==================================================
Building and Distributing Packages with Setuptools
==================================================
``Setuptools`` is a collection of enhancements to the Python ``distutils``
(for Python 2.6 and up) that allow developers to more easily build and
distribute Python packages, especially ones that have dependencies on other
packages.
Packages built and distributed using ``setuptools`` look to the user like
ordinary Python packages based on the ``distutils``. Your users don't need to
install or even know about setuptools in order to use them, and you don't
have to include the entire setuptools package in your distributions. By
including just a single `bootstrap module`_ (a 12K .py file), your package will
automatically download and install ``setuptools`` if the user is building your
package from source and doesn't have a suitable version already installed.
.. _bootstrap module: https://bootstrap.pypa.io/ez_setup.py
Feature Highlights:
* Automatically find/download/install/upgrade dependencies at build time using
the `EasyInstall tool <easy_install.html>`_,
which supports downloading via HTTP, FTP, Subversion, and SourceForge, and
automatically scans web pages linked from PyPI to find download links. (It's
the closest thing to CPAN currently available for Python.)
* Create `Python Eggs <http://peak.telecommunity.com/DevCenter/PythonEggs>`_ -
a single-file importable distribution format
* Enhanced support for accessing data files hosted in zipped packages.
* Automatically include all packages in your source tree, without listing them
individually in setup.py
* Automatically include all relevant files in your source distributions,
without needing to create a ``MANIFEST.in`` file, and without having to force
regeneration of the ``MANIFEST`` file when your source tree changes.
* Automatically generate wrapper scripts or Windows (console and GUI) .exe
files for any number of "main" functions in your project. (Note: this is not
a py2exe replacement; the .exe files rely on the local Python installation.)
* Transparent Pyrex support, so that your setup.py can list ``.pyx`` files and
still work even when the end-user doesn't have Pyrex installed (as long as
you include the Pyrex-generated C in your source distribution)
* Command aliases - create project-specific, per-user, or site-wide shortcut
names for commonly used commands and options
* PyPI upload support - upload your source distributions and eggs to PyPI
* Deploy your project in "development mode", such that it's available on
``sys.path``, yet can still be edited directly from its source checkout.
* Easily extend the distutils with new commands or ``setup()`` arguments, and
distribute/reuse your extensions for multiple projects, without copying code.
* Create extensible applications and frameworks that automatically discover
extensions, using simple "entry points" declared in a project's setup script.
.. contents:: **Table of Contents**
.. _ez_setup.py: `bootstrap module`_
-----------------
Developer's Guide
-----------------
Installing ``setuptools``
=========================
Please follow the `EasyInstall Installation Instructions`_ to install the
current stable version of setuptools. In particular, be sure to read the
section on `Custom Installation Locations`_ if you are installing anywhere
other than Python's ``site-packages`` directory.
.. _EasyInstall Installation Instructions: easy_install.html#installation-instructions
.. _Custom Installation Locations: easy_install.html#custom-installation-locations
If you want the current in-development version of setuptools, you should first
install a stable version, and then run::
ez_setup.py setuptools==dev
This will download and install the latest development (i.e. unstable) version
of setuptools from the Python Subversion sandbox.
Basic Use
=========
For basic use of setuptools, just import things from setuptools instead of
the distutils. Here's a minimal setup script using setuptools::
from setuptools import setup, find_packages
setup(
name="HelloWorld",
version="0.1",
packages=find_packages(),
)
As you can see, it doesn't take much to use setuptools in a project.
Run that script in your project folder, alongside the Python packages
you have developed.
Invoke that script to produce eggs, upload to
PyPI, and automatically include all packages in the directory where the
setup.py lives. See the `Command Reference`_ section below to see what
commands you can give to this setup script. For example,
to produce a source distribution, simply invoke::
python setup.py sdist
Of course, before you release your project to PyPI, you'll want to add a bit
more information to your setup script to help people find or learn about your
project. And maybe your project will have grown by then to include a few
dependencies, and perhaps some data files and scripts::
from setuptools import setup, find_packages
setup(
name="HelloWorld",
version="0.1",
packages=find_packages(),
scripts=['say_hello.py'],
# Project uses reStructuredText, so ensure that the docutils get
# installed or upgraded on the target machine
install_requires=['docutils>=0.3'],
package_data={
# If any package contains *.txt or *.rst files, include them:
'': ['*.txt', '*.rst'],
# And include any *.msg files found in the 'hello' package, too:
'hello': ['*.msg'],
},
# metadata for upload to PyPI
author="Me",
author_email="me@example.com",
description="This is an Example Package",
license="PSF",
keywords="hello world example examples",
url="http://example.com/HelloWorld/", # project home page, if any
# could also include long_description, download_url, classifiers, etc.
)
In the sections that follow, we'll explain what most of these ``setup()``
arguments do (except for the metadata ones), and the various ways you might use
them in your own project(s).
Specifying Your Project's Version
---------------------------------
Setuptools can work well with most versioning schemes; there are, however, a
few special things to watch out for, in order to ensure that setuptools and
EasyInstall can always tell what version of your package is newer than another
version. Knowing these things will also help you correctly specify what
versions of other projects your project depends on.
A version consists of an alternating series of release numbers and pre-release
or post-release tags. A release number is a series of digits punctuated by
dots, such as ``2.4`` or ``0.5``. Each series of digits is treated
numerically, so releases ``2.1`` and ``2.1.0`` are different ways to spell the
same release number, denoting the first subrelease of release 2. But ``2.10``
is the *tenth* subrelease of release 2, and so is a different and newer release
from ``2.1`` or ``2.1.0``. Leading zeros within a series of digits are also
ignored, so ``2.01`` is the same as ``2.1``, and different from ``2.0.1``.
Following a release number, you can have either a pre-release or post-release
tag. Pre-release tags make a version be considered *older* than the version
they are appended to. So, revision ``2.4`` is *newer* than revision ``2.4c1``,
which in turn is newer than ``2.4b1`` or ``2.4a1``. Postrelease tags make
a version be considered *newer* than the version they are appended to. So,
revisions like ``2.4-1`` and ``2.4pl3`` are newer than ``2.4``, but are *older*
than ``2.4.1`` (which has a higher release number).
A pre-release tag is a series of letters that are alphabetically before
"final". Some examples of prerelease tags would include ``alpha``, ``beta``,
``a``, ``c``, ``dev``, and so on. You do not have to place a dot or dash
before the prerelease tag if it's immediately
没有合适的资源?快使用搜索试试~ 我知道了~
setuptools-34.1.0.zip
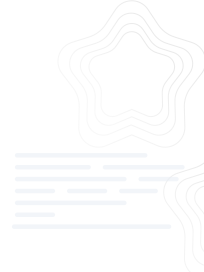
共144个文件
py:98个
txt:18个
exe:6个

0 下载量 184 浏览量
2024-05-14
23:05:34
上传
评论
收藏 607KB ZIP 举报
温馨提示
Node.js,简称Node,是一个开源且跨平台的JavaScript运行时环境,它允许在浏览器外运行JavaScript代码。Node.js于2009年由Ryan Dahl创立,旨在创建高性能的Web服务器和网络应用程序。它基于Google Chrome的V8 JavaScript引擎,可以在Windows、Linux、Unix、Mac OS X等操作系统上运行。 Node.js的特点之一是事件驱动和非阻塞I/O模型,这使得它非常适合处理大量并发连接,从而在构建实时应用程序如在线游戏、聊天应用以及实时通讯服务时表现卓越。此外,Node.js使用了模块化的架构,通过npm(Node package manager,Node包管理器),社区成员可以共享和复用代码,极大地促进了Node.js生态系统的发展和扩张。 Node.js不仅用于服务器端开发。随着技术的发展,它也被用于构建工具链、开发桌面应用程序、物联网设备等。Node.js能够处理文件系统、操作数据库、处理网络请求等,因此,开发者可以用JavaScript编写全栈应用程序,这一点大大提高了开发效率和便捷性。 在实践中,许多大型企业和组织已经采用Node.js作为其Web应用程序的开发平台,如Netflix、PayPal和Walmart等。它们利用Node.js提高了应用性能,简化了开发流程,并且能更快地响应市场需求。
资源推荐
资源详情
资源评论
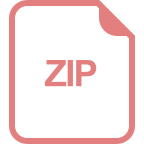
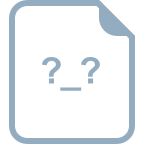
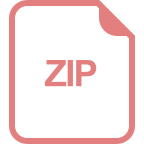
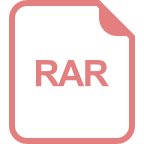
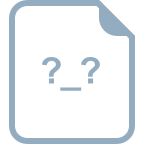
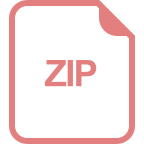
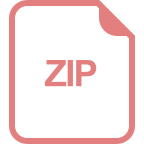
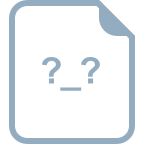
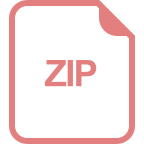
收起资源包目录

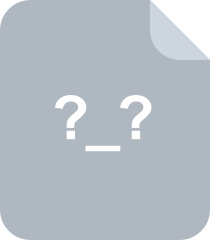
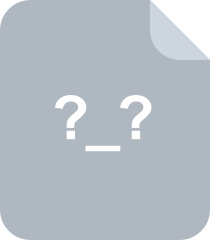
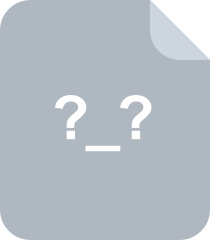
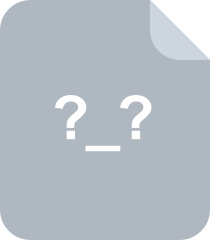
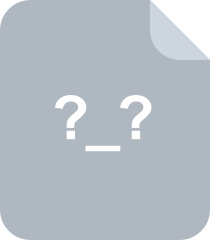
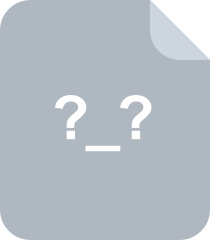
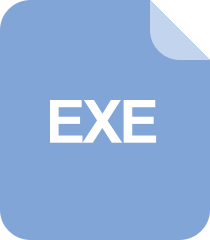
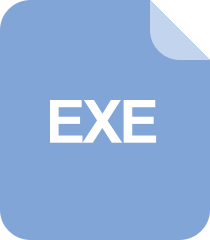
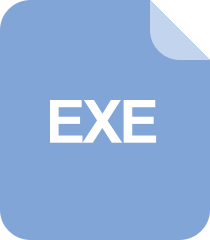
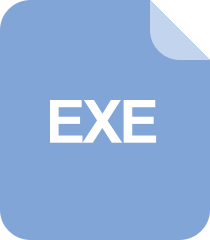
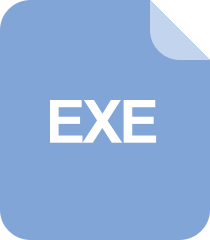
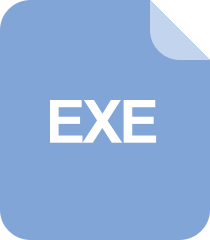
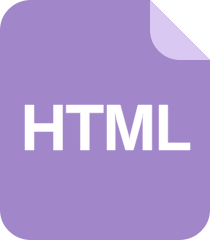
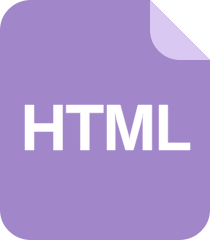
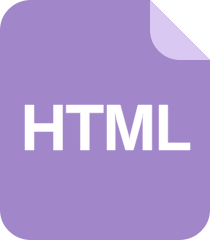
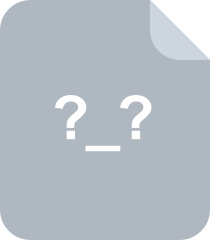
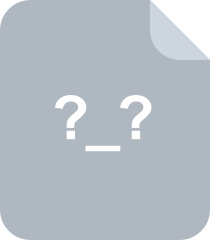
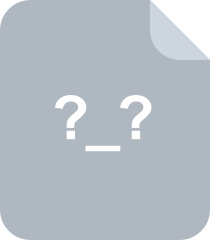
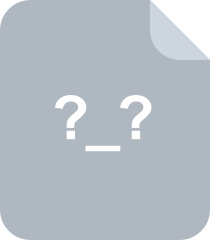
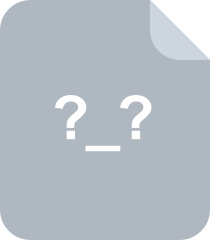
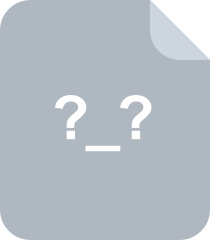
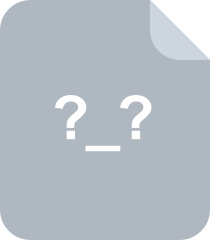
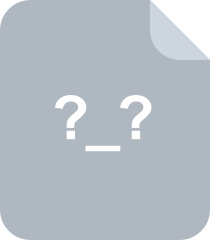
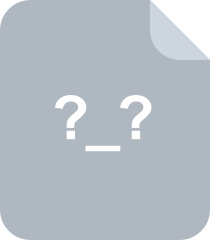
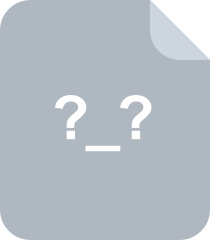
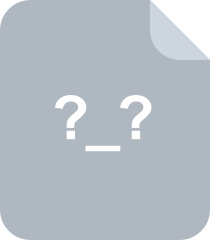
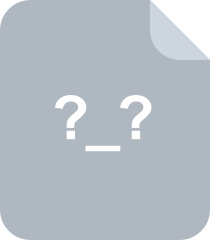
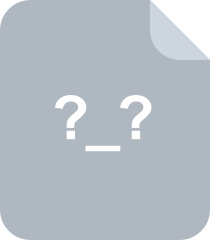
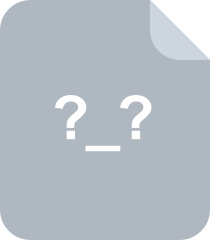
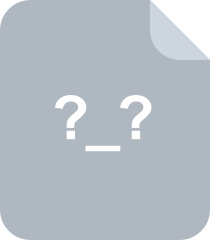
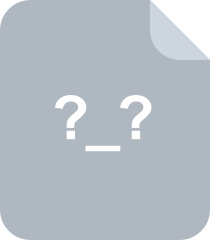
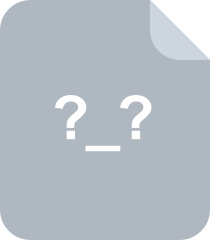
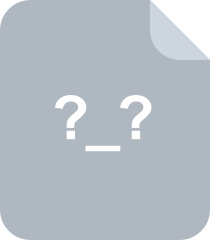
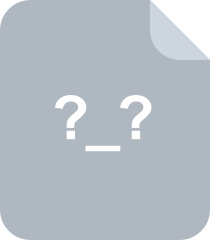
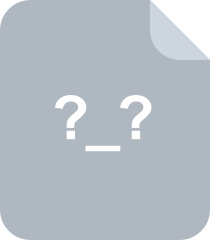
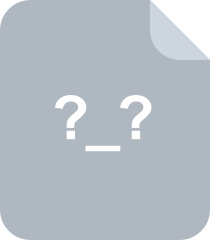
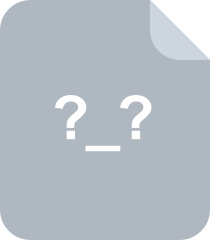
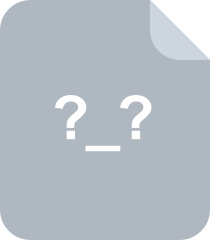
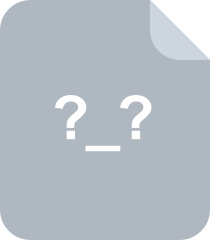
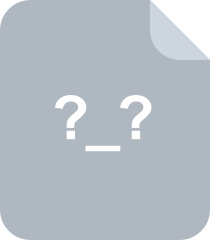
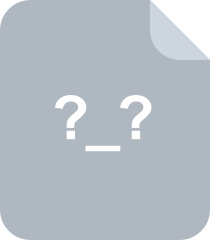
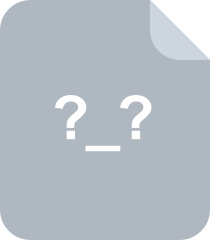
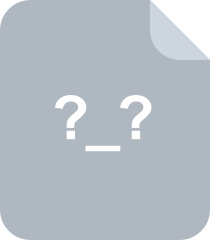
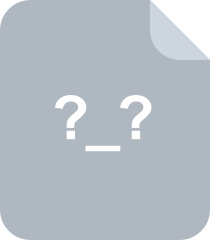
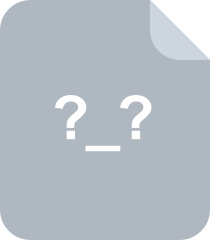
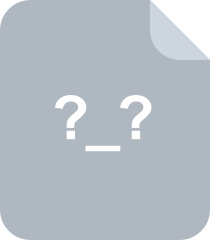
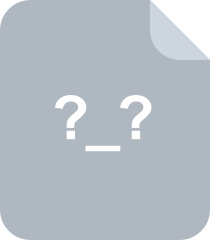
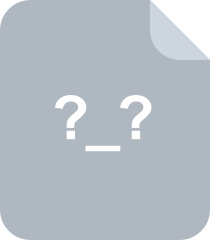
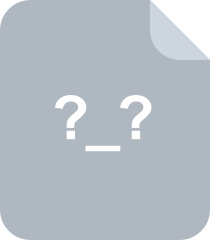
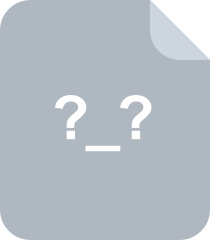
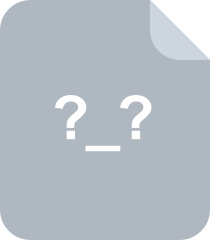
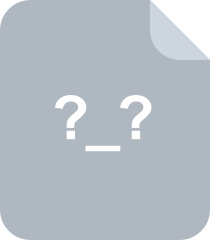
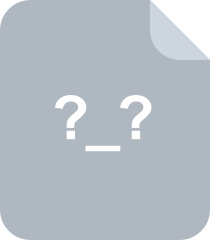
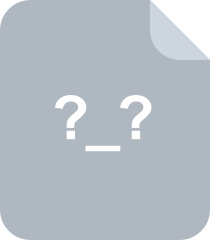
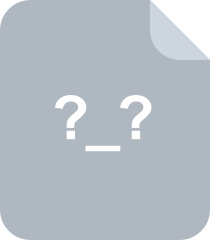
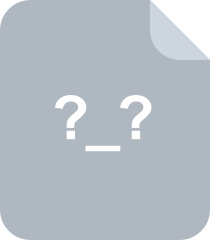
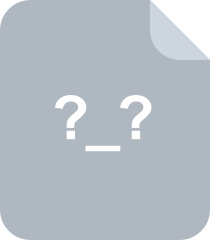
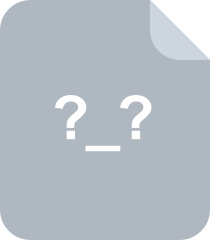
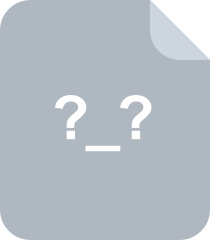
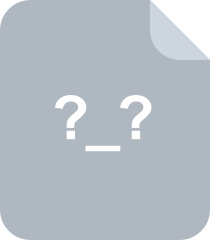
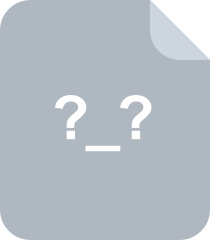
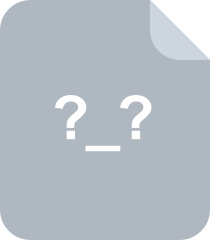
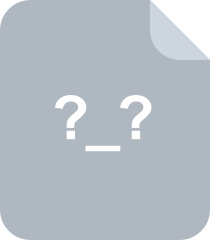
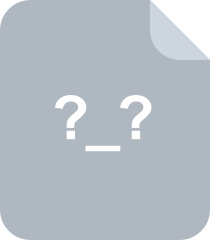
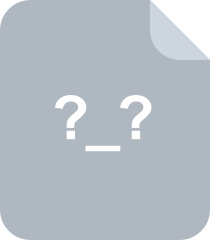
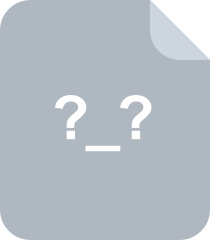
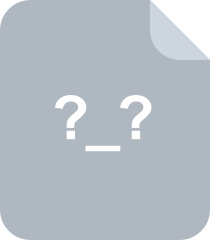
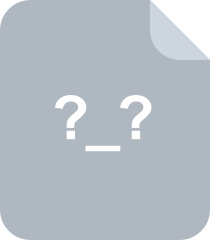
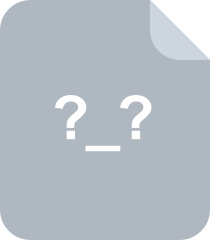
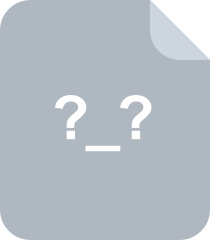
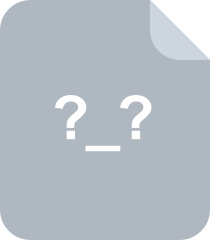
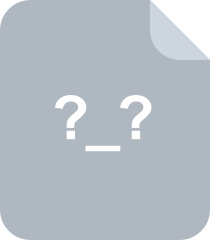
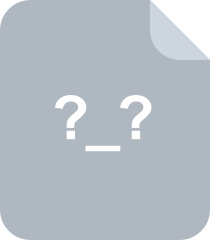
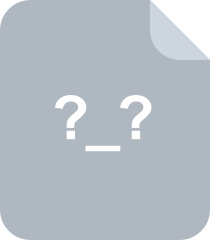
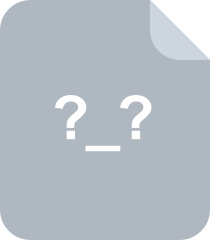
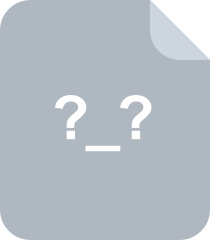
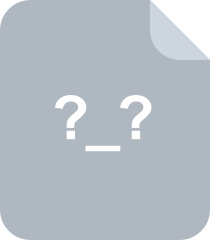
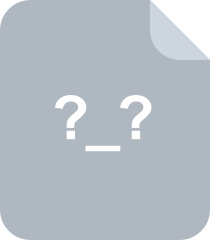
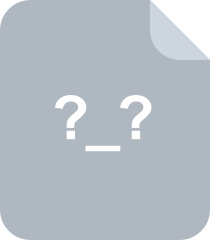
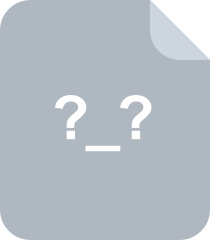
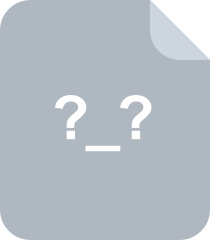
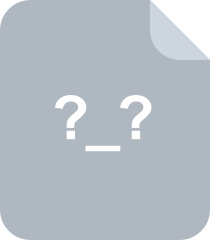
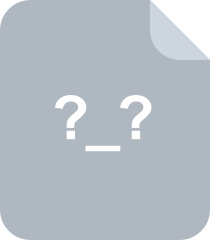
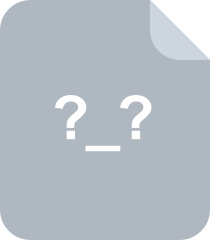
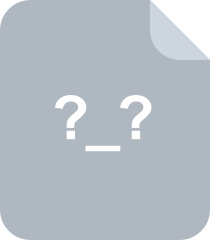
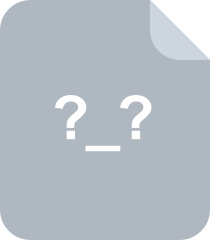
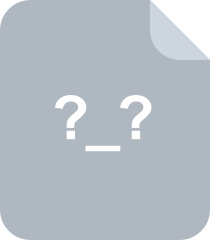
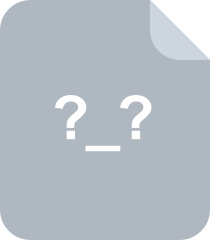
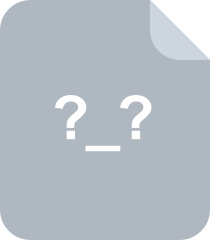
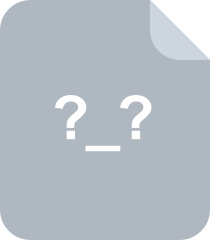
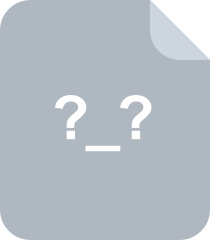
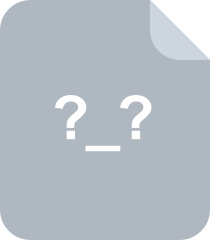
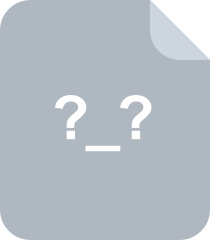
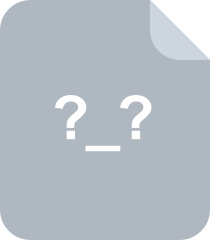
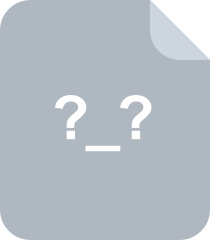
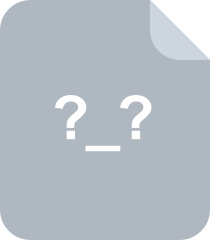
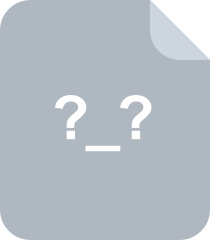
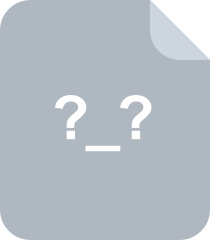
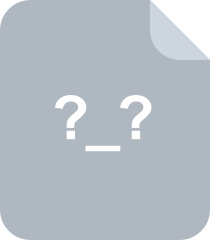
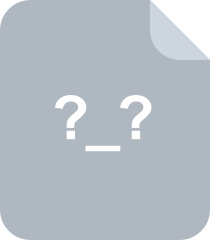
共 144 条
- 1
- 2
资源评论
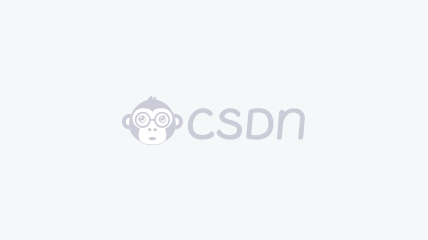

程序员Chino的日记
- 粉丝: 2936
- 资源: 4万+
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

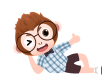
最新资源
- 使用PYTHON编写一个程序,识别数字和字母的程序
- chuanghanshu.m
- 3-1same.cbp
- 电路硬件PCB设计-EMC电磁兼容PCB防干扰与防静电设计pcb电磁兼容EMC设计等文档资料合集(18个).zip
- tudou-android-release (2).apk
- 三维重建-基于NeRF实现的稳定+实时3D说话人脸生成-附项目源码-优质项目实战.zip
- oracle-10g-32bit.zip
- com.aesq.zb_v1.0.35_danji100.com.apk
- 760996331259605建立门派1.360.apk
- 下面提供一些C语言的入门示例代码,并附有注释,以帮助理解每个部分的功能 1. Hello World程序 #include
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


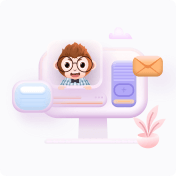
安全验证
文档复制为VIP权益,开通VIP直接复制
