function varargout = Gui_Main(varargin)
% GUI_MAIN MATLAB code for Gui_Main.fig
% GUI_MAIN, by itself, creates a new GUI_MAIN or raises the existing
% singleton*.
%
% H = GUI_MAIN returns the handle to a new GUI_MAIN or the handle to
% the existing singleton*.
%
% GUI_MAIN('CALLBACK',hObject,eventData,handles,...) calls the local
% function named CALLBACK in GUI_MAIN.M with the given input arguments.
%
% GUI_MAIN('Property','Value',...) creates a new GUI_MAIN or raises the
% existing singleton*. Starting from the left, property value pairs are
% applied to the GUI before Gui_Main_OpeningFcn gets called. An
% unrecognized property name or invalid value makes property application
% stop. All inputs are passed to Gui_Main_OpeningFcn via varargin.
%
% *See GUI Options on GUIDE's Tools menu. Choose "GUI allows only one
% instance to run (singleton)".
%
% See also: GUIDE, GUIDATA, GUIHANDLES
% Edit the above text to modify the response to help Gui_Main
% Last Modified by GUIDE v2.5 27-Apr-2011 09:29:29
% Begin initialization code - DO NOT EDIT
gui_Singleton = 1;
gui_State = struct('gui_Name', mfilename, ...
'gui_Singleton', gui_Singleton, ...
'gui_OpeningFcn', @Gui_Main_OpeningFcn, ...
'gui_OutputFcn', @Gui_Main_OutputFcn, ...
'gui_LayoutFcn', [] , ...
'gui_Callback', []);
if nargin && ischar(varargin{1})
gui_State.gui_Callback = str2func(varargin{1});
end
if nargout
[varargout{1:nargout}] = gui_mainfcn(gui_State, varargin{:});
else
gui_mainfcn(gui_State, varargin{:});
end
% End initialization code - DO NOT EDIT
% --- Executes just before Gui_Main is made visible.
function Gui_Main_OpeningFcn(hObject, eventdata, handles, varargin)
% This function has no output args, see OutputFcn.
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% varargin command line arguments to Gui_Main (see VARARGIN)
% Choose default command line output for Gui_Main
clc; warning off all;
axes(handles.axes1); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes2); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes3); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes4); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
handles.output = hObject;
handles.file = [];
handles.MStitch = [];
handles.grayResult = [];
handles.RGBResult = [];
handles.grayListResult = [];
handles.RGBListResult = [];
% Update handles structure
guidata(hObject, handles);
% UIWAIT makes Gui_Main wait for user response (see UIRESUME)
% uiwait(handles.figure1);
% --- Outputs from this function are returned to the command line.
function varargout = Gui_Main_OutputFcn(hObject, eventdata, handles)
% varargout cell array for returning output args (see VARARGOUT);
% hObject handle to figure
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% Get default command line output from handles structure
varargout{1} = handles.output;
% --------------------------------------------------------------------
function uipushtool1_ClickedCallback(hObject, eventdata, handles)
% hObject handle to uipushtool1 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
[filename, pathname] = uiputfile({'*.jpg;*.tif;*.png;*.gif','All Image Files';...
'*.*','All Files' }, '保存结果', ...
'Result\result_gui.jpg');
if isempty(filename)
return;
end
file = fullfile(pathname, filename);
f = getframe(gcf);
f = frame2im(f);
imwrite(f, file);
msgbox('保存GUI结果图像成功!', '提示信息', 'modal');
% --------------------------------------------------------------------
function uipushtool2_ClickedCallback(hObject, eventdata, handles)
% hObject handle to uipushtool2 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
axes(handles.axes1); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes2); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes3); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes4); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
handles.file = [];
handles.MStitch = [];
handles.grayResult = [];
handles.RGBResult = [];
handles.grayListResult = [];
handles.RGBListResult = [];
[filename, pathname, filterindex] = uigetfile({'*.jpg;*.tif;*.png;*.gif;*.bmp','All Image Files';...
'*.*','All Files' }, '选择待处理图像', ...
'.\\images\\', 'MultiSelect', 'on');
if ~isa(filename, 'cell') && isequal(filename, 0)
return;
end
file = File_Process(filename, pathname);
if length(file) < 2
msgbox('请选择至少两幅图像!', '提示信息', 'modal');
return;
end
Img1 = imread(file{1});
Img2 = ImageList(file);
axes(handles.axes1);
imshow(Img1); title('图像序列1', 'FontWeight', 'Bold');
axes(handles.axes2);
imshow(Img2); title('图像序列2', 'FontWeight', 'Bold');
handles.Img1 = Img1;
handles.Img2 = Img2;
handles.file = file;
guidata(hObject, handles);
% --- Executes on button press in pushbutton1.
function pushbutton1_Callback(hObject, eventdata, handles)
% hObject handle to pushbutton1 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
axes(handles.axes1); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes2); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes3); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
axes(handles.axes4); cla reset; box on; set(gca, 'XTickLabel', [], 'YTickLabel', []);
handles.file = [];
handles.MStitch = [];
handles.grayResult = [];
handles.RGBResult = [];
dname = uigetdir('.\\images\\风景图像', '请选择待处理图像文件夹:');
if dname == 0
return;
end
df = ls(dname);
if length(df) > 2
for i = 1 : size(df, 1)
if strfind(df(i, :), '.db');
df(i, :) = [];
break;
end
end
if length(df) > 2
filename = fullfile(dname, df(end, :));
pathname = [dname '\'];
else
msgbox('请选择至少两幅图像!', '提示信息', 'modal');
return;
end
else
msgbox('请选择至少两幅图像!', '提示信息', 'modal');
return;
end
file = File_Process(filename, pathname);
if length(file) < 2
msgbox('请选择至少两幅图像!', '提示信息', 'modal');
return;
end
Img1 = imread(file{1});
Img2 = ImageList(file);
axes(handles.axes1);
imshow(Img1); title('图像序列1', 'FontWeight', 'Bold');
axes(handles.axes2);
imshow(Img2); title('图像序列2', 'FontWeight', 'Bold');
handles.Img1 = Img1;
handles.Img2 = Img2;
handles.file = file;
guidata(hObject, handles);
% --- If Enable == 'on', executes on mouse press in 5 pixel border.
% --- Otherwise, executes on mouse press in 5 pixel border or over pushbutton1.
function pushbutton1_ButtonDownFcn(hObject, eventdata, handles)
% hObject handle to pushbutton1 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% --- Executes on mouse press over axes background.
function axes1_ButtonDownFcn(hObject, eventdata, handles)
% hObject handle to axes1 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with handles and user data (see GUIDATA)
% --- Executes on mouse press over axes background.
function axes2_ButtonDownFcn(hObject, eventdata, handles)
% hObject handle to axes2 (see GCBO)
% eventdata reserved - to be defined in a future version of MATLAB
% handles structure with h


fpga和matlab
- 粉丝: 17w+
- 资源: 2638
最新资源
- 06-【培训手册】05-新员工入职培训手册.docx
- 07-【培训考试】01-新入职员工培训考试试题.docx
- 07-【培训考试】03-新员工培训考试试题.docx
- 08-【考核管理】04-新员工培训考核方案.docx
- 08-【考核管理】01-新员工培训和考核管理制度.doc.docx
- 08-【考核管理】07-新员工培训考核表.doc.docx
- 08-【考核管理】10-新员工培训评估表.doc.docx
- 11-【其他】04-新员工岗前培训试题.docx
- 09-【确认书】01-新员工入职培训确认书.docx
- 11-【其他】01-新员工入职培训引导表.docx
- 11-【其他】08-新员工入职培训考试试卷.docx
- 11-【其他】07-新员工入职培训跟踪表.docx
- 中职学校《计算机应用基础》课程标准及教学指导(2024年版)
- 【培训实施】-02-培训计划实施方案.docx
- 【培训实施】-01-公司年度培训实施方案.docx.doc
- 【培训管理】员工培训实施制度.docx
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


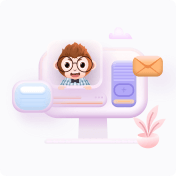