c#自定义透明按钮控件
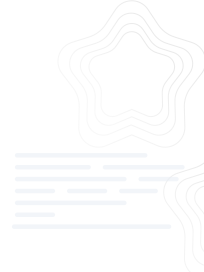


在C#编程中,自定义控件是一种常见的需求,它允许开发者根据项目需求创建具有特定功能或视觉效果的用户界面元素。"c#自定义透明按钮控件"就是这样一个实例,它展示了如何利用C#语言和.NET Framework或.NET Core来扩展系统默认的Button控件,以实现透明的效果。下面我们将深入探讨这个主题。 自定义控件的创建通常涉及继承已有的控件类,并在其基础上添加新的属性、方法和事件。在C#中,我们可以从System.Windows.Forms.Button类派生,然后重写或扩展其功能。例如: ```csharp using System.Drawing; using System.Windows.Forms; public class CustomTransparentButton : Button { public CustomTransparentButton() { this.SetStyle(ControlStyles.SupportsTransparentBackColor, true); this.BackColor = Color.Transparent; } protected override void OnPaint(PaintEventArgs e) { base.OnPaint(e); // 在这里可以自定义绘制按钮的外观,例如添加边框、文字等 } } ``` 在这个例子中,我们设置了控件支持透明背景(`SetStyle(ControlStyles.SupportsTransparentBackColor, true)`),并将背景颜色设为透明(`BackColor = Color.Transparent`)。为了实现透明效果,还需要处理控件的OnPaint事件,以自定义绘制按钮的外观。 透明按钮的设计可能包括绘制按钮的文字、图标以及边框。在`OnPaint`方法中,可以使用Graphics对象(`e.Graphics`)进行绘制。例如,使用`DrawString`方法绘制文本,使用`DrawRectangle`或`DrawPolygon`绘制边框。 ```csharp protected override void OnPaint(PaintEventArgs e) { base.OnPaint(e); // 绘制边框 Pen borderPen = new Pen(Color.Black, 2f); e.Graphics.DrawRectangle(borderPen, 0, 0, this.Width - 1, this.Height - 1); // 绘制文本 SolidBrush textBrush = new SolidBrush(Color.White); StringFormat sf = new StringFormat(); sf.Alignment = StringAlignment.Center; sf.LineAlignment = StringAlignment.Center; e.Graphics.DrawString(this.Text, this.Font, textBrush, new RectangleF(0, 0, this.Width, this.Height), sf); // 清理资源 borderPen.Dispose(); textBrush.Dispose(); } ``` 此外,为了实现点击效果,可能还需要处理鼠标事件,如`MouseEnter`, `MouseLeave`, `MouseDown`和`MouseUp`,并根据这些事件改变按钮的外观。例如,当鼠标进入按钮时,可以改变边框颜色使其看起来被高亮。 ```csharp private bool isMouseOver = false; protected override void OnMouseEnter(EventArgs e) { base.OnMouseEnter(e); isMouseOver = true; this.Invalidate(); } protected override void OnMouseLeave(EventArgs e) { base.OnMouseLeave(e); isMouseOver = false; this.Invalidate(); } protected override void OnMouseDown(MouseEventArgs e) { base.OnMouseDown(e); this.Invalidate(); } protected override void OnMouseUp(MouseEventArgs e) { base.OnMouseUp(e); this.Invalidate(); } protected override void OnPaint(PaintEventArgs e) { // ... if (isMouseOver) { borderPen.Color = Color.Gray; // 鼠标悬停时的边框颜色 } else { borderPen.Color = Color.Black; // 默认边框颜色 } // ... } ``` `thirdbuttons`可能是这个项目的源代码文件夹,包含其他可能的按钮样式或扩展功能。例如,可能会有多个类文件,每个类代表一种不同样式的透明按钮,或者包含一个专门处理按钮集合的容器控件。 总结起来,"c#自定义透明按钮控件"是C#编程中的一个实践案例,它展示了如何通过继承和自定义绘制来扩展系统控件,以满足特定设计或交互需求。通过这种方式,开发者可以创建出既美观又符合应用风格的用户界面。
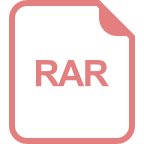
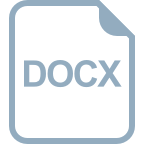
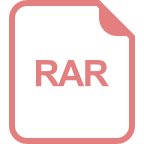
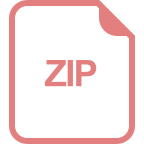
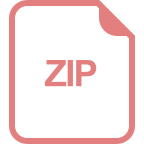
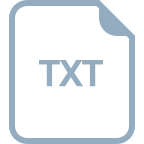
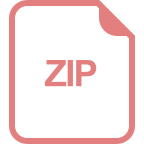
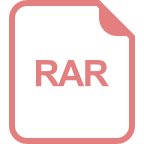
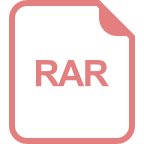
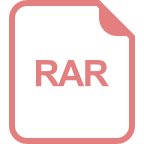
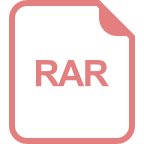



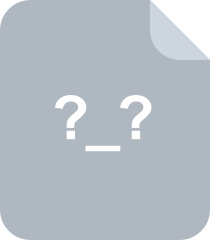


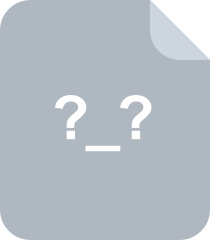
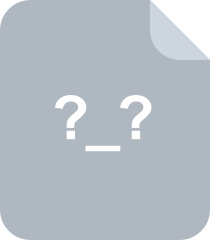
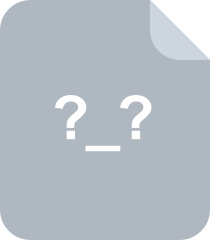



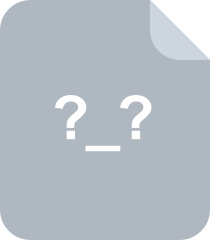
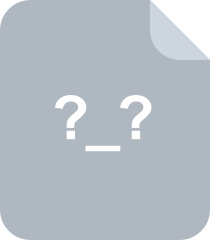

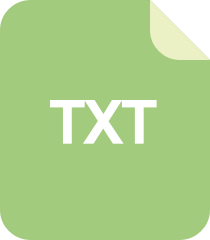

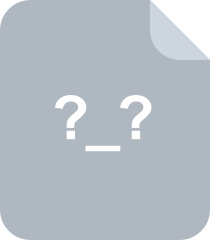
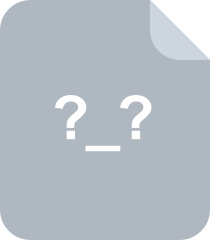
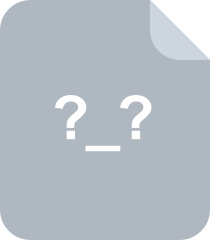
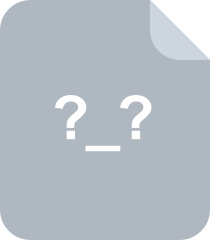
- 1
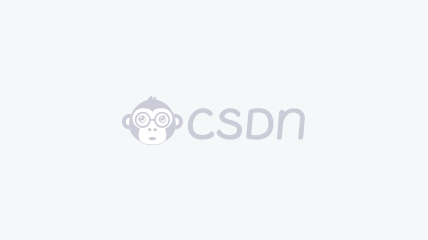
- 范晓天2012-10-21满足不了需求,背景是可变的flash或者视频文件的时候达不到透明效果
- songguanjun2014-01-05但是感觉外观一般
- 七号神人2015-06-13感觉外观一般,但是还可以参考参考
- 孙宏凌2013-10-11按钮可用, 还是感谢分享。
- coolba20462012-06-20按钮可用,但是感觉外观一般,还是感谢分享。

- 粉丝: 0
- 资源: 43
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

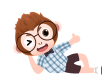
最新资源

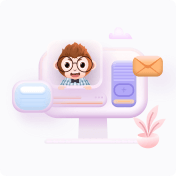
