package com.example.demo;
import org.geotools.data.*;
import org.geotools.data.collection.ListFeatureCollection;
import org.geotools.data.simple.SimpleFeatureCollection;
import org.geotools.data.simple.SimpleFeatureSource;
import org.geotools.data.simple.SimpleFeatureStore;
import org.geotools.factory.CommonFactoryFinder;
import org.geotools.feature.simple.SimpleFeatureBuilder;
import org.geotools.util.factory.GeoTools;
import org.locationtech.jts.geom.Coordinate;
import org.locationtech.jts.geom.GeometryFactory;
import org.locationtech.jts.geom.LineString;
import org.locationtech.jts.geom.MultiLineString;
import org.locationtech.jts.geom.MultiPolygon;
import org.locationtech.jts.geom.Point;
import org.locationtech.jts.geom.Polygon;
import org.opengis.feature.simple.SimpleFeature;
import org.opengis.feature.simple.SimpleFeatureType;
import org.opengis.filter.Filter;
import org.opengis.filter.FilterFactory;
import org.opengis.filter.identity.FeatureId;
import java.io.IOException;
import java.util.*;
import java.util.List;
public class PostGISDataStore {
public static void main(String[] args) throws Exception {
DataStore dataStore=getDataStore();
String tableName="ddf2";
Double x=116.181123075;
Double y=33.2329145104;
insertPoint(dataStore,tableName,x,y);
// removePoint(dataStore,tableName,"34");
// updatePoint(dataStore, tableName, x, y, "30");
// SimpleFeatureCollection querydataCollection=queryFeatures(dataStore,tableName);
// System.out.println(querydataCollection.getID());
// String tableName="lixian2";
// GeometryFactory geometryFactory =new GeometryFactory();
// double xy[][]= {{116.17952546,33.23436815},{116.18216085,33.23460989},{116.18216085,33.23460035},{116.17952546,33.23436815}};
// LineString[] lines = new LineString[1];
// Point point = geometryFactory.createPoint(new Coordinate(xy[0][0],xy[0][1]));
// Point point2 = geometryFactory.createPoint(new Coordinate(xy[1][0],xy[1][1]));
// Point point3 = geometryFactory.createPoint(new Coordinate(xy[2][0],xy[2][1]));
// lines[0] =geometryFactory.createLineString(new Coordinate[] {new Coordinate(xy[0][0], xy[0][1]), new Coordinate(xy[1][0], xy[1][1]),new Coordinate(xy[2][0], xy[2][1])});
// insertLine(dataStore,tableName,lines);
// updateLine(dataStore,tableName,lines,"20");
// removeLine(dataStore, tableName, "23");
// Polygon[] pp=new Polygon[1];
// String tableNameString="mianys2";
// pp[0]=geometryFactory.createPolygon((new Coordinate[] {new Coordinate(xy[0][0], xy[0][1]), new Coordinate(xy[1][0], xy[1][1]),new Coordinate(xy[2][0], xy[2][1]),new Coordinate(xy[3][0], xy[3][1])}));
// insertMultiPolygon(dataStore,tableNameString,pp);
// updateMultiPolygon(dataStore,tableNameString,pp,"3");
// removeMultiPolygon(dataStore, tableNameString, "3");
dataStore.dispose();
}
public static DataStore getDataStore() throws IOException {
Map<String, Object> params = new HashMap<>();
params.put("dbtype", "postgis");
params.put("host", "192.168.102.33");
params.put("port", 5432);
params.put("schema", "public");
params.put("database", "sample");
params.put("user", "postgres");
params.put("passwd", "sinoso");
DataStore dataStore = DataStoreFinder.getDataStore(params);
return dataStore;
}
public static void insertMultiPolygon(DataStore dataStore,String tableName,Polygon[] pp) throws IOException {
SimpleFeatureSource simpleFeatureSource = null;
MultiPolygon polygonString = null;
//构建要素
SimpleFeature feature = null;
if (dataStore != null) {
GeometryFactory geometryFactory=new GeometryFactory();
simpleFeatureSource = dataStore.getFeatureSource(tableName);
SimpleFeatureType type = simpleFeatureSource.getSchema();
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(type);
polygonString = geometryFactory.createMultiPolygon(pp);
List<Object> resultList = new ArrayList<>();
resultList.add(0);
resultList.add(polygonString);
featureBuilder.addAll(resultList);
feature = featureBuilder.buildFeature("polygon");
List<SimpleFeature> features = new ArrayList<>();
features.add(feature);
if( simpleFeatureSource instanceof SimpleFeatureStore){
SimpleFeatureStore featureStore = (SimpleFeatureStore) simpleFeatureSource;
SimpleFeatureCollection featureCollection = new ListFeatureCollection(type,features);
//创建事务
Transaction session = new DefaultTransaction("Adding");
featureStore.setTransaction( session );
try {
List<FeatureId> added = featureStore.addFeatures( featureCollection );
System.out.println( "Added "+added );
//提交事务
session.commit();
}
catch (Throwable t){
System.out.println( "Failed to add features: "+t );
try {
//事务回归
session.rollback();
} catch (IOException e) {
e.printStackTrace();
}finally {
session.close();
}
}
}
} else {
throw new IOException("数据库连接未成功");
}
}
public static void insertLine(DataStore dataStore,String tableName,LineString[] pp) throws IOException {
SimpleFeatureSource simpleFeatureSource = null;
MultiLineString lineString = null;
//构建要素
SimpleFeature feature = null;
if (dataStore != null) {
GeometryFactory geometryFactory=new GeometryFactory();
simpleFeatureSource = dataStore.getFeatureSource(tableName);
SimpleFeatureType type = simpleFeatureSource.getSchema();
SimpleFeatureBuilder featureBuilder = new SimpleFeatureBuilder(type);
lineString = geometryFactory.createMultiLineString(pp);
List<Object> resultList = new ArrayList<>();
resultList.add(0);
resultList.add(null);
resultList.add(null);
resultList.add(null);
resultList.add(null);
resultList.add(lineString);
featureBuilder.addAll(resultList);
feature = featureBuilder.buildFeature("line");
List<SimpleFeature> features = new ArrayList<>();
features.add(feature);
if( simpleFeatureSource instanceof SimpleFeatureStore){
SimpleFeatureStore featureStore = (SimpleFeatureStore) simpleFeatureSource;
SimpleFeatureCollection featureCollection = new ListFeatureCollection(type,features);
//创建事务
Transaction session = new DefaultTransaction("Adding");
featureStore.setTransaction( session );
try {
List<FeatureId> added = featureStore.addFeatures( featureCollection );
System.out.println( "Added "+added );
//提交事务
session.commit();
}
catch (Throwable t){
System.out.println( "Failed to add features: "+t );
try {
//事务回归
session.rollback();
} catch (IOException e) {
e.printStackTrace();
}finally {
session.close();
}
}
}
} else {
throw new IOException("数据库连接未成功");
}
}
public static void insertPoint(DataStore dataStore,String tableName,Doub
没有合适的资源?快使用搜索试试~ 我知道了~
资源推荐
资源详情
资源评论
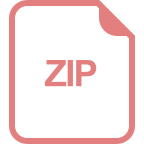
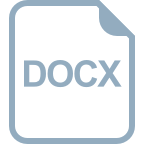
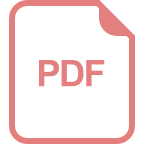
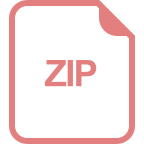
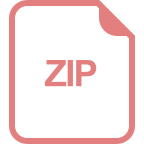
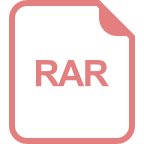
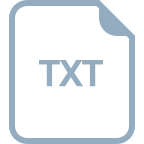
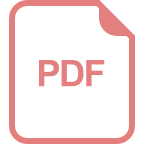
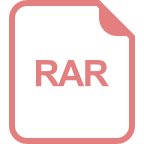
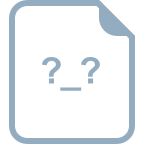
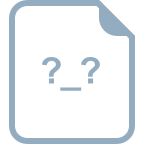
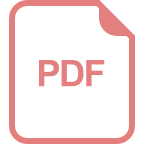
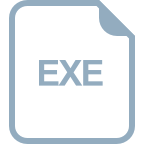
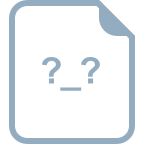
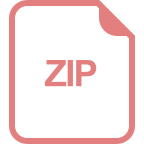
收起资源包目录

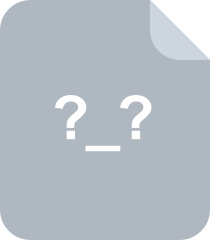
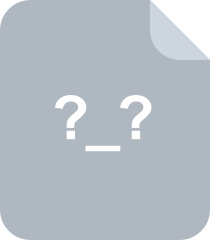
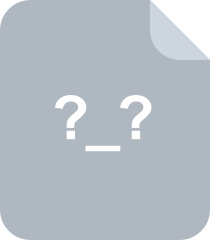
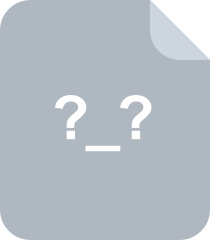
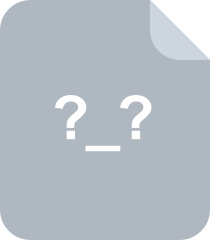
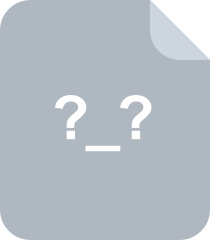
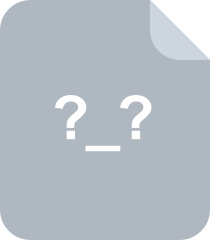
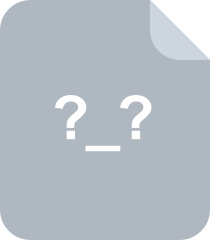
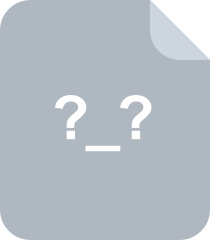
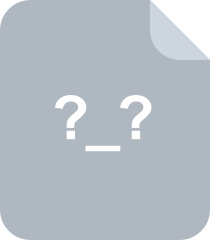
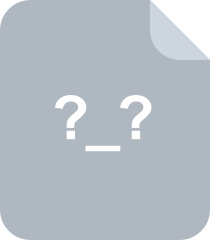
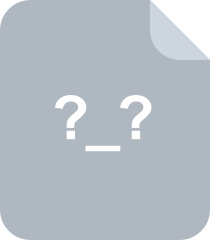
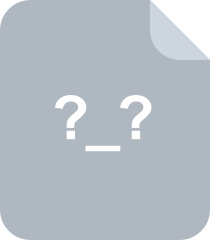
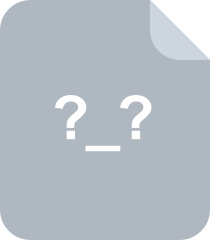
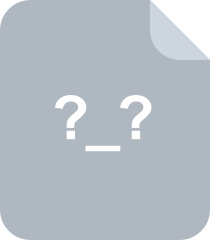
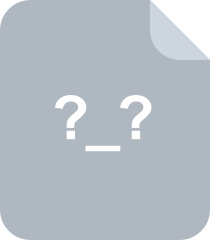
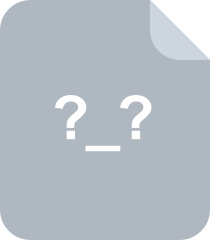
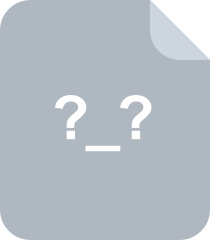
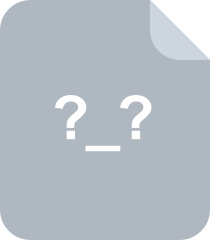
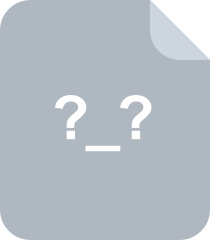
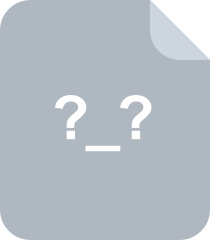
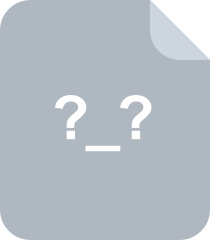
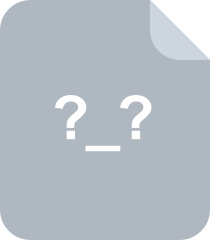
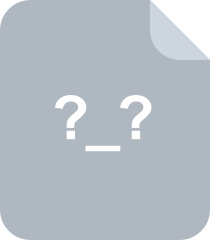
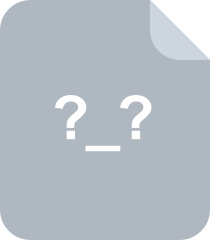
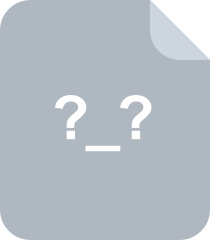
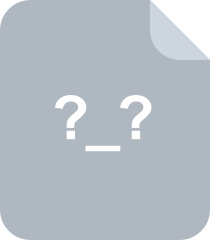
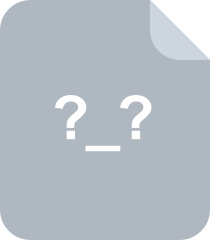
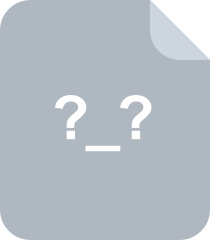
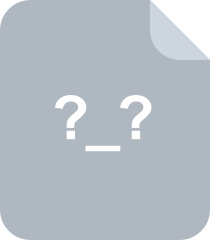
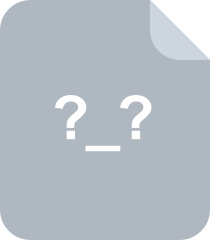
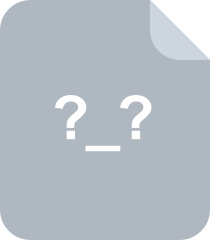
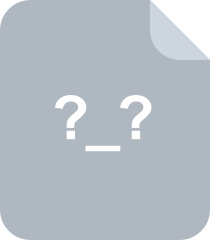
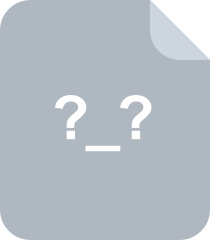
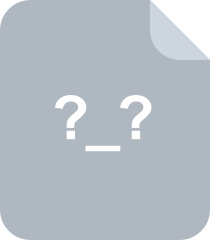
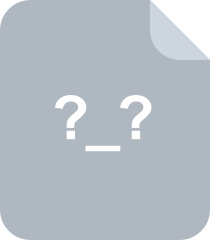
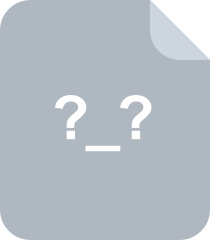
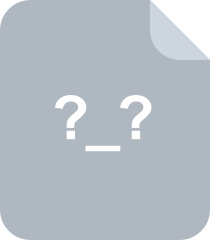
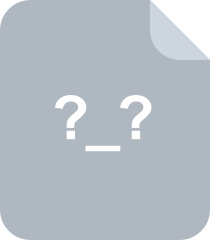
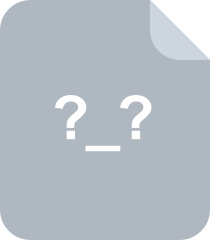
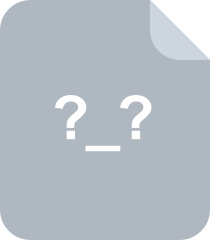
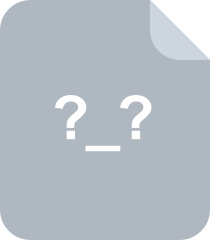
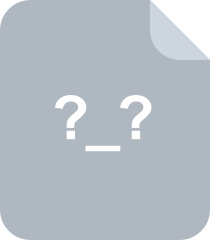
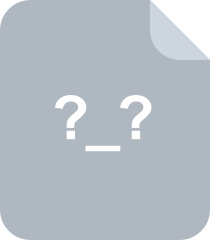
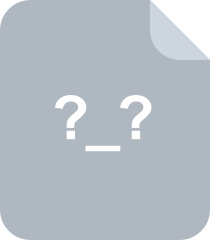
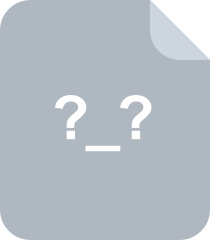
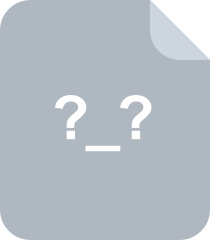
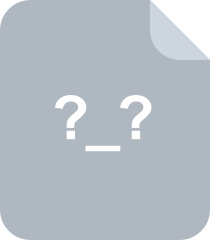
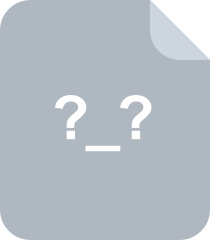
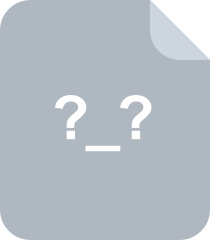
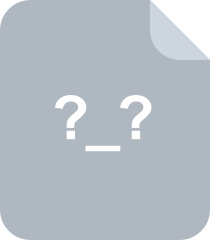
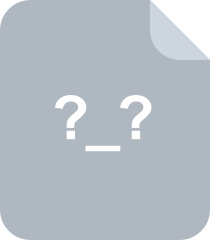
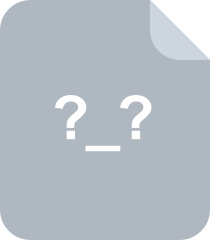
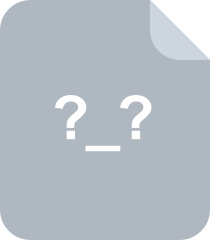
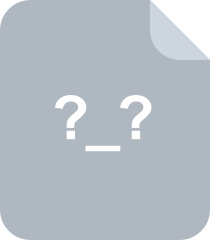
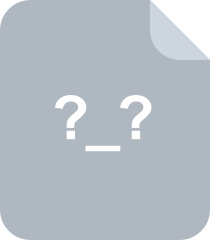
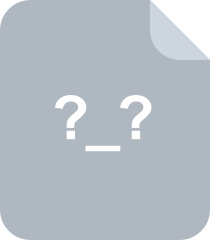
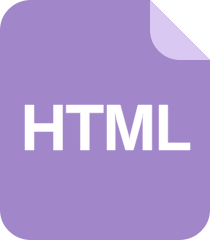
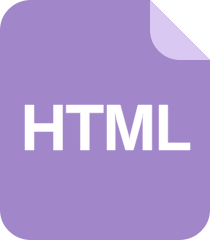
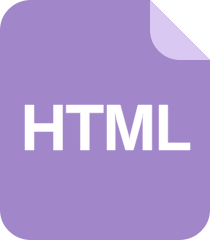
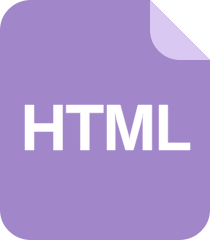
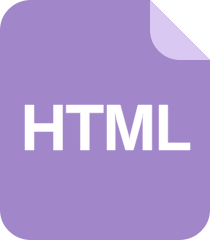
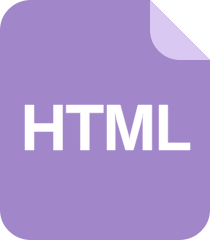
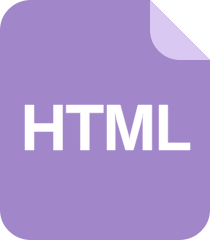
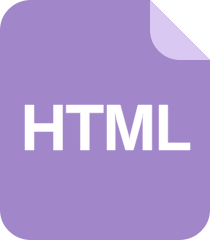
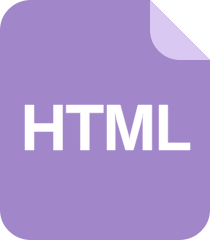
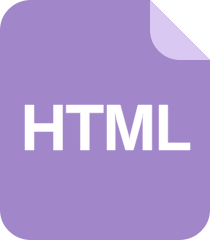
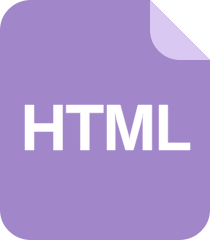
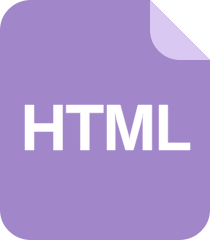
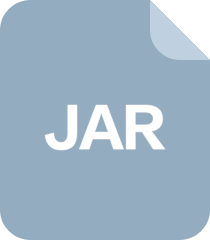
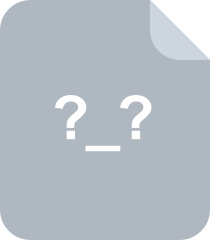
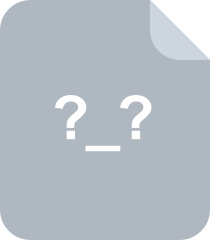
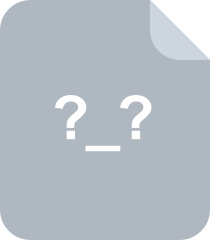
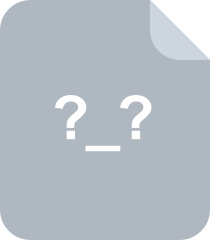
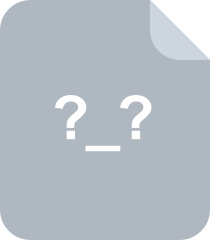
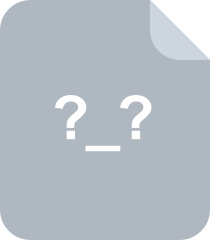
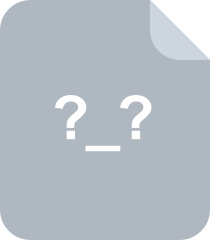
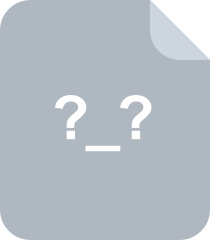
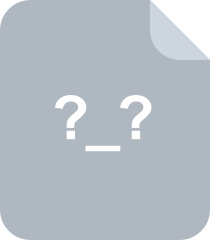
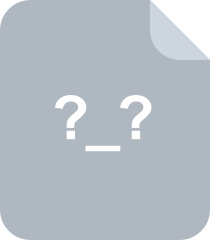
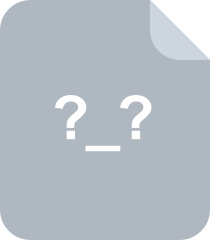
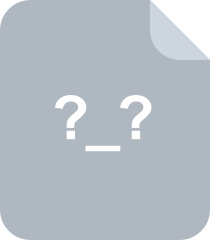
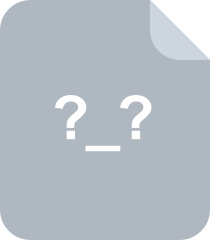
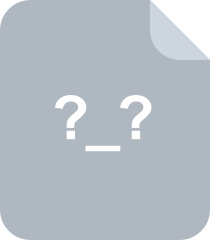
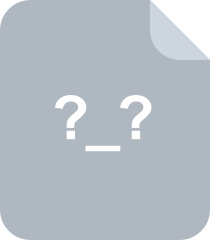
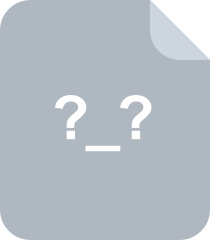
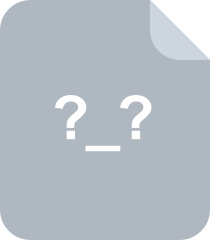
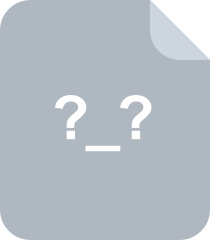
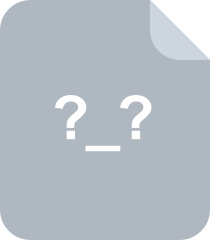
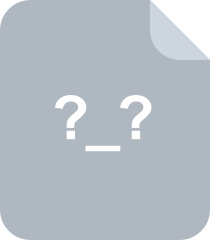
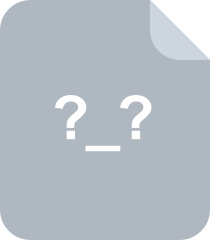
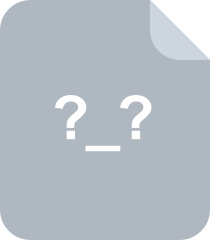
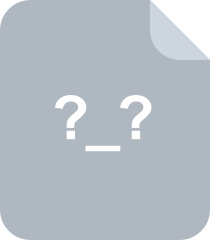
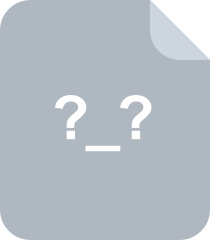
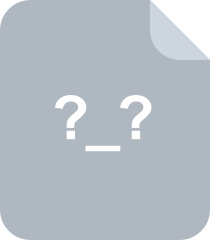
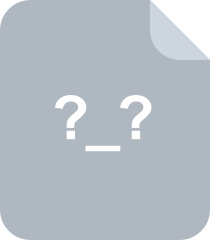
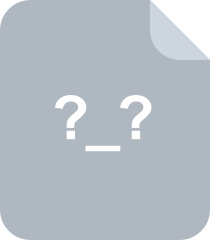
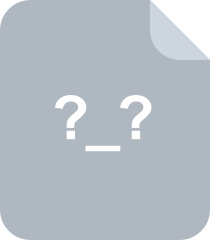
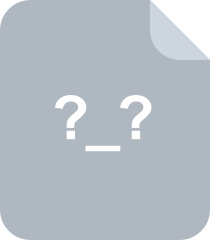
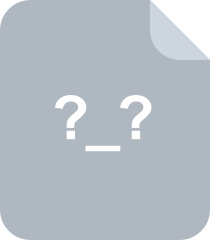
共 162 条
- 1
- 2
资源评论
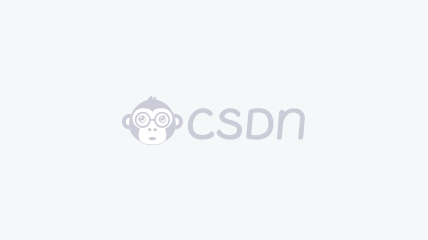

曹小象
- 粉丝: 1
- 资源: 2
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

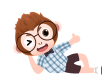
最新资源
- xyctf:从入门到精通的实用指南.zip
- mmqrcode1714153659780.png
- Screenshot_2024-04-27-06-08-58-486_com.baidu.xin.aiqicha.jpg
- 基于Javaweb+Tomcat+MySQL的大学生公寓管理系统+sql文件.zip
- 实训作业基于javaweb的订单管理系统源码+数据库+实训报告.zip
- 多机调度问题贪心算法基于最小堆和贪心算法求解多机调度问题.zip
- 基于同态加密技术的匿名电子投票系统源码.zip
- Pyqt5项目框架-PyQt项目开发实践
- 基于C通过MQTT的智能农业大棚管理系统(本科毕业设计)
- python+CNN的网络入侵检测算法源码.zip
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


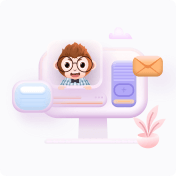
安全验证
文档复制为VIP权益,开通VIP直接复制
