# [scikit-opt](https://github.com/guofei9987/scikit-opt)
[](https://pypi.org/project/scikit-opt/)
[](https://github.com/guofei9987/scikit-opt)
[](https://travis-ci.com/guofei9987/scikit-opt)
[](https://codecov.io/gh/guofei9987/scikit-opt)
[](https://pepy.tech/project/scikit-opt)
[](https://github.com/guofei9987/scikit-opt/stargazers)
[](https://github.com/guofei9987/scikit-opt/network/members)
[](https://gitter.im/guofei9987/scikit-opt?utm_source=badge&utm_medium=badge&utm_campaign=pr-badge&utm_content=badge)
一个封装了7种启发式算法的 Python 代码库
(差分进化算法、遗传算法、粒子群算法、模拟退火算法、蚁群算法、鱼群算法、免疫优化算法)
# 安装
```bash
pip install scikit-opt
```
或者直接把源代码中的 `sko` 文件夹下载下来放本地也调用可以
# 特性
## 特性1:UDF(用户自定义算子)
举例来说,你想出一种新的“选择算子”,如下
-> Demo code: [examples/demo_ga_udf.py#s1](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_udf.py#L1)
```python
# step1: define your own operator:
def selection_tournament(algorithm, tourn_size):
FitV = algorithm.FitV
sel_index = []
for i in range(algorithm.size_pop):
aspirants_index = np.random.choice(range(algorithm.size_pop), size=tourn_size)
sel_index.append(max(aspirants_index, key=lambda i: FitV[i]))
algorithm.Chrom = algorithm.Chrom[sel_index, :] # next generation
return algorithm.Chrom
```
导入包,并且创建遗传算法实例
-> Demo code: [examples/demo_ga_udf.py#s2](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_udf.py#L12)
```python
import numpy as np
from sko.GA import GA, GA_TSP
demo_func = lambda x: x[0] ** 2 + (x[1] - 0.05) ** 2 + (x[2] - 0.5) ** 2
ga = GA(func=demo_func, n_dim=3, size_pop=100, max_iter=500, prob_mut=0.001,
lb=[-1, -10, -5], ub=[2, 10, 2], precision=[1e-7, 1e-7, 1])
```
把你的算子注册到你创建好的遗传算法实例上
-> Demo code: [examples/demo_ga_udf.py#s3](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_udf.py#L20)
```python
ga.register(operator_name='selection', operator=selection_tournament, tourn_size=3)
```
scikit-opt 也提供了十几个算子供你调用
-> Demo code: [examples/demo_ga_udf.py#s4](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_udf.py#L22)
```python
from sko.operators import ranking, selection, crossover, mutation
ga.register(operator_name='ranking', operator=ranking.ranking). \
register(operator_name='crossover', operator=crossover.crossover_2point). \
register(operator_name='mutation', operator=mutation.mutation)
```
做遗传算法运算
-> Demo code: [examples/demo_ga_udf.py#s5](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_udf.py#L28)
```python
best_x, best_y = ga.run()
print('best_x:', best_x, '\n', 'best_y:', best_y)
```
> 现在 **udf** 支持遗传算法的这几个算子: `crossover`, `mutation`, `selection`, `ranking`
> Scikit-opt 也提供了十来个算子,参考[这里](https://github.com/guofei9987/scikit-opt/tree/master/sko/operators)
> 提供一个面向对象风格的自定义算子的方法,供进阶用户使用:
-> Demo code: [examples/demo_ga_udf.py#s6](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_udf.py#L31)
```python
class MyGA(GA):
def selection(self, tourn_size=3):
FitV = self.FitV
sel_index = []
for i in range(self.size_pop):
aspirants_index = np.random.choice(range(self.size_pop), size=tourn_size)
sel_index.append(max(aspirants_index, key=lambda i: FitV[i]))
self.Chrom = self.Chrom[sel_index, :] # next generation
return self.Chrom
ranking = ranking.ranking
demo_func = lambda x: x[0] ** 2 + (x[1] - 0.05) ** 2 + (x[2] - 0.5) ** 2
my_ga = MyGA(func=demo_func, n_dim=3, size_pop=100, max_iter=500, lb=[-1, -10, -5], ub=[2, 10, 2],
precision=[1e-7, 1e-7, 1])
best_x, best_y = my_ga.run()
print('best_x:', best_x, '\n', 'best_y:', best_y)
```
## 特性2:断点继续运行
例如,先跑10代,然后在此基础上再跑20代,可以这么写:
```python
from sko.GA import GA
func = lambda x: x[0] ** 2
ga = GA(func=func, n_dim=1)
ga.run(10)
ga.run(20)
```
## 特性3:4种加速方法
- [x] 矢量化计算:vectorization
- [x] 多线程计算:multithreading,适用于 IO 密集型目标函数
- [x] 多进程计算:multiprocessing,适用于 CPU 密集型目标函数
- [x] 缓存化计算:cached,适用于目标函数的每次输入有大量重复
see [https://github.com/guofei9987/scikit-opt/blob/master/examples/example_function_modes.py](https://github.com/guofei9987/scikit-opt/blob/master/examples/example_function_modes.py)
## 特性4: GPU 加速
GPU加速功能还比较简单,将会在 1.0.0 版本大大完善。
有个 demo 已经可以在现版本运行了: [https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_gpu.py](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga_gpu.py)
# 快速开始
## 1. 差分进化算法
**Step1**:定义你的问题,这个demo定义了有约束优化问题
-> Demo code: [examples/demo_de.py#s1](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_de.py#L1)
```python
'''
min f(x1, x2, x3) = x1^2 + x2^2 + x3^2
s.t.
x1*x2 >= 1
x1*x2 <= 5
x2 + x3 = 1
0 <= x1, x2, x3 <= 5
'''
def obj_func(p):
x1, x2, x3 = p
return x1 ** 2 + x2 ** 2 + x3 ** 2
constraint_eq = [
lambda x: 1 - x[1] - x[2]
]
constraint_ueq = [
lambda x: 1 - x[0] * x[1],
lambda x: x[0] * x[1] - 5
]
```
**Step2**: 做差分进化算法
-> Demo code: [examples/demo_de.py#s2](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_de.py#L25)
```python
from sko.DE import DE
de = DE(func=obj_func, n_dim=3, size_pop=50, max_iter=800, lb=[0, 0, 0], ub=[5, 5, 5],
constraint_eq=constraint_eq, constraint_ueq=constraint_ueq)
best_x, best_y = de.run()
print('best_x:', best_x, '\n', 'best_y:', best_y)
```
## 2. 遗传算法
**第一步**:定义你的问题
-> Demo code: [examples/demo_ga.py#s1](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga.py#L1)
```python
import numpy as np
def schaffer(p):
'''
This function has plenty of local minimum, with strong shocks
global minimum at (0,0) with value 0
https://en.wikipedia.org/wiki/Test_functions_for_optimization
'''
x1, x2 = p
part1 = np.square(x1) - np.square(x2)
part2 = np.square(x1) + np.square(x2)
return 0.5 + (np.square(np.sin(part1)) - 0.5) / np.square(1 + 0.001 * part2)
```
**第二步**:运行遗传算法
-> Demo code: [examples/demo_ga.py#s2](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga.py#L16)
```python
from sko.GA import GA
ga = GA(func=schaffer, n_dim=2, size_pop=50, max_iter=800, prob_mut=0.001, lb=[-1, -1], ub=[1, 1], precision=1e-7)
best_x, best_y = ga.run()
print('best_x:', best_x, '\n', 'best_y:', best_y)
```
**第三步**:用 matplotlib 画出结果
-> Demo code: [examples/demo_ga.py#s3](https://github.com/guofei9987/scikit-opt/blob/master/examples/demo_ga.py#L22)
```python
import pandas as pd
import matplotlib.pyplot as plt
Y_history = pd.
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
基于遗传算法、粒子群算法、模拟退火、蚁群算法、免疫优化算法、鱼群算法,旅行商问题仿真(完整源码+数据).zip 已获导师指导并通过的97分的高分课程设计项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。 基于遗传算法、粒子群算法、模拟退火、蚁群算法、免疫优化算法、鱼群算法,旅行商问题仿真(完整源码+数据).zip 已获导师指导并通过的97分的高分课程设计项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。基于遗传算法、粒子群算法、模拟退火、蚁群算法、免疫优化算法、鱼群算法,旅行商问题仿真(完整源码+数据).zip 已获导师指导并通过的97分的高分课程设计项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。基于遗传算法、粒子群算法、模拟退火、蚁群算法、免疫优化算法、鱼群算法,旅行商问题仿真(完整源码+数据).zip 已获导师指导并通过的97分的高分课程设计项目,可作为课程设计和期末大作业,下载即用无需修改,项目完整确保可以运行。基于遗传算法、粒子群算法、模拟退火、蚁群算法、免疫优化算法、鱼群算法,旅行商问题仿真
资源推荐
资源详情
资源评论
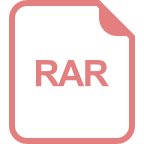
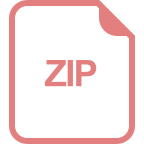
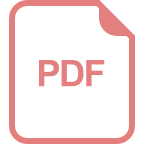
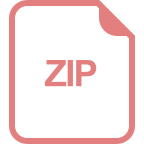
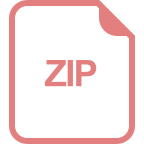
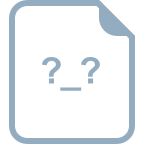
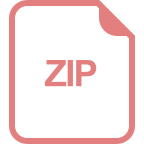
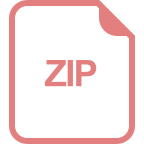
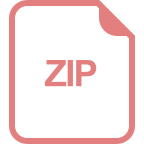
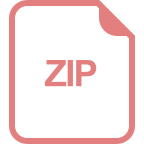
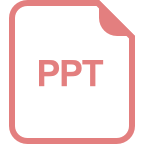
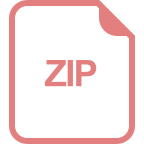
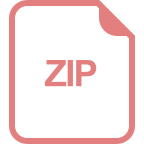
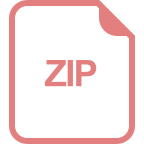
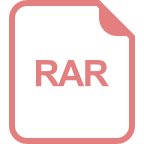
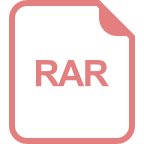
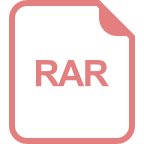
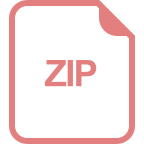
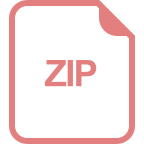
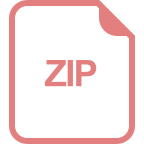
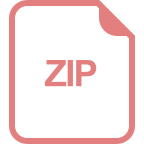
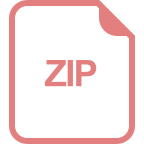
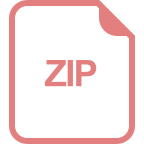
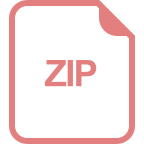
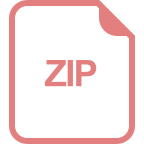
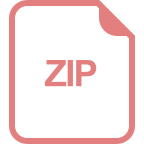
收起资源包目录



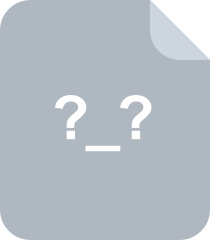
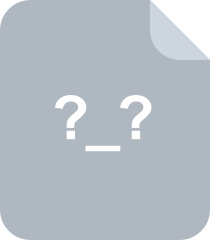
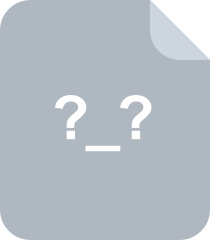

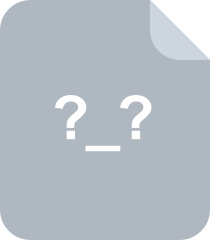
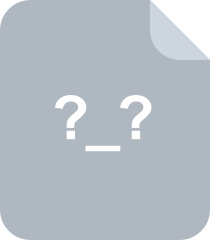
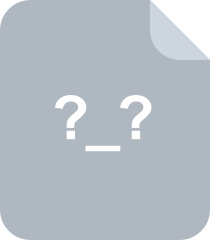
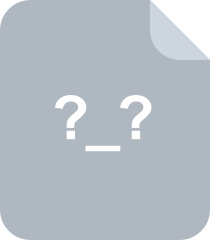
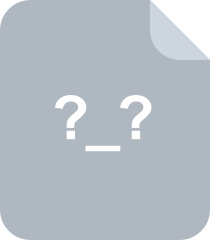
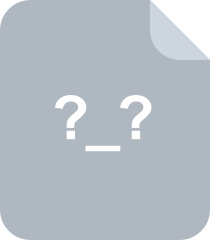

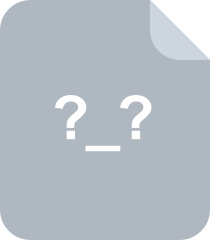
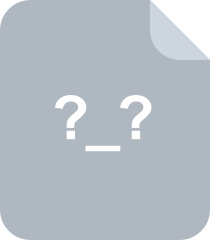
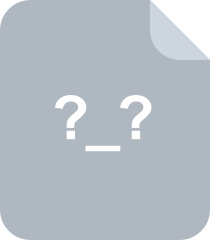
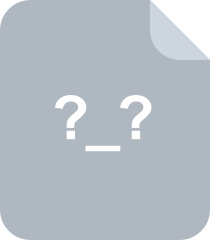
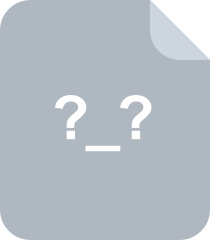
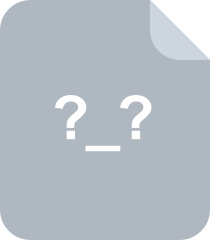
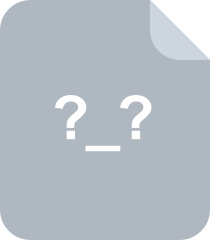
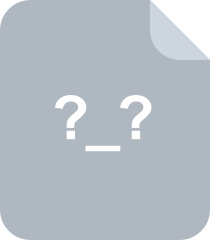
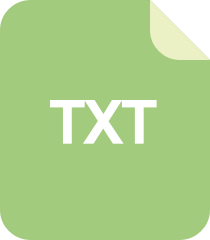
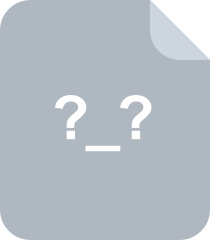
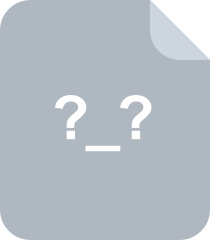
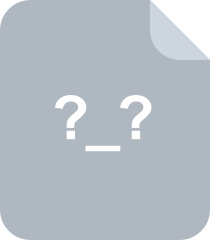
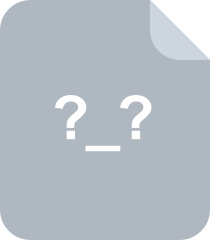
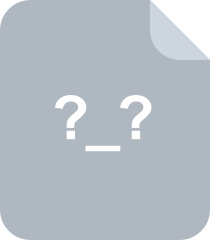
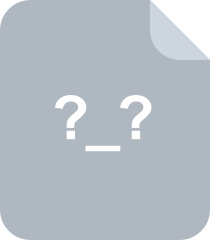
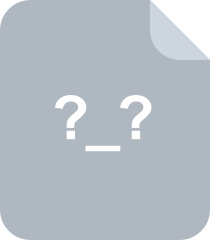

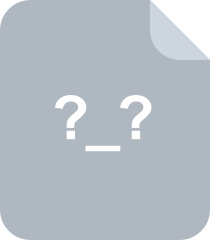
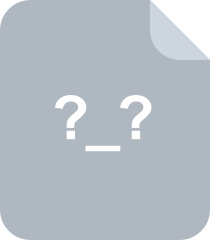
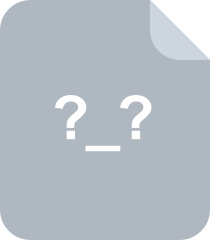
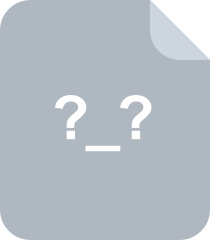

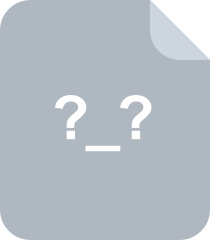
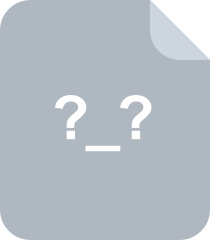
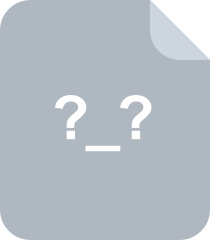
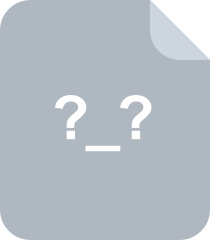

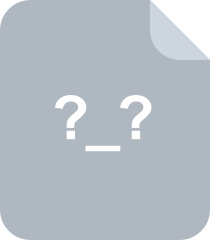
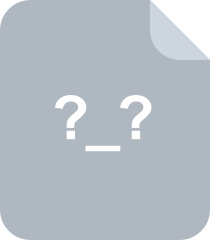
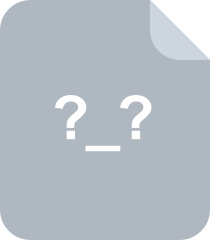
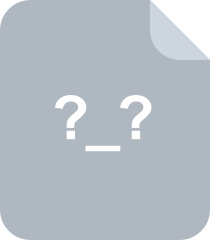
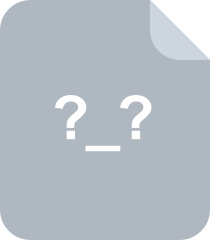
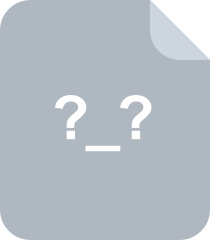
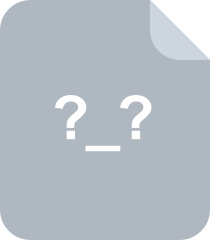
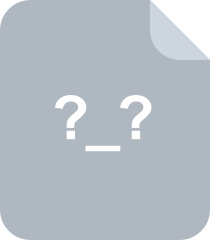
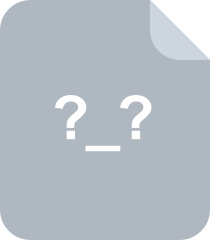
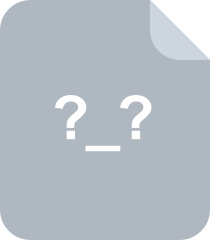
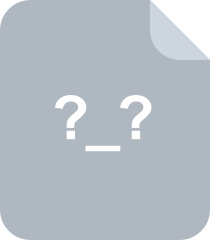
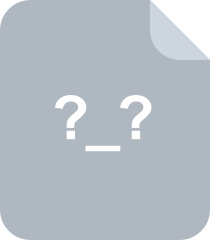
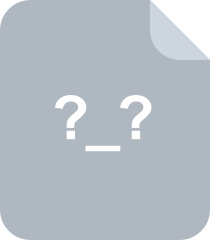
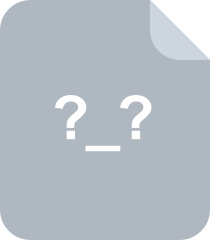
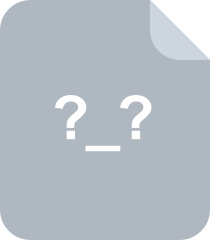
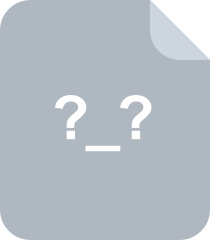

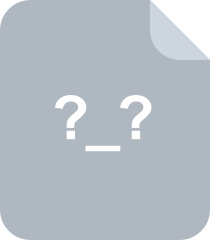
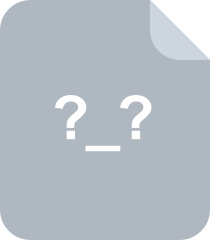
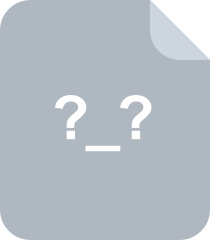
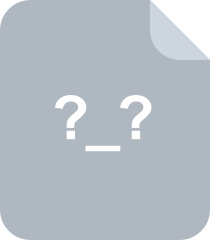
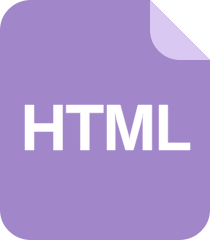
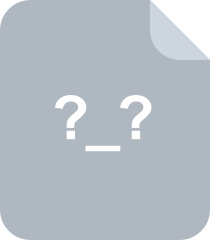
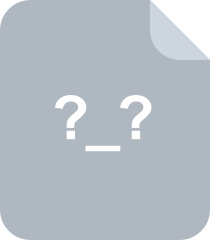

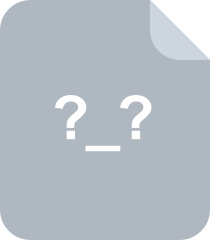
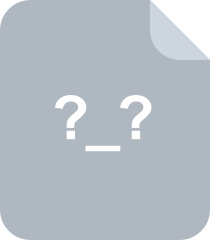
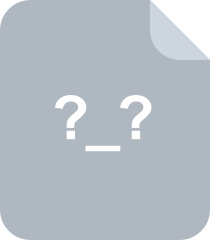
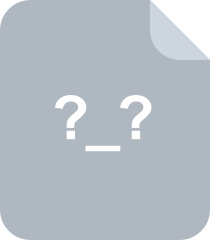
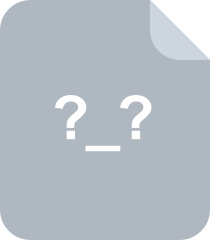
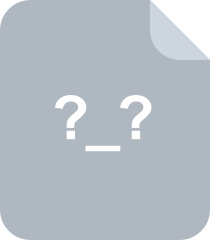
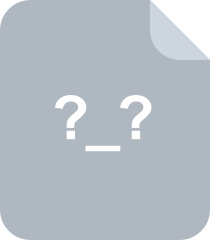
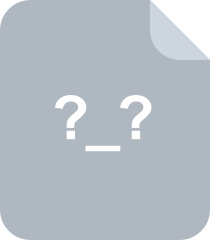
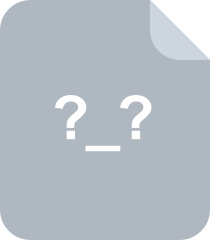
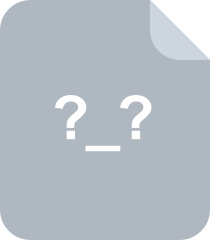

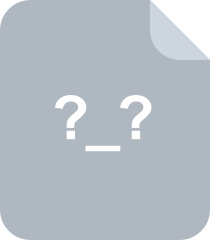
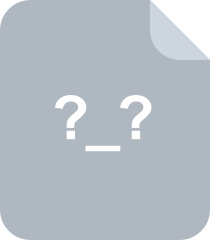
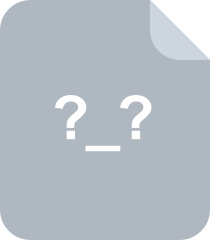
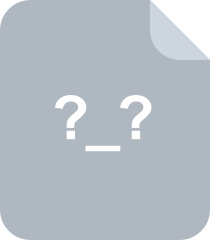
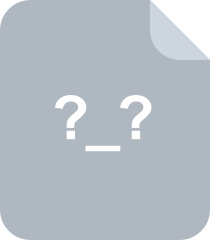
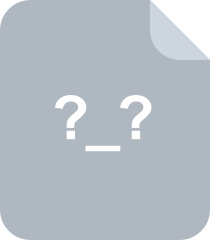
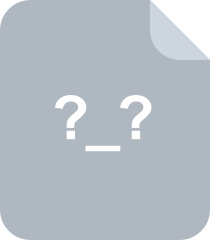
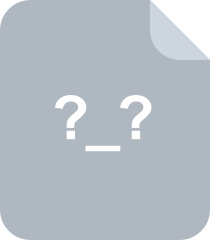
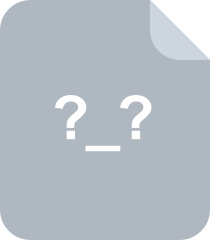
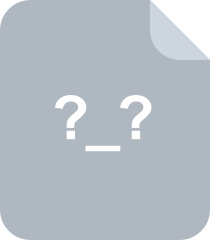
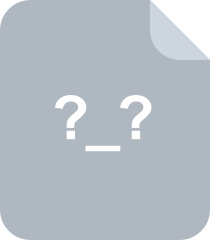
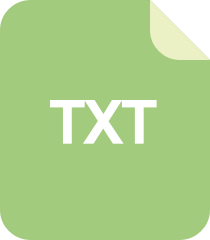
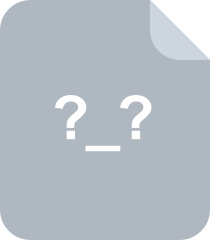
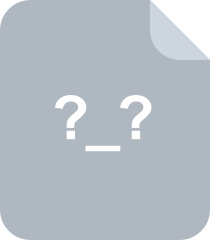
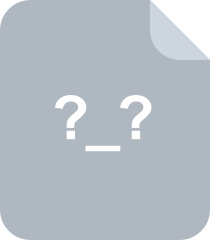
共 81 条
- 1
资源评论
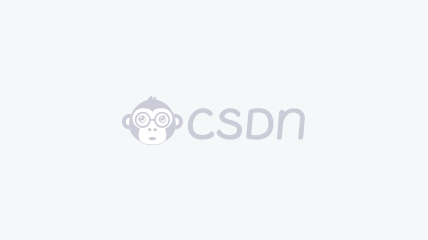
- 2301_774810552024-05-17发现一个宝藏资源,赶紧冲冲冲!支持大佬~
- 2301_763135602024-04-24资源使用价值高,内容详实,给了我很多新想法,感谢大佬分享~

猰貐的新时代
- 粉丝: 1w+
- 资源: 2886
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

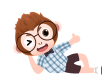
最新资源
- (33269446)全国省市县经纬度xml数据(全)
- ip地址查询城市php代码
- jieba分词自定义分词词表
- (6340824)C语言学生信息管理系统
- 床、自行车、瓶子、碗、公交车、食堂、小型车检测12-YOLO(v5至v11)、COCO、CreateML、Paligemma、TFRecord、VOC数据集合集.rar
- (6351410)c++经典程序200例
- (7276248)c语言图书管理系统
- (9368016)C++ STL使用
- (10377004)C语言下实现的学生管理系统
- (15341010)经典C程序一百例
- (174549194)ANSYS Fluent Tutorial Guide
- (175909636)全国293个地级市的经纬度信息
- 尚硅谷宋红康C语言精讲.zip
- 视图库级联抓包,支持GA/T1400-2018版,包括Register, keepalive, subscribe, subscribeNotification等
- ip地址查询区域代码包括php c++ python golang java rust代码使用例子
- C语言结构体精讲,结构体在内存中的访问
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


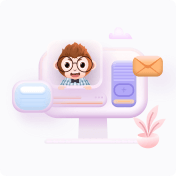
安全验证
文档复制为VIP权益,开通VIP直接复制
