CUDA_C_Programming_Guide.pdf
需积分: 0 62 浏览量
2021-07-11
11:20:17
上传
评论
收藏 2.86MB PDF 举报


chend926
- 粉丝: 1
- 资源: 11
最新资源
- HM2305B-VB一款P-Channel沟道SOT23的MOSFET晶体管参数介绍与应用说明
- 基于52单片机、ADC0832、LCD1602、两个74HC393和一个74HC08的频率测量计 不能用,请私我
- HM2302-VB一款N-Channel沟道SOT23的MOSFET晶体管参数介绍与应用说明
- python实战项目-学生成绩管理系统(基础版)
- 微信小程序源码 实现查公交 滴滴公交 app 源码下载
- HM2302E-VB一款N-Channel沟道SOT23的MOSFET晶体管参数介绍与应用说明
- 基于C#图片相似度比较,感知哈希算法
- VR开发的概要介绍与分析
- 自动驾驶定位系列教程七:点云畸变补偿.pdf
- HM2302D-VB一款N-Channel沟道SOT23的MOSFET晶体管参数介绍与应用说明
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


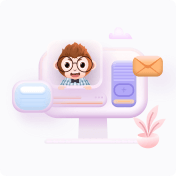