function varargout = mapMatch(varargin)
%*********************************************
%地图匹配的入门程序
%ma.yanbin@hotmail.com
%*********************************************
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @mapMatch_OpeningFcn, ...
'gui_OutputFcn', @mapMatch_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before mapMatch is made visible.
function mapMatch_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to mapMatch (see VARARGIN)
% Choose default command line output for mapMatch
handles.output = hObject;
% Update handles structure
guidata(hObject, handles);
% UIWAIT makes mapMatch wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% --- Outputs from this function are returned to the command line.
function varargout = mapMatch_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
% --- Executes on button press in loadMap.
function loadMap_Callback(hObject, eventdata, handles)
[fileName,pathName]=uigetfile('*.jpg','载入地图');
fullName=strcat(pathName,fileName);
mapData=imread(fullName);
handles.mapData=mapData;
guidata(hObject,handles);
h=mywaitbar(0.1, 'loading...', mapMatch,100,60);
for i=1:99
mywaitbar(i/100, h,['loaded ',num2str(i) '%']);
pause(0.1);
end
mywaitbar(1, h,'load success');
pause(0.2)
delete(h);
axes(handles.mapAxes);
imshow(mapData);
function h = mywaitbar(x, whichbar, varargin)
if ischar(whichbar) || iscellstr(whichbar) %调用格式为h = mywaitbar(p, 'title' , h_figure, x, y)
if nargin == 5
h_f = waitbar(x, whichbar, 'visible', 'off'); %创建一个临时进度条
h1 = findall(h_f, 'type', 'axes'); %查找进度条内的坐标轴
h_axs = copyobj(h1, varargin{1}); %将进度条内的坐标轴及其子对象拷贝到指定窗口内
delete(h_f);
pos = get(h_axs, 'position');
set(h_axs, 'position', [varargin{2}, varargin{3}, pos(3 : 4)])
end
elseif isnumeric(whichbar) %调用格式为mywaitbar(p, h)或mywaitbar(p, h, 'title')
h_axs = whichbar;
p = findobj(h_axs,'Type','patch');
set(p, 'XData', [0 100*x 100*x 0])
if nargin == 3 %调用格式为mywaitbar(p, h, 'title')
hTitle = get(h_axs, 'title');
set(hTitle, 'string', varargin{1});
end
end
if nargout == 1 %返回内嵌进度条的句柄
h = h_axs;
end
% --- Executes on button press in mapMatching.
function mapMatching_Callback(hObject, eventdata, handles)
image=handles.mapData;
image_2=im2bw(image,0.56);%进行阈值化处理 参数可改
image_d=double(image_2);
obj_row=55;%设定原始行位置 可改
obj_col=35;%设定原始列位置 可改
input_angle=30;%设定原始速度方向 可改
%-----------------------------------------
A=8;%A为分块大小 分为A*A块大小
[m,n]=size(image_d);
row=fix(m/A);%row为分块后的行数
col=fix(n/A);%col为分块后的列数
image_cell=mat2cell(image_d,ones(row,1)*A,ones(col,1)*A);
image_array=ones(A,A,row*col);
for k=1:row*col
image_array(:,:,k)=image_cell{k}(:,:);
end
%-----------------------------------------
temp_row=ceil((obj_row/A))+1;
temp_col=ceil((obj_col/A))+1;
number=(temp_col-1)*row+temp_row;%number为目标点所在的块的编号
%-----------------------------------------
near(1)=number-1-row;%获得邻近块编号
near(2)=number-1;
near(3)=number-1+row;
near(4)=number-row;
near(5)=number;
near(6)=number+row;
near(7)=number+1-row;
near(8)=number+1;
near(9)=number+1+row;
%-----------------------------------------
small=1000;
final_i=0;
fianl_j=0;
final_k=0;
flag=0;%标志是否找到匹配点
for k=1:9
for i=2:A-1
for j=2:A-1
if image_array(i,j,near(k))==0
temp_col_2=1+floor(near(k)/row);
temp_row_2=near(k)-(temp_col_2-1)*row;
temp_row_3=(temp_row_2-1)*A+i;
temp_col_3=(temp_col_2-1)*A+j;
dis=distance(temp_row_3,temp_col_3,obj_row,obj_col);
temp=image_array(:,:,near(k));
angle=find_near_node(temp,i,j,k,input_angle);
if deside(temp_col_3,temp_row_3,obj_col,obj_row)
all=dis*0.6+angle*0.4;
if all<=small
small=dis;
final_i=i;%最终匹配点
fianl_j=j;%最终匹配点
final_k=near(k);%最终匹配点
flag=1;
end
else
flag=0;
end
end
end
end
end
%-----------------------------------------
final_col=floor(final_k/row)+1;
final_row=final_k-(final_col-1)*row;
final_x=(final_row-1)*A+final_i;
final_y=(final_col-1)*A+fianl_j;
%-----------------------------------------
axes(handles.mapAxes);
hold on
plot(obj_col,obj_row,'o');
place_x_o=num2str(obj_row);
place_y_o=num2str(obj_col);
clc;
temp=['原始点位置为',place_x_o,'行',place_y_o,'列'];
disp(temp);
if flag==1
plot(final_y,final_x,'*')
place_x=num2str(final_x);
place_y=num2str(final_y);
temp=['找到匹配点位置为',place_x,'行',place_y,'列'];
disp(temp);
else
disp('附近没有可匹配的点,请尝试修改网格大小');
end

MCU学习笔记
- 粉丝: 492
- 资源: 6
最新资源
- wrapt-1.11.2-cp27-cp27m-win32.whl.rar
- wrapt-1.11.2-cp34-cp34m-win_amd64.whl.rar
- wrapt-1.12.1-cp36-cp36m-win_amd64.whl.rar
- wrapt-1.12.1-cp36-cp36m-win32.whl.rar
- wrapt-1.11.2-cp34-cp34m-win32.whl.rar
- wrapt-1.11.2-cp35-cp35m-win32.whl.rar
- wrapt-1.11.2-cp35-cp35m-win_amd64.whl.rar
- wrapt-1.14.0-cp37-cp37m-win_amd64.whl.rar
- wrapt-1.14.1-cp38-cp38-win_amd64.whl.rar
- wrapt-1.14.1-cp38-cp38-win32.whl.rar
- wrapt-1.14.0-cp37-cp37m-win32.whl.rar
- wrapt-1.14.1-cp39-cp39-win_amd64.whl.rar
- wrapt-1.14.1-cp39-cp39-win32.whl.rar
- wrapt-1.14.1-cp310-cp310-win_amd64.whl.rar
- wrapt-1.14.1-cp311-cp311-win_amd64.whl.rar
- wrapt-1.14.1-cp311-cp311-win32.whl.rar
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


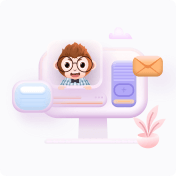