
bool GameScene::initReadyBubble()
{
bool bRet = false;
do
{
m_curReady = randomBubble();
CC_BREAK_IF(!m_curReady);
CCSize size = CCDirector::sharedDirector()->getWinSize();
m_curReady->setPosition(ccp(size.width / 2, size.height / 10));
this->addChild(m_curReady);
bRet = true;
} while (0);
return bRet;
}
bool GameScene::initWaitBubble()
{
bool bRet = false;
do
{
for (int i = 0; i < MAX_WAIT_BUBBLE; i++)
{
Bubble *pBubble = randomBubble();
CC_BREAK_IF(!pBubble);
CCSize size = CCDirector::sharedDirector()->getWinSize();
pBubble->setPosition(ccp(size.width/2 + (i+1) * BUBBLE_RADIUS * 2, size.height/20));
m_wait[i] = pBubble;
this->addChild(pBubble);
bRet = true;
}
} while (0);
return bRet;
}
Bubble* GameScene::randomBubble()
{
BUBBLE_COLOR color = static_cast<BUBBLE_COLOR>(rand() % (COLOR_COUNT/* - 2*/));
Bubble *pBubble = NULL;
do
{
pBubble = Bubble::create();
if(pBubble && pBubble->initWithFile(g_bubbleName[color].c_str()))
{
pBubble->setBubbleColor(color);
}
else
{
CC_SAFE_DELETE(pBubble);
}
} while (0);
return pBubble;
}
Bubble* GameScene::createBubble(BUBBLE_COLOR color)
{
Bubble *pBubble = NULL;
do
{
pBubble = Bubble::create();
if(pBubble && pBubble->initWithFile(g_bubbleName[color].c_str()))
{
pBubble->setBubbleColor(color);
}
else
{
CC_SAFE_DELETE(pBubble);
}
} while (0);
return pBubble;
}
void GameScene::clear()
{
for (int nRow = 0; nRow < MAX_ROWS; nRow++)
{
for (int nCol = 0; nCol < MAX_COLS - nRow % 2; nCol++)
{
CC_SAFE_DELETE(m_board[nRow][nCol]);
}
}
m_listBubble.clear();
}
bool GameScene::isCollisionWithBorder() //是否和边缘碰撞
{
CCSize size = CCDirector::sharedDirector()->getWinSize();
CCPoint pos = m_curReady->getPosition();
if (pos.x < BUBBLE_RADIUS || pos.x > size.width - BUBBLE_RADIUS)
{
return true;
}
return false;
}
bool GameScene::isCollisionWithTopBorder(Bubble *pBubble)
{
if (pBubble == NULL)
{
return false;
}
CCPoint pos = pBubble->getPosition();
CCSize size = CCDirector::sharedDirector()->getWinSize();
if (pos.y > size.height - BUBBLE_RADIUS)
{
return true;
}
return false;
}
bool GameScene::isCollisionWithBubble(CCPoint pos1, float radius1, CCPoint pos2, float radius2) //是否和上方的球碰撞
{
return pow(pos1.x - pos2.x, 2) + pow(pos1.y - pos2.y, 2) < pow(radius1 + radius2, 2); //判断两圆是否相交, 公式:(x1-x2)^2 + (y1-y2)^2 < (r1 + r2)^2
}
bool GameScene::isCollision()
{
bool bRet = false;
CCSize size = CCDirector::sharedDirector()->getWinSize();
if (m_curReady->getPosition().y > size.height - BUBBLE_RADIUS)
{
bRet = true;
return bRet;
}
for (BUBBLE_LIST::reverse_iterator iterBubble = m_listBubble.rbegin(); iterBubble != m_listBubble.rend(); ++iterBubble)
{
Bubble *pBubble = *iterBubble;
if (pBubble && isCollisionWithBubble(pBubble->getPosition(), BUBBLE_RADIUS, m_curReady->getPosition(), BUBBLE_RADIUS))
{
bRet = true;
return bRet;
}
}
return bRet;
}
void GameScene::setEnable()
{
CCDirector::sharedDirector()->getTouchDispatcher()->addTargetedDelegate(this, 0, true);
}
void GameScene::setDisableEnable()
{
CCDirector::sharedDirector()->getTouchDispatcher()->removeDelegate(this);
}
bool GameScene::ccTouchBegan(CCTouch *pTouch, CCEvent *pEvent)
{
return true;
}
void GameScene::ccTouchMoved(CCTouch *pTouch, CCEvent *pEvent)
{
}
void GameScene::ccTouchEnded(CCTouch *pTouch, CCEvent *pEvent)
{
// if (m_state == GS_FLY || m_state == GS_FALL)
// {
// return ;
// }
m_state = GS_FLY;
CCPoint pos = pTouch->getLocation();
m_real = ccpNormalize(ccpSub(pos, m_curReady->getPosition()));
setDisableEnable();
this->scheduleUpdate();
}
void GameScene::loop(float dt)
{
}
void GameScene::update(float delta)
{
if (isCollisionWithBorder())
{
m_real.x = -m_real.x;
}
CCPoint pos = m_curReady->getPosition();
m_curReady->setPosition(ccp(pos.x + m_real.x * BUBBLE_SPEED, pos.y + m_real.y * BUBBLE_SPEED));
if (isCollision()) //如果和球或者上边缘碰撞了, 做相应的处理
{
m_real = CCPointZero;
adjustBubblePosition();
//根据同样的球数量作出相应的清理处理
execClearBubble(m_curReady);
//清除球之后,掉落处于悬挂状态的球
ROWCOL_LIST fallList = checkFallBubble();
FallBubble(fallList);
this->unscheduleUpdate();
changeWaitToReady();
setEnable();
}
}
void GameScene::adjustBubblePosition()
{
CCPoint curPos = m_curReady->getPosition();
RowCol rowcol_index = GetRowColByPos(curPos.x, curPos.y);
CCPoint adjustPos = getPosByRowAndCol(rowcol_index.m_nRow, rowcol_index.m_nCol);
m_curReady->setPosition(adjustPos);
m_curReady->setRowColIndex(rowcol_index.m_nRow, rowcol_index.m_nCol);
m_board[rowcol_index.m_nRow][rowcol_index.m_nCol] = m_curReady;
m_listBubble.push_back(m_curReady);
}
//将wait状态的球换成ready状态
void GameScene::changeWaitToReady()
{
m_curReady = m_wait[0];
m_curReady->setPosition(READY_BUBBLE_POS);
CCSize size = CCDirector::sharedDirector()->getWinSize();
for (int index = 0; index < MAX_WAIT_BUBBLE - 1; index++)
{
m_wait[index] = m_wait[index + 1];
m_wait[index]->setPosition(ccp(size.width/2 + (index+1) * BUBBLE_RADIUS * 2, size.height/20));
}
m_wait[MAX_WAIT_BUBBLE - 1] = randomBubble();
m_wait[MAX_WAIT_BUBBLE - 1]->setPosition(ccp(size.width/2+MAX_WAIT_BUBBLE * BUBBLE_RADIUS *2, size.height/20));
this->addChild(m_wait[MAX_WAIT_BUBBLE - 1]);
}
ROWCOL_LIST GameScene::findClearBubble(Bubble *pReadyBubble)
{
ROWCOL_LIST clearRowCollist;
if (pReadyBubble == NULL)
{
return clearRowCollist;
}
if (pReadyBubble->getBubbleColo

普通网友
- 粉丝: 0
- 资源: 5
最新资源
- 纯 Python Java 解析器和工具.zip
- YOLO标记口罩数据集 (YOLO 格式注释)
- uniapp+vue3+云开发全栈开发同城配送鲜花小程序任意商城教程
- 客户需求快速小程序项目开发技巧
- java项目,课程设计-医疗服务系统.zip
- YOLO 注释风力涡轮机表面损坏-以 YOLO 格式注释风力涡轮机表面损伤 一万六千多文件
- 第一个适用于 Java 的 REST API 框架.zip
- Nvidia GeForce GT 1030显卡驱动(Win7)
- TIA PORTAL V17 UPD8- 更新包(最新版本2024.09)-链接地址.txt
- 示例应用程序展示了客户端和服务器上 JavaFX 和 Spring 技术的集成.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


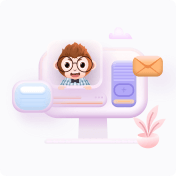
- 1
- 2
- 3
- 4
前往页